

O.2 — Bitwise operators
The bitwise operators
C++ provides 6 bit manipulation operators, often called bitwise operators:
Author’s note
In the following examples, we will largely be working with 4-bit binary values. This is for the sake of convenience and keeping the examples simple. In actual programs, the number of bits used is based on the size of the object (e.g. a 2 byte object would store 16 bits).
For readability, we’ll also omit the 0b prefix outside of code examples (e.g. instead of 0b0101, we’ll just use 0101).
The bitwise operators are defined for integral types and std::bitset. We’ll use std::bitset in our examples because it’s easier to print the output in binary.
Avoid using the bitwise operators with signed operands, as many operators will return implementation-defined results prior to C++20 or have other potential gotchas that are easily avoided by using unsigned operands (or std::bitset).
Best practice
To avoid surprises, use the bitwise operators with unsigned operands or std::bitset.
Bitwise left shift (<<) and bitwise right shift (>>) operators
The bitwise left shift (<<) operator shifts bits to the left. The left operand is the expression to shift the bits of, and the right operand is an integer number of bits to shift left by.
So when we say x << 1 , we are saying “shift the bits in the variable x left by 1 place”. New bits shifted in from the right side receive the value 0.
0011 << 1 is 0110 0011 << 2 is 1100 0011 << 3 is 1000
Note that in the third case, we shifted a bit off the end of the number! Bits that are shifted off the end of the binary number are lost forever.
The bitwise right shift (>>) operator shifts bits to the right.
1100 >> 1 is 0110 1100 >> 2 is 0011 1100 >> 3 is 0001
Note that in the third case we shifted a bit off the right end of the number, so it is lost.
Here’s an example of doing some bit shifting:
This prints:
For advanced readers
Bit-shifting in C++ is endian -agnostic. Left-shift is always towards the most significant bit, and right-shift towards the least significant bit.
What!? Aren’t operator<< and operator>> used for input and output?
They sure are.
Programs today typically do not make much use of the bitwise left and right shift operators to shift bits. Rather, you tend to see the bitwise left shift operator used with std::cout (or other stream objects) to output text. Consider the following program:
This program prints:
In the above program, how does operator<< know to shift bits in one case and output x in another case? The answer is that std::cout has overloaded (provided an alternate definition for) operator<< that does console output rather than bit shifting.
When the compiler sees that the left operand of operator<< is std::cout, it knows that it should call the version of operator<< that std::cout overloaded to do output. If the left operand is an integral type, then operator<< knows it should do its usual bit-shifting behavior.
The same applies for operator>>.
Note that if you’re using operator << for both output and left shift, parenthesization is required:
The first line prints the value of x (0110), and then the literal 1. The second line prints the value of x left-shifted by 1 (1100).
We will talk more about operator overloading in a future chapter, including discussion of how to overload operators for your own purposes.
Bitwise NOT
The bitwise NOT operator (~) is perhaps the easiest to understand of all the bitwise operators. It simply flips each bit from a 0 to a 1, or vice versa. Note that the result of a bitwise NOT is dependent on what size your data type is.
Flipping 4 bits: ~0100 is 1011
Flipping 8 bits: ~0000 0100 is 1111 1011
In both the 4-bit and 8-bit cases, we start with the same number (binary 0100 is the same as 0000 0100 in the same way that decimal 7 is the same as 07), but we end up with a different result.
We can see this in action in the following program:
This prints: 1011 11111011
Bitwise OR (|) works much like its logical OR counterpart. However, instead of applying the OR to the operands to produce a single result, bitwise OR applies to each bit! For example, consider the expression 0b0101 | 0b0110 .
To do (any) bitwise operations, it is easiest to line the two operands up like this:
and then apply the operation to each column of bits.
If you remember, logical OR evaluates to true (1) if either the left, right, or both operands are true (1) , and 0 otherwise. Bitwise OR evaluates to 1 if either the left, right, or both bits are 1 , and 0 otherwise. Consequently, the expression evaluates like this:
Our result is 0111 binary.
We can do the same thing to compound OR expressions, such as 0b0111 | 0b0011 | 0b0001 . If any of the bits in a column are 1 , the result of that column is 1 .
Here’s code for the above:
Bitwise AND
Bitwise AND (&) works similarly to the above. Logical AND evaluates to true if both the left and right operand evaluate to true . Bitwise AND evaluates to true (1) if both bits in the column are 1 . Consider the expression 0b0101 & 0b0110 . Lining each of the bits up and applying an AND operation to each column of bits:
Similarly, we can do the same thing to compound AND expressions, such as 0b0001 & 0b0011 & 0b0111 . If all of the bits in a column are 1, the result of that column is 1.
Bitwise XOR
The last operator is the bitwise XOR (^), also known as exclusive or .
When evaluating two operands, XOR evaluates to true (1) if one and only one of its operands is true (1) . If neither or both are true, it evaluates to 0 . Consider the expression 0b0110 ^ 0b0011 :
It is also possible to evaluate compound XOR expression column style, such as 0b0001 ^ 0b0011 ^ 0b0111 . If there are an even number of 1 bits in a column, the result is 0 . If there are an odd number of 1 bits in a column, the result is 1 .
Bitwise assignment operators
Similar to the arithmetic assignment operators, C++ provides bitwise assignment operators in order to facilitate easy modification of variables.
For example, instead of writing x = x >> 1; , you can write x >>= 1; .
As an aside…
There is no bitwise NOT assignment operator. This is because the other bitwise operators are binary, but bitwise NOT is unary (so what would go on the right-hand side of a ~= operator?). If you want to flip all of the bits, you can use normal assignment here: x = ~x;
Summarizing how to evaluate bitwise operations utilizing the column method:
When evaluating bitwise OR , if any bit in a column is 1, the result for that column is 1. When evaluating bitwise AND , if all bits in a column are 1, the result for that column is 1. When evaluating bitwise XOR , if there are an odd number of 1 bits in a column, the result for that column is 1.
In the next lesson, we’ll explore how these operators can be used in conjunction with bit masks to facilitate bit manipulation.
Question #1
a) What does 0110 >> 2 evaluate to in binary?
Show Solution
0110 >> 2 evaluates to 0001
b) What does the following evaluate to in binary: 0011 | 0101?
c) What does the following evaluate to in binary: 0011 & 0101?
d) What does the following evaluate to in binary (0011 | 0101) & 1001?
Question #2
A bitwise rotation is like a bitwise shift, except that any bits shifted off one end are added back to the other end. For example 0b1001 << 1 would be 0b0010 , but a left rotate by 1 would result in 0b0011 instead. Implement a function that does a left rotate on a std::bitset<4> . For this one, it’s okay to use test() and set().
The following code should execute:
and print the following:
We have named the function “rotl” rather than “rotateLeft”, because “rotl” is a well-established name in computer science and also the name of the standard function, std::rotl .
Question #3
Extra credit: Redo quiz #2 but don’t use the test and set functions (use bitwise operators).
- Skip to main content
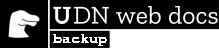
- Bitwise XOR assignment (^=)
The bitwise XOR assignment operator ( ^= ) uses the binary representation of both operands, does a bitwise XOR operation on them and assigns the result to the variable.
The source for this interactive example is stored in a GitHub repository. If you'd like to contribute to the interactive examples project, please clone https://github.com/mdn/interactive-examples and send us a pull request.
Using bitwise XOR assignment
Specifications, browser compatibility.
- Assignment operators in the JS guide
- Bitwise XOR operator
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Using promises
- Iterators and generators
- Meta programming
- JavaScript modules
- Client-side JavaScript frameworks
- Client-side web APIs
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- AggregateError
- ArrayBuffer
- AsyncFunction
- BigInt64Array
- BigUint64Array
- FinalizationRegistry
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- ReferenceError
- SharedArrayBuffer
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Addition (+)
- Addition assignment (+=)
- Assignment (=)
- Bitwise AND (&)
- Bitwise AND assignment (&=)
- Bitwise NOT (~)
- Bitwise OR (|)
- Bitwise OR assignment (|=)
- Bitwise XOR (^)
- Comma operator (,)
- Conditional (ternary) operator
- Decrement (--)
- Destructuring assignment
- Division (/)
- Division assignment (/=)
- Equality (==)
- Exponentiation (**)
- Exponentiation assignment (**=)
- Function expression
- Greater than (>)
- Greater than or equal (>=)
- Grouping operator ( )
- Increment (++)
- Inequality (!=)
- Left shift (<<)
- Left shift assignment (<<=)
- Less than (<)
- Less than or equal (<=)
- Logical AND (&&)
- Logical AND assignment (&&=)
- Logical NOT (!)
- Logical OR (||)
- Logical OR assignment (||=)
- Logical nullish assignment (??=)
- Multiplication (*)
- Multiplication assignment (*=)
- Nullish coalescing operator (??)
- Object initializer
- Operator precedence
- Optional chaining (?.)
- Pipeline operator (|>)
- Property accessors
- Remainder (%)
- Remainder assignment (%=)
- Right shift (>>)
- Right shift assignment (>>=)
- Spread syntax (...)
- Strict equality (===)
- Strict inequality (!==)
- Subtraction (-)
- Subtraction assignment (-=)
- Unary negation (-)
- Unary plus (+)
- Unsigned right shift (>>>)
- Unsigned right shift assignment (>>>=)
- async function expression
- class expression
- delete operator
- function* expression
- in operator
- new operator
- void operator
- async function
- for await...of
- function declaration
- import.meta
- try...catch
- Arrow function expressions
- Default parameters
- Method definitions
- Rest parameters
- The arguments object
- Private class fields
- Public class fields
- constructor
- Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: can't access lexical declaration "x" before initialization
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the "delete" operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: "x" is not iterable
- TypeError: More arguments needed
- TypeError: Reduce of empty array with no initial value
- TypeError: X.prototype.y called on incompatible type
- TypeError: can't access dead object
- TypeError: can't access property "x" of "y"
- TypeError: can't assign to property "x" on "y": not an object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cannot use "in" operator to search for "x" in "y"
- TypeError: cyclic object value
- TypeError: invalid "instanceof" operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- Skip to main content
- Select language
- Skip to search
- Assignment operators
An assignment operator assigns a value to its left operand based on the value of its right operand.
The basic assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x . The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
Simple assignment operator which assigns a value to a variable. The assignment operation evaluates to the assigned value. Chaining the assignment operator is possible in order to assign a single value to multiple variables. See the example.
Addition assignment
The addition assignment operator adds the value of the right operand to a variable and assigns the result to the variable. The types of the two operands determine the behavior of the addition assignment operator. Addition or concatenation is possible. See the addition operator for more details.
Subtraction assignment
The subtraction assignment operator subtracts the value of the right operand from a variable and assigns the result to the variable. See the subtraction operator for more details.
Multiplication assignment
The multiplication assignment operator multiplies a variable by the value of the right operand and assigns the result to the variable. See the multiplication operator for more details.
Division assignment
The division assignment operator divides a variable by the value of the right operand and assigns the result to the variable. See the division operator for more details.
Remainder assignment
The remainder assignment operator divides a variable by the value of the right operand and assigns the remainder to the variable. See the remainder operator for more details.
Exponentiation assignment
This is an experimental technology, part of the ECMAScript 2016 (ES7) proposal. Because this technology's specification has not stabilized, check the compatibility table for usage in various browsers. Also note that the syntax and behavior of an experimental technology is subject to change in future version of browsers as the spec changes.
The exponentiation assignment operator evaluates to the result of raising first operand to the power second operand. See the exponentiation operator for more details.
Left shift assignment
The left shift assignment operator moves the specified amount of bits to the left and assigns the result to the variable. See the left shift operator for more details.
Right shift assignment
The right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the right shift operator for more details.
Unsigned right shift assignment
The unsigned right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the unsigned right shift operator for more details.
Bitwise AND assignment
The bitwise AND assignment operator uses the binary representation of both operands, does a bitwise AND operation on them and assigns the result to the variable. See the bitwise AND operator for more details.
Bitwise XOR assignment
The bitwise XOR assignment operator uses the binary representation of both operands, does a bitwise XOR operation on them and assigns the result to the variable. See the bitwise XOR operator for more details.
Bitwise OR assignment
The bitwise OR assignment operator uses the binary representation of both operands, does a bitwise OR operation on them and assigns the result to the variable. See the bitwise OR operator for more details.
Left operand with another assignment operator
In unusual situations, the assignment operator (e.g. x += y ) is not identical to the meaning expression (here x = x + y ). When the left operand of an assignment operator itself contains an assignment operator, the left operand is evaluated only once. For example:
Specifications
Browser compatibility.
- Arithmetic operators
Document Tags and Contributors
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Array comprehensions
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical Operators
- Object initializer
- Operator precedence
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- function declaration
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
- Java - Home
- Java - Introduction
- Java - Syntax
- Java - Comments
- Java - Data Types
- Java - Type Casting
- Java - Operators
- Java - Strings
- Java - Booleans
- Java - If Else
- Java - Switch
- Java - While Loop
- Java - For Loop
- Java - Continue Statement
- Java - Break Statement
- Java - Label Statement
- Java - Arrays
- Java - Methods
- Java - Exceptions
- Java - Classes/Objects
- Java - Constructors
- Java - Encapsulation
- Java - Inheritance
- Java - Polymorphism
- Java.lang Package
- Java Utility Package
- Java - Keywords
- Java - String Methods
- Java - Math Methods
- Java - Data Structures
- Java - Examples
- Java - Interview Questions
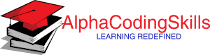
- Programming Languages
- Web Technologies
- Database Technologies
- Microsoft Technologies
- Python Libraries
- Data Structures
- Interview Questions
- PHP & MySQL
- C++ Standard Library
- C Standard Library
- Java Utility Library
- Java Default Package
- PHP Function Reference
Java - Bitwise XOR and assignment operator
The Bitwise XOR and assignment operator (^=) assigns the first operand a value equal to the result of Bitwise XOR operation of two operands.
(x ^= y) is equivalent to (x = x ^ y)
The Bitwise XOR operator (^) is a binary operator which takes two bit patterns of equal length and performs the logical exclusive OR operation on each pair of corresponding bits. It returns 1 if only one of the bits is 1, else returns 0.
The example below describes how bitwise XOR operator works:
The code of using Bitwise XOR and assignment operator (^=) is given below:
The output of the above code will be:
Example: Swap two numbers without using temporary variable
The bitwise XOR and assignment operator can be used to swap the value of two variables. Consider the example below.
The above code will give the following output:
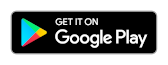
- Data Structures Tutorial
- Algorithms Tutorial
- JavaScript Tutorial
- Python Tutorial
- MySQLi Tutorial
- Java Tutorial
- Scala Tutorial
- C++ Tutorial
- C# Tutorial
- PHP Tutorial
- MySQL Tutorial
- SQL Tutorial
- PHP Function reference
- C++ - Standard Library
- Ruby Tutorial
- Rust Tutorial
- Swift Tutorial
- Perl Tutorial
- HTML Tutorial
- CSS Tutorial
- AJAX Tutorial
- XML Tutorial
- Online Compilers
- QuickTables
- NumPy Tutorial
- Pandas Tutorial
- Matplotlib Tutorial
- SciPy Tutorial
- Seaborn Tutorial
- TutorialKart
- SAP Tutorials
- Salesforce Admin
- Salesforce Developer
- Visualforce
- Informatica
- Kafka Tutorial
- Spark Tutorial
- Tomcat Tutorial
- Python Tkinter
Programming
- Bash Script
- Julia Tutorial
- CouchDB Tutorial
- MongoDB Tutorial
- PostgreSQL Tutorial
- Android Compose
- Flutter Tutorial
- Kotlin Android
Web & Server
- Selenium Java
- Java Basics
- Java Tutorial
- Java HelloWorld Program
- Java Program Structure
- Java Datatypes
- Java Variable Types
- Java Access Modifiers
- Java Operators
- Java Decision Making
- Print array
- Initialize array
- Array of integers
- Array of strings
- Array of objects
- Array of arrays
- Iterate over array
- Array For loop
- Array while loop
- Append element to array
- Check if array is empty
- Array average
- Check if array contains
- Array ForEach
- Array - Find Index of Item
- Concatenate arrays
- Find smallest number in array
- Find largest number in array
- Array reverse
- Classes and Objects
- Inheritance
- Polymorphism
- Method Overloading
- Method Overriding/
- Abstraction
- Abstract methods and classes
- Encapsulation
- Print string
- Read string from console
- Create string from Char array
- Create string from Byte array
- Concatenate two strings
- Get index of the first Occurrence of substring
- Get index of nth occurrence of substring
- Check if two strings are equal
- Check if string ends with specific suffix
- Check if string starts with specific prefix
- Check if string is blank
- Check if string is empty
- Check if string contains search substring
- Validate if string is a Phone Number
- Character Level
- Get character at specific index in string
- Get first character in string
- Get last character from string
- Transformations
- Replace first occurrence of string
- Replace all occurrences of a string
- Join strings
- Join strings in string array
- Join strings in ArrayList
- Reverse a string
- Trim string
- Split string
- Remove whitespaces in string
- Replace multiple spaces with single space
- Comparisons
- Compare strings lexicographically
- Compare String and CharSequence
- Compare String and StringBuffer
- Java Exception Handling StringIndexOutOfBoundsException
- Convert string to int
- Convert string to float
- Convert string to double
- Convert string to long
- Convert string to boolean
- Convert int to string
- Convert int to float
- Convert int to double
- Convert int to long
- Convert int to char
- Convert float to string
- Convert float to int
- Convert float to double
- Convert float to long
- Convert long to string
- Convert long to float
- Convert long to double
- Convert long to int
- Convert double to string
- Convert double to float
- Convert double to int
- Convert double to long
- Convert char to int
- Convert boolean to string
- Create a file
- Read file as string
- Write string to file
- Delete File
- Rename File
- Download File from URL
- Replace a String in File
- Filter list of files or directories
- Check if file is readable
- Check if file is writable
- Check if file is executable
- Read contents of a file line by line using BufferedReader
- Read contents of a File line by line using Stream
- Check if n is positive or negative
- Read integer from console
- Add two integers
- Count digits in number
- Largest of three numbers
- Smallest of three numbers
- Even numbers
- Odd numbers
- Reverse a number
- Prime Number
- Print All Prime Numbers
- Factors of a Number
- Check Palindrome number
- Check Palindrome string
- Swap two numbers
- Even or Odd number
- Java Classes
- ArrayList add()
- ArrayList addAll()
- ArrayList clear()
- ArrayList clone()
- ArrayList contains()
- ArrayList ensureCapacity()
- ArrayList forEach()
- ArrayList get()
- ArrayList indexOf()
- ArrayList isEmpty()
- ArrayList iterator()
- ArrayList lastIndexOf()
- ArrayList listIterator()
- ArrayList remove()
- ArrayList removeAll()
- ArrayList removeIf()
- ArrayList removeRange()
- ArrayList retainAll()
- ArrayList set()
- ArrayList size()
- ArrayList spliterator()
- ArrayList subList()
- ArrayList toArray()
- ArrayList trimToSize()
- HashMap clear()
- HashMap clone()
- HashMap compute()
- HashMap computeIfAbsent()
- HashMap computeIfPresent()
- HashMap containsKey()
- HashMap containsValue()
- HashMap entrySet()
- HashMap get()
- HashMap isEmpty()
- HashMap keySet()
- HashMap merge()
- HashMap put()
- HashMap putAll()
- HashMap remove()
- HashMap size()
- HashMap values()
- HashSet add()
- HashSet clear()
- HashSet clone()
- HashSet contains()
- HashSet isEmpty()
- HashSet iterator()
- HashSet remove()
- HashSet size()
- HashSet spliterator()
- Integer bitCount()
- Integer byteValue()
- Integer compare()
- Integer compareTo()
- Integer compareUnsigned()
- Integer decode()
- Integer divideUnsigned()
- Integer doubleValue()
- Integer equals()
- Integer floatValue()
- Integer getInteger()
- Integer hashCode()
- Integer highestOneBit()
- Integer intValue()
- Integer longValue()
- Integer lowestOneBit()
- Integer max()
- Integer min()
- Integer numberOfLeadingZeros()
- Integer numberOfTrailingZeros()
- Integer parseInt()
- Integer parseUnsignedInt()
- Integer remainderUnsigned()
- Integer reverse()
- Integer reverseBytes()
- Integer rotateLeft()
- Integer rotateRight()
- Integer shortValue()
- Integer signum()
- Integer sum()
- Integer toBinaryString()
- Integer toHexString()
- Integer toOctalString()
- Integer toString()
- Integer toUnsignedLong()
- Integer toUnsignedString()
- Integer valueOf()
- StringBuilder append()
- StringBuilder appendCodePoint()
- StringBuilder capacity()
- StringBuilder charAt()
- StringBuilder chars()
- StringBuilder codePointAt()
- StringBuilder codePointBefore()
- StringBuilder codePointCount()
- StringBuilder codePoints()
- StringBuilder delete()
- StringBuilder deleteCharAt()
- StringBuilder ensureCapacity()
- StringBuilder getChars()
- StringBuilder indexOf()
- StringBuilder insert()
- StringBuilder lastIndexOf()
- StringBuilder length()
- StringBuilder offsetByCodePoints()
- StringBuilder replace()
- StringBuilder reverse()
- StringBuilder setCharAt()
- StringBuilder setLength()
- StringBuilder subSequence()
- StringBuilder substring()
- StringBuilder toString()
- StringBuilder trimToSize()
- Arrays.asList()
- Arrays.binarySearch()
- Arrays.copyOf()
- Arrays.copyOfRange()
- Arrays.deepEquals()
- Arrays.deepToString()
- Arrays.equals()
- Arrays.fill()
- Arrays.hashCode()
- Arrays.sort()
- Arrays.toString()
- Random doubles()
- Random ints()
- Random longs()
- Random next()
- Random nextBoolean()
- Random nextBytes()
- Random nextDouble()
- Random nextFloat()
- Random nextGaussian()
- Random nextInt()
- Random nextLong()
- Random setSeed()
- Math random
- Math signum
- Math toDegrees
- Math toRadians
- Java Date & Time
- ❯ Java Tutorial
Java Bitwise XOR Assignment (^=) Operator
Java bitwise xor assignment.
In Java, Bitwise XOR Assignment Operator is used to compute the Bitwise XOR operation between left and right operands, and assign the result back to left operand.
In this tutorial, we will learn how to use Bitwise XOR Assignment operator in Java, with examples.
The syntax to compute bitwise XOR a value of 2 and value in variable x , and assign the result back to x using Bitwise XOR Assignment Operator is
In the following example, we take a variable x with an initial value of 9 , add bitwise XOR it with value of 2 , and assign the result to x , using Bitwise XOR Assignment Operator.
In this Java Tutorial , we learned about Bitwise XOR Assignment Operator in Java, with examples.
Popular Courses by TutorialKart
App developement, web development, online tools.
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Bitwise and shift operators (C# reference)
- 9 contributors
The bitwise and shift operators include unary bitwise complement, binary left and right shift, unsigned right shift, and the binary logical AND, OR, and exclusive OR operators. These operands take operands of the integral numeric types or the char type.
- Unary ~ (bitwise complement) operator
- Binary << (left shift) , >> (right shift) , and >>> (unsigned right shift) operators
- Binary & (logical AND) , | (logical OR) , and ^ (logical exclusive OR) operators
Those operators are defined for the int , uint , long , and ulong types. When both operands are of other integral types ( sbyte , byte , short , ushort , or char ), their values are converted to the int type, which is also the result type of an operation. When operands are of different integral types, their values are converted to the closest containing integral type. For more information, see the Numeric promotions section of the C# language specification . The compound operators (such as >>= ) don't convert their arguments to int or have the result type as int .
The & , | , and ^ operators are also defined for operands of the bool type. For more information, see Boolean logical operators .
Bitwise and shift operations never cause overflow and produce the same results in checked and unchecked contexts.
- Bitwise complement operator ~
The ~ operator produces a bitwise complement of its operand by reversing each bit:
You can also use the ~ symbol to declare finalizers. For more information, see Finalizers .
Left-shift operator <<
The << operator shifts its left-hand operand left by the number of bits defined by its right-hand operand. For information about how the right-hand operand defines the shift count, see the Shift count of the shift operators section.
The left-shift operation discards the high-order bits that are outside the range of the result type and sets the low-order empty bit positions to zero, as the following example shows:
Because the shift operators are defined only for the int , uint , long , and ulong types, the result of an operation always contains at least 32 bits. If the left-hand operand is of another integral type ( sbyte , byte , short , ushort , or char ), its value is converted to the int type, as the following example shows:
Right-shift operator >>
The >> operator shifts its left-hand operand right by the number of bits defined by its right-hand operand. For information about how the right-hand operand defines the shift count, see the Shift count of the shift operators section.
The right-shift operation discards the low-order bits, as the following example shows:
The high-order empty bit positions are set based on the type of the left-hand operand as follows:
If the left-hand operand is of type int or long , the right-shift operator performs an arithmetic shift: the value of the most significant bit (the sign bit) of the left-hand operand is propagated to the high-order empty bit positions. That is, the high-order empty bit positions are set to zero if the left-hand operand is non-negative and set to one if it's negative.
If the left-hand operand is of type uint or ulong , the right-shift operator performs a logical shift: the high-order empty bit positions are always set to zero.
Use the unsigned right-shift operator to perform a logical shift on operands of signed integer types. This is preferred to casting a left-hand operand to an unsigned type and then casting the result of a shift operation back to a signed type.
Unsigned right-shift operator >>>
Available in C# 11 and later, the >>> operator shifts its left-hand operand right by the number of bits defined by its right-hand operand. For information about how the right-hand operand defines the shift count, see the Shift count of the shift operators section.
The >>> operator always performs a logical shift. That is, the high-order empty bit positions are always set to zero, regardless of the type of the left-hand operand. The >> operator performs an arithmetic shift (that is, the value of the most significant bit is propagated to the high-order empty bit positions) if the left-hand operand is of a signed type. The following example demonstrates the difference between >> and >>> operators for a negative left-hand operand:
- Logical AND operator &
The & operator computes the bitwise logical AND of its integral operands:
For bool operands, the & operator computes the logical AND of its operands. The unary & operator is the address-of operator .
- Logical exclusive OR operator ^
The ^ operator computes the bitwise logical exclusive OR, also known as the bitwise logical XOR, of its integral operands:
For bool operands, the ^ operator computes the logical exclusive OR of its operands.
- Logical OR operator |
The | operator computes the bitwise logical OR of its integral operands:
For bool operands, the | operator computes the logical OR of its operands.
- Compound assignment
For a binary operator op , a compound assignment expression of the form
is equivalent to
except that x is only evaluated once.
The following example demonstrates the usage of compound assignment with bitwise and shift operators:
Because of numeric promotions , the result of the op operation might be not implicitly convertible to the type T of x . In such a case, if op is a predefined operator and the result of the operation is explicitly convertible to the type T of x , a compound assignment expression of the form x op= y is equivalent to x = (T)(x op y) , except that x is only evaluated once. The following example demonstrates that behavior:
Operator precedence
The following list orders bitwise and shift operators starting from the highest precedence to the lowest:
- Shift operators << , >> , and >>>
Use parentheses, () , to change the order of evaluation imposed by operator precedence:
For the complete list of C# operators ordered by precedence level, see the Operator precedence section of the C# operators article.
Shift count of the shift operators
For the built-in shift operators << , >> , and >>> , the type of the right-hand operand must be int or a type that has a predefined implicit numeric conversion to int .
For the x << count , x >> count , and x >>> count expressions, the actual shift count depends on the type of x as follows:
If the type of x is int or uint , the shift count is defined by the low-order five bits of the right-hand operand. That is, the shift count is computed from count & 0x1F (or count & 0b_1_1111 ).
If the type of x is long or ulong , the shift count is defined by the low-order six bits of the right-hand operand. That is, the shift count is computed from count & 0x3F (or count & 0b_11_1111 ).
The following example demonstrates that behavior:
As the preceding example shows, the result of a shift operation can be non-zero even if the value of the right-hand operand is greater than the number of bits in the left-hand operand.
Enumeration logical operators
The ~ , & , | , and ^ operators are also supported by any enumeration type. For operands of the same enumeration type, a logical operation is performed on the corresponding values of the underlying integral type. For example, for any x and y of an enumeration type T with an underlying type U , the x & y expression produces the same result as the (T)((U)x & (U)y) expression.
You typically use bitwise logical operators with an enumeration type that is defined with the Flags attribute. For more information, see the Enumeration types as bit flags section of the Enumeration types article.
Operator overloadability
A user-defined type can overload the ~ , << , >> , >>> , & , | , and ^ operators. When a binary operator is overloaded, the corresponding compound assignment operator is also implicitly overloaded. A user-defined type can't explicitly overload a compound assignment operator.
If a user-defined type T overloads the << , >> , or >>> operator, the type of the left-hand operand must be T . In C# 10 and earlier, the type of the right-hand operand must be int ; beginning with C# 11, the type of the right-hand operand of an overloaded shift operator can be any.
C# language specification
For more information, see the following sections of the C# language specification :
- Bitwise complement operator
- Shift operators
- Logical operators
- Numeric promotions
- C# 11 - Relaxed shift requirements
- C# 11 - Logical right-shift operator
- C# operators and expressions
- Boolean logical operators
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Specifications
Browser compatibility.
An assignment operator assigns a value to its left operand based on the value of its right operand.
The source for this interactive example is stored in a GitHub repository. If you'd like to contribute to the interactive examples project, please clone https://github.com/mdn/interactive-examples and send us a pull request.
The basic assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x . The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
Simple assignment operator is used to assign a value to a variable. The assignment operation evaluates to the assigned value. Chaining the assignment operator is possible in order to assign a single value to multiple variables. See the example.
Addition assignment
The addition assignment operator adds the value of the right operand to a variable and assigns the result to the variable. The types of the two operands determine the behavior of the addition assignment operator. Addition or concatenation is possible. See the addition operator for more details.
Subtraction assignment
The subtraction assignment operator subtracts the value of the right operand from a variable and assigns the result to the variable. See the subtraction operator for more details.
Multiplication assignment
The multiplication assignment operator multiplies a variable by the value of the right operand and assigns the result to the variable. See the multiplication operator for more details.
Division assignment
The division assignment operator divides a variable by the value of the right operand and assigns the result to the variable. See the division operator for more details.
Remainder assignment
The remainder assignment operator divides a variable by the value of the right operand and assigns the remainder to the variable. See the remainder operator for more details.
Exponentiation assignment
The exponentiation assignment operator evaluates to the result of raising first operand to the power second operand. See the exponentiation operator for more details.
Left shift assignment
The left shift assignment operator moves the specified amount of bits to the left and assigns the result to the variable. See the left shift operator for more details.
Right shift assignment
The right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the right shift operator for more details.
Unsigned right shift assignment
The unsigned right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the unsigned right shift operator for more details.
Bitwise AND assignment
The bitwise AND assignment operator uses the binary representation of both operands, does a bitwise AND operation on them and assigns the result to the variable. See the bitwise AND operator for more details.
Bitwise XOR assignment
The bitwise XOR assignment operator uses the binary representation of both operands, does a bitwise XOR operation on them and assigns the result to the variable. See the bitwise XOR operator for more details.

Bitwise OR assignment
The bitwise OR assignment operator uses the binary representation of both operands, does a bitwise OR operation on them and assigns the result to the variable. See the bitwise OR operator for more details.
Left operand with another assignment operator
In unusual situations, the assignment operator (e.g. x += y ) is not identical to the meaning expression (here x = x + y ). When the left operand of an assignment operator itself contains an assignment operator, the left operand is evaluated only once. For example:
- Arithmetic operators
Document Tags and Contributors
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Using promises
- Iterators and generators
- Meta programming
- JavaScript modules
- Client-side web APIs
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.ListFormat
- Intl.Locale
- Intl.NumberFormat
- Intl.PluralRules
- Intl.RelativeTimeFormat
- ReferenceError
- SharedArrayBuffer
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Array comprehensions
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical operators
- Object initializer
- Operator precedence
- (currently at stage 1) pipes the value of an expression into a function. This allows the creation of chained function calls in a readable manner. The result is syntactic sugar in which a function call with a single argument can be written like this:">Pipeline operator
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for await...of
- for each...in
- function declaration
- import.meta
- try...catch
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- The arguments object
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: can't access lexical declaration`X' before initialization
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: 'x' is not iterable
- TypeError: More arguments needed
- TypeError: Reduce of empty array with no initial value
- TypeError: can't access dead object
- TypeError: can't access property "x" of "y"
- TypeError: can't assign to property "x" on "y": not an object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cannot use 'in' operator to search for 'x' in 'y'
- TypeError: cyclic object value
- TypeError: invalid 'instanceof' operand 'x'
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- X.prototype.y called on incompatible type
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
Learn the best of web development
Get the latest and greatest from MDN delivered straight to your inbox.
Thanks! Please check your inbox to confirm your subscription.
If you haven’t previously confirmed a subscription to a Mozilla-related newsletter you may have to do so. Please check your inbox or your spam filter for an email from us.
PrepBytes Blog
ONE-STOP RESOURCE FOR EVERYTHING RELATED TO CODING
Sign in to your account
Forgot your password?
Login via OTP
We will send you an one time password on your mobile number
An OTP has been sent to your mobile number please verify it below
Register with PrepBytes
Assignment operator in python.
Last Updated on June 8, 2023 by Prepbytes
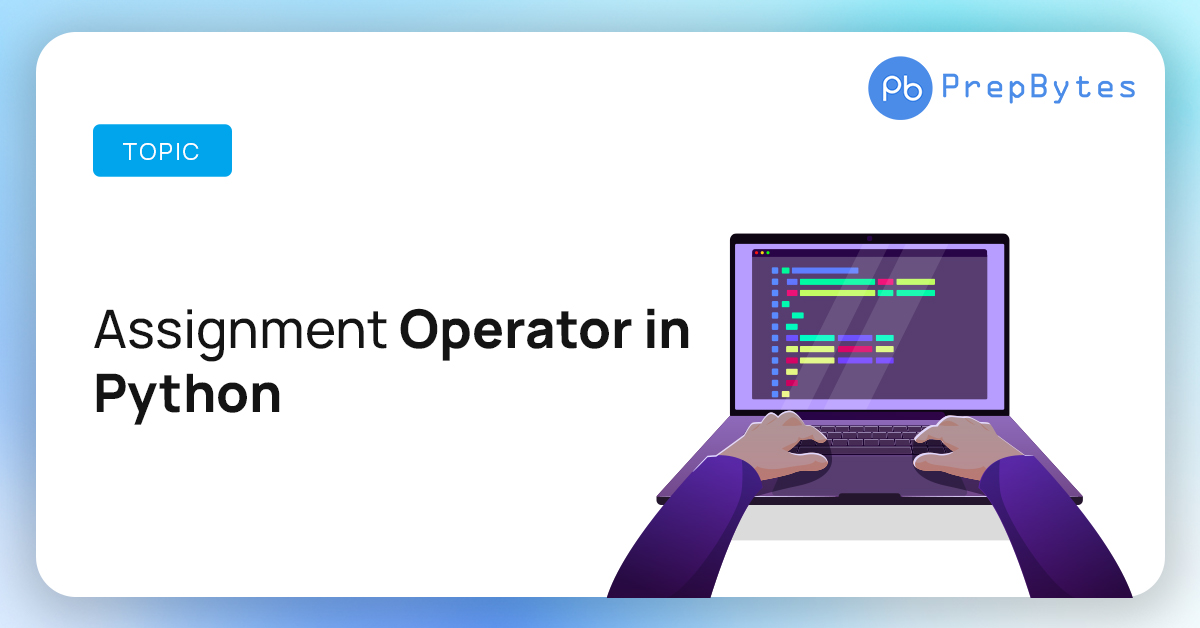
To fully comprehend the assignment operators in Python, it is important to have a basic understanding of what operators are. Operators are utilized to carry out a variety of operations, including mathematical, bitwise, and logical operations, among others, by connecting operands. Operands are the values that are acted upon by operators. In Python, the assignment operator is used to assign a value to a variable. The assignment operator is represented by the equals sign (=), and it is the most commonly used operator in Python. In this article, we will explore the assignment operator in Python, how it works, and its different types.
What is an Assignment Operator in Python?
The assignment operator in Python is used to assign a value to a variable. The assignment operator is represented by the equals sign (=), and it is used to assign a value to a variable. When an assignment operator is used, the value on the right-hand side is assigned to the variable on the left-hand side. This is a fundamental operation in programming, as it allows developers to store data in variables that can be used throughout their code.
For example, consider the following line of code:
Explanation: In this case, the value 10 is assigned to the variable a using the assignment operator. The variable a now holds the value 10, and this value can be used in other parts of the code. This simple example illustrates the basic usage and importance of assignment operators in Python programming.
Types of Assignment Operator in Python
There are several types of assignment operator in Python that are used to perform different operations. Let’s explore each type of assignment operator in Python in detail with the help of some code examples.
1. Simple Assignment Operator (=)
The simple assignment operator is the most commonly used operator in Python. It is used to assign a value to a variable. The syntax for the simple assignment operator is:
Here, the value on the right-hand side of the equals sign is assigned to the variable on the left-hand side. For example
Explanation: In this case, the value 25 is assigned to the variable a using the simple assignment operator. The variable a now holds the value 25.
2. Addition Assignment Operator (+=)
The addition assignment operator is used to add a value to a variable and store the result in the same variable. The syntax for the addition assignment operator is:
Here, the value on the right-hand side is added to the variable on the left-hand side, and the result is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is incremented by 5 using the addition assignment operator. The result, 15, is then printed to the console.
3. Subtraction Assignment Operator (-=)
The subtraction assignment operator is used to subtract a value from a variable and store the result in the same variable. The syntax for the subtraction assignment operator is
Here, the value on the right-hand side is subtracted from the variable on the left-hand side, and the result is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is decremented by 5 using the subtraction assignment operator. The result, 5, is then printed to the console.
4. Multiplication Assignment Operator (*=)
The multiplication assignment operator is used to multiply a variable by a value and store the result in the same variable. The syntax for the multiplication assignment operator is:
Here, the value on the right-hand side is multiplied by the variable on the left-hand side, and the result is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is multiplied by 5 using the multiplication assignment operator. The result, 50, is then printed to the console.
5. Division Assignment Operator (/=)
The division assignment operator is used to divide a variable by a value and store the result in the same variable. The syntax for the division assignment operator is:
Here, the variable on the left-hand side is divided by the value on the right-hand side, and the result is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is divided by 5 using the division assignment operator. The result, 2.0, is then printed to the console.
6. Modulus Assignment Operator (%=)
The modulus assignment operator is used to find the remainder of the division of a variable by a value and store the result in the same variable. The syntax for the modulus assignment operator is
Here, the variable on the left-hand side is divided by the value on the right-hand side, and the remainder is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is divided by 3 using the modulus assignment operator. The remainder, 1, is then printed to the console.
7. Floor Division Assignment Operator (//=)
The floor division assignment operator is used to divide a variable by a value and round the result down to the nearest integer, and store the result in the same variable. The syntax for the floor division assignment operator is:
Here, the variable on the left-hand side is divided by the value on the right-hand side, and the result is rounded down to the nearest integer. The rounded result is then stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is divided by 3 using the floor division assignment operator. The result, 3, is then printed to the console.
8. Exponentiation Assignment Operator (**=)
The exponentiation assignment operator is used to raise a variable to the power of a value and store the result in the same variable. The syntax for the exponentiation assignment operator is:
Here, the variable on the left-hand side is raised to the power of the value on the right-hand side, and the result is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is raised to the power of 3 using the exponentiation assignment operator. The result, 8, is then printed to the console.
9. Bitwise AND Assignment Operator (&=)
The bitwise AND assignment operator is used to perform a bitwise AND operation on the binary representation of a variable and a value, and store the result in the same variable. The syntax for the bitwise AND assignment operator is:
Here, the variable on the left-hand side is ANDed with the value on the right-hand side using the bitwise AND operator, and the result is stored back in the variable on the left-hand side. For example,
Explanation: In this case, the value of a is ANDed with 3 using the bitwise AND assignment operator. The result, 2, is then printed to the console.
10. Bitwise OR Assignment Operator (|=)
The bitwise OR assignment operator is used to perform a bitwise OR operation on the binary representation of a variable and a value, and store the result in the same variable. The syntax for the bitwise OR assignment operator is:
Here, the variable on the left-hand side is ORed with the value on the right-hand side using the bitwise OR operator, and the result is stored back in the variable on the left-hand side. For example,
Explanation: In this case, the value of a is ORed with 3 using the bitwise OR assignment operator. The result, 7, is then printed to the console.
11. Bitwise XOR Assignment Operator (^=)
The bitwise XOR assignment operator is used to perform a bitwise XOR operation on the binary representation of a variable and a value, and store the result in the same variable. The syntax for the bitwise XOR assignment operator is:
Here, the variable on the left-hand side is XORed with the value on the right-hand side using the bitwise XOR operator, and the result are stored back in the variable on the left-hand side. For example,
Explanation: In this case, the value of a is XORed with 3 using the bitwise XOR assignment operator. The result, 5, is then printed to the console.
12. Bitwise Right Shift Assignment Operator (>>=)
The bitwise right shift assignment operator is used to shift the bits of a variable to the right by a specified number of positions, and store the result in the same variable. The syntax for the bitwise right shift assignment operator is:
Here, the variable on the left-hand side has its bits shifted to the right by the number of positions specified by the value on the right-hand side, and the result is stored back in the variable on the left-hand side. For example,
Explanation: In this case, the value of a is shifted 2 positions to the right using the bitwise right shift assignment operator. The result, 2, is then printed to the console.
13. Bitwise Left Shift Assignment Operator (<<=)
The bitwise left shift assignment operator is used to shift the bits of a variable to the left by a specified number of positions, and store the result in the same variable. The syntax for the bitwise left shift assignment operator is:
Here, the variable on the left-hand side has its bits shifted to the left by the number of positions specified by the value on the right-hand side, and the result is stored back in the variable on the left-hand side. For example,
Conclusion Assignment operator in Python is used to assign values to variables, and it comes in different types. The simple assignment operator (=) assigns a value to a variable. The augmented assignment operators (+=, -=, *=, /=, %=, &=, |=, ^=, >>=, <<=) perform a specified operation and assign the result to the same variable in one step. The modulus assignment operator (%) calculates the remainder of a division operation and assigns the result to the same variable. The bitwise assignment operators (&=, |=, ^=, >>=, <<=) perform bitwise operations and assign the result to the same variable. The bitwise right shift assignment operator (>>=) shifts the bits of a variable to the right by a specified number of positions and stores the result in the same variable. The bitwise left shift assignment operator (<<=) shifts the bits of a variable to the left by a specified number of positions and stores the result in the same variable. These operators are useful in simplifying and shortening code that involves assigning and manipulating values in a single step.
Here are some Frequently Asked Questions on Assignment Operator in Python:
Q1 – Can I use the assignment operator to assign multiple values to multiple variables at once? Ans – Yes, you can use the assignment operator to assign multiple values to multiple variables at once, separated by commas. For example, "x, y, z = 1, 2, 3" would assign the value 1 to x, 2 to y, and 3 to z.
Q2 – Is it possible to chain assignment operators in Python? Ans – Yes, you can chain assignment operators in Python to perform multiple operations in one line of code. For example, "x = y = z = 1" would assign the value 1 to all three variables.
Q3 – How do I perform a conditional assignment in Python? Ans – To perform a conditional assignment in Python, you can use the ternary operator. For example, "x = a (if a > b) else b" would assign the value of a to x if a is greater than b, otherwise it would assign the value of b to x.
Q4 – What happens if I use an undefined variable in an assignment operation in Python? Ans – If you use an undefined variable in an assignment operation in Python, you will get a NameError. Make sure you have defined the variable before trying to assign a value to it.
Q5 – Can I use assignment operators with non-numeric data types in Python? Ans – Yes, you can use assignment operators with non-numeric data types in Python, such as strings or lists. For example, "my_list += [4, 5, 6]" would append the values 4, 5, and 6 to the end of the list named my_list.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Linked List
- Segment Tree
- Backtracking
- Dynamic Programming
- Greedy Algorithm
- Operating System
- Company Placement
- Interview Tips
- General Interview Questions
- Data Structure
- Other Topics
- Computational Geometry
- Game Theory
Related Post
Python list functions & python list methods, python interview questions, namespaces and scope in python, what is the difference between append and extend in python, python program to check for the perfect square, python program to find the sum of first n natural numbers.
- Practice Bitwise Algorithms
- MCQs on Bitwise Algorithms
- Tutorial on Biwise Algorithms
- Binary Representation
- Bitwise Operators
- Bit Swapping
- Bit Manipulation
- Count Set bits
- Setting a Bit
- Clear a Bit
- Toggling a Bit
- Left & Right Shift
- Checking Power of 2
- Important Tactics
- Bit Manipulation for CP
- Fast Exponentiation
- C Programming Language Tutorial
- C Language Introduction
- Features of C Programming Language
- C Programming Language Standard
- C Hello World Program
- Compiling a C Program: Behind the Scenes
- Tokens in C
- Keywords in C
C Variables and Constants
- C Variables
- Constants in C
- Const Qualifier in C
- Different ways to declare variable as constant in C
- Scope rules in C
- Internal Linkage and External Linkage in C
- Global Variables in C
C Data Types
- Data Types in C
- Literals in C
- Escape Sequence in C
- Integer Promotions in C
- Character Arithmetic in C
- Type Conversion in C
C Input/Output
- Basic Input and Output in C
- Format Specifiers in C
- printf in C
- Scansets in C
- Formatted and Unformatted Input/Output functions in C with Examples
C Operators
- Operators in C
- Arithmetic Operators in C
- Unary operators in C
- Relational Operators in C
Bitwise Operators in C
- C Logical Operators
- Assignment Operators in C
- Increment and Decrement Operators in C
- Conditional or Ternary Operator (?:) in C
- sizeof operator in C
- Operator Precedence and Associativity in C
C Control Statements Decision-Making
- Decision Making in C (if , if..else, Nested if, if-else-if )
- C - if Statement
- C if...else Statement
- C if else if ladder
- Switch Statement in C
- Using Range in switch Case in C
- while loop in C
- do...while Loop in C
- For Versus While
- Continue Statement in C
- Break Statement in C
- goto Statement in C
- C Functions
- User-Defined Function in C
- Parameter Passing Techniques in C
- Function Prototype in C
- How can I return multiple values from a function?
- main Function in C
- Implicit return type int in C
- Callbacks in C
- Nested functions in C
- Variadic functions in C
- _Noreturn function specifier in C
- Predefined Identifier __func__ in C
- C Library math.h Functions
C Arrays & Strings
- Properties of Array in C
- Multidimensional Arrays in C
- Initialization of Multidimensional Array in C
- Pass Array to Functions in C
- How to pass a 2D array as a parameter in C?
- What are the data types for which it is not possible to create an array?
- How to pass an array by value in C ?
- Strings in C
- Array of Strings in C
- What is the difference between single quoted and double quoted declaration of char array?
- C String Functions
- Pointer Arithmetics in C with Examples
- C - Pointer to Pointer (Double Pointer)
- Function Pointer in C
- How to declare a pointer to a function?
- Pointer to an Array | Array Pointer
- Difference between constant pointer, pointers to constant, and constant pointers to constants
- Pointer vs Array in C
- Dangling, Void , Null and Wild Pointers in C
- Near, Far and Huge Pointers in C
- restrict keyword in C
C User-Defined Data Types
- C Structures
- dot (.) Operator in C
- Structure Member Alignment, Padding and Data Packing
- Flexible Array Members in a structure in C
- Bit Fields in C
- Difference Between Structure and Union in C
- Anonymous Union and Structure in C
- Enumeration (or enum) in C
C Storage Classes
- Storage Classes in C
- extern Keyword in C
- Static Variables in C
- Initialization of static variables in C
- Static functions in C
- Understanding "volatile" qualifier in C | Set 2 (Examples)
- Understanding "register" keyword in C
C Memory Management
- Memory Layout of C Programs
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc()
- Difference Between malloc() and calloc() with Examples
- What is Memory Leak? How can we avoid?
- Dynamic Array in C
- How to dynamically allocate a 2D array in C?
- Dynamically Growing Array in C
C Preprocessor
- C Preprocessors
- C Preprocessor Directives
- How a Preprocessor works in C?
- Header Files in C
- What’s difference between header files "stdio.h" and "stdlib.h" ?
- How to write your own header file in C?
- Macros and its types in C
- Interesting Facts about Macros and Preprocessors in C
- # and ## Operators in C
- How to print a variable name in C?
- Multiline macros in C
- Variable length arguments for Macros
- Branch prediction macros in GCC
- typedef versus #define in C
- Difference between #define and const in C?
C File Handling
- Basics of File Handling in C
- C fopen() function with Examples
- EOF, getc() and feof() in C
- fgets() and gets() in C language
- fseek() vs rewind() in C
- What is return type of getchar(), fgetc() and getc() ?
- Read/Write Structure From/to a File in C
- C Program to print contents of file
- C program to delete a file
- C Program to merge contents of two files into a third file
- What is the difference between printf, sprintf and fprintf?
- Difference between getc(), getchar(), getch() and getche()
Miscellaneous
- time.h header file in C with Examples
- Input-output system calls in C | Create, Open, Close, Read, Write
- Signals in C language
- Program error signals
- Socket Programming in C
- _Generics Keyword in C
- Multithreading in C
C Interview Questions
- C Programming Interview Questions (2024)
- Commonly Asked C Programming Interview Questions | Set 1
- Commonly Asked C Programming Interview Questions | Set 2
- Commonly Asked C Programming Interview Questions | Set 3
In C, the following 6 operators are bitwise operators (also known as bit operators as they work at the bit-level). They are used to perform bitwise operations in C.
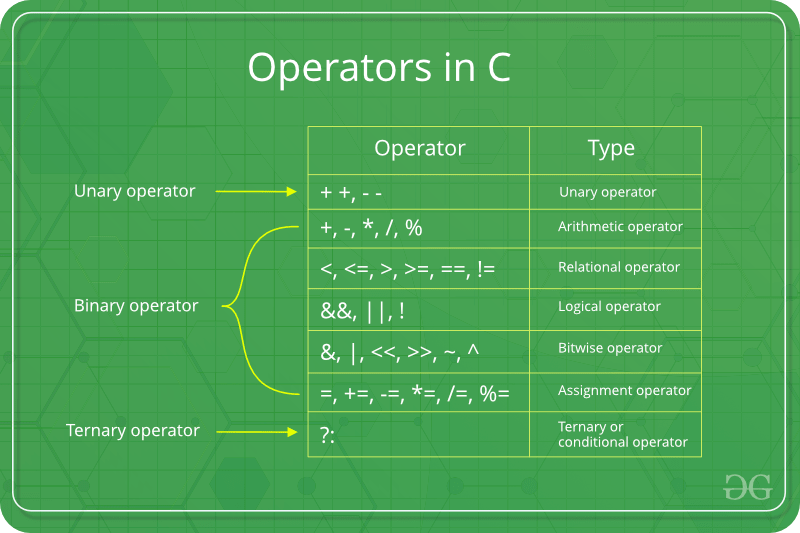
- The & (bitwise AND) in C takes two numbers as operands and does AND on every bit of two numbers. The result of AND is 1 only if both bits are 1.
- The | (bitwise OR) in C takes two numbers as operands and does OR on every bit of two numbers. The result of OR is 1 if any of the two bits is 1.
- The ^ (bitwise XOR) in C takes two numbers as operands and does XOR on every bit of two numbers. The result of XOR is 1 if the two bits are different.
- The << (left shift) in C takes two numbers, the left shifts the bits of the first operand, and the second operand decides the number of places to shift.
- The >> (right shift) in C takes two numbers, right shifts the bits of the first operand, and the second operand decides the number of places to shift.
- The ~ (bitwise NOT) in C takes one number and inverts all bits of it.
Let’s look at the truth table of the bitwise operators.
Example of Bitwise Operators in C
The following program uses bitwise operators to perform bit operations in C.
Time Complexity: O(1) Auxiliary Space: O(1)
Interesting Facts About Bitwise Operators
1. The left-shift and right-shift operators should not be used for negative numbers .
If the second operand(which decides the number of shifts) is a negative number, it results in undefined behavior in C. For example, results of both 1 <<- 1 and 1 >> -1 are undefined. Also, if the number is shifted more than the size of the integer, the behavior is undefined. For example, 1 << 33 is undefined if integers are stored using 32 bits. Another thing is NO shift operation is performed if the additive expression (operand that decides no of shifts) is 0. See this for more details.
2. The bitwise OR of two numbers is just the sum of those two numbers if there is no carry involved, otherwise, you just add their bitwise AND.
Let’s say, we have a=5(101) and b=2(010), since there is no carry involved, their sum is just a|b. Now, if we change ‘a’ to 6 which is 110 in binary, their sum would change to a|b + a&b since there is a carry involved.
3. The bitwise XOR operator is the most useful operator from a technical interview perspective.
It is used in many problems. A simple example could be “Given a set of numbers where all elements occur an even number of times except one number, find the odd occurring number” This problem can be efficiently solved by doing XOR to all numbers.
Below program demonstrates the use XOR operator to find odd occcuring elements in an array.
Time Complexity: O(n) Auxiliary Space: O(1)
The following are many other interesting problems using the XOR operator.
- Find the Missing Number
- Swap two numbers without using a temporary variable
- A Memory-Efficient Doubly Linked List
- Find the two non-repeating elements
- Find the two numbers with odd occurrences in an unsorted array
- Add two numbers without using arithmetic operators .
- Swap bits in a given number
- Count the number of bits to be flipped to convert a to b
- Find the element that appears once
- Detect if two integers have opposite signs
4. The Bitwise operators should not be used in place of logical operators.
The result of logical operators (&&, || and !) is either 0 or 1, but bitwise operators return an integer value. Also, the logical operators consider any non-zero operand as 1. For example, consider the following program, the results of & & && are different for the same operands.
The below program demonstrates the difference between & and && operators.
5. The left-shift and right-shift operators are equivalent to multiplication and division by 2 respectively.
As mentioned in point 1, it works only if numbers are positive.
The below example demonstrates the use of left-shift and right-shift operators.
6. The & operator can be used to quickly check if a number is odd or even.
The value of the expression (x & 1) would be non-zero only if x is odd, otherwise, the value would be zero.
The below example demonstrates the use bitwise & operator to find if the given number is even or odd.
7. The ~ operator should be used carefully.
The result of the ~ operator on a small number can be a big number if the result is stored in an unsigned variable. The result may be a negative number if the result is stored in a signed variable (assuming that the negative numbers are stored in 2’s complement form where the leftmost bit is the sign bit).
The below example demonstrates the use of bitwise NOT operator.
Note The output of the above program is compiler dependent
Related Articles
- Bits manipulation (Important tactics)
- Bitwise Hacks for Competitive Programming
- Bit Tricks for Competitive Programming
Please Login to comment...
Similar reads.
- Bitwise-XOR
- C-Operators
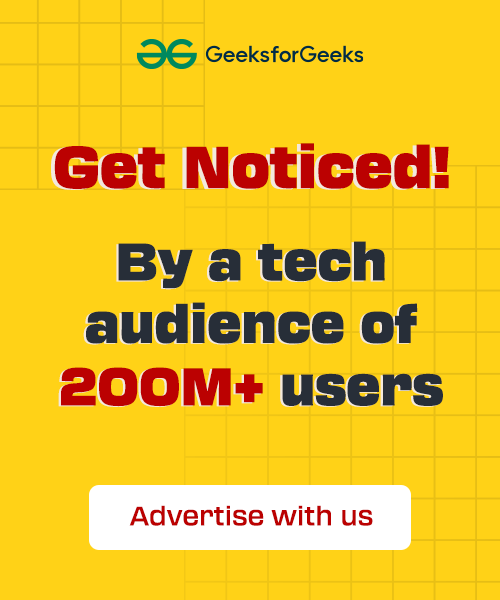
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

IMAGES
VIDEO
COMMENTS
The bitwise XOR assignment (^=) operator performs bitwise XOR on the two operands and assigns the result to the left operand. Try it. Syntax. js. x ^= y Description. x ^= y is equivalent to x = x ^ y, except that the expression x is only evaluated once. Examples. Using bitwise XOR assignment. js.
Bitwise XOR (exclusive OR) is a binary operation that takes two equal-length binary representations and performs the logical XOR operation on each pair of corresponding bits. The result in each position is 1 if only one of the two bits is 1 but will be 0 if both are 0 or both are 1. The truth table for the XOR (exclusive OR) operation is as ...
The Javascript Bitwise XOR assignment is represented by (^=). It is used to perform a bitwise XOR operation on both operands and assign the result to the left operand. Syntax: a ^= b. // a = a ^ b. Where -. a = First operand. b = Second operand.
There are two important things about these operators: 1) they guarantee short-circuit evaluation, 2) they introduce a sequence point, 3) they evaluate their operands only once. XOR evaluation, as you understand, cannot be short-circuited since the result always depends on both operands. So 1 is out of question.
In C++, Bitwise XOR Assignment Operator is used to compute the Bitwise XOR operation between left and right operands, and assign the result back to left operand. The syntax to compute bitwise XOR a value of 2 and value in variable x, and assign the result back to x using Bitwise XOR Assignment Operator is. x ^= 2.
The bitwise operators are defined for integral types and std::bitset. We'll use std::bitset in our examples because it's easier to print the output in binary. ... Similar to the arithmetic assignment operators, C++ provides bitwise assignment operators in order to facilitate easy modification of variables. Operator Symbol Form Operation ...
The bitwise XOR assignment operator ( ^=) uses the binary representation of both operands, does a bitwise XOR operation on them and assigns the result to the variable. JavaScript Demo: Expressions - Bitwise XOR assignment. 9.
Expressions and operators. This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more. A complete and detailed list of operators and expressions is also available in the reference.
An assignment operator assigns a value to its left operand based on the value of its right operand.. Overview. The basic assignment operator is equal (=), which assigns the value of its right operand to its left operand.That is, x = y assigns the value of y to x.The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
The ^ operator is overloaded for two types of operands: number and BigInt.For numbers, the operator returns a 32-bit integer. For BigInts, the operator returns a BigInt. It first coerces both operands to numeric values and tests the types of them. It performs BigInt XOR if both operands become BigInts; otherwise, it converts both operands to 32-bit integers and performs number bitwise XOR.
The Bitwise XOR and assignment operator (^=) assigns the first operand a value equal to the result of Bitwise XOR operation of two operands. (x ^= y) is equivalent to (x = x ^ y) The Bitwise XOR operator (^) is a binary operator which takes two bit patterns of equal length and performs the logical exclusive OR operation on each pair of ...
In Java, Bitwise XOR Assignment Operator is used to compute the Bitwise XOR operation between left and right operands, and assign the result back to left operand. In this tutorial, we will learn how to use Bitwise XOR Assignment operator in Java, with examples. The syntax to compute bitwise XOR a value of 2 and value in variable x, and assign ...
In C++, the addition assignment operator (+=) combines the addition operation with the variable assignment allowing you to increment the value of variable by a specified expression in a concise and efficient way. Syntax. variable += value; This above expression is equivalent to the expression: variable = variable + value; Example.
For the complete list of C# operators ordered by precedence level, see the Operator precedence section of the C# operators article. Operator overloadability. A user-defined type can overload the !, &, |, and ^ operators. When a binary operator is overloaded, the corresponding compound assignment operator is also implicitly overloaded.
Unsigned right-shift operator >>> Available in C# 11 and later, the >>> operator shifts its left-hand operand right by the number of bits defined by its right-hand operand. For information about how the right-hand operand defines the shift count, see the Shift count of the shift operators section.. The >>> operator always performs a logical shift. That is, the high-order empty bit positions ...
The basic assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x. The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples. Name. Shorthand operator.
In JavaScript, the bitwise XOR (^) Operator is used to compare two operands and return a new binary number which is 1 if both the bits in operators are different and 0 if both the bits in operads are the same. The operation is represented by the "|" symbol. This operator can be used to find the missing numbers in an array of natural numbers.
The bitwise XOR assignment operator is used to perform a bitwise XOR operation on the binary representation of a variable and a value, and store the result in the same variable. The syntax for the bitwise XOR assignment operator is: variable ^= value.
Bitwise Operators in C. In C, the following 6 operators are bitwise operators (also known as bit operators as they work at the bit-level). They are used to perform bitwise operations in C. The & (bitwise AND) in C takes two numbers as operands and does AND on every bit of two numbers. The result of AND is 1 only if both bits are 1.