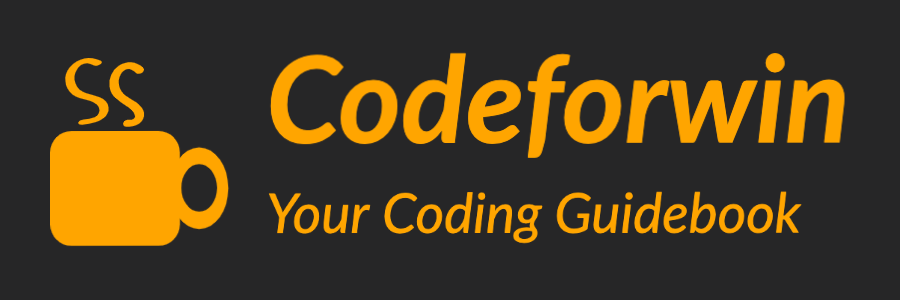

Assignment and shorthand assignment operator in C
Quick links.
- Shorthand assignment
Assignment operator is used to assign value to a variable (memory location). There is a single assignment operator = in C. It evaluates expression on right side of = symbol and assigns evaluated value to left side the variable.
For example consider the below assignment table.
The RHS of assignment operator must be a constant, expression or variable. Whereas LHS must be a variable (valid memory location).
Shorthand assignment operator
C supports a short variant of assignment operator called compound assignment or shorthand assignment. Shorthand assignment operator combines one of the arithmetic or bitwise operators with assignment operator.
For example, consider following C statements.
The above expression a = a + 2 is equivalent to a += 2 .
Similarly, there are many shorthand assignment operators. Below is a list of shorthand assignment operators in C.
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
Assignment Operators in Programming
- Binary Operators in Programming
- Operator Associativity in Programming
- C++ Assignment Operator Overloading
- What are Operators in Programming?
- Assignment Operators In C++
- Bitwise AND operator in Programming
- Increment and Decrement Operators in Programming
- Types of Operators in Programming
- Logical AND operator in Programming
- Modulus Operator in Programming
- Solidity - Assignment Operators
- Augmented Assignment Operators in Python
- Pre Increment and Post Increment Operator in Programming
- Right Shift Operator (>>) in Programming
- JavaScript Assignment Operators
- Move Assignment Operator in C++ 11
- Assignment Operators in Python
- Assignment Operators in C
- Subtraction Assignment( -=) Operator in Javascript
Assignment operators in programming are symbols used to assign values to variables. They offer shorthand notations for performing arithmetic operations and updating variable values in a single step. These operators are fundamental in most programming languages and help streamline code while improving readability.
Table of Content
What are Assignment Operators?
- Types of Assignment Operators
- Assignment Operators in C++
- Assignment Operators in Java
- Assignment Operators in C#
- Assignment Operators in Javascript
- Application of Assignment Operators
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign ( = ), which assigns the value on the right side of the operator to the variable on the left side.
Types of Assignment Operators:
- Simple Assignment Operator ( = )
- Addition Assignment Operator ( += )
- Subtraction Assignment Operator ( -= )
- Multiplication Assignment Operator ( *= )
- Division Assignment Operator ( /= )
- Modulus Assignment Operator ( %= )
Below is a table summarizing common assignment operators along with their symbols, description, and examples:
Assignment Operators in C:
Here are the implementation of Assignment Operator in C language:
Assignment Operators in C++:
Here are the implementation of Assignment Operator in C++ language:
Assignment Operators in Java:
Here are the implementation of Assignment Operator in java language:
Assignment Operators in Python:
Here are the implementation of Assignment Operator in python language:
Assignment Operators in C#:
Here are the implementation of Assignment Operator in C# language:
Assignment Operators in Javascript:
Here are the implementation of Assignment Operator in javascript language:
Application of Assignment Operators:
- Variable Initialization : Setting initial values to variables during declaration.
- Mathematical Operations : Combining arithmetic operations with assignment to update variable values.
- Loop Control : Updating loop variables to control loop iterations.
- Conditional Statements : Assigning different values based on conditions in conditional statements.
- Function Return Values : Storing the return values of functions in variables.
- Data Manipulation : Assigning values received from user input or retrieved from databases to variables.
Conclusion:
In conclusion, assignment operators in programming are essential tools for assigning values to variables and performing operations in a concise and efficient manner. They allow programmers to manipulate data and control the flow of their programs effectively. Understanding and using assignment operators correctly is fundamental to writing clear, efficient, and maintainable code in various programming languages.
Please Login to comment...
Similar reads.
- Programming
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Home » Learn C Programming from Scratch » C Assignment Operators
C Assignment Operators
Summary : in this tutorial, you’ll learn about the C assignment operators and how to use them effectively.
Introduction to the C assignment operators
An assignment operator assigns the vale of the right-hand operand to the left-hand operand. The following example uses the assignment operator (=) to assign 1 to the counter variable:
After the assignmment, the counter variable holds the number 1.
The following example adds 1 to the counter and assign the result to the counter:
The = assignment operator is called a simple assignment operator. It assigns the value of the left operand to the right operand.
Besides the simple assignment operator, C supports compound assignment operators. A compound assignment operator performs the operation specified by the additional operator and then assigns the result to the left operand.
The following example uses a compound-assignment operator (+=):
The expression:
is equivalent to the following expression:
The following table illustrates the compound-assignment operators in C:
- A simple assignment operator assigns the value of the left operand to the right operand.
- A compound assignment operator performs the operation specified by the additional operator and then assigns the result to the left operand.

- C Programming Tutorial
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Assignment Operators in C
In C language, the assignment operator stores a certain value in an already declared variable. A variable in C can be assigned the value in the form of a literal, another variable, or an expression.
The value to be assigned forms the right-hand operand, whereas the variable to be assigned should be the operand to the left of the " = " symbol, which is defined as a simple assignment operator in C.
In addition, C has several augmented assignment operators.
The following table lists the assignment operators supported by the C language −
Simple Assignment Operator (=)
The = operator is one of the most frequently used operators in C. As per the ANSI C standard, all the variables must be declared in the beginning. Variable declaration after the first processing statement is not allowed.
You can declare a variable to be assigned a value later in the code, or you can initialize it at the time of declaration.
You can use a literal, another variable, or an expression in the assignment statement.
Once a variable of a certain type is declared, it cannot be assigned a value of any other type. In such a case the C compiler reports a type mismatch error.
In C, the expressions that refer to a memory location are called "lvalue" expressions. A lvalue may appear as either the left-hand or right-hand side of an assignment.
On the other hand, the term rvalue refers to a data value that is stored at some address in memory. A rvalue is an expression that cannot have a value assigned to it which means an rvalue may appear on the right-hand side but not on the left-hand side of an assignment.
Variables are lvalues and so they may appear on the left-hand side of an assignment. Numeric literals are rvalues and so they may not be assigned and cannot appear on the left-hand side. Take a look at the following valid and invalid statements −
Augmented Assignment Operators
In addition to the = operator, C allows you to combine arithmetic and bitwise operators with the = symbol to form augmented or compound assignment operator. The augmented operators offer a convenient shortcut for combining arithmetic or bitwise operation with assignment.
For example, the expression "a += b" has the same effect of performing "a + b" first and then assigning the result back to the variable "a".
Run the code and check its output −
Similarly, the expression "a <<= b" has the same effect of performing "a << b" first and then assigning the result back to the variable "a".
Here is a C program that demonstrates the use of assignment operators in C −
When you compile and execute the above program, it will produce the following result −
To Continue Learning Please Login
Next: Execution Control Expressions , Previous: Arithmetic , Up: Top [ Contents ][ Index ]
7 Assignment Expressions
As a general concept in programming, an assignment is a construct that stores a new value into a place where values can be stored—for instance, in a variable. Such places are called lvalues (see Lvalues ) because they are locations that hold a value.
An assignment in C is an expression because it has a value; we call it an assignment expression . A simple assignment looks like
We say it assigns the value of the expression value-to-store to the location lvalue , or that it stores value-to-store there. You can think of the “l” in “lvalue” as standing for “left,” since that’s what you put on the left side of the assignment operator.
However, that’s not the only way to use an lvalue, and not all lvalues can be assigned to. To use the lvalue in the left side of an assignment, it has to be modifiable . In C, that means it was not declared with the type qualifier const (see const ).
The value of the assignment expression is that of lvalue after the new value is stored in it. This means you can use an assignment inside other expressions. Assignment operators are right-associative so that
is equivalent to
This is the only useful way for them to associate; the other way,
would be invalid since an assignment expression such as x = y is not valid as an lvalue.
Warning: Write parentheses around an assignment if you nest it inside another expression, unless that is a conditional expression, or comma-separated series, or another assignment.
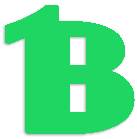
- Learn C Programming
Introduction
- Historical Development of C
- Importance of C
- Basic Structure of C Program
- Executing a C Program
- Compiler, Assembler, and Interpreter
Problem Solving Using Computer
- Problem Analysis
- Types of Errors
- Debugging, Testing, and Program Documentation
- Setting up C Programming Environment
C Fundamentals
- Character Set
- Identifiers and Keywords
- Data Types
- Constants and Variables
- Variable/Constant Declaration
- Pre-processor Directive
- Symbolic Constant
C Operators and Expressions
- Operators and Types
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Assignment Operators
- Conditional Operator
- Increment and Decrement Operators
- Bitwise Operators
- Special Operators
- Precedence and Associativity
C Input and Output
- Input and Output functions
- Unformatted I/O
- Formatted I/O
C Decision-making Statements
- Decision-making Statements in C
- Nested if else
- Else-if ladder
- Switch Case
- Loop Control Statements in C
C Functions
- Get Started
- First Program

Assignment Operators in C
Assignment operators are used to assigning the result of an expression to a variable. Up to now, we have used the shorthand assignment operator “=”, which assigns the result of a right-hand expression to the left-hand variable. For example, in the expression x = y + z, the sum of y and z is assigned to x.
Another form of assignment operator is variable operator_symbol= expression ; which is equivalent to variable = variable operator_symbol expression;
We have the following different types of assignment and assignment short-hand operators.
Expected Output:
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
C Assignment Operators
- 6 contributors
An assignment operation assigns the value of the right-hand operand to the storage location named by the left-hand operand. Therefore, the left-hand operand of an assignment operation must be a modifiable l-value. After the assignment, an assignment expression has the value of the left operand but isn't an l-value.
assignment-expression : conditional-expression unary-expression assignment-operator assignment-expression
assignment-operator : one of = *= /= %= += -= <<= >>= &= ^= |=
The assignment operators in C can both transform and assign values in a single operation. C provides the following assignment operators:
In assignment, the type of the right-hand value is converted to the type of the left-hand value, and the value is stored in the left operand after the assignment has taken place. The left operand must not be an array, a function, or a constant. The specific conversion path, which depends on the two types, is outlined in detail in Type Conversions .
- Assignment Operators
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
Learn C practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c interactively, c introduction.
- Getting Started with C
- Your First C Program
C Fundamentals
- C Variables, Constants and Literals
- C Data Types
- C Input Output (I/O)
C Programming Operators
C flow control.
C if...else Statement
- C while and do...while Loop
- C break and continue
- C switch Statement
- C goto Statement
- C Functions
- C User-defined functions
- Types of User-defined Functions in C Programming
- C Recursion
- C Storage Class
C Programming Arrays
- C Multidimensional Arrays
- Pass arrays to a function in C
C Programming Pointers
- Relationship Between Arrays and Pointers
- C Pass Addresses and Pointers
- C Dynamic Memory Allocation
- C Array and Pointer Examples
- C Programming Strings
- String Manipulations In C Programming Using Library Functions
- String Examples in C Programming
C Structure and Union
- C structs and Pointers
- C Structure and Function
C Programming Files
- C File Handling
- C Files Examples
C Additional Topics
- C Keywords and Identifiers
C Precedence And Associativity Of Operators

C Bitwise Operators
- C Preprocessor and Macros
- C Standard Library Functions
C Tutorials
Bitwise Operators in C Programming
- Compute Quotient and Remainder
- Find the Size of int, float, double and char
- Make a Simple Calculator Using switch...case
An operator is a symbol that operates on a value or a variable. For example: + is an operator to perform addition.
C has a wide range of operators to perform various operations.
C Arithmetic Operators
An arithmetic operator performs mathematical operations such as addition, subtraction, multiplication, division etc on numerical values (constants and variables).
Example 1: Arithmetic Operators
The operators + , - and * computes addition, subtraction, and multiplication respectively as you might have expected.
In normal calculation, 9/4 = 2.25 . However, the output is 2 in the program.
It is because both the variables a and b are integers. Hence, the output is also an integer. The compiler neglects the term after the decimal point and shows answer 2 instead of 2.25 .
The modulo operator % computes the remainder. When a=9 is divided by b=4 , the remainder is 1 . The % operator can only be used with integers.
Suppose a = 5.0 , b = 2.0 , c = 5 and d = 2 . Then in C programming,
C Increment and Decrement Operators
C programming has two operators increment ++ and decrement -- to change the value of an operand (constant or variable) by 1.
Increment ++ increases the value by 1 whereas decrement -- decreases the value by 1. These two operators are unary operators, meaning they only operate on a single operand.
Example 2: Increment and Decrement Operators
Here, the operators ++ and -- are used as prefixes. These two operators can also be used as postfixes like a++ and a-- . Visit this page to learn more about how increment and decrement operators work when used as postfix .
C Assignment Operators
An assignment operator is used for assigning a value to a variable. The most common assignment operator is =
Example 3: Assignment Operators
C relational operators.
A relational operator checks the relationship between two operands. If the relation is true, it returns 1; if the relation is false, it returns value 0.
Relational operators are used in decision making and loops .
Example 4: Relational Operators
C logical operators.
An expression containing logical operator returns either 0 or 1 depending upon whether expression results true or false. Logical operators are commonly used in decision making in C programming .
Example 5: Logical Operators
Explanation of logical operator program
- (a == b) && (c > 5) evaluates to 1 because both operands (a == b) and (c > b) is 1 (true).
- (a == b) && (c < b) evaluates to 0 because operand (c < b) is 0 (false).
- (a == b) || (c < b) evaluates to 1 because (a = b) is 1 (true).
- (a != b) || (c < b) evaluates to 0 because both operand (a != b) and (c < b) are 0 (false).
- !(a != b) evaluates to 1 because operand (a != b) is 0 (false). Hence, !(a != b) is 1 (true).
- !(a == b) evaluates to 0 because (a == b) is 1 (true). Hence, !(a == b) is 0 (false).
During computation, mathematical operations like: addition, subtraction, multiplication, division, etc are converted to bit-level which makes processing faster and saves power.
Bitwise operators are used in C programming to perform bit-level operations.
Visit bitwise operator in C to learn more.
Other Operators
Comma operator.
Comma operators are used to link related expressions together. For example:
The sizeof operator
The sizeof is a unary operator that returns the size of data (constants, variables, array, structure, etc).
Example 6: sizeof Operator
Other operators such as ternary operator ?: , reference operator & , dereference operator * and member selection operator -> will be discussed in later tutorials.
Table of Contents
- Arithmetic Operators
- Increment and Decrement Operators
- Assignment Operators
- Relational Operators
- Logical Operators
- sizeof Operator
Video: Arithmetic Operators in C
Sorry about that.
Related Tutorials
Programtopia
C Programming Operators and Expressions
In this Section, you will learn about Operators in C Programming (all valid operators available in C), expressions (combination of operators, variables and constants) and precedence of operators (which operator has higher priority and which operator has lower priority).
C Operators
- Expressions in C
- C Operator Precedence
Operators are the symbols which tell the computer to execute certain mathematical or logical operations. A mathematical or logical expression is generally formed with the help of an operator. C programming offers a number of operators which are classified into 8 categories viz.
- Arithmetic operators
- Relational operators
- Logical operators
- Assignment operators
- Increment and Decrement operators
- Conditional operators
- Bitwise operators
- Special operators
1. Arithmetic Operators
C programming language provides all basic arithmetic operators: +, -, *, / and %.
Note: ‘/’ is integer division which only gives integer part as result after division. ‘%’ is modulo division which gives the remainder of integer division as result.
Some examples of arithmetic operators are:
In these examples, a and b are variables and are called operands.
Note: ‘%’ cannot be used on floating data type.
2. Relational Operators
Relational operators are used when we have to make comparisons. C programming offers 6 relational operators.
Relational expression is an expression which contains the relational operator. Relational operators are most commonly used in decision statements like if , while , etc. Some simple relational expressions are:
Note: Arithmetic operators have higher priority than relational operators meaning that if arithmetic expressions are present on two sides of a relational operator then arithmetic expressions will be calculated first and then the result will be compared.
3. Logical Operators
Logical operators are used when more than one conditions are to be tested and based on that result, decisions have to be made. C programming offers three logical operators. They are:
For example:
An expression which combines two or more relational expressions is known as logical expression.
Note: Relative precedence of relational and logical operators are as follows
4. Assignment Operators
Assignment operators are used to assign result of an expression to a variable. ‘=’ is the assignment operator in C. Furthermore, C also allows the use of shorthand assignment operators. Shorthand operators take the form:
where var is a variable, op is arithmetic operator, exp is an expression. In this case, ‘op=’ is known as shorthand assignment operator.
The above assignment
is the same as the assignment
Consider an example:
Here, the above statement means the same as
Note: Shorthand assignment can be used with all arithmetic operators.
5. Increment and Decrement Operators
C programming allows the use of ++ and – operators which are increment and decrement operators respectively. Both the increment and decrement operators are unary operators. The increment operator ++ adds 1 to the operand and the decrement operator – subtracts 1 from the operand. The general syntax of these operators are:
Increment Operator: m++ or ++m ;
Decrement Operator: m–or –m ;
In the example above, m++ simply means m=m+1; and m– simply means m=m-1;
Increment and decrement operators are mostly used in for and while loops.
++m and m++ performs the same operation when they form statements independently but they function differently when they are used in right hand side of an expression.
++m is known as prefix operator and m++ is known as postfix operator. A prefix operator firstly adds 1 to the operand and then the result is assigned to the variable on the left whereas a postfix operator firstly assigns value to the variable on the left and then increases the operand by 1. Same is in the case of decrement operator.
For example,
X=10; Y=++X;
In this case, the value of X and Y will be 6.
In this case, the value of Y will be 10 and the value of X will be 11.
6. Conditional Operator
The operator pair “?” and “:” is known as conditional operator. These pair of operators are ternary operators. The general syntax of conditional operator is:
This syntax can be understood as a substitute of if else statement.
Consider an if else statement as:
Now, this if else statement can be written by using conditional operator as:
7. Bitwise Operator
In C programming, bitwise operators are used for testing the bits or shifting them left or right. The bitwise operators available in C are:
8. Special Operators
C programming supports special operators like comma operator, sizeof operator, pointer operators (& and *) and member selection operators (. and ->). The comma operator and sizeof operator are discussed in this section whereas the pointer and member selection operators are discussed in later sections.
1. Comma Operator
The comma operator can be used to link the related expressions together. A comma linked expression is evaluated from left to right and the value of the right most expression is the value of the combined expression.
In this example, the expression is evaluated from left to right. So at first, variable a is assigned value 2, then variable b is assigned value 4 and then value 6 is assigned to the variable x. Comma operators are commonly used in for loops, while loops, while exchanging values, etc.
2 .Sizeof() operator
The sizeof operator is usually used with an operand which may be variable, constant or a data type qualifier. This operator returns the number of bytes the operand occupies. Sizeof operator is a compile time operator. Some examples of use of sizeof operator are:
The sizeof operator is usually used to determine the length of arrays and structures when their sizes are not known. It is also used in dynamic memory allocation.
9. C Expressions
Arithmetic expression in C is a combination of variables, constants and operators written in a proper syntax. C can easily handle any complex mathematical expressions but these mathematical expressions have to be written in a proper syntax. Some examples of mathematical expressions written in proper syntax of C are:
Note: C does not have any operator for exponentiation.
10. C Operator Precedence
At first, the expressions within parenthesis are evaluated. If no parenthesis is present, then the arithmetic expression is evaluated from left to right. There are two priority levels of operators in C.
High priority: * / % Low priority: + –
The evaluation procedure of an arithmetic expression includes two left to right passes through the entire expression. In the first pass, the high priority operators are applied as they are encountered and in the second pass, low priority operations are applied as they are encountered.
Suppose, we have an arithmetic expression as:
This expression is evaluated in two left to right passes as:
But when parenthesis is used in the same expression, the order of evaluation gets changed.
When parentheses are present then the expression inside the parenthesis are evaluated first from left to right. The expression is now evaluated in three passes as:
Second Pass
There may even arise a case where nested parentheses are present (i.e. parenthesis inside parenthesis). In such case, the expression inside the innermost set of parentheses is evaluated first and then the outer parentheses are evaluated.
For example, we have an expression as:
The expression is now evaluated as:
First Pass:
Note: The number of evaluation steps is equal to the number of operators in the arithmetic expression.

- school Campus Bookshelves
- menu_book Bookshelves
- perm_media Learning Objects
- login Login
- how_to_reg Request Instructor Account
- hub Instructor Commons
Margin Size
- Download Page (PDF)
- Download Full Book (PDF)
- Periodic Table
- Physics Constants
- Scientific Calculator
- Reference & Cite
- Tools expand_more
- Readability
selected template will load here
This action is not available.
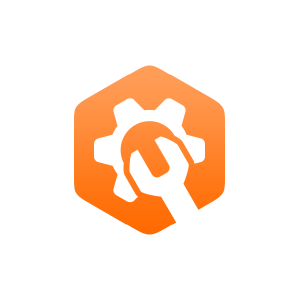
4.6: Assignment Operator
- Last updated
- Save as PDF
- Page ID 29038
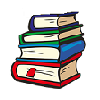
- Patrick McClanahan
- San Joaquin Delta College
\( \newcommand{\vecs}[1]{\overset { \scriptstyle \rightharpoonup} {\mathbf{#1}} } \)
\( \newcommand{\vecd}[1]{\overset{-\!-\!\rightharpoonup}{\vphantom{a}\smash {#1}}} \)
\( \newcommand{\id}{\mathrm{id}}\) \( \newcommand{\Span}{\mathrm{span}}\)
( \newcommand{\kernel}{\mathrm{null}\,}\) \( \newcommand{\range}{\mathrm{range}\,}\)
\( \newcommand{\RealPart}{\mathrm{Re}}\) \( \newcommand{\ImaginaryPart}{\mathrm{Im}}\)
\( \newcommand{\Argument}{\mathrm{Arg}}\) \( \newcommand{\norm}[1]{\| #1 \|}\)
\( \newcommand{\inner}[2]{\langle #1, #2 \rangle}\)
\( \newcommand{\Span}{\mathrm{span}}\)
\( \newcommand{\id}{\mathrm{id}}\)
\( \newcommand{\kernel}{\mathrm{null}\,}\)
\( \newcommand{\range}{\mathrm{range}\,}\)
\( \newcommand{\RealPart}{\mathrm{Re}}\)
\( \newcommand{\ImaginaryPart}{\mathrm{Im}}\)
\( \newcommand{\Argument}{\mathrm{Arg}}\)
\( \newcommand{\norm}[1]{\| #1 \|}\)
\( \newcommand{\Span}{\mathrm{span}}\) \( \newcommand{\AA}{\unicode[.8,0]{x212B}}\)
\( \newcommand{\vectorA}[1]{\vec{#1}} % arrow\)
\( \newcommand{\vectorAt}[1]{\vec{\text{#1}}} % arrow\)
\( \newcommand{\vectorB}[1]{\overset { \scriptstyle \rightharpoonup} {\mathbf{#1}} } \)
\( \newcommand{\vectorC}[1]{\textbf{#1}} \)
\( \newcommand{\vectorD}[1]{\overrightarrow{#1}} \)
\( \newcommand{\vectorDt}[1]{\overrightarrow{\text{#1}}} \)
\( \newcommand{\vectE}[1]{\overset{-\!-\!\rightharpoonup}{\vphantom{a}\smash{\mathbf {#1}}}} \)
Assignment Operator
The assignment operator allows us to change the value of a modifiable data object (for beginning programmers this typically means a variable). It is associated with the concept of moving a value into the storage location (again usually a variable). Within C++ programming language the symbol used is the equal symbol. But bite your tongue, when you see the = symbol you need to start thinking: assignment. The assignment operator has two operands. The one to the left of the operator is usually an identifier name for a variable. The one to the right of the operator is a value.
The value 21 is moved to the memory location for the variable named: age. Another way to say it: age is assigned the value 21.
The item to the right of the assignment operator is an expression. The expression will be evaluated and the answer is 14. The value 14 would assigned to the variable named: total_cousins.
The expression to the right of the assignment operator contains some identifier names. The program would fetch the values stored in those variables; add them together and get a value of 44; then assign the 44 to the total_students variable.
As we have seen, assignment operators are used to assigning value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of the variable on the left side otherwise the compiler will raise an error. Different types of assignment operators are shown below:
- “=” : This is the simplest assignment operator, which was discussed above. This operator is used to assign the value on the right to the variable on the left. For example: a = 10; b = 20; ch = 'y';
If initially the value 5 is stored in the variable a, then: (a += 6) is equal to 11. (the same as: a = a + 6)
If initially value 8 is stored in the variable a, then (a -= 6) is equal to 2. (the same as a = a - 6)
If initially value 5 is stored in the variable a,, then (a *= 6) is equal to 30. (the same as a = a * 6)
If initially value 6 is stored in the variable a, then (a /= 2) is equal to 3. (the same as a = a / 2)
Below example illustrates the various Assignment Operators:
Definitions
Adapted from: "Assignment Operator" by Kenneth Leroy Busbee , (Download for free at http://cnx.org/contents/[email protected] ) is licensed under CC BY 4.0
cppreference.com
Assignment operators.
Assignment and compound assignment operators are binary operators that modify the variable to their left using the value to their right.
[ edit ] Simple assignment
The simple assignment operator expressions have the form
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs .
Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non-lvalue (so that expressions such as ( a = b ) = c are invalid).
rhs and lhs must satisfy one of the following:
- both lhs and rhs have compatible struct or union type, or..
- rhs must be implicitly convertible to lhs , which implies
- both lhs and rhs have arithmetic types , in which case lhs may be volatile -qualified or atomic (since C11)
- both lhs and rhs have pointer to compatible (ignoring qualifiers) types, or one of the pointers is a pointer to void, and the conversion would not add qualifiers to the pointed-to type. lhs may be volatile or restrict (since C99) -qualified or atomic (since C11) .
- lhs is a (possibly qualified or atomic (since C11) ) pointer and rhs is a null pointer constant such as NULL or a nullptr_t value (since C23)
[ edit ] Notes
If rhs and lhs overlap in memory (e.g. they are members of the same union), the behavior is undefined unless the overlap is exact and the types are compatible .
Although arrays are not assignable, an array wrapped in a struct is assignable to another object of the same (or compatible) struct type.
The side effect of updating lhs is sequenced after the value computations, but not the side effects of lhs and rhs themselves and the evaluations of the operands are, as usual, unsequenced relative to each other (so the expressions such as i = ++ i ; are undefined)
Assignment strips extra range and precision from floating-point expressions (see FLT_EVAL_METHOD ).
In C++, assignment operators are lvalue expressions, not so in C.
[ edit ] Compound assignment
The compound assignment operator expressions have the form
The expression lhs @= rhs is exactly the same as lhs = lhs @ ( rhs ) , except that lhs is evaluated only once.
[ edit ] References
- C17 standard (ISO/IEC 9899:2018):
- 6.5.16 Assignment operators (p: 72-73)
- C11 standard (ISO/IEC 9899:2011):
- 6.5.16 Assignment operators (p: 101-104)
- C99 standard (ISO/IEC 9899:1999):
- 6.5.16 Assignment operators (p: 91-93)
- C89/C90 standard (ISO/IEC 9899:1990):
- 3.3.16 Assignment operators
[ edit ] See Also
Operator precedence
[ edit ] See also
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 19 August 2022, at 09:36.
- This page has been accessed 58,085 times.
- Privacy policy
- About cppreference.com
- Disclaimers


COMMENTS
C supports a short variant of assignment operator called compound assignment or shorthand assignment. Shorthand assignment operator combines one of the arithmetic or bitwise operators with assignment operator. For example, consider following C statements. The above expression a = a + 2 is equivalent to a += 2.
Different types of assignment operators are shown below: 1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: a = 10; b = 20; ch = 'y'; 2. "+=": This operator is combination of '+' and '=' operators. This operator first adds the current ...
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
Summary: in this tutorial, you'll learn about the C assignment operators and how to use them effectively.. Introduction to the C assignment operators. An assignment operator assigns the vale of the right-hand operand to the left-hand operand. The following example uses the assignment operator (=) to assign 1 to the counter variable:
These operators allow you to write concise and easy t... In this video, we'll teach you how to use the shorthand assignment operators in C programming language.
Simple assignment operator. Assigns values from right side operands to left side operand. C = A + B will assign the value of A + B to C. +=. Add AND assignment operator. It adds the right operand to the left operand and assign the result to the left operand. C += A is equivalent to C = C + A. -=.
Shorthand assignment operator is used express the syntax that are already available in shorter wayCheck out our website: http://www.telusko.comFollow Telusko...
7 Assignment Expressions. As a general concept in programming, an assignment is a construct that stores a new value into a place where values can be stored—for instance, in a variable. Such places are called lvalues (see Lvalues) because they are locations that hold a value. An assignment in C is an expression because it has a value; we call it an assignment expression.
The += assignment operator is a combination of + arithmetic operator and = simple assignment operator. For example, x += y; is equivalent to x = x+y;. It adds the right side value to the value of left side operand and assign the result back to the left-hand side operand. #include <stdio.h> int main () { int x = 100, y = 20, z = 50 ...
Assignment operators are used to assigning the result of an expression to a variable. Up to now, we have used the shorthand assignment operator "=", which assigns the result of a right-hand expression to the left-hand variable. For example, in the expression x = y + z, the sum of y and z is assigned to x.
The assignment operators in C can both transform and assign values in a single operation. C provides the following assignment operators: | =. In assignment, the type of the right-hand value is converted to the type of the left-hand value, and the value is stored in the left operand after the assignment has taken place.
The Assignment operators in C are some of the Programming operators that are useful for assigning the values to the declared variables. Equals (=) operator is the most commonly used assignment operator. For example: int i = 10; The below table displays all the assignment operators present in C Programming with an example. C Assignment Operators.
C Programming & Data Structures: Assignment Operators in CTopics discussed:1. Introduction to Assignment Operators in C language.2. Types of Shorthand Assign...
An operator is a symbol that operates on a value or a variable. For example: + is an operator to perform addition. In this tutorial, you will learn about different C operators such as arithmetic, increment, assignment, relational, logical, etc. with the help of examples.
Shorthand operators take the form: var op = exp; where var is a variable, op is arithmetic operator, exp is an expression. In this case, 'op=' is known as shorthand assignment operator. The above assignment. var op = exp; is the same as the assignment. var = var op exp; Consider an example: x += y; Here, the above statement means the same ...
This operator first subtracts the current value of the variable on left from the value on the right and then assigns the result to the variable on the left. Example: (a -= b) can be written as (a = a - b) If initially value 8 is stored in the variable a, then (a -= 6) is equal to 2. (the same as a = a - 6)
Introduction to C Programming Operators The Simple Assignment Operator. The equals sign, =, is known as the assignment operator in C; The purpose of the assignment operator is to take the value from the right hand side of the operator ( the RHS value ), and store it in the variable on the left hand side ( the LHS ). Note the following examples:
Shorthand Operator in C - C has a special operator called shorthand that simplifies coding a certain type of assignment statements. Example for Shorthand Operator in C. a = a+2; can be written as: a += 2; The operator += tells the compiler that a is assigned the value of a + 2; This shorthand works for all binary operators in C.
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs . Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non ...
Assignment Operators in C Language | Shorthand operators in C Language. Assignment/shorthand operators are used to update the value of a variable in an easy way. There are various assignment operators provided by C language.
Relational Operators. Shorthand Operators are operators that combine one of the arithmetic or bitwise operators with the assignment operator. Shorthand Operators are a shorter way of expressing something that is already available in the programming statements. Expressions or Values can be assigned to the variable using Shorthand Operators.
c++; assignment-operator; shorthand; Share. Improve this question. Follow asked Sep 12, 2019 at 16:15. Kshitiz Kamal Kshitiz Kamal. 141 1 1 silver badge 6 6 bronze badges. 3. 3. With integer division: 3/2 is 1. - Jarod42. ... Example of emacs setup code written in elisp
It's most commonly used in assignment operations, although it has other uses as well. The ternary operator ? is a way of shortening an if-else clause, and is also called an immediate-if statement in other languages ( IIf(condition,true-clause,false-clause) in VB, for example). For example: bool Three = SOME_VALUE;