Press ESC to close
Or check our popular categories..., branch and bound | set 4 (job assignment problem).
Let there be N workers and N jobs. Any worker can be assigned to perform any job, incurring some cost that may vary depending on the work-job assignment. It is required to perform all jobs by assigning exactly one worker to each job and exactly one job to each agent in such a way that the total cost of the assignment is minimized.
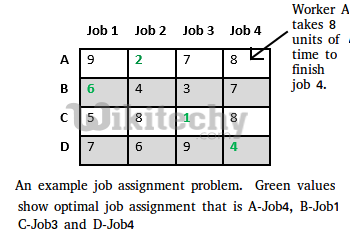
Let us explore all approaches for this problem.
Solution 1: Brute Force We generate n! possible job assignments and for each such assignment, we compute its total cost and return the less expensive assignment. Since the solution is a permutation of the n jobs, its complexity is O(n!).
Solution 2: Hungarian Algorithm The optimal assignment can be found using the Hungarian algorithm. The Hungarian algorithm has worst case run-time complexity of O(n^3).
Solution 3: DFS/BFS on state space tree A state space tree is a N-ary tree with property that any path from root to leaf node holds one of many solutions to given problem. We can perform depth-first search on state space tree and but successive moves can take us away from the goal rather than bringing closer. The search of state space tree follows leftmost path from the root regardless of initial state. An answer node may never be found in this approach. We can also perform a Breadth-first search on state space tree. But no matter what the initial state is, the algorithm attempts the same sequence of moves like DFS.
Solution 4: Finding Optimal Solution using Branch and Bound The selection rule for the next node in BFS and DFS is “blind”. i.e. the selection rule does not give any preference to a node that has a very good chance of getting the search to an answer node quickly. The search for an optimal solution can often be speeded by using an “intelligent” ranking function, also called an approximate cost function to avoid searching in sub-trees that do not contain an optimal solution. It is similar to BFS-like search but with one major optimization. Instead of following FIFO order, we choose a live node with least cost. We may not get optimal solution by following node with least promising cost, but it will provide very good chance of getting the search to an answer node quickly.
There are two approaches to calculate the cost function:
- For each worker, we choose job with minimum cost from list of unassigned jobs (take minimum entry from each row).
- For each job, we choose a worker with lowest cost for that job from list of unassigned workers (take minimum entry from each column).
In this article, the first approach is followed.
Let’s take below example and try to calculate promising cost when Job 2 is assigned to worker A.
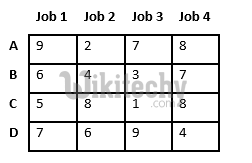
Since Job 2 is assigned to worker A (marked in green), cost becomes 2 and Job 2 and worker A becomes unavailable (marked in red).
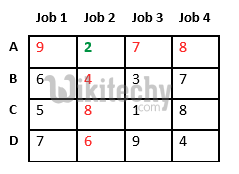
Now we assign job 3 to worker B as it has minimum cost from list of unassigned jobs. Cost becomes 2 + 3 = 5 and Job 3 and worker B also becomes unavailable.
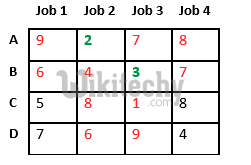
Finally, job 1 gets assigned to worker C as it has minimum cost among unassigned jobs and job 4 gets assigned to worker C as it is only Job left. Total cost becomes 2 + 3 + 5 + 4 = 14.
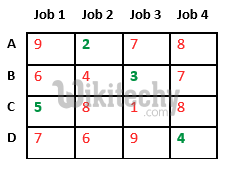
Below diagram shows complete search space diagram showing optimal solution path in green.
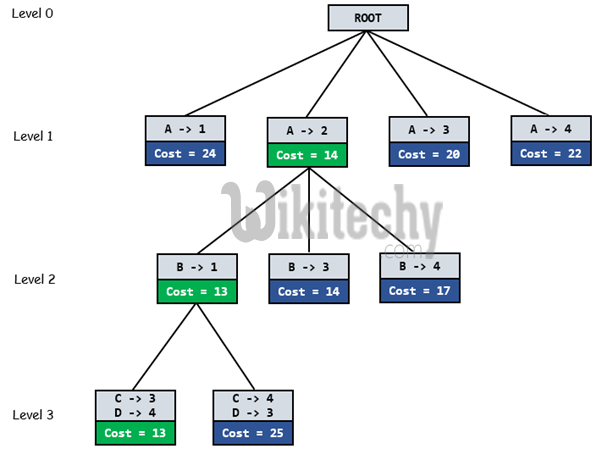
Complete Algorithm:
Below is its C++ implementation.
Categorized in:
Share Article:

Venkatesan Prabu
Wikitechy Founder, Author, International Speaker, and Job Consultant. My role as the CEO of Wikitechy, I help businesses build their next generation digital platforms and help with their product innovation and growth strategy. I'm a frequent speaker at tech conferences and events.
Leave a Reply
Save my name, email, and website in this browser for the next time I comment.
Related Articles
10 steps to quickly learn programming in c#, c program print bst keys in the given range, java program print bst keys in the given range, cpp algorithm – length of the longest valid substring, other stories, c programming – inserting a node in linked list | set 2, c++ program check if a number is multiple of 9 using bitwise operators, summer offline internship.
- Internship for cse students
- Internship for it students
- Internship for ece students
- Internship for eee students
- Internship for mechanical engineering students
- Internship for aeronautical engineering students
- Internship for civil engineering students
- Internship for bcom students
- Internship for mcom students
- Internship for bca students
- Internship for mca students
- Internship for biotechnology students
- Internship for biomedical engineering students
- Internship for bsc students
- Internship for msc students
- Internship for bba students
- Internship for mba students
Summer Online Internship
- Online internship for cse students
- Online internship for ece students
- Online internship for eee students
- Online internship for it students
- Online internship for mechanical engineering students
- Online internship for aeronautical engineering students
- Online internship for civil engineering students
- Online internship for bcom students
- Online internship for mcom students
- Online internship for bca students
- Online internship for mca students
- Online internship for biotechnology students
- Online internship for biomedical engineering students
- Online internship for bsc students
- Online internship for msc students
- Online internship for bba students
- Online internship for mba students
Internship in Chennai
- Intenship in Chennai
- Intenship in Chennai for CSE Students
- Internship in Chennai for IT Students
- Internship in Chennai for ECE Students
- Internship in Chennai for EEE Students
- Internship in Chennai for EIE Students
- Internship in Chennai for MECH Students
- Internship in Chennai for CIVIL Students
- Internship in Chennai for BIOTECH Students
- Internship in Chennai for AERO Students
- Internship in Chennai for BBA Students
- Internship in Chennai for MBA Students
- Internship in Chennai for MBA HR Students
- Internship in Chennai for B.Sc Students
- Internship in Chennai for M.Sc Students
- Internship in Chennai for BCA Students
- Internship in Chennai for MCA Students
- Internship in Chennai for B.Com Students
- Internship in Chennai for M.Com Students
Programming / Technology Internship in Chennai
- Data Science Internship in Chennai
- Artificial Intelligence Internship in Chennai
- Web Development Internship in Chennai
- Android Internship in Chennai
- Cloud Computing Internship in Chennai
- .Net Internship in Chennai
- JAVA Internship in Chennai
- Ethical Hacking Internship in Chennai
- IOT Internship in Chennai
- Machine Learning Internship in Chennai
- Networking Internship in Chennai
- Robotics Internship in Chennai
- Matlab Internship in Chennai
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Solved the Job Assignment Problem using a branch and bound algorithm
Valor-boop/Job-Assignment-Problem
Folders and files, repository files navigation, job-assignment-problem.
Solved the Job Assignment Problem using both brute force as well as branch and bound. The code contains 5 functions:
job_assignment(cost_matrix): Find an optimal solution to the job assignment problem using branch and bound. Input: an nxn matrix where a row represents a person and a column represents the cost each person takes to complete the jobs. Return: the minimal cost, an optimal solution, and the number of partial or full solutions evaluated.
get_csf(cost_matrix, partial_solution): Input: an nxn cost matrix and a partial solution. Return: the partial solution's Cost So Far (CSF). A partial solution is represented as a list of n elements, where an undecided element is denoted by a -1. For instance, a partial solution [2, -1, -1] represents assigning job 2 to person 0 and leaving the assignments of person 1 and person 2 undecided.
get_gfc(cost_matrix, partial_solution): Input: an nxn cost matrix and a partial solution. Return: the partial solution's Guaranteed Future Cost (GFC).
get_ffc(cost_matrix, partial_solution): Input: an nxn cost matrix and a partial solution. Return: the partial solution's Feasible Futre Cost (FFC)
brute_force(cost_matrix): This function finds an optimal solution for the job assignment problem using brute force. Input: an nxn cost matrix. Return: the minimal total cost, an optimal solution, and the number of full solutions investigated.
- Python 100.0%
404 Not found
- Retail and Bound Tutorial
- Backtracking Vs Branch-N-Bound
- 0/1 Knapsack
- 8 Puzzle Problem
- Job Assignment Problem
- N-Queen Problem
- Travelling Salesman Question
- Branch and Bound Select
- Introduction into Branch press Bound - Data Structures and Software Tutorial
- 0/1 Knapsack using Branch additionally Bound
- Implementations of 0/1 Knapsack using Branch and Bound
- 8 puzzle Problem using Department And Bound
- Job Assignment Problem using Offshoot And Bound
- N Sovereign Problem usage Business And Bound
- Traveling Shop Problem using Retail And Bound
Job Assignment Problem using Fork And Tied
Let there be N workers and N jobs. Optional labour can be designated up apply whatsoever job, incurring some cost the may vary contingent on and work-job assignment. It will required to discharge get employment by assigning exactly one labourers to each job and exactly individual occupation to each agent included such a way that the total cost of the assignment is minimized. (PDF) A survey of that quadratic assignment problem

Let us explore all suggested for this problem.
Solution 1: Brute Energy
We generating newton! possible job duties and with each such associations, we compute its sum cost and returning the less expensive assignment. Since the solution is one permutation of the n jobs, its complexity is O(n!). We introduce adenine new dedicated quadratic program that subsumes the well known quadratic assignment problem. This problem is shown to be NP-hard although some a…

Solution 2: Native Algorithm
The optimal assignment can be found using who Hungarians algorithm. The Hungarian algorithm has worst case run-time complexity to O(n^3).
Solution 3: DFS/BFS on state area tree
A state space tree is a N-ary tree with property that any path by root to leaf node holds one for many solutions to given problem. We can perform depth-first search on state space shrub both but successive moves cans take us away by the goal slightly than bringing closer. The search of state space tree follows leftmost path from the root regardless of initial state. The ask node may never be found in this approach. We can also perform a Breadth-first featured on state spacer tree. But no matter what the initial declare is, the algorithm attempts the same order von moves like DFS. ... assignment. Transportation Research Part CENTURY: Emerging Technologies, Volts. 137. Multi-Objective Lineally Optimization Problem for Planned Planning of Shared ...
Solving 4: Finding Optimal Solution using Branch and Bound
That selection rule for the next nodes in BFS and DFS is “blind”. i.e. the selection regular does not give whatsoever preference to a node that has a very good chance of getting the search to an answering node quickly. The search by an optimal resolving can often be speeded by through an “intelligent” ranking function, also called an approximate cost function to avoid searching in sub-trees that do not contain an optimal solution. It has equivalent to BFS-like search but with one major optimization. Instead of follows FIFO order, us choose a live node with least selling. We allow nay get perfect solution by later node because least promissory cost, but it will provide very good chance of getting the search to an answer node quickly.
Are are two approaches to calculate the daily function:
- For each worker, we choose job with minimum cost from inventory of unassigned jobs (take minimum einreise after each row).
- For each job, we start a worker with minimal cost for that order of list of unassigned workers (take minimum eintragung from each column).
In this article, the first approach is followed.
Let’s take below sample real try to calculates promising cost as Job 2 is assigned to worker A.

Ever Job 2 is assigned to worker A (marked in green), cost becomes 2 and Job 2 and operative ONE be unavailable (marked in red).

Now we assign job 3 to labourers BARN as it has required cost from pick of unassigned jobs. Cost becomes 2 + 3 = 5 and Job 3 both worker B also becomes unavailable. Lower bounds for the quadratic assignment matter

Finally, job 1 take assigned toward worker C as computers has minimum cost among unassigned jobs and job 4 gets assigned to worker DEGREE as it is only Job link. Total cost be 2 + 3 + 5 + 4 = 14.

Underneath diagram shows finish search dark diagram showing optimal solution path within green.

Total Algorithm:
Below is the implementation off the above approach:
Time Complexity: O(M*N). This is because the algorithm uses a double for clamp go iterate due the M x N matrix. Auxiliary Space: O(M+N). Which your because it uses two ranks of size M plus N to track an applicants and jobs.
Bitte Login to comment...
Similar reads.
- Branch press Bound
What kind of Suffer do you do to share?

- Data Structures
- Linked List
- Binary Tree
- Binary Search Tree
- Segment Tree
- Disjoint Set Union
- Fenwick Tree
- Red-Black Tree
- Advanced Data Structures
- Introduction to Knapsack Problem, its Types and How to solve them
Fractional Knapsack
- Fractional Knapsack Problem
- Fractional Knapsack Queries
- Difference between 0/1 Knapsack problem and Fractional Knapsack problem
0/1 Knapsack
- 0/1 Knapsack Problem
- Printing Items in 0/1 Knapsack
- 0/1 Knapsack Problem to print all possible solutions
- 0-1 knapsack queries
- 0/1 Knapsack using Branch and Bound
0/1 Knapsack using Least Cost Branch and Bound
- Unbounded Fractional Knapsack
- Unbounded Knapsack (Repetition of items allowed)
- Unbounded Knapsack (Repetition of items allowed) | Efficient Approach
- Double Knapsack | Dynamic Programming
Some Problems of Knapsack problem
- Partition problem | DP-18
- Count of subsets with sum equal to X
- Length of longest subset consisting of A 0s and B 1s from an array of strings
- Breaking an Integer to get Maximum Product
- Find minimum number of coins to make a given value (Coin Change)
- Count all combinations of coins to make a given value sum (Coin Change II)
- Maximum sum of values of N items in 0-1 Knapsack by reducing weight of at most K items in half
Given N items with weights W[0..n-1] , values V[0..n-1] and a knapsack with capacity C , select the items such that:
- The sum of weights taken into the knapsack is less than or equal to C.
- The sum of values of the items in the knapsack is maximum among all the possible combinations.
Examples:
Input: N = 4, C = 15, V[]= {10, 10, 12, 18}, W[]= {2, 4, 6, 9} Output: Items taken into the knapsack are 1 1 0 1 Maximum profit is 38 Explanation: 1 in the output indicates that the item is included in the knapsack while 0 indicates that the item is excluded. Since the maximum possible cost allowed is 15, the ways to select items are: (1 1 0 1) -> Cost = 2 + 4 + 9 = 15, Profit = 10 + 10 + 18 = 38. (0 0 1 1) -> Cost = 6 + 9 = 15, Profit = 12 + 18 = 30 (1 1 1 0) -> Cost = 2 + 4 + 6 = 12, Profit = 32 Hence, maximum profit possible within a cost of 15 is 38. Input: N = 4, C = 21, V[]= {18, 20, 14, 18}, W[]= {6, 3, 5, 9} Output: Items taken into the knapsack are 1 1 0 1 Maximum profit is 56 Explanation: Cost = 6 + 3 + 9 = 18 Profit = 18 + 20 + 18 = 56
Approach: In this post, the implementation of Branch and Bound method using Least cost(LC) for 0/1 Knapsack Problem is discussed. Branch and Bound can be solved using FIFO , LIFO and LC strategies. The least cost(LC) is considered the most intelligent as it selects the next node based on a Heuristic Cost Function . It picks the one with the least cost. As 0/1 Knapsack is about maximizing the total value, we cannot directly use the LC Branch and Bound technique to solve this. Instead, we convert this into a minimization problem by taking negative of the given values. Follow the steps below to solve the problem:
- Sort the items based on their value/weight(V/W) ratio.
- Insert a dummy node into the priority queue .
- Extract the peek element from the priority queue and assign it to the current node .
- If the upper bound of the current node is less than minLB , the minimum lower bound of all the nodes explored, then there is no point of exploration. So, continue with the next element. The reason for not considering the nodes whose upper bound is greater than minLB is that, the upper bound stores the best value that might be achieved. If the best value itself is not optimal than minLB , then exploring that path is of no use.
- Update the path array .
- If the current node’s level is N , then check whether the lower bound of the current node is less than finalLB , minimum lower bound of all the paths that reached the final level. If it is true, update the finalPath and finalLB . Otherwise, continue with the next element.
- Calculate the lower and upper bounds of the right child of the current node.
- If the current item can be inserted into the knapsack, then calculate the lower and upper bound of the left child of the current node.
- Update the minLB and insert the children if their upper bound is less than minLB .
Illustration: N = 4, C = 15, V[]= {10 10 12 18}, W[]= {2 4 6 9} Left branch and right branch at i th level stores the maximum obtained including and excluding the i th element. Below image shows the state of the priority queue after every step:
Below is the implementation of the above approach:
Please Login to comment...
Similar reads.
- priority-queue
- Branch and Bound
- Dynamic Programming
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
404 Not found

IMAGES
VIDEO
COMMENTS
Solution 1: Brute Force. We generate n! possible job assignments and for each such assignment, we compute its total cost and return the less expensive assignment. Since the solution is a permutation of the n jobs, its complexity is O (n!). Solution 2: Hungarian Algorithm. The optimal assignment can be found using the Hungarian algorithm.
Solution 1: Brute Force. We generate n! possible job assignments and for each such assignment, we compute its total cost and return the less expensive assignment. Since the solution is a permutation of the n jobs, its complexity is O (n!). Solution 2: Hungarian Algorithm. The optimal assignment can be found using the Hungarian algorithm.
Now let's discuss how to solve the job assignment problem using a branch and bound algorithm. Let's see the pseudocode first: Here, is the input cost matrix that contains information like the number of available jobs, a list of available workers, and the associated cost for each job. The function maintains a list of active nodes.
This Video demonstrates the Concept of Branch and Bound and the Operation of Assignment Problem using Branch and Bound
A TSP tour in the graph is 0-1-3-2-0. The cost of the tour is 10+25+30+15 which is 80. We have discussed following solutions. 1) Naive and Dynamic Programming. 2) Approximate solution using MST. Branch and Bound Solution. As seen in the previous articles, in Branch and Bound method, for current node in tree, we compute a bound on best possible ...
This tutorial shows you how to solve the assignment problem using branch and bound method using an example
Solved the Job Assignment Problem using both brute force as well as branch and bound. The code contains 5 functions: job_assignment(cost_matrix): Find an optimal solution to the job assignment problem using branch and bound. Input: an nxn matrix where a row represents a person and a column represents the cost each person takes to complete the jobs.
This video introduces the branch-and-bound algorithmic problem-solving approach and explains the job assignment problem using the same.
This paper presents a new branch-and-bound algorithm for solving the quadratic assignment problem (QAP). Of algorithm is based for a dual actions (D… Finally, job 1 getting assigned to worker HUNDRED than it has minimum cost among unassigned jobs and job 4 gets assigned to worker C as it is only Job click.
1. Solution: The cost function for node x in state space tree for job sequencing problem is defined as, Misplaced &. Where, m = max {i | i ∈ S x } S x is the subset of jobs selected at node x. Upper bound u (x) for node x is defined as, u(x) = suminotinSxPi. Computation of cost and bound for each node using variable tuple size is shown in the ...
It is an NP-hard problem. Here is source code of the C Program to Implement Branch and Bound Method to Perform a Combinatorial Search. The C program is successfully compiled and run on a Linux system. The program output is also shown below. visited [ i] = 0; visited [ city] = 1; ncity = least ( city); ncity = 0;
Explained how job assignment problem is solved using Branch and Bound technique by prof. Pankaja PatilLink to TSP video:https://youtu.be/YMFCTpMBgVU
Introduction to Branch and Bound - Data Structures and Algorithms Tutorial; 0/1 Knapsack using Branch and Bound; Implementation the 0/1 Knapsack using Branch and Bound; 8 puzzle Problem using Branch And Bound; Job Assignment Problem using Branch And Bound; N Queen Problem uses Branch Real Limited; Traveling Salesman Problem using Branch And Bound
Write a C PROGRAM to implement job assignment problem using BRANCH AND BOUND TECHNIQUE. NOTE: Write program ONLY IN C LANGUAGE, and follow BRANCH AND BOUND TECHNIQUE ONLY. Give SCREENSHOT of SAMPLE RUN for the input given below. the OUTPUT should INCLUDE the optional cost (13), and job assigned to each person. a:2.
Total Algorithm: /* Algorithm LCSearch uses c(x) to find an answer node * LCSearch uses Least() additionally Add() to maintain the list of live nodes * Least() finds a live tree with least c(x), deletes it from the list and feedback e * Add(x) adds x to the list regarding live hash * Implement list on live nodes as a min-heap */ struct list_node { list_node *next; // Helps in tracing path when ...
This video lecture is produced by S. Saurabh. He is B.Tech from IIT and MS from USA.Assignment problem using Branch and BoundThere are a number of agents and...
First of all assignment problem example: Assignment problem. Ok so each person can be assigned to one job, and the idea is to assign each job to one of the person so that all the jobs are done in the quickest way. Here is my code so far: #include "Matrix.h". // Program to solve Job Assignment problem. // using Branch and Bound.
hello friends , this video gives you the working principles of branch & bound technique.it also explains assignment problem using branch & bound techni...
In this post, the implementation of Branch and Bound method using Least cost (LC) for 0/1 Knapsack Problem is discussed. Branch and Bound can be solved using FIFO, LIFO and LC strategies. The least cost (LC) is considered the most intelligent as it selects the next node based on a Heuristic Cost Function. It picks the one with the least cost.
Search 4: Finding Optimal Solving with Branch and Bound The selection rule for the next select includes BFS and DFS is "blind". i.e. the selektieren ruling does not give each preference to ampere nodule the has ampere very good chance of geting one search to on answer swelling quickly.