JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript assignment, javascript assignment operators.
Assignment operators assign values to JavaScript variables.

Shift Assignment Operators
Bitwise assignment operators, logical assignment operators, the = operator.
The Simple Assignment Operator assigns a value to a variable.
Simple Assignment Examples
The += operator.
The Addition Assignment Operator adds a value to a variable.
Addition Assignment Examples
The -= operator.
The Subtraction Assignment Operator subtracts a value from a variable.
Subtraction Assignment Example
The *= operator.
The Multiplication Assignment Operator multiplies a variable.
Multiplication Assignment Example
The **= operator.
The Exponentiation Assignment Operator raises a variable to the power of the operand.
Exponentiation Assignment Example
The /= operator.
The Division Assignment Operator divides a variable.
Division Assignment Example
The %= operator.
The Remainder Assignment Operator assigns a remainder to a variable.
Remainder Assignment Example
Advertisement
The <<= Operator
The Left Shift Assignment Operator left shifts a variable.
Left Shift Assignment Example
The >>= operator.
The Right Shift Assignment Operator right shifts a variable (signed).
Right Shift Assignment Example
The >>>= operator.
The Unsigned Right Shift Assignment Operator right shifts a variable (unsigned).
Unsigned Right Shift Assignment Example
The &= operator.
The Bitwise AND Assignment Operator does a bitwise AND operation on two operands and assigns the result to the the variable.
Bitwise AND Assignment Example
The |= operator.
The Bitwise OR Assignment Operator does a bitwise OR operation on two operands and assigns the result to the variable.
Bitwise OR Assignment Example
The ^= operator.
The Bitwise XOR Assignment Operator does a bitwise XOR operation on two operands and assigns the result to the variable.
Bitwise XOR Assignment Example
The &&= operator.
The Logical AND assignment operator is used between two values.
If the first value is true, the second value is assigned.
Logical AND Assignment Example
The &&= operator is an ES2020 feature .
The ||= Operator
The Logical OR assignment operator is used between two values.
If the first value is false, the second value is assigned.
Logical OR Assignment Example
The ||= operator is an ES2020 feature .
The ??= Operator
The Nullish coalescing assignment operator is used between two values.
If the first value is undefined or null, the second value is assigned.
Nullish Coalescing Assignment Example
The ??= operator is an ES2020 feature .
Test Yourself With Exercises
Use the correct assignment operator that will result in x being 15 (same as x = x + y ).
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Specifications
Browser compatibility.
An assignment operator assigns a value to its left operand based on the value of its right operand.
The source for this interactive example is stored in a GitHub repository. If you'd like to contribute to the interactive examples project, please clone https://github.com/mdn/interactive-examples and send us a pull request.
The basic assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x . The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
Simple assignment operator is used to assign a value to a variable. The assignment operation evaluates to the assigned value. Chaining the assignment operator is possible in order to assign a single value to multiple variables. See the example.
Addition assignment
The addition assignment operator adds the value of the right operand to a variable and assigns the result to the variable. The types of the two operands determine the behavior of the addition assignment operator. Addition or concatenation is possible. See the addition operator for more details.
Subtraction assignment
The subtraction assignment operator subtracts the value of the right operand from a variable and assigns the result to the variable. See the subtraction operator for more details.
Multiplication assignment
The multiplication assignment operator multiplies a variable by the value of the right operand and assigns the result to the variable. See the multiplication operator for more details.
Division assignment
The division assignment operator divides a variable by the value of the right operand and assigns the result to the variable. See the division operator for more details.
Remainder assignment
The remainder assignment operator divides a variable by the value of the right operand and assigns the remainder to the variable. See the remainder operator for more details.
Exponentiation assignment
The exponentiation assignment operator evaluates to the result of raising first operand to the power second operand. See the exponentiation operator for more details.
Left shift assignment
The left shift assignment operator moves the specified amount of bits to the left and assigns the result to the variable. See the left shift operator for more details.
Right shift assignment
The right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the right shift operator for more details.
Unsigned right shift assignment
The unsigned right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the unsigned right shift operator for more details.
Bitwise AND assignment
The bitwise AND assignment operator uses the binary representation of both operands, does a bitwise AND operation on them and assigns the result to the variable. See the bitwise AND operator for more details.

Bitwise XOR assignment
The bitwise XOR assignment operator uses the binary representation of both operands, does a bitwise XOR operation on them and assigns the result to the variable. See the bitwise XOR operator for more details.
Bitwise OR assignment
The bitwise OR assignment operator uses the binary representation of both operands, does a bitwise OR operation on them and assigns the result to the variable. See the bitwise OR operator for more details.
Left operand with another assignment operator
In unusual situations, the assignment operator (e.g. x += y ) is not identical to the meaning expression (here x = x + y ). When the left operand of an assignment operator itself contains an assignment operator, the left operand is evaluated only once. For example:
- Arithmetic operators
Document Tags and Contributors
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Using promises
- Iterators and generators
- Meta programming
- JavaScript modules
- Client-side web APIs
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.ListFormat
- Intl.Locale
- Intl.NumberFormat
- Intl.PluralRules
- Intl.RelativeTimeFormat
- ReferenceError
- SharedArrayBuffer
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Array comprehensions
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical operators
- Object initializer
- Operator precedence
- (currently at stage 1) pipes the value of an expression into a function. This allows the creation of chained function calls in a readable manner. The result is syntactic sugar in which a function call with a single argument can be written like this:">Pipeline operator
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for await...of
- for each...in
- function declaration
- import.meta
- try...catch
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- The arguments object
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: can't access lexical declaration`X' before initialization
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: 'x' is not iterable
- TypeError: More arguments needed
- TypeError: Reduce of empty array with no initial value
- TypeError: can't access dead object
- TypeError: can't access property "x" of "y"
- TypeError: can't assign to property "x" on "y": not an object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cannot use 'in' operator to search for 'x' in 'y'
- TypeError: cyclic object value
- TypeError: invalid 'instanceof' operand 'x'
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- X.prototype.y called on incompatible type
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
Learn the best of web development
Get the latest and greatest from MDN delivered straight to your inbox.
Thanks! Please check your inbox to confirm your subscription.
If you haven’t previously confirmed a subscription to a Mozilla-related newsletter you may have to do so. Please check your inbox or your spam filter for an email from us.
The <<= operator shifts the bits of result left by the number of bits specified in expression . For example:
The variable temp has a value of 56 because 14 (00001110 in binary) shifted left two bits equals 56 (00111000 in binary). Bits are filled in with zeroes when shifting.
For information on when a run-time error is generated by the <<= operator, see the Operator Behavior table.
See also: << Operator , >> Operator , >>> Operator , Operator Behavior , Operator Precedence , Operator Summary
- Python - Home
- Python - Introduction
- Python - Syntax
- Python - Comments
- Python - Variables
- Python - Data Types
- Python - Numbers
- Python - Type Casting
- Python - Operators
- Python - Booleans
- Python - Strings
- Python - Lists
- Python - Tuples
- Python - Sets
- Python - Dictionary
- Python - If Else
- Python - While Loop
- Python - For Loop
- Python - Continue Statement
- Python - Break Statement
- Python - Functions
- Python - Lambda Function
- Python - Scope of Variables
- Python - Modules
- Python - Date & Time
- Python - Iterators
- Python - JSON
- Python - File Handling
- Python - Try Except
- Python - Arrays
- Python - Classes/Objects
- Python - Inheritance
- Python - Decorators
- Python - RegEx
- Python - Operator Overloading
- Python - Built-in Functions
- Python - Keywords
- Python - String Methods
- Python - File Handling Methods
- Python - List Methods
- Python - Tuple Methods
- Python - Set Methods
- Python - Dictionary Methods
- Python - Math Module
- Python - cMath Module
- Python - Data Structures
- Python - Examples
- Python - Q&A
- Python - Interview Questions
- Python - NumPy
- Python - Pandas
- Python - Matplotlib
- Python - SciPy
- Python - Seaborn
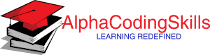
- Programming Languages
- Web Technologies
- Database Technologies
- Microsoft Technologies
- Python Libraries
- Data Structures
- Interview Questions
- PHP & MySQL
- C++ Standard Library
- C Standard Library
- Java Utility Library
- Java Default Package
- PHP Function Reference
Python - left shift operator assignment
The Bitwise left shift assignment operator (<<=) assigns the first operand a value equal to the result of Bitwise left shift operation of two operands.
(x <<= y) is equivalent to (x = x << y)
The Bitwise left shift operator (<<) takes the two numbers and left shift the bits of first operand by number of place specified by second operand. For example: for left shifting the bits of x by y places, the expression ( x<<y ) can be used. It is equivalent to multiplying x by 2 y .
The example below describes how left shift operator works:
The code of using left shift operator (<<) is given below:
The output of the above code will be:
Example: Count number of 1 Bits in a positive integer
Consider an integer 1000. In the bit-wise format, it can be written as 1111101000. However, all bits are not written here. A complete representation will be 32 bit representation as given below:
Bitwise AND operation with 1 at any bit results into 1 if the bit is 1 or 0 if the bit is 0. Performing such operation at every bit, and counting the number of 1 gives the count of 1 bits in the given positive integer. To achieve this bitwise left shift assignment operator can be used as shown in the example below:
The above code will give the following output:
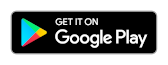
- Data Structures Tutorial
- Algorithms Tutorial
- JavaScript Tutorial
- Python Tutorial
- MySQLi Tutorial
- Java Tutorial
- Scala Tutorial
- C++ Tutorial
- C# Tutorial
- PHP Tutorial
- MySQL Tutorial
- SQL Tutorial
- PHP Function reference
- C++ - Standard Library
- Java.lang Package
- Ruby Tutorial
- Rust Tutorial
- Swift Tutorial
- Perl Tutorial
- HTML Tutorial
- CSS Tutorial
- AJAX Tutorial
- XML Tutorial
- Online Compilers
- QuickTables
- NumPy Tutorial
- Pandas Tutorial
- Matplotlib Tutorial
- SciPy Tutorial
- Seaborn Tutorial
- Skip to main content
- Select language
- Skip to search
- Assignment operators
An assignment operator assigns a value to its left operand based on the value of its right operand.
The basic assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x . The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
Simple assignment operator which assigns a value to a variable. The assignment operation evaluates to the assigned value. Chaining the assignment operator is possible in order to assign a single value to multiple variables. See the example.
Addition assignment
The addition assignment operator adds the value of the right operand to a variable and assigns the result to the variable. The types of the two operands determine the behavior of the addition assignment operator. Addition or concatenation is possible. See the addition operator for more details.
Subtraction assignment
The subtraction assignment operator subtracts the value of the right operand from a variable and assigns the result to the variable. See the subtraction operator for more details.
Multiplication assignment
The multiplication assignment operator multiplies a variable by the value of the right operand and assigns the result to the variable. See the multiplication operator for more details.
Division assignment
The division assignment operator divides a variable by the value of the right operand and assigns the result to the variable. See the division operator for more details.
Remainder assignment
The remainder assignment operator divides a variable by the value of the right operand and assigns the remainder to the variable. See the remainder operator for more details.
Exponentiation assignment
This is an experimental technology, part of the ECMAScript 2016 (ES7) proposal. Because this technology's specification has not stabilized, check the compatibility table for usage in various browsers. Also note that the syntax and behavior of an experimental technology is subject to change in future version of browsers as the spec changes.
The exponentiation assignment operator evaluates to the result of raising first operand to the power second operand. See the exponentiation operator for more details.
Left shift assignment
The left shift assignment operator moves the specified amount of bits to the left and assigns the result to the variable. See the left shift operator for more details.
Right shift assignment
The right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the right shift operator for more details.
Unsigned right shift assignment
The unsigned right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the unsigned right shift operator for more details.
Bitwise AND assignment
The bitwise AND assignment operator uses the binary representation of both operands, does a bitwise AND operation on them and assigns the result to the variable. See the bitwise AND operator for more details.
Bitwise XOR assignment
The bitwise XOR assignment operator uses the binary representation of both operands, does a bitwise XOR operation on them and assigns the result to the variable. See the bitwise XOR operator for more details.
Bitwise OR assignment
The bitwise OR assignment operator uses the binary representation of both operands, does a bitwise OR operation on them and assigns the result to the variable. See the bitwise OR operator for more details.
Left operand with another assignment operator
In unusual situations, the assignment operator (e.g. x += y ) is not identical to the meaning expression (here x = x + y ). When the left operand of an assignment operator itself contains an assignment operator, the left operand is evaluated only once. For example:
Specifications
Browser compatibility.
- Arithmetic operators
Document Tags and Contributors
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Array comprehensions
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical Operators
- Object initializer
- Operator precedence
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- function declaration
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
JS Arithmetic Operators
- Addition(+) Arithmetic Operator in JavaScript
- Subtraction(-) Arithmetic Operator in JavaScript
- Multiplication(*) Arithmetic Operator in JavaScript
- Division(/) Arithmetic Operator in JavaScript
- Modulus(%) Arithmetic Operator in JavaScript
- Exponentiation(**) Arithmetic Operator in JavaScript
- Increment(+ +) Arithmetic Operator in JavaScript
- Decrement(--) Arithmetic Operator in JavaScript
- JavaScript Arithmetic Unary Plus(+) Operator
- JavaScript Arithmetic Unary Negation(-) Operator
JS Assignment Operators
- Addition Assignment (+=) Operator in Javascript
- Subtraction Assignment( -=) Operator in Javascript
- Multiplication Assignment(*=) Operator in JavaScript
- Division Assignment(/=) Operator in JavaScript
- JavaScript Remainder Assignment(%=) Operator
- Exponentiation Assignment(**=) Operator in JavaScript
- Left Shift Assignment (<<=) Operator in JavaScript
Right Shift Assignment(>>=) Operator in JavaScript
- Bitwise AND Assignment (&=) Operator in JavaScript
- Bitwise OR Assignment (|=) Operator in JavaScript
- Bitwise XOR Assignment (^=) Operator in JavaScript
- JavaScript Logical AND assignment (&&=) Operator
- JavaScript Logical OR assignment (||=) Operator
- Nullish Coalescing Assignment (??=) Operator in JavaScript
JS Comparison Operators
- Equality(==) Comparison Operator in JavaScript
- Inequality(!=) Comparison Operator in JavaScript
- Strict Equality(===) Comparison Operator in JavaScript
- Strict Inequality(!==) Comparison Operator in JavaScript
- Greater than(>) Comparison Operator in JavaScript
- Greater Than or Equal(>=) Comparison Operator in JavaScript
- Less Than or Equal(
JS Logical Operators
- NOT(!) Logical Operator inJavaScript
- AND(&&) Logical Operator in JavaScript
- OR(||) Logical Operator in JavaScript
JS Bitwise Operators
- AND(&) Bitwise Operator in JavaScript
- OR(|) Bitwise Operator in JavaScript
- XOR(^) Bitwise Operator in JavaScript
- NOT(~) Bitwise Operator in JavaScript
- Left Shift (
- Right Shift (>>) Bitwise Operator in JavaScript
- Zero Fill Right Shift (>>>) Bitwise Operator in JavaScript
JS Unary Operators
- JavaScript typeof Operator
- JavaScript delete Operator
JS Relational Operators
- JavaScript in Operator
- JavaScript Instanceof Operator
JS Other Operators
- JavaScript String Operators
- JavaScript yield Operator
- JavaScript Pipeline Operator
- JavaScript Grouping Operator
The Right Shift Assignment Operator is represented by “>>=”. This operator shifts the first operand to the right and assigns the result to the variable. It can also be explained as shifting the first operand to the right in a specified amount of bits which is the second operand integer and then assigning the result to the first operand.
Where –
- a is the first operand, and
- b is the second operand.
Example 1: In this example, we will see the implementation of the right shift assignment.
Example 2: In this example, we will see assigning the right shift operator to the variable.
We have a complete list of Javascript Assignment Operators, Please check this article Javascript Assignment Operator .
Supported Browser:
Please Login to comment...
Similar reads.
- Web Technologies

Improve your Coding Skills with Practice
What kind of Experience do you want to share?

IMAGES
VIDEO
COMMENTS
The left shift assignment (<<=) operator performs left shift on the two operands and assigns the result to the left operand. Try it. Syntax. js. x <<= y Description. x <<= y is equivalent to x = x << y, except that the expression x is only evaluated once. Examples. Using left shift assignment. js.
The Left Shift Assignment Operator is represented by "<<=". This operator moves the specified number of bits to the left and assigns that result to the variable. We can fill the vacated place by 0. The left shift operator treats the integer stored in the variable to the operator's left as a 32-bit binary number. This can also be explained ...
Bitwise Shift Operators. They are classified into two categories left shift and the right shift. Left Shift(<<): The left shift operator, shifts all of the bits in value to the left a specified number of times. Syntax: value << num. Here num specifies the number of position to left-shift the value in value.
The Bitwise left shift assignment operator (<<=) assigns the first operand a value equal to the result of Bitwise left shift operation of two operands. The Bitwise left shift operator (<<) takes the two numbers and left shift the bits of first operand by number of place specified by second operand. For example: for left shifting the bits of x ...
Use the correct assignment operator that will result in x being 15 (same as x = x + y ). Start the Exercise. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
The left shift assignment operator (<<=) moves the specified amount of bits to the left and assigns the result to the variable. The source for this interactive example is stored in a GitHub repository. If you'd like to contribute to the interactive examples project, ...
The basic assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x. The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples. Name. Shorthand operator.
The Bitwise left shift assignment operator (<<=) assigns the first operand a value equal to the result of Bitwise left shift operation of two operands. The Bitwise left shift operator (<<) takes the two numbers and left shift the bits of first operand by number of place specified by second operand. For example: for left shifting the bits of x ...
The <<= operator shifts the bits of result left by the number of bits specified in expression. For example: temp = 14. temp <<= 2. document.write (temp); To run the code above, paste it into JavaScript Editor, and click the Execute button. The variable temp has a value of 56 because 14 (00001110 in binary) shifted left two bits equals 56 ...
The bitwise left shift operator can be used to clear a specific bit in an integer to 0. This is done by left shifting 1 by the desired bit position, taking the bitwise complement (~) of the result, and then using the bitwise AND (&) operator to clear the bit. // Clear the nth bit to 0. int bitmask = ~(1 << n);
Left Shift (<<) It is a binary operator that takes two numbers, left shifts the bits of the first operand, and the second operand decides the number of places to shift. In other words, left-shifting an integer " a " with an integer " b " denoted as ' (a<<b)' is equivalent to multiplying a with 2^b (2 raised to power b). Syntax: a << b;
Expressions and operators. Left shift (<<) The left shift operator ( <<) shifts the first operand the specified number of bits to the left. Excess bits shifted off to the left are discarded. Zero bits are shifted in from the right. JavaScript Demo: Expressions - Left shift operator. 9.
We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to the variable on the left side. Types of Assignment Operators: Simple ...
The Bitwise left shift assignment operator (<<=) assigns the first operand a value equal to the result of Bitwise left shift operation of two operands. The Bitwise left shift operator (<<) takes the two numbers and left shift the bits of first operand by number of place specified by second operand. For example: for left shifting the bits of x ...
An assignment operator assigns a value to its left operand based on the value of its right operand.. Overview. The basic assignment operator is equal (=), which assigns the value of its right operand to its left operand.That is, x = y assigns the value of y to x.The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
In the following expression, the left shift assignment operation is carried. int x = 7; x <<= 32; printf("x = %d\n",x); ... Precedence while using right and left shift operators. 39. Why does it make a difference if left and right shift are used together in one expression or not? 1.
The syntax of the left-shift operator in Java is given below, Syntax: x << n. Here, x: an integer. n: a non-negative integer. Return type: An integer after shifting x by n positions toward left. Exception: When n is negative the output is undefined. Below is the program to illustrate how we can use the left shift operator in Java.
I've been trying to implement a lightweight unsigned big numbers (big endian) class in c++, and am storing the numbers as base-2^32 numbers in a uint32_t array. But i've have been having some troub...
The Right Shift Assignment Operator is represented by ">>=".This operator shifts the first operand to the right and assigns the result to the variable. It can also be explained as shifting the first operand to the right in a specified amount of bits which is the second operand integer and then assigning the result to the first operand.