- Python Course
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries

Different Forms of Assignment Statements in Python
We use Python assignment statements to assign objects to names. The target of an assignment statement is written on the left side of the equal sign (=), and the object on the right can be an arbitrary expression that computes an object.
There are some important properties of assignment in Python :-
- Assignment creates object references instead of copying the objects.
- Python creates a variable name the first time when they are assigned a value.
- Names must be assigned before being referenced.
- There are some operations that perform assignments implicitly.
Assignment statement forms :-
1. Basic form:
This form is the most common form.
2. Tuple assignment:
When we code a tuple on the left side of the =, Python pairs objects on the right side with targets on the left by position and assigns them from left to right. Therefore, the values of x and y are 50 and 100 respectively.
3. List assignment:
This works in the same way as the tuple assignment.
4. Sequence assignment:
In recent version of Python, tuple and list assignment have been generalized into instances of what we now call sequence assignment – any sequence of names can be assigned to any sequence of values, and Python assigns the items one at a time by position.
5. Extended Sequence unpacking:
It allows us to be more flexible in how we select portions of a sequence to assign.
Here, p is matched with the first character in the string on the right and q with the rest. The starred name (*q) is assigned a list, which collects all items in the sequence not assigned to other names.
This is especially handy for a common coding pattern such as splitting a sequence and accessing its front and rest part.
6. Multiple- target assignment:
In this form, Python assigns a reference to the same object (the object which is rightmost) to all the target on the left.
7. Augmented assignment :
The augmented assignment is a shorthand assignment that combines an expression and an assignment.
There are several other augmented assignment forms:
Please Login to comment...
Similar reads.
- Python Programs
- python-basics
- How to Get a Free SSL Certificate
- Best SSL Certificates Provider in India
- Elon Musk's xAI releases Grok-2 AI assistant
- What is OpenAI SearchGPT? How it works and How to Get it?
- Full Stack Developer Roadmap [2024 Updated]
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Assignment Operators
Add and assign, subtract and assign, multiply and assign, divide and assign, floor divide and assign, exponent and assign, modulo and assign.
to | |||
to and assigns the result to | |||
from and assigns the result to | |||
by and assigns the result to | |||
with and assigns the result to ; the result is always a float | |||
with and assigns the result to ; the result will be dependent on the type of values used | |||
to the power of and assigns the result to | |||
is divided by and assigns the result to |
For demonstration purposes, let’s use a single variable, num . Initially, we set num to 6. We can apply all of these operators to num and update it accordingly.
Assigning the value of 6 to num results in num being 6.
Expression: num = 6
Adding 3 to num and assigning the result back to num would result in 9.
Expression: num += 3
Subtracting 3 from num and assigning the result back to num would result in 6.
Expression: num -= 3
Multiplying num by 3 and assigning the result back to num would result in 18.
Expression: num *= 3
Dividing num by 3 and assigning the result back to num would result in 6.0 (always a float).
Expression: num /= 3
Performing floor division on num by 3 and assigning the result back to num would result in 2.
Expression: num //= 3
Raising num to the power of 3 and assigning the result back to num would result in 216.
Expression: num **= 3
Calculating the remainder when num is divided by 3 and assigning the result back to num would result in 2.
Expression: num %= 3
We can effectively put this into Python code, and you can experiment with the code yourself! Click the “Run” button to see the output.
The above code is useful when we want to update the same number. We can also use two different numbers and use the assignment operators to apply them on two different values.
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python assignment operators.
Assignment operators are used to assign values to variables:
Operator | Example | Same As | Try it |
---|---|---|---|
= | x = 5 | x = 5 | |
+= | x += 3 | x = x + 3 | |
-= | x -= 3 | x = x - 3 | |
*= | x *= 3 | x = x * 3 | |
/= | x /= 3 | x = x / 3 | |
%= | x %= 3 | x = x % 3 | |
//= | x //= 3 | x = x // 3 | |
**= | x **= 3 | x = x ** 3 | |
&= | x &= 3 | x = x & 3 | |
|= | x |= 3 | x = x | 3 | |
^= | x ^= 3 | x = x ^ 3 | |
>>= | x >>= 3 | x = x >> 3 | |
<<= | x <<= 3 | x = x << 3 |
Related Pages

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Python Assignment Operators: A Beginner’s Guide with Examples
Python offers a variety of tools to manipulate and manage data. Among these are assignment operators, which enable programmers to assign values to variables in a concise and efficient manner. Whether you’re a newcomer to programming or just getting started with Python, understanding assignment operators is a fundamental step toward becoming a proficient coder. In this article, we’ll explore the basics of Python assignment operators with clear examples to help beginners grasp their usage effectively.
Table of Contents
1. What are Assignment Operators?
2. examples of assignment operators., 2.1 basic assignment (=)., 2.2 add and assign (+=)., 2.3 subtract and assign (-=)., 2.4 multiply and assign (*=)., 2.5 divide and assign (/=)., 2.6 modulus and assign (%=)., 3. conclusion., leave a comment cancel reply.
This site uses Akismet to reduce spam. Learn how your comment data is processed .
- Module 2: The Essentials of Python »
- Variables & Assignment
- View page source
Variables & Assignment
There are reading-comprehension exercises included throughout the text. These are meant to help you put your reading to practice. Solutions for the exercises are included at the bottom of this page.
Variables permit us to write code that is flexible and amendable to repurpose. Suppose we want to write code that logs a student’s grade on an exam. The logic behind this process should not depend on whether we are logging Brian’s score of 92% versus Ashley’s score of 94%. As such, we can utilize variables, say name and grade , to serve as placeholders for this information. In this subsection, we will demonstrate how to define variables in Python.
In Python, the = symbol represents the “assignment” operator. The variable goes to the left of = , and the object that is being assigned to the variable goes to the right:
Attempting to reverse the assignment order (e.g. 92 = name ) will result in a syntax error. When a variable is assigned an object (like a number or a string), it is common to say that the variable is a reference to that object. For example, the variable name references the string "Brian" . This means that, once a variable is assigned an object, it can be used elsewhere in your code as a reference to (or placeholder for) that object:
Valid Names for Variables
A variable name may consist of alphanumeric characters ( a-z , A-Z , 0-9 ) and the underscore symbol ( _ ); a valid name cannot begin with a numerical value.
var : valid
_var2 : valid
ApplePie_Yum_Yum : valid
2cool : invalid (begins with a numerical character)
I.am.the.best : invalid (contains . )
They also cannot conflict with character sequences that are reserved by the Python language. As such, the following cannot be used as variable names:
for , while , break , pass , continue
in , is , not
if , else , elif
def , class , return , yield , raises
import , from , as , with
try , except , finally
There are other unicode characters that are permitted as valid characters in a Python variable name, but it is not worthwhile to delve into those details here.
Mutable and Immutable Objects
The mutability of an object refers to its ability to have its state changed. A mutable object can have its state changed, whereas an immutable object cannot. For instance, a list is an example of a mutable object. Once formed, we are able to update the contents of a list - replacing, adding to, and removing its elements.
To spell out what is transpiring here, we:
Create (initialize) a list with the state [1, 2, 3] .
Assign this list to the variable x ; x is now a reference to that list.
Using our referencing variable, x , update element-0 of the list to store the integer -4 .
This does not create a new list object, rather it mutates our original list. This is why printing x in the console displays [-4, 2, 3] and not [1, 2, 3] .
A tuple is an example of an immutable object. Once formed, there is no mechanism by which one can change of the state of a tuple; and any code that appears to be updating a tuple is in fact creating an entirely new tuple.
Mutable & Immutable Types of Objects
The following are some common immutable and mutable objects in Python. These will be important to have in mind as we start to work with dictionaries and sets.
Some immutable objects
numbers (integers, floating-point numbers, complex numbers)
“frozen”-sets
Some mutable objects
dictionaries
NumPy arrays
Referencing a Mutable Object with Multiple Variables
It is possible to assign variables to other, existing variables. Doing so will cause the variables to reference the same object:
What this entails is that these common variables will reference the same instance of the list. Meaning that if the list changes, all of the variables referencing that list will reflect this change:
We can see that list2 is still assigned to reference the same, updated list as list1 :
In general, assigning a variable b to a variable a will cause the variables to reference the same object in the system’s memory, and assigning c to a or b will simply have a third variable reference this same object. Then any change (a.k.a mutation ) of the object will be reflected in all of the variables that reference it ( a , b , and c ).
Of course, assigning two variables to identical but distinct lists means that a change to one list will not affect the other:
Reading Comprehension: Does slicing a list produce a reference to that list?
Suppose x is assigned a list, and that y is assigned a “slice” of x . Do x and y reference the same list? That is, if you update part of the subsequence common to x and y , does that change show up in both of them? Write some simple code to investigate this.
Reading Comprehension: Understanding References
Based on our discussion of mutable and immutable objects, predict what the value of y will be in the following circumstance:
Reading Comprehension Exercise Solutions:
Does slicing a list produce a reference to that list?: Solution
Based on the following behavior, we can conclude that slicing a list does not produce a reference to the original list. Rather, slicing a list produces a copy of the appropriate subsequence of the list:
Understanding References: Solutions
Integers are immutable, thus x must reference an entirely new object ( 9 ), and y still references 3 .
Multiple assignment in Python: Assign multiple values or the same value to multiple variables
In Python, the = operator is used to assign values to variables.
You can assign values to multiple variables in one line.
Assign multiple values to multiple variables
Assign the same value to multiple variables.
You can assign multiple values to multiple variables by separating them with commas , .
You can assign values to more than three variables, and it is also possible to assign values of different data types to those variables.
When only one variable is on the left side, values on the right side are assigned as a tuple to that variable.
If the number of variables on the left does not match the number of values on the right, a ValueError occurs. You can assign the remaining values as a list by prefixing the variable name with * .
For more information on using * and assigning elements of a tuple and list to multiple variables, see the following article.
- Unpack a tuple and list in Python
You can also swap the values of multiple variables in the same way. See the following article for details:
- Swap values in a list or values of variables in Python
You can assign the same value to multiple variables by using = consecutively.
For example, this is useful when initializing multiple variables with the same value.
After assigning the same value, you can assign a different value to one of these variables. As described later, be cautious when assigning mutable objects such as list and dict .
You can apply the same method when assigning the same value to three or more variables.
Be careful when assigning mutable objects such as list and dict .
If you use = consecutively, the same object is assigned to all variables. Therefore, if you change the value of an element or add a new element in one variable, the changes will be reflected in the others as well.
If you want to handle mutable objects separately, you need to assign them individually.
after c = []; d = [] , c and d are guaranteed to refer to two different, unique, newly created empty lists. (Note that c = d = [] assigns the same object to both c and d .) 3. Data model — Python 3.11.3 documentation
You can also use copy() or deepcopy() from the copy module to make shallow and deep copies. See the following article.
- Shallow and deep copy in Python: copy(), deepcopy()
Related Categories
Related articles.
- NumPy: arange() and linspace() to generate evenly spaced values
- Chained comparison (a < x < b) in Python
- pandas: Get first/last n rows of DataFrame with head() and tail()
- pandas: Filter rows/columns by labels with filter()
- Get the filename, directory, extension from a path string in Python
- Sign function in Python (sign/signum/sgn, copysign)
- How to flatten a list of lists in Python
- None in Python
- Create calendar as text, HTML, list in Python
- NumPy: Insert elements, rows, and columns into an array with np.insert()
- Shuffle a list, string, tuple in Python (random.shuffle, sample)
- Add and update an item in a dictionary in Python
- Cartesian product of lists in Python (itertools.product)
- Remove a substring from a string in Python
- pandas: Extract rows that contain specific strings from a DataFrame
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, python introduction.
- Get Started With Python
- Your First Python Program
- Python Comments
Python Fundamentals
- Python Variables and Literals
- Python Type Conversion
- Python Basic Input and Output
- Python Operators
Python Flow Control
- Python if...else Statement
- Python for Loop
- Python while Loop
- Python break and continue
- Python pass Statement
Python Data types
- Python Numbers and Mathematics
- Python List
- Python Tuple
- Python String
Python Dictionary
- Python Functions
- Python Function Arguments
- Python Variable Scope
- Python Global Keyword
- Python Recursion
- Python Modules
- Python Package
- Python Main function
Python Files
- Python Directory and Files Management
- Python CSV: Read and Write CSV files
- Reading CSV files in Python
- Writing CSV files in Python
- Python Exception Handling
- Python Exceptions
- Python Custom Exceptions
Python Object & Class
- Python Objects and Classes
- Python Inheritance
- Python Multiple Inheritance
- Polymorphism in Python
- Python Operator Overloading

Python Advanced Topics
- List comprehension
- Python Lambda/Anonymous Function
- Python Iterators
- Python Generators
- Python Namespace and Scope
- Python Closures
- Python Decorators
- Python @property decorator
- Python RegEx
Python Date and Time
- Python datetime
- Python strftime()
- Python strptime()
- How to get current date and time in Python?
- Python Get Current Time
- Python timestamp to datetime and vice-versa
- Python time Module
- Python sleep()
Additional Topic
- Precedence and Associativity of Operators in Python
- Python Keywords and Identifiers
- Python Asserts
- Python Json
- Python *args and **kwargs
Python Tutorials
Python reversed()
Python Dictionary clear()
- Python Dictionary items()
- Python Dictionary keys()
Python Dictionary fromkeys()
- Python Nested Dictionary
A Python dictionary is a collection of items, similar to lists and tuples. However, unlike lists and tuples, each item in a dictionary is a key-value pair (consisting of a key and a value).
- Create a Dictionary
We create a dictionary by placing key: value pairs inside curly brackets {} , separated by commas. For example,
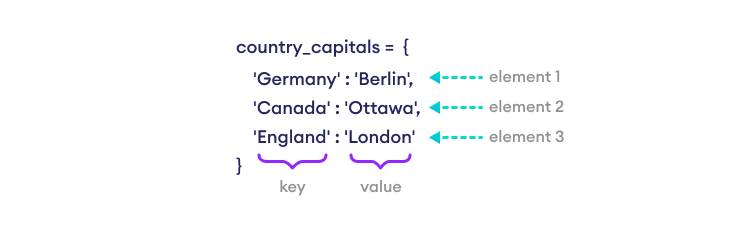
- Dictionary keys must be immutable, such as tuples, strings, integers, etc. We cannot use mutable (changeable) objects such as lists as keys.
- We can also create a dictionary using a Python built-in function dict() . To learn more, visit Python dict() .
Valid and Invalid Dictionaries
Immutable objects can't be changed once created. Some immutable objects in Python are integer, tuple and string.
In this example, we have used integers, tuples, and strings as keys for the dictionaries. When we used a list as a key, an error message occurred due to the list's mutable nature.
Note: Dictionary values can be of any data type, including mutable types like lists.
The keys of a dictionary must be unique. If there are duplicate keys, the later value of the key overwrites the previous value.
Here, the key Harry Potter is first assigned to Gryffindor . However, there is a second entry where Harry Potter is assigned to Slytherin .
As duplicate keys are not allowed in a dictionary, the last entry Slytherin overwrites the previous value Gryffindor .
- Access Dictionary Items
We can access the value of a dictionary item by placing the key inside square brackets.
Note: We can also use the get() method to access dictionary items.
- Add Items to a Dictionary
We can add an item to a dictionary by assigning a value to a new key. For example,
- Remove Dictionary Items
We can use the del statement to remove an element from a dictionary. For example,
Note : We can also use the pop() method to remove an item from a dictionary.
If we need to remove all items from a dictionary at once, we can use the clear() method.
- Change Dictionary Items
Python dictionaries are mutable (changeable). We can change the value of a dictionary element by referring to its key. For example,
Note : We can also use the update() method to add or change dictionary items.
- Iterate Through a Dictionary
A dictionary is an ordered collection of items (starting from Python 3.7), therefore it maintains the order of its items.
We can iterate through dictionary keys one by one using a for loop .
- Find Dictionary Length
We can find the length of a dictionary by using the len() function.
- Python Dictionary Methods
Here are some of the commonly used dictionary methods .
Function | Description |
---|---|
Removes the item with the specified key. | |
Adds or changes dictionary items. | |
Remove all the items from the dictionary. | |
Returns all the dictionary's keys. | |
Returns all the dictionary's values. | |
Returns the value of the specified key. | |
Returns the last inserted key and value as a tuple. | |
Returns a copy of the dictionary. |
- Dictionary Membership Test
We can check whether a key exists in a dictionary by using the in and not in operators.
Note: The in operator checks whether a key exists; it doesn't check whether a value exists or not.
Table of Contents
Before we wrap up, let’s put your knowledge of Python dictionary to the test! Can you solve the following challenge?
Write a function to merge two dictionaries.
- Merge dict1 and dict2 , then return the merged dictionary.
Video: Python Dictionaries to Store key/value Pairs
Sorry about that.
Our premium learning platform, created with over a decade of experience and thousands of feedbacks .
Learn and improve your coding skills like never before.
- Interactive Courses
- Certificates
- 2000+ Challenges
Related Tutorials
Python Library
Python Tutorial
Python Data Types

Python Conditional Assignment
When you want to assign a value to a variable based on some condition, like if the condition is true then assign a value to the variable, else assign some other value to the variable, then you can use the conditional assignment operator.
In this tutorial, we will look at different ways to assign values to a variable based on some condition.
1. Using Ternary Operator
The ternary operator is very special operator in Python, it is used to assign a value to a variable based on some condition.
It goes like this:
Here, the value of variable will be value_if_true if the condition is true, else it will be value_if_false .
Let's see a code snippet to understand it better.
You can see we have conditionally assigned a value to variable c based on the condition a > b .
2. Using if-else statement
if-else statements are the core part of any programming language, they are used to execute a block of code based on some condition.
Using an if-else statement, we can assign a value to a variable based on the condition we provide.
Here is an example of replacing the above code snippet with the if-else statement.
3. Using Logical Short Circuit Evaluation
Logical short circuit evaluation is another way using which you can assign a value to a variable conditionally.
The format of logical short circuit evaluation is:
It looks similar to ternary operator, but it is not. Here the condition and value_if_true performs logical AND operation, if both are true then the value of variable will be value_if_true , or else it will be value_if_false .
Let's see an example:
But if we make condition True but value_if_true False (or 0 or None), then the value of variable will be value_if_false .
So, you can see that the value of c is 20 even though the condition a < b is True .
So, you should be careful while using logical short circuit evaluation.
While working with lists , we often need to check if a list is empty or not, and if it is empty then we need to assign some default value to it.
Let's see how we can do it using conditional assignment.
Here, we have assigned a default value to my_list if it is empty.
Assign a value to a variable conditionally based on the presence of an element in a list.
Now you know 3 different ways to assign a value to a variable conditionally. Any of these methods can be used to assign a value when there is a condition.
The cleanest and fastest way to conditional value assignment is the ternary operator .
if-else statement is recommended to use when you have to execute a block of code based on some condition.
Happy coding! 😊
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
All the assignment code submission.
007kumar/Python-Assignment
Folders and files.
Name | Name | |||
---|---|---|---|---|
2 Commits | ||||
- Python 100.0%
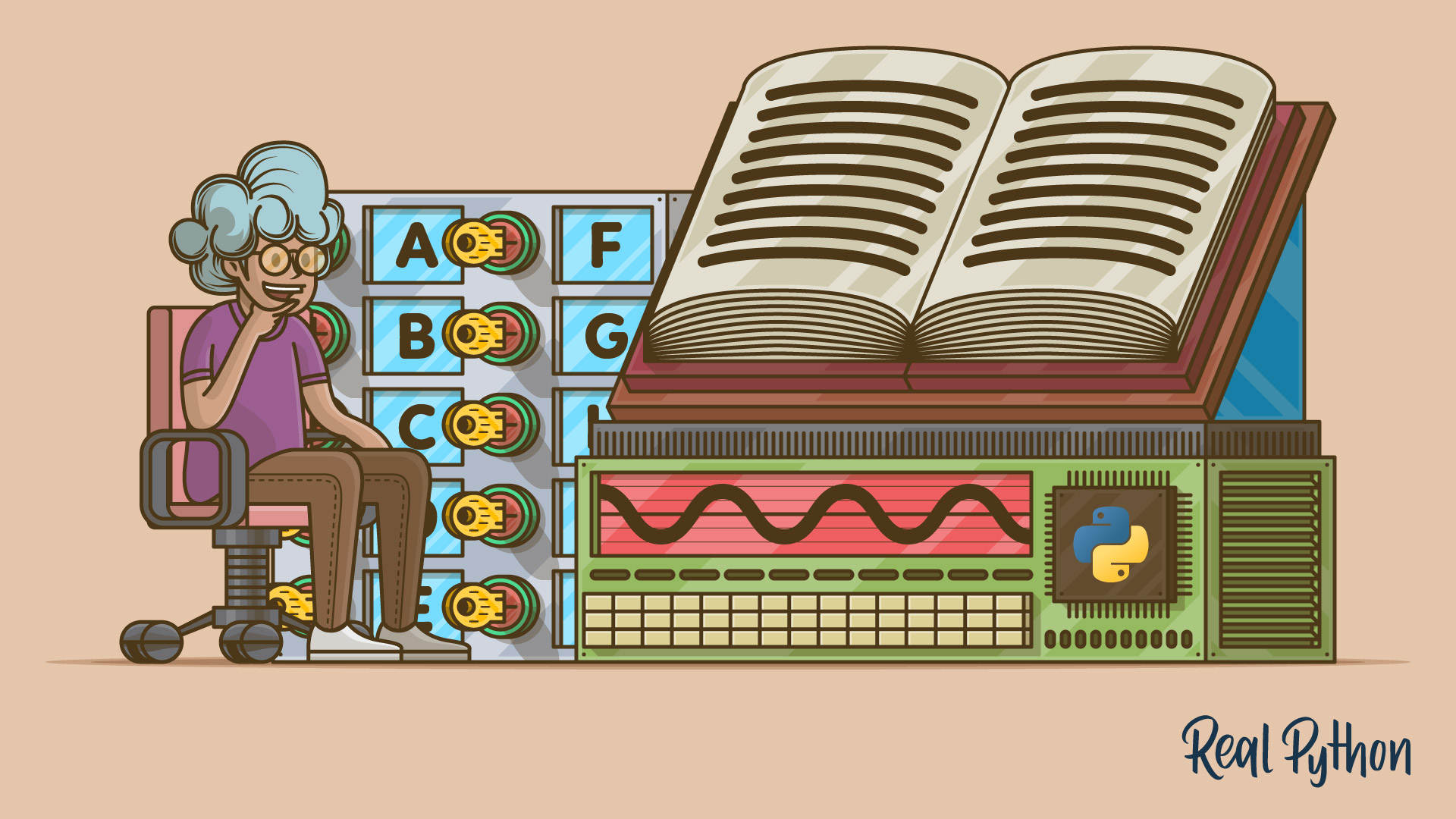
Dictionaries in Python
Table of Contents
Defining a Dictionary
Accessing dictionary values, dictionary keys vs. list indices, building a dictionary incrementally, restrictions on dictionary keys, restrictions on dictionary values, operators and built-in functions, d.get(<key>[, <default>]), d.pop(<key>[, <default>]), d.popitem(), d.update(<obj>).
Watch Now This tutorial has a related video course created by the Real Python team. Watch it together with the written tutorial to deepen your understanding: Dictionaries in Python
Python provides another composite data type called a dictionary , which is similar to a list in that it is a collection of objects.
Here’s what you’ll learn in this tutorial: You’ll cover the basic characteristics of Python dictionaries and learn how to access and manage dictionary data. Once you have finished this tutorial, you should have a good sense of when a dictionary is the appropriate data type to use, and how to do so.
Dictionaries and lists share the following characteristics:
- Both are mutable.
- Both are dynamic. They can grow and shrink as needed.
- Both can be nested. A list can contain another list. A dictionary can contain another dictionary. A dictionary can also contain a list, and vice versa.
Dictionaries differ from lists primarily in how elements are accessed:
- List elements are accessed by their position in the list, via indexing.
- Dictionary elements are accessed via keys.
Take the Quiz: Test your knowledge with our interactive “Python Dictionaries” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Test your understanding of Python dictionaries
Dictionaries are Python’s implementation of a data structure that is more generally known as an associative array. A dictionary consists of a collection of key-value pairs. Each key-value pair maps the key to its associated value.
You can define a dictionary by enclosing a comma-separated list of key-value pairs in curly braces ( {} ). A colon ( : ) separates each key from its associated value:
The following defines a dictionary that maps a location to the name of its corresponding Major League Baseball team:
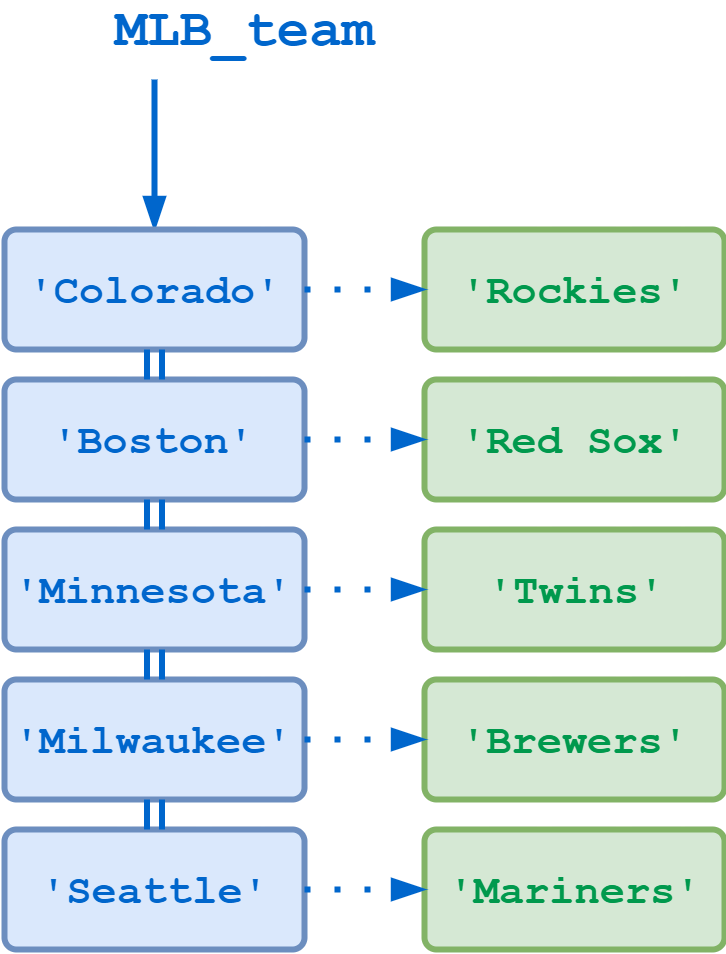
You can also construct a dictionary with the built-in dict() function. The argument to dict() should be a sequence of key-value pairs. A list of tuples works well for this:
MLB_team can then also be defined this way:
If the key values are simple strings, they can be specified as keyword arguments. So here is yet another way to define MLB_team :
Once you’ve defined a dictionary, you can display its contents, the same as you can do for a list. All three of the definitions shown above appear as follows when displayed:
The entries in the dictionary display in the order they were defined. But that is irrelevant when it comes to retrieving them. Dictionary elements are not accessed by numerical index:
Perhaps you’d still like to sort your dictionary. If that’s the case, then check out Sorting a Python Dictionary: Values, Keys, and More .
Of course, dictionary elements must be accessible somehow. If you don’t get them by index, then how do you get them?
A value is retrieved from a dictionary by specifying its corresponding key in square brackets ( [] ):
If you refer to a key that is not in the dictionary, Python raises an exception:
Adding an entry to an existing dictionary is simply a matter of assigning a new key and value:
If you want to update an entry, you can just assign a new value to an existing key:
To delete an entry, use the del statement , specifying the key to delete:
Begone, Seahawks! Thou art an NFL team.
You may have noticed that the interpreter raises the same exception, KeyError , when a dictionary is accessed with either an undefined key or by a numeric index:
In fact, it’s the same error. In the latter case, [1] looks like a numerical index, but it isn’t.
You will see later in this tutorial that an object of any immutable type can be used as a dictionary key. Accordingly, there is no reason you can’t use integers:
In the expressions MLB_team[1] , d[0] , and d[2] , the numbers in square brackets appear as though they might be indices. But they have nothing to do with the order of the items in the dictionary. Python is interpreting them as dictionary keys. If you define this same dictionary in reverse order, you still get the same values using the same keys:
The syntax may look similar, but you can’t treat a dictionary like a list:
Note: Although access to items in a dictionary does not depend on order, Python does guarantee that the order of items in a dictionary is preserved. When displayed, items will appear in the order they were defined, and iteration through the keys will occur in that order as well. Items added to a dictionary are added at the end. If items are deleted, the order of the remaining items is retained.
You can only count on this preservation of order very recently. It was added as a part of the Python language specification in version 3.7 . However, it was true as of version 3.6 as well—by happenstance as a result of the implementation but not guaranteed by the language specification.
Defining a dictionary using curly braces and a list of key-value pairs, as shown above, is fine if you know all the keys and values in advance. But what if you want to build a dictionary on the fly?
You can start by creating an empty dictionary, which is specified by empty curly braces. Then you can add new keys and values one at a time:
Once the dictionary is created in this way, its values are accessed the same way as any other dictionary:
Retrieving the values in the sublist or subdictionary requires an additional index or key:
This example exhibits another feature of dictionaries: the values contained in the dictionary don’t need to be the same type. In person , some of the values are strings, one is an integer, one is a list, and one is another dictionary.
Just as the values in a dictionary don’t need to be of the same type, the keys don’t either:
Here, one of the keys is an integer, one is a float, and one is a Boolean . It’s not obvious how this would be useful, but you never know.
Notice how versatile Python dictionaries are. In MLB_team , the same piece of information (the baseball team name) is kept for each of several different geographical locations. person , on the other hand, stores varying types of data for a single person.
You can use dictionaries for a wide range of purposes because there are so few limitations on the keys and values that are allowed. But there are some. Read on!
Almost any type of value can be used as a dictionary key in Python. You just saw this example, where integer, float, and Boolean objects are used as keys:
You can even use built-in objects like types and functions:
However, there are a couple restrictions that dictionary keys must abide by.
First, a given key can appear in a dictionary only once. Duplicate keys are not allowed. A dictionary maps each key to a corresponding value, so it doesn’t make sense to map a particular key more than once.
You saw above that when you assign a value to an already existing dictionary key, it does not add the key a second time, but replaces the existing value:
Similarly, if you specify a key a second time during the initial creation of a dictionary, the second occurrence will override the first:
Begone, Timberwolves! Thou art an NBA team. Sort of.
Secondly, a dictionary key must be of a type that is immutable. You have already seen examples where several of the immutable types you are familiar with—integer, float, string, and Boolean—have served as dictionary keys.
A tuple can also be a dictionary key, because tuples are immutable:
(Recall from the discussion on tuples that one rationale for using a tuple instead of a list is that there are circumstances where an immutable type is required. This is one of them.)
However, neither a list nor another dictionary can serve as a dictionary key, because lists and dictionaries are mutable :
Technical Note: Why does the error message say “unhashable”?
Technically, it is not quite correct to say an object must be immutable to be used as a dictionary key. More precisely, an object must be hashable , which means it can be passed to a hash function. A hash function takes data of arbitrary size and maps it to a relatively simpler fixed-size value called a hash value (or simply hash), which is used for table lookup and comparison.
Python’s built-in hash() function returns the hash value for an object which is hashable, and raises an exception for an object which isn’t:
All of the built-in immutable types you have learned about so far are hashable, and the mutable container types (lists and dictionaries) are not. So for present purposes, you can think of hashable and immutable as more or less synonymous.
In future tutorials, you will encounter mutable objects which are also hashable.
By contrast, there are no restrictions on dictionary values. Literally none at all. A dictionary value can be any type of object Python supports, including mutable types like lists and dictionaries, and user-defined objects, which you will learn about in upcoming tutorials.
There is also no restriction against a particular value appearing in a dictionary multiple times:
You have already become familiar with many of the operators and built-in functions that can be used with strings , lists , and tuples . Some of these work with dictionaries as well.
For example, the in and not in operators return True or False according to whether the specified operand occurs as a key in the dictionary:
You can use the in operator together with short-circuit evaluation to avoid raising an error when trying to access a key that is not in the dictionary:
In the second case, due to short-circuit evaluation, the expression MLB_team['Toronto'] is not evaluated, so the KeyError exception does not occur.
The len() function returns the number of key-value pairs in a dictionary:
Built-in Dictionary Methods
As with strings and lists, there are several built-in methods that can be invoked on dictionaries. In fact, in some cases, the list and dictionary methods share the same name. (In the discussion on object-oriented programming, you will see that it is perfectly acceptable for different types to have methods with the same name.)
The following is an overview of methods that apply to dictionaries:
Clears a dictionary.
d.clear() empties dictionary d of all key-value pairs:
Returns the value for a key if it exists in the dictionary.
The Python dictionary .get() method provides a convenient way of getting the value of a key from a dictionary without checking ahead of time whether the key exists, and without raising an error.
d.get(<key>) searches dictionary d for <key> and returns the associated value if it is found. If <key> is not found, it returns None :
If <key> is not found and the optional <default> argument is specified, that value is returned instead of None :
Returns a list of key-value pairs in a dictionary.
d.items() returns a list of tuples containing the key-value pairs in d . The first item in each tuple is the key, and the second item is the key’s value:
Returns a list of keys in a dictionary.
d.keys() returns a list of all keys in d :
Returns a list of values in a dictionary.
d.values() returns a list of all values in d :
Any duplicate values in d will be returned as many times as they occur:
Technical Note: The .items() , .keys() , and .values() methods actually return something called a view object . A dictionary view object is more or less like a window on the keys and values. For practical purposes, you can think of these methods as returning lists of the dictionary’s keys and values.
Removes a key from a dictionary, if it is present, and returns its value.
If <key> is present in d , d.pop(<key>) removes <key> and returns its associated value:
d.pop(<key>) raises a KeyError exception if <key> is not in d :
If <key> is not in d , and the optional <default> argument is specified, then that value is returned, and no exception is raised:
Removes a key-value pair from a dictionary.
d.popitem() removes the last key-value pair added from d and returns it as a tuple:
If d is empty, d.popitem() raises a KeyError exception:
Note: In Python versions less than 3.6, popitem() would return an arbitrary (random) key-value pair since Python dictionaries were unordered before version 3.6.
Merges a dictionary with another dictionary or with an iterable of key-value pairs.
If <obj> is a dictionary, d.update(<obj>) merges the entries from <obj> into d . For each key in <obj> :
- If the key is not present in d , the key-value pair from <obj> is added to d .
- If the key is already present in d , the corresponding value in d for that key is updated to the value from <obj> .
Here is an example showing two dictionaries merged together:
In this example, key 'b' already exists in d1 , so its value is updated to 200 , the value for that key from d2 . However, there is no key 'd' in d1 , so that key-value pair is added from d2 .
<obj> may also be a sequence of key-value pairs, similar to when the dict() function is used to define a dictionary. For example, <obj> can be specified as a list of tuples:
Or the values to merge can be specified as a list of keyword arguments:
In this tutorial, you covered the basic properties of the Python dictionary and learned how to access and manipulate dictionary data.
Lists and dictionaries are two of the most frequently used Python types. As you have seen, they have several similarities, but differ in how their elements are accessed. Lists elements are accessed by numerical index based on order, and dictionary elements are accessed by key
Because of this difference, lists and dictionaries tend to be appropriate for different circumstances. You should now have a good feel for which, if either, would be best for a given situation.
Next you will learn about Python sets . The set is another composite data type, but it is quite different from either a list or dictionary.
🐍 Python Tricks 💌
Get a short & sweet Python Trick delivered to your inbox every couple of days. No spam ever. Unsubscribe any time. Curated by the Real Python team.
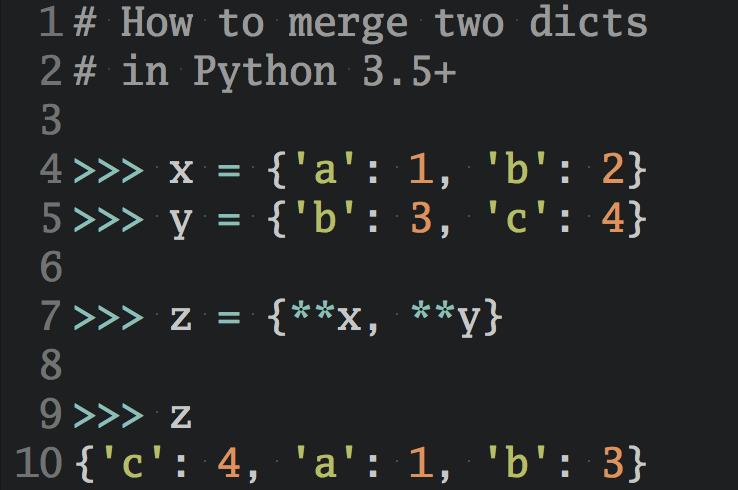
About John Sturtz
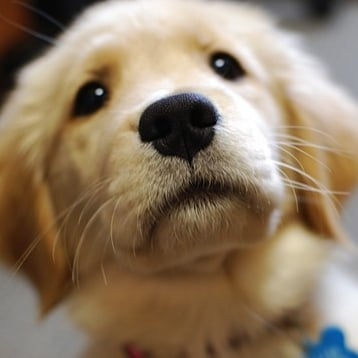
John is an avid Pythonista and a member of the Real Python tutorial team.
Each tutorial at Real Python is created by a team of developers so that it meets our high quality standards. The team members who worked on this tutorial are:
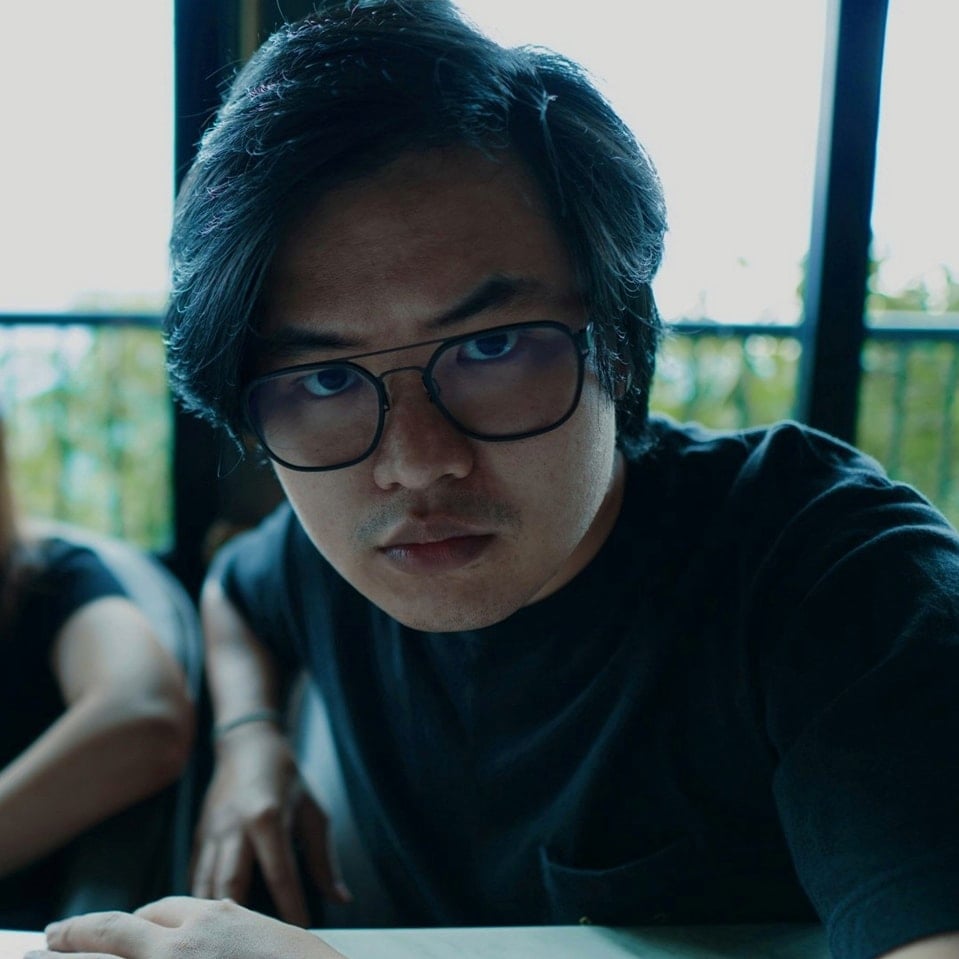
Master Real-World Python Skills With Unlimited Access to Real Python
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
What Do You Think?
What’s your #1 takeaway or favorite thing you learned? How are you going to put your newfound skills to use? Leave a comment below and let us know.
Commenting Tips: The most useful comments are those written with the goal of learning from or helping out other students. Get tips for asking good questions and get answers to common questions in our support portal . Looking for a real-time conversation? Visit the Real Python Community Chat or join the next “Office Hours” Live Q&A Session . Happy Pythoning!
Keep Learning
Related Topics: basics python
Recommended Video Course: Dictionaries in Python
Keep reading Real Python by creating a free account or signing in:
Already have an account? Sign-In
Exceptions ¶
Raised when an implicitly defined __setattr__() or __delattr__() is called on a dataclass which was defined with frozen=True . It is a subclass of AttributeError .
Python初心者がマスターしておきたい辞書の活用テクニック10選!
みずほリサーチ&テクノロジーズの @fujine です。
本記事では、 Pythonの辞書をより便利に使うためのテクニック10個を解説 していきます。基本的な操作も多く含まれていますが、
- パフォーマンスを意識して実装する
- キーの数に依存せず、短い行数で簡潔に実装する
ことに重点を置きました。 Pythonの辞書に一通り慣れた初心者が、チーム開発やより複雑なプログラム開発へステップアップする際に活用 いただけたらと思います。
複数の辞書を1つに結合する
初期値を持つ辞書を作成する, 辞書の要素を取得・更新・追加・削除する, 要素の追加と取得を一度に実行する, 辞書から複数の要素を同時に取得する, 辞書をキーや値でソートする, 辞書を読み取り専用にする, カスタム辞書を作成する.
実行環境はWindows10(64bit) 22H2、Pythonバージョンは以下の通りです。
波括弧 {} 、もしくは組み込み関数 dict() で辞書を作成できます。
辞書の作成時間は {} の方が3倍近く高速 です。大量の辞書を作成する場合やパフォーマンス重視のアプリケーションでは、 {} を使用しましょう。
辞書内包表記でも辞書を作成できます。リスト等のシーケンスオブジェクトを辞書に変換する時に便利です。
辞書と集合( set )はどちらも同じ波括弧( {} )を使用するため、辞書内包表記にしたつもりが集合内包表記とならないよう注意しましょう。
複数の辞書を結合して、新たな辞書を生成できます。 説明にあたり、結合前の辞書を3つ用意します。
| 演算子で辞書同士を結合した新たな辞書オブジェクトを生成できます。キーが重複する場合、後から結合した値で上書きされます。3つ以上の結合も可能です。
| 演算子による結合はPython3.9から利用可能です。それ以前のバージョンでは、以下の実装でも同じ結果を得ることができます。
update メソッドでも結合可能です。 | 演算子との違いとして、 update はインプレース操作です。また、キーが重複した場合は TypeError が送出されます。
- 予期しない上書きを検知したい場合は update
- 上書きを許容する場合は | 演算子
という使い分けが良さそうです。
よくある操作の1つに、「辞書に特定のキーが存在しない場合、そのキーと値(初期値)を設定する」があります。
以下のように愚直に代入することもできますが、
- キーの数が多いとコードが長くなり保守しづらい
- 処理結果の辞書はキー順序が不定になる
という問題があります。
代わりに、初期値を持った d_init を定義して入力データ d_input とマージしましょう。これなら、 初期値が複数ある場合も一括設定できる上、キーの順序もコントロールできます 。
全てのキーで初期値が同じ場合は、 fromkeys クラスメソッドを使いましょう。第1引数にキーのシーケンスオブジェクト、第2引数に初期値の値(省略時は None )を設定することで、 全てのキーの同じ値の辞書を生成 できます。
パフォーマンスは、辞書内包表記と fromkeys のどちらも同程度です。
fromkeys の初期値はイミュータブルオブジェクトのみを指定して下さい。リストや辞書のようなミュータブルオブジェクトを指定すると、辞書の値は全て同じオブジェクトを参照するようになります。
ミュータブルオブジェクトを初期値にしたい場合は、代わりに辞書内包表記を使用しましょう。
辞書の基本的な取得、更新、追加、削除の操作は以下の通りです。
存在しないキーを取得しようとすると KeyError が送出されます。 get メソッドを使えば、キーが存在しない場合はデフォルト値(省略時は None )が応答され、例外は発生しません。
存在しないキーを削除しようとしても KeyError が送出されます。 pop メソッドを使うと、キーが存在しない場合は代わりにデフォルト値が応答されます。
get と異なり、 pop ではキーが存在せずデフォルト値も指定されない場合は None ではなく KeyError が送出されます。 pop ではデフォルト値を指定しましょう。
よくある操作の1つに、「特定のキーがあれば値を取得し、なければキーと初期値を追加してから値を取得する」というのもあります。
以下にキー名と初期値を定義します。
よくある実装例は以下です。 if 文でキーの有無を判定して値を追加しています。
setdefault メソッドを使えば、同じ処理を1行でシンプルに実装できます。 if 文は必要ありません。
setdefault は便利ですが、 set なのに get もしており、命名があまり直感的ではありません。私は setdefault → set default (and get) という独自の覚え方をしています。
通常、辞書に指定可能なキーは一度に1つのみです。 d[key] では複数の値を同時に指定・取得することはできません。
operator.itemgetter を使えば、 複数の値を同時に取得することが可能 です。 itemgetter の引数にキーを複数指定すると、指定したキーの順番で値を取得できます。
これを応用すれば、辞書から特定のキーのみをフィルタリングすることも簡単にできます。
辞書 d に対し、 sorted(d) では昇順ソートされたキー名だけが出力されます(これは sorted(d.keys()) と同じです)。 sorted(d.items()) とすれば、キーと値の両方が出力されます。この場合、 d.items() によりキー・値のペア (key, value) が展開され、その0番目である key がソート対象となります。
この仕組みを利用し、辞書内包表記と dict() の2通りでキー順によるソートが可能です。
値でソートする場合は、 sorted の key 引数を用います。 lambda 関数か itemgetter(1) を使用して、値をソート対象とします。
パフォーマンスを比較すると、キーと値のどちらでソートする場合も、辞書内包表記の方が速い結果となりました。これは、 d.items() でキーと値のペアを生成する処理コストの方が大きいためと考えられます。
types.MappingProxyType を使うことで、辞書の読み取り専用ビューを作成することができます。ビューに対しては参照操作のみが可能です。
更新・追加・削除操作は TypeError となるため、 「辞書オブジェクトへの予期しない変更」をガードしたい場面で効果的 です。
辞書に独自の処理をカスタムしたい場合は、 collections.UserDict を使用します。
例えば、 KeyError 発生時に現在のキー情報を例外メッセージに加えた FriendlyDict クラスを定義してみます。 UserDict を継承し、キーが存在しない場合に呼び出される __missing__() 特殊メソッド内の例外メッセージをオーバーライドします。
存在しないキーでアクセスすると、期待通りの例外メッセージを得ることができました。既存のキー情報を出力することで、デバッグの効率化にもつながりそうです。
UserDict の代わりに、組み込みクラスである dict を継承する方法もあります。 UserDict を使用したのは、特殊メソッドのオーバーライドによる他特殊メソッドへの意図しない影響を最小化するためです。
辞書に型ヒントを定義すると、想定する型や値を明示することができ、プログラムの可読性が向上します。また、型ヒントチェッカーにより想定外データの混入が検知できるため、バグや不良を早期発見しやすくなります。
pythonの型ヒントは静的解析時のみ検査されるため、プログラム実行時には検査されず、エラーも発生しません。 実行時にも型を検査したい場合は、 pydantic などの外部パッケージをご使用下さい。
型ヒントチェッカーとして、 mypy をインストールします。
まずはシンプルに、 変数: dict としてみましょう。この変数に辞書以外のオブジェクトを代入するとチェックエラーになります。
続いて、辞書のキーと値のデータ型を指定します。任意のデータ型は Any 、複数のデータ型を許容する場合は | で連結します。
キーや値に特定のリテラル値のみを許容するには Literal を使用します。指定したリテラル値以外の代入はチェックエラーになります。 Literal に float や Any 、変数や式を指定することはできません。 なお AllowedKeys と AllowedValues は辞書の全要素に適用されるため、特定のキーに特定のリテラル値を制約付けたい場合は後述する TypedDict を使用します。
TypedDict を継承したクラスを定義することで、キー毎にデータ型を指定することができます。 デフォルトでは全てのキーは必須 となります。
クラス継承の引数に total=False を追加すると、 全てのキーは任意 となります。
必須と任意を混在させるには、 Required と NotRequired を使用します。 a と b を必須とし、 c のみ任意とする場合は以下のように定義します。
この Sample3 クラスは、以下のように定義しても同じです。 「必須キーと任意キーのどちらが多いか」によって使い分けても良いでしょう。
本記事では、辞書を活用するためのテクニックを10個紹介させていただきました。
初心者のみならず、辞書を使い慣れた中級者の方にとっても、新たな発見が1つでもありましたら幸いです。
Go to list of comments
Register as a new user and use Qiita more conveniently
- You get articles that match your needs
- You can efficiently read back useful information
- You can use dark theme
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
What is the best way to do automatic attribute assignment in Python, and is it a good idea?
Instead of writing code like this every time I define a class:
I could use this recipe for automatic attribute assignment .
Two questions :
- Are there drawbacks or pitfalls associated with this shortcut?
Is there a better way to achieve similar convenience?
- 4 +1 this is a recurrent pattern in Python. I don't see any drawback in using the autoassign decorator, as it lets you define a list of argument not to assign. – pberkes Commented Sep 6, 2010 at 16:55
- 1 stackoverflow.com/questions/1389180/… – pmav99 Commented May 24, 2014 at 12:32
9 Answers 9
There are some things about the autoassign code that bug me (mostly stylistic, but one more serious problem):
autoassign does not assign an 'args' attribute:
autoassign acts like a decorator. But autoassign(*argnames) calls a function which returns a decorator. To achieve this magic, autoassign needs to test the type of its first argument. If given a choice, I prefer functions not test the type of its arguments.
There seems to be a considerable amount of code devoted to setting up sieve , lambdas within lambdas, ifilters, and lots of conditions.
I think there might be a simpler way. (See below).
for _ in itertools.starmap(assigned.setdefault, defaults): pass . I don't think map or starmap was meant to call functions, whose only purpose is their side effects. It could have been written more clearly with the mundane:
Here is an alternative simpler implementation which has the same functionality as autoassign (e.g. can do includes and excludes), and which addresses the above points:
And here is the unit test I used to check its behavior:
PS. Using autoassign or autoargs is compatible with IPython code completion.
- 1 I also found your autoargs incredibly handy. One issue I noticed is that it seems to throw an error if defaults is None , because the call to reversed fails. Am I right in thinking that this should be fixable by adding an if defaults: in front of the line for attr,val in zip(reversed(attrs),reversed(defaults)): , or does that have unintended sideeffects? The unit test passes. – m01 Commented Feb 12, 2014 at 9:32
- @m01: Sorry for the very belated response. Thanks for the correction! – unutbu Commented Aug 2, 2017 at 15:38
- @unutbu Just a nit but in 3.10.x inspect.getargspec(func) getargspec is deprecated. you should use inspect.getfullargspec(func) instead – Frank C. Commented Jan 10, 2023 at 20:46
From Python 3.7+ you can use a Data Class , which achieves what you want and more.
It allows you to define fields for your class, which are attributes automatically assigned.
It would look something like that:
The __init__ method will be automatically created in your class, and it will assign the arguments of instance creation to those attributes (and validate the arguments).
Note that here type hinting is required , that is why I have used int and str in the example. If you don't know the type of your field, you can use Any from the typing module .

- 1 Backport for Python 3.6 : The PEP 557 references a sample implementation ( permalink as of 2019-10-28 ) on GitHub which has a pypi/pip module as well. Therefore it can be installed by pip install dataclasses . – luckydonald Commented Oct 28, 2019 at 21:19
I don't know if it is necessarily better, but you could do this:
Courtesy Peter Norvig's Python: Infrequently Answered Questions .
- 7 This is a quick solution to the problem, but it has two drawbacks: 1) you have to add much more code if you want to make sure that some arguments are passed to the function; 2) the signature of the function is completely opaque, and it cannot be used to understand how to call the function; one will need to rely on the docstring instead – pberkes Commented Sep 6, 2010 at 16:57
One drawback: many IDEs parse __init__.py to discover an object's attributes. If you want automatic code completion in your IDE to be more functional, then you may be better off spelling it out the old-fashioned way.
If you have a lot of variables, you could pass one single configuration dict or object.
Similar to the above, though not the same... the following is very short, deals with args and kwargs :
Use like this:
This a simple implementation by judy2k :
In this package you can now find
- @autoargs inspired by answer-3653049
- @autoprops to transform the fields generated by @autoargs into @property , for use in combination with a validation library such as enforce or pycontracts .
Note that this has been validated for python 3.5+

You just can't use *args, but you can store in some instance list (like self.args, don't know)
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged python attributes decorator or ask your own question .
- The Overflow Blog
- LLMs evolve quickly. Their underlying architecture, not so much.
- From PHP to JavaScript to Kubernetes: how one backend engineer evolved over time
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- What does a new user need in a homepage experience on Stack Overflow?
- Feedback requested: How do you use tag hover descriptions for curating and do...
- Staging Ground Reviewer Motivation
Hot Network Questions
- "Authorized ESTA After Incorrectly Answering Criminal Offense Question: What Should I Do?"
- Need strftime() output in the buffer
- What's the proper way to shut down after a kernel panic?
- Stealth Mosquitoes?
- How could Bangladesh protect itself from Indian dams and barrages?
- Repeating zsh brace expansion values in zsh to download multiple files using wget2
- Reduce String Length With Thread Safety & Concurrency
- Where should the chess pieces be placed in 4x4, 6x6 and 9x9 chessboards?
- Polyline to polygon
- Flight left while checked in passenger queued for boarding
- Canceling factors in a ratio of factorials
- Is it OK to make an "offshape" bid if you can handle any likely response?
- Which cards use −5 V and −12 V in IBM PC compatible systems?
- A short story about a SF author telling his friends, as a joke, a harebrained SF story, and what follows
- How Can this Limit be really Evaluated?
- R Squared Causal Inference
- Order of connection using digital multimeter wall outlet
- How can you trust a forensic scientist to have maintained the chain of custody?
- Why cant we save the heat rejected in a heat engine?
- Is 2'6" within the size constraints of small, and what would the weight of a fairy that size be?
- default-valued optional (boolean) parameter for a new command in tikz
- What was I thinking when I made this grid?
- What are the limits of Terms of Service as a legal shield for a company?
- How to ensure a BSD licensed open source project is not closed in the future?

IMAGES
COMMENTS
Python's assignment operators allow you to define assignment statements. This type of statement lets you create, initialize, and update variables throughout your code. Variables are a fundamental cornerstone in every piece of code, and assignment statements give you complete control over variable creation and mutation.
Although the definition of assignment implies that overlaps between the left-hand side and the right-hand side are 'simultaneous' (for example a, b = b, a swaps two variables), overlaps within the collection of assigned-to variables occur left-to-right, sometimes resulting in confusion. For instance, the following program prints [0, 2]:
In Python dictionaries, the assignment operator = is used to assign a new key-value pair to the dictionary or update the value of an existing key. Here's how you might use it: my_dict = {} # Create an empty dictionary. my_dict['key1'] = 'value1' # Assign a new key-value pair. my_dict['key1'] = 'updated value' # Update the value of an existing ...
Multiple- target assignment: x = y = 75. print(x, y) In this form, Python assigns a reference to the same object (the object which is rightmost) to all the target on the left. OUTPUT. 75 75. 7. Augmented assignment : The augmented assignment is a shorthand assignment that combines an expression and an assignment.
The author selected the COVID-19 Relief Fund to receive a donation as part of the Write for DOnations program.. Introduction. Python 3.8, released in October 2019, adds assignment expressions to Python via the := syntax. The assignment expression syntax is also sometimes called "the walrus operator" because := vaguely resembles a walrus with tusks. ...
Since Python 3.8, code can use the so-called "walrus" operator (:=), documented in PEP 572, for assignment expressions. This seems like a really substantial new feature, since it allows this form of assignment within comprehensions and lambdas. What exactly are the syntax, semantics, and grammar specifications of assignment expressions?
Login to Save. Assignment operators in Python. The above code is useful when we want to update the same number. We can also use two different numbers and use the assignment operators to apply them on two different values. num_one = 6. num_two = 3. print(num_one) num_one += num_two. print(num_one)
In this lesson, you'll learn about the biggest change in Python 3.8: the introduction of assignment expressions.Assignment expression are written with a new notation (:=).This operator is often called the walrus operator as it resembles the eyes and tusks of a walrus on its side.. Assignment expressions allow you to assign and return a value in the same expression.
Python Assignment Operators. Assignment operators are used to assign values to variables: Operator. Example. Same As. Try it. =. x = 5. x = 5.
Python offers a variety of tools to manipulate and manage data. Among these are assignment operators, which enable programmers to assign values to variables in a concise and efficient manner. Whether you're a newcomer to programming or just getting started with Python, understanding assignment operators is a fundamental step toward becoming a proficient coder. In […]
Valid Names for Variables . A variable name may consist of alphanumeric characters (a-z, A-Z, 0-9) and the underscore symbol (_); a valid name cannot begin with a numerical value.var: valid _var2: valid. ApplePie_Yum_Yum: valid. 2cool: invalid (begins with a numerical character). I.am.the.best: invalid (contains .. They also cannot conflict with character sequences that are reserved by the ...
In Python, variables need not be declared or defined in advance, as is the case in many other programming languages. To create a variable, you just assign it a value and then start using it. Assignment is done with a single equals sign ( = ): Python. >>> n = 300. This is read or interpreted as " n is assigned the value 300 .".
None in Python; Create calendar as text, HTML, list in Python; NumPy: Insert elements, rows, and columns into an array with np.insert() Shuffle a list, string, tuple in Python (random.shuffle, sample) Add and update an item in a dictionary in Python; Cartesian product of lists in Python (itertools.product) Remove a substring from a string in Python
A Python dictionary is a collection of items, similar to lists and tuples. However, unlike lists and tuples, each item in a dictionary is a key-value pair (consisting of a key and a value). Create a Dictionary. We create a dictionary by placing key: value pairs inside curly brackets {}, separated by commas. For example,
4. More Control Flow Tools¶. As well as the while statement just introduced, Python uses a few more that we will encounter in this chapter.. 4.1. if Statements¶. Perhaps the most well-known statement type is the if statement. For example: >>> x = int (input ("Please enter an integer: ")) Please enter an integer: 42 >>> if x < 0:...
Let's see a code snippet to understand it better. a = 10. b = 20 # assigning value to variable c based on condition. c = a if a > b else b. print(c) # output: 20. You can see we have conditionally assigned a value to variable c based on the condition a > b. 2. Using if-else statement.
Note how the local assignment (which is default) didn't change scope_test's binding of spam.The nonlocal assignment changed scope_test's binding of spam, and the global assignment changed the module-level binding.. You can also see that there was no previous binding for spam before the global assignment.. 9.3. A First Look at Classes¶. Classes introduce a little bit of new syntax, three new ...
PEP 572 seeks to add assignment expressions (or "inline assignments") to the language, but it has seen a prolonged discussion over multiple huge threads on the python-dev mailing list—even after multiple rounds on python-ideas.
All the assignment code submission. Contribute to 007kumar/Python-Assignment development by creating an account on GitHub.
Defining a Dictionary. Dictionaries are Python's implementation of a data structure that is more generally known as an associative array. A dictionary consists of a collection of key-value pairs. Each key-value pair maps the key to its associated value. You can define a dictionary by enclosing a comma-separated list of key-value pairs in ...
In this practical, we will learn about the programming language Python as well as NumPy and Matplotlib, two fundamental tools for data science and machine learning in Python. ... are the strict less and greater than operators, while == is the equality operator (not to be confused with =, the variable assignment operator). The operators <= and ...
Exactly what that means depends on the nature of the assignment target (more details on that below). When the assignment target is an identifier, @'' or @"" can be used to mean "the assignment target as a string" (useful for APIs like collections.NamedTuple and typing.NewType
There are various mistakes in your code. First you forgot the = in the first line. Additionally in a dict definition you have to use : to separate the keys from the values.. Next thing is that you have to define new_variable first before you can add something to it.. This will work:
Module-level decorators, classes, and functions¶ @dataclasses.dataclass (*, init=True, repr=True, eq=True, order=False, unsafe_hash=False, frozen=False) ¶ This function is a decorator that is used to add generated special method s to classes, as described below.. The dataclass() decorator examines the class to find field s. A field is defined as class variable that has a type annotation.
はじめにみずほリサーチ&テクノロジーズの @fujine です。本記事では、Pythonの辞書をより便利に使うためのテクニック10個を解説していきます。基本的な操作も多く含まれていますが、パフ…
From Python 3.7+ you can use a Data Class, which achieves what you want and more.. It allows you to define fields for your class, which are attributes automatically assigned.. It would look something like that: @dataclass class Foo: a: str b: int c: str ... The __init__ method will be automatically created in your class, and it will assign the arguments of instance creation to those attributes ...