Home » JavaScript Tutorial » JavaScript Assignment Operators

JavaScript Assignment Operators
Summary : in this tutorial, you will learn how to use JavaScript assignment operators to assign a value to a variable.
Introduction to JavaScript assignment operators
An assignment operator ( = ) assigns a value to a variable. The syntax of the assignment operator is as follows:
In this syntax, JavaScript evaluates the expression b first and assigns the result to the variable a .
The following example declares the counter variable and initializes its value to zero:
The following example increases the counter variable by one and assigns the result to the counter variable:
When evaluating the second statement, JavaScript evaluates the expression on the right-hand first ( counter + 1 ) and assigns the result to the counter variable. After the second assignment, the counter variable is 1 .
To make the code more concise, you can use the += operator like this:
In this syntax, you don’t have to repeat the counter variable twice in the assignment.
The following table illustrates assignment operators that are shorthand for another operator and the assignment:
Destructuring assignment
The two most used data structures in JavaScript are Object and Array .
- Objects allow us to create a single entity that stores data items by key.
- Arrays allow us to gather data items into an ordered list.
However, when we pass these to a function, we may not need all of it. The function might only require certain elements or properties.
Destructuring assignment is a special syntax that allows us to “unpack” arrays or objects into a bunch of variables, as sometimes that’s more convenient.
Destructuring also works well with complex functions that have a lot of parameters, default values, and so on. Soon we’ll see that.
Array destructuring
Here’s an example of how an array is destructured into variables:
Now we can work with variables instead of array members.
It looks great when combined with split or other array-returning methods:
As you can see, the syntax is simple. There are several peculiar details though. Let’s see more examples to understand it better.
It’s called “destructuring assignment,” because it “destructurizes” by copying items into variables. However, the array itself is not modified.
It’s just a shorter way to write:
Unwanted elements of the array can also be thrown away via an extra comma:
In the code above, the second element of the array is skipped, the third one is assigned to title , and the rest of the array items are also skipped (as there are no variables for them).
…Actually, we can use it with any iterable, not only arrays:
That works, because internally a destructuring assignment works by iterating over the right value. It’s a kind of syntax sugar for calling for..of over the value to the right of = and assigning the values.
We can use any “assignables” on the left side.
For instance, an object property:
In the previous chapter, we saw the Object.entries(obj) method.
We can use it with destructuring to loop over the keys-and-values of an object:
The similar code for a Map is simpler, as it’s iterable:
There’s a well-known trick for swapping values of two variables using a destructuring assignment:
Here we create a temporary array of two variables and immediately destructure it in swapped order.
We can swap more than two variables this way.
The rest ‘…’
Usually, if the array is longer than the list at the left, the “extra” items are omitted.
For example, here only two items are taken, and the rest is just ignored:
If we’d like also to gather all that follows – we can add one more parameter that gets “the rest” using three dots "..." :
The value of rest is the array of the remaining array elements.
We can use any other variable name in place of rest , just make sure it has three dots before it and goes last in the destructuring assignment.
Default values
If the array is shorter than the list of variables on the left, there will be no errors. Absent values are considered undefined:
If we want a “default” value to replace the missing one, we can provide it using = :
Default values can be more complex expressions or even function calls. They are evaluated only if the value is not provided.
For instance, here we use the prompt function for two defaults:
Please note: the prompt will run only for the missing value ( surname ).
Object destructuring
The destructuring assignment also works with objects.
The basic syntax is:
We should have an existing object on the right side, that we want to split into variables. The left side contains an object-like “pattern” for corresponding properties. In the simplest case, that’s a list of variable names in {...} .
For instance:
Properties options.title , options.width and options.height are assigned to the corresponding variables.
The order does not matter. This works too:
The pattern on the left side may be more complex and specify the mapping between properties and variables.
If we want to assign a property to a variable with another name, for instance, make options.width go into the variable named w , then we can set the variable name using a colon:
The colon shows “what : goes where”. In the example above the property width goes to w , property height goes to h , and title is assigned to the same name.
For potentially missing properties we can set default values using "=" , like this:
Just like with arrays or function parameters, default values can be any expressions or even function calls. They will be evaluated if the value is not provided.
In the code below prompt asks for width , but not for title :
We also can combine both the colon and equality:
If we have a complex object with many properties, we can extract only what we need:
The rest pattern “…”
What if the object has more properties than we have variables? Can we take some and then assign the “rest” somewhere?
We can use the rest pattern, just like we did with arrays. It’s not supported by some older browsers (IE, use Babel to polyfill it), but works in modern ones.
It looks like this:
In the examples above variables were declared right in the assignment: let {…} = {…} . Of course, we could use existing variables too, without let . But there’s a catch.
This won’t work:
The problem is that JavaScript treats {...} in the main code flow (not inside another expression) as a code block. Such code blocks can be used to group statements, like this:
So here JavaScript assumes that we have a code block, that’s why there’s an error. We want destructuring instead.
To show JavaScript that it’s not a code block, we can wrap the expression in parentheses (...) :
Nested destructuring
If an object or an array contains other nested objects and arrays, we can use more complex left-side patterns to extract deeper portions.
In the code below options has another object in the property size and an array in the property items . The pattern on the left side of the assignment has the same structure to extract values from them:
All properties of options object except extra which is absent in the left part, are assigned to corresponding variables:
Finally, we have width , height , item1 , item2 and title from the default value.
Note that there are no variables for size and items , as we take their content instead.
Smart function parameters
There are times when a function has many parameters, most of which are optional. That’s especially true for user interfaces. Imagine a function that creates a menu. It may have a width, a height, a title, an item list and so on.
Here’s a bad way to write such a function:
In real-life, the problem is how to remember the order of arguments. Usually, IDEs try to help us, especially if the code is well-documented, but still… Another problem is how to call a function when most parameters are ok by default.
That’s ugly. And becomes unreadable when we deal with more parameters.
Destructuring comes to the rescue!
We can pass parameters as an object, and the function immediately destructurizes them into variables:
We can also use more complex destructuring with nested objects and colon mappings:
The full syntax is the same as for a destructuring assignment:
Then, for an object of parameters, there will be a variable varName for the property incomingProperty , with defaultValue by default.
Please note that such destructuring assumes that showMenu() does have an argument. If we want all values by default, then we should specify an empty object:
We can fix this by making {} the default value for the whole object of parameters:
In the code above, the whole arguments object is {} by default, so there’s always something to destructurize.
Destructuring assignment allows for instantly mapping an object or array onto many variables.
The full object syntax:
This means that property prop should go into the variable varName and, if no such property exists, then the default value should be used.
Object properties that have no mapping are copied to the rest object.
The full array syntax:
The first item goes to item1 ; the second goes into item2 , and all the rest makes the array rest .
It’s possible to extract data from nested arrays/objects, for that the left side must have the same structure as the right one.
We have an object:
Write the destructuring assignment that reads:
- name property into the variable name .
- years property into the variable age .
- isAdmin property into the variable isAdmin (false, if no such property)
Here’s an example of the values after your assignment:
The maximal salary
There is a salaries object:
Create the function topSalary(salaries) that returns the name of the top-paid person.
- If salaries is empty, it should return null .
- If there are multiple top-paid persons, return any of them.
P.S. Use Object.entries and destructuring to iterate over key/value pairs.
Open a sandbox with tests.
Open the solution with tests in a sandbox.
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript tutorial.
JavaScript is the world's most popular programming language.
JavaScript is the programming language of the Web.
JavaScript is easy to learn.
This tutorial will teach you JavaScript from basic to advanced.
Examples in Each Chapter
With our "Try it Yourself" editor, you can edit the source code and view the result.
My First JavaScript
Try it Yourself »
Use the Menu
We recommend reading this tutorial, in the sequence listed in the menu.
If you have a large screen, the menu will always be present on the left.
If you have a small screen, open the menu by clicking the top menu sign ☰ .
Learn by Examples
Examples are better than 1000 words. Examples are often easier to understand than text explanations.
This tutorial supplements all explanations with clarifying "Try it Yourself" examples.
If you try all the examples, you will learn a lot about JavaScript, in a very short time!
Why Study JavaScript?
JavaScript is one of the 3 languages all web developers must learn:
1. HTML to define the content of web pages
2. CSS to specify the layout of web pages
3. JavaScript to program the behavior of web pages
This tutorial covers every version of JavaScript:
- The Original JavaScript ES1 ES2 ES3 (1997-1999)
- The First Main Revision ES5 (2009)
- The Second Revision ES6 (2015)
- The Yearly Additions (2016, 2017 ... 2021, 2022)
Advertisement
Learning Speed
In this tutorial, the learning speed is your choice.
Everything is up to you.
If you are struggling, take a break, or re-read the material.
Always make sure you understand all the "Try-it-Yourself" examples.
The only way to become a clever programmer is to: Practice. Practice. Practice. Code. Code. Code !
Test Yourself With Exercises
Create a variable called carName and assign the value Volvo to it.
Start the Exercise
Commonly Asked Questions
- How do I get JavaScript?
- Where can I download JavaScript?
- Is JavaScript Free?
You don't have to get or download JavaScript.
JavaScript is already running in your browser on your computer, on your tablet, and on your smart-phone.
JavaScript is free to use for everyone.
My Learning
Track your progress with the free "My Learning" program here at W3Schools.
Log in to your account, and start earning points!
This is an optional feature. You can study at W3Schools without using My Learning.

JavaScript References
W3Schools maintains a complete JavaScript reference, including all HTML and browser objects.
The reference contains examples for all properties, methods and events, and is continuously updated according to the latest web standards.
JavaScript Quiz Test
Test your JavaScript skills at W3Schools!
Start JavaScript Quiz!
JavaScript Exam - Get Your Diploma!
Kickstart your career.
Get certified by completing the course

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Free Javascript challenges
Learn Javascript online by solving coding exercises.
Javascript for all levels
Solve Javascript tasks from beginner to advanced levels.
Accross various subjects
Select your topic of interest and start practicing.
Start your learning path here
Why jschallenger, a hands-on javascript experience.
JSchallenger provides a variety of JavaScript exercises, including coding tasks, coding challenges, lessons, and quizzes.
Structured learning path
JSchallenger provides a structured learning path that gradually increases in complexity. Build your skills and knowledge at your own pace.
Build a learning streak
JSchallenger saves your learning progress. This feature helps to stay motivated and makes it easy for you to pick up where you left off.
Type and execute code yourself
Type real JavaScript and see the output of your code. JSchallenger provides syntax highlighting for easy understanding.
Join 1.000s of users around the world
Most popular challenges, most failed challenges, what users say about jschallenger.
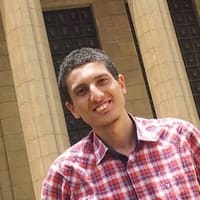
Mohamed Ibrahim
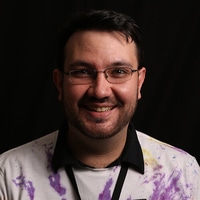
Tobin Shields
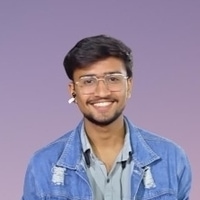
The Online Coding & Design School With A
- Entry Level Tech Jobs
- Front End Developer
- Full Stack Developer
- UI/UX Designer
- Learn to Code
- Get Hired in Tech
21 Easy JavaScript Projects for Beginners (Code included!)
Looking to get some practice with JavaScript or to build your portfolio to impress hiring managers? You can level up your JavaScript skills with our instructor approved, curated project tutorials.
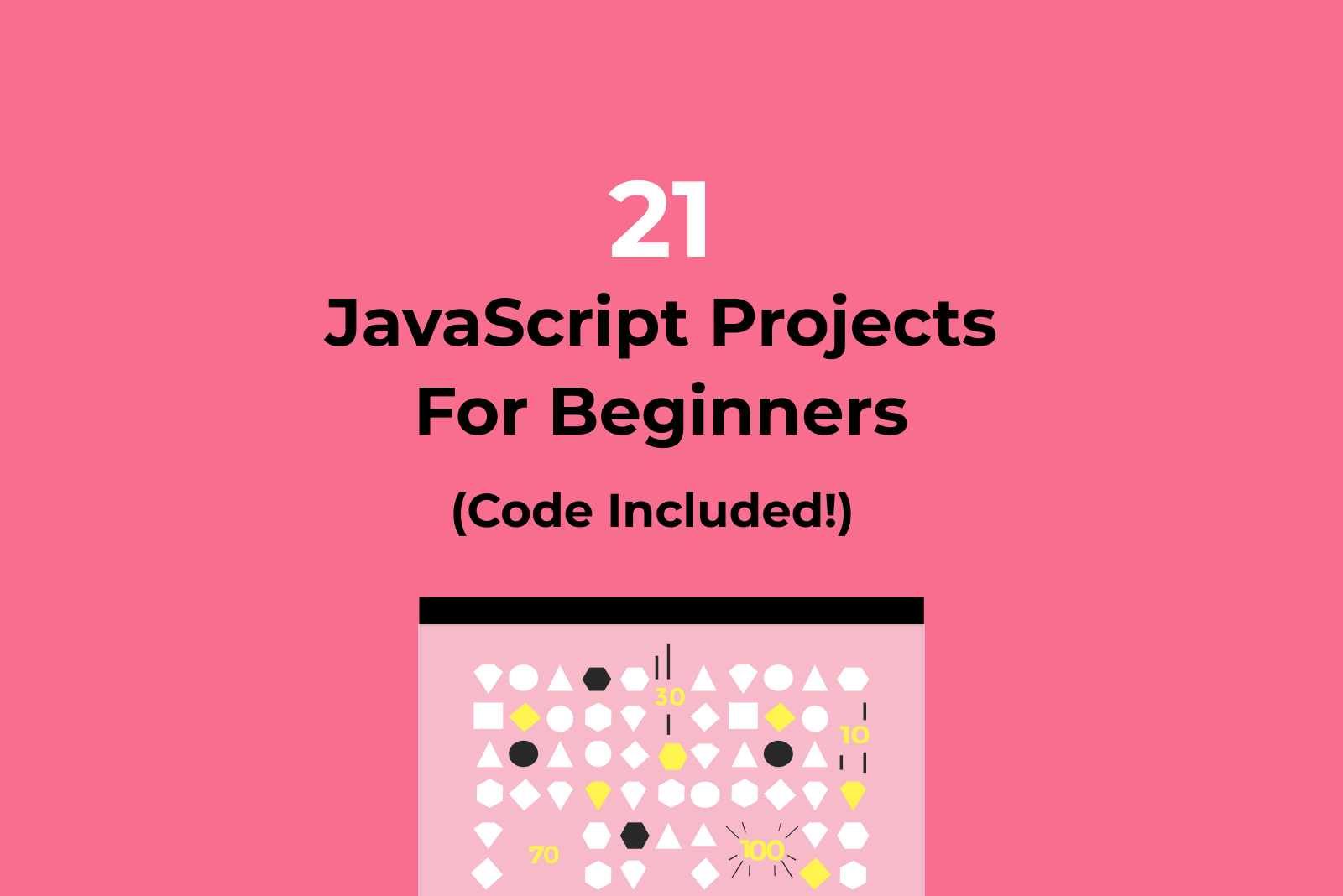
According to the 2023 Stack Overflow Developer Survey, JavaScript has spent eleven years in a row as the most commonly used programming language, with 66% of developers using it extensively. If you’re interested in becoming a web developer ( front end, back end, or full stack ), JavaScript is one of the best programming languages you can learn. One way to do that is to do some JavaScript projects for beginners.
Vanilla JavaScript, also known as “plain” JavaScript — meaning without any frameworks — is one of the foundations of coding and web development. Most web pages you see are built using a combination of HTML, CSS, vanilla JavaScript, and API calls.
Getting familiar with JavaScript basics means using those JavaScript skills to build JavaScript practice projects. Luckily, we know some fun JavaScript beginner projects you can do to hone your skills from the thousands of students we’ve trained in our front end development job training program, Break into Tech .
What we see with our successful students who have landed jobs at companies like GoDaddy, Toast, Asics Digital, 1Password, Figure and Apple is that they all have JavaScript practice projects, portfolios that show off their front end developer skills , and are very well versed in JavaScript. Doing JavaScript projects for beginners is a great way to get some practice and build your portfolio.
If you want to practice your front end developer skills, we’ve put together a list of 20+ JavaScript beginner projects you can start working on right now. When you find a JavaScript practice project that piques your interest, follow the project link. Each of these open source JavaScript projects for beginners (and above) have their source code listed on their homepage for you to use as a guide.
Lisa Savoie, one of Skillcrush’s JavaScript instructors, recommends reading the source code out loud to understand it better, looking for features to incorporate into other projects , or even retyping it to work on your muscle memory of how to write functions, variables, and loops.
She says, “You can Google methods you’re unfamiliar with to learn more about them or even break the code and fix it — It’s fun to break things😀.”
Table of Contents
- JavaScript Projects Common Mistakes
- Beginner JavaScript Projects
- Mouseover Element
- Magic 8 Ball
- Build a To-Do List
- Epic Mix Playlist
- Speech Detection
- Sticky Navigation
- Geolocation
Intermediate JavaScript Projects
- Election Map
- Login Authentication
- Guess the Word
- Terminalizer
- Tic Tac Toe Game
- Hotel Booking App
- Advanced JavaScript Project
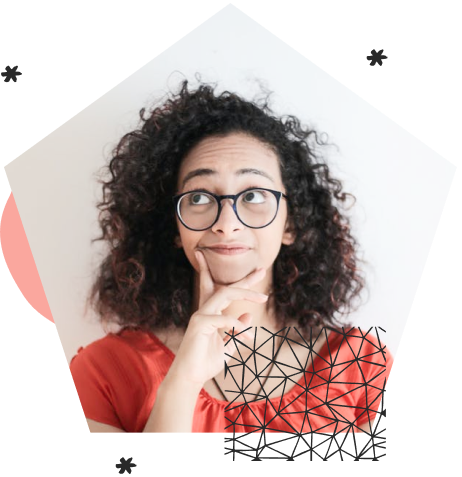
Want to learn to code? Join our FREE Coding Camp!
You Will Learn: HTML & CSS JavaScript User Experience Design Python—the language of AI 🤖 PLUS How to decide what tech job role is right for you!
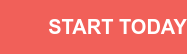
JavaScript Beginner Projects Common Mistakes
- Read what the console is telling you, especially if you keep getting error messages
- Are you working with the right type of variable? Is var, let, or const the right choice?
- Is your logic sound? Are you properly using your array methods (when those are appropriate)?
- Are you factoring in edge cases like empty inputs or negative integers?
- Are you passing the right kind/type of argument to a function?
JavaScript Projects for Beginners
1. mouseover element.
Why do this project?
- You’ll see how JavaScript functions work and practice your JavaScript logic
- It’s a fun beginner JavaScript project to do to liven up your user experience
- Learn more about using random, functions, and event listeners.
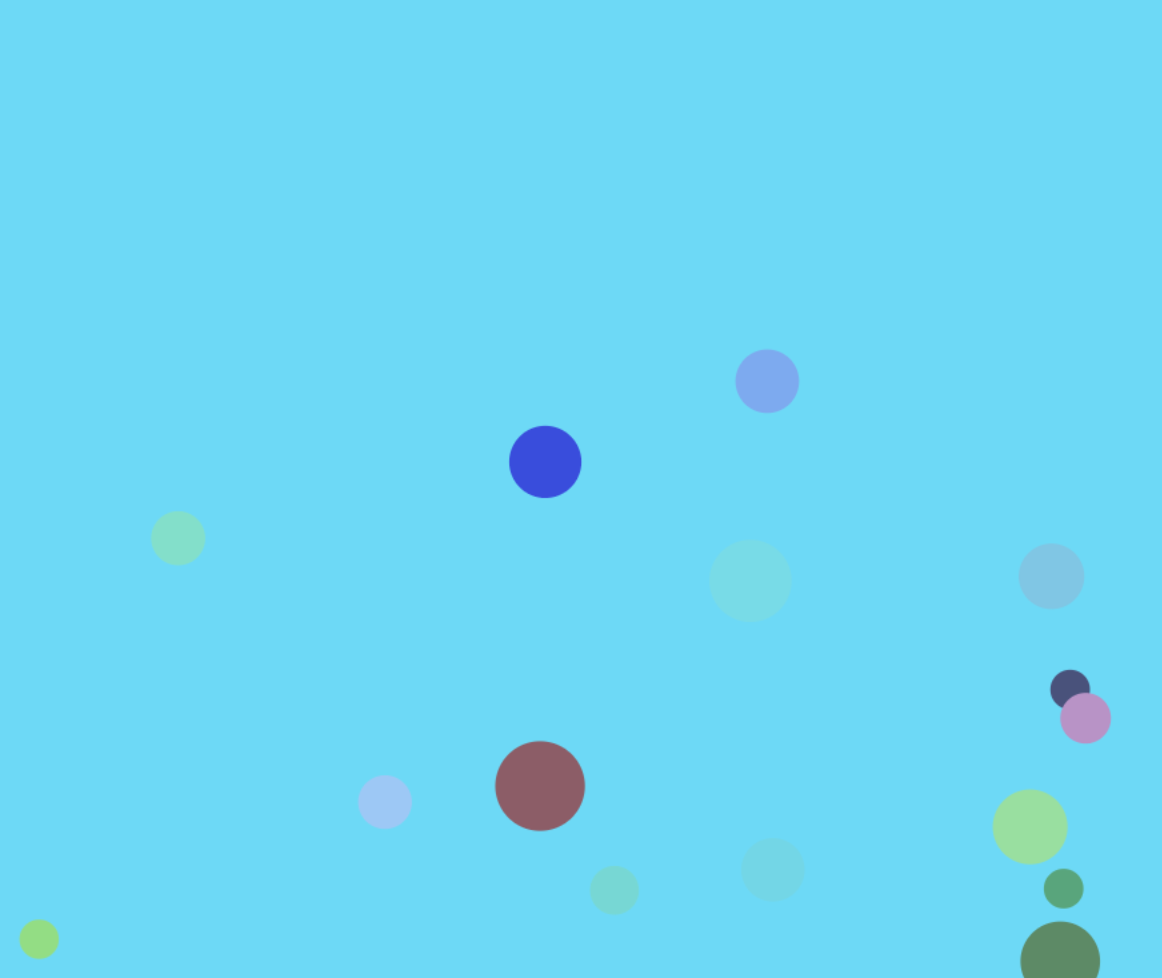
Mouseover Element Source Code
Key concepts covered:
- Functions and if-else statements
- Event listeners
What to do:
- Copy the source code from CodePen.
- Implement the JavaScript code so that when you hover your mouse over a colored ball, it gets bigger.
Bonus Challenge:
To test your understanding of the concepts behind the mouseover element, your bonus challenge is this custom cursor project .
2. JavaScript Clock
- You get hands-on with the kind of actual work you’ll be doing as a JavaScript developer
- You’ll apply key JavaScript concepts and have an adorable project for your portfolio
- Practice concepts like variables, conditional logic, and date API
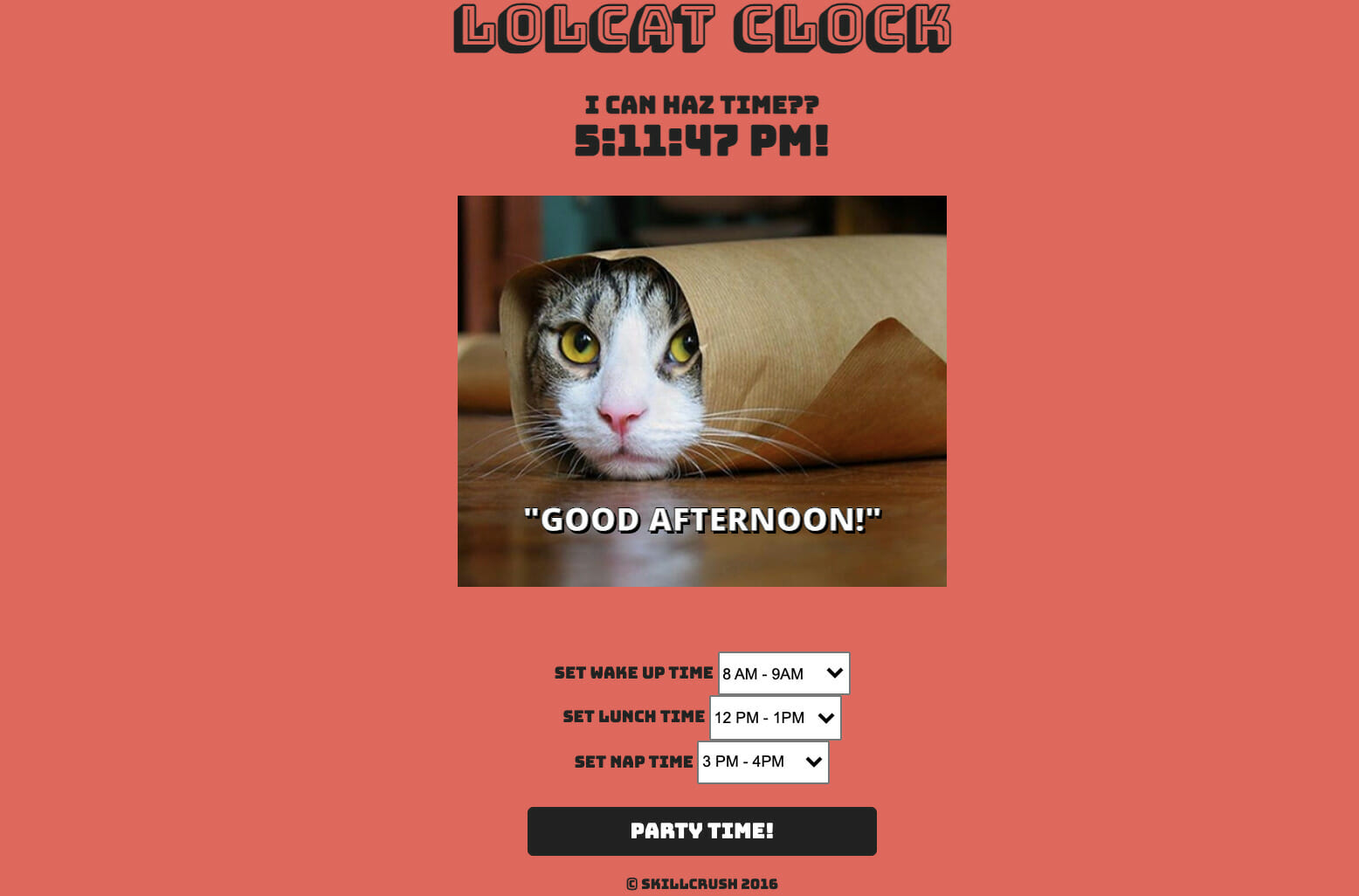
JavaScript Clock Source Code
- Conditional logic
- Program flow
- DOM elements
- Implement the JavaScript code so that every time you click the “Party Time!” button in the time range that you specify, you get a different LOLcat. You can set different times to see different images — there are four images total.
Bonus challenge: Now that you’ve mastered the Lolcat JavaScript clock, challenge yourself by building your own countdown timer.

3. Magic 8 Ball
- It gives you a solid foundation for how to use Math.random to produce randomized results for JavaScript projects
- You can use this project to impress your friends and make small life decisions
- Learn more about how to use the random function, nested functions, and event listeners
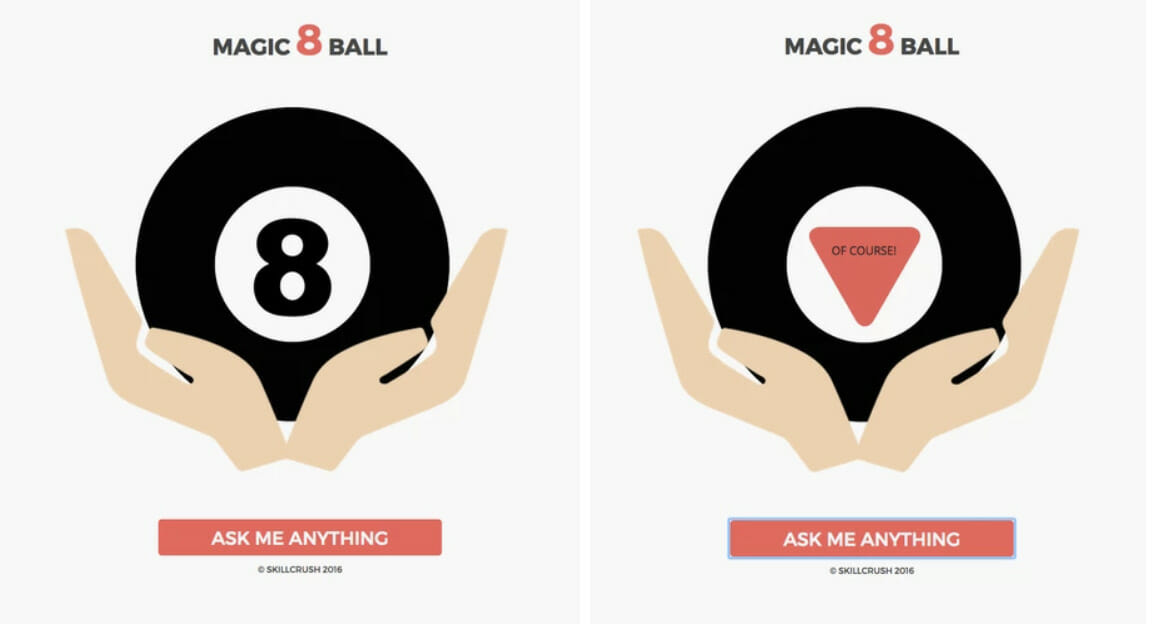
Magic 8 Ball Source Code
- Math.random
- Nested functions
- Copy the source code from GitHub for HTML and CSS.
- Implement the JavaScript code so that you can grab the 8 ball, ask it a question, shake it, and have the 8 ball respond with a somewhat enigmatic, somewhat applicable answer.
To test your understanding of the concepts behind the Magic 8 Ball, your bonus challenge is this dad joke generator.
4. To-Do List
- You’ll beef up your skills at coding interactive lists, which lets users add, remove, and group items
- You can use this beginner JavaScript project in your daily life and add it to your portfolio
- Learn more about how to use arrays, nested functions, and local storage API
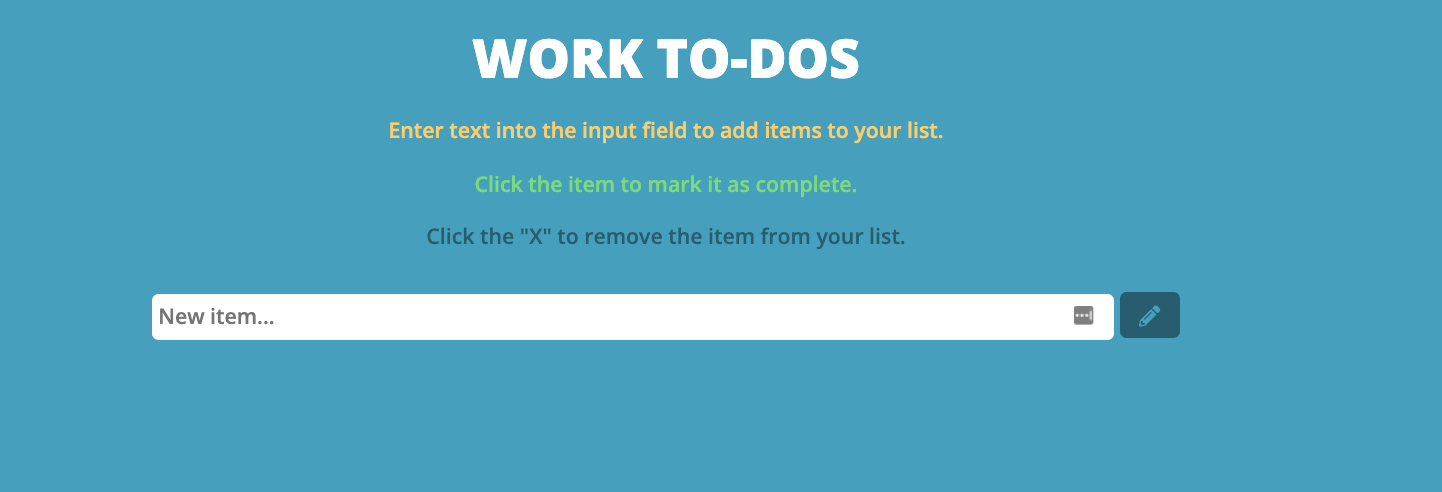
To-Do List Source Code
- Local storage API
- DOM manipulation
- Implement the JavaScript code so that you can add new items and use the buttons to toggle completed items, edit items, or delete items.
To test your understanding of the concepts behind the To-Do list, your bonus challenge is this JavaScript grocery list.
5. Epic Mix Playlist
- It helps you practice core JavaScript skills, such as forEach loops and event listeners, and work with functions and lists
- You can use this project to show people looking at your portfolio your great taste in music
- Learn more about how to classList, innerHTML, forEach, and template literals
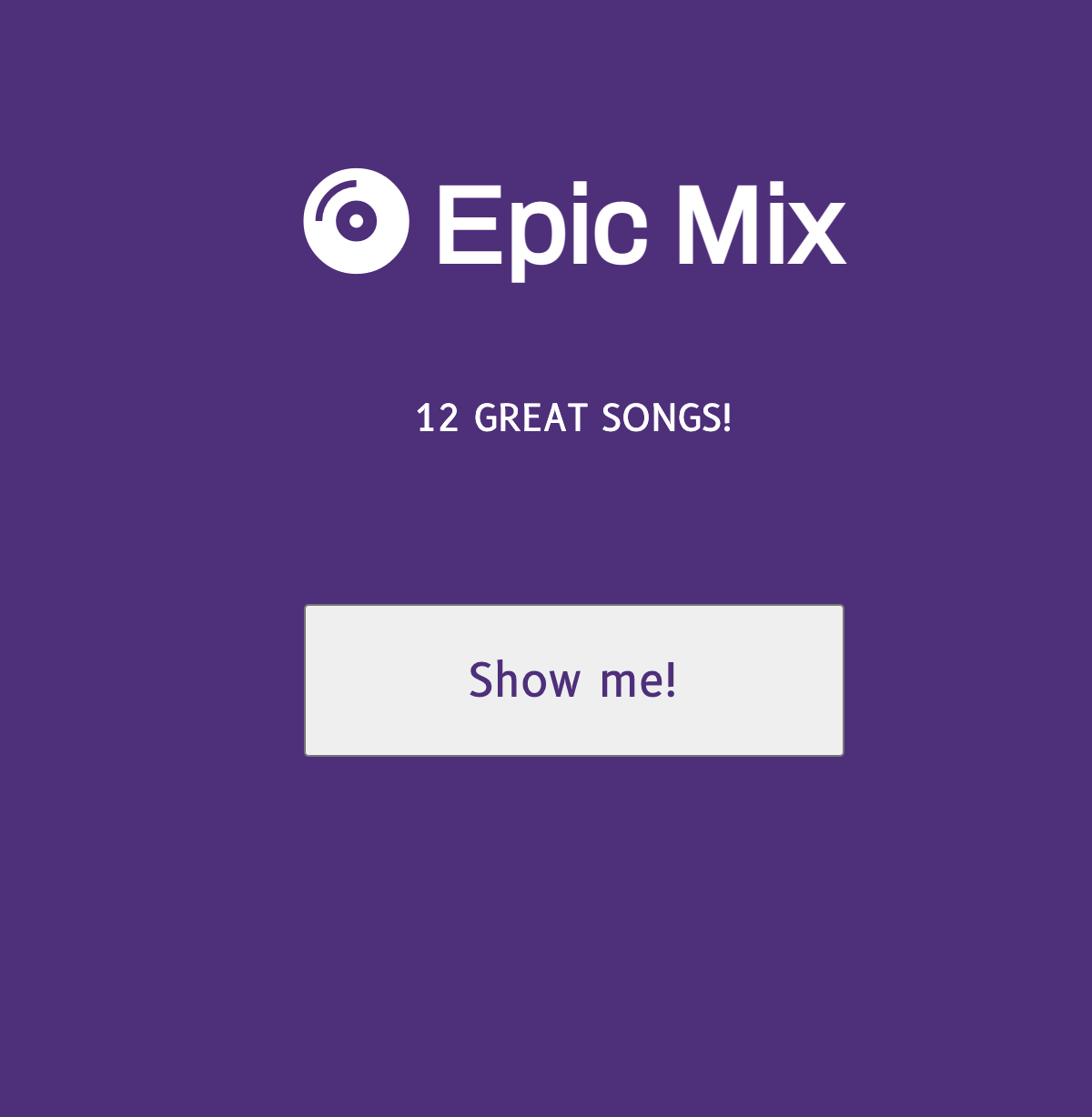
Epic Mix Playlist Source Code
- Array loops (forEach)
- document.createElement
- Append to an array
- Template literals
- Copy the source code from CodeSandbox.
- Implement the JavaScript code so that you can create an auto-generated list of your favorite songs and their indexes.
To test your understanding of the concepts behind the epic mix playlist, your bonus challenge is this favorite movie list.
6. Pet Rescue
- It gives you a solid foundation for understanding factory function patterns and parameters
- You can use this project to contribute to pet rescues or display inventory for products on a ecommerce site
- Learn more about how to use factory functions, parameters, methods, and objects
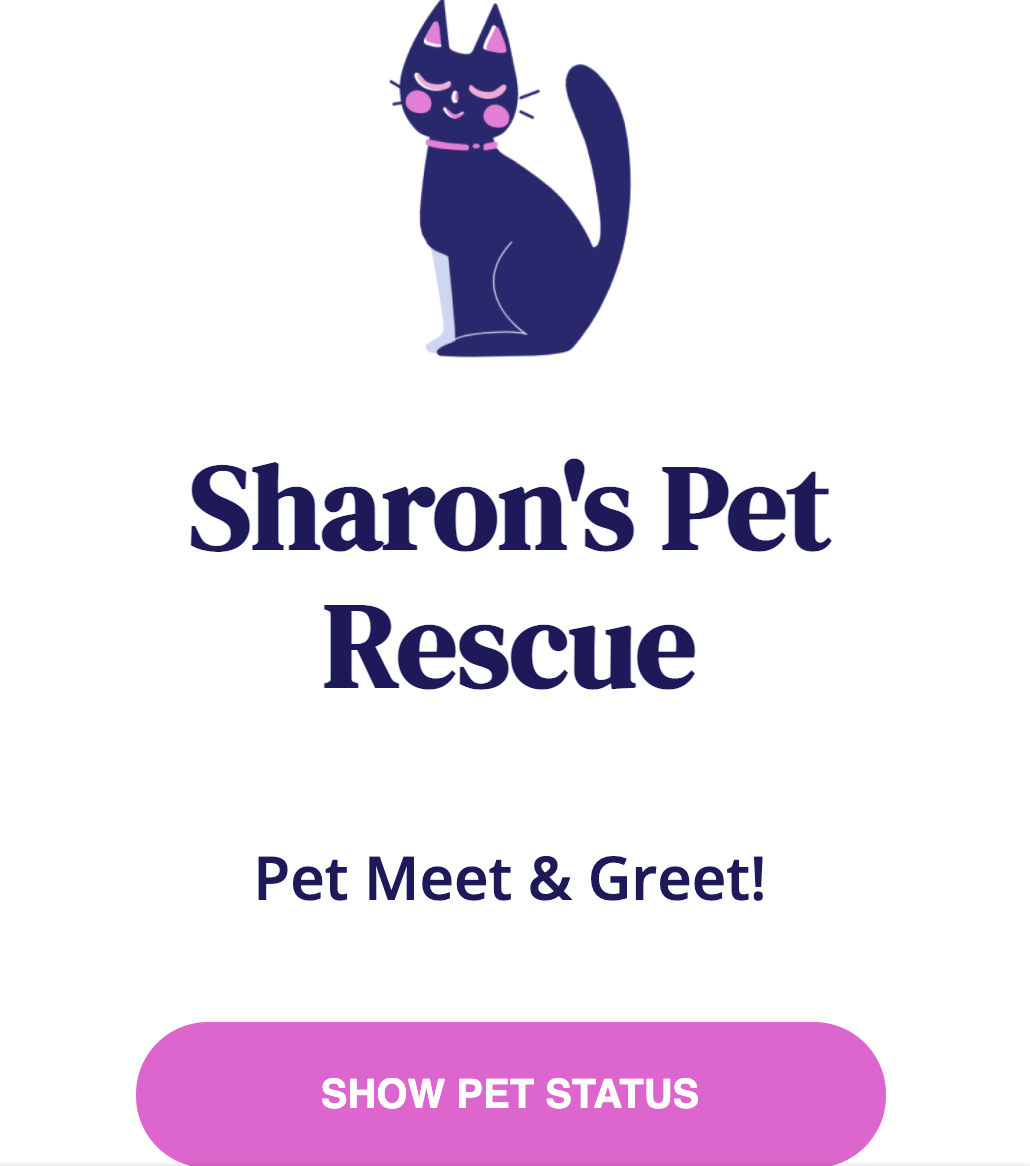
Pet Rescue Source Code
- Factory functions
- Object methods and properties
- Implement the JavaScript code so that you can accept an animal’s name, species, and energy level as a parameter and indicate each animal’s status (whether they are sleeping or awake).
To test your understanding of the concepts behind Pet Rescue, your bonus challenge is this inventory system.
7. Drum Kit
- You can see the interaction between seeing elements on the page and listening to sound and learning how to add and remove elements
- You can use this project to practice your drum skills with your JavaScript skills
- Learn more about how to use audio tags and document.querySelector
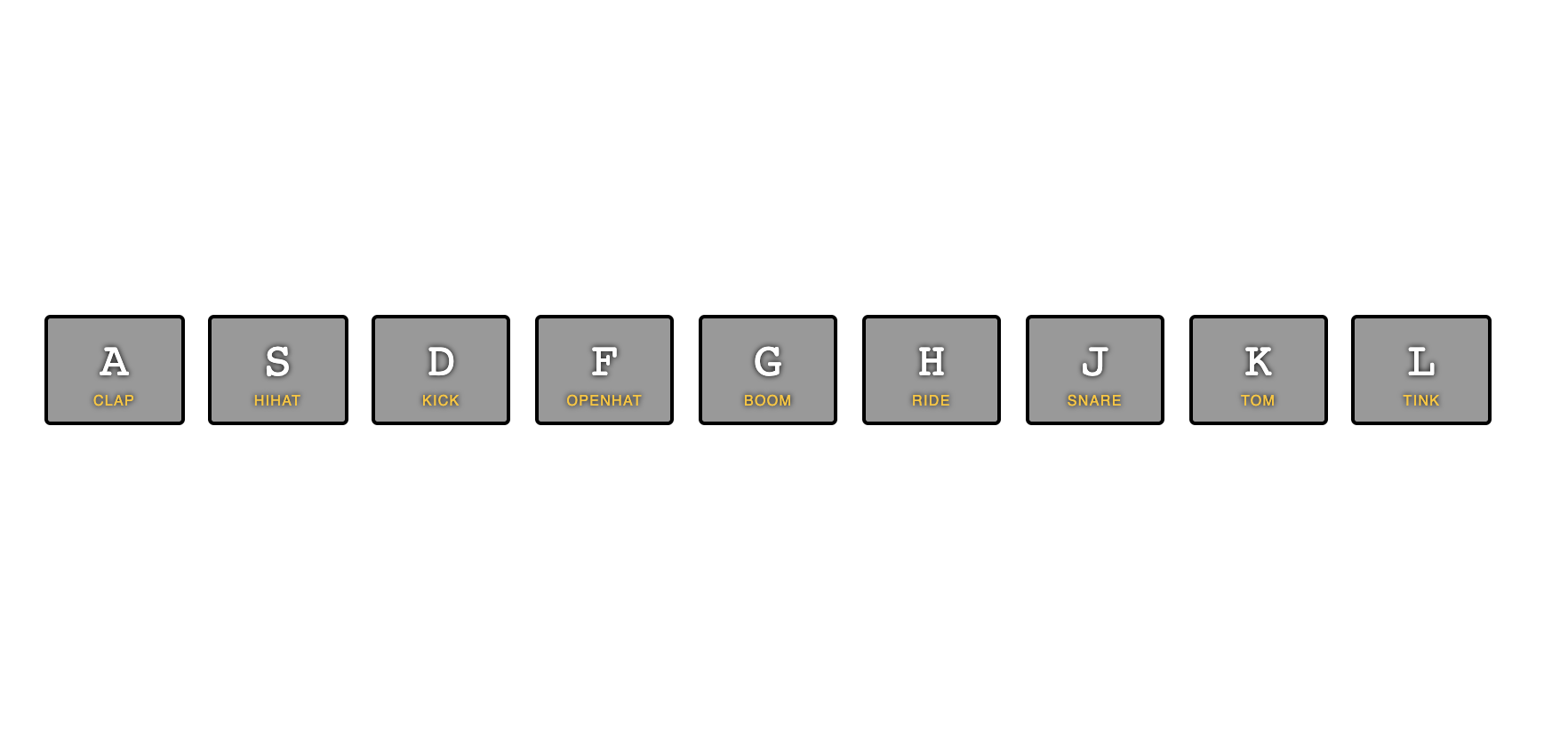
Drum Kit Source Code
- Event Listener
- document.querySelector
- Clone the GitHub repo.
- Implement the JavaScript code so that you can play the drums by pressing specific keys in the browser.
To test your understanding of the concepts behind the drum kit, your bonus challenge is this JavaScript piano player.
8. Speech Detection
- It gives you a basis for understanding how speech detection works, which is good (and very cool!) to know
- You’ll have a nifty notetaking method straight in your browser
- Learn more about speech recognition, text content, and event listeners
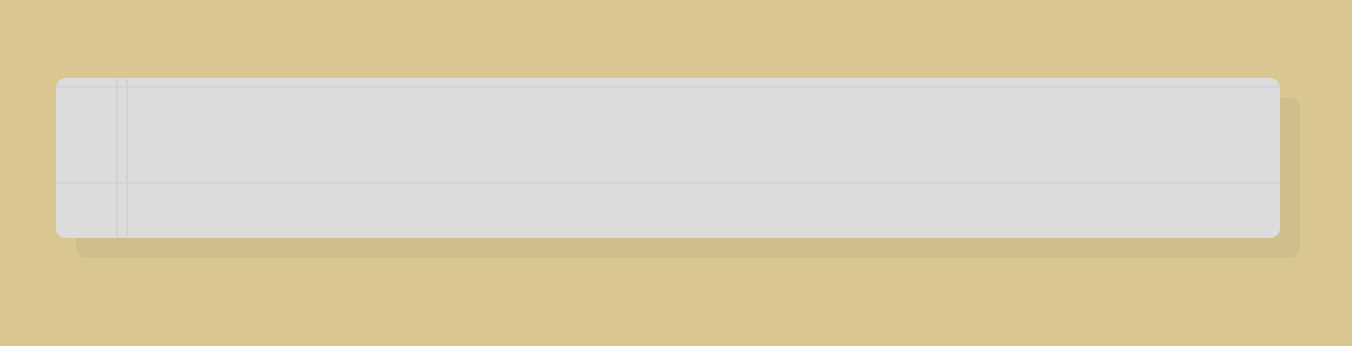
Speech Detection Source Code
- Window.SpeechRecognition
- .textContent
- Copy the source code from GitHub.
- Implement the JavaScript code so that when you speak, whatever you say is transcribed on the lined paper on your screen.
To test your understanding of the concepts behind speech detection, your bonus challenge is this text to speech in different languages.
9. Sticky Navigation
- It teaches you how to keep your elements in a fixed position on a page, which is a skill you might need in your front end developer role
- You can use this to upgrade the design of your website or portfolio
- Learn more about how to use query electors, event listeners, and offsetting
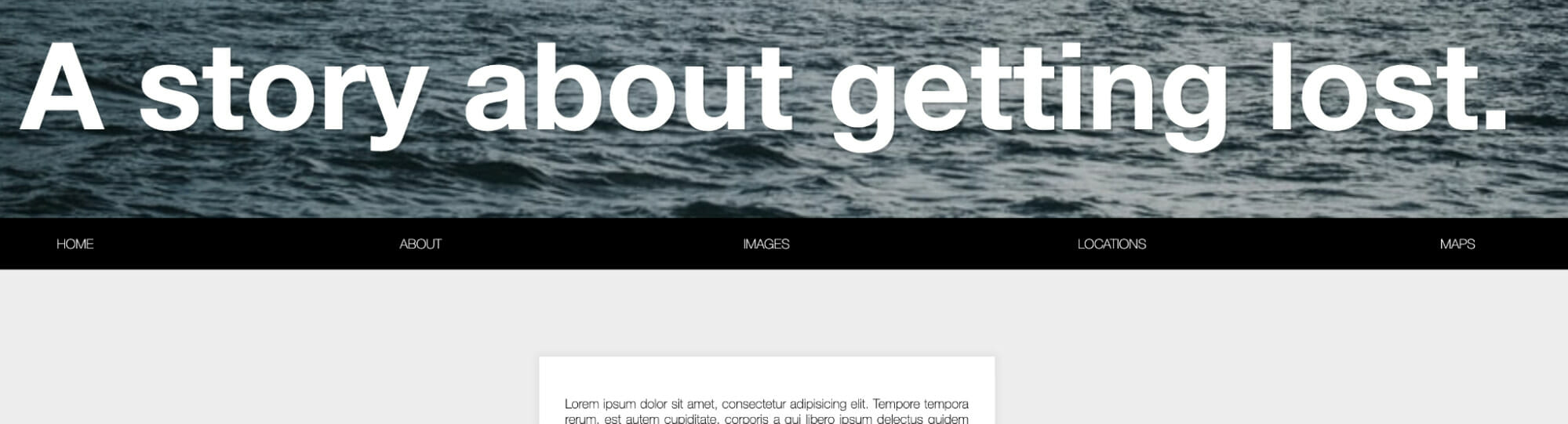
Sticky Navigation Source Code
- .querySelector
- Implement the JavaScript code so that when you scroll up or down the page, your navigation bar remains in a fixed and visible position.
To test your understanding of the concepts behind sticky navigation, your bonus challenge is to create a sliding navigation.
10. Geolocation
- You can learn more about how to develop for mobile, how geolocation works, and how to test your code using simulators or a different device
- You can use this project in your daily life (if you get lost easily)
- Learn more about how to use query selectors, watch position, and coordinates
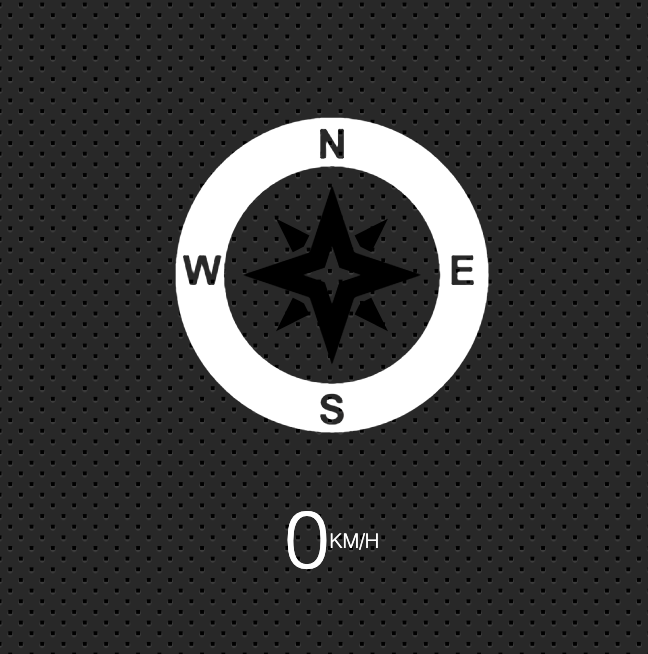
Geolocation Source Code
- .watchPosition
- Install packages with npm and run your local server.
- Implement the JavaScript code so that you see your location in real time. You will need iOS or an iOS simulator to test whether or not your code works.
To test your understanding of the concepts behind geolocation, your bonus challenge is this weather forecast tool.
11. Movie App
- It gives you a solid foundation of how JavaScript works with HTML, CSS, and other tools
- You can use this project to make your own custom movie app
- Learn more about how to use JavaScript with jQuery, Bootstrap, and API calls
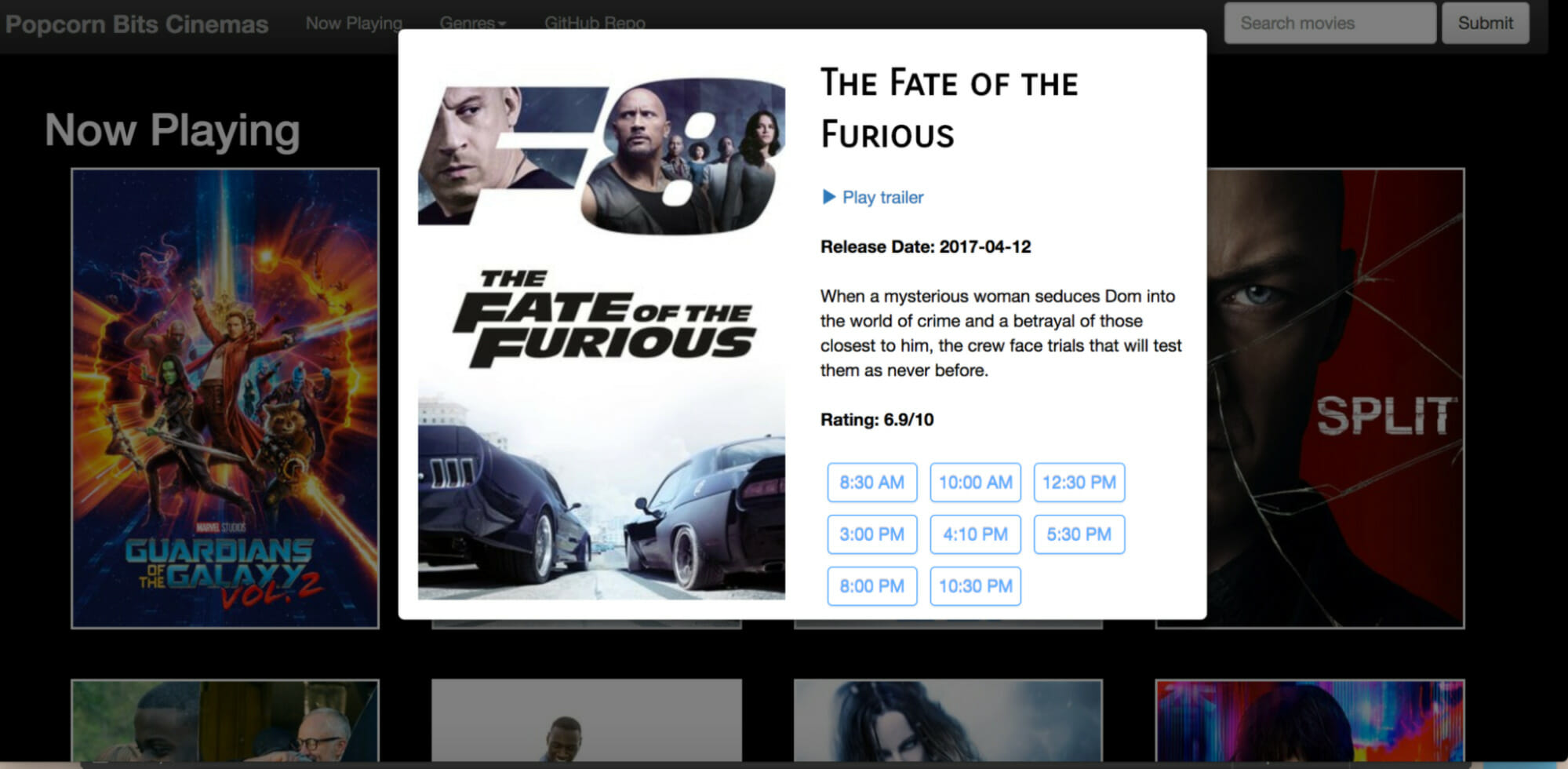
Movie App Source Code
- On click event
- You’ll need to get yourself an API key!
- Implement the JavaScript code so that you can make your own movie app that lets you view the most popular movies, sort them by genre, and search for other movies.
To test your understanding of the concepts behind the movie app, your bonus challenge is to build an ecommerce landing page.
12. Name Tags
- As a beginner JavaScript project, it lets you practice using React to create a practical and useful tool
- You can use this project to hone your React skills and key concepts
- Learn more about how to handle user input, work with stateful components, and render array data models
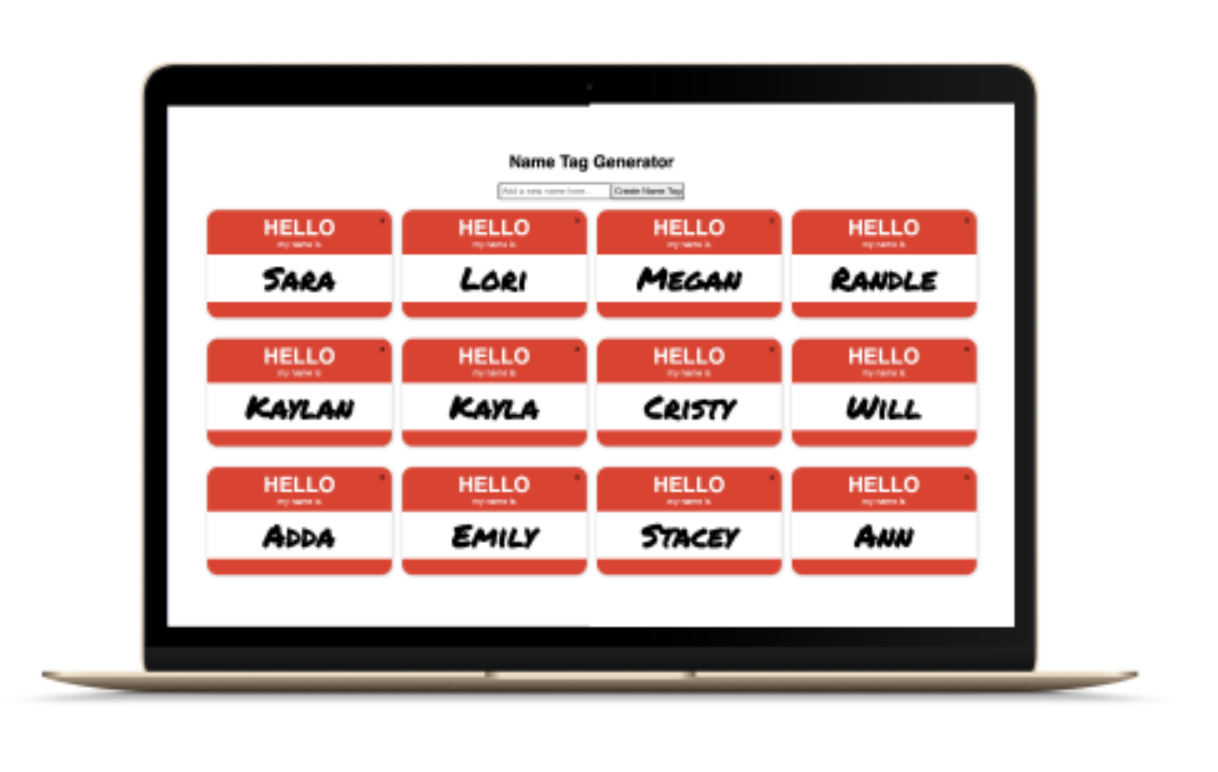
Name Tag Generator Source Code
- Handling user input
- Stateful components
- Render array data models
- Implement the JavaScript code so that you can build a text box and button to let users input text and have it respond to the input by generating a new name tag!
To test your understanding of the concepts behind the name tag generator, your bonus challenge is this Pokemon React generator.
(Back to top.)
13. Tone.js
- You’ll learn how to create interactive web browser music with advanced scheduling capabilities, synths and effects, and intuitive musical abstractions built on top of the Web Audio API
- You can use this project to be your own personal DJ and music producer, for free!
- Learn more about how to use event listeners, triggerAttack, triggerRelease, and different kinds of synths

Tone.js Source Code
- triggerAttack and triggerRelease
- Copy the source code from the Tone.js GitHub.io page.
- Implement the JavaScript code so that you can create interactive music in your own browser.
14. Election Map
- It gives you a solid foundation of the difference between JavaScript, HTML, and CSS, especially what JavaScript does and how it does it
- You can use this project in your daily life and add it to your portfolio
- Learn more about how to use arrays and nested functions
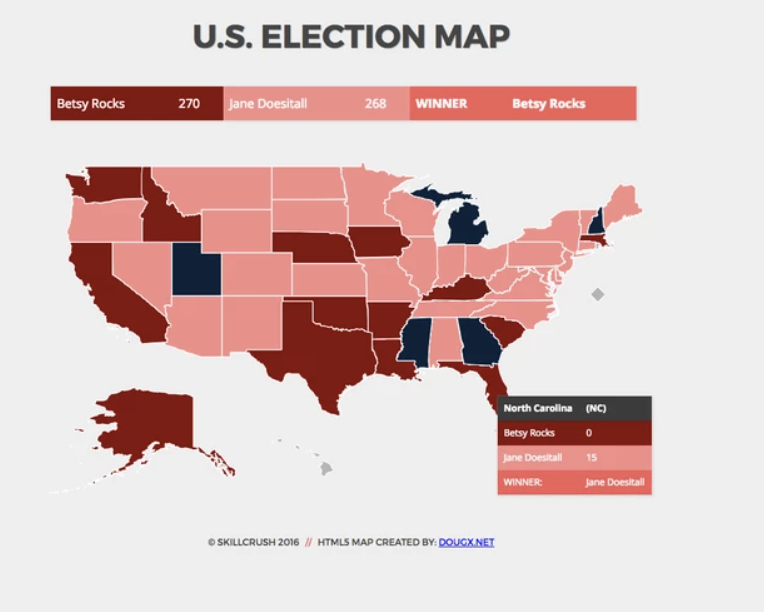
Election Map Source Code
- If-else and function practice
- Parent-child relationships
- getElementById
- Copy the source code from JSBin.
- Implement the JavaScript code so that you can add two candidates running for president and display the number of votes they received from each state, both in a table on the bottom right and when you mouse over a state.
To test your understanding of the concepts behind the election map, your bonus challenge is this interactive map.
15. Login Authentication
- It introduces you to Angular JS and more advanced JavaScript concepts
- You can see how login authentication works behind the scenes
- Learn more about how to use function states, app controllers, and dependencies
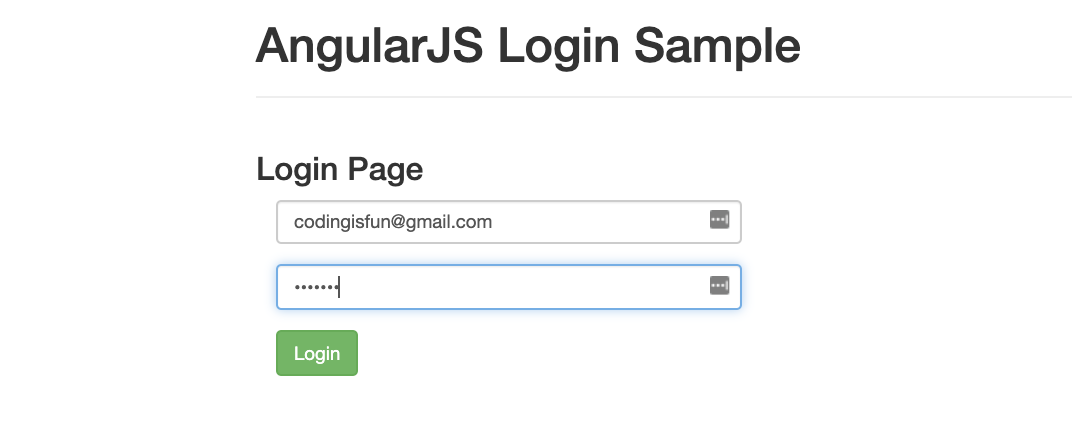
Login Authenticator Source Code
- State management
- App controller
- Dependencies
- Implement the JavaScript code so that you can enter an email address and password, and have the system tell you if the email address is invalid when you press the “Submit” button.
To test your understanding of the concepts behind the Login Authenticator, your bonus challenge is this password generator.
16. Guess the Word
- It gives you a solid foundation for methods, creating global variables, and working with event listeners
- You can use this project as a party game or challenge with your friends and coworkers
- Learn more about how to use methods, functions, and regular expressions
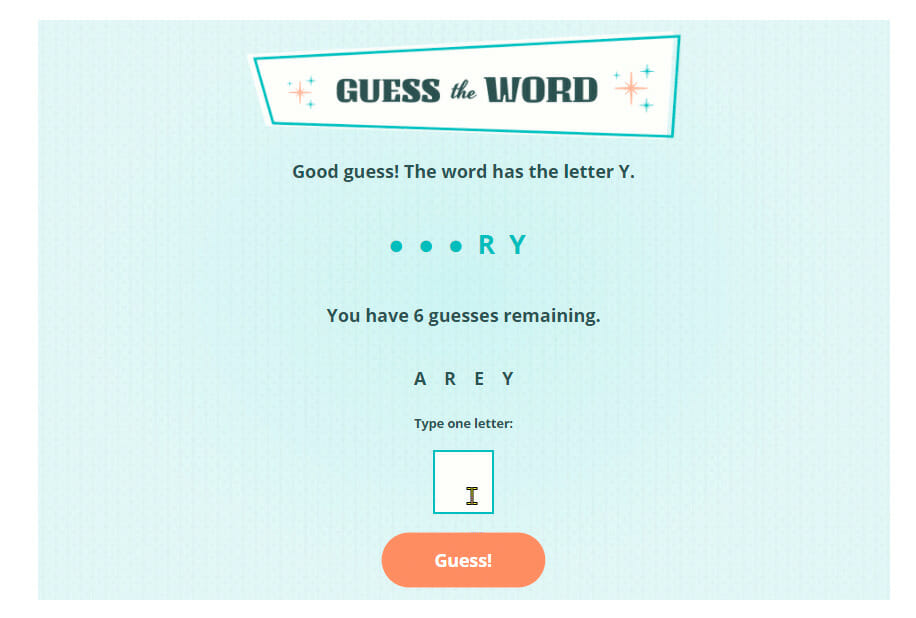
Guess the Word Source Code
- Methods like match(), split(), trim(), and join()
- Regular expressions
- Implement the JavaScript code so that players start by entering a letter. If they guess correctly, the letter appears in the word. If they guess incorrectly, they learn how many guesses they have remaining. The player can also see which letters they’ve already guessed. The game lets the player know if they’ve already guessed a letter or entered a non-alphabetic character!
To test your understanding of the concepts behind the Guess the Word game, your bonus challenge is this Wordled game.
Warning: the difficulty level for this is advanced, so if you’re having trouble with it, it’s not you!
17. Terminalizer
- It teaches you how to record your terminal screen and share your work with others
- You can use this project to debug and work on coding projects with friends
- Learn more about how to use recording files, frame delays, and set idle times
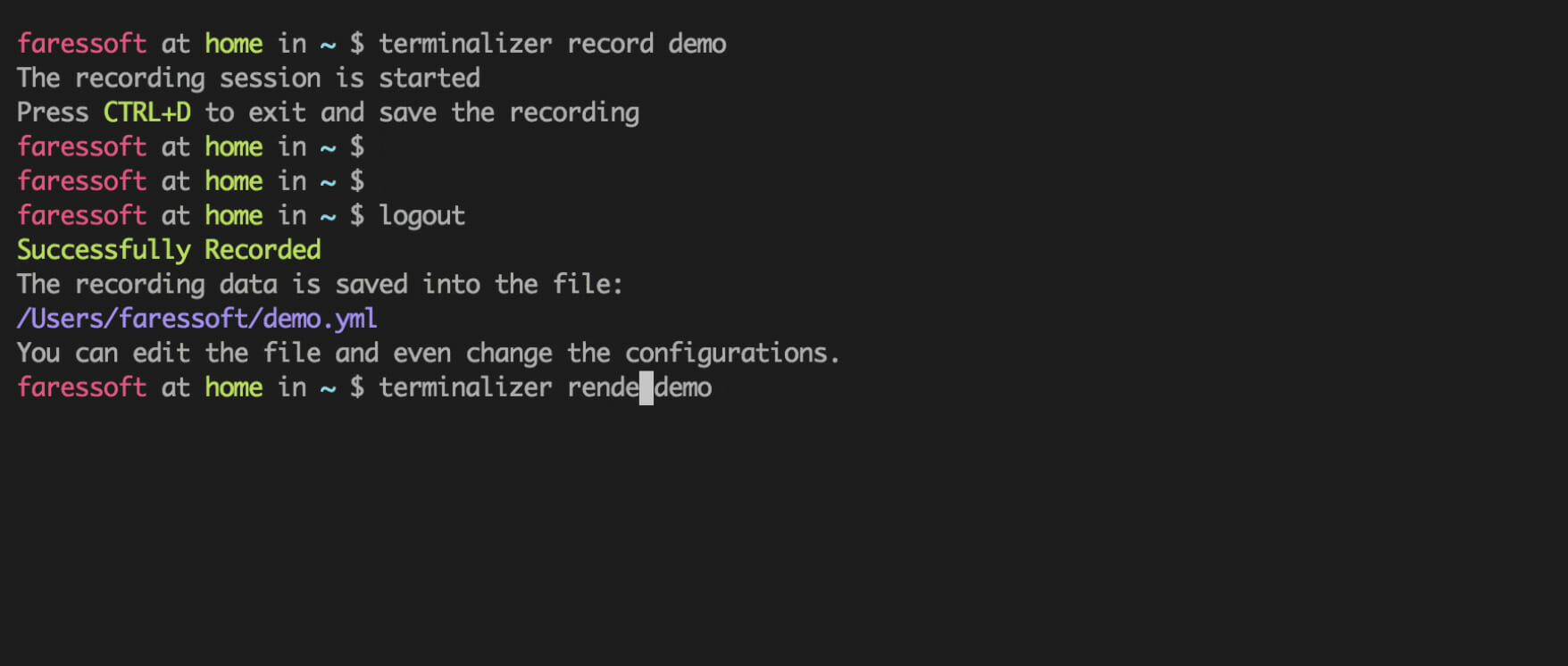
Terminalizer Source Code
- Recording files
- Using frameDelay
- Setting idle time
- Implement the JavaScript code so that you can record your terminal and generate animated GIF images or share web player links.
To test your understanding of the concepts behind Terminalizer, your bonus challenge is to figure out how to play/pause GIFs.
18. Chat App
- It gives you a solid foundation of how JavaScript and its front and back end libraries can be used to create applications
- You can add this project to your portfolio to show your knowledge of programming concepts as a full stack developer
- Learn more about how to use ReactJS, NodeJS, and sockets along with JavaScript
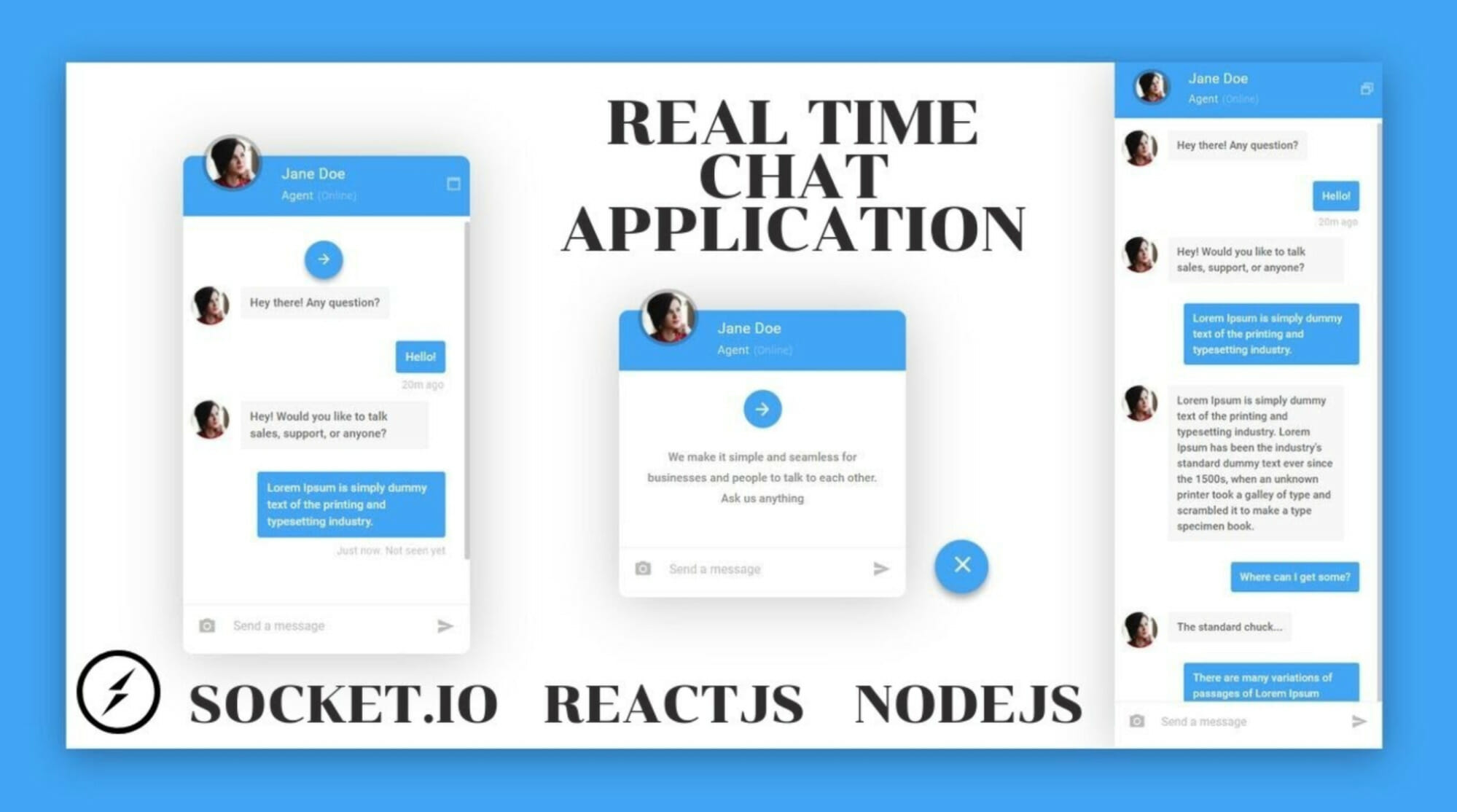
Chat App Source Code and YouTube tutorial link
- You won’t be able to clone and run the project straight from GitHub because there is no package.json file, but you can build this using the YouTube tutorial
- Implement the JavaScript code so that you can build a real time chat application that lets you send and receive messages using web sockets
To test your understanding of the concepts behind the chat application, your bonus challenge is this React text editor.
19. Tic Tac Toe Game
- This project will test your HTML semantics and your JavaScript logic skills
- You can use this as a practice project and for something to do while your code compiles
- Learn more about how to use arrays, grid methods, and event listeners
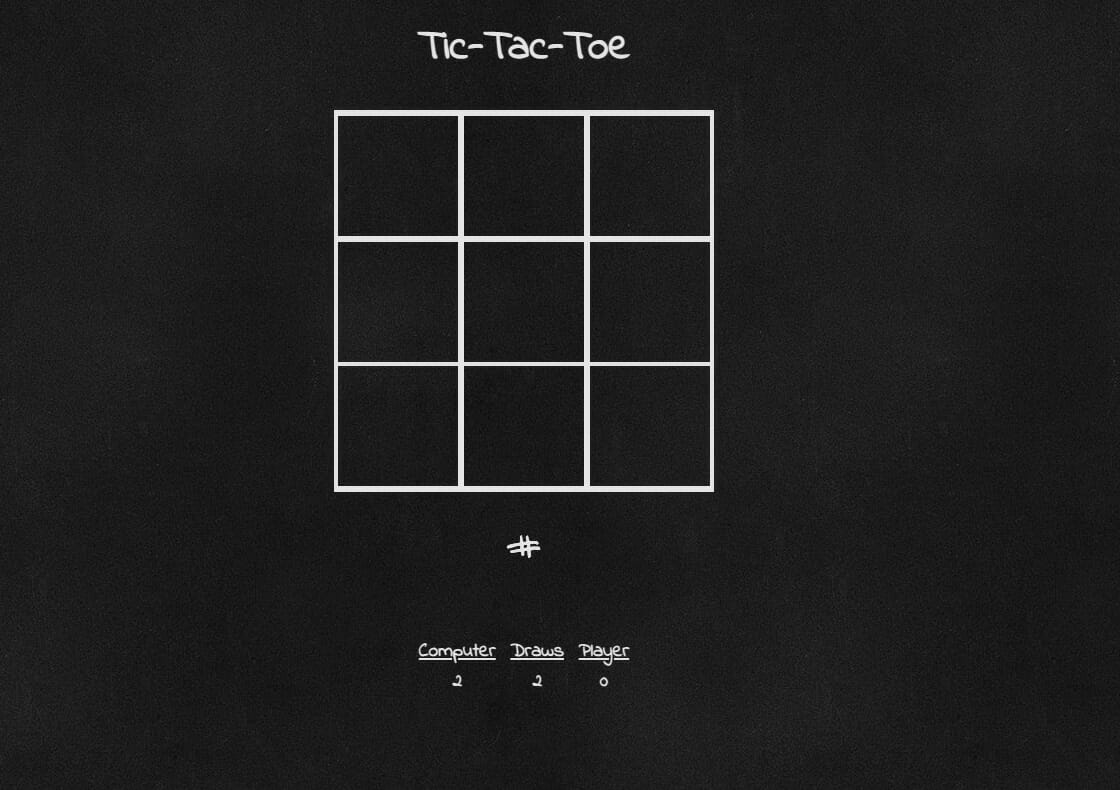
Tic-Tac-Toe Source Code
- Implement the JavaScript code so that the player can set difficulty level as easy or hard, choose whether to play as X or O, play a game with the computer, have it remember who won or drew, and play again.
To test your understanding of the concepts behind the Tic-Tac-Toe game, your bonus challenge is this hangman game.
20. Hotel Booking App
- You’ll get to practice key concepts in JavaScript ES6 and React JSX
- You can use this project to practice building sites with more functionality and use some of your creativity by making it look really spiffy
- Learn more about how to manage data flow and manipulate the DOM
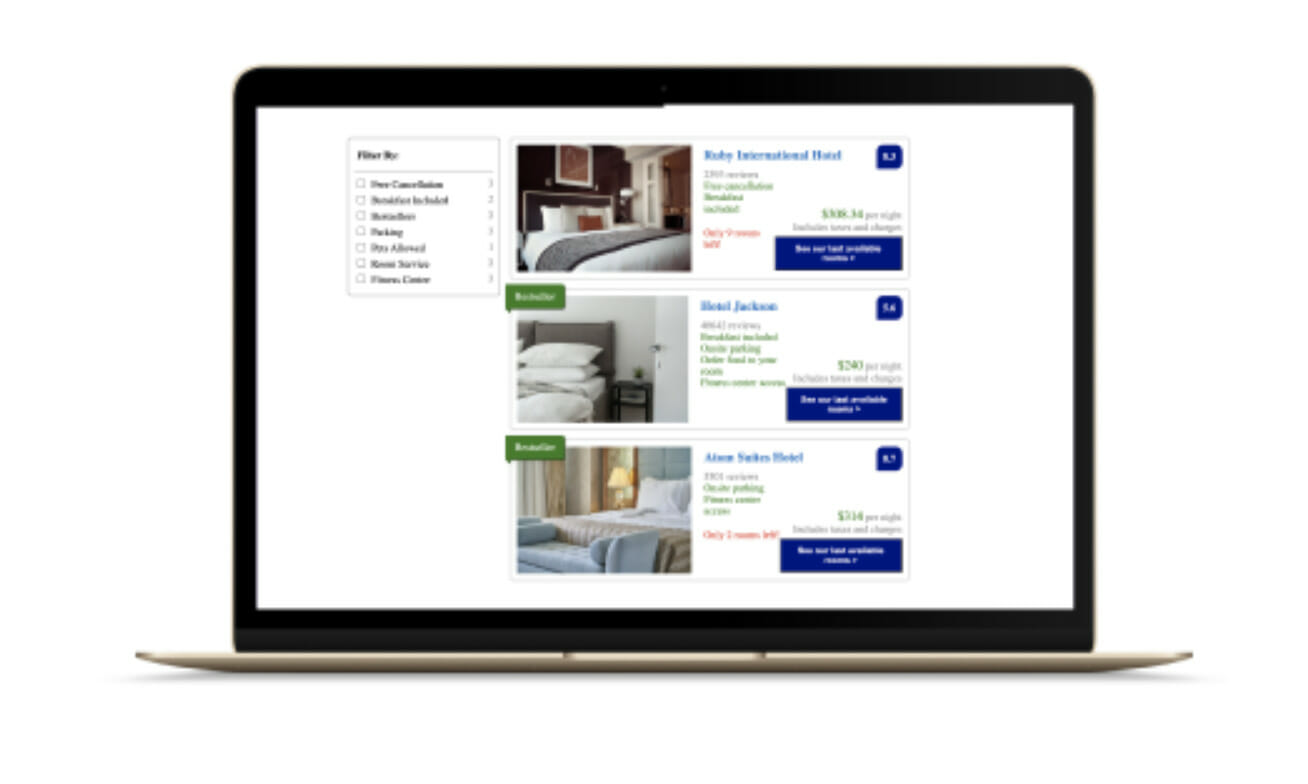
Hotel Booking App Source Code
- ES6 and React JSX practice
- Managing data flow
- Implement the JavaScript code so that you can create a sample booking on a hotel site, complete with filtered search results, room inventory display, and hotel ratings.
To test your understanding of the concepts behind the Hotel Booking App, your bonus challenge is this ticket booking app.
Advanced JavaScript Projects
21. maze game.
- It gives you a solid idea of how JavaScript core skills and functions can be used to create interactive games
- You can put this project in your portfolio and challenge friends
- Learn more about how to use JavaScript math concepts, create elements, and use loops
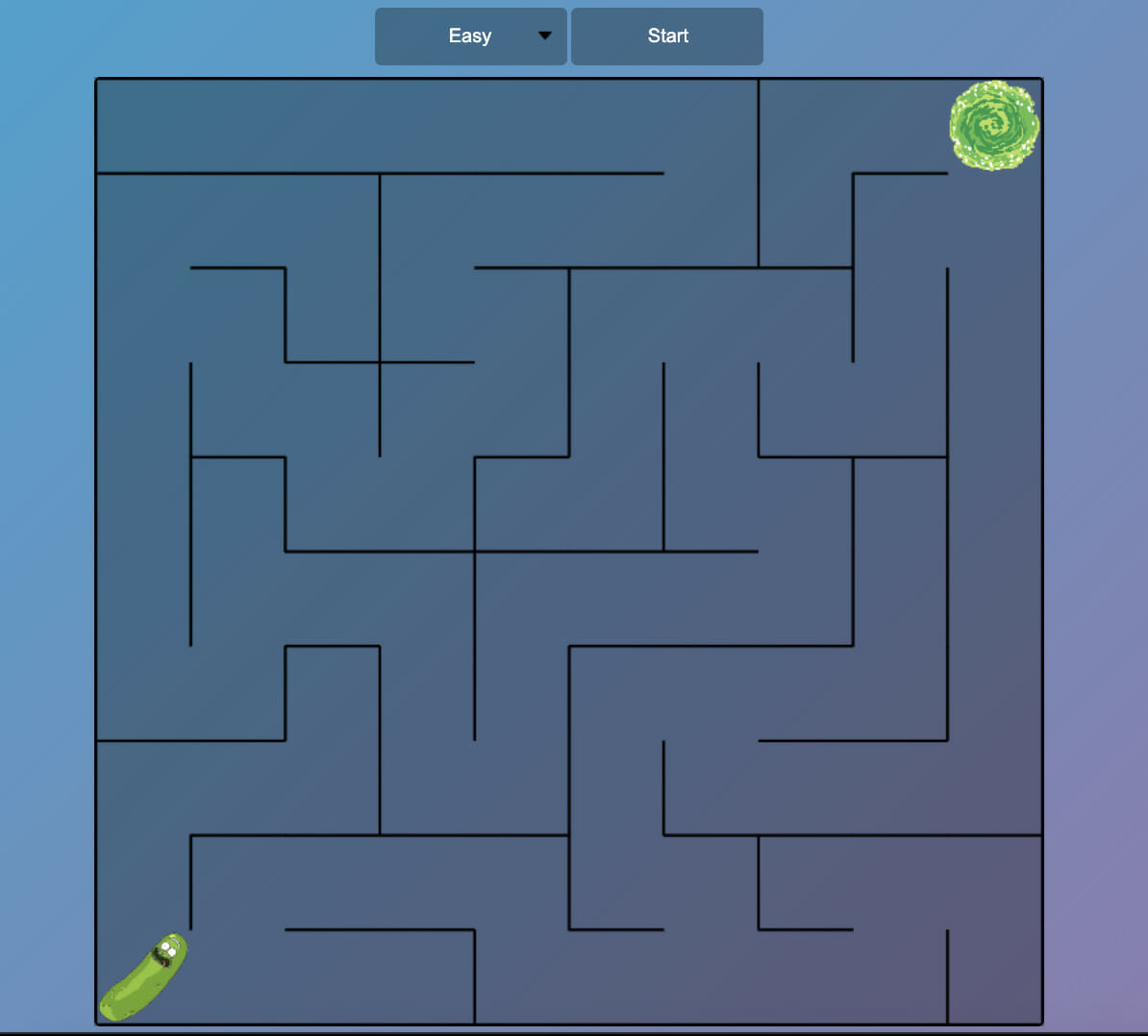
Maze Game Source Code
- Random and Floor
- Element creation
- Implement the JavaScript code so that you can get the pickle from one end of the maze to another using the arrow functions (or AWSD) on your keyboard, have it display the number of steps it took to complete the maze, and be able to set the difficulty level.
To test your understanding of the concepts behind the maze game, your bonus challenge is this Tetris game.
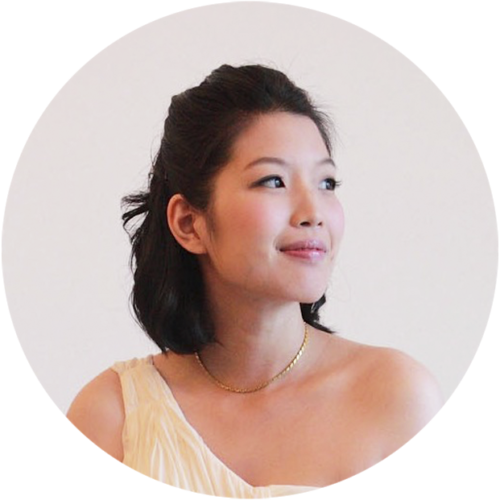
Justina Hwang
Category: Blog , Coding Languages and Tools , Entry Level Tech Jobs , Front End Developer , Full Stack Developer , JavaScript , Learn to Code
Related Articles
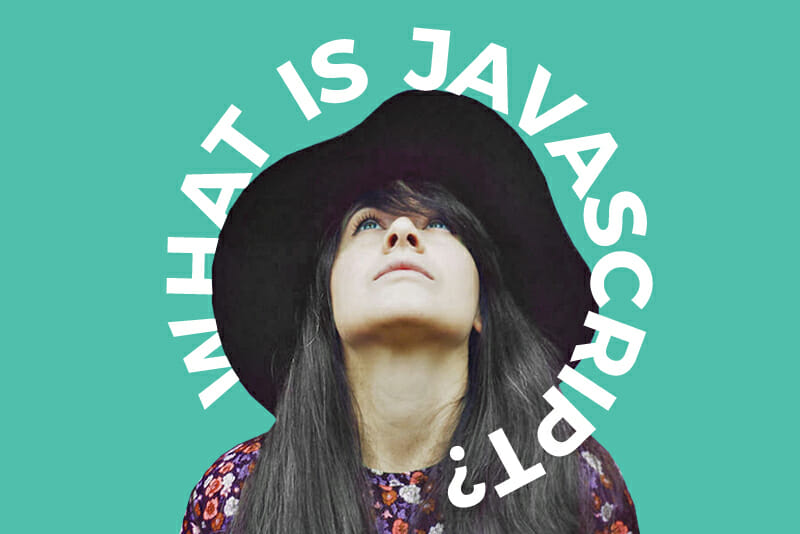
How to Use If Statements in JavaScript – a Beginner's Guide

JavaScript is a powerful and versatile programming language that is widely used for creating dynamic and interactive web pages.
One of the fundamental building blocks of JavaScript programming is the if statement. if statements allow developers to control the flow of their programs by making decisions based on certain conditions.
In this article, we will explore the basics of if statements in JavaScript, understand their syntax, and see how they can be used to create more responsive and intelligent code.
What is an if Statement?
An if statement is a conditional statement that allows you to execute a block of code only if a specified condition is true. In other words, it provides a way to make decisions in your code.
For example, you might want to display a message to the user if they have entered the correct password, or you might want to perform a certain action only if a variable has a specific value.
Here's a simple example to illustrate the basic structure of an if statement:
In this example, the if statement checks whether the value of the temperature variable is greater than 20. If the condition is true, the code inside the curly braces ( {} ) is executed, and the message "It's a warm day!" is logged to the console.
Syntax of if Statements
The syntax of an if statement in JavaScript is straightforward. It consists of the if keyword followed by a set of parentheses containing a condition. If the condition evaluates to true , the code inside the curly braces is executed. Here is the basic syntax:
Let's break down the components of the if statement:
- if keyword: This is the keyword that starts the if statement and is followed by a set of parentheses.
- Condition: Inside the parentheses, you provide the condition that you want to evaluate. This condition should result in a boolean value ( true or false ).
- Code block: The code block is enclosed in curly braces {} . If the condition is true, the code inside the block is executed.
It's important to note that the curly braces are essential, even if the block contains only one statement. Including the braces makes your code more readable and helps prevent bugs that can occur when adding more statements to the block later.
Simple if Statements
Let's dive deeper into simple if statements by exploring some examples. Simple if statements consist of a single condition and a block of code that executes when the condition is true.
Example 1: Checking a Number
In this example, the if statement checks whether the value of the number variable is greater than 0. If the condition is true, the message "The number is positive." is logged to the console.
Example 2: Verifying User Input
This example uses the prompt function to get the user's age as input. The if statement then checks whether the entered age is greater than or equal to 18. If true, the message "You are eligible to vote." is logged to the console.
Example 3: Checking Equality
In this example, the if statement checks whether the value of the password variable is exactly equal to the string "secure123". If the condition is true, the message "Access granted." is logged to the console.
If-Else Statements
While simple if statements are useful, often you'll want to provide an alternative action when the condition is false. This is where the if-else statement comes in handy. The else block contains code that executes when the if condition is false.
Example: If-Else Statement
In this example, the if statement checks whether the value of the hour variable is less than 12. If true, it logs "Good morning!" to the console. Otherwise, it logs "Good afternoon!".
If-Else If Statements
Sometimes, you may have multiple conditions to check. In such cases, you can use else if statements to add additional conditions. The code inside the first true condition block encountered will be executed, and subsequent conditions will be ignored.
Example: If-Else If Statement
In this example, the code determines a student's grade based on their score. It first checks if the grade is greater than or equal to 90, and if true, it logs "A". If not, it moves to the next condition and checks if the grade is greater than or equal to 80, and so on. If none of the conditions are true, it logs "D".
Nested if Statements
You can also nest if statements inside other if statements to create more complex decision structures. Each if statement within the nested structure adds an additional layer of conditions.
Example: Nested if Statement
In this example, the outer if statement checks if it's summer. If true, it enters the nested structure and checks the temperature. Depending on the temperature, it logs different messages. If it's not summer, it logs "It's not summer."
Logical Operators in if Statements
JavaScript provides logical operators ( && , || , ! ) that allow you to combine multiple conditions in a single if statement.
Example: Logical Operators
In this example, the && (logical AND) operator combines two conditions: the person's age being 18 or older and having a valid license. If both conditions are true, it logs "You are eligible to drive." Otherwise, it logs "You are not eligible to drive."
Switch Statements
In situations where you have multiple possible conditions to check against a single value, a switch statement can be more concise than a series of if-else if statements.
Example: Switch Statement
In this example, the switch statement checks the value of dayOfWeek and executes the corresponding block of code. If none of the cases match, the default block is executed.
Common Mistakes with if Statements
While using if statements, beginners often make some common mistakes. Let's address a few of them:
Mistake 1: Forgetting the Equality Operator
The above code is incorrect because it uses the assignment operator = instead of the equality operator === inside the if condition. The correct code should be if (x === 10) .
Mistake 2: Misplacing Curly Braces
In this example, only the first console.log statement is part of the if block because the curly braces are missing. To include both statements in the if block, use curly braces:
Mistake 3: Confusing = and ==
The above code is incorrect because it uses the assignment operator = instead of the equality operator == inside the if condition. The correct code should be if (z == 5) .
Understanding and mastering if statements is essential for any JavaScript developer. These conditional statements provide the logic that enables your programs to make decisions and respond dynamically to different situations.
Whether you're validating user input, controlling the flow of your application, or handling different cases, if statements are a fundamental tool in your programming arsenal.
As you continue your journey in JavaScript development, practice using if statements in various scenarios. Experiment with different conditions, nesting structures, and logical operators to become comfortable and proficient in utilizing these powerful tools.
With a solid understanding of if statements, you'll be well-equipped to build more dynamic and responsive web applications.
frontend developer || technical writer
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
Destructuring Assignment in JavaScript
- How to swap variables using destructuring assignment in JavaScript ?
- Division Assignment(/=) Operator in JavaScript
- Exponentiation Assignment(**=) Operator in JavaScript
- What is a Destructuring assignment and explain it in brief in JavaScript ?
- Describe closure concept in JavaScript
- Copy Constructor in JavaScript
- Classes In JavaScript
- JavaScript Function() Constructor
- Multiple Class Constructors in JavaScript
- Implementation of Array class in JavaScript
- Functions in JavaScript
- JavaScript TypeError - Invalid assignment to const "X"
- Scoping & Hoisting in JavaScript
- How to set default values when destructuring an object in JavaScript ?
- What is Parameter Destructuring in TypeScript ?
- JavaScript Hoisting
- Addition Assignment (+=) Operator in Javascript
- Array of functions in JavaScript
- Enums in JavaScript
Destructuring Assignment is a JavaScript expression that allows to unpack values from arrays, or properties from objects, into distinct variables data can be extracted from arrays, objects, nested objects and assigning to variables . In Destructuring Assignment on the left-hand side defined that which value should be unpacked from the sourced variable. In general way implementation of the extraction of the array is as shown below: Example:
- Array destructuring:
Object destructuring:
Array destructuring: Using the Destructuring Assignment in JavaScript array possible situations, all the examples are listed below:
- Example 1: When using destructuring assignment the same extraction can be done using below implementations.
- Example 2: The array elements can be skipped as well using a comma separator. A single comma can be used to skip a single array element. One key difference between the spread operator and array destructuring is that the spread operator unpacks all array elements into a comma-separated list which does not allow us to pick or choose which elements we want to assign to variables. To skip the whole array it can be done using the number of commas as there is a number of array elements.
- Example 3: In order to assign some array elements to variable and rest of the array elements to only a single variable can be achieved by using rest operator (…) as in below implementation. But one limitation of rest operator is that it works correctly only with the last elements implying a subarray cannot be obtained leaving the last element in the array.
- Example 4: Values can also be swapped using destructuring assignment as below:
- Example 5: Data can also be extracted from an array returned from a function. One advantage of using a destructuring assignment is that there is no need to manipulate an entire object in a function but just the fields that are required can be copied inside the function.
- Example 6: In ES5 to assign variables from objects its implementation is
- Example 7: The above implementation in ES6 using destructuring assignment is.
- Example1: The Nested objects can also be destructured using destructuring syntax.
- Example2: Nested objects can also be destructuring
Please Login to comment...
Similar reads.
- JavaScript-Questions
- Web Technologies
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

IMAGES
VIDEO
COMMENTS
The assignment (=) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. ... JavaScript does not have implicit or undeclared variables. It just conflates the global object with the global scope and allows omitting the global object qualifier during property creation.
Use the correct assignment operator that will result in x being 15 (same as x = x + y ). Start the Exercise. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
An assignment operator ( =) assigns a value to a variable. The syntax of the assignment operator is as follows: let a = b; Code language: JavaScript (javascript) In this syntax, JavaScript evaluates the expression b first and assigns the result to the variable a. The following example declares the counter variable and initializes its value to zero:
An assignment operator assigns a value to its left operand based on the value of its right operand.. Overview. The basic assignment operator is equal (=), which assigns the value of its right operand to its left operand.That is, x = y assigns the value of y to x.The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
An operator is binary if it has two operands. The same minus exists in binary form as well: let x = 1, y = 3; alert( y - x ); // 2, binary minus subtracts values. Formally, in the examples above we have two different operators that share the same symbol: the negation operator, a unary operator that reverses the sign, and the subtraction ...
This is made to assign a default value, in this case the value of y, if the x variable is falsy. The boolean operators in JavaScript can return an operand, and not always a boolean result as in other languages. The Logical OR operator ( ||) returns the value of its second operand, if the first one is falsy, otherwise the value of the first ...
Catastrophic backtracking. Sticky flag "y", searching at position. Methods of RegExp and String. Modern JavaScript Tutorial: simple, but detailed explanations with examples and tasks, including: closures, document and events, object oriented programming and more.
40 JavaScript Projects for Beginners - Easy Ideas to Get Started Coding JS. The best way to learn a new programming language is to build projects. I have created a list of 40 beginner friendly project tutorials in Vanilla JavaScript, React, and TypeScript. My advice for tutorials would be to watch the video, build the project, break it apart ...
Embark on your JavaScript learning journey with our online practice portal. Start by selecting quizzes tailored to your skill level. Engage in hands-on coding exercises, receive real-time feedback, and monitor your progress. With our user-friendly platform, mastering JavaScript becomes an enjoyable and personalized experience.
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
It's called "destructuring assignment," because it "destructurizes" by copying items into variables. However, the array itself is not modified. It's just a shorter way to write: // let [firstName, surname] = arr; let firstName = arr [0]; let surname = arr [1]; Ignore elements using commas.
Javascript operators are used to perform different types of mathematical and logical computations. Examples: The Assignment Operator = assigns values. The Addition Operator + adds values. The Multiplication Operator * multiplies values. The Comparison Operator > compares values
JavaScript is a cross-platform, object-oriented scripting language. It is a small and lightweight language. Inside a host environment ( a web browser), JavaScript can be connected to the objects of its environment to provide programmatic control over them. JavaScript contains a standard library of objects, such as Array, Date, and Math, and a ...
There is a single operator in JavaScript, capable of providing the remainder of a division operation. Two numbers are passed as parameters. The first parameter divided by the second parameter will have a remainder, possibly zero. Return that value. Examples remainder(1, 3) 1 remainder(3, 4) 3 remainder(-9, 45) -9 r …
JavaScript is one of the 3 languages all web developers must learn: 1. HTML to define the content of web pages. 2. CSS to specify the layout of web pages. 3. JavaScript to program the behavior of web pages. This tutorial covers every version of JavaScript: The Original JavaScript ES1 ES2 ES3 (1997-1999)
JSchallenger recognizes what skill level you're on and adjusts the difficulty of the next challenges automatically. Making you continuously improve your Javascript skills in a short amount of time. JSchallenger. Free Javascript challenges. Learn Javascript online by solving coding exercises. Javascript for all levels.
Implement the JavaScript code so that when you speak, whatever you say is transcribed on the lined paper on your screen. Bonus Challenge: To test your understanding of the concepts behind speech detection, your bonus challenge is this text to speech in different languages. 9. Sticky Navigation.
JavaScript Addition assignment operator (+=) adds a value to a variable, The Addition Assignment (+ =) Sums up left and right operand values and then assigns the result to the left operand. The two major operations that can be performed using this operator are the addition of numbers and the concatenation of strings. Syntax: a += b.
JavaScript is a powerful and versatile programming language that is widely used for creating dynamic and interactive web pages. One of the fundamental building blocks of JavaScript programming is the if statement.if statements allow developers to control the flow of their programs by making decisions based on certain conditions.. In this article, we will explore the basics of if statements in ...
Destructuring Assignment is a JavaScript expression that allows to unpack values from arrays, or properties from objects, into distinct variables data can be extracted from arrays, objects, nested objects and assigning to variables. In Destructuring Assignment on the left-hand side defined that which value should be unpacked from the sourced ...
9 videos • Total 43 minutes. Course Introduction: Web Development with HTML, CSS, and JavaScript • 1 minute • Preview module. Overview of Web and Cloud Development • 4 minutes. Insiders' Viewpoints: Aspects of the Web Development Lifecycle • 2 minutes. Learning Front-End Development • 6 minutes.