If...Else Statement in C Explained
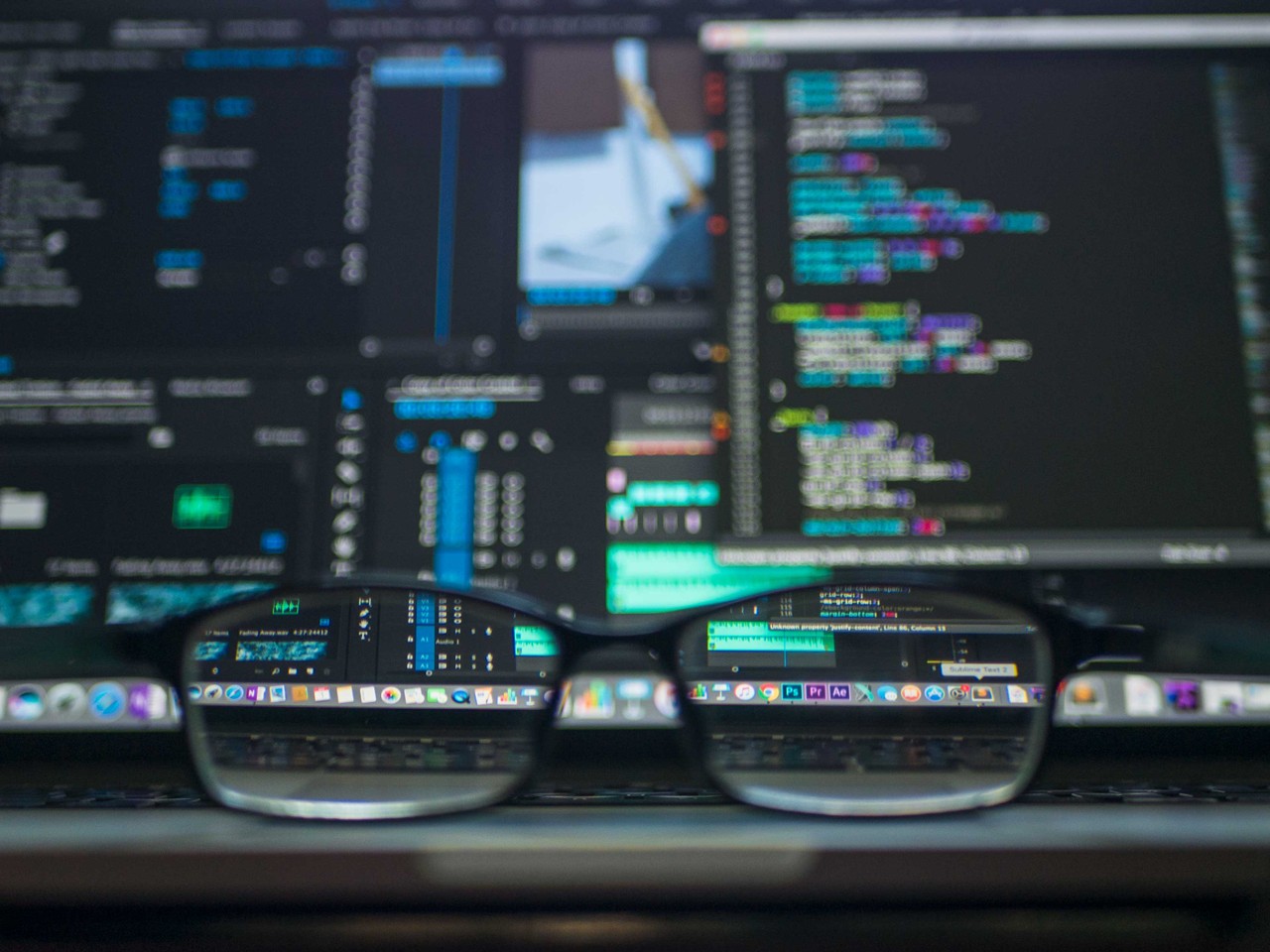
Conditional code flow is the ability to change the way a piece of code behaves based on certain conditions. In such situations you can use if statements.
The if statement is also known as a decision making statement, as it makes a decision on the basis of a given condition or expression. The block of code inside the if statement is executed is the condition evaluates to true. However, the code inside the curly braces is skipped if the condition evaluates to false, and the code after the if statement is executed.

Syntax of an if statement
A simple example.
Let’s look at an example of this in action:
If the code inside parenthesis of the if statement is true, everything within the curly braces is executed. In this case, true evaluates to true, so the code runs the printf function.
if..else statements
In an if...else statement, if the code in the parenthesis of the if statement is true, the code inside its brackets is executed. But if the statement inside the parenthesis is false, all the code within the else statement's brackets is executed instead.
Of course, the example above isn't very useful in this case because true always evaluates to true. Here's another that's a bit more practical:
There are a few important differences here. First, stdbool.h hasn’t been included. That's okay because true and false aren't being used like in the first example. In C, like in other programming languages, you can use statements that evaluate to true or false rather than using the boolean values true or false directly.
Also notice the condition in the parenthesis of the if statement: n == 3 . This condition compares n and the number 3. == is the comparison operator, and is one of several comparison operations in C.
Nested if...else
The if...else statement allows a choice to be made between two possibilities. But sometimes you need to choose between three or more possibilities.
For example the sign function in mathematics returns -1 if the argument is less than zero, +1 if the argument is greater than zero, and returns zero if the argument is zero.
The following code implements this function:
As you can see, a second if...else statement is nested within else statement of the first if..else .
If x is less than 0, then sign is set to -1. However, if x is not less than 0, the second if...else statement is executed. There, if x is equal to 0, sign is also set to 0. But if x is greater than 0, sign is instead set to 1.
Rather than a nested if...else statement, beginners often use a string of if statements:
While this works, it's not recommended since it's unclear that only one of the assignment statements ( sign = ... ) is meant to be executed depending on the value of x . It's also inefficient – every time the code runs, all three conditions are tested, even if one or two don't have to be.
else...if statements
if...else statements are an alternative to a string of if statements. Consider the following:
If the condition for the if statement evaluates to false, the condition for the else...if statement is checked. If that condition evaluates to true, the code inside the else...if statement's curly braces is run.
Comparison Operators
Logical operators.
We might want a bit of code to run if something is not true, or if two things are true. For that we have logical operators:
For example:
An important note about C comparisons
While we mentioned earlier that each comparison is checking if something is true or false, but that's only half true. C is very light and close to the hardware it's running on. With hardware it's easy to check if something is 0 or false, but anything else is much more difficult.
Instead it's much more accurate to say that the comparisons are really checking if something is 0 / false, or if it is any other value.
For example, his if statement is true and valid:
By design, 0 is false, and by convention, 1 is true. In fact, here’s a look at the stdbool.h library:
While there's a bit more to it, this is the core of how booleans work and how the library operates. These two lines instruct the compiler to replace the word false with 0, and true with 1.
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Next: Execution Control Expressions , Previous: Arithmetic , Up: Top [ Contents ][ Index ]
7 Assignment Expressions
As a general concept in programming, an assignment is a construct that stores a new value into a place where values can be stored—for instance, in a variable. Such places are called lvalues (see Lvalues ) because they are locations that hold a value.
An assignment in C is an expression because it has a value; we call it an assignment expression . A simple assignment looks like
We say it assigns the value of the expression value-to-store to the location lvalue , or that it stores value-to-store there. You can think of the “l” in “lvalue” as standing for “left,” since that’s what you put on the left side of the assignment operator.
However, that’s not the only way to use an lvalue, and not all lvalues can be assigned to. To use the lvalue in the left side of an assignment, it has to be modifiable . In C, that means it was not declared with the type qualifier const (see const ).
The value of the assignment expression is that of lvalue after the new value is stored in it. This means you can use an assignment inside other expressions. Assignment operators are right-associative so that
is equivalent to
This is the only useful way for them to associate; the other way,
would be invalid since an assignment expression such as x = y is not valid as an lvalue.
Warning: Write parentheses around an assignment if you nest it inside another expression, unless that is a conditional expression, or comma-separated series, or another assignment.

C Conditional Statement: IF, IF Else and Nested IF Else with Example

What is a Conditional Statement in C?
Conditional Statements in C programming are used to make decisions based on the conditions. Conditional statements execute sequentially when there is no condition around the statements. If you put some condition for a block of statements, the execution flow may change based on the result evaluated by the condition. This process is called decision making in ‘C.’
In ‘C’ programming conditional statements are possible with the help of the following two constructs:
1. If statement
2. If-else statement
It is also called as branching as a program decides which statement to execute based on the result of the evaluated condition.
If statement
The condition evaluates to either true or false. True is always a non-zero value, and false is a value that contains zero. Instructions can be a single instruction or a code block enclosed by curly braces { }.
Following program illustrates the use of if construct in ‘C’ programming:
The above program illustrates the use of if construct to check equality of two numbers.
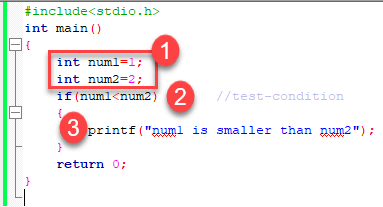
- In the above program, we have initialized two variables with num1, num2 with value as 1, 2 respectively.
- Then, we have used if with a test-expression to check which number is the smallest and which number is the largest. We have used a relational expression in if construct. Since the value of num1 is smaller than num2, the condition will evaluate to true.
- Thus it will print the statement inside the block of If. After that, the control will go outside of the block and program will be terminated with a successful result.
Relational Operators
C has six relational operators that can be used to formulate a Boolean expression for making a decision and testing conditions, which returns true or false :
< less than
<= less than or equal to
> greater than
>= greater than or equal to
== equal to
!= not equal to
Notice that the equal test (==) is different from the assignment operator (=) because it is one of the most common problems that a programmer faces by mixing them up.
For example:
Keep in mind that a condition that evaluates to a non-zero value is considered as true.
The If-Else statement
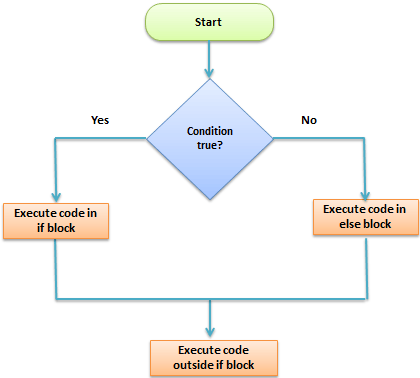
The if-else is statement is an extended version of If. The general form of if-else is as follows:
n this type of a construct, if the value of test-expression is true, then the true block of statements will be executed. If the value of test-expression if false, then the false block of statements will be executed. In any case, after the execution, the control will be automatically transferred to the statements appearing outside the block of If.
Let’s start.
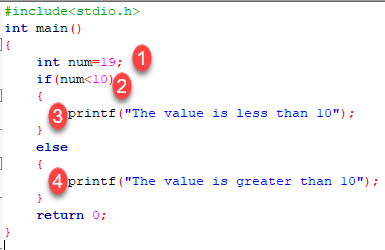
- We have initialized a variable with value 19. We have to find out whether the number is bigger or smaller than 10 using a ‘C’ program. To do this, we have used the if-else construct.
- Here we have provided a condition num<10 because we have to compare our value with 10.
- As you can see the first block is always a true block which means, if the value of test-expression is true then the first block which is If, will be executed.
- The second block is an else block. This block contains the statements which will be executed if the value of the test-expression becomes false. In our program, the value of num is greater than ten hence the test-condition becomes false and else block is executed. Thus, our output will be from an else block which is “The value is greater than 10”. After the if-else, the program will terminate with a successful result.
In ‘C’ programming we can use multiple if-else constructs within each other which are referred to as nesting of if-else statements.
Conditional Expressions
There is another way to express an if-else statement is by introducing the ?: operator. In a conditional expression the ?: operator has only one statement associated with the if and the else.
Nested If-else Statements
When a series of decision is required, nested if-else is used. Nesting means using one if-else construct within another one.
Let’s write a program to illustrate the use of nested if-else.
The above program checks if a number is less or greater than 10 and prints the result using nested if-else construct.
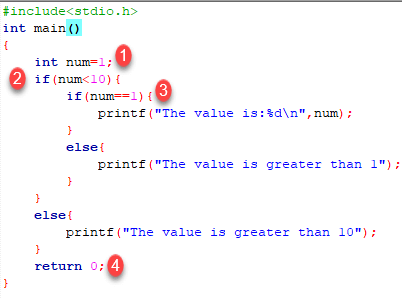
- Firstly, we have declared a variable num with value as 1. Then we have used if-else construct.
- In the outer if-else, the condition provided checks if a number is less than 10. If the condition is true then and only then it will execute the inner loop . In this case, the condition is true hence the inner block is processed.
- In the inner block, we again have a condition that checks if our variable contains the value 1 or not. When a condition is true, then it will process the If block otherwise it will process an else block. In this case, the condition is true hence the If a block is executed and the value is printed on the output screen.
- The above program will print the value of a variable and exit with success.
Try changing the value of variable see how the program behaves.
NOTE: In nested if-else, we have to be careful with the indentation because multiple if-else constructs are involved in this process, so it becomes difficult to figure out individual constructs. Proper indentation makes it easy to read the program.
Nested Else-if statements
Nested else-if is used when multipath decisions are required.
The general syntax of how else-if ladders are constructed in ‘C’ programming is as follows:
This type of structure is known as the else-if ladder. This chain generally looks like a ladder hence it is also called as an else-if ladder. The test-expressions are evaluated from top to bottom. Whenever a true test-expression if found, statement associated with it is executed. When all the n test-expressions becomes false, then the default else statement is executed.
Let us see the actual working with the help of a program.
The above program prints the grade as per the marks scored in a test. We have used the else-if ladder construct in the above program.
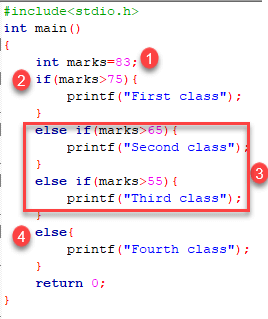
- We have initialized a variable with marks. In the else-if ladder structure, we have provided various conditions.
- The value from the variable marks will be compared with the first condition since it is true the statement associated with it will be printed on the output screen.
- If the first test condition turns out false, then it is compared with the second condition.
- This process will go on until the all expression is evaluated otherwise control will go out of the else-if ladder, and default statement will be printed.
Try modifying the value and notice the change in the output.
- Decision making or branching statements are used to select one path based on the result of the evaluated expression.
- It is also called as control statements because it controls the flow of execution of a program.
- ‘C’ provides if, if-else constructs for decision-making statements.
- We can also nest if-else within one another when multiple paths have to be tested.
- The else-if ladder is used when we have to check various ways based upon the result of the expression.
- Dynamic Memory Allocation in C using malloc(), calloc() Functions
- Type Casting in C: Type Conversion, Implicit, Explicit with Example
- C Programming Tutorial PDF for Beginners
- 13 BEST C Programming Books for Beginners (2024 Update)
- Difference Between C and Java
- Difference Between Structure and Union in C
- Top 100 C Programming Interview Questions and Answers (PDF)
- calloc() Function in C Library with Program EXAMPLE
cppreference.com
Assignment operators.
Assignment and compound assignment operators are binary operators that modify the variable to their left using the value to their right.
[ edit ] Simple assignment
The simple assignment operator expressions have the form
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs .
Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non-lvalue (so that expressions such as ( a = b ) = c are invalid).
rhs and lhs must satisfy one of the following:
- both lhs and rhs have compatible struct or union type, or..
- rhs must be implicitly convertible to lhs , which implies
- both lhs and rhs have arithmetic types , in which case lhs may be volatile -qualified or atomic (since C11)
- both lhs and rhs have pointer to compatible (ignoring qualifiers) types, or one of the pointers is a pointer to void, and the conversion would not add qualifiers to the pointed-to type. lhs may be volatile or restrict (since C99) -qualified or atomic (since C11) .
- lhs is a (possibly qualified or atomic (since C11) ) pointer and rhs is a null pointer constant such as NULL or a nullptr_t value (since C23)
[ edit ] Notes
If rhs and lhs overlap in memory (e.g. they are members of the same union), the behavior is undefined unless the overlap is exact and the types are compatible .
Although arrays are not assignable, an array wrapped in a struct is assignable to another object of the same (or compatible) struct type.
The side effect of updating lhs is sequenced after the value computations, but not the side effects of lhs and rhs themselves and the evaluations of the operands are, as usual, unsequenced relative to each other (so the expressions such as i = ++ i ; are undefined)
Assignment strips extra range and precision from floating-point expressions (see FLT_EVAL_METHOD ).
In C++, assignment operators are lvalue expressions, not so in C.
[ edit ] Compound assignment
The compound assignment operator expressions have the form
The expression lhs @= rhs is exactly the same as lhs = lhs @ ( rhs ) , except that lhs is evaluated only once.
[ edit ] References
- C17 standard (ISO/IEC 9899:2018):
- 6.5.16 Assignment operators (p: 72-73)
- C11 standard (ISO/IEC 9899:2011):
- 6.5.16 Assignment operators (p: 101-104)
- C99 standard (ISO/IEC 9899:1999):
- 6.5.16 Assignment operators (p: 91-93)
- C89/C90 standard (ISO/IEC 9899:1990):
- 3.3.16 Assignment operators
[ edit ] See Also
Operator precedence
[ edit ] See also
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 19 August 2022, at 09:36.
- This page has been accessed 58,085 times.
- Privacy policy
- About cppreference.com
- Disclaimers


- C Programming Tutorial
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Nested Structures
- C - Structure Padding and Packing
- C - Anonymous Structure and Union
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Assignment Operators in C
In C language, the assignment operator stores a certain value in an already declared variable. A variable in C can be assigned the value in the form of a literal, another variable, or an expression.
The value to be assigned forms the right-hand operand, whereas the variable to be assigned should be the operand to the left of the " = " symbol, which is defined as a simple assignment operator in C.
In addition, C has several augmented assignment operators.
The following table lists the assignment operators supported by the C language −
Simple Assignment Operator (=)
The = operator is one of the most frequently used operators in C. As per the ANSI C standard, all the variables must be declared in the beginning. Variable declaration after the first processing statement is not allowed.
You can declare a variable to be assigned a value later in the code, or you can initialize it at the time of declaration.
You can use a literal, another variable, or an expression in the assignment statement.
Once a variable of a certain type is declared, it cannot be assigned a value of any other type. In such a case the C compiler reports a type mismatch error.
In C, the expressions that refer to a memory location are called "lvalue" expressions. A lvalue may appear as either the left-hand or right-hand side of an assignment.
On the other hand, the term rvalue refers to a data value that is stored at some address in memory. A rvalue is an expression that cannot have a value assigned to it which means an rvalue may appear on the right-hand side but not on the left-hand side of an assignment.
Variables are lvalues and so they may appear on the left-hand side of an assignment. Numeric literals are rvalues and so they may not be assigned and cannot appear on the left-hand side. Take a look at the following valid and invalid statements −
Augmented Assignment Operators
In addition to the = operator, C allows you to combine arithmetic and bitwise operators with the = symbol to form augmented or compound assignment operator. The augmented operators offer a convenient shortcut for combining arithmetic or bitwise operation with assignment.
For example, the expression "a += b" has the same effect of performing "a + b" first and then assigning the result back to the variable "a".
Run the code and check its output −
Similarly, the expression "a <<= b" has the same effect of performing "a << b" first and then assigning the result back to the variable "a".
Here is a C program that demonstrates the use of assignment operators in C −
When you compile and execute the above program, it will produce the following result −
To Continue Learning Please Login
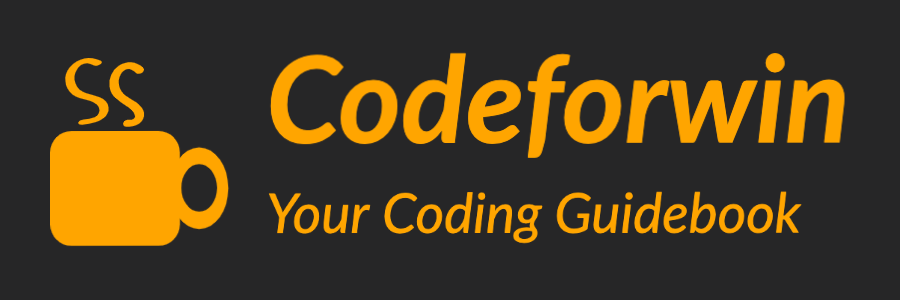
If else programming exercises and solutions in C
if...else is a branching statement . It is used to take an action based on some condition. For example – if user inputs valid account number and pin, then allow money withdrawal.
If statement works like “If condition is met, then execute the task” . It is used to compare things and take some action based on the comparison. Relational and logical operators supports this comparison.
C language supports three variants of if statement.
- Simple if statement
- if…else and if…else…if statement
- Nested if…else statement
As a programmer you must have a good control on program execution flow. In this exercise we will focus to control program flow using if...else statements.
Always feel free to drop your queries and suggestions below in the comments section . I will try to get back to you asap.
Required knowledge
Basic C programming , Relational operators , Logical operators
List of if...else programming exercises
- Write a C program to find maximum between two numbers.
- Write a C program to find maximum between three numbers.
- Write a C program to check whether a number is negative, positive or zero.
- Write a C program to check whether a number is divisible by 5 and 11 or not.
- Write a C program to check whether a number is even or odd.
- Write a C program to check whether a year is leap year or not.
- Write a C program to check whether a character is alphabet or not.
- Write a C program to input any alphabet and check whether it is vowel or consonant.
- Write a C program to input any character and check whether it is alphabet, digit or special character.
- Write a C program to check whether a character is uppercase or lowercase alphabet .
- Write a C program to input week number and print week day .
- Write a C program to input month number and print number of days in that month.
- Write a C program to count total number of notes in given amount .
- Write a C program to input angles of a triangle and check whether triangle is valid or not.
- Write a C program to input all sides of a triangle and check whether triangle is valid or not.
- Write a C program to check whether the triangle is equilateral, isosceles or scalene triangle.
- Write a C program to find all roots of a quadratic equation .
- Write a C program to calculate profit or loss.
- Write a C program to input marks of five subjects Physics, Chemistry, Biology, Mathematics and Computer. Calculate percentage and grade according to following: Percentage >= 90% : Grade A Percentage >= 80% : Grade B Percentage >= 70% : Grade C Percentage >= 60% : Grade D Percentage >= 40% : Grade E Percentage < 40% : Grade F
- Write a C program to input basic salary of an employee and calculate its Gross salary according to following: Basic Salary <= 10000 : HRA = 20%, DA = 80% Basic Salary <= 20000 : HRA = 25%, DA = 90% Basic Salary > 20000 : HRA = 30%, DA = 95%
- Write a C program to input electricity unit charges and calculate total electricity bill according to the given condition: For first 50 units Rs. 0.50/unit For next 100 units Rs. 0.75/unit For next 100 units Rs. 1.20/unit For unit above 250 Rs. 1.50/unit An additional surcharge of 20% is added to the bill
Learn C++ practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c++ interactively, introduction to c++.
- Getting Started With C++
- Your First C++ Program
- C++ Comments
C++ Fundamentals
- C++ Keywords and Identifiers
- C++ Variables, Literals and Constants
- C++ Data Types
- C++ Type Modifiers
- C++ Constants
- C++ Basic Input/Output
- C++ Operators
Flow Control
- C++ Relational and Logical Operators
C++ if, if...else and Nested if...else
- C++ for Loop
C++ while and do...while Loop
C++ break Statement
C++ continue Statement
- C++ goto Statement
- C++ switch..case Statement
C++ Ternary Operator
- C++ Functions
- C++ Programming Default Arguments
- C++ Function Overloading
- C++ Inline Functions
- C++ Recursion
Arrays and Strings
- C++ Array to Function
- C++ Multidimensional Arrays
- C++ String Class
Pointers and References
- C++ Pointers
- C++ Pointers and Arrays
- C++ References: Using Pointers
- C++ Call by Reference: Using pointers
- C++ Memory Management: new and delete
Structures and Enumerations
- C++ Structures
- C++ Structure and Function
- C++ Pointers to Structure
- C++ Enumeration
Object Oriented Programming
- C++ Classes and Objects
- C++ Constructors
- C++ Constructor Overloading
- C++ Destructors
- C++ Access Modifiers
- C++ Encapsulation
- C++ friend Function and friend Classes
Inheritance & Polymorphism
- C++ Inheritance
- C++ Public, Protected and Private Inheritance
- C++ Multiple, Multilevel and Hierarchical Inheritance
- C++ Function Overriding
- C++ Virtual Functions
- C++ Abstract Class and Pure Virtual Function
STL - Vector, Queue & Stack
- C++ Standard Template Library
- C++ STL Containers
- C++ std::array
- C++ Vectors
- C++ Forward List
- C++ Priority Queue
STL - Map & Set
- C++ Multimap
- C++ Multiset
- C++ Unordered Map
- C++ Unordered Set
- C++ Unordered Multiset
- C++ Unordered Multimap
STL - Iterators & Algorithms
- C++ Iterators
- C++ Algorithm
- C++ Functor

Additional Topics
- C++ Exceptions Handling
- C++ File Handling
- C++ Ranged for Loop
- C++ Nested Loop
- C++ Function Template
- C++ Class Templates
- C++ Type Conversion
- C++ Type Conversion Operators
- C++ Operator Overloading
Advanced Topics
- C++ Namespaces
- C++ Preprocessors and Macros
- C++ Storage Class
- C++ Bitwise Operators
- C++ Buffers
- C++ istream
- C++ ostream
C++ Tutorials
- Display Factors of a Number
In computer programming, we use the if...else statement to run one block of code under certain conditions and another block of code under different conditions.
For example, assigning grades (A, B, C) based on marks obtained by a student.
- if the percentage is above 90 , assign grade A
- if the percentage is above 75 , assign grade B
- if the percentage is above 65 , assign grade C
- C++ if Statement
The if statement evaluates the condition inside the parentheses ( ) .
- If the condition evaluates to true , the code inside the body of if is executed.
- If the condition evaluates to false , the code inside the body of if is skipped.
Note: The code inside { } is the body of the if statement.
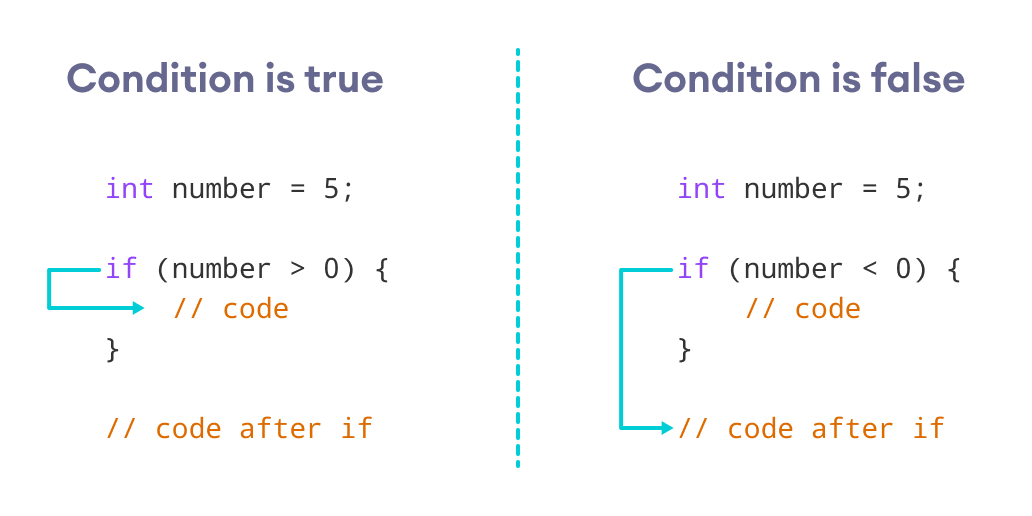
Example 1: C++ if Statement
When the user enters 5 , the condition number > 0 is evaluated to true and the statement inside the body of if is executed.
When the user enters -5 , the condition number > 0 is evaluated to false and the statement inside the body of if is not executed.
- C++ if...else
The if statement can have an optional else clause.
The if..else statement evaluates the condition inside the parenthesis.
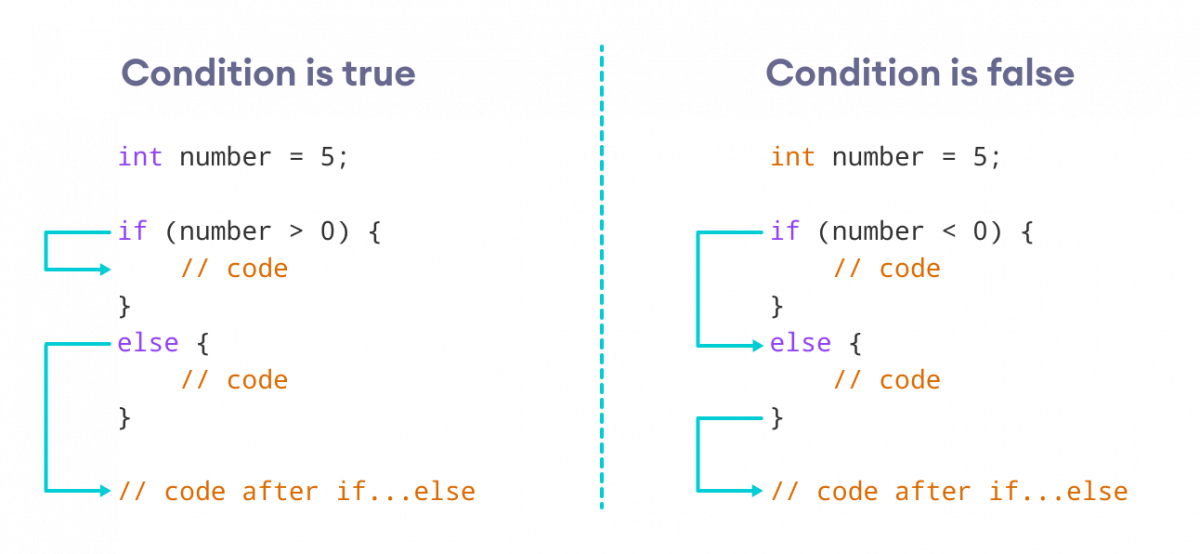
If the condition evaluates true ,
- the code inside the body of if is executed
- the code inside the body of else is skipped from execution
If the condition evaluates false ,
- the code inside the body of else is executed
- the code inside the body of if is skipped from execution
Example 2: C++ if...else Statement
In the above program, we have the condition number >= 0 . If we enter the number greater or equal to 0 , then the condition evaluates true .
Here, we enter 4 . So, the condition is true . Hence, the statement inside the body of if is executed.
Here, we enter -4 . So, the condition is false . Hence, the statement inside the body of else is executed.
- C++ if...else...else if statement
The if...else statement is used to execute a block of code among two alternatives. However, if we need to make a choice between more than two alternatives, we use the if...else if...else statement.
- If condition1 evaluates to true , the code block 1 is executed.
- If condition1 evaluates to false , then condition2 is evaluated.
- If condition2 is true , the code block 2 is executed.
- If condition2 is false , the code block 3 is executed.
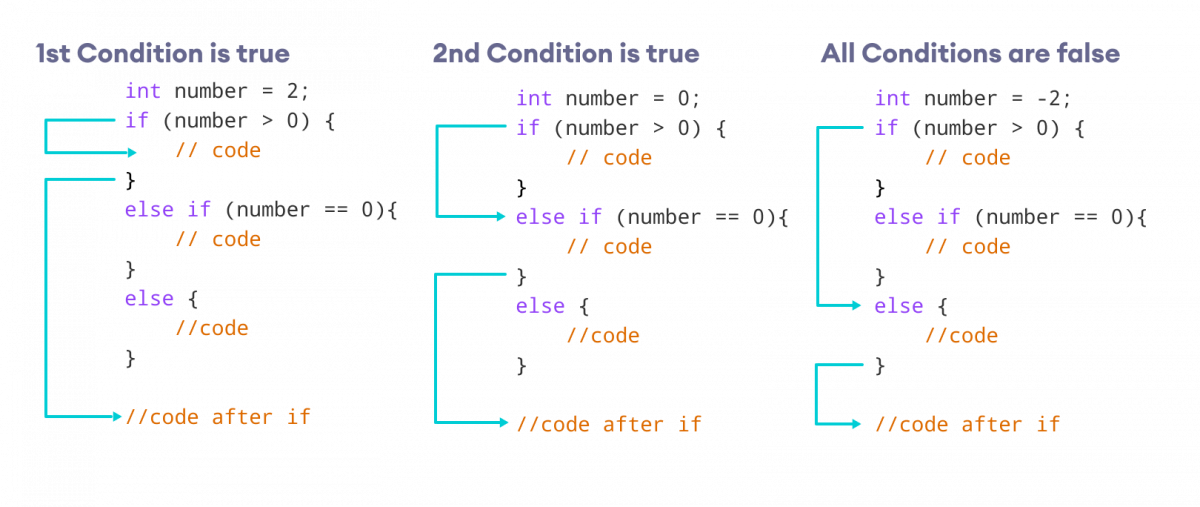
Note: There can be more than one else if statement but only one if and else statements.
Example 3: C++ if...else...else if
In this program, we take a number from the user. We then use the if...else if...else ladder to check whether the number is positive, negative, or zero.
If the number is greater than 0 , the code inside the if block is executed. If the number is less than 0 , the code inside the else if block is executed. Otherwise, the code inside the else block is executed.
- C++ Nested if...else
Sometimes, we need to use an if statement inside another if statement. This is known as nested if statement.
Think of it as multiple layers of if statements. There is a first, outer if statement, and inside it is another, inner if statement.
- We can add else and else if statements to the inner if statement as required.
- The inner if statement can also be inserted inside the outer else or else if statements (if they exist).
- We can nest multiple layers of if statements.
Example 4: C++ Nested if
In the above example,
- We take an integer as an input from the user and store it in the variable num .
- If true , then the inner if...else statement is executed.
- If false , the code inside the outer else condition is executed, which prints "The number is 0 and it is neither positive nor negative."
- If true , then we print a statement saying that the number is positive.
- If false , we print that the number is negative.
Note: As you can see, nested if...else makes your logic complicated. If possible, you should always try to avoid nested if...else .
Body of if...else With Only One Statement
If the body of if...else has only one statement, you can omit { } in the program. For example, you can replace
The output of both programs will be the same.
Note: Although it's not necessary to use { } if the body of if...else has only one statement, using { } makes your code more readable.
- More on Decision Making
The ternary operator is a concise, inline method used to execute one of two expressions based on a condition. To learn more, visit C++ Ternary Operator .
If we need to make a choice between more than one alternatives based on a given test condition, the switch statement can be used. To learn more, visit C++ switch .
- C++ Program to Check Whether Number is Even or Odd
- C++ Program to Check Whether a Character is Vowel or Consonant.
- C++ Program to Find Largest Number Among Three Numbers
Table of Contents
- Introduction
Sorry about that.
Related Tutorials
C++ Tutorial

- C All Exercises & Assignments
Write a C program to check whether a number is even or odd
Description:
Write a C program to check whether a number is even or odd.
Note: Even number is divided by 2 and give the remainder 0 but odd number is not divisible by 2 for eg. 4 is divisible by 2 and 9 is not divisible by 2.
Conditions:
- Create a variable with name of number.
- Take value from user for number variable.
Enter the Number=9 Number is Odd.
Write a C program to swap value of two variables using the third variable.
You need to create a C program to swap values of two variables using the third variable.
You can use a temp variable as a blank variable to swap the value of x and y.
- Take three variables for eg. x, y and temp.
- Swap the value of x and y variable.
Write a C program to check whether a user is eligible to vote or not.
You need to create a C program to check whether a user is eligible to vote or not.
- Minimum age required for voting is 18.
- You can use decision making statement.
Enter your age=28 User is eligible to vote
Write a C program to check whether an alphabet is Vowel or Consonant
You need to create a C program to check whether an alphabet is Vowel or Consonant.
- Create a character type variable with name of alphabet and take the value from the user.
- You can use conditional statements.
Enter an alphabet: O O is a vowel.
Write a C program to find the maximum number between three numbers
You need to write a C program to find the maximum number between three numbers.
- Create three variables in c with name of number1, number2 and number3
- Find out the maximum number using the nested if-else statement
Enter three numbers: 10 20 30 Number3 is max with value of 30
Write a C program to check whether number is positive, negative or zero
You need to write a C program to check whether number is positive, negative or zero
- Create variable with name of number and the value will taken by user or console
- Create this c program code using else if ladder statement
Enter a number : 10 10 is positive
Write a C program to calculate Electricity bill.
You need to write a C program to calculate electricity bill using if-else statements.
- For first 50 units – Rs. 3.50/unit
- For next 100 units – Rs. 4.00/unit
- For next 100 units – Rs. 5.20/unit
- For units above 250 – Rs. 6.50/unit
- You can use conditional statements.
Enter the units consumed=278.90 Electricity Bill=1282.84 Rupees
Write a C program to print 1 to 10 numbers using the while loop
You need to create a C program to print 1 to 10 numbers using the while loop
- Create a variable for the loop iteration
- Use increment operator in while loop
1 2 3 4 5 6 7 8 9 10
- C Exercises Categories
- C Top Exercises
- C Decision Making
- SI SWIMSUIT
- SI SPORTSBOOK
Atlanta Braves Designate Infielder Joey Wendle For Assignment 3 Days After Signing Him
Sam connon | 2 hours ago.
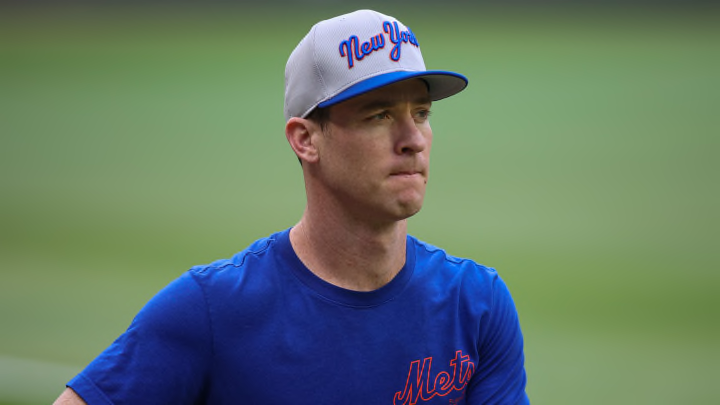
- Atlanta Braves
- New York Mets
- Tampa Bay Rays
- Miami Marlins
The Atlanta Braves have designated veteran infielder Joey Wendle for assignment, the team announced Monday.
The move was made as part of a major roster reshuffling that sent reigning NL MVP Ronald Acuña Jr. to the 10-day injured list with a torn left ACL. Atlanta called up JP Martínez to take Acuña's place on the outfield depth chart, while also reinstating All-Star catcher Sean Murphy from the injured list.
That left no room for Wendle on the 40-man roster. As a result, he got booted before ever appearing in a game for the Braves.
Atlanta signed Wendle to a one-year deal on Friday, just a few days after he had been released by the division rival New York Mets . New York designated Wendle for assignment on May 15.
Wendle was batting .222 with one double, one RBI, one stolen base, a .493 OPS and a -0.1 WAR on the season, only earning 10 starts in his 18 appearances.
The #Braves today returned C Sean Murphy from his rehabilitation assignment and reinstated him from the injured list, and placed OF Ronald Acuña Jr. on the 10-day IL with a torn left ACL. Additionally, the club recalled OF J.P. Martínez to Atlanta and designated INF Joey Wendle… — Atlanta Braves (@Braves) May 27, 2024
The Mets signed Wendle to a one-year, $2 million contract in November.
Before landing in Queens, Wendle enjoyed a moderately successful career elsewhere.
The Oakland Athletics traded Wendle to the Tampa Bay Rays in December 2017. He immediately became an everyday player for the Rays, batting .300 with a .789 OPS and 4.9 WAR, which helped him place fourth in AL Rookie of the Year voting.
Wendle missed half of 2019 with hamstring and wrist injuries, then put up solid numbers again in the COVID-shortened 2020 season. The utility infielder took a major leap in 2021, making his first All-Star Game and posting a 3.6 WAR.
In November 2021, the Rays flipped Wendle to the Miami Marlins . Wendle racked up a 2.4 WAR in just 101 appearances in 2022, but he saw his WAR drop to -0.2 in 112 games the following year.
Wendle looked more like his 2023 self than his 2018, 2021 or 2022 self in the early goings of 2024, which explains why he fell out of favor with the Mets and why the Braves hardly gave him a chance. Now, the 34-year-old will either hit the waiver wire, accept a minor league assignment or re-enter free agency.
Follow Fastball on FanNation on social media
Continue to follow our Fastball on FanNation coverage on social media by liking us on Facebook and by following us on Twitter @FastballFN .
You can also follow Sam Connon on Twitter @SamConnon .
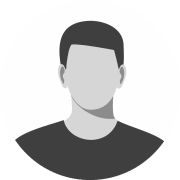
Sam Connon is a Staff Writer for Fastball on the Sports Illustrated/FanNation networks. He previously covered UCLA Athletics for Sports Illustrated/FanNation's All Bruins, 247Sports' Bruin Report Online, Rivals' Bruin Blitz, the Bleav Podcast Network and the Daily Bruin, with his work as a sports columnist receiving awards from the College Media Association and Society of Professional Journalists. Connon also wrote for Sports Illustrated/FanNation's New England Patriots site, Patriots Country, and he was on the Patriots and Boston Red Sox beats at Prime Time Sports Talk.
Follow SamConnon
Detroit Tigers reliever Shelby Miller gears up for rehab assignment with Triple-A Toledo

Detroit Tigers reliever Shelby Miller believes he has dodged major injury.
Miller, a veteran right-hander, expects to begin a rehab assignment Tuesday with Triple-A Toledo. The 33-year-old has recovered from right ulnar nerve inflammation, which required a hydrodissection procedure. Miller has thrown three bullpens in his build-up process, including his latest Sunday at Comerica Park.
"Just getting some strength around the nerve and breaking up the scar tissue," Miller said. "I think the needle, or whatever it's called, the water thing, probably could have helped a little bit. You can't really say what helped with it, but it's feeling a lot better. I know that. It's not nearly as stiff as it was, so that's a positive."
The Tigers placed Miller on in the injured list May 13, retroactive to May 12 .
MORE ABOUT HIM: Tigers' Shelby Miller had elite fastball, but Dodgers helped him complement it
Miller has a 4.41 ERA, six walks and 15 strikeouts across 16⅓ innings in 15 relief appearances for the Tigers this season. He struggled throughout his past 10 outings, with a 7.71 ERA. His fastball velocity typically averages 93-94 mph, but it was at 91-92 mph in the two outings before landing on the injured list.
The decrease in velocity concerned him.
"Nothing was hurting when I was throwing," Miller said. "It was more of like, 'I don't throw 91,' so something was off. I'm assuming that it was just fatigue in there. It wasn't ready to perform at this level. ... It just wasn't there, but it's been a lot better lately."
KERRY CRUSH: Tigers' Kerry Carpenter is a great hitter, and now a reliable outfielder too
Miller had trouble bouncing back between appearances.
He dealt with symptoms of stiffness and soreness.
"Performance was down," he said. "I chalked it up to getting myself better. It's still early in the season. There's no reason to push it and injure something else further. We all sat down and thought that was the wisest decision."
In the offseason, the Tigers signed Miller — a 12-year MLB veteran who used to be a starter — to a one-year, $3.25 million contract , which includes a $4.25 million team option for 2025.
Contact Evan Petzold at [email protected] or follow him @EvanPetzold .
Listen to our weekly Tigers show "Days of Roar" every Monday afternoon on demand at freep.com, Apple , Spotify or wherever you listen to podcasts. And catch all of our podcasts and daily voice briefing at freep.com/podcasts .
C Data Types
C operators.
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
C File Handling
- C Cheatsheet
C Interview Questions
- C Programming Language Tutorial
- C Language Introduction
- Features of C Programming Language
- C Programming Language Standard
- C Hello World Program
- Compiling a C Program: Behind the Scenes
- Tokens in C
- Keywords in C
C Variables and Constants
- C Variables
- Constants in C
- Const Qualifier in C
- Different ways to declare variable as constant in C
- Scope rules in C
- Internal Linkage and External Linkage in C
- Global Variables in C
- Data Types in C
- Literals in C
- Escape Sequence in C
- Integer Promotions in C
- Character Arithmetic in C
- Type Conversion in C
C Input/Output
- Basic Input and Output in C
- Format Specifiers in C
- printf in C
- Scansets in C
- Formatted and Unformatted Input/Output functions in C with Examples
- Operators in C
- Arithmetic Operators in C
- Unary operators in C
- Relational Operators in C
- Bitwise Operators in C
- C Logical Operators
Assignment Operators in C
- Increment and Decrement Operators in C
- Conditional or Ternary Operator (?:) in C
- sizeof operator in C
- Operator Precedence and Associativity in C
C Control Statements Decision-Making
- Decision Making in C (if , if..else, Nested if, if-else-if )
- C - if Statement
- C if...else Statement
- C if else if ladder
- Switch Statement in C
- Using Range in switch Case in C
- while loop in C
- do...while Loop in C
- For Versus While
- Continue Statement in C
- Break Statement in C
- goto Statement in C
- User-Defined Function in C
- Parameter Passing Techniques in C
- Function Prototype in C
- How can I return multiple values from a function?
- main Function in C
- Implicit return type int in C
- Callbacks in C
- Nested functions in C
- Variadic functions in C
- _Noreturn function specifier in C
- Predefined Identifier __func__ in C
- C Library math.h Functions
C Arrays & Strings
- Properties of Array in C
- Multidimensional Arrays in C
- Initialization of Multidimensional Array in C
- Pass Array to Functions in C
- How to pass a 2D array as a parameter in C?
- What are the data types for which it is not possible to create an array?
- How to pass an array by value in C ?
- Strings in C
- Array of Strings in C
- What is the difference between single quoted and double quoted declaration of char array?
- C String Functions
- Pointer Arithmetics in C with Examples
- C - Pointer to Pointer (Double Pointer)
- Function Pointer in C
- How to declare a pointer to a function?
- Pointer to an Array | Array Pointer
- Difference between constant pointer, pointers to constant, and constant pointers to constants
- Pointer vs Array in C
- Dangling, Void , Null and Wild Pointers in C
- Near, Far and Huge Pointers in C
- restrict keyword in C
C User-Defined Data Types
- C Structures
- dot (.) Operator in C
- Structure Member Alignment, Padding and Data Packing
- Flexible Array Members in a structure in C
- Bit Fields in C
- Difference Between Structure and Union in C
- Anonymous Union and Structure in C
- Enumeration (or enum) in C
C Storage Classes
- Storage Classes in C
- extern Keyword in C
- Static Variables in C
- Initialization of static variables in C
- Static functions in C
- Understanding "volatile" qualifier in C | Set 2 (Examples)
- Understanding "register" keyword in C
C Memory Management
- Memory Layout of C Programs
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc()
- Difference Between malloc() and calloc() with Examples
- What is Memory Leak? How can we avoid?
- Dynamic Array in C
- How to dynamically allocate a 2D array in C?
- Dynamically Growing Array in C
C Preprocessor
- C Preprocessor Directives
- How a Preprocessor works in C?
- Header Files in C
- What’s difference between header files "stdio.h" and "stdlib.h" ?
- How to write your own header file in C?
- Macros and its types in C
- Interesting Facts about Macros and Preprocessors in C
- # and ## Operators in C
- How to print a variable name in C?
- Multiline macros in C
- Variable length arguments for Macros
- Branch prediction macros in GCC
- typedef versus #define in C
- Difference between #define and const in C?
- Basics of File Handling in C
- C fopen() function with Examples
- EOF, getc() and feof() in C
- fgets() and gets() in C language
- fseek() vs rewind() in C
- What is return type of getchar(), fgetc() and getc() ?
- Read/Write Structure From/to a File in C
- C Program to print contents of file
- C program to delete a file
- C Program to merge contents of two files into a third file
- What is the difference between printf, sprintf and fprintf?
- Difference between getc(), getchar(), getch() and getche()
Miscellaneous
- time.h header file in C with Examples
- Input-output system calls in C | Create, Open, Close, Read, Write
- Signals in C language
- Program error signals
- Socket Programming in C
- _Generics Keyword in C
- Multithreading in C
- C Programming Interview Questions (2024)
- Commonly Asked C Programming Interview Questions | Set 1
- Commonly Asked C Programming Interview Questions | Set 2
- Commonly Asked C Programming Interview Questions | Set 3
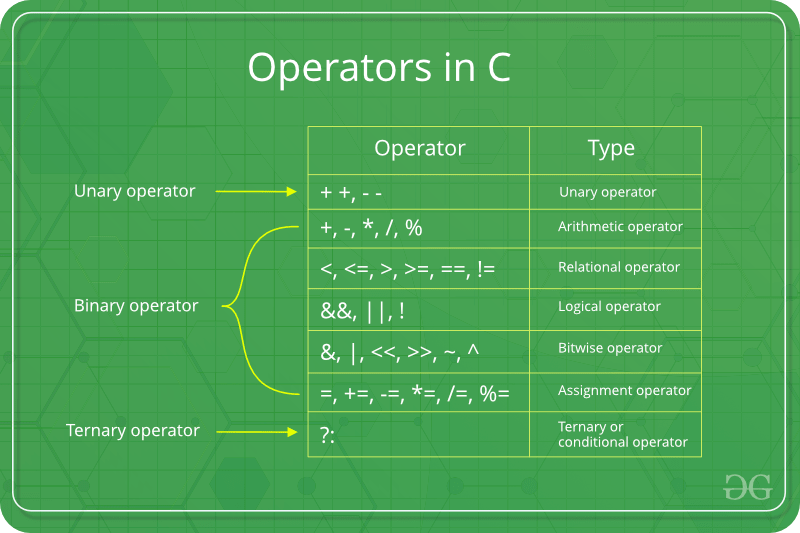
Assignment operators are used for assigning value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of the variable on the left side otherwise the compiler will raise an error.
Different types of assignment operators are shown below:
1. “=”: This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example:
2. “+=” : This operator is combination of ‘+’ and ‘=’ operators. This operator first adds the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a += 6) = 11.
3. “-=” This operator is combination of ‘-‘ and ‘=’ operators. This operator first subtracts the value on the right from the current value of the variable on left and then assigns the result to the variable on the left. Example:
If initially value stored in a is 8. Then (a -= 6) = 2.
4. “*=” This operator is combination of ‘*’ and ‘=’ operators. This operator first multiplies the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a *= 6) = 30.
5. “/=” This operator is combination of ‘/’ and ‘=’ operators. This operator first divides the current value of the variable on left by the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 6. Then (a /= 2) = 3.
Below example illustrates the various Assignment Operators:
Please Login to comment...
Similar reads.
- C-Operators
- cpp-operator
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Ronald Acuna Jr. Suffers Torn ACL, Will Miss Rest Of 2024 Season
- Ronald Acuna Jr. Exits Game With Apparent Knee Injury
- Report: MLB Teams Planning For Roki Sasaki Posting This Offseason
- Red Sox Sign Brad Keller
- Garrett Whitlock Likely To Undergo Internal Brace Procedure
- Giants Sign Drew Pomeranz To Major League Deal
- Hoops Rumors
- Pro Football Rumors
- Pro Hockey Rumors
MLB Trade Rumors
Rangers Designate Shaun Anderson For Assignment
By Nick Deeds | May 26, 2024 at 9:58am CDT
The Rangers announced this morning that they’ve designated right-hander Shaun Anderson for assignment. In a corresponding move, right-hander Gerson Garabito had his contract selected from the minors. Garabito is scheduled to start today’s game against the Twins, which would be his major league debut.
Anderson was selected to the club’s roster earlier this month after signing on a minor league deal back in April. A third-round pick by the Red Sox in the 2016 draft, Anderson made his big league debut with San Francisco in 2019 and pitched to mediocre results in a swing role, with a 5.44 ERA and 4.77 FIP in 96 innings of work across 28 appearances (16 starts). Major league innings have been hard to come by for the righty since then, as he made just 35 big league appearances across five organizations between 2020 and 2022. The results left something to be desired, as well; he pitched to a 6.85 ERA with a 5.57 FIP in that time.
The righty’s struggles at the big league level led him to try his luck overseas during the 2023 campaign, and he signed with the Korea Baseball Organization’s KIA Tigers. Anderson pitched as a starter with the club and found some success overseas, with a 3.76 ERA in his 14 appearances. He returned to stateside ball late in the campaign on a minor league deal with the Phillies, but his improved results in Korea did not carry over as he surrendered 28 runs (including 11 homers) in 11 starts for the club’s Triple-A affiliate down the stretch.
That didn’t stop the Rangers from signing Anderson to a minor league deal back in April, however, and he was selected to the roster earlier this month. Anderson ultimately made just two appearances in a Rangers uniform before being DFA’d. In 3 1/3 innings of work, he allowed two runs on six hits and a walk while striking out three in a performance that was good for a 5.40 ERA and 2.23 FIP. The Rangers will now have seven days to trade Anderson or attempt to pass him through waivers. As a player who has already been outrighted previously in his career, Anderson would have the opportunity to reject an outright assignment in favor of free agency should he clear waivers.
Replacing Anderson on the club’s roster is Garabito, a 28-year-old righty who made his pro debut with the Royals back in 2013. Garabito worked his way through the minor leagues across seven seasons in the Royals system before electing minor league free agency and joining the Giants, for whom he pitched to a 4.71 ERA in 11 appearances at the Triple-A level during 2021 season. Garabito subsequently left affiliated ball to pitch in Nicaragua, Venezuela, and the Dominican Republic over the next three seasons, racking up a 2.86 ERA in 69 1/3 innings of work during that time.
Garabito returned to stateside ball when he landed with the Rangers on a minor league deal prior to the 2024 season and has impressed in seven appearances split between the Double- and Triple-A levels with a 2.05 ERA in 30 2/3 innings of work with an enticing 30.8% strikeout rate. The Rangers evidently have seen enough for Garabito to offer him his first shot at big league action, which figures to come against Minnesota later today amid a slew of injuries to the Rangers rotation that have left key pieces such as Nathan Eovaldi and Jon Gray sidelined.
12 hours ago
This poor fella needs an updated image.
Wow, what a great story and we needed this spark. I hope CY keeps him in the majors to see if he can continue to contribute.

Was one of nine Anderson pitchers on mlb rosters. Ten if you include Pete Fairbanks who was born Peter Anderson. Gotta be a record of some sort ?
Leave a Reply Cancel reply
Please login to leave a reply.
Log in Register

- Feeds by Team
- Commenting Policy
- Privacy Policy
MLB Trade Rumors is not affiliated with Major League Baseball, MLB or MLB.com
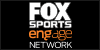
Username or Email Address
Remember Me

Royce Lewis ready for rehab assignment, close to rejoining Twins

MINNEAPOLIS — Royce Lewis is getting close.
Sidelined since Opening Day with a severe right quadriceps strain, Lewis has been cleared to begin a minor-league rehab assignment with Triple-A St. Paul, the Minnesota Twins announced Friday.
Lewis worked out in front of the Twins’ training staff Friday at Target Field and will join the Saints on their road trip to Buffalo, N.Y. He’s slated to start at designated hitter Saturday in what will be Lewis’ first game action since leaving the March 28 season opener in the third inning. Initially expected to miss approximately two months, Lewis is on target for that timetable.
Advertisement
In his absence, Jose Miranda has emerged as the Twins’ main fill-in third baseman, starting Friday for the 28th time in 49 games since Lewis’ injury. Kyle Farmer (11 starts) and Willi Castro (10) have also seen regular action at the hot corner, and collectively the Twins’ third basemen rank 14th out of 30 teams in OPS this season.
Lewis suffered back-to-back season-ending knee injuries in 2021 and 2022 and also missed time last year with oblique and hamstring injuries. When healthy enough to stay in the Twins’ lineup, he’s played at an MVP level by hitting .313/.369/.564 with 18 homers and 58 RBIs in 71 games, plus another four homers in six playoff games.
“He’s a difference-maker and a game-changer,” shortstop Carlos Correa said. “The fact that we will get him back soon is exciting. Adding him to our lineup, it’s going to boost the lineup in a big way. I’m excited for him to just go out there, take it day by day, and go through his things, show everybody that he’s ready, and then come back here and help us.”
Royce Lewis with the first Twins HR this year… because of course 😂 pic.twitter.com/QH5ihNkPNk — Minnesota Twins (@Twins) March 28, 2024
Lewis has described this rehab process as more mentally challenging than even the much longer recoveries from knee surgery, because it halted what he hoped would finally be his first full, healthy season with the Twins. And teammates and coaches have sensed the always optimistic 24-year-old’s restlessness, joking about keeping Lewis patient and sane.
“He’s been antsier than what I remember in the past,” bench coach Jayce Tingler said. “He’s been charged up, ready to get out there. I think it’s a bit challenging for him because he wants to be out there so bad with the guys. … He’s extremely eager to get out and get going. I think everybody is kind of charged up and excited for him.”
Lewis will no doubt be pushing to return as soon as possible. “He’s been wanting to play since last month,” Correa joked, and he’s previously been able to produce at the plate right away after returning from lengthy absences, but the Twins will likely want to see how his quadriceps responds to multiple games of base running and fielding.
“Royce seems to be the type of guy that doesn’t need really a lot of at-bats, just from a rhythm and a timing standpoint,” Tingler said. “I say that just because of the layoff he had at the end of last year, and then to go into the playoffs and to not miss a beat. It’s going to be about getting some strength, some stamina under the legs and get built up properly that way.”
Lewis batted third in the Twins’ order on Opening Day and going without his impact bat has forced manager Rocco Baldelli to mix and match, both at third base and with the lineup in general. His return would free up Miranda to play more first base and DH and enable Castro to play more left field, three spots where the Twins have struggled to get consistent production.
“Hopefully, when he comes back, he stays with us for the entire season,” Correa said. “And you know what he can do when he’s on the field.”
(Photo of Royce Lewis and Carlos Correa: Brace Hemmelgarn / Minnesota Twins / Getty Images)
Get all-access to exclusive stories.
Subscribe to The Athletic for in-depth coverage of your favorite players, teams, leagues and clubs. Try a week on us.

Aaron Gleeman is a staff writer for The Athletic covering the Minnesota Twins. He was previously the editor-in-chief of Baseball Prospectus and a senior writer for NBC Sports. He was named the 2021 NSMA Minnesota Sportswriter of the Year and co-hosts the "Gleeman and The Geek" podcast. Follow Aaron on Twitter @ AaronGleeman

IMAGES
VIDEO
COMMENTS
Basically C evaluates expressions. In. s = data[q] The value of data[q] is the the value of expression here and the condition is evaluated based on that. The assignment. s <- data[q] is just a side-effect.
The working of the if statement in C is as follows: STEP 1: When the program control comes to the if statement, the test expression is evaluated. STEP 2A: If the condition is true, the statements inside the if block are executed. STEP 2B: If the expression is false, the statements inside the if body are not executed. STEP 3: Program control moves out of the if block and the code after the if ...
The if else statement essentially means that " if this condition is true do the following thing, else do this thing instead". If the condition inside the parentheses evaluates to true, the code inside the if block will execute. However, if that condition evaluates to false, the code inside the else block will execute.
C if-else Statement. The if-else statement is a decision-making statement that is used to decide whether the part of the code will be executed or not based on the specified condition (test expression). If the given condition is true, then the code inside the if block is executed, otherwise the code inside the else block is executed.
In C, like in other programming languages, you can use statements that evaluate to true or false rather than using the boolean values true or false directly. Also notice the condition in the parenthesis of the if statement: n == 3. This condition compares n and the number 3. == is the comparison operator, and is one of several comparison ...
C Programming Strings. C Programming Strings; String Manipulations In C Programming Using Library Functions; String Examples in C Programming; C Structure and Union. C struct; C structs and Pointers; C Structure and Function; C Unions; C Programming Files. C File Handling; C Files Examples; C Additional Topics. C Keywords and Identifiers
7 Assignment Expressions. As a general concept in programming, an assignment is a construct that stores a new value into a place where values can be stored—for instance, in a variable. Such places are called lvalues (see Lvalues) because they are locations that hold a value. An assignment in C is an expression because it has a value; we call it an assignment expression.
This process is called decision making in 'C.'. In 'C' programming conditional statements are possible with the help of the following two constructs: 1. If statement. 2. If-else statement. It is also called as branching as a program decides which statement to execute based on the result of the evaluated condition.
The conditional operator in C is kind of similar to the if-else statement as it follows the same algorithm as of if-else statement but the conditional operator takes less space and helps to write the if-else statements in the shortest way possible. It is also known as the ternary operator in C as it operates on three operands.. Syntax of Conditional/Ternary Operator in C
Conditional execution of instructions is the basic requirement of a computer program. The if statement in C is the primary conditional statement. C allows an optional else keyword to specify the statements to be executed if the if condition is false.. C - if Statement. The if statement is a fundamental decision control statement in C programming. One or more statements in a block will get ...
Explanation. If the condition yields true after conversion to bool, statement-true is executed.. If the else part of the if statement is present and condition yields false after conversion to bool, statement-false is executed.. In the second form of if statement (the one including else), if statement-true is also an if statement then that inner if statement must contain an else part as well ...
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs . Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non ...
Simple assignment operator. Assigns values from right side operands to left side operand. C = A + B will assign the value of A + B to C. +=. Add AND assignment operator. It adds the right operand to the left operand and assign the result to the left operand. C += A is equivalent to C = C + A. -=.
Yes, you can assign in a condition, and it's very useful when you need it, and it's a perpetual trap for new C programmers. The result of the assignment, 0, is compared to see whether it is not equal to 0; it is equal to 0, so the if is false and the else is executed. Basic C pitfall.
If else programming exercises and solutions in C. if...else is a branching statement. It is used to take an action based on some condition. For example - if user inputs valid account number and pin, then allow money withdrawal. If statement works like "If condition is met, then execute the task". It is used to compare things and take some ...
In computer programming, we use the if...else statement to run one block of code under certain conditions and another block of code under different conditions.. For example, assigning grades (A, B, C) based on marks obtained by a student. if the percentage is above 90, assign grade A; if the percentage is above 75, assign grade B; if the percentage is above 65, assign grade C
Write a C program to find the maximum number between three numbers . Description: You need to write a C program to find the maximum number between three numbers. Conditions: Create three variables in c with name of number1, number2 and number3; Find out the maximum number using the nested if-else statement
Nationals Designate Victor Robles For Assignment. By Darragh McDonald | May 27, 2024 at 11:23am CDT. The Nationals announced today that outfielder Lane Thomas has been reinstated from the injured ...
Cleveland Guardians: Steven Kwan goes 1-for-3 with a three-run home run in his first rehab assignment with the Lake County Captains.
The #Braves today returned C Sean Murphy from his rehabilitation assignment and reinstated him from the injured list, and placed OF Ronald Acuña Jr. on the 10-day IL with a torn left ACL ...
0:00. 4:08. Detroit Tigers reliever Shelby Miller believes he has dodged major injury. Miller, a veteran right-hander, expects to begin a rehab assignment Tuesday with Triple-A Toledo. The 33-year ...
Royce Lewis' two-hit game in rehab assignment. May 26, 2024 | 00:00:19. Reels. Twins infielder Royce Lewis collects two hits in a rehab game with Triple-A St. Paul. Royce Lewis. highlight. rehab. Minor League Baseball. Twins affiliate.
Different types of assignment operators are shown below: 1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: a = 10; b = 20; ch = 'y'; 2. "+=": This operator is combination of '+' and '=' operators. This operator first adds the current ...
By Nick Deeds | May 26, 2024 at 9:58am CDT. The Rangers announced this morning that they've designated right-hander Shaun Anderson for assignment. In a corresponding move, right-hander Gerson ...
The reason is: Performance improvement (sometimes) Less code (always) Take an example: There is a method someMethod() and in an if condition you want to check whether the return value of the method is null. If not, you are going to use the return value again. If(null != someMethod()){. String s = someMethod();
Updated:10:58 PM EDT May 26, 2024. MOOSIC, Pa. — New York Yankees' DJ LeMahieu saw 6 at bats over two games with the RailRiders and was walked twice. LeMahieu also had some on field reps playing ...
See where your favorite Cup Series driver will pit during Sunday's race at Charlotte Motor Speedway (6 p.m. ET, FOX).
0 likes, 0 comments - assignment___helper____ on May 25, 2024: "For any kind of assignment contact Whatsapp +923065729900 or link in bio #assignmenthelp #onlneassignmenthelp".
May 24, 2024. 10. MINNEAPOLIS — Royce Lewis is getting close. Sidelined since Opening Day with a severe right quadriceps strain, Lewis has been cleared to begin a minor-league rehab assignment ...
2. Consider the following Code, int i; while (i=0) printf ("Hello"); Now Generally speaking i=0 is an assignment and not a condition for while to check. But the GCC compiler lets it go with a warning and even evaluates it correctly (does not execute the print statement).