- MapReduce Algorithm
- Linear Programming using Pyomo
- Networking and Professional Development for Machine Learning Careers in the USA
- Predicting Employee Churn in Python
- Airflow Operators


Solving Assignment Problem using Linear Programming in Python
Learn how to use Python PuLP to solve Assignment problems using Linear Programming.
In earlier articles, we have seen various applications of Linear programming such as transportation, transshipment problem, Cargo Loading problem, and shift-scheduling problem. Now In this tutorial, we will focus on another model that comes under the class of linear programming model known as the Assignment problem. Its objective function is similar to transportation problems. Here we minimize the objective function time or cost of manufacturing the products by allocating one job to one machine.
If we want to solve the maximization problem assignment problem then we subtract all the elements of the matrix from the highest element in the matrix or multiply the entire matrix by –1 and continue with the procedure. For solving the assignment problem, we use the Assignment technique or Hungarian method, or Flood’s technique.
The transportation problem is a special case of the linear programming model and the assignment problem is a special case of transportation problem, therefore it is also a special case of the linear programming problem.
In this tutorial, we are going to cover the following topics:
Assignment Problem
A problem that requires pairing two sets of items given a set of paired costs or profit in such a way that the total cost of the pairings is minimized or maximized. The assignment problem is a special case of linear programming.
For example, an operation manager needs to assign four jobs to four machines. The project manager needs to assign four projects to four staff members. Similarly, the marketing manager needs to assign the 4 salespersons to 4 territories. The manager’s goal is to minimize the total time or cost.
Problem Formulation
A manager has prepared a table that shows the cost of performing each of four jobs by each of four employees. The manager has stated his goal is to develop a set of job assignments that will minimize the total cost of getting all 4 jobs.
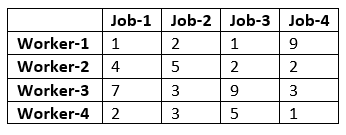
Initialize LP Model
In this step, we will import all the classes and functions of pulp module and create a Minimization LP problem using LpProblem class.
Define Decision Variable
In this step, we will define the decision variables. In our problem, we have two variable lists: workers and jobs. Let’s create them using LpVariable.dicts() class. LpVariable.dicts() used with Python’s list comprehension. LpVariable.dicts() will take the following four values:
- First, prefix name of what this variable represents.
- Second is the list of all the variables.
- Third is the lower bound on this variable.
- Fourth variable is the upper bound.
- Fourth is essentially the type of data (discrete or continuous). The options for the fourth parameter are LpContinuous or LpInteger .
Let’s first create a list route for the route between warehouse and project site and create the decision variables using LpVariable.dicts() the method.
Define Objective Function
In this step, we will define the minimum objective function by adding it to the LpProblem object. lpSum(vector)is used here to define multiple linear expressions. It also used list comprehension to add multiple variables.
Define the Constraints
Here, we are adding two types of constraints: Each job can be assigned to only one employee constraint and Each employee can be assigned to only one job. We have added the 2 constraints defined in the problem by adding them to the LpProblem object.
Solve Model
In this step, we will solve the LP problem by calling solve() method. We can print the final value by using the following for loop.
From the above results, we can infer that Worker-1 will be assigned to Job-1, Worker-2 will be assigned to job-3, Worker-3 will be assigned to Job-2, and Worker-4 will assign with job-4.
In this article, we have learned about Assignment problems, Problem Formulation, and implementation using the python PuLp library. We have solved the Assignment problem using a Linear programming problem in Python. Of course, this is just a simple case study, we can add more constraints to it and make it more complicated. You can also run other case studies on Cargo Loading problems , Staff scheduling problems . In upcoming articles, we will write more on different optimization problems such as transshipment problem, balanced diet problem. You can revise the basics of mathematical concepts in this article and learn about Linear Programming in this article .
- Solving Blending Problem in Python using Gurobi
- Transshipment Problem in Python Using PuLP
You May Also Like
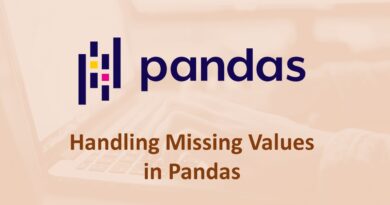
Handling Missing Values in Pandas
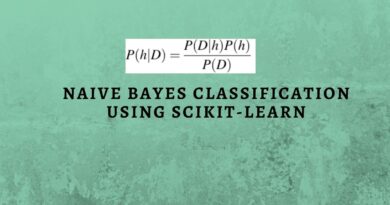
Naive Bayes Classification using Scikit-learn
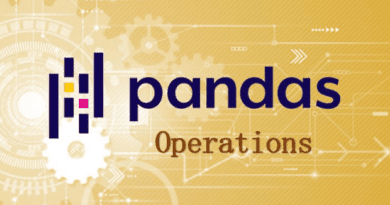
Pandas Basic Operations
Your Article Library
Assignment problem in linear programming : introduction and assignment model.
ADVERTISEMENTS:
Assignment problem is a special type of linear programming problem which deals with the allocation of the various resources to the various activities on one to one basis. It does it in such a way that the cost or time involved in the process is minimum and profit or sale is maximum. Though there problems can be solved by simplex method or by transportation method but assignment model gives a simpler approach for these problems.
In a factory, a supervisor may have six workers available and six jobs to fire. He will have to take decision regarding which job should be given to which worker. Problem forms one to one basis. This is an assignment problem.
1. Assignment Model :
Suppose there are n facilitates and n jobs it is clear that in this case, there will be n assignments. Each facility or say worker can perform each job, one at a time. But there should be certain procedure by which assignment should be made so that the profit is maximized or the cost or time is minimized.

In the table, Co ij is defined as the cost when j th job is assigned to i th worker. It maybe noted here that this is a special case of transportation problem when the number of rows is equal to number of columns.
Mathematical Formulation:
Any basic feasible solution of an Assignment problem consists (2n – 1) variables of which the (n – 1) variables are zero, n is number of jobs or number of facilities. Due to this high degeneracy, if we solve the problem by usual transportation method, it will be a complex and time consuming work. Thus a separate technique is derived for it. Before going to the absolute method it is very important to formulate the problem.
Suppose x jj is a variable which is defined as
1 if the i th job is assigned to j th machine or facility
0 if the i th job is not assigned to j th machine or facility.
Now as the problem forms one to one basis or one job is to be assigned to one facility or machine.

The total assignment cost will be given by

The above definition can be developed into mathematical model as follows:
Determine x ij > 0 (i, j = 1,2, 3…n) in order to

Subjected to constraints

and x ij is either zero or one.
Method to solve Problem (Hungarian Technique):
Consider the objective function of minimization type. Following steps are involved in solving this Assignment problem,
1. Locate the smallest cost element in each row of the given cost table starting with the first row. Now, this smallest element is subtracted form each element of that row. So, we will be getting at least one zero in each row of this new table.
2. Having constructed the table (as by step-1) take the columns of the table. Starting from first column locate the smallest cost element in each column. Now subtract this smallest element from each element of that column. Having performed the step 1 and step 2, we will be getting at least one zero in each column in the reduced cost table.
3. Now, the assignments are made for the reduced table in following manner.
(i) Rows are examined successively, until the row with exactly single (one) zero is found. Assignment is made to this single zero by putting square □ around it and in the corresponding column, all other zeros are crossed out (x) because these will not be used to make any other assignment in this column. Step is conducted for each row.
(ii) Step 3 (i) in now performed on the columns as follow:- columns are examined successively till a column with exactly one zero is found. Now , assignment is made to this single zero by putting the square around it and at the same time, all other zeros in the corresponding rows are crossed out (x) step is conducted for each column.
(iii) Step 3, (i) and 3 (ii) are repeated till all the zeros are either marked or crossed out. Now, if the number of marked zeros or the assignments made are equal to number of rows or columns, optimum solution has been achieved. There will be exactly single assignment in each or columns without any assignment. In this case, we will go to step 4.
4. At this stage, draw the minimum number of lines (horizontal and vertical) necessary to cover all zeros in the matrix obtained in step 3, Following procedure is adopted:
(iii) Now tick mark all the rows that are not already marked and that have assignment in the marked columns.
(iv) All the steps i.e. (4(i), 4(ii), 4(iii) are repeated until no more rows or columns can be marked.
(v) Now draw straight lines which pass through all the un marked rows and marked columns. It can also be noticed that in an n x n matrix, always less than ‘n’ lines will cover all the zeros if there is no solution among them.
5. In step 4, if the number of lines drawn are equal to n or the number of rows, then it is the optimum solution if not, then go to step 6.
6. Select the smallest element among all the uncovered elements. Now, this element is subtracted from all the uncovered elements and added to the element which lies at the intersection of two lines. This is the matrix for fresh assignments.
7. Repeat the procedure from step (3) until the number of assignments becomes equal to the number of rows or number of columns.
Related Articles:
- Two Phase Methods of Problem Solving in Linear Programming: First and Second Phase
- Linear Programming: Applications, Definitions and Problems
No comments yet.
Leave a reply click here to cancel reply..
You must be logged in to post a comment.
Assignment Problem: Linear Programming
The assignment problem is a special type of transportation problem , where the objective is to minimize the cost or time of completing a number of jobs by a number of persons.
In other words, when the problem involves the allocation of n different facilities to n different tasks, it is often termed as an assignment problem.
The model's primary usefulness is for planning. The assignment problem also encompasses an important sub-class of so-called shortest- (or longest-) route models. The assignment model is useful in solving problems such as, assignment of machines to jobs, assignment of salesmen to sales territories, travelling salesman problem, etc.
It may be noted that with n facilities and n jobs, there are n! possible assignments. One way of finding an optimal assignment is to write all the n! possible arrangements, evaluate their total cost, and select the assignment with minimum cost. But, due to heavy computational burden this method is not suitable. This chapter concentrates on an efficient method for solving assignment problems that was developed by a Hungarian mathematician D.Konig.
"A mathematician is a device for turning coffee into theorems." -Paul Erdos
Formulation of an assignment problem
Suppose a company has n persons of different capacities available for performing each different job in the concern, and there are the same number of jobs of different types. One person can be given one and only one job. The objective of this assignment problem is to assign n persons to n jobs, so as to minimize the total assignment cost. The cost matrix for this problem is given below:
The structure of an assignment problem is identical to that of a transportation problem.
To formulate the assignment problem in mathematical programming terms , we define the activity variables as
for i = 1, 2, ..., n and j = 1, 2, ..., n
In the above table, c ij is the cost of performing jth job by ith worker.
Generalized Form of an Assignment Problem
The optimization model is
Minimize c 11 x 11 + c 12 x 12 + ------- + c nn x nn
subject to x i1 + x i2 +..........+ x in = 1 i = 1, 2,......., n x 1j + x 2j +..........+ x nj = 1 j = 1, 2,......., n
x ij = 0 or 1
In Σ Sigma notation
x ij = 0 or 1 for all i and j
An assignment problem can be solved by transportation methods, but due to high degree of degeneracy the usual computational techniques of a transportation problem become very inefficient. Therefore, a special method is available for solving such type of problems in a more efficient way.
Assumptions in Assignment Problem
- Number of jobs is equal to the number of machines or persons.
- Each man or machine is assigned only one job.
- Each man or machine is independently capable of handling any job to be done.
- Assigning criteria is clearly specified (minimizing cost or maximizing profit).
Share this article with your friends
Operations Research Simplified Back Next
Goal programming Linear programming Simplex Method Transportation Problem

- school Campus Bookshelves
- menu_book Bookshelves
- perm_media Learning Objects
- login Login
- how_to_reg Request Instructor Account
- hub Instructor Commons
Margin Size
- Download Page (PDF)
- Download Full Book (PDF)
- Periodic Table
- Physics Constants
- Scientific Calculator
- Reference & Cite
- Tools expand_more
- Readability
selected template will load here
This action is not available.
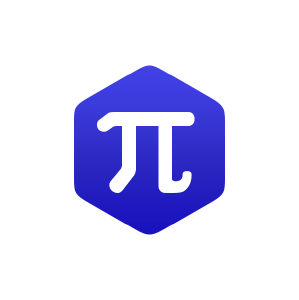
4.3: Linear Programming - Maximization Applications
- Last updated
- Save as PDF
- Page ID 40134
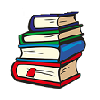
- Rupinder Sekhon and Roberta Bloom
- De Anza College
\( \newcommand{\vecs}[1]{\overset { \scriptstyle \rightharpoonup} {\mathbf{#1}} } \)
\( \newcommand{\vecd}[1]{\overset{-\!-\!\rightharpoonup}{\vphantom{a}\smash {#1}}} \)
\( \newcommand{\id}{\mathrm{id}}\) \( \newcommand{\Span}{\mathrm{span}}\)
( \newcommand{\kernel}{\mathrm{null}\,}\) \( \newcommand{\range}{\mathrm{range}\,}\)
\( \newcommand{\RealPart}{\mathrm{Re}}\) \( \newcommand{\ImaginaryPart}{\mathrm{Im}}\)
\( \newcommand{\Argument}{\mathrm{Arg}}\) \( \newcommand{\norm}[1]{\| #1 \|}\)
\( \newcommand{\inner}[2]{\langle #1, #2 \rangle}\)
\( \newcommand{\Span}{\mathrm{span}}\)
\( \newcommand{\id}{\mathrm{id}}\)
\( \newcommand{\kernel}{\mathrm{null}\,}\)
\( \newcommand{\range}{\mathrm{range}\,}\)
\( \newcommand{\RealPart}{\mathrm{Re}}\)
\( \newcommand{\ImaginaryPart}{\mathrm{Im}}\)
\( \newcommand{\Argument}{\mathrm{Arg}}\)
\( \newcommand{\norm}[1]{\| #1 \|}\)
\( \newcommand{\Span}{\mathrm{span}}\) \( \newcommand{\AA}{\unicode[.8,0]{x212B}}\)
\( \newcommand{\vectorA}[1]{\vec{#1}} % arrow\)
\( \newcommand{\vectorAt}[1]{\vec{\text{#1}}} % arrow\)
\( \newcommand{\vectorB}[1]{\overset { \scriptstyle \rightharpoonup} {\mathbf{#1}} } \)
\( \newcommand{\vectorC}[1]{\textbf{#1}} \)
\( \newcommand{\vectorD}[1]{\overrightarrow{#1}} \)
\( \newcommand{\vectorDt}[1]{\overrightarrow{\text{#1}}} \)
\( \newcommand{\vectE}[1]{\overset{-\!-\!\rightharpoonup}{\vphantom{a}\smash{\mathbf {#1}}}} \)
Learning Objectives
In this section, you will learn to:
- Recognize the typical form of a linear programming problem.
- Formulate maximization linear programming problems.
- Graph feasible regions for maximization linear programming problems.
- Determine optimal solutions for maximization linear programming problems.
Prerequisite Skills
Before you get started, take this prerequisite quiz.
1. Graph this system of inequalities:
\(\left\{\begin{array} {l} 2x+4y\leq 10\\3x−5y<15\end{array}\right.\)
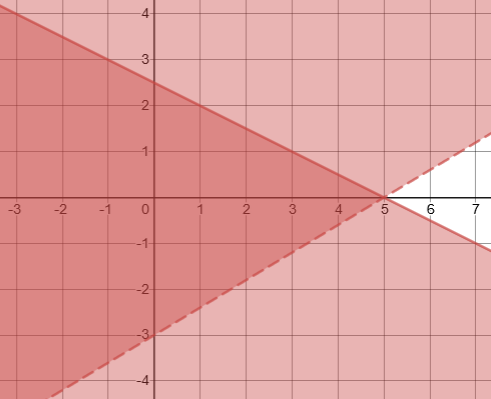
If you missed this problem, review Section 4.2 . (Note that this will open in a new window.)
2. Graph this system of inequalities:
\(\left\{\begin{array} {l} y\leq 2x+1\\y\leq-3x+6\\x\geq0\\y\geq0\end{array}\right.\)
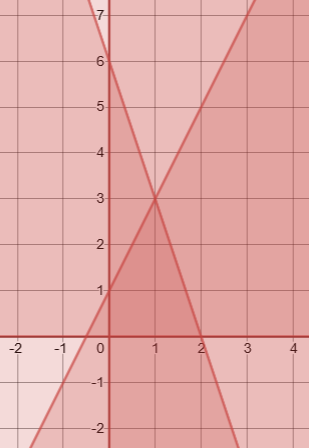
(The solution is in the center region.)
Application problems in business, economics, and social and life sciences often ask us to make decisions on the basis of certain conditions. The conditions or constraints often take the form of inequalities. In this section, we will begin to formulate, analyze, and solve such problems, at a simple level, to understand the many components of such a problem.
A typical linear programming problem consists of finding an extreme value of a linear function subject to certain constraints. We are either trying to maximize or minimize the value of this linear function, such as to maximize profit or revenue, or to minimize cost. That is why these linear programming problems are classified as maximization or minimization problems , or just optimization problems . The function we are trying to optimize is called an objective function , and the conditions that must be satisfied are called constraints .
When we graph all constraints, the area of the graph that satisfies all constraints is called the feasible region . The Fundamental Theorem of Linear Programming states that the maximum (or minimum) value of the objective function always takes place at the vertices of the feasible region. We call these vertices critical points. These are found using any methods from Chapter 3 as we are looking for the points where any two of the boundary lines intersect.
A typical example is to maximize profit from producing several products, subject to limitations on materials or resources needed for producing these items; the problem requires us to determine the amount of each item produced. Another type of problem involves scheduling; we need to determine how much time to devote to each of several activities in order to maximize income from (or minimize cost of) these activities, subject to limitations on time and other resources available for each activity.
In this chapter, we will work with problems that involve only two variables, and therefore, can be solved by graphing. Here are the steps we'll follow:
The Maximization Linear Programming Problems
- Define the unknowns.
- Write the objective function that needs to be maximized.
- For the standard maximization linear programming problems, constraints are of the form: \(ax + by ≤ c\)
- Since the variables are non-negative, we include the constraints: \(x ≥ 0\); \(y ≥ 0\).
- Graph the constraints.
- Shade the feasible region.
- Find the corner points.
- Find the value of the objective function at each corner point to determine the corner point that gives the maximum value.
Example \(\PageIndex{1}\)
Niki holds two part-time jobs, Job I and Job II. She never wants to work more than a total of 12 hours a week. She has determined that for every hour she works at Job I, she needs 2 hours of preparation time, and for every hour she works at Job II, she needs one hour of preparation time, and she cannot spend more than 16 hours for preparation.
If Niki makes $40 an hour at Job I, and $30 an hour at Job II, how many hours should she work per week at each job to maximize her income?
We start by defining our unknowns.
- Let the number of hours per week Niki will work at Job I = \(x\).
- Let the number of hours per week Niki will work at Job II = \(y\).
Now we write the objective function. Since Niki gets paid $40 an hour at Job I, and $30 an hour at Job II, her total income I is given by the following equation.
\[I = 40x + 30y \nonumber\]
Our next task is to find the constraints. The second sentence in the problem states, "She never wants to work more than a total of 12 hours a week." This translates into the following constraint:
\[x + y \leq 12 \nonumber \]
The third sentence states, "For every hour she works at Job I, she needs 2 hours of preparation time, and for every hour she works at Job II, she needs one hour of preparation time, and she cannot spend more than 16 hours for preparation." The translation follows.
\[2x + y \leq 16 \nonumber\]
The fact that \(x\) and \(y\) can never be negative is represented by the following two constraints:
\[x \geq 0 \text{, and } y \geq 0 \nonumber.\]
Well, good news! We have formulated the problem. We restate it as
\[\begin{array}{ll} \textbf { Maximize } & \mathrm{I}=40 \mathrm{x}+30 \mathrm{y} \\ \textbf { Subject to: } & \mathrm{x}+\mathrm{y} \leq 12 \\ & 2 \mathrm{x}+\mathrm{y} \leq 16 \\ & \mathrm{x} \geq 0 ; \mathrm{y} \geq 0 \end{array}\nonumber\]
In order to solve the problem, we graph the constraints and shade the region that satisfies all the inequality constraints.
Any appropriate method can be used to graph the lines for the constraints. However often the easiest method is to graph the line by plotting the x-intercept and y-intercept.
The line for a constraint will divide the plane into two region, one of which satisfies the inequality part of the constraint. A test point is used to determine which portion of the plane to shade to satisfy the inequality. Any point on the plane that is not on the line can be used as a test point.
- If the test point satisfies the inequality, then the region of the plane that satisfies the inequality is the region that contains the test point.
- If the test point does not satisfy the inequality, then the region that satisfies the inequality lies on the opposite side of the line from the test point.
In the graph below, after the lines representing the constraints were graphed, the point (0,0) was used as a test point to determine that
- (0,0) satisfies the constraint \(x + y \leq 12\) because \(0 + 0 < 12\)
- (0,0) satisfies the constraint \(2x + y \leq 16\) because \(2(0) + 0 < 16\)
Therefore, in this example, we shade the region that is below and to the left of both constraint lines, but also above the x axis and to the right of the y axis, in order to further satisfy the constraints \(x \geq 0\) and \(y \geq 0\).
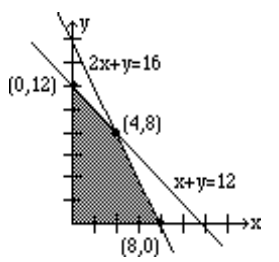
The shaded region where all conditions are satisfied is the feasible region or the feasible polygon.
The Fundamental Theorem of Linear Programming states that the maximum (or minimum) value of the objective function always takes place at the vertices of the feasible region.
Therefore, we will identify all the vertices (corner points) of the feasible region. These are found using any methods from Chapter 3 as we are looking for the points where any two of the boundary lines intersect. They are listed as (0, 0), (0, 12), (4, 8), (8, 0). To maximize Niki's income, we will substitute these points in the objective function to see which point gives us the highest income per week. We list the results below.
Clearly, the point (4, 8) gives the most profit: $400.
Therefore, we conclude that Niki should work 4 hours at Job I, and 8 hours at Job II.
Example \(\PageIndex{2}\)
A factory manufactures two types of gadgets, regular and premium. Each gadget requires the use of two operations, assembly and finishing, and there are at most 12 hours available for each operation. A regular gadget requires 1 hour of assembly and 2 hours of finishing, while a premium gadget needs 2 hours of assembly and 1 hour of finishing. Due to other restrictions, the company can make at most 7 gadgets a day. If a profit of $20 is realized for each regular gadget and $30 for a premium gadget, how many of each should be manufactured to maximize profit?
We define our unknowns:
- Let the number of regular gadgets manufactured each day = \(x\).
- and the number of premium gadgets manufactured each day = \(y\).
The objective function is
\[P = 20x + 30y \nonumber\]
We now write the constraints. The fourth sentence states that the company can make at most 7 gadgets a day. This translates as
\[x + y \leq 7 \nonumber\]
Since the regular gadget requires one hour of assembly and the premium gadget requires two hours of assembly, and there are at most 12 hours available for this operation, we get
\[x + 2y \leq 12 \nonumber\]
Similarly, the regular gadget requires two hours of finishing and the premium gadget one hour. Again, there are at most 12 hours available for finishing. This gives us the following constraint.
\[2x + y \leq 12 \nonumber \]
We have formulated the problem as follows:
\[\begin{array}{ll} \textbf { Maximize } & \mathrm{P}=20 \mathrm{x}+30 \mathrm{y} \\ \textbf { Subject to: } & \mathrm{x}+\mathrm{y} \leq 7 \\ & \mathrm{x}+2\mathrm{y} \leq 12 \\ & 2\mathrm{x} +\mathrm{y} \leq 12 \\ & \mathrm{x} \geq 0 ; \mathrm{y} \geq 0 \end{array} \nonumber\]
In order to solve the problem, we next graph the constraints and feasible region.
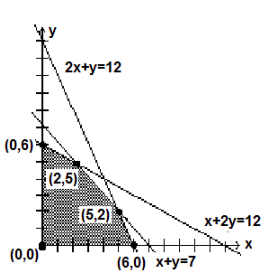
Again, we have shaded the feasible region, where all constraints are satisfied.
Since the extreme value of the objective function always takes place at the vertices of the feasible region, we identify all the critical points. They are listed as (0, 0), (0, 6), (2, 5), (5, 2), and (6, 0). To maximize profit, we will substitute these points in the objective function to see which point gives us the maximum profit each day. The results are listed below.
The point (2, 5) gives the most profit, and that profit is $190. Therefore, we conclude that we should manufacture 2 regular gadgets and 5 premium gadgets daily to obtain the maximum profit of $190.
So far we have focused on “ standard maximization problems ” in which
- The objective function is to be maximized
- All constraints are of the form \(ax + by \leq c\)
- All variables are constrained to by non-negative (\(x ≥ 0\), \(y ≥ 0\))
We will next consider an example where that is not the case. Our next problem is said to have “ mixed constraints ”, since some of the inequality constraints are of the form \(ax + by ≤ c\) and some are of the form \(ax + by ≥ c\). The non-negativity constraints are still an important requirement in any linear program.
Example \(\PageIndex{3}\)
Solve the following maximization problem graphically.
\[\begin{array}{ll} \textbf { Maximize } & \mathrm{P}=10 \mathrm{x}+15 \mathrm{y} \\ \textbf { Subject to: } & \mathrm{x}+\mathrm{y} \geq 1 \\ & \mathrm{x}+2\mathrm{y} \leq 6 \\ & 2\mathrm{x} +\mathrm{y} \leq 6 \\ & \mathrm{x} \geq 0 ; \mathrm{y} \geq 0 \end{array} \nonumber\]
The graph is shown below.
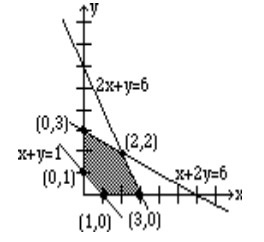
The five critical points are listed in the above figure. The reader should observe that the first constraint \(x + y ≥ 1\) requires that the feasible region must be bounded below by the line \(x + y =1\); the test point (0,0) does not satisfy \(x + y ≥ 1\), so we shade the region on the opposite side of the line from test point (0,0).
Clearly, the point (2, 2) maximizes the objective function to a maximum value of 50.
It is important to observe that that if the point (0,0) lies on the line for a constraint, then (0,0) could not be used as a test point. We would need to select any other point we want that does not lie on the line to use as a test point in that situation.
Finally, we address an important question. Is it possible to determine the point that gives the maximum value without calculating the value at each critical point?
The answer is yes.
We summarize:
Quantitative Techniques: Theory and Problems by P. C. Tulsian, Vishal Pandey
Get full access to Quantitative Techniques: Theory and Problems and 60K+ other titles, with a free 10-day trial of O'Reilly.
There are also live events, courses curated by job role, and more.
WHAT IS ASSIGNMENT PROBLEM
Assignment Problem is a special type of linear programming problem where the objective is to minimise the cost or time of completing a number of jobs by a number of persons.
The assignment problem in the general form can be stated as follows:
“Given n facilities, n jobs and the effectiveness of each facility for each job, the problem is to assign each facility to one and only one job in such a way that the measure of effectiveness is optimised (Maximised or Minimised).”
Several problems of management has a structure identical with the assignment problem.
Example I A manager has four persons (i.e. facilities) available for four separate jobs (i.e. jobs) and the cost of assigning (i.e. effectiveness) each job to each ...
Get Quantitative Techniques: Theory and Problems now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.
Don’t leave empty-handed
Get Mark Richards’s Software Architecture Patterns ebook to better understand how to design components—and how they should interact.
It’s yours, free.
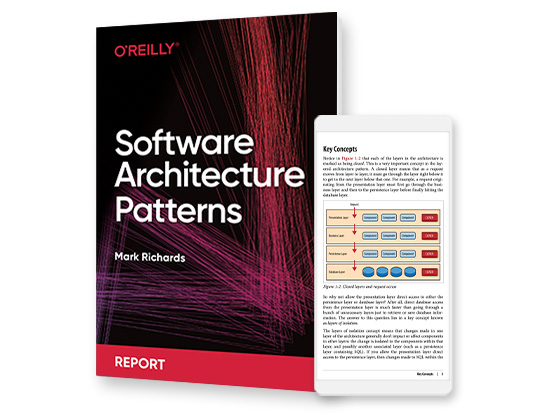
Check it out now on O’Reilly
Dive in for free with a 10-day trial of the O’Reilly learning platform—then explore all the other resources our members count on to build skills and solve problems every day.
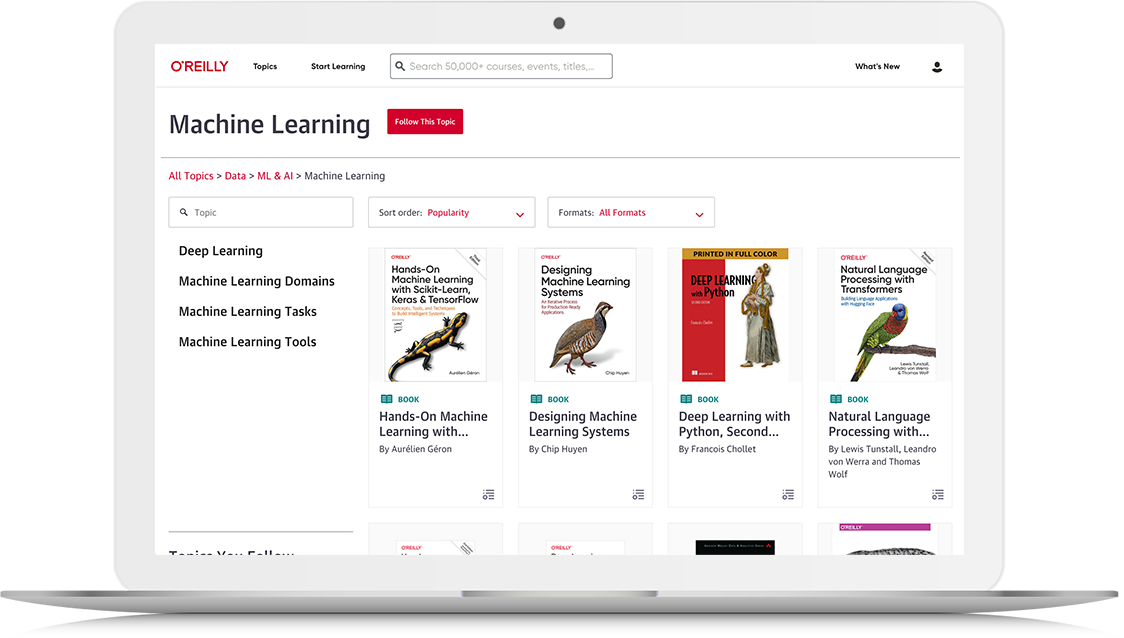
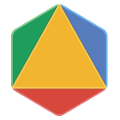
- Google OR-Tools
- Español – América Latina
- Português – Brasil
- Tiếng Việt
Solving an Assignment Problem
This section presents an example that shows how to solve an assignment problem using both the MIP solver and the CP-SAT solver.
In the example there are five workers (numbered 0-4) and four tasks (numbered 0-3). Note that there is one more worker than in the example in the Overview .
The costs of assigning workers to tasks are shown in the following table.
The problem is to assign each worker to at most one task, with no two workers performing the same task, while minimizing the total cost. Since there are more workers than tasks, one worker will not be assigned a task.
MIP solution
The following sections describe how to solve the problem using the MPSolver wrapper .
Import the libraries
The following code imports the required libraries.
Create the data
The following code creates the data for the problem.
The costs array corresponds to the table of costs for assigning workers to tasks, shown above.
Declare the MIP solver
The following code declares the MIP solver.
Create the variables
The following code creates binary integer variables for the problem.
Create the constraints
Create the objective function.
The following code creates the objective function for the problem.
The value of the objective function is the total cost over all variables that are assigned the value 1 by the solver.
Invoke the solver
The following code invokes the solver.
Print the solution
The following code prints the solution to the problem.
Here is the output of the program.
Complete programs
Here are the complete programs for the MIP solution.
CP SAT solution
The following sections describe how to solve the problem using the CP-SAT solver.
Declare the model
The following code declares the CP-SAT model.
The following code sets up the data for the problem.
The following code creates the constraints for the problem.
Here are the complete programs for the CP-SAT solution.
Except as otherwise noted, the content of this page is licensed under the Creative Commons Attribution 4.0 License , and code samples are licensed under the Apache 2.0 License . For details, see the Google Developers Site Policies . Java is a registered trademark of Oracle and/or its affiliates.
Last updated 2023-01-02 UTC.
Assignment Model | Linear Programming Problem (LPP) | Introduction
What is assignment model.
→ Assignment model is a special application of Linear Programming Problem (LPP) , in which the main objective is to assign the work or task to a group of individuals such that;
i) There is only one assignment.
ii) All the assignments should be done in such a way that the overall cost is minimized (or profit is maximized, incase of maximization).
→ In assignment problem, the cost of performing each task by each individual is known. → It is desired to find out the best assignments, such that overall cost of assigning the work is minimized.
For example:
Suppose there are 'n' tasks, which are required to be performed using 'n' resources.
The cost of performing each task by each resource is also known (shown in cells of matrix)
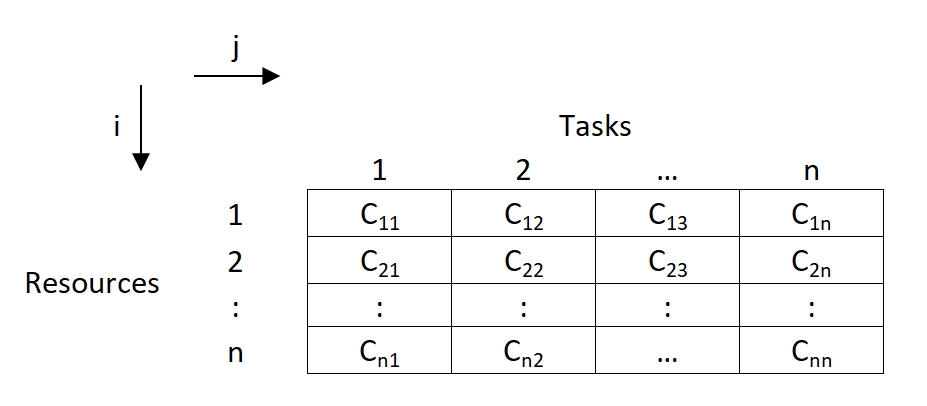
- In the above asignment problem, we have to provide assignments such that there is one to one assignments and the overall cost is minimized.

How Assignment Problem is related to LPP? OR Write mathematical formulation of Assignment Model.
→ Assignment Model is a special application of Linear Programming (LP).
→ The mathematical formulation for Assignment Model is given below:
→ Let, C i j \text {C}_{ij} C ij denotes the cost of resources 'i' to the task 'j' ; such that
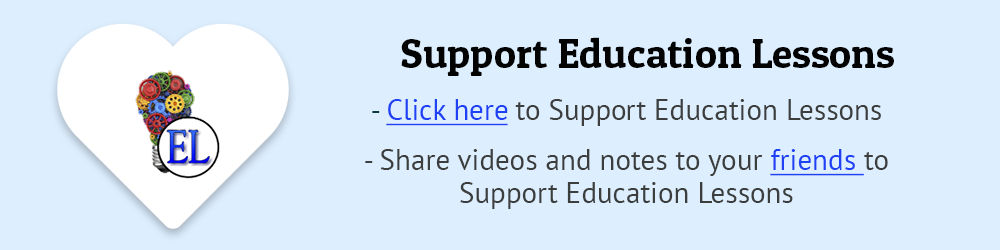
→ Now assignment problems are of the Minimization type. So, our objective function is to minimize the overall cost.
→ Subjected to constraint;
(i) For all j t h j^{th} j t h task, only one i t h i^{th} i t h resource is possible:
(ii) For all i t h i^{th} i t h resource, there is only one j t h j^{th} j t h task possible;
(iii) x i j x_{ij} x ij is '0' or '1'.
Types of Assignment Problem:
(i) balanced assignment problem.
- It consist of a suqare matrix (n x n).
- Number of rows = Number of columns
(ii) Unbalanced Assignment Problem
- It consist of a Non-square matrix.
- Number of rows ≠ \not= = Number of columns
Methods to solve Assignment Model:
(i) integer programming method:.
In assignment problem, either allocation is done to the cell or not.
So this can be formulated using 0 or 1 integer.
While using this method, we will have n x n decision varables, and n+n equalities.
So even for 4 x 4 matrix problem, it will have 16 decision variables and 8 equalities.
So this method becomes very lengthy and difficult to solve.
(ii) Transportation Methods:
As assignment problem is a special case of transportation problem, it can also be solved using transportation methods.
In transportation methods ( NWCM , LCM & VAM), the total number of allocations will be (m+n-1) and the solution is known as non-degenerated. (For eg: for 3 x 3 matrix, there will be 3+3-1 = 5 allocations)
But, here in assignment problems, the matrix is a square matrix (m=n).
So total allocations should be (n+n-1), i.e. for 3 x 3 matrix, it should be (3+3-1) = 5
But, we know that in 3 x 3 assignment problem, maximum possible possible assignments are 3 only.
So, if are we will use transportation methods, then the solution will be degenerated as it does not satisfy the condition of (m+n-1) allocations.
So, the method becomes lengthy and time consuming.
(iii) Enumeration Method:
It is a simple trail and error type method.
Consider a 3 x 3 assignment problem. Here the assignments are done randomly and the total cost is found out.
For 3 x 3 matrix, the total possible trails are 3! So total 3! = 3 x 2 x 1 = 6 trails are possible.
The assignments which gives minimum cost is selected as optimal solution.
But, such trail and error becomes very difficult and lengthy.
If there are more number of rows and columns, ( For eg: For 6 x 6 matrix, there will be 6! trails. So 6! = 6 x 5 x 4 x 3 x 2 x 1 = 720 trails possible) then such methods can't be applied for solving assignments problems.
(iv) Hungarian Method:
It was developed by two mathematicians of Hungary. So, it is known as Hungarian Method.
It is also know as Reduced matrix method or Flood's technique.
There are two main conditions for applying Hungarian Method:
(1) Square Matrix (n x n). (2) Problem should be of minimization type.
Suggested Notes:
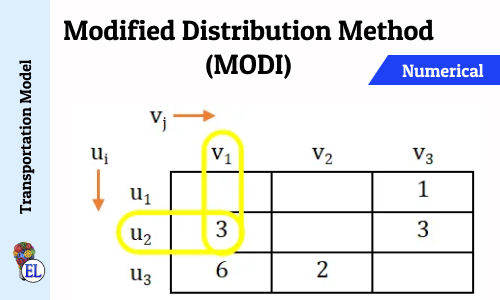
Modified Distribution Method (MODI) | Transportation Problem | Transportation Model
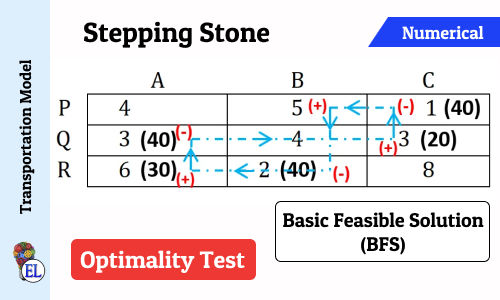
Stepping Stone | Transportation Problem | Transportation Model
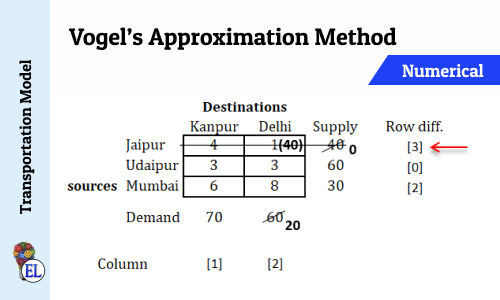
Vogel’s Approximation Method (VAM) | Method to Solve Transportation Problem | Transportation Model
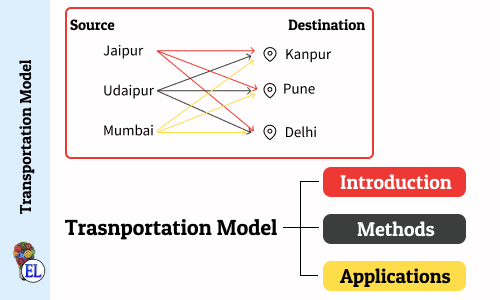
Transportation Model - Introduction
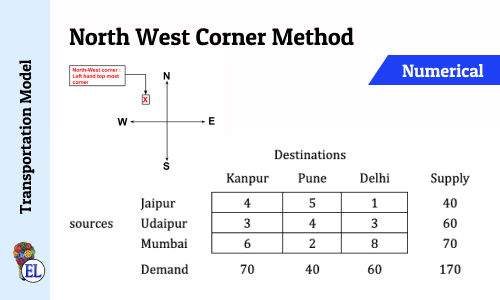
North West Corner Method | Method to Solve Transportation Problem | Transportation Model
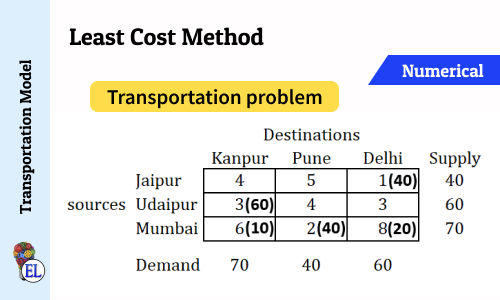
Least Cost Method | Method to Solve Transportation Problem | Transportation Model
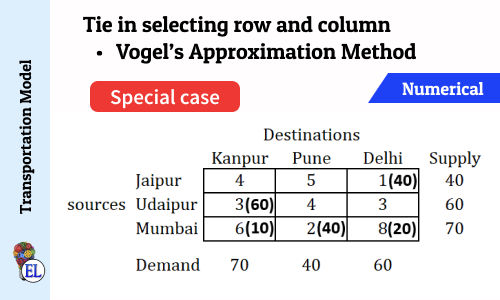
Tie in selecting row and column (Vogel's Approximation Method - VAM) | Numerical | Solving Transportation Problem | Transportation Model
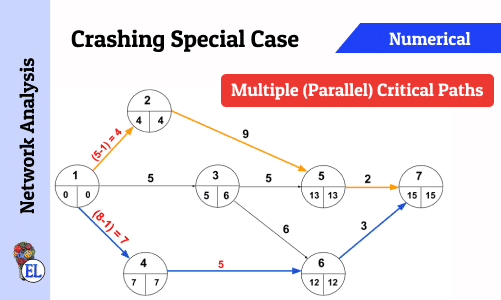
Crashing Special Case - Multiple (Parallel) Critical Paths
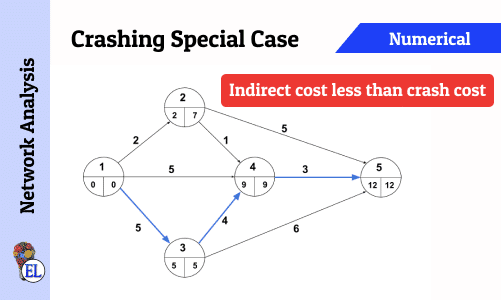
Crashing Special Case - Indirect cost less than Crash Cost
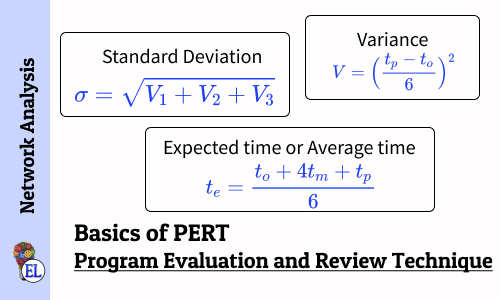
Basics of Program Evaluation and Review Technique (PERT)
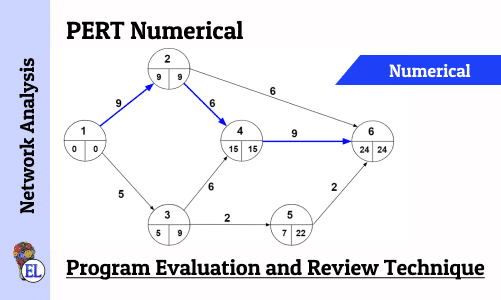
Numerical on PERT (Program Evaluation and Review Technique)
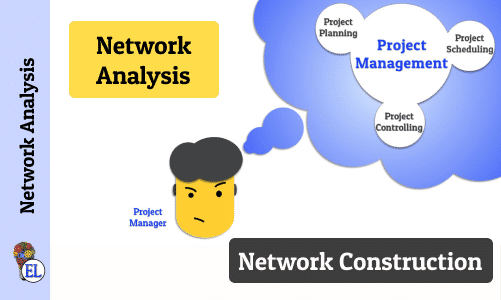
Network Analysis - Dealing with Network Construction Basics
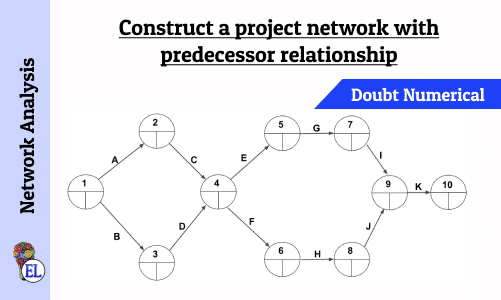
Construct a project network with predecessor relationship | Operation Research | Numerical
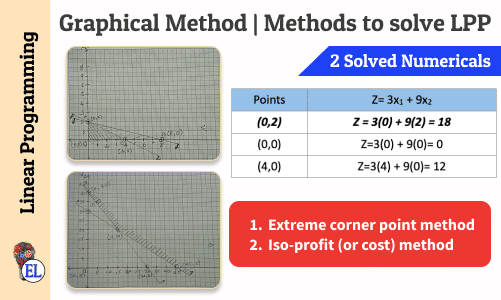
Graphical Method | Methods to solve LPP | Linear Programming
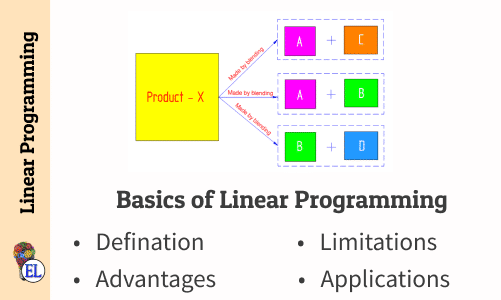
Basics of Linear Programming
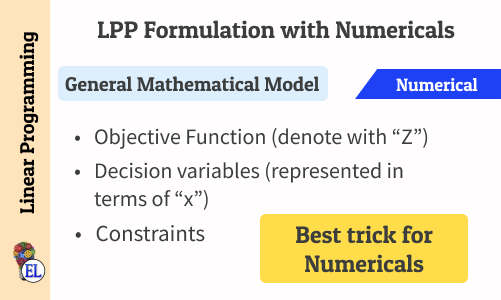
Linear Programming Problem (LPP) Formulation with Numericals

All comments that you add will await moderation. We'll publish all comments that are topic related, and adhere to our Code of Conduct .
Want to tell us something privately? Contact Us
Post comment
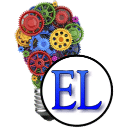
Education Lessons
Stay in touch, [notes] operation research, [notes] dynamics of machinery, [notes] maths, [notes] science, [notes] computer aided design.
Linear Programming Problems and Questions
How are linear programming problems and word problems solved? Below are links to many examples on how to formulate and solve optimization problems in linear programming.
- Solve Inequalities with Two Variables .
- Solve Systems of Inequalities with Two Variables .
- Linear Programming and Optimization .
- Linear Programming: Word Problems and Applications .
- School Guide
- Mathematics
- Number System and Arithmetic
- Trigonometry
- Probability
- Mensuration
- Maths Formulas
- Class 8 Maths Notes
- Class 9 Maths Notes
- Class 10 Maths Notes
- Class 11 Maths Notes
- Class 12 Maths Notes
- Graphical Solution of Linear Programming Problems
- Linear Programming
- Solving Linear Inequalities Word Problems
- Stars and Bars Algorithms for Competitive Programming
- Python | Linear search on list or tuples
- Top 50 Dynamic Programming Coding Problems for Interviews
- Dynamic Programming (DP) Tutorial with Problems
- Program to find all types of Matrix
- Dynamic Programming | High-effort vs. Low-effort Tasks Problem
- Python | Linear Programming in Pulp
- C++ Program for the Fractional Knapsack Problem
- Transportation Problem | Set 1 (Introduction)
- Algorithms | Dynamic Programming | Question 7
- Algorithms | Dynamic Programming | Question 4
- Algorithms | Dynamic Programming | Question 3
- Algorithms | Dynamic Programming | Question 2
- How to begin with Competitive Programming?
- Dynamic Programming (DP) on Grids
- Knuth's Optimization in Dynamic Programming
Types of Linear Programming Problems
Linear programming is a mathematical technique for optimizing operations under a given set of constraints. The basic goal of linear programming is to maximize or minimize the total numerical value. It is regarded as one of the most essential strategies for determining optimum resource utilization. Linear programming challenges include a variety of problems involving cost minimization and profit maximization, among others. They will be briefly discussed in this article.
The purpose of this article is to provide students with a comprehensive understanding of the different types of linear programming problems and their solutions.
What is Linear Programming?
Linear programming (LP) is a mathematical optimization technique used to maximize or minimize a linear objective function, subject to a set of linear equality and inequality constraints. It is widely used in various fields such as economics, engineering, operations research, and management science to find the best possible outcome given limited resources.
Components of Linear Programming
Components of linear programming include:
- Objective Function: This is a linear function that needs to be optimized (maximized or minimized). It represents the quantity to be maximized or minimized, such as profit, cost, time, etc.
- Decision Variables: These are the variables that represent the choices or decisions to be made. They are the unknown quantities that the optimization process seeks to determine. Decision variables must be continuous and can take any real value within a specified range.
- Constraints: These are restrictions or limitations on the decision variables that must be satisfied. Constraints can be expressed as linear equations or inequalities. They represent the limitations imposed by available resources, capacity constraints, demand requirements, etc.
- Feasible Region: The feasible region is the set of all possible combinations of decision variables that satisfy all constraints. It is defined by the intersection of the constraint boundaries.
- Optimal Solution: This is the best possible solution that maximizes or minimizes the objective function while satisfying all constraints. In graphical terms, it is the point within the feasible region that maximizes or minimizes the objective function.
Linear programming provides a systematic and efficient approach to decision-making in situations where resources are limited and objectives need to be optimized.
Different Types of Linear Programming Problems
The following are the types of linear programming problems:
- Manufacturing problems
- Diet problems
- Transportation problems
- Optimal alignment problem
Let’s discuss more about each of them.
Manufacturing Problems
In these problems, we evaluate the number of units of various items that should be produced and sold by a company when each product requires a given number of workforce, machine hours, labour hours per unit of product, warehouse space per unit of output, and so on, to maximize profit.
Manufacturing problems involve maximizing the production rate or net profits of manufactured products, which might measure the available workspace, the number of workers, machine hours, packing materials used, raw materials required, the product’s market value, and other factors. These are commonly used in the industrial sector to anticipate a company’s future capital increase over time.
Diet Problems
In these challenges, we assess how many components or nutrients a diet should contain in order to lower the cost of the desired diet while guaranteeing the minimal amount of each vitamin.
As the name suggests, diet-related problems can be resolved by eating more particular foods that are rich in essential nutrients and can support the adoption of a particular diet plan. Finding a set of meals that will satisfy a set of daily nutritional demands for the least amount of money is the aim of a diet problem.
Transportation Problems
In these problems , we create a transportation schedule to discover the most cost-effective method of carrying a product from various plants/factories to various markets.
The study of transportation routes or how items from diverse production sources are transported to various markets to minimize the total transportation cost is linked to transportation difficulties. Analyzing such challenges is crucial for large firms with several production units and a broad customer base.
Optimal Assignment Problems
This problem addresses a company’s completion of a given task/assignment by selecting a specific number of employees to complete the assignment within the required timeframe, assuming that each person works on only one job. Event planning and management in major organizations, for example, are examples of such problems.
Constraints and Objective Function of Each Linear Programming Problem
Steps for solving linear programming problems.
Step 1: Identify the decision variables : The first step is to determine which choice factors control the behaviour of the objective function. A function that needs to be optimised is an objective function. Determine the decision variables and designate them with X, Y, and Z symbols.
Step 2: Form an objective function : Using the decision variables, write out an algebraic expression that displays the quantity we aim to maximize.
Step 3: Identify the constraints : Choose and write the given linear inequalities from the data.
Step 4: Draw the graph for the given data : Construct the graph by using constraints for solving the linear programming problem.
Step 5: Draw the feasible region : Every constraint on the problem is satisfied by this portion of the graph. Anywhere in the feasible zone is a viable solution for the objective function.
Step 6: Choosing the optimal point : Choose the point for which the given function has maximum or minimum values.
Solved Problems of Linear Programming Problems
Question 1. A factory manufactures two types of gadgets, regular and premium. Each gadget requires the use of two operations, assembly and finishing, and there are at most 12 hours available for each operation. A regular gadget requires 1 hour of assembly and 2 hours of finishing, while a premium gadget needs 2 hours of assembly and 1 hour of finishing. Due to other restrictions, the company can make at most 7 gadgets a day. If a profit of $20 is realized for each regular gadget and $30 for a premium gadget, how many of each should be manufactured to maximize profit?
We define our unknowns:
Let the number of regular gadgets manufactured each day = x
and the number of premium gadgets manufactured each day = y
The objective function is
P = 20x + 30y
We now write the constraints. The fourth sentence states that the company can make at most 7 gadgets a day. This translates as
Since the regular gadget requires one hour of assembly and the premium gadget requires two hours of assembly, and there are at most 12 hours available for this operation, we get
x + 2y ≤ 12
Similarly, the regular gadget requires two hours of finishing and the premium gadget one hour. Again, there are at most 12 hours available for finishing. This gives us the following constraint.
2x + y ≤ 12
The fact that x and y can never be negative is represented by the following two constraints:
x ≥ 0, and y ≥ 0.
We have formulated the problem as follows :
Maximize P=20x + 30y Subject to : x + y ≤ 7, x + 2y ≤ 122, x + y ≤ 12, x ≥ 0, y ≥ 0
In order to solve the problem, we next graph the constraints and feasible region.
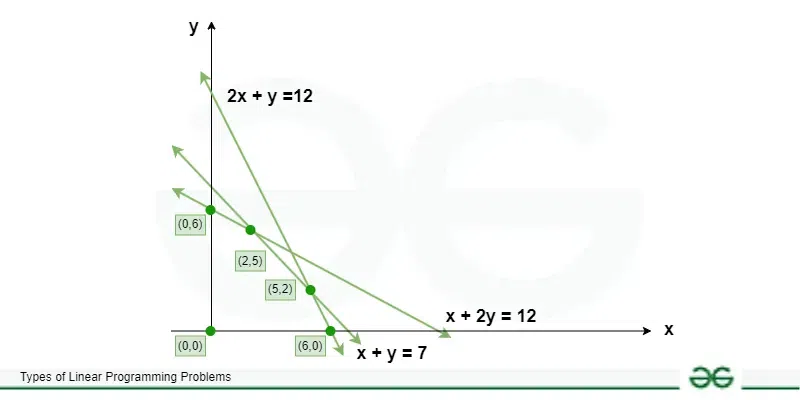
Again, we have shaded the feasible region, where all constraints are satisfied.
Since the extreme value of the objective function always takes place at the vertices of the feasible region, we identify all the critical points. They are listed as (0, 0), (0, 6), (2, 5), (5, 2), and (6, 0). To maximize profit, we will substitute these points in the objective function to see which point gives us the maximum profit each day. The results are listed below.
FAQ on Linear programming
How many methods are there in lpp.
There are different methods to solve a linear programming problem. Such as Graphical method, Simplex method, Ellipsoid method, Interior point methods.
What are the four 4 special cases in linear programming?
Four special cases and difficulties arise at times when using the graphical approach to solving LP problems: (1) infeasibility, (2) unboundedness, (3) redundancy, and (4) alternate optimal solutions.
What are the 3 components of linear programming?
The basic components of the LP are as follows: Decision Variables. Constraints. Objective Functions.
What are the applications of LPP?
LPP applications may include production scheduling, inventory policies, investment portfolio, allocation of advertising budget, construction of warehouses, etc.
What are the limitations of LPP?
Constraints (limitations) should be expressed in mathematical form. Relationships between two or more variables should be linear. The values of the variables should always be non-negative or zero. There should always be finite and infinite inputs and output numbers.
Please Login to comment...
Similar reads.
- Maths-Class-12
- School Learning
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

COMMENTS
Assignment Problem. A problem that requires pairing two sets of items given a set of paired costs or profit in such a way that the total cost of the pairings is minimized or maximized. The assignment problem is a special case of linear programming. For example, an operation manager needs to assign four jobs to four machines.
Worked example of assigning tasks to an unequal number of workers using the Hungarian method. The assignment problem is a fundamental combinatorial optimization problem. In its most general form, the problem is as follows: The problem instance has a number of agents and a number of tasks.Any agent can be assigned to perform any task, incurring some cost that may vary depending on the agent ...
Linear Programming Examples. In this section, you'll see two examples of linear programming problems: A small problem that illustrates what linear programming is; A practical problem related to resource allocation that illustrates linear programming concepts in a real-world scenario; You'll use Python to solve these two problems in the next ...
Step 1: Mark the decision variables in the problem. Step 2: Build the objective function of the problem and check if the function needs to be minimized or maximized. Step 3: Write down all the constraints of the linear problems. Step 4: Ensure non-negative restrictions of the decision variables.
Formulating Linear Programming Models LP Example #4 (Assignment Problem) The coach of a swim team needs to assign swimmers to a 200-yard medley relay team (four swimmers, each swims 50 yards of one of the four strokes). Since most of the best swimmers are very fast in more than one stroke, it is not clear which
In which we introduce linear programming. 1 Linear Programming A linear program is an optimization problem in which we have a collection of variables, ... to an assignment is called the cost of the assignment. For example, x 1:= 1 3 and x 2:= 1 3 is a feasible solution, of cost 2 3. Note that if x 1;x
Enhance accuracy of solving linear systems of equations. 4 Bipartite matching. Can solve via reduction to max flow. Flow. During Ford-Fulkerson, all capacities and flows are 0/1. Flow ... Equivalent Assignment Problem c(x, y) 00312 01015 43330 00110 12204 cp(x, y) 3891510 41071614 913111910 813122013 175119 8 13 11 19 13 5 4 3 0 8 9 + 8 - 13 10
1.2 Concepts in Linear Programming The term linear programming arises from the fact that the objective function is a linear combination of decision variables and parameters that one seeks to maximize or minimize. For example, classic problems seek to maximize profits and flow and to minimize cost or time. The
Assignment problem is a special type of linear programming problem which deals with the allocation of the various resources to the various activities on one to one basis. It does it in such a way that the cost or time involved in the process is minimum and profit or sale is maximum. Though there problems can be solved by simplex method or by ...
Lecture 15: Linear Programming. Linear programming (LP) is a method to achieve the optimum outcome under some requirements represented by linear relationships. More precisely, LP can solve the problem of maximizing or minimizing a linear objective function subject to some linear constraints. In general, the standard form of LP consists of.
Assignment Problem: Linear Programming. The assignment problem is a special type of transportation problem, where the objective is to minimize the cost or time of completing a number of jobs by a number of persons. In other words, when the problem involves the allocation of n different facilities to n different tasks, it is often termed as an ...
Time complexity : O(n^3), where n is the number of workers and jobs. This is because the algorithm implements the Hungarian algorithm, which is known to have a time complexity of O(n^3). Space complexity : O(n^2), where n is the number of workers and jobs.This is because the algorithm uses a 2D cost matrix of size n x n to store the costs of assigning each worker to a job, and additional ...
Linear Programming Transportation and Assignment problems are taught by example with the best tips and techniques. The Least Cost Method (also called the min...
Linear Programming deals with the problem of optimizing a linear objective function subject to linear equality and inequality constraints on the decision variables. ... A certain amount of each nutrient is required per day. For example, here is the data corresponding to a civilization with just two types of grains (G1 and G2) and three types of ...
For the standard maximization linear programming problems, constraints are of the form: ax + by ≤ c a x + b y ≤ c. Since the variables are non-negative, we include the constraints: x ≥ 0 x ≥ 0; y ≥ 0 y ≥ 0. Graph the constraints. Shade the feasible region. Find the corner points.
Assignment Problem is a special type of linear programming problem where the objective is to minimise the cost or time of completing a number of jobs by a number of persons. The assignment problem in the general form can be stated as follows: "Given n facilities, n jobs and the effectiveness of each facility for each job, the problem is to ...
This section presents an example that shows how to solve an assignment problem using both the MIP solver and the CP-SAT solver. Example. In the example there are five workers (numbered 0-4) and four tasks (numbered 0-3). Note that there is one more worker than in the example in the Overview.
The Quadratic Assignment Problem (QAP), ... As an example, ... plants as well as the volume of goods between locations to maximize revenue is what distinguishes this from other linear programming assignment problems like the Knapsack Problem. The Backboard Wiring Problem.
LINEAR PROGRAMMING: EXERCISES - V. Kostoglou 18 PROBLEM 10 Solve using the Simplex method, the following linear programming problem: max f(X) = 7/6x 1 + 13/10x 2 with structure limitations : x 1 /30 + x 2 /40 1 x 1 /28 + x 2 /35 1 x 1 /30 + x 2 /25 1 and x 1, x 2 0
The assignment problem represents a special case of linear programming problem used for allocating resources (mostly workforce) in an optimal way; it is a highly useful tool for operation and project managers for optimizing costs. The lpSolve R package allows us to solve LP assignment problems with just very few lines of code.
There are two main conditions for applying Hungarian Method: (1) Square Matrix (n x n). (2) Problem should be of minimization type. Assignment model is a special application of Linear Programming Problem (LPP), in which the main objective is to assign the work or task to a group of individuals such that; i) There is only one assignment.
Below are links to many examples on how to formulate and solve optimization problems in linear programming. Solve Inequalities with Two Variables . Solve Systems of Inequalities with Two Variables . Linear Programming and Optimization . Linear Programming: Word Problems and Applications . Mathematics Tutorials and Problems. {ezoic-ad-1}
What is Linear Programming? Linear programming (LP) is a mathematical optimization technique used to maximize or minimize a linear objective function, subject to a set of linear equality and inequality constraints. It is widely used in various fields such as economics, engineering, operations research, and management science to find the best possible outcome given limited resources.