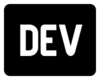

DEV Community

Posted on Oct 11, 2021 • Updated on Mar 25, 2023
6 ways to find the largest number in a python list.
Howdy, folks! I certainly hope you are having a great time and staying safe from that ugly Covid19 virus! Have you ever wonder what'd be the best approach to find the largest element of a list of numbers in python? Well, let's find out this together, buddies.
As you might've already guessed, know or even imagine, there are certainly several ways to find the largest number in a list in python, as in any other coding scenario. Let's break down some of the most common ones I've used when it comes to this type of situation. Are you ready, pals?
6 most common solutions
First, let's create a simple integer numbers list.
- Using the max() Function Now is time to put together a couple of those approaches into action. Let's start with the fastest solution to this:
- Find the largest element manually For some other pals out there, they would have preferred to built this solution by manually looking for that largest element, well, let's code something like that as follows:
- Find the largest item using list comprehension Well, that was that, wasn't it? If you find that a bit too large or too much code to write, let's simplify things on this case by using the list comprehension approach, shall we?
- Sorting list ascendantly & getting the last item Oh yeah, cowboys, that was a bit too short, and for some, a bit hard to read and understand, well, that is the magic of python, it has colors for every taste: Are you that kind of guy that prefers to go as simple as possible? Well, I am! So let's get back to the matter but with a simpler solution, bud!
- Using built-in sorted() function Hey, now, do you still think that was too iffy for a solution? Well, don't worry, let's add another cup of coffee to this breakfast, shall we? Let's use the sorted built-in function.
- Using a reverse list shortcut Last, but not least, this method is not 100% recommended because it might not give you the expected results in some versions of python, or the compiler as well, nevertheless, it is worth to bring it to the table.
Final Source Code
Now that we have fractionated all of these solutions, let's put together a final source code fo you guys to test it out and play with it. Please leave your comments with some other approaches or better solutions, to update the post if needed. Thank you so much for reading us, folks!
Thanks for reading this article, I hope you enjoyed it as much as I did writing it. Until next time, dear readers!
β€οΈ Your enjoyment of this article encourages me to write further. π¬ Kindly share your valuable opinion by leaving a comment. π Bookmark this article for future reference. π If this article has truly helped you, please share it.
Top comments (2)
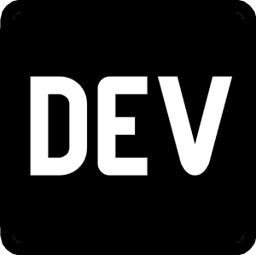
Templates let you quickly answer FAQs or store snippets for re-use.

- Joined Jun 6, 2022
max_num = numbers_lst[0] [max_num := n for n in numbers_lst if n >= max_num] print("List Comprehension: ", max_num) Doesn't work, can anyone help?

- Location Carazo, Nicaragua
- Pronouns He/Him
- Work Front End Web Developer
- Joined May 17, 2021
You are probably missing the list itself on this example, check this out:
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
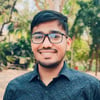
Promices and Async Await
Rohit - Apr 16
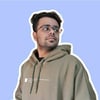
4 Habits to Avoid Bugs related to Hoisting in your JavaScript Code
Shubh Sharma - Apr 15
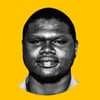
The One Thing Nobody Seems To Be Talking About
Karl Esi - Apr 15
How to debug axios requests
Paulund - Apr 15
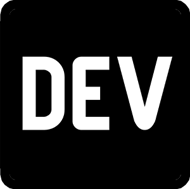
We're a place where coders share, stay up-to-date and grow their careers.

- Online Python Compiler
- Hello World
- Console Operations
- Conditional Statements
- Loop Statements
- Builtin Functions
- Type Conversion
Collections
- Classes and Objects
- File Operations
- Global Variables
- Regular Expressions
- Multi-threading
- phonenumbers
- Breadcrumbs
- βΊ Python Examples
- βΊ βΊ List Operations
- βΊ βΊ βΊ Python Program to Find Largest Number in a List
- Python Strings
- Python List Tutorials
- Python Lists
- Python List Operations
- Create Lists
- Python β Create an empty list
- Python β Create a list of size n
- Python β Create a list of numbers from 1 to n
- Python β Create a list of strings
- Python β Create a list of objects
- Python β Create a list of empty lists
- Access Lists
- Python List β Access elements
- Python List β Get item at specific index
- Python List β Get first element
- Python List β Get last element
- Python List β Iterate with index
- Python List β While loop
- Python List β For loop
- Python List β Loop through items
- Python β Unpack List
- List Properties
- Python β List length
- List Methods
- Python List Methods
- Python List append()
- Python List clear()
- Python List copy()
- Python List count()
- Python List extend()
- Python List index()
- Python List insert()
- Python List pop()
- Python List remove()
- Python List reverse()
- Python List sort()
- Update / Transform Lists
- Python β Add item to list
- Python β Remove specific item from list
- Python β Remove item at specific index from list
- Python β Remove all occurrences of an item from list
- Python β Remove duplicates from list
- Python β Append a list to another list
- Python β Reverse list
- Python β Sort list
- Python β Sort list in descending order
- Python β Shuffle list
- Python β Convert list into list of lists
- Checking Operations on Lists
- Python - Check if list is empty
- Python - Check if element is present in list
- Python - Check if value is in list using "in" operator
- Python - Check if list contains all elements of another list
- List Finding Operations
- Python β Count the occurrences of items in a List with a specific value
- Python β Find duplicates in list
- Python β Find unique elements in list
- Python β Find index of specific item in list
- Python β Insert item at specific position in list
- Python β Find element with most number of occurrences in the list
- Python β Find element with least number of occurrences in the list
- List Comprehension Operations
- Python List Comprehension
- Python List Comprehension with two lists
- Python List Comprehension with If condition
- Python List Comprehension with multiple conditions
- Python List Comprehension with List of Lists
- Python List Comprehension with nested For loops
- List Filtering
- Python β Filter list
- Python β Filter list of strings that start with specific prefix string
- Python β Filter list based on datatype
- Python β Get list without last element
- Python β Traverse list except last element
- Python List β Get first N elements
- Python List β Get last N elements
- Python List β Slice
- Python List β Slice first N elements
- Python List β Slice last N elements
- Python List β Slice all but last
- Python List β Slice reverse
- List Conversions to other Types
- Python β Convert List to Dictionary
- Python β Convert dictionary keys to list
- Python β Convert dictionary values to list
- Python β Convert list to tuple
- Python β Convert tuple to list
- Python β Convert list to set
- Python β Convert set to list
- Python β Convert list to string
- Python β Convert CSV string to list
- Python β Convert list to CSV string
- Python β Convert string (numeric range) to a list
- Python β Convert list of lists to a flat list
- Python - Convert list of strings into list of integers
- Python - Convert list of strings into list of floats
- Python - Convert list of integers into list of strings
- Python - Convert list of floats into list of strings
- Numeric Lists
- Python β Find average of numbers in list
- Python β Find largest number in list
- Python β Find smallest number in list
- Python β Find sum of all numbers in numeric list
- Python β Find product of all numbers in numeric list
- Python β Sort numbers
- String Lists
- Python β Compare lists lexicographically
- Python β Find shortest string in list
- Python β Find longest string in list
- Python β Find most occurred string in a list
- Python β Find least occurred string in a list
- Python β Sort list of strings based on length
- Python β Sort a list alphabetically
- Python - Join list with new line
- Python - Join list with comma
- Python - Join list with space
- Printing Lists
- Python - Print list
- Python - Print list without brackets
- Python - Print list without commas
- Python - Print list without quotes
- Python - Print list without spaces
- Python - Print list without brackets and commas
- Python - Print list without brackets and quotes
- Python - Print list without quotes and commas
- Python - Print list in reverse order
- Python - Print list in JSON format
- Python - Print list with double quotes
- Python - Print list with index
- Python - Print list as column
- Python - Print list as lines
- Python - Print elements at even indices in List
- Python - Print elements at odd indices in List
- Exceptions with Lists
- Python List ValueError: too many values to unpack
- Python List ValueError: not enough values to unpack
- List Other Topics
- Python List of Lists
- Python β Print a list
- Python List vs Set
- Python Dictionary
Python Program to Find Largest Number in a List
- Find the largest number in given list using sort()
- Find largest number in list using For loop
Find Largest Number in Python List
You can find largest number of a list in Python using sort() function or more basic for loop.
Using sort() function is kind of concise, but using For Loop is efficient. We will go through these two approaches with examples.
1. Find the largest number in given list using sort()
We know that sort() builtin function sorts a list in ascending or descending order. After you sort a list, you have your largest number at the end of the list if you have sorted in ascending order or at the start of the list if you have sorted in descending order.
In the following example, we will sort the given list in ascending order. Of course, last number of the sorted list is our required largest number.
Python Program
a[-1] fetches the last element in the list.
2. Find largest number in list using For loop
Though finding the largest number using sort() function is easy, using Python For Loop does it relatively faster with less number of operations. Also, the contents of the list are unchanged.
In this example, we have assumed a list and initialized largest number ln variable to the first element of the list. If there are no elements in the list, ln is initialized with None .
Iterate the loop for each element in the list. During each iteration, we check if the largest number is less than this element. If that is the case, we update our largest number with the element.
When you complete traversing the list, you will end up with the largest number of the list in your variable.
In this tutorial of Python Examples , we learned some of the ways to find largest number in Python list with the help of well detailed Python example programs.
Related Tutorials

- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Python program to find the largest number in a list
In this article, we will learn about the solution to the problem statement given below.
Problem statement − We are given a list, we need to calculate the largest element of the list.
Here we will take the help of built-in functions to reach the solution of the problem statement
Using sort() function
Using max() function.
Live Demo
We can also take input from the user by the code given below
In this article, we have learned how to get the largest element from the list.

Related Articles
- Python program to find largest number in a list
- Python program to find the second largest number in a list
- Python Program to Find the Second Largest Number in a List Using Bubble Sort
- Python Program to Find the Largest Element in a Doubly Linked List
- Program to find lexicographically largest mountain list in Python
- Python program to find Largest, Smallest, Second Largest, and Second Smallest in a List?
- Python program to find N largest elements from a list
- Golang Program to Print the Largest Even and Largest Odd Number in a List
- Python program to find the smallest number in a list
- Program to find the largest grouping of anagrams from a word list in Python
- C# program to find Largest, Smallest, Second Largest, Second Smallest in a List
- Program to find largest kth index value of one list in Python
- Python Program for Find largest prime factor of a number
- Program to find largest sum of non-adjacent elements of a list in Python
- Python Program to find the largest element in a tuple
Kickstart Your Career
Get certified by completing the course
- How it works
- Homework answers
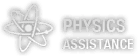
Answer to Question #225605 in Python for Reclimet
Largest Number in the List
This Program name is Largest Number in the List. Write a Python program to Largest Number in the List, it has two test cases
The below link contains Largest Number in the List question, explanation and test cases
https://docs.google.com/document/d/120K51mYmZkwWPZ-KiP4VWxPG52b8P7tB/edit?usp=sharing&ouid=104486799211107564921&rtpof=true&sd=true
We need exact output when the code was run
Need a fast expert's response?
and get a quick answer at the best price
for any assignment or question with DETAILED EXPLANATIONS !

Leave a comment
Ask your question, related questions.
- 1. Product of Elements in the ListThis Program name is Product of Elements in the List. Write a Python
- 2. Reverse the SentenceThis Program name is Reverse the Sentence. Write a Python program to Reverse the
- 3. DiamondGiven an integer value N, write a program to print a number diamond of 2*N -1 rows as shown b
- 4. Sum of N terms in Harmonic series:Given integer N as input, write a program to display the sum of th
- 5. Diamond CrystalGiven an integer value N, write a program to print a diamond pattern of 2*N rows as s
- 6. The game is played as follows:The game consists of 3 turns, and the aim of the game is to score as
- 7. Given a M x N matrix, write a program to print the matrix after ordering all the elements of the mat
- Programming
- Engineering
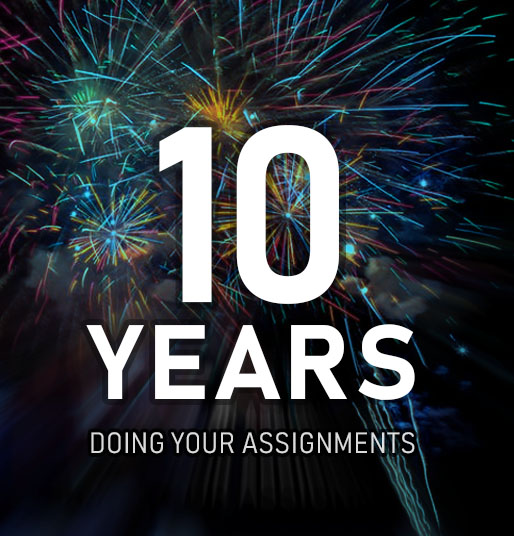
Who Can Help Me with My Assignment
There are three certainties in this world: Death, Taxes and Homework Assignments. No matter where you study, and no matter…
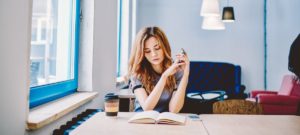
How to Finish Assignments When You Can’t
Crunch time is coming, deadlines need to be met, essays need to be submitted, and tests should be studied for.…
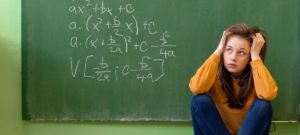
How to Effectively Study for a Math Test
Numbers and figures are an essential part of our world, necessary for almost everything we do every day. As important…
5 Best Ways to Find the Largest Number Possible from a List of Numbers in Python
π‘ Problem Formulation: You are given a list of non-negative integers and your task is to arrange them such that they form the largest possible number. For instance, given [3, 34, 302, 50, 31] the largest formed number would be "5034330231" .
Method 1: Custom Sort with String Comparison
To determine the correct order of numbers, we compare them as strings. Specifically, for any two numbers, say x and y, we compare the concatenated strings “xy” and “yx”, and arrange the numbers based on which concatenation yields a larger number. This method relies on the Python sorted function with a custom comparator.
Here’s an example:
Output: "5034330231"
This code snippet defines a function largest_num which first verifies that all elements in the list are non-negative integers, then sorts the numbers as strings, concatenating them in the order that produces the largest possible number. Zero-padding handling is included to ensure the output is not ‘0’ repeated.
Method 2: Using functools.cmp_to_key
The functools module provides a cmp_to_key function that converts a comparison function into a key function suitable for the sorted() method. The key function must be able to take two arguments and return a negative, zero, or positive number depending on the desired order. By defining a custom compare function, we can sort the numbers as per their concatenation results.
The compare function implements the desired ordering by calculating the difference between the two possible concatenations. The largest_num function sorts the array of strings using this comparator, then joins and returns the result.
Method 3: Using a Max Heap
This method entails building a max heap from the list after transforming each number into a string. By comparing these strings lexicographically, we maintain a heap in which the top element represents the largest number when combined with others. The built-in library heapq can be used here.
The largest_num function creates a max-heap from the list of stringified numbers and then pops out elements from the heap to form the largest number. Keep in mind that the underscore functions of heapq are used for illustrative purposes; they are considered private and may change in future Python releases.
Method 4: Recursive Backtracking
Recursive backtracking is a brute force method which involves generating all possible permutations of the list’s elements to find the maximum number. This method guarantees the result but is not efficient for large lists due to its factorial time complexity.
The snippet uses itertools.permutations which provides all possible arrangements of the list elements. The one which leads to the largest concatenated number is selected as max_num and returned.
Bonus Method 5: Concise One-Liner
The previous methods can be condensed into a one-liner using Python’s sorted() function combined with the join operation. This method provides a quick and easy solution but may sacrifice a bit of readability.
This one-liner performs a similar custom sort as Method 1 but is presented in a compact form. Notice the lambda x: x*5 trick, which ensures that the comparisons are made by sufficiently long concatenated string repetitions so that the order is determined correctly.
Summary/Discussion
- Method 1: Custom Sort with String Comparison. Itβs efficient and easy to understand. However, it may not be intuitive for those unfamiliar with sorting algorithms.
- Method 2: Using functools.cmp_to_key . Works well and leverages Pythonβs standard library, making the code clean. Can be tricky to handle edge cases like leading zeros.
- Method 3: Using a Max Heap. Provides good performance. However, it requires understanding of heap data structures, and the use of Pythonβs private heap methods is not recommended.
- Method 4: Recursive Backtracking. Though it’s not efficient for large lists, it can be useful for small sets and is straightforward in its approach.
- Method 5: Concise One-Liner. Itβs a quick, clever solution for simple scenarios. But it may be less readable and harder to debug.
Emily Rosemary Collins is a tech enthusiast with a strong background in computer science, always staying up-to-date with the latest trends and innovations. Apart from her love for technology, Emily enjoys exploring the great outdoors, participating in local community events, and dedicating her free time to painting and photography. Her interests and passion for personal growth make her an engaging conversationalist and a reliable source of knowledge in the ever-evolving world of technology.
- Free Python 3 Tutorial
- Control Flow
- Exception Handling
- Python Programs
- Python Projects
- Python Interview Questions
- Python Database
- Data Science With Python
- Machine Learning with Python
- Python | Delete elements in range
- Python | Ways to format elements of given list
- Find sum and average of List in Python
- Python | Merging duplicates to list of list
- Python Program to get Maximum product of elements of list in a 2D list
- Python | Interleave multiple lists of same length
- Python | Combine two lists by maintaining duplicates in first list
- Python | Optional padding in list elements
- Python | List Initialization with alternate 0s and 1s
- Python | Operation to each element in list
- Python | Integrity Sorting in two lists
- Python | Dividing two lists
- Python | Remove all digits from a list of strings
- Python | Convert a list into tuple of lists
- Python program to find the Strongest Neighbour
- Python | Associating a single value with all list items
- Counting number of unique values in a Python list
- Python | Merge elements of sublists
- Python Program to Swap Two Elements in a List
Python program to find second largest number in a list
Given a list of numbers, the task is to write a Python program to find the second largest number in the given list.
Examples:
Input: list1 = [10, 20, 4] Output: 10 Input: list2 = [70, 11, 20, 4, 100] Output: 70
Method 1: Sorting is an easier but less optimal method. Given below is an O(n) algorithm to do the same.
The time complexity of this code is O(n) as it makes a single linear scan of the list to find the second largest element. The operations performed in each iteration have a constant time complexity, so the overall complexity is O(n). The space complexity is O(1) as the code only uses a constant amount of extra space.
Method 2: Sort the list in ascending order and print the second last element in the list.
Time Complexity: O(nlogn) Auxiliary Space: O(1)
Method 3: By removing the max element from the list
Time Complexity: O(n) Auxiliary Space: O(1)
Method 4: Find the max list element on inputs provided by the user
Time Complexity: O(NlogN) where N is the number of elements in the Array.
Auxiliary Space: O(1)
Method 5: Traverse once to find the largest and then once again to find the second largest.
Time Complexity: O(N)
Method 6 : Using list comprehension
Method: Using lambda function
Method: Using enumerate function
Method : Using heap
One approach is to use a heap data structure. A heap is a complete binary tree that satisfies the heap property: the value of each node is at least as great as the values of its children. This property allows us to efficiently find the largest or smallest element in the heap in O(1) time.
To find the second largest element in a list using a heap, we can first build a max heap using the elements in the list. Then, we can remove the root element (which is the largest element in the heap) and find the new root element, which will be the second largest element in the heap.
Here is an example of how this can be done in Python:
This approach has a time complexity of O(n log n) for building the heap and O(log n) for finding the second largest element, making it more efficient than the methods mentioned in the article which have a time complexity of O(n).
Method : Using numpy.argsort() function.
note: install numpy module using command “pip install numpy”
Here’s the implementation of finding the second largest number in a list using numpy.argsort() function.
Create a numpy array from the given list. Use numpy.argsort() function to find the indices that would sort the array. Find the second last index from the sorted indices. Return the element at that index from the original array.
The time complexity of the code is O(nlogn), where n is the number of elements in the list. This is because the numpy.argsort() function has a time complexity of O(nlogn) for sorting the input list, and selecting the second largest element from the sorted list takes constant time.
The auxiliary space of the code is O(n), where n is the number of elements in the list. This is because the numpy.argsort() function creates a sorted copy of the input list and stores it in memory.
Method : Using sorted()
Algorithm :
- The list of integers is initialized with values [2, 1, 8, 7, 3, 0].
- The original list is printed using the print() function and the list variable.
- The sorted() function is used to sort the list in descending order, and the sorted list is assigned to a new variable named list1.
- The sorted list is printed using the print() function and the list1 variable.
- The second largest number is printed using the print() function and the index of the second largest number in the sorted list (which is 1, since list indexes start from 0).
Time Complexity : O(n log n)
Auxiliary Space : O(n)
Please Login to comment...
Similar reads.
- Python list-programs
- python-list
- 5 Reasons to Start Using Claude 3 Instead of ChatGPT
- 6 Ways to Identify Who an Unknown Caller
- 10 Best Lavender AI Alternatives and Competitors 2024
- The 7 Best AI Tools for Programmers to Streamline Development in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
CopyAssignment
We are Python language experts, a community to solve Python problems, we are a 1.2 Million community on Instagram, now here to help with our blogs.
Largest Number in the list in Python
Problem statement:.
In the problem of largest number in the list in python, we are given a list and we need to find the largest number from that list. We can use any method like max or sort . Also, there are many solutions and approaches to this problem, you can try any one but we are going to use max(built-in method).
Code to find largest number in the list in python:
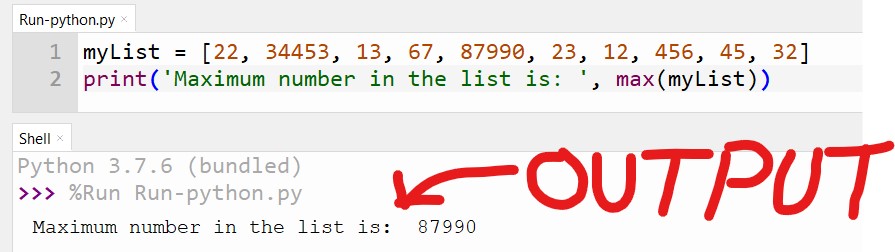
- Hyphenate Letters in Python
- Earthquake in Python | Easy Calculation
- Striped Rectangle in Python
- Perpendicular Words in Python
- Free shipping in Python
- Raj has ordered two electronic items Python | Assignment Expert
- Team Points in Python
- Ticket selling in Cricket Stadium using Python | Assignment Expert
- Split the sentence in Python
- String Slicing in JavaScript
- First and Last Digits in Python | Assignment Expert
- List Indexing in Python
- Date Format in Python | Assignment Expert
- New Year Countdown in Python
- Add Two Polynomials in Python
- Sum of even numbers in Python | Assignment Expert
- Evens and Odds in Python
- A Game of Letters in Python
- Sum of non-primes in Python
- Smallest Missing Number in Python
- String Rotation in Python
- Secret Message in Python
- Word Mix in Python
- Single Digit Number in Python
- Shift Numbers in Python | Assignment Expert
- Weekend in Python
- Temperature Conversion in Python
- Special Characters in Python
- Sum of Prime Numbers in the Input in Python
Author: Harry

Search….
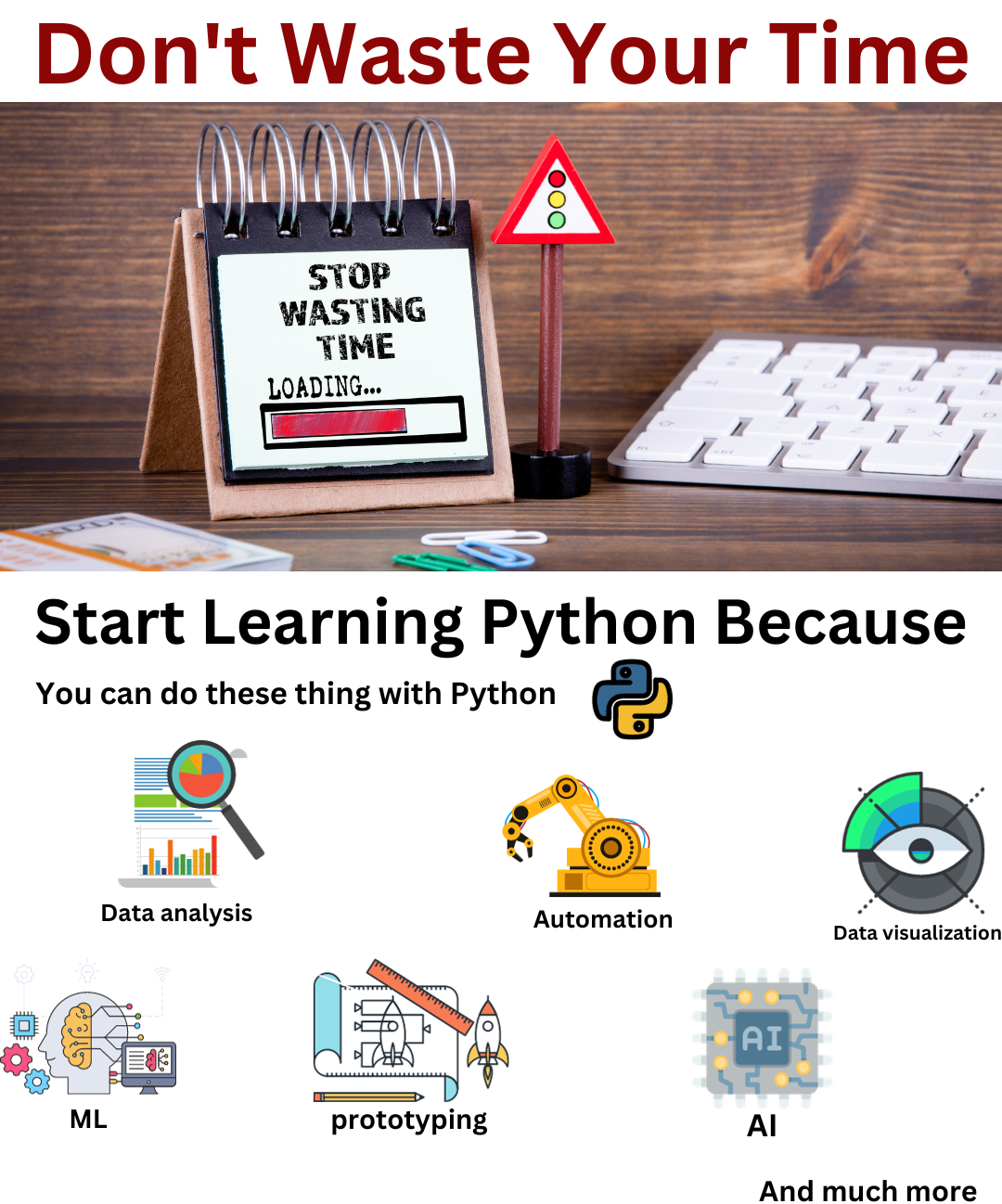
Machine Learning
Data Structures and Algorithms(Python)
Python Turtle
Games with Python
All Blogs On-Site
Python Compiler(Interpreter)
Online Java Editor
Online C++ Editor
Online C Editor
All Editors
Services(Freelancing)
Recent Posts
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
Β© Copyright 2019-2023 www.copyassignment.com. All rights reserved. Developed by copyassignment

IMAGES
VIDEO
COMMENTS
Question #228701. Largest Number in the List. You are given space -separated integers as input. Write a program to print the maximum number among the given numbers. Input. The first line of input contains space -separated integers. Explanation. In the example, the integers given are. 1, 0, 3, 2, 9, 8.
Largest Number in the List: You are given space-separated integers as input. Write a program to print the maximum number among the given numbers. Input: The first line of input contains space-separated integers. Explanation: In the example, the integers given are . 1, 0, 3, 2, 9, 8. The maximum number present among them is 9. So, the output ...
Auxiliary Space: O(k), where k is the number of largest elements to be returned. In this case, k is 1, so the auxiliary space is O(1). Find Largest Number in a List Using np.max() method. Initialize the test list. Use np.array() method to convert the list to numpy array. Use np.max() method on numpy array which gives the max element in the list.
Python Program to find the Largest Number in a List Using the sort function. The sort function sorts the List elements in ascending order. Next, we use the Index position to print the Last element in a List. a = [10, 50, 60, 80, 20, 15] a.sort() print(a[5]) Output. 80 Using sort() function example 2. This largest list number program is the same ...
# 4- Sort the list in ascending order and print the last element in the list. numbers_lst. sort # printing the last element, which is in this case the largest one print (" Using the sort list method: ", numbers_lst [-1])
π‘ Problem Formulation: When working with lists in Python, a common task is to determine the largest number within a collection. Imagine we have a list such as [15, 30, 56, 9, 18]; our aim is to identify the greatest value, which, in this case, is 56.. Method 1: Using Python's max() function. The most straightforward method to find the largest number in a Python list is to use the built-in ...
As you might've already guessed, know or even imagine, there are certainly several ways to find the largest number in a list in Python, as in any other coding scenario. Let's break down some of the most common ones I've used when it comes to this type of situation.
Find largest number in list using For loop. Though finding the largest number using sort () function is easy, using Python For Loop does it relatively faster with less number of operations. Also, the contents of the list are unchanged. Python Program. a = [18, 52, 23, 41, 32] # Variable to store largest number.
In this video, we are going to see 3 different approaches to finding the largest number in a list in Python. Approach: 1) Initialize a max variable with the first element of the list and traverse. the whole list and simultaneously update the max variable. 2) Sorting the list places the largest number in the last position, we can sort the list ...
z = num. return z. high() print(z) I want a function that returns the highest number from a list without using the max () inbuilt python function. Whenever I try and run this, i get this error: line 6, in high. if num > z: UnboundLocalError: local variable 'z' referenced before assignment.
Python program to find the largest number in a list - In this article, we will learn about the solution to the problem statement given below.Problem statement β We are given a list, we need to calculate the largest element of the list.Here we will take the help of built-in functions to reach the solution of the problem statementUsing sort() function
Product of Elements in the ListThis Program name is Product of Elements in the List. Write a Python ; 2. Reverse the SentenceThis Program name is Reverse the Sentence. Write a Python program to Reverse the; 3. DiamondGiven an integer value N, write a program to print a number diamond of 2*N -1 rows as shown b; 4.
This approach is a clever one-liner that cleverly uses Python's list comprehension and heapq.nlargest() function to find all elements except the 'n-1' largest, then applies max() to get the nth largest. It's an elegant and minimalistic approach but may be inefficient for large 'n' due to repeated calculations.
π‘ Problem Formulation: You are given a list of non-negative integers and your task is to arrange them such that they form the largest possible number. For instance, given [3, 34, 302, 50, 31] the largest formed number would be "5034330231".. Method 1: Custom Sort with String Comparison. To determine the correct order of numbers, we compare them as strings.
Write a Python program to get the largest number from a list. Sample Output: In the above code -. def max_num_in_list ( list ): -> This code defines a function called max_num_in_list that takes a single argument list. This function will be used to find the maximum number in the list. max = list [ 0 ] -> We assume the list is not empty.
Output: 45. The time complexity of the code is O(nlogn), where n is the number of elements in the list. This is because the numpy.argsort() function has a time complexity of O(nlogn) for sorting the input list, and selecting the second largest element from the sorted list takes constant time.
Code to find largest number in the list in python: myList = [22, 34453, 13, 67, 87990, 23, 12, 456, 45, 32] print ('Maximum number in the list is: ', max (myList))
x = [6,5,5,4] z = [5,5,4,3] I want to find the largest number in each list that is not 5. So the max of x is 6 and the max of z is 4. My ideas: Remove all 5 from both lists then find the max. OR. If 5 is the max, then return the second-highest number. If 5 is not the max, return the max.
I need to create a function that will print the smallest, largest,and average of n amount of numbers inputed by user.. My function, so far, can print the average and largest value of n amount of numbers entered by user but I'm stuck on finding the smallest value.. This is what I have so far: