- Branch and Bound Tutorial
- Backtracking Vs Branch-N-Bound
- 0/1 Knapsack
- 8 Puzzle Problem
- Job Assignment Problem
- N-Queen Problem
- Travelling Salesman Problem

Job Assignment Problem using Branch And Bound
Let there be N workers and N jobs. Any worker can be assigned to perform any job, incurring some cost that may vary depending on the work-job assignment. It is required to perform all jobs by assigning exactly one worker to each job and exactly one job to each agent in such a way that the total cost of the assignment is minimized.
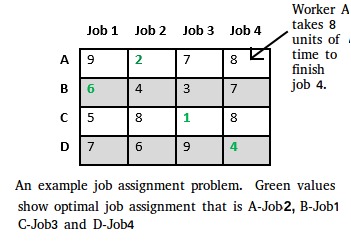
Let us explore all approaches for this problem.
Solution 1: Brute Force
We generate n! possible job assignments and for each such assignment, we compute its total cost and return the less expensive assignment. Since the solution is a permutation of the n jobs, its complexity is O(n!).
Solution 2: Hungarian Algorithm
The optimal assignment can be found using the Hungarian algorithm. The Hungarian algorithm has worst case run-time complexity of O(n^3).
Solution 3: DFS/BFS on state space tree
A state space tree is a N-ary tree with property that any path from root to leaf node holds one of many solutions to given problem. We can perform depth-first search on state space tree and but successive moves can take us away from the goal rather than bringing closer. The search of state space tree follows leftmost path from the root regardless of initial state. An answer node may never be found in this approach. We can also perform a Breadth-first search on state space tree. But no matter what the initial state is, the algorithm attempts the same sequence of moves like DFS.
Solution 4: Finding Optimal Solution using Branch and Bound
The selection rule for the next node in BFS and DFS is “blind”. i.e. the selection rule does not give any preference to a node that has a very good chance of getting the search to an answer node quickly. The search for an optimal solution can often be speeded by using an “intelligent” ranking function, also called an approximate cost function to avoid searching in sub-trees that do not contain an optimal solution. It is similar to BFS-like search but with one major optimization. Instead of following FIFO order, we choose a live node with least cost. We may not get optimal solution by following node with least promising cost, but it will provide very good chance of getting the search to an answer node quickly.
There are two approaches to calculate the cost function:
- For each worker, we choose job with minimum cost from list of unassigned jobs (take minimum entry from each row).
- For each job, we choose a worker with lowest cost for that job from list of unassigned workers (take minimum entry from each column).
In this article, the first approach is followed.
Let’s take below example and try to calculate promising cost when Job 2 is assigned to worker A.
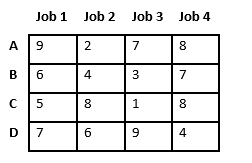
Since Job 2 is assigned to worker A (marked in green), cost becomes 2 and Job 2 and worker A becomes unavailable (marked in red).
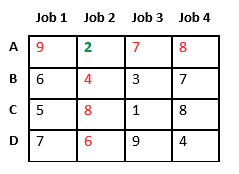
Now we assign job 3 to worker B as it has minimum cost from list of unassigned jobs. Cost becomes 2 + 3 = 5 and Job 3 and worker B also becomes unavailable.
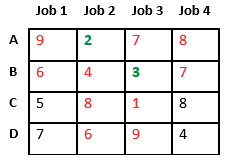
Finally, job 1 gets assigned to worker C as it has minimum cost among unassigned jobs and job 4 gets assigned to worker D as it is only Job left. Total cost becomes 2 + 3 + 5 + 4 = 14.
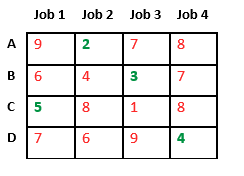
Below diagram shows complete search space diagram showing optimal solution path in green.
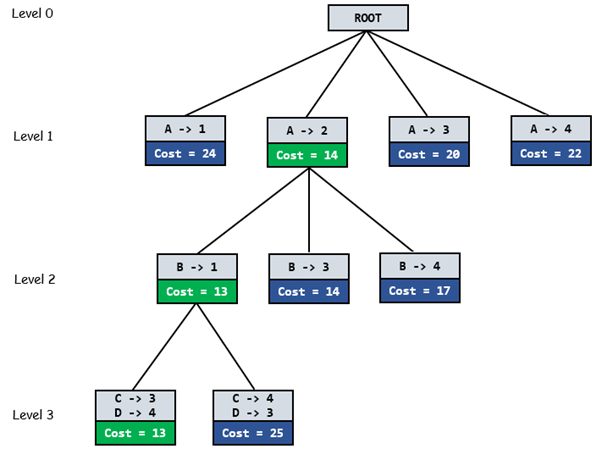
Complete Algorithm:
Below is the implementation of the above approach:
Time Complexity: O(M*N). This is because the algorithm uses a double for loop to iterate through the M x N matrix. Auxiliary Space: O(M+N). This is because it uses two arrays of size M and N to track the applicants and jobs.
Similar Reads
- Branch and Bound
Please Login to comment...
- PS5 Digital vs. Disc : Which PlayStation 5 Should You Buy in 2024?
- AMD vs. Nvidia Graphics Cards 2024: Which GPU Should You Choose?
- Top IPTV-Anbieter in Österreich für günstiges Streaming in 2024
- IPTV Austria: Top 10 IPTV Subscription Providers for Austrian and International Channels
- GeeksforGeeks Practice - Leading Online Coding Platform
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Hungarian Method
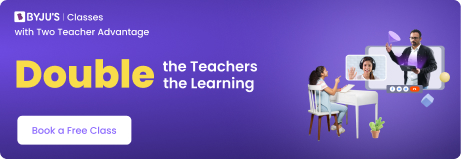
The Hungarian method is a computational optimization technique that addresses the assignment problem in polynomial time and foreshadows following primal-dual alternatives. In 1955, Harold Kuhn used the term “Hungarian method” to honour two Hungarian mathematicians, Dénes Kőnig and Jenő Egerváry. Let’s go through the steps of the Hungarian method with the help of a solved example.
Hungarian Method to Solve Assignment Problems
The Hungarian method is a simple way to solve assignment problems. Let us first discuss the assignment problems before moving on to learning the Hungarian method.
What is an Assignment Problem?
A transportation problem is a type of assignment problem. The goal is to allocate an equal amount of resources to the same number of activities. As a result, the overall cost of allocation is minimised or the total profit is maximised.
Because available resources such as workers, machines, and other resources have varying degrees of efficiency for executing different activities, and hence the cost, profit, or loss of conducting such activities varies.
Assume we have ‘n’ jobs to do on ‘m’ machines (i.e., one job to one machine). Our goal is to assign jobs to machines for the least amount of money possible (or maximum profit). Based on the notion that each machine can accomplish each task, but at variable levels of efficiency.
Hungarian Method Steps
Check to see if the number of rows and columns are equal; if they are, the assignment problem is considered to be balanced. Then go to step 1. If it is not balanced, it should be balanced before the algorithm is applied.
Step 1 – In the given cost matrix, subtract the least cost element of each row from all the entries in that row. Make sure that each row has at least one zero.
Step 2 – In the resultant cost matrix produced in step 1, subtract the least cost element in each column from all the components in that column, ensuring that each column contains at least one zero.
Step 3 – Assign zeros
- Analyse the rows one by one until you find a row with precisely one unmarked zero. Encircle this lonely unmarked zero and assign it a task. All other zeros in the column of this circular zero should be crossed out because they will not be used in any future assignments. Continue in this manner until you’ve gone through all of the rows.
- Examine the columns one by one until you find one with precisely one unmarked zero. Encircle this single unmarked zero and cross any other zero in its row to make an assignment to it. Continue until you’ve gone through all of the columns.
Step 4 – Perform the Optimal Test
- The present assignment is optimal if each row and column has exactly one encircled zero.
- The present assignment is not optimal if at least one row or column is missing an assignment (i.e., if at least one row or column is missing one encircled zero). Continue to step 5. Subtract the least cost element from all the entries in each column of the final cost matrix created in step 1 and ensure that each column has at least one zero.
Step 5 – Draw the least number of straight lines to cover all of the zeros as follows:
(a) Highlight the rows that aren’t assigned.
(b) Label the columns with zeros in marked rows (if they haven’t already been marked).
(c) Highlight the rows that have assignments in indicated columns (if they haven’t previously been marked).
(d) Continue with (b) and (c) until no further marking is needed.
(f) Simply draw the lines through all rows and columns that are not marked. If the number of these lines equals the order of the matrix, then the solution is optimal; otherwise, it is not.
Step 6 – Find the lowest cost factor that is not covered by the straight lines. Subtract this least-cost component from all the uncovered elements and add it to all the elements that are at the intersection of these straight lines, but leave the rest of the elements alone.
Step 7 – Continue with steps 1 – 6 until you’ve found the highest suitable assignment.
|
---|
Hungarian Method Example
Use the Hungarian method to solve the given assignment problem stated in the table. The entries in the matrix represent each man’s processing time in hours.
\(\begin{array}{l}\begin{bmatrix} & I & II & III & IV & V \\1 & 20 & 15 & 18 & 20 & 25 \\2 & 18 & 20 & 12 & 14 & 15 \\3 & 21 & 23 & 25 & 27 & 25 \\4 & 17 & 18 & 21 & 23 & 20 \\5 & 18 & 18 & 16 & 19 & 20 \\\end{bmatrix}\end{array} \)
With 5 jobs and 5 men, the stated problem is balanced.
\(\begin{array}{l}A = \begin{bmatrix}20 & 15 & 18 & 20 & 25 \\18 & 20 & 12 & 14 & 15 \\21 & 23 & 25 & 27 & 25 \\17 & 18 & 21 & 23 & 20 \\18 & 18 & 16 & 19 & 20 \\\end{bmatrix}\end{array} \)
Subtract the lowest cost element in each row from all of the elements in the given cost matrix’s row. Make sure that each row has at least one zero.
\(\begin{array}{l}A = \begin{bmatrix}5 & 0 & 3 & 5 & 10 \\6 & 8 & 0 & 2 & 3 \\0 & 2 & 4 & 6 & 4 \\0 & 1 & 4 & 6 & 3 \\2 & 2 & 0 & 3 & 4 \\\end{bmatrix}\end{array} \)
Subtract the least cost element in each Column from all of the components in the given cost matrix’s Column. Check to see if each column has at least one zero.
\(\begin{array}{l}A = \begin{bmatrix}5 & 0 & 3 & 3 & 7 \\6 & 8 & 0 & 0 & 0 \\0 & 2 & 4 & 4 & 1 \\0 & 1 & 4 & 4 & 0 \\2 & 2 & 0 & 1 & 1 \\\end{bmatrix}\end{array} \)
When the zeros are assigned, we get the following:
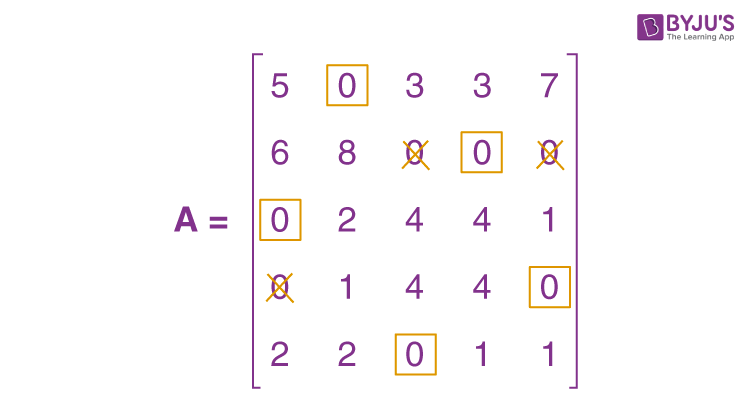
The present assignment is optimal because each row and column contain precisely one encircled zero.
Where 1 to II, 2 to IV, 3 to I, 4 to V, and 5 to III are the best assignments.
Hence, z = 15 + 14 + 21 + 20 + 16 = 86 hours is the optimal time.
Practice Question on Hungarian Method
Use the Hungarian method to solve the following assignment problem shown in table. The matrix entries represent the time it takes for each job to be processed by each machine in hours.
\(\begin{array}{l}\begin{bmatrix}J/M & I & II & III & IV & V \\1 & 9 & 22 & 58 & 11 & 19 \\2 & 43 & 78 & 72 & 50 & 63 \\3 & 41 & 28 & 91 & 37 & 45 \\4 & 74 & 42 & 27 & 49 & 39 \\5 & 36 & 11 & 57 & 22 & 25 \\\end{bmatrix}\end{array} \)
Stay tuned to BYJU’S – The Learning App and download the app to explore all Maths-related topics.
Frequently Asked Questions on Hungarian Method
What is hungarian method.
The Hungarian method is defined as a combinatorial optimization technique that solves the assignment problems in polynomial time and foreshadowed subsequent primal–dual approaches.

What are the steps involved in Hungarian method?
The following is a quick overview of the Hungarian method: Step 1: Subtract the row minima. Step 2: Subtract the column minimums. Step 3: Use a limited number of lines to cover all zeros. Step 4: Add some more zeros to the equation.
What is the purpose of the Hungarian method?
When workers are assigned to certain activities based on cost, the Hungarian method is beneficial for identifying minimum costs.
MATHS Related Links | |
Leave a Comment Cancel reply
Your Mobile number and Email id will not be published. Required fields are marked *
Request OTP on Voice Call
Post My Comment

Register with BYJU'S & Download Free PDFs
Register with byju's & watch live videos.
Assignment Problem: Meaning, Methods and Variations | Operations Research
After reading this article you will learn about:- 1. Meaning of Assignment Problem 2. Definition of Assignment Problem 3. Mathematical Formulation 4. Hungarian Method 5. Variations.
Meaning of Assignment Problem:
An assignment problem is a particular case of transportation problem where the objective is to assign a number of resources to an equal number of activities so as to minimise total cost or maximize total profit of allocation.
The problem of assignment arises because available resources such as men, machines etc. have varying degrees of efficiency for performing different activities, therefore, cost, profit or loss of performing the different activities is different.
Thus, the problem is “How should the assignments be made so as to optimize the given objective”. Some of the problem where the assignment technique may be useful are assignment of workers to machines, salesman to different sales areas.
Definition of Assignment Problem:
ADVERTISEMENTS:
Suppose there are n jobs to be performed and n persons are available for doing these jobs. Assume that each person can do each job at a term, though with varying degree of efficiency, let c ij be the cost if the i-th person is assigned to the j-th job. The problem is to find an assignment (which job should be assigned to which person one on-one basis) So that the total cost of performing all jobs is minimum, problem of this kind are known as assignment problem.
The assignment problem can be stated in the form of n x n cost matrix C real members as given in the following table:

www.springer.com The European Mathematical Society
- StatProb Collection
- Recent changes
- Current events
- Random page
- Project talk
- Request account
- What links here
- Related changes
- Special pages
- Printable version
- Permanent link
- Page information
- View source
Assignment problem
The problem of optimally assigning $ m $ individuals to $ m $ jobs. It can be formulated as a linear programming problem that is a special case of the transport problem :
maximize $ \sum _ {i,j } c _ {ij } x _ {ij } $
$$ \sum _ { j } x _ {ij } = a _ {i} , i = 1 \dots m $$
(origins or supply),
$$ \sum _ { i } x _ {ij } = b _ {j} , j = 1 \dots n $$
(destinations or demand), where $ x _ {ij } \geq 0 $ and $ \sum a _ {i} = \sum b _ {j} $, which is called the balance condition. The assignment problem arises when $ m = n $ and all $ a _ {i} $ and $ b _ {j} $ are $ 1 $.
If all $ a _ {i} $ and $ b _ {j} $ in the transposed problem are integers, then there is an optimal solution for which all $ x _ {ij } $ are integers (Dantzig's theorem on integral solutions of the transport problem).
In the assignment problem, for such a solution $ x _ {ij } $ is either zero or one; $ x _ {ij } = 1 $ means that person $ i $ is assigned to job $ j $; the weight $ c _ {ij } $ is the utility of person $ i $ assigned to job $ j $.
The special structure of the transport problem and the assignment problem makes it possible to use algorithms that are more efficient than the simplex method . Some of these use the Hungarian method (see, e.g., [a5] , [a1] , Chapt. 7), which is based on the König–Egervary theorem (see König theorem ), the method of potentials (see [a1] , [a2] ), the out-of-kilter algorithm (see, e.g., [a3] ) or the transportation simplex method.
In turn, the transportation problem is a special case of the network optimization problem.
A totally different assignment problem is the pole assignment problem in control theory.
[a1] | D.B. Yudin, E.G. Gol'shtein, "Linear programming" , Israel Program Sci. Transl. (1965) (In Russian) |
[a2] | R. Frisch, "La résolution des problèmes de programme linéaire par la méthode du potentiel logarithmique" , (1956) pp. 20–23 |
[a3] | K. Murtz, "Linear and combinatorial programming" , Wiley (1976) |
[a4] | M. Grötschel, L. Lovász, A. Schrijver, "Geometric algorithms and combinatorial optimization" , Springer (1987) |
[a5] | C.H. Papadimitriou, K. Steiglitz, "Combinatorial optimization" , Prentice-Hall (1982) |
- This page was last edited on 5 April 2020, at 18:48.
- Privacy policy
- About Encyclopedia of Mathematics
- Disclaimers
- Impressum-Legal

IMAGES
VIDEO
COMMENTS
The assignment problem consists of finding, in a weighted bipartite graph, a matching of a given size, in which the sum of weights of the edges is minimum. If the numbers of agents and tasks are equal, then the problem is called balanced assignment. Otherwise, it is called unbalanced assignment. [1] .
Solution 1: Brute Force. We generate n! possible job assignments and for each such assignment, we compute its total cost and return the less expensive assignment. Since the solution is a permutation of the n jobs, its complexity is O (n!). Solution 2: Hungarian Algorithm. The optimal assignment can be found using the Hungarian algorithm.
Assignment problem. ipartite graph G = (L. ! Goal: find a perfect matching of min weight. 2. Bipartite Matching. redu. ies and flows are 0/1. Flow corresponds t. Residual graph GM simplifies to: ! If (x, y) " M, then (x, y) is in GM. ! If (x, y) # M, the (y, x) is in GM. 1. s t. X Y. Augmenting path simplifies to:
What is an Assignment Problem? A transportation problem is a type of assignment problem. The goal is to allocate an equal amount of resources to the same number of activities. As a result, the overall cost of allocation is minimised or the total profit is maximised.
An assignment problem is a particular case of transportation problem where the objective is to assign a number of resources to an equal number of activities so as to minimise total cost or maximize total profit of allocation.
The assignment problem is particularly interesting because many seemingly different problems may be solved as assignment problems. Moreover, it is an important example of a combinatorial optimization problem; that is, a problem that seeks the best possible arrangement of objects among a specified set of many possible arrangements.
Assignment problem. Also known as weighted bipartite matching problem. Bipartite graph. Has two sets of nodes , ⇒ = ∪. And a set of edges connecting them. A matching on a bipartite graph G = (S, T, E) is a subset of edges ∈ ∋ no two edges in are incident to the same node. Nodes 1, 2, 3, 4. Node of are matched or covered.
The “assignment problem” is one that can be solved using simple techniques, at least for small problem sizes, and is easy to see how it could be applied to the real world. Assignment Problem. Pretend for a moment that you are writing software for a famous ride sharing application.
In this lesson we learn what is an assignment problem and how we can solve it using the Hungarian method.
History. Assignment problem. The problem of optimally assigning $ m $ individuals to $ m $ jobs. It can be formulated as a linear programming problem that is a special case of the transport problem: maximize $ \sum _ {i,j } c _ {ij } x _ {ij } $ subject to. $$ \sum _ { j } x _ {ij } = a _ {i} , i = 1 \dots m $$ (origins or supply),