The Modal component is a basic way to present content above an enclosing view.

View Props
Inherits View Props .
Deprecated. Use the animationType prop instead.
animationType
The animationType prop controls how the modal animates.
Possible values:
- slide slides in from the bottom,
- fade fades into view,
- none appears without an animation.
Type | Default |
---|---|
enum( , , ) |
hardwareAccelerated Android
The hardwareAccelerated prop controls whether to force hardware acceleration for the underlying window.
Type | Default |
---|---|
bool |
onDismiss iOS
The onDismiss prop allows passing a function that will be called once the modal has been dismissed.
Type |
---|
function |
onOrientationChange iOS
The onOrientationChange callback is called when the orientation changes while the modal is being displayed. The orientation provided is only 'portrait' or 'landscape'. This callback is also called on initial render, regardless of the current orientation.
onRequestClose
The onRequestClose callback is called when the user taps the hardware back button on Android or the menu button on Apple TV. Because of this required prop, be aware that BackHandler events will not be emitted as long as the modal is open. On iOS, this callback is called when a Modal is being dismissed using a drag gesture when presentationStyle is pageSheet or formSheet
Type |
---|
function |
The onShow prop allows passing a function that will be called once the modal has been shown.
presentationStyle iOS
The presentationStyle prop controls how the modal appears (generally on larger devices such as iPad or plus-sized iPhones). See https://developer.apple.com/reference/uikit/uimodalpresentationstyle for details.
- fullScreen covers the screen completely
- pageSheet covers portrait-width view centered (only on larger devices)
- formSheet covers narrow-width view centered (only on larger devices)
- overFullScreen covers the screen completely, but allows transparency
Type | Default |
---|---|
enum( , , , ) | if if |
statusBarTranslucent Android
The statusBarTranslucent prop determines whether your modal should go under the system statusbar.
supportedOrientations iOS
The supportedOrientations prop allows the modal to be rotated to any of the specified orientations. On iOS, the modal is still restricted by what's specified in your app's Info.plist's UISupportedInterfaceOrientations field.
When using presentationStyle of pageSheet or formSheet , this property will be ignored by iOS.
Type | Default |
---|---|
array of enums( , , , , ) |
transparent
The transparent prop determines whether your modal will fill the entire view. Setting this to true will render the modal over a transparent background.
The visible prop determines whether your modal is visible.
- animationType
- hardwareAccelerated Android
- onDismiss iOS
- onOrientationChange iOS
- onRequestClose
- presentationStyle iOS
- statusBarTranslucent Android
- supportedOrientations iOS
- transparent
Create a modal
Edit this page
In this tutorial, learn how to create a modal from React Native to select an image.
In this chapter, we'll create a modal that shows an emoji picker list.
Declare a state variable to show buttons
Before implementing the modal, we are going to add three new buttons. These buttons will only be visible when the user picks an image from the media library or decides to use the placeholder image. One of these buttons will trigger the emoji picker modal.
Add buttons
Let's break down the layout of the option buttons we will implement in this chapter. The design looks like this:
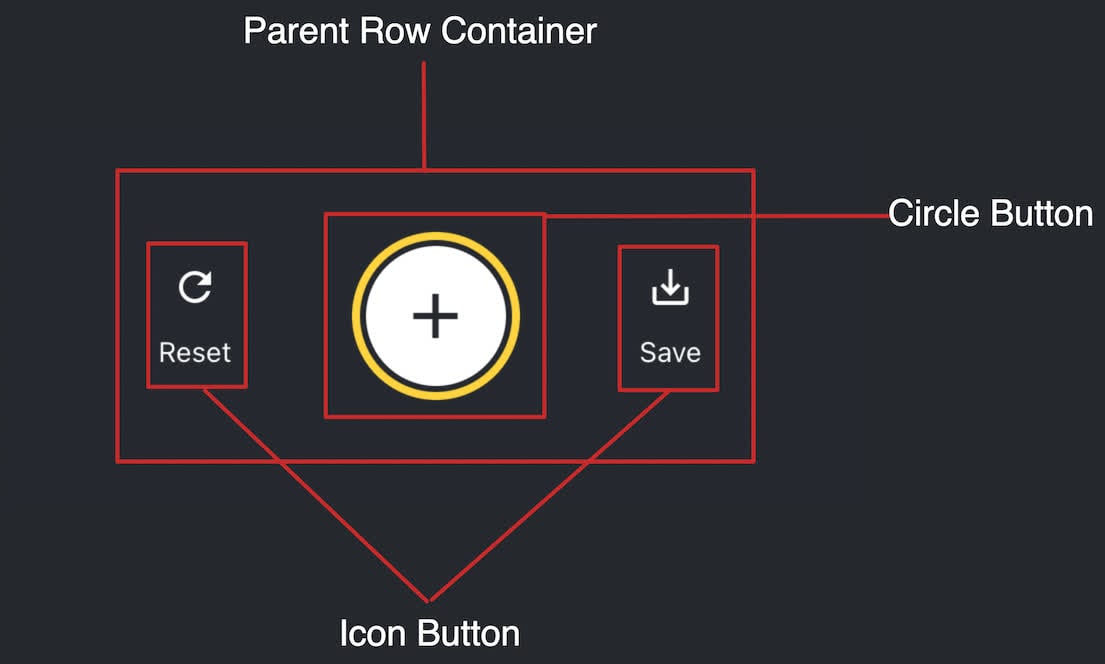
- label : the text label displayed on the button.
- onPress : the function called when the button is pressed.
Open in Snack
Let's take a look at our app on Android, iOS and the web:
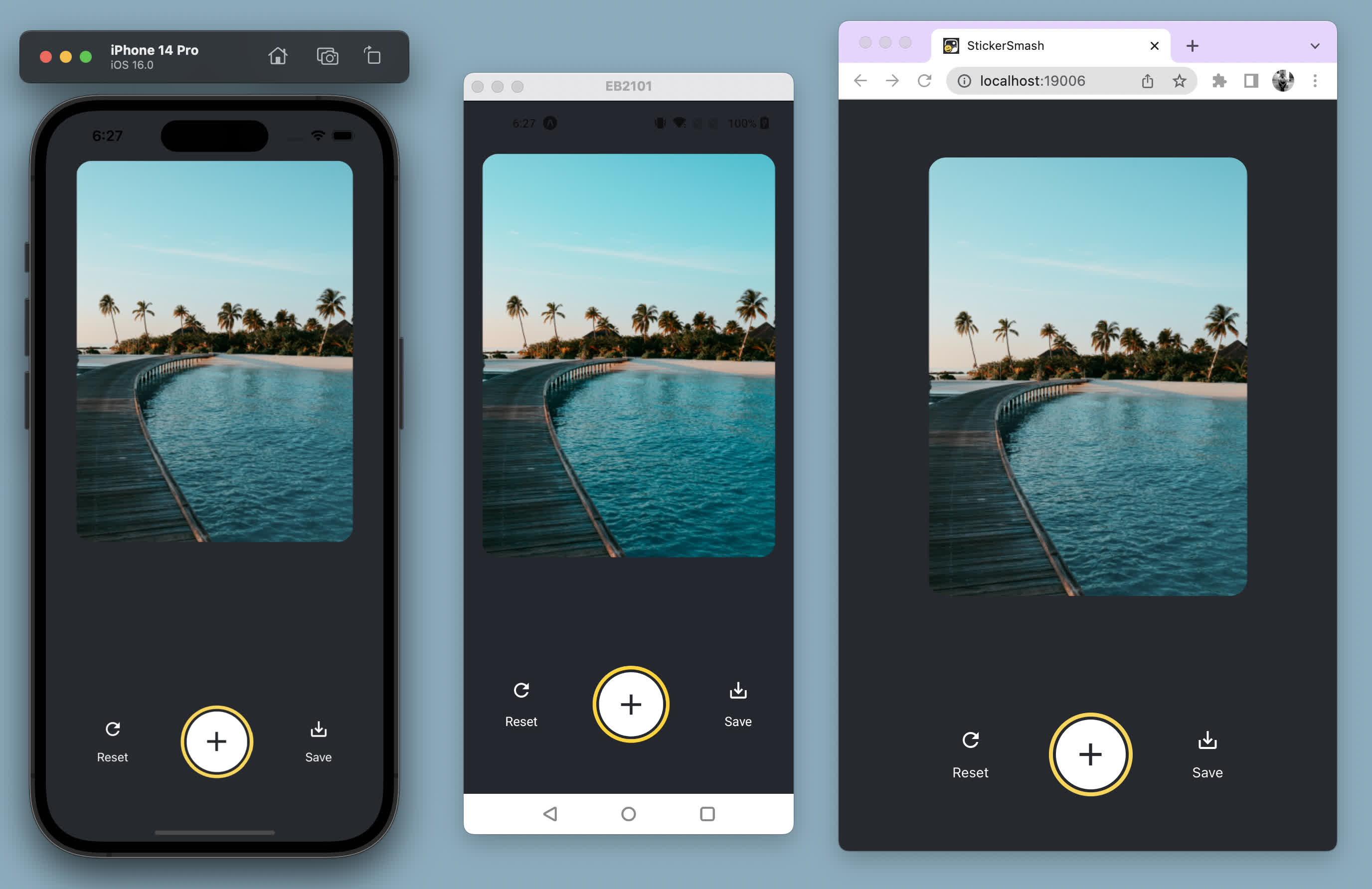
Create an emoji picker modal
- onClose : a function that closes the modal.
- children : used later to display a list of emoji.
Let's learn what the above code does.
- Its visible prop takes the value of isVisible and controls if the modal is open or closed.
- Its transparent prop is a boolean value that determines whether the modal fills the entire view.
- Its animationType prop determines how it enters and leaves the screen. In this case, it is sliding from the bottom of the screen.
- Lastly, the <EmojiPicker> onClose prop is called when the user presses the close <Pressable> .
- Then, create an isModalVisible state variable with the useState hook. It has a default value of false to ensure that the modal is hidden until the user presses the button to open it.
- Replace the comment in the onAddSticker() function to update the isModalVisible variable to true when the user presses the button. This will open the emoji picker.
- Create a onModalClose() function to update the isModalVisible state variable.
- Place the <EmojiPicker> component at the bottom of the <App> component, above the <StatusBar> component.
Here is the result after this step:
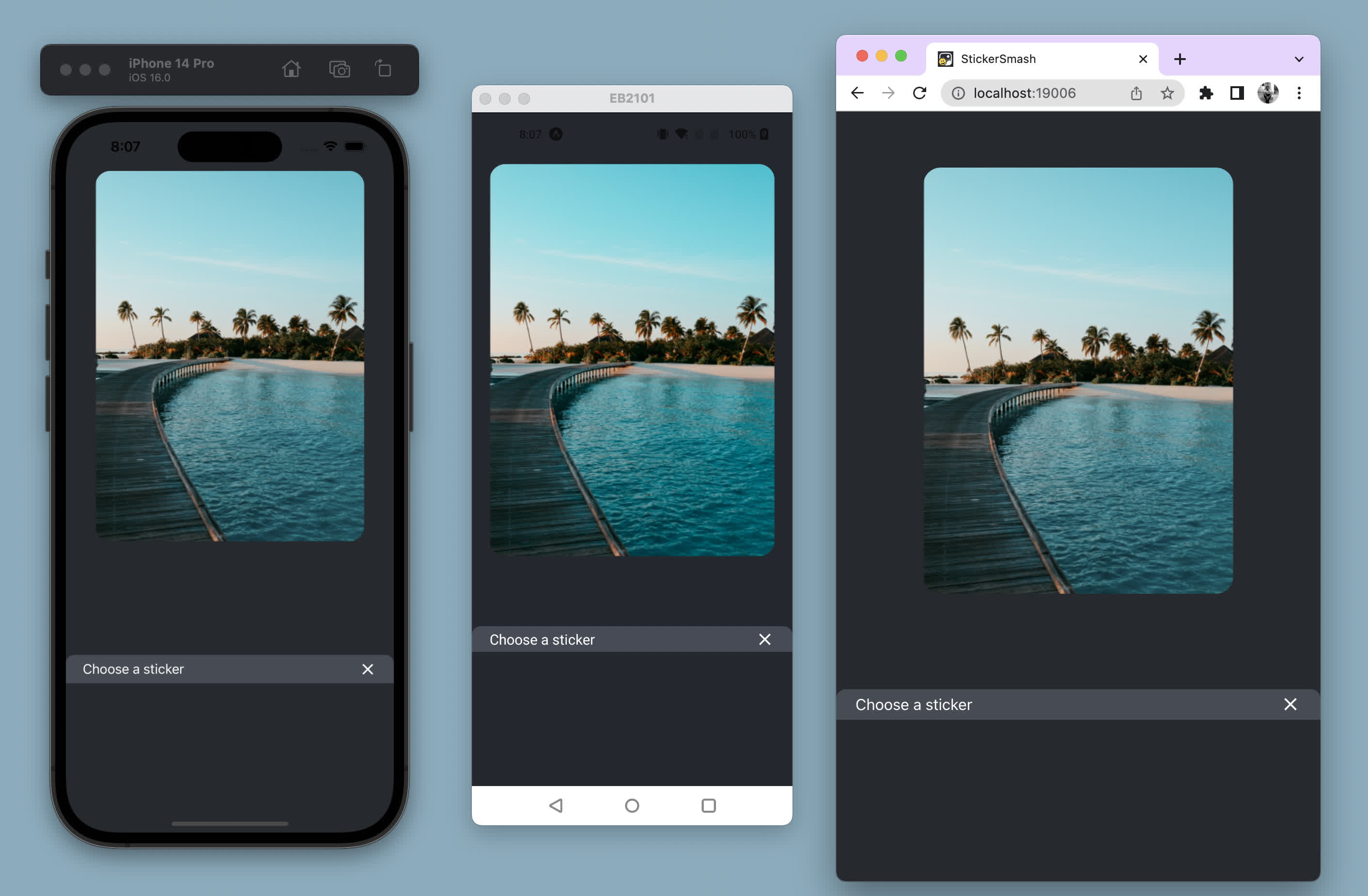
Display a list of emoji
Display the selected emoji.
Now we'll put the emoji sticker on the image.
This component receives two props:
- stickerSource : the source of the selected emoji image.
We successfully created the emoji picker modal and implemented the logic to select an emoji and display it over the image.
In the next chapter, let's add user interactions with gestures to drag the emoji and scale the size by tapping it.
Advisory boards aren’t only for executives. Join the LogRocket Content Advisory Board today →
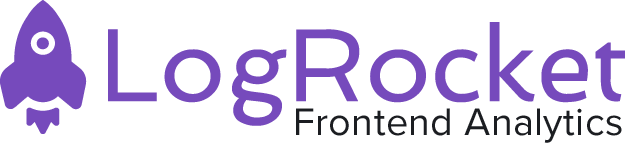
- Product Management
- Solve User-Reported Issues
- Find Issues Faster
- Optimize Conversion and Adoption
- Start Monitoring for Free
Creating a pop-up modal in React Native
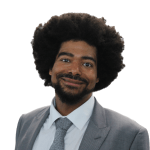
Modals are a great tool for improving user interfaces. Essentially, a modal is a screen that appears above another screen, directing a user’s attention towards critical information or guiding them to the next step in a process. An active modal disables the original screen, which is still visible, prompting the user to interact with the new one.
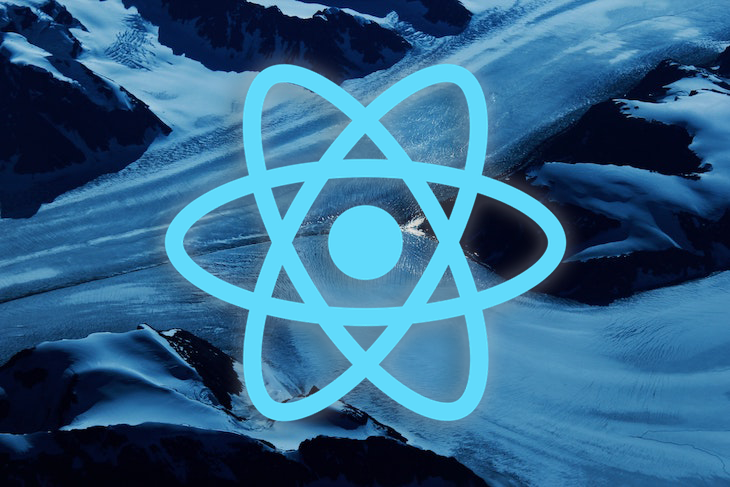
In this tutorial, we’ll look at some practical use cases for modals and improve our understanding of them by creating the following in React Native:
- A modal that opens when a user clicks a button
- A reusable modal with child components
- A pop-up modal that opens when a user navigates to a screen
You can find the source code for this project on my GitHub .
Initialize the project
First, to initialize the project, type the following code into your terminal:
If you’re adding the modal to an existing project instead of starting from scratch, skip this step, add react-native-modal to your project, and select the title option.
Select the tabs (TypeScript) option, which should look like the code block below:
A browser window will pop up with instructions for opening the Expo project. Now, you can open your favorite IDE. If you’re using VS Code, enter code . in your terminal to open it.
Install react-native-modal
react-native-modal is what you would get if you took React Native’s modal component to the beauty salon and asked for a full makeover. It builds on React Native’s modal component, providing beautiful animations and customizable styling options.
Run the following code to install react-native-modal :
Creating a basic modal
A modal is triggered by a preceding action, like navigating to/from a screen or clicking a button.
Open screens/TabOneScreen . Change the code to look like the code block below:
Let’s take a closer look.
The React useState Hook returns isModalVisible , a stateful value, and setIsModalVisible , a function to update it.
The initial state is false , so we can’t see the modal until we change the state. In our case, we’ll use a button.
handleModal
Pressing the button will call handleModal and return the opposite state. True becomes false, and false becomes true.
<Modal />
Modal has a property called isVisible that accepts a Boolean value. Because we pass isModalVisible to isVisible , the modal knows when it should appear.
Your finished modal will look like the image below:
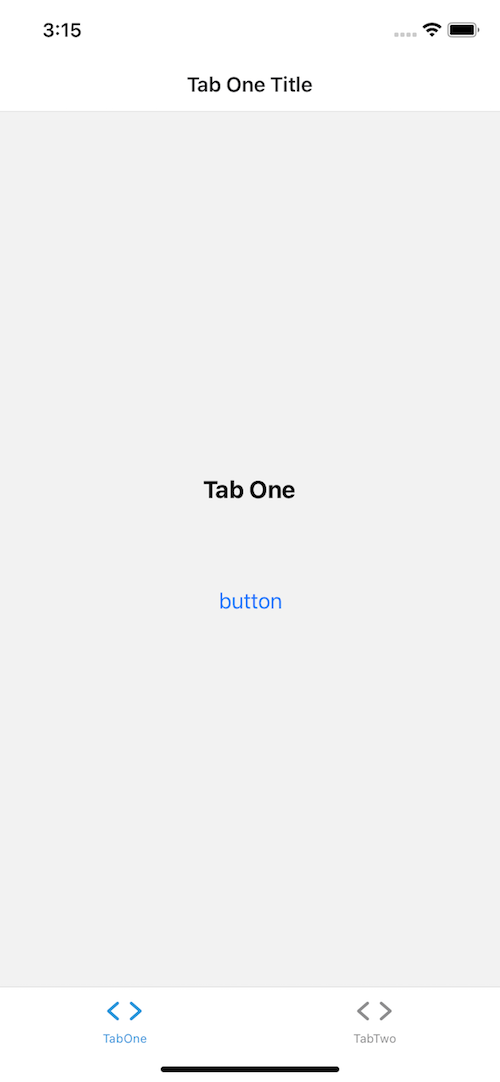
Creating a reusable modal
The previous example created a modal and buttons for opening and closing it. Now that we know these steps, let’s build a custom modal and a button for booting it.
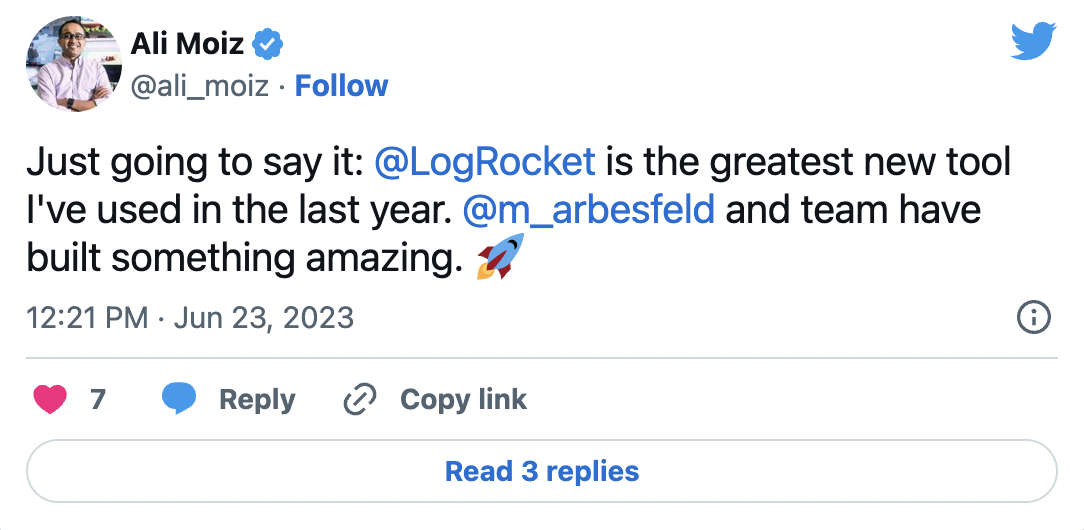
Over 200k developers use LogRocket to create better digital experiences

Let’s start with a simple button to open and close our modal. In the components folder, create a new file called Button.tsx and add the following code:
Your output should look like the image below:
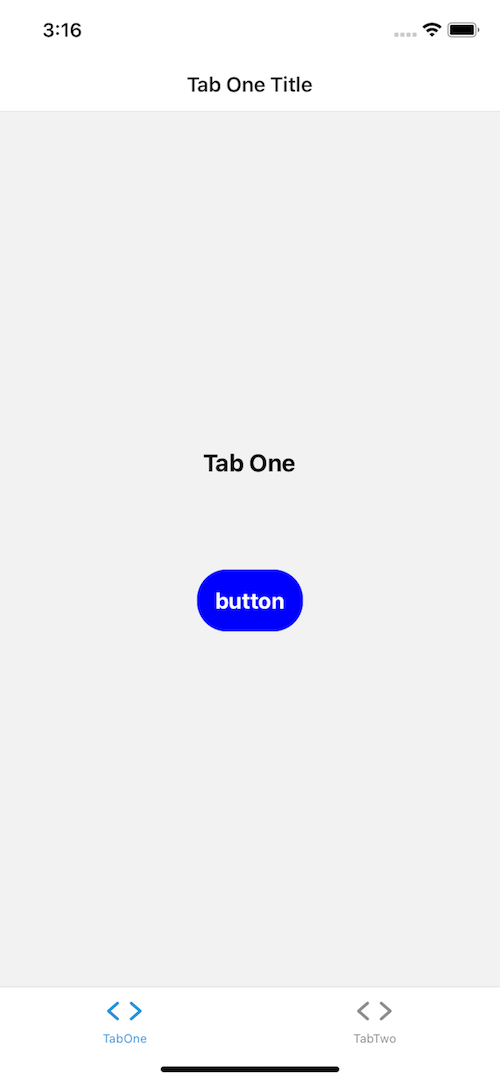
Now that we’ve built a button, we’ll create our modal. In your components folder, create a file called Modal.tsx and add the following code:
We’ve exported the original modal component from the first example as Modal . Did you notice the animation and backdropTransitions props? These props are what give the native modal component that makeover I was talking about.
For a full list of available props, check out the documentation .
Child components and dot notation
Using dot notation , we’ll export Modal as a module with child components. If you’d like to add a header, you can access the header component with Modal.Header .
ModalHeader accepts a string in the title prop. Modal.Container , Modal.Body , and Modal.Footer accept children. A child component can be anything you want it to be, like an image, some text, buttons, or a ScrollView .
Now that we have a modal and a button, head back over to screens/TabOneScreen . In just two steps, we’ll put it all together.
Import our new button and modal by changing the import statements from the code block above to the code block below, respectively:
Do the same for the modal component in the return statement:
The result should look similar to the image below:
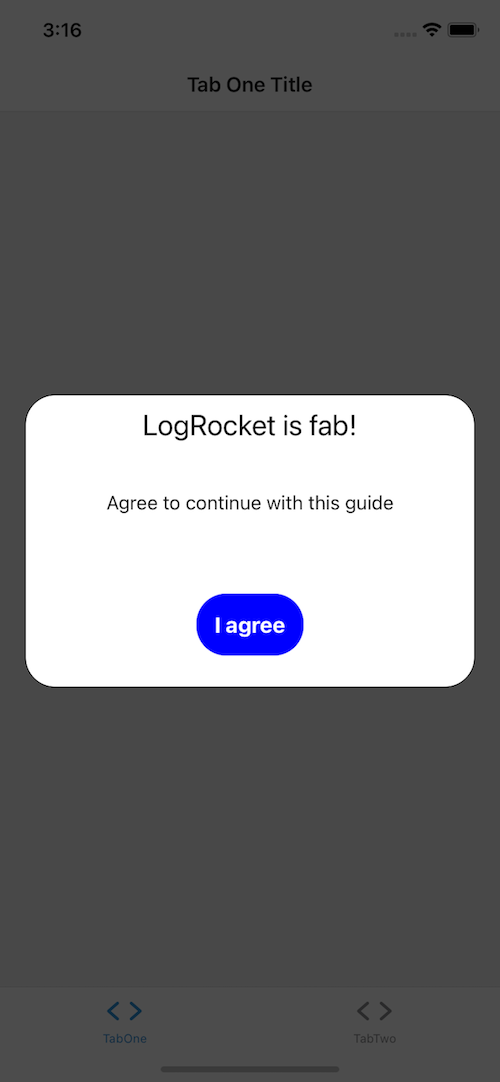
You can find all the changes on GitHub.
Creating a pop-up modal
Now, we have a modal that appears when we tell it to. Let’s add pop-ups that appear when we want to prompt the user to see or do something. To start, we’ll create a call to action scenario.
Let’s pretend that a user must subscribe to have access to a particular screen. We want to create a call to action if the user lands on the screen but isn’t subscribed.
Head over to screens/TabTwoScreen and swap out the current code for the code below:
useEffect re-renders when the dependencies in the dependency array change, indicated by [] . useEffect causes the Modal component to appear when you navigate to the screen.
Since we haven’t passed any dependencies, useEffect will only render once. setTimeout is used to simulate checking if the user is subscribed:
Your modal should look like the image below:
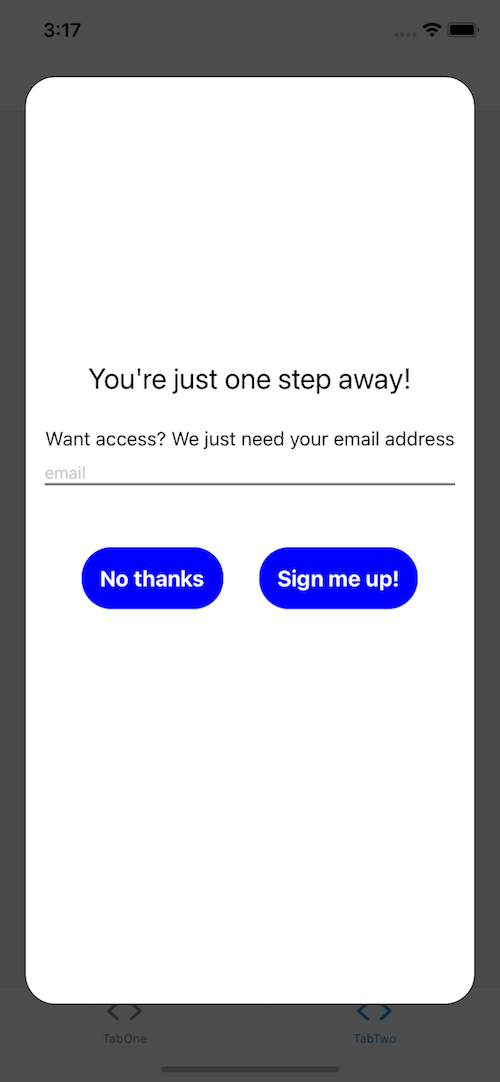
In this tutorial, we’ve learned what modals are and how they work. We then created three custom modals that can improve any React Native application.
As you can see, modals are a great tool for implementing calls to action. There are several other libraries that work similarly, like r eact-native-modal-datetime-picker , so it’s worth browsing on npm . Depending on your use case, you might save some time building a customized component!
I hope you’ve enjoyed learning about modals in this tutorial. Happy coding!
LogRocket : Instantly recreate issues in your React Native apps
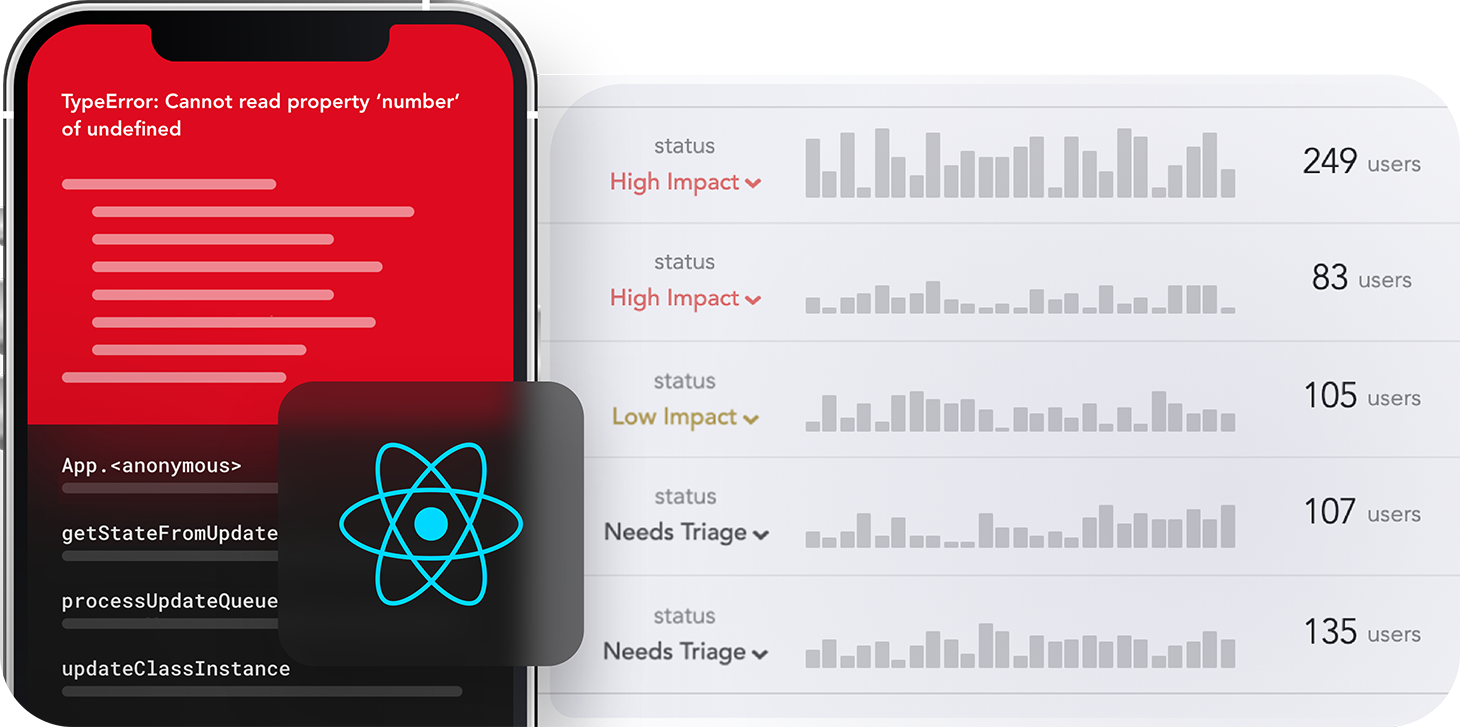
LogRocket is a React Native monitoring solution that helps you reproduce issues instantly, prioritize bugs, and understand performance in your React Native apps.
LogRocket also helps you increase conversion rates and product usage by showing you exactly how users are interacting with your app. LogRocket's product analytics features surface the reasons why users don't complete a particular flow or don't adopt a new feature.
Start proactively monitoring your React Native apps — try LogRocket for free .
Share this:
- Click to share on Twitter (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Facebook (Opens in new window)
- #react native
Hey there, want to help make our blog better?
Join LogRocket’s Content Advisory Board. You’ll help inform the type of content we create and get access to exclusive meetups, social accreditation, and swag.
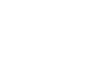
Stop guessing about your digital experience with LogRocket
Recent posts:.
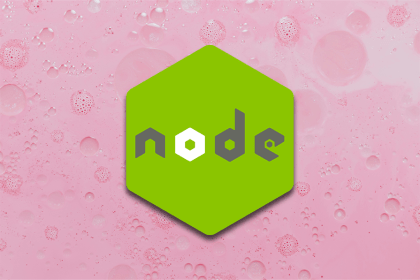
How to implement Coolify, the self-hosted alternative to Heroku
If you’ve been active on Twitter or Reddit lately, you’ve likely seen discussions surrounding Vercel’s pricing model, which has turned […]
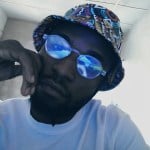
Zustand adoption guide: Overview, examples, and alternatives
Learn about Zustand’s simplistic approach to managing state and how it compares to existing tools such as Mobx and Redux.
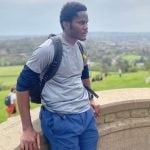
Working with custom elements in React
Dive into custom HTML elements, the challenges of using them with React, and the changes in React 19 that address these challenges.
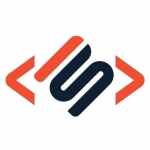
How to build a simple Svelte app
Developers can use both Svelte and React to effectively build web applications. While they serve the same overall purpose, there are distinct differences in how they work.
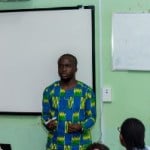
Leave a Reply Cancel reply
- Privacy Policy
React Native Modal Tutorial with Examples
A Modal is a pre-defined component that helps in creating the modal popup to React Native. Ordinarily, a Modal component is a primary way to present content above an enclosing view.
If you need more control over how to present modals over the rest of your app, then consider using a top-level Navigator.
Working with modal popups has been made easy by built-in React Native Modal component. In this tutorial, we will check out the various react-native modal examples, and show you how you can add different controls over the modal component to customize it to the next level.
Table of Contents
Prerequisite, initiate react native project, run react native app with xcode emulator, add button to open modal dialog, show react native modal, close react native modal popup, modal methods & properties, react native basic modal, react native modal example.
Following tools, frameworks, and packages required to get started with this tutorial.
- React-Native
- React-Native CLI
- Text Editor or IDE
- Xcode or Android Studio
Run command in the terminal to globally Install the latest React Native CLI version.
Let’s start installing the React Native app first.
Head over to the project directory.
Run the command to check the installed React Native version:
The Xcode IDE is at the center of the Apple development experience. Robustly blended with the Cocoa and Cocoa Touch frameworks, Xcode is an astonishingly productive environment for developing apps for Mac, iPhone, iPad, Apple Watch, and Apple TV.
Here is the process to download Xcode from Apple site.
- Visit Apple Developer
- Click on develop
- Click on downloads and sign in
- Scroll to the bottom and click see more downloads
- Use the search bar to search up Xcode
- Click the little plus icon on the row with the Xcode version you want
- Click download on the zip file
Or even you can visit the following URL to download Xcode .
Next, set the Xcode path for command line tool. Go to Xcode > Preferences > Locations Here set the Command Line Tools: Xcode xx.x.x (version number) from the dropdown.

Next, run the following command to install cocoapods .
Next, get inside the ios folder of your project.
Now, run the command while staying in the ios folder.
Then, we are all set with configuration, come out from the iOS folder and run the command in the terminal to start the app in the iOS emulator using Xcode.

To run your app in an Android device or emulator, you have to set the android development environment in your machine and run the following command.
We are about to add a cool stylish button in our react-native application, and this button will be responsible for opening the Modal when the user clicks on this button.
However, we are not going to use the Button component. Instead, we will use the TouchableOpacity component to create and add a custom styling in a button.
Open the App.js file and replace with the following code.
In this step we will learn how to implement Simple Modal in React Native with some content (Image and Text). Open the App.js file and place the following code in it.
We imported the Modal component along with Image and Text components.
We defined the initial state of the Modal, the displayModal() is a function that set the Modal state to true if its false.
In the following react native modal example, we implemented the animated Modal by just setting the animation property to ‘slide’ . Apart from that, you can also choose ‘fade’ or ‘none’ prop to animate the modal.
To set the React Native Modal’s transparent background, we set the transparent property to false.
We are showing The image and text in the Modal and added the style for the components using { styles.className } property.
Next, we will understand how to close the React Native Modal, add the following code inside the Modal component.
Here is the style for close button.
And, here is the final App.js code.
There are various other properties which can allow us to customize Modal to some extent. We will have a look at the useful methods and properties to work with Modal. However, you can visit the following URL to check out the full details about Modal props.
Visible – This property makes sure whether your modal should be visible or not. It takes two parameters, either true or false.
supportedOrientations: The supportedOrientations prop lets the modal to be wheeled to any of the specified orientations.
On iOS, the modal is still restricted by what’s specified in your app’s Info.plist’s UISupportedInterfaceOrientations field. When using presentationStyle of pageSheet or formSheet, this property will be ignored by iOS.
animationType: The animationType prop manages how modal should be animated. However, the default prop is set to be none.
- fade into view
- slides in from the bottom
- shows without animation
hardwareAccelerated: This property examines whether to enforce hardware acceleration for the underlying window.
presentationStyle: This value examines how the modal should appear (usually on larger devices like iPad or larger iPhones).
- fullScreen comprises the screen perfectly
- formSheet overlays narrow-width composition centered
- pageSheet covers portrait-width view centered (exclusively on larger devices)
- overFullScreen includes the screen effectively but concedes clarity
Have you thought of a modal that we can observe in a lot of simplistic applications? In such cases, the perceptibility of the modal is handled privately by some component’s local state.
Possibly something similar to this:
Thats it for now, finally we have completed React Native Modal tutorial in this tutorial we have learned how to display modal in iOS and Android app with some data. We have learned how to show and hide modal popup in React native app and also focused on basic styling of components using style.classname property in React Native.
You can get the full code of this tutorial on this GitHub repo .
Happy Coding!
- CSS Animations
- CodeIgniter
- Angular Material
- React Native
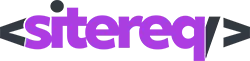
Sign in to your account or register new one to start publishing your guest posts, directory submissions and more.

React Native Modal Box - Everything you Need to Know
You can go to our GitHub Repository through this link .
Although there are several other plugins, that we will mention throughout this post and the differences between them, that accomplish the same objective but, we decided to build our React Native Modal demo app using just the built-in Modal component shipped with the ‘react-native’ library.
Creating a React Native Modal Reusable Component
Second, create a new component class called CustomModal in a new directory called “components” exactly as structured in the demo repository that you cloned. The CustomModal component will act as the reusable component that the other parent screens will call.
First, the imports of this component are nothing more than a React Native View , Modal , StyleSheet , Dimensions and TouchableWithoutFeedback . It’s clear that CustomModal uses only the basic React Native components as shown below.
React Native Modal Style Example
A full overview of the StyleSheetFactory class is like the following code snippet:
React Native Modal Full Screen
Those navigation parameters will act as the CustomModal configurations.
Setting the transparent navigation parameter to false , will let the screen to show the modal box as follows:
The CustomModal hosted inside the BasicScreen should look like the following code snippet:
React Native Modal Not in Full Screen Mode
This behavior is the opposite configuration of the full screen mode. Simply if the transparent navigation parameter is set to true . The background screen will start to show and the modal will appear as a popover above it as shown in the below screenshot:
Bottom-Half React Native Modal
Showing a React Native Modal in the bottom-half section of the screen with our setup is beyond simple. You could have already noticed that there is a boolean property inside CustomModal called bottomHalf that could be set to true or false to show the modal in the bottom-half mode.
Closing the React Native Modal on Clicking Outside
Then, CustomModal delegates the click event of the touchable to the calling parent components that will then, decide to do some action if the touchable is clicked. This action, in our case, will be setting the visible local state to false in BasicScreen .
Other React Native Modal Plugins
We found both plugins interesting, and we added some useful examples how to include them and call them in your project. As we know, some plugins have always advantages over the others and what we found through integrating both plugins is very interesting.
Here’s a chart that emphasizes the popularity difference:
React-Native-Modal Integration Results
The next step is to import the plugin using the following import statement:
The next step is calling the modal plugin you’ve just imported in order to display a modal on the screen.
React-Native-Modals Integration Results
The following imports might be needed according to your requirements.
To call the modal component, you can place the following code snippet in your project as a simple example:
As a Conclusion
react-native-modal
- 2 Dependencies
- 612 Dependents
- 104 Versions
Announcements
- 📣 We're looking for maintainers and contributors! See #598
- 💡 We're brainstorming if/how we can make a JavaScript-only version of react-native-modal . See #597
- 🙏 If you have a question, please start a new discussion instead of opening a new issue.
If you're new to the React Native world, please notice that React Native itself offers a component that works out-of-the-box .
An enhanced, animated, customizable React Native modal.
The goal of react-native-modal is expanding the original React Native <Modal> component by adding animations, style customization options, and new features, while still providing a simple API.
- Smooth enter/exit animations
- Plain simple and flexible APIs
- Customizable backdrop opacity, color and timing
- Listeners for the modal animations ending
- Resize itself correctly on device rotation
This library is available on npm, install it with: npm i react-native-modal or yarn add react-native-modal .
Since react-native-modal is an extension of the original React Native modal , it works in a similar fashion.
- Import react-native-modal :
- Create a <Modal> component and nest its content inside of it:
- Then, show the modal by setting the isVisible prop to true :
The isVisible prop is the only prop you'll really need to make the modal work: you should control this prop value by saving it in your wrapper component state and setting it to true or false when needed.
A complete example
The following example consists in a component ( ModalTester ) with a button and a modal. The modal is controlled by the isModalVisible state variable and it is initially hidden, since its value is false . Pressing the button sets isModalVisible to true, making the modal visible. Inside the modal there is another button that, when pressed, sets isModalVisible to false, hiding the modal.
For a more complex example take a look at the /example directory.
Available props
Name | Type | Default | Description |
---|---|---|---|
or | Modal show animation | ||
Timing for the modal show animation (in ms) | |||
or | Modal hide animation | ||
Timing for the modal hide animation (in ms) | |||
Move the modal up if the keyboard is open | |||
Will use RN component to cover the entire screen wherever the modal is mounted in the component hierarchy | |||
Render the backdrop | |||
The backdrop background color | |||
The backdrop opacity when the modal is visible | |||
The backdrop show timing (in ms) | |||
The backdrop hide timing (in ms) | |||
The custom backdrop element | |||
The modal content | |||
Device height (useful on devices that can hide the navigation bar) | |||
Device width (useful on devices that can hide the navigation bar) | |||
Show the modal? | |||
Called when the Android back button is pressed | |||
Called when the backdrop is pressed | |||
Called before the modal hide animation begins | |||
Called when the modal is completely hidden | |||
Called before the modal show animation begins | |||
Called when the modal is completely visible | |||
Called when the swipe action started | |||
Called on each swipe event | |||
Called when the has been reached | |||
Called when the has not been reached | |||
The threshold for when the panResponder should pick up swipe events | |||
When > 0, disables swipe-to-close, in order to implement scrollable content | |||
Used to implement overscroll feel when content is scrollable. See directory | |||
Used to implement scrollable modal. See directory for reference on how to use it | |||
Set to true if your scrollView is horizontal (for a correct scroll handling) | |||
Swiping threshold that when reached calls | |||
or | Defines the direction where the modal can be swiped. Can be 'up', 'down', 'left, or 'right', or a combination of them like | ||
Defines if animations should use native driver | |||
Defines if animations for backdrop should use native driver (to avoid flashing on android) | |||
Enhances the performance by hiding the modal content until the animations complete | |||
or | Allows swipe events to propagate to children components (eg a ScrollView inside a modal) | ||
Style applied to the modal |
Frequently Asked Questions
The component is not working as expected.
Under the hood react-native-modal uses react-native original Modal component . Before reporting a bug, try swapping react-native-modal with react-native original Modal component and, if the issue persists, check if it has already been reported as a react-native issue .
The backdrop is not completely filled/covered on some Android devices (Galaxy, for one)
React-Native has a few issues detecting the correct device width/height of some devices. If you're experiencing this issue, you'll need to install react-native-extra-dimensions-android . Then, provide the real window height (obtained from react-native-extra-dimensions-android ) to the modal:
How can I hide the modal by pressing outside of its content?
The prop onBackdropPress allows you to handle this situation:
How can I hide the modal by swiping it?
The prop onSwipeComplete allows you to handle this situation (remember to set swipeDirection too!):
Note that when using useNativeDriver={true} the modal won't drag correctly. This is a known issue .
The modal flashes in a weird way when animating
Unfortunately this is a known issue that happens when useNativeDriver=true and must still be solved. In the meanwhile as a workaround you can set the hideModalContentWhileAnimating prop to true : this seems to solve the issue. Also, do not assign a backgroundColor property directly to the Modal. Prefer to set it on the child container.
The modal background doesn't animate properly
Are you sure you named the isVisible prop correctly? Make sure it is spelled correctly: isVisible , not visible .
The modal doesn't change orientation
Add a supportedOrientations={['portrait', 'landscape']} prop to the component, as described in the React Native documentation .
Also, if you're providing the deviceHeight and deviceWidth props you'll have to manually update them when the layout changes.
I can't show multiple modals one after another
Unfortunately right now react-native doesn't allow multiple modals to be displayed at the same time. This means that, in react-native-modal , if you want to immediately show a new modal after closing one you must first make sure that the modal that your closing has completed its hiding animation by using the onModalHide prop.
I can't show multiple modals at the same time
See the question above. Showing multiple modals (or even alerts/dialogs) at the same time is not doable because of a react-native bug. That said, I would strongly advice against using multiple modals at the same time because, most often than not, this leads to a bad UX, especially on mobile (just my opinion).
The StatusBar style changes when the modal shows up
This issue has been discussed here . The TLDR is: it's a know React-Native issue with the Modal component 😞
The modal is not covering the entire screen
The modal style applied by default has a small margin. If you want the modal to cover the entire screen you can easily override it this way:
I can't scroll my ScrollView inside of the modal
Enable propagateSwipe to allow your child components to receive swipe events:
Please notice that this is still a WIP fix and might not fix your issue yet, see issue #236 .
The modal enter/exit animation flickers
Make sure your animationIn and animationOut are set correctly. We noticed that, for example, using fadeIn as an exit animation makes the modal flicker (it should be fadeOut !). Also, some users have noticed that setting backdropTransitionOutTiming={0} can fix the flicker without affecting the animation.
The custom backdrop doesn't fill the entire screen
You need to specify the size of your custom backdrop component. You can also make it expand to fill the entire screen by adding a flex: 1 to its style:
The custom backdrop doesn't dismiss the modal on press
You can provide an event handler to the custom backdrop element to dismiss the modal. The prop onBackdropPress is not supported for a custom backdrop.
Available animations
Take a look at react-native-animatable to see the dozens of animations available out-of-the-box. You can also pass in custom animation definitions and have them automatically register with react-native-animatable. For more information on creating custom animations, see the react-native-animatable animation definition schema .
Alternatives
- React Native's built-in <Modal> component
- React Native Paper <Modal> component
- React Native Modalfy
Acknowledgements
Thanks @oblador for react-native-animatable, @brentvatne for the npm namespace and to anyone who contributed to this library!
Pull requests, feedbacks and suggestions are welcome!
- react-native
Package Sidebar
npm i react-native-modal
Git github.com/react-native-community/react-native-modal
github.com/react-native-community/react-native-modal
Downloads Weekly Downloads
Unpacked size, total files, last publish.
2 years ago
Collaborators
Native Stack Navigator
Native Stack Navigator provides a way for your app to transition between screens where each new screen is placed on top of a stack.
This navigator uses the native APIs UINavigationController on iOS and Fragment on Android so that navigation built with createNativeStackNavigator will behave exactly the same and have the same performance characteristics as apps built natively on top of those APIs. It also offers basic Web support using react-native-web .
One thing to keep in mind is that while @react-navigation/native-stack offers native performance and exposes native features such as large title on iOS etc., it may not be as customizable as @react-navigation/stack depending on your needs. So if you need more customization than what's possible in this navigator, consider using @react-navigation/stack instead - which is a more customizable JavaScript based implementation.
Installation
To use this navigator, ensure that you have @react-navigation/native and its dependencies (follow this guide) , then install @react-navigation/native-stack :
API Definition
💡 If you encounter any bugs while using createNativeStackNavigator , please open issues on react-native-screens rather than the react-navigation repository!
To use this navigator, import it from @react-navigation/native-stack :
The Stack.Navigator component accepts following props:
Optional unique ID for the navigator. This can be used with navigation.getParent to refer to this navigator in a child navigator.
initialRouteName
The name of the route to render on first load of the navigator.
screenOptions
Default options to use for the screens in the navigator.
The following options can be used to configure the screens in the navigator:
String that can be used as a fallback for headerTitle .
headerBackButtonMenuEnabled
Boolean indicating whether to show the menu on longPress of iOS >= 14 back button. Defaults to true .
Requires react-native-screens version >=3.3.0.
Only supported on iOS.
headerBackVisible
Whether the back button is visible in the header. You can use it to show a back button alongside headerLeft if you have specified it.
This will have no effect on the first screen in the stack.
headerBackTitle
Title string used by the back button on iOS. Defaults to the previous scene's title, or "Back" if there's not enough space. Use headerBackTitleVisible: false to hide it.
headerBackTitleVisible
Whether the back button title should be visible or not.
headerBackTitleStyle
Style object for header back title. Supported properties:
headerBackImageSource
Image to display in the header as the icon in the back button. Defaults to back icon image for the platform
- A chevron on iOS
- An arrow on Android

headerLargeStyle
Style of the header when a large title is shown. The large title is shown if headerLargeTitle is true and the edge of any scrollable content reaches the matching edge of the header.
Supported properties:
- backgroundColor
headerLargeTitle
Whether to enable header with large title which collapses to regular header on scroll.
For large title to collapse on scroll, the content of the screen should be wrapped in a scrollable view such as ScrollView or FlatList . If the scrollable area doesn't fill the screen, the large title won't collapse on scroll. You also need to specify contentInsetAdjustmentBehavior="automatic" in your ScrollView , FlatList etc.
headerLargeTitleShadowVisible
Whether drop shadow of header is visible when a large title is shown.
headerLargeTitleStyle
Style object for large title in header. Supported properties:
headerShown
Whether to show the header. The header is shown by default. Setting this to false hides the header.
headerStyle
Style object for header. Supported properties:
headerShadowVisible
Whether to hide the elevation shadow (Android) or the bottom border (iOS) on the header.
headerTransparent
Boolean indicating whether the navigation bar is translucent.
Defaults to false . Setting this to true makes the header absolutely positioned - so that the header floats over the screen so that it overlaps the content underneath, and changes the background color to transparent unless specified in headerStyle .
This is useful if you want to render a semi-transparent header or a blurred background.
Note that if you don't want your content to appear under the header, you need to manually add a top margin to your content. React Navigation won't do it automatically.
To get the height of the header, you can use HeaderHeightContext with React's Context API or useHeaderHeight .
headerBlurEffect
Blur effect for the translucent header. The headerTransparent option needs to be set to true for this to work.
Supported values:
- systemUltraThinMaterial
- systemThinMaterial
- systemMaterial
- systemThickMaterial
- systemChromeMaterial
- systemUltraThinMaterialLight
- systemThinMaterialLight
- systemMaterialLight
- systemThickMaterialLight
- systemChromeMaterialLight
- systemUltraThinMaterialDark
- systemThinMaterialDark
- systemMaterialDark
- systemThickMaterialDark
- systemChromeMaterialDark
headerBackground
Function which returns a React Element to render as the background of the header. This is useful for using backgrounds such as an image or a gradient.
headerTintColor
Tint color for the header. Changes the color of back button and title.
headerLeft
Function which returns a React Element to display on the left side of the header. This replaces the back button. See headerBackVisible to show the back button along side left element.
headerRight
Function which returns a React Element to display on the right side of the header.
headerTitle
String or a function that returns a React Element to be used by the header. Defaults to title or name of the screen.
When a function is passed, it receives tintColor and children in the options object as an argument. The title string is passed in children .
Note that if you render a custom element by passing a function, animations for the title won't work.
headerTitleAlign
How to align the header title. Possible values:
Defaults to left on platforms other than iOS.
Not supported on iOS. It's always center on iOS and cannot be changed.
headerTitleStyle
Style object for header title. Supported properties:
headerSearchBarOptions
Options to render a native search bar on iOS. Search bars are rarely static so normally it is controlled by passing an object to headerSearchBarOptions navigation option in the component's body. You also need to specify contentInsetAdjustmentBehavior="automatic" in your ScrollView , FlatList etc. If you don't have a ScrollView , specify headerTransparent: false .
Only supported on iOS and Android.
Supported properties are described below.
autoCapitalize
Controls whether the text is automatically auto-capitalized as it is entered by the user. Possible values:
Defaults to sentences .
autoFocus
Whether to automatically focus search bar when it's shown. Defaults to false .
Only supported on Android.
barTintColor
The search field background color. By default bar tint color is translucent.
tintColor
The color for the cursor caret and cancel button text.
cancelButtonText
The text to be used instead of default Cancel button text.
disableBackButtonOverride
Whether the back button should close search bar's text input or not. Defaults to false .
hideNavigationBar
Boolean indicating whether to hide the navigation bar during searching. Defaults to true .
hideWhenScrolling
Boolean indicating whether to hide the search bar when scrolling. Defaults to true .
inputType
The type of the input. Defaults to "text" .
- "text"
- "phone"
- "number"
- "email"
obscureBackground
Boolean indicating whether to obscure the underlying content with semi-transparent overlay. Defaults to true .
placeholder
Text displayed when search field is empty.
textColor
The color of the text in the search field.
hintTextColor
The color of the hint text in the search field.
headerIconColor
The color of the search and close icons shown in the header
shouldShowHintSearchIcon
Whether to show the search hint icon when search bar is focused. Defaults to true .
A callback that gets called when search bar has lost focus.
onCancelButtonPress
A callback that gets called when the cancel button is pressed.
onChangeText
A callback that gets called when the text changes. It receives the current text value of the search bar.
Custom header to use instead of the default header.
This accepts a function that returns a React Element to display as a header. The function receives an object containing the following properties as the argument:
- navigation - The navigation object for the current screen.
- route - The route object for the current screen.
- options - The options for the current screen
- back - Options for the back button, contains an object with a title property to use for back button label.
To set a custom header for all the screens in the navigator, you can specify this option in the screenOptions prop of the navigator.
Note that if you specify a custom header, the native functionality such as large title, search bar etc. won't work.
statusBarAnimation
Sets the status bar animation (similar to the StatusBar component). Defaults to fade on iOS and none on Android.
- "fade"
- "none"
- "slide"
On Android, setting either fade or slide will set the transition of status bar color. On iOS, this option applies to appereance animation of the status bar.
Requires setting View controller-based status bar appearance -> YES (or removing the config) in your Info.plist file.
Only supported on Android and iOS.
statusBarHidden
Whether the status bar should be hidden on this screen.
statusBarStyle
Sets the status bar color (similar to the StatusBar component). Defaults to auto .
- "auto"
- "inverted" (iOS only)
- "dark"
- "light"
statusBarColor
Sets the status bar color (similar to the StatusBar component). Defaults to initial status bar color.
statusBarTranslucent
Sets the translucency of the status bar (similar to the StatusBar component). Defaults to false .
contentStyle
Style object for the scene content.
customAnimationOnGesture
Whether the gesture to dismiss should use animation provided to animation prop. Defaults to false .
Doesn't affect the behavior of screens presented modally.
fullScreenGestureEnabled
Whether the gesture to dismiss should work on the whole screen. Using gesture to dismiss with this option results in the same transition animation as simple_push . This behavior can be changed by setting customAnimationOnGesture prop. Achieving the default iOS animation isn't possible due to platform limitations. Defaults to false .
gestureEnabled
Whether you can use gestures to dismiss this screen. Defaults to true . Only supported on iOS.
animationTypeForReplace
The type of animation to use when this screen replaces another screen. Defaults to pop .
- push : the new screen will perform push animation.
- pop : the new screen will perform pop animation.
animation
How the screen should animate when pushed or popped.
- default : use the platform default animation
- fade : fade screen in or out
- fade_from_bottom : fade the new screen from bottom
- flip : flip the screen, requires presentation: "modal" (iOS only)
- simple_push : default animation, but without shadow and native header transition (iOS only, uses default animation on Android)
- slide_from_bottom : slide in the new screen from bottom
- slide_from_right : slide in the new screen from right (Android only, uses default animation on iOS)
- slide_from_left : slide in the new screen from left (Android only, uses default animation on iOS)
- none : don't animate the screen
presentation
How should the screen be presented.
- card : the new screen will be pushed onto a stack, which means the default animation will be slide from the side on iOS, the animation on Android will vary depending on the OS version and theme.
- modal : the new screen will be presented modally. this also allows for a nested stack to be rendered inside the screen.
- transparentModal : the new screen will be presented modally, but in addition, the previous screen will stay so that the content below can still be seen if the screen has translucent background.
- containedModal : will use "UIModalPresentationCurrentContext" modal style on iOS and will fallback to "modal" on Android.
- containedTransparentModal : will use "UIModalPresentationOverCurrentContext" modal style on iOS and will fallback to "transparentModal" on Android.
- fullScreenModal : will use "UIModalPresentationFullScreen" modal style on iOS and will fallback to "modal" on Android. A screen using this presentation style can't be dismissed by gesture.
- formSheet : will use "UIModalPresentationFormSheet" modal style on iOS and will fallback to "modal" on Android.
orientation
The display orientation to use for the screen.
- default - resolves to "all" without "portrait_down" on iOS. On Android, this lets the system decide the best orientation.
- all : all orientations are permitted.
- portrait : portrait orientations are permitted.
- portrait_up : right-side portrait orientation is permitted.
- portrait_down : upside-down portrait orientation is permitted.
- landscape : landscape orientations are permitted.
- landscape_left : landscape-left orientation is permitted.
- landscape_right : landscape-right orientation is permitted.
autoHideHomeIndicator
Boolean indicating whether the home indicator should prefer to stay hidden. Defaults to false .
gestureDirection
Sets the direction in which you should swipe to dismiss the screen.
- vertical – dismiss screen vertically
- horizontal – dismiss screen horizontally (default)
When using vertical option, options fullScreenGestureEnabled: true , customAnimationOnGesture: true and animation: 'slide_from_bottom' are set by default.
animationDuration
Changes the duration (in milliseconds) of slide_from_bottom , fade_from_bottom , fade and simple_push transitions on iOS. Defaults to 350 .
The duration of default and flip transitions isn't customizable.
navigationBarColor
Sets the navigation bar color. Defaults to initial status bar color.
navigationBarHidden
Boolean indicating whether the navigation bar should be hidden. Defaults to false .
freezeOnBlur
Boolean indicating whether to prevent inactive screens from re-rendering. Defaults to false . Defaults to true when enableFreeze() from react-native-screens package is run at the top of the application.
Requires react-native-screens version >=3.16.0.
The navigator can emit events on certain actions. Supported events are:
transitionStart
This event is fired when the transition animation starts for the current screen.
Event data:
- e.data.closing - Boolean indicating whether the screen is being opened or closed.
transitionEnd
This event is fired when the transition animation ends for the current screen.
- e.data.closing - Boolean indicating whether the screen was opened or closed.
The native stack navigator adds the following methods to the navigation prop:
Replaces the current screen with a new screen in the stack. The method accepts following arguments:
- name - string - Name of the route to push onto the stack.
- params - object - Screen params to pass to the destination route.
Pushes a new screen to top of the stack and navigate to it. The method accepts following arguments:
Pops the current screen from the stack and navigates back to the previous screen. It takes one optional argument ( count ), which allows you to specify how many screens to pop back by.
Pops all of the screens in the stack except the first one and navigates to it.
- Installation
Get the Reddit app
A community for learning and developing native mobile applications using React Native by Facebook.
Is there a way to get this type of iOS modal in react native?
By continuing, you agree to our User Agreement and acknowledge that you understand the Privacy Policy .
Enter the 6-digit code from your authenticator app
You’ve set up two-factor authentication for this account.
Enter a 6-digit backup code
Create your username and password.
Reddit is anonymous, so your username is what you’ll go by here. Choose wisely—because once you get a name, you can’t change it.
Reset your password
Enter your email address or username and we’ll send you a link to reset your password
Check your inbox
An email with a link to reset your password was sent to the email address associated with your account
Choose a Reddit account to continue
- Before you start
- Installation
- Playground app
- Launching the app
- Basic navigation
- Advanced navigation
- Screen Lifecycle
- Passing data to components
- Using functional components
- Bottom tabs
- External Component
- Orientation
- Changing fonts
- Community libraries
- React Context API
- Contributing
In human-centered design, when we speak about a modal (or modality), we often refer to a set of techniques, aimed at bringing user attention to a specific event / screen / action / etc. Those often require user input. A pop-up on a website, a file deletion confirmation dialogue on your computer, or an alert asking you to enable location service on your phone - these can all be considered modals.
A modal is a term used in native iOS world ( full description here ), while on Android, dialogs are often used to create similar or identical behavior, alongside other techniques.
Presenting modals #
Modal can be displayed by invoking the Navigation.showModal() command, as shown in the example below:
Here's how the Modal looks on both platforms.
Adding a dismiss button #
Modals should always have a dismiss button, on left-to-right devices it's typically placed as a left button in the TopBar.
After we've added our dismiss button, we need to dismiss the modal when the button is pressed.
For the LeftButton to be visible, the screen must be displayed in a Stack.
Transparent modals #
Showing transparent modals is a nice technique to increase immersiveness while keeping the user in the same context. When a modal is displayed the content behind it (either root or modal) is removed from hierarchy. While this behavior improves performance, it's undesired when showing transparent modals as we need to see the content behind it.
To configure this behaviour, we'll need to change the modalPresentationStyle option to overCurrentContext and change the layout background color to 'transparent' .
Preventing a Modal from being dismissed #
Preventing a modal from being dismissed is done differently for each platform. While preventing the user from dismissing the modal is possible, the modal could still be dismissed programmatically by calling Navigation.dismissModal()
If the modal has a dismiss button, of course you'll need to handle it your self and avoid calling Navigation.dismissModal() when the button is pressed.
On Android, modals can be dismissed with the hardware back button. You can handle the back press your self by adding a BackHandler
While iOS devices don't have a hardware back button, PageSheet modals can be dismissed by swipe gesture from the top of the screen. To disable it, set swipeToDismiss option to false.
Presentation Style #
The presentation style determines the look and feel of a screen displayed as modal.
- Presenting modals
- Adding a dismiss button
- Transparent modals
- Presentation Style
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
IOS Modal Presentation Style. #1226
arunabhverma commented Dec 12, 2022 • edited Loading
If it is possible with the current version of this library then please guide me on how to can I implement it. Because this is a native ios style to show a full-screen modal. And this library provides us with all things like the bottom sheet and modal if there is a way to implement ios modal presentation it will be the cherry on the cake. I'll prefer to use reanimated for this type of animation.
|
The text was updated successfully, but these errors were encountered: |
- 👍 1 reaction
github-actions bot commented Dec 12, 2022
: hello! 👋 This issue is being automatically closed because it does not follow the issue template. |
Sorry, something went wrong.
nrwinner commented Dec 21, 2022
Why not just use a regular Modal from React Native here? you can set the prop to "pageSheet" to get the look you're after. |
- 👍 2 reactions
github-actions bot commented Jan 21, 2023
This issue is stale because it has been open 30 days with no activity. Remove stale label or comment or this will be closed in 5 days. |
github-actions bot commented Jan 26, 2023
This issue was closed because it has been stalled for 5 days with no activity. |
arunabhverma commented Feb 1, 2023 • edited Loading
you can set the prop to "pageSheet" to get the look you're after. This is a good idea but if we go for this we are not able to use the swipe down to close in android. We have to build another component for this. |
nrwinner commented Feb 1, 2023
Hmm, in that case I'd recommend checking out the native modals in or . This repo is specifically for bottom sheets and most likely will not implement iOS modals directly. |
- ❤️ 1 reaction
No branches or pull requests
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
react-navigation native-stack, how to change the modal height?
navigation.js
otherscreen.js
this is my current screen for modal
i wannna make like this this is my target
is there any possible to change the height using the react-navigation native-stack v6? i already look at the documentation which it does not mention any styling which can change the height of modal
im expecting the height of modal can be change
- react-native
- react-navigation
- react-navigation-native-stack
2 Answers 2
Adding a to the root of my app seemed to interfere with the that was already there (which already uses PortalProvider under the hood). Only every other bottom sheet I opened would become visible.
I got mine working simply by wrapping my react-navigation modal screen's contents in a :

- i already did based on your code, however the height still same as picture given. My expectation is i want to control the height modal. <BottomSheetModalProvider> for this one, which library that you used? – One Commented Jan 30 at 12:52
- I just update please try it should work – Mahammad Momin Commented Jan 30 at 12:58
- thanks for update, however i want to use library react-navigation. i dont want to use other library – One Commented Jan 31 at 7:42
The modal's height should now match the value you entered in the styles' height attribute.modalCard object.
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged ios react-native react-navigation react-navigation-native-stack or ask your own question .
- The Overflow Blog
- Where does Postgres fit in a world of GenAI and vector databases?
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- What does a new user need in a homepage experience on Stack Overflow?
- Feedback requested: How do you use tag hover descriptions for curating and do...
- Staging Ground Reviewer Motivation
Hot Network Questions
- Who was the "Dutch author", "Bumstone Bumstone"?
- What is an intuitive way to rename a column in a Dataset?
- How long does it take to achieve buoyancy in a body of water?
- Centering tikz graphic in OpTeX
- Completely introduce your friends
- Why an Out Parameter can be left unassigned in .NET 6 but not .NET 8 (CS0177)?
- Canonical ensemble, ergodicity and Liouville’s equation
- What prevents a browser from saving and tracking passwords entered to a site?
- Why does a halfing's racial trait lucky specify you must use the next roll?
- Can a rope thrower act as a propulsion method for land based craft?
- Is there a phrase for someone who's really bad at cooking?
- What would be non-slang equivalent of "copium"?
- Is there a faster way of expanding multiple polynomials with power?
- Briefly stopped at US Border because I read books
- What explanations can be offered for the extreme see-sawing in Montana's senate race polling?
- How much payload could the Falcon 9 send to geostationary orbit?
- Optimal Bath Fan Location
- The size of elementary particles
- Are my internal systems susceptible to MitM if the root/chain is shared amongst all customers?
- How much missing data is too much (part 2)? statistical power, effective sample size
- How do I make a command that makes a comma-separated list where all the items are bold?
- Using "no" at the end of a statement instead of "isn't it"?
- If inflation/cost of living is such a complex difficult problem, then why has the price of drugs been absoultly perfectly stable my whole life?
- Is it possible to have a planet that's gaslike in some areas and rocky in others?

IMAGES
VIDEO
COMMENTS
The onRequestClose callback is called when the user taps the hardware back button on Android or the menu button on Apple TV. Because of this required prop, be aware that BackHandler events will not be emitted as long as the modal is open. On iOS, this callback is called when a Modal is being dismissed using a drag gesture when presentationStyle ...
14. The accepted answer states: If you want a full-screen screen, you probably don't want a modal. That's not necessarily true. Use. screenOptions={{ presentation: 'transparentModal' }} or. screenOptions={{ presentation: 'fullScreenModal' }} for a full screen modal animation you can't dismiss on iOS.
When presentation is set to modal, the screens behave like a modal, i.e. they have a bottom to top transition and may show part of the previous screen in the background. Setting presentation: 'modal' on a group makes all the screens in the group modals, so to use non-modal transitions on other screens, we add another group with the default ...
An enhanced, animated, customizable React Native modal. The goal of react-native-modal is expanding the original React Native <Modal> component by adding animations, style customization options, and new features, while still providing a simple API.
Presenting modals. Modal can be displayed by invoking the Navigation.showModal() command, as shown in the example below: Or by declaring modal as a Component inside render function: Available for Android only, for iOS please use Modal from react-native. ( Full code can be found in the Playground ).
React Native provides a <Modal> component that presents content above the rest of your app. In general, modals are used to draw a user's attention toward critical information or guide them to take action. For example, in the second chapter, we used alert() to display a placeholder when a button is pressed. That's how a modal component displays an overlay.
Initialize the project. First, to initialize the project, type the following code into your terminal: expo init projectName && cd projectName && expo start. If you're adding the modal to an existing project instead of starting from scratch, skip this step, add react- native-modal to your project, and select the title option.
The mode prop for stack navigator can be either card (default) or modal. The modal behavior slides the screen in from the bottom on iOS and allows the user to swipe down from the top to dismiss it. The modal prop has no effect on Android because full-screen modals don't have any different transition behavior on the platform.
In the following react native modal example, we implemented the animated Modal by just setting the animation property to 'slide'. Apart from that, you can also choose 'fade' or 'none' prop to animate the modal. To set the React Native Modal's transparent background, we set the transparent property to false.
To use this navigator, ensure that you have @react-navigation/native and its dependencies (follow this guide), then install @react-navigation/stack: npm. Yarn. pnpm. npm install @react-navigation/stack. yarn add @react-navigation/stack. pnpm add @react-navigation/stack. Then, you need to install and configure the libraries that are required by ...
The react-native-modal plugin actually uses the Modal plugin shipped with React Native as mentioned in the usage section in the plugin documentation. The plugin allows both full-screen and a non-full-screen modes out of the box using the coverScreen property. It also provides a way to dismiss the modal when a click is tapped outside the modal box.
In this tutorial we look at how to achieve the standard modal design requirement for your React Native Apps.Thanks to all the channel supporters, I can creat...
Frequently Asked Questions The component is not working as expected. Under the hood react-native-modal uses react-native original Modal component. Before reporting a bug, try swapping react-native-modal with react-native original Modal component and, if the issue persists, check if it has already been reported as a react-native issue.. The backdrop is not completely filled/covered on some ...
A screen using this presentation style can't be dismissed by gesture. formSheet: will use "UIModalPresentationFormSheet" modal style on iOS and will fallback to "modal" on Android. ... from react-native-screens package is run at the top of the application.
Yes, you can use the Modal component from React Native: https: ... Reply reply jsizzle96 • Just a note that the UI varies for iOS and Android using this presentation style. On iOS pageSheet is user dismissible (via drag) in iOS13+ so keep that in mind as well. Not sure if a prop is exposed to prevent this. ...
In human-centered design, when we speak about a modal (or modality), we often refer to a set of techniques, aimed at bringing user attention to a specific event / screen / action / etc. ... This is documentation for React Native Navigation 6.12.2, ... Presentation Style# The presentation style determines the look and feel of a screen displayed ...
Because this is a native ios style to show a full-screen modal. And this library provides us with all things like the bottom sheet and modal if there is a way to implement ios modal presentation it will be the cherry on the cake. Possible implementation. I'll prefer to use reanimated for this type of animation. Code sample
It seems like you need to specify this information for a group, rather than for the specific screen. You were also assigning properties which the typescript compiler did not think were valid for React Navigation 6. Check that your text editor is showing the compiler errors as you type. <Stack.Group. screenOptions={{.
The modal's height should now match the value you entered in the styles' height attribute.modalCard object. import { StyleSheet } from 'react-native'; const styles = StyleSheet.create({.