- Python Home
- ▼Python Exercises
- Exercises Home
- ▼Python Basic
- Basic - Part-I
- Basic - Part-II
- Python Programming Puzzles
- Mastering Python
- ▼Python Advanced
- Python Advanced Exercises
- ▼Python Control Flow
- Condition Statements and Loops
- ▼Python Data Types
- List Advanced
- Collections
- ▼Python Class
- ▼Python Concepts
- Python Unit test
- Python Exception Handling
- Python Object-Oriented Programming
- ▼Functional Programming
- Filter Function
- ▼Date and Time
- Pendulum Module
- ▼File Handling
- CSV Read, Write
- ▼Regular Expressions
- Regular Expression
- ▼Data Structures and Algorithms
- Search and Sorting
- Linked List
- Binary Search Tree
- Heap queue algorithm
- ▼Advanced Python Data Types
- Boolean Data Type
- None Data Type
- Bytes and Byte Arrays
- Memory Views exercises
- Frozenset Views exercises
- NamedTuple exercises
- OrderedDict exercises
- Counter exercises
- Ellipsis exercises
- ▼Concurrency and Threading
- Asynchronous
- ▼Python Modules
- Operating System Services
- SQLite Database
- ▼Miscellaneous
- Cyber Security
- Generators Yield
- ▼Python GUI Tkinter, PyQt
- Tkinter Home
- Tkinter Basic
- Tkinter Layout Management
- Tkinter Widgets
- Tkinter Dialogs and File Handling
- Tkinter Canvas and Graphics
- Tkinter Events and Event Handling
- Tkinter Custom Widgets and Themes
- Tkinter File Operations and Integration
- PyQt Widgets
- PyQt Connecting Signals to Slots
- PyQt Event Handling
- ▼Python NumPy
- Python NumPy Home
- ▼Python urllib3
- Python urllib3 Home
- ▼Python Metaprogramming
- Python Metaprogramming Home
- ▼Python GeoPy
- Python GeoPy Home
- ▼BeautifulSoup
- BeautifulSoup Home
- ▼Arrow Module
- ▼Python Pandas
- Python Pandas Home
- ▼Pandas and NumPy Exercises
- Pandas and NumPy Home
- ▼Python Machine Learning
- Machine Learning Home
- TensorFlow Basic
- ▼Python Web Scraping
- Web Scraping
- ▼Python Challenges
- Challenges-1
- ▼Python Mini Project
- Python Projects
- ▼Python Natural Language Toolkit
- Python NLTK
- ▼Python Project
- Novel Coronavirus (COVID-19)
- ..More to come..

Python Math: - Exercises, Practice, Solution
Python math [94 exercises with solution].
[ An editor is available at the bottom of the page to write and execute the scripts. Go to the editor ]
1. Write a Python program to convert degrees to radians. Note : The radian is the standard unit of angular measure, used in many areas of mathematics. An angle's measurement in radians is numerically equal to the length of a corresponding arc of a unit circle; one radian is just under 57.3 degrees (when the arc length is equal to the radius). Test Data: Degree : 15 Expected Result in radians: 0.2619047619047619 Click me to see the sample solution
3. Write a Python program to calculate the area of a trapezoid. Note : A trapezoid is a quadrilateral with two sides parallel. The trapezoid is equivalent to the British definition of the trapezium. An isosceles trapezoid is a trapezoid in which the base angles are equal so. Test Data: Height : 5 Base, first value : 5 Base, second value : 6 Expected Output: Area is : 27.5 Click me to see the sample solution
5. Write a Python program to calculate the surface volume and area of a cylinder. Note: A cylinder is one of the most basic curvilinear geometric shapes, the surface formed by the points at a fixed distance from a given straight line, the axis of the cylinder. Test Data: volume : Height (4), Radius(6) Expected Output: Volume is : 452.57142857142856 Surface Area is : 377.1428571428571 Click me to see the sample solution
6. Write a Python program to calculate the surface volume and area of a sphere. Note: A sphere is a perfectly round geometrical object in three-dimensional space that is the surface of a completely round ball. Test Data: Radius of sphere : .75 Expected Output : Surface Area is : 7.071428571428571 Volume is : 1.7678571428571428 Click me to see the sample solution
7. Write a Python program to calculate the arc length of an angle. Note: In a planar geometry, an angle is the figure formed by two rays, called the sides of the angle, sharing a common endpoint, called the vertex of the angle. Angles formed by two rays lie in a plane, but this plane does not have to be a Euclidean plane. Test Data: Diameter of a circle : 8 Angle measure : 45 Expected Output : Arc Length is : 3.142857142857143 Click me to see the sample solution
8. Write a Python program to calculate the area of the sector. Note: A circular sector or circle sector, is the portion of a disk enclosed by two radii and an arc, where the smaller area is known as the minor sector and the larger being the major sector. Test Data: Radius of a circle : 4 Angle measure : 45 Expected Output: Sector Area: 6.285714285714286 Click me to see the sample solution
9. Write a Python program to calculate the discriminant value. Note: The discriminant is the name given to the expression that appears under the square root (radical) sign in the quadratic formula. Test Data: The x value : 4 The y value : 0 The z value : -4 Expected Output: Two Solutions. Discriminant value is : 64.0 Click me to see the sample solution
10. Write a Python program to find the smallest multiple of the first n numbers. Also, display the factors. Test Data: If n = (13) Expected Output : [13, 12, 11, 10, 9, 8, 7] 360360 Click me to see the sample solution
11. Write a Python program to calculate the difference between the squared sum of the first n natural numbers and the sum of squared first n natural numbers.(default value of number=2). Test Data: If sum_difference(12) Expected Output : 5434 Click me to see the sample solution
12. Write a Python program to calculate the sum of all digits of the base to the specified power. Test Data: If power_base_sum(2, 100) Expected Output : 115 Click me to see the sample solution
13. Write a Python program to find out if the given number is abundant. Note: In number theory, an abundant number or excessive number is a number for which the sum of its proper divisors is greater than the number itself. The integer 12 is the first abundant number. Its proper divisors are 1, 2, 3, 4 and 6 for a total of 16. Test Data: If is_abundant(12) If is_abundant(13) Expected Output: True False Click me to see the sample solution
14. Write a Python program to find out if the given number is abundant. Note: Amicable numbers are two different numbers so related that the sum of the proper divisors of each is equal to the other number. (A proper divisor of a number is a positive factor of that number other than the number itself. For example, the proper divisors of 6 are 1, 2, and 3.) Test Data: If amicable_numbers_sum(9999) If amicable_numbers_sum(999) If amicable_numbers_sum(99) Expected Output: 31626 504 0 Click me to see the sample solution
15. Write a Python program to return the sum of all divisors of a number. Test Data: If number = 8 If number = 12 Expected Output: 7 16 Click me to see the sample solution
16. Write a Python program to print all permutations of a given string (including duplicates). Click me to see the sample solution
17. Write a Python program to print the first n lucky numbers. Lucky numbers are defined via a sieve as follows. Begin with a list of integers starting with 1 : 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, . . . . Now eliminate every second number : 1, 3, 5, 7, 9, 11, 13, 15, 17, 19, 21, 23, 25, ... The second remaining number is 3, so remove every 3rd number: 1, 3, 7, 9, 13, 15, 19, 21, 25, ... The next remaining number is 7, so remove every 7th number: 1, 3, 7, 9, 13, 15, 21, 25, ... Next, remove every 9th number and so on. Finally, the resulting sequence is the lucky numbers. Click me to see the sample solution
18. Write a Python program to compute square roots using the Babylonian method. Perhaps the first algorithm used for approximating √S is known as the Babylonian method, named after the Babylonians, or "Hero's method", named after the first-century Greek mathematician Hero of Alexandria who gave the first explicit description of the method. It can be derived from (but predates by 16 centuries) Newton's method. The basic idea is that if x is an overestimate to the square root of a non-negative real number S then S / x will be an underestimate and so the average of these two numbers may reasonably be expected to provide a better approximation. Click me to see the sample solution
19. Write a Python program to multiply two integers without using the * operator. Click me to see the sample solution
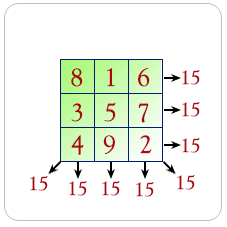
21. Write a Python program to print all primes (Sieve_of_Eratosthenes) smaller than or equal to a specified number. In mathematics, the sieve of Eratosthenes, one of a number of prime number sieves, is a simple, ancient algorithm for finding all prime numbers up to any given limit. It does so by iteratively marking as composite (i.e., not prime) the multiples of each prime, starting with the multiples of 2. Click me to see the sample solution
22. Write a Python program to find the next smallest palindrome of a specified number. Click me to see the sample solution
23. Write a Python program to find the next and previous palindromes of a specified number. Click me to see the sample solution
24. Write a Python program to convert a float to ratio.
Expected Output :
Click me to see the sample solution
25. Write a Python program for the nth Catalan numbers. In combinatorial mathematics, the Catalan numbers form a sequence of natural numbers that occur in various counting problems, often involving recursively-defined objects. They are named after the Belgian mathematician Eugène Charles Catalan (1814 –1894). Click me to see the sample solution
26. Write a Python program to display numbers separated by commas as thousands. Click me to see the sample solution
27. Write a Python program to calculate the distance between two points using latitude and longitude.
28. Write a Python program to calculate the area of a regular polygon.
29. Write a Python program to calculate the wind chill index.
30. Write a Python program to find the roots of a quadratic function.
31. Write a Python program to convert a decimal number to a binary number.
32. Write a Python program to print a complex number and its real and imaginary parts.
33. Write a Python program to add, subtract, multiply, and divide two complex numbers.
34. Write a Python program to get the length and the angle of a complex number.
35. Write a Python program to convert Polar coordinates to rectangular coordinates.
36. Write a Python program to find the maximum and minimum numbers from the specified decimal numbers.
Decimal numbers : 2.45, 2.69, 2.45, 3.45, 2.00, 0.04, 7.25
37. Write a Python program to find the sum of the following decimal numbers and display the numbers in sorted order.
38. Write a Python program to get the square root and exponential of a given decimal number.
Decimal number : 1.44
39. Write a Python program to retrieve the current global context (public properties) for all decimal.
40. Write a Python program to round a specified decimal by setting precision (between 1 and 4).
Sample Number : 0.26598 Original Number : 0.26598 Precision- 1 : 0.3 Precision- 2 : 0.27 Precision- 3 : 0.266 Precision- 4 : 0.2660
41. Write a Python program to round a specified number upwards towards infinity and down towards negative infinity with precision 4.
42. Write a Python program to get the local and default precision.
43. Write a Python program to display fraction instances of the string representation of a number.
Sample data : '0.7', '2.5', '9.32', '7e-1'
44. Write a Python program to create fraction instances of float numbers.
Sample numbers: 0.2, 0.7, 6.5, 6.0
45. Write a Python program to create fraction instances of decimal numbers.
Sample decimal.2' number: Decimal('0), Decimal('0.7'), Decimal('2.5'), Decimal('3.0')
46. Write a Python program to add, subtract, multiply and divide two fractions.
47. Write a Python program to convert a floating point number (PI) to an approximate rational value on the various denominators.
Note: max_denominator=1000000
48. Write a Python program to generate random floating numbers in a specific numerical range.
49. Write a Python program to generate random integers in a specific numerical range.
50. Write a Python program to generate random even integers in a specific numerical range.
51. Write a Python program to get a single random element from a specified string.
52. Write a Python program to shuffle the following elements randomly.
Sample elements : [1, 2, 3, 4, 5, 6, 7]
53. Write a Python program to flip a coin 1000 times and count heads and tails.
54. Write a Python program to print a random sample of words from the system dictionary.
55. Write a Python program to randomly select an item from a list.
56. Write a Python program to calculate the absolute value of a floating point number.
57. Write a Python program to calculate the standard deviation of the following data.
58. Write a Python program to print the floating point of the mantissa, exponent pair.
59. Write a Python program to split the fractional and integer parts of a floating point number.
60. Write a Python program to parse math formulas and put parentheses around multiplication and division.
61. Write a Python program to describe linear regression.
62. Write a Python program to calculate a grid of hexagon coordinates of the given radius given lower-left and upper-right coordinates. The function will return a list of lists containing 6 tuples of x, y point coordinates. These can be used to construct valid regular hexagonal polygons.
63. Write a Python program to create a simple math quiz.
64. Write a Python program to calculate the volume of a tetrahedron.
Note: In geometry, a tetrahedron (plural: tetrahedra or tetrahedrons) is a polyhedron composed of four triangular faces, six straight edges, and four vertex corners. The tetrahedron is the simplest of all the ordinary convex polyhedra and the only one that has fewer than 5 faces.
65. Write a Python program to compute the value of e(2.718281827...) using an infinite series.
66. Write a Python program to create an ASCII waveform.
67. Write a Python program to create a dot string.
68. Write a Python program to create a Pythagorean theorem calculator.
69. Write a Python function to round up a number to specified digits.
70. Write a Python program for a casino simulation.
71. Write a Python program to reverse a range.
72. Write a Python program to create a range for floating numbers.
73. Write a Python program to generate (given an integer n) a square matrix filled with elements from 1 to n raised to the power of 2 in spiral order.
74. Write a Python program to select a random date in the current year.
75. Write a Python program to calculate clusters using the Hierarchical Clustering method.
76. Write a Python program to implement the Euclidean Algorithm to compute the greatest common divisor (GCD).
77. Write a Python program to convert RGB color to HSV color.
78. Write a Python program to find perfect squares between two given numbers.
79. Write a Python program to compute Euclidean distances.
80. Write a Python program to convert an integer to a 2 byte Hex value.
81. Write a Python program to generate a series of distinct random numbers.
82. Write a Python program to convert a given float value to a ratio.
83. Write a Python program to calculate the aliquot sum of a given number.
84. Write a Python program to get the nth tetrahedral number from a given integer(n) value.
85. Write a Python program to get the sum of the powers of all the numbers from start to end (both inclusive).
86. Write a Python program to calculate the Hamming distance between two given values.
87. Write a Python program to cap a number within the inclusive range specified by the given boundary values x and y.
88. Write a Python program to check whether a given number is a Disarium number or an unhappy number.
89. Write a Python program to check if a given number is a repdigit number or not. If the given number is repdigit return true otherwise false. Sample Data: (0) -> True (1) -> True (-1111) -> False (9999999) -> True Click me to see the sample solution
90. Write a Python program to check if a given number is a Harshad number or not. Return True if the number is Harshad otherwise False. Sample Data: (666) -> True (11) -> False (-144) -> None (200) -> True Click me to see the sample solution
91. Write a Python program that accepts an integer number with distinct digits and displays the next number containing only distinct digits. Sample Data: 12345) -> 12346 (99999) -> 102345 (100) -> 102 Click me to see the sample solution
92. Write a Python program that checks whether the absolute difference between two consecutive digits is two or not. Return true otherwise false. Sample Data: (666) -> False (3579) -> True (2468) -> True (20420) -> False Click me to see the sample solution
93. Write a Python program that takes an integer and rearranges the digits to create two maximum and minimum numbers. Click me to see the sample solution
94. Write a Python program to calculate the sum of all prime numbers in a given list of positive integers. Sample Data: ([1, 3, 4, 7, 9]) -> 10 ([]) -> Empty list! ([11, 37, 444]) -> 48 Click me to see the sample solution
Python Code Editor:
More to Come !
Test your Python skills with w3resource's quiz
Follow us on Facebook and Twitter for latest update.
- Weekly Trends and Language Statistics
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Simplest way to solve mathematical equations in Python
I want to solve a set of equations, linear, or sometimes quadratic. I don't have a specific problem, but often, I have been in this situation often.
It is simple to use wolframalpha.com , the web equivalent of Mathematica, to solve them. But that doesn't provide the comfort and convenience of an iPython shell.
Is there a simple library to work on linear and quadratic equations from a python shell?
Personally, I find it extremely convenient to use the Casio 991 MS scientific calculator. I know how to set variables, solve equations, and do a lot. I want such a tool preferably usable from within an ipython shell. I am surprised not to have found any. I'm not impressed enough by sage; perhaps I am missing something.

- Do you know the Maxima Language and the WxMaxima interface? I find it cozier than IPython – Foad S. Farimani Commented Nov 24, 2017 at 9:31
16 Answers 16
sympy is exactly what you're looking for.
- Upvoted. Let's see a demo! I just posted an answer with a demo of sympy to help get people started in it. – Gabriel Staples Commented Aug 20, 2022 at 7:26
You discount the best answer as unacceptable.
Your question is "I want a free Computer Algebra System that I can use in Python."
The answer is "SAGE does that."
Have you looked at maxima/macsyma? SAGE provides bindings for it, and that's one of the more powerful free ones.
http://maxima.sourceforge.net/
Here is how to solve your original question using Python (via Sage). This basically clarifies the remark Paul McMillan makes above.
For inexact solutions, read up on linear programming and sequential quadratic optimization , then search for Python libraries that performs such optimizations for you.
If the equations require integer solutions, you should search for Diophantine equation solvers for Python.
Just note that using a simple solver for Project Euler is missing the point. The fun, and educational part, is learning how to solve it yourself using primitive methods!
- they can give exact solution - depends on model, constraints & tolerance ... for Continuous Problems - use Gradient Descend Algos... for Mixed Integer Problems see Branch and Bound Method - but it is used in LP Solvers internally and you should not care about algo itself, find libs (scipy.optimize, pulp, GEKKO, Networkx etc depending on task to solve) – JeeyCi Commented Nov 13, 2023 at 6:42
A free web-service for solving large-scale systems of nonlinear equations (1 million+) is APMonitor.com. There is a browser interface and an API to Python / MATLAB. The API to Python is a single script (apm.py) that is available for download from the apmonitor.com homepage. Once the script is loaded into a Python code, it gives the ability to solve problems of:
- Nonlinear equations
- Differential and algebraic equations
- Least squares model fitting
- Moving horizon estimation
- Nonlinear model predictive control
For the new user, the APM Python software has a Google Groups forum where a user can post questions. There are bi-weekly webinars that showcase optimization problems in operations research and engineering.
Below is an example of an optimization problem (hs71.apm).
The optimization problem is solved with the following Python script:
Have you looked at SciPy ?
It has an example in the tutorials on solving linear algebra:
http://docs.scipy.org/doc/scipy/reference/tutorial/linalg.html#solving-linear-system

For reference: Wolfram Alpha's solution :
In python, using sympy's solver module (note that it assumes all equations are set equal to zero):
And of course, a != 1000, as a-1000 is the denominator of the two equations.
I have just started using GNU Scientific Library , which however is C library. Looks like there are Python bindings too. So, it might be worth looking at.
I'd use Octave for this but I agree, the syntax of Octave isn't what I'd call thrilling (and the docs always confuse me more than they help, too).
SymPy symbolic Python library demo: symbolically solving math and integrals & pretty-printing the output
From @Autoplectic:
While I have a tendency to write some of the longest answers on Stack Overflow, that is the shortest answer I've seen on Stack Overflow.
Let's add a basic demo.
References and tutorials you'll need:
- Basic Operations
- Simplification
Calculus demo I came up with (see here for the main tutorial I looked at to get started):
Short version:
Longer version:
eRCaGuy_hello_world/math/sympy_integral_and_printing.py :
Note that using pprint(integral) is the same as print(pretty(integral)) .
Output of running the above commands:
The SymPy symbolic math library in Python can do pretty much any kind of math, solving equations, simplifying, factoring, substituting values for variables, pretty printing, converting to LaTeX format, etc. etc. It seems to be a pretty robust solver in my very limited use so far. I recommend trying it out.
Installing it, for me (tested on Linux Ubuntu), was as simple as:

I don't think there is a unified way of dealing with both linear and quadratic (or generally nonlinear) equations simultaneously. With linear systems, python has bindings to linear algebra and matrix packages. Nonlinear problems tend to be solved on a case by case basis.
- SAGE's maxima bindings can deal with pretty much anything you throw at them. – Paul McMillan Commented Oct 29, 2009 at 9:00
It depends on your needs:
If you want an interactive graphical interface, then sage is probably the best solution.
If you want to avoid using a graphical interface, but you still want to do computer algebra, then sympy or maxima may cover your needs. (sympy looks very promising, but it still have a long way to go before they can replace mathematica).
If you don't really need symbolic algrebra, but you need a way to program with matrices, solve differential equations, and minimize functions, then scipy or octave are excelent starting points.
Take a look at this:
http://openopt.org/FuncDesignerDoc#Solving_systems_of_nonlinear_equations
It is extremely easy to use and quite powerful
Well, I just googled into this page by accident. I see many suggestions regarding this and that software tool, but does any tool actually provide an answer? The actual answer is:
[a,b,c] = [200,375,425]
How did I get this? By writing a quick program in the Maxima programming language to find it via "brute force" searching. It only took about 10 minutes to write, seeing as how I'm familiar with the Maxima language. It took a few seconds for the program to run. Here is the program:
euler_solve():= block ( [ a, b, A, B, end:1000],
You can just cut and paste the above code into the wxMaxima user interface, which I run under Ubuntu and not MS Windows. Then you just enter the function name: euler_solve(), hit return, wait a few seconds, and out pops the answer. This particular kind of problem is so simple that you could use any general-purpose programming language to do the search.
- 2 [200, 375, 425] is one potential solution, but there's an infinite set of solutions to this set of equations. [-499000, 999, 499001] is another. One numeric solution is probably not what the OP is looking for. – Nate Commented Mar 16, 2012 at 15:41
- This answer is not relevant since the question is specific Python. The comment about infinite solutions isn't either since the problem that this question refers to (Project Euler 9) has constraints and specifically limits its solution to a single tuple. – anddam Commented Jun 4, 2014 at 10:10
Try applying Bisection method in py to find the root given an interval:

On second thoughts, I went through sage in detail and clearly it is the best math free software available.
Just some of the different python math related libraries, it integrates is absolutely awesome.
Mathematics packages contained in Sage:
Other packages contained in Sage:
- 11 It's impolite to answer your own question with a duplicate of answers provided by others, and then to accept your own answer. Instead, accept someone else's correct answer, and edit your question to include the information you found useful, or reply in a comment. – Paul McMillan Commented Jan 6, 2011 at 6:57
- Paul, these points are not the primary driving force of me, nor the site, I think. Get over that obsession. People are here for sharing information. – lprsd Commented Jan 6, 2011 at 12:25
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged python math numpy scipy equation or ask your own question .
- Featured on Meta
- Announcing a change to the data-dump process
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
Hot Network Questions
- Are elements above 137 possible?
- What to do about chain rubbing on unusually placed chainstay?
- What are the not-winglet wingtips on the CL-415?
- Electron "clouds" in an atom
- Does forgetful functor commute with limits?
- More Square Root
- How did Middleton's Microcynicon survive the Bishop's Ban of 1599?
- How can life which cannot live on the surface of a planet naturally reach the supermajority of the planet's caves?
- Find the newest element
- Report a police emergency at home while abroad
- Is it illegal in Australia to add "chocolate or sultanas" to an Anzac biscuit?
- Tcolorbox theorem with boxed title to the left of the body of the theorem
- Can a general recursive function be defined by Pr(x)?
- Why doesn't Philip directly answer Jesus' query in John 6:5-7?
- Identifying transistor topology in discrete phono preamp
- Why is much harder to encrypt emails, compared to web pages?
- Camera on me and my computer screen all day
- Structure of the headline "Olympics organizers scramble as furor over woke blasphemy grows."
- Airshow pilots not making radio calls - best course of action
- Justify switch after centering switch
- Isn't manual port forwarding actually less safe than UPnP?
- Can't get started with Tao Ex 3.6.6 regarding cardinality of power sets.
- Why try to explain the unexplainable?
- Why is my internet speed slowing by 10x when using this coax cable and splitter setup?
How to Solve Algebraic Equations Using Python
- Python How-To's
- How to Solve Algebraic Equations Using …
Solve Algebraic Equations in One Variable Using the solve() Method From the SymPy Package
Solve algebraic equations in multiple variables using the sympy package, solving algebraic equations in two multiple variables, solving algebraic equations in three multiple variables.
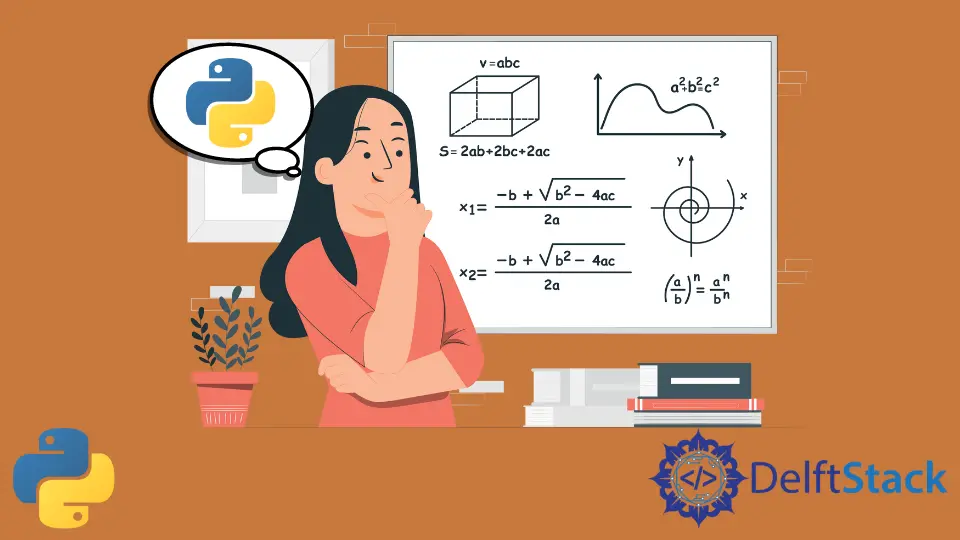
Python has a library for symbolic mathematics, namely, SymPy . This library contains utilities for solving complex mathematical problems and concepts such as matrices, calculus, geometry, discrete mathematics, integrals, cryptography, algebra, etc.
We can use this library to solve algebraic equations. This article will show how to use SymPy to solve algebraic equations in Python.
Following are some ways using which we can install the SymPy module on our machines.
Using the pip Package Manager to Install Sympy
Use the following command to install the SymPy package using pip .
Using Anaconda to Install Sympy
Anaconda is a free Python distribution that includes the SymPy library in its environment by default. One can update the existing version using the following command.
To get a detailed overview of the methods discussed above and some other available methods to install the SymPy library, refer to the official documentation here .
The SymPy library has a solve() function that can solve algebraic equations. This function accepts the following main arguments.
- f : An algebraic equation.
- symbols : The variables for which the equation has to be solved.
- dict : A boolean flag for returning a list of solutions mappings.
- set : A boolean flag for a list of symbols and set of tuples of solutions.
- check : A boolean flag for testing the obtained solutions in the algebraic expression.
- minimal : A boolean flag for fast and minimal testing of the solution.
Now that we are done with some brief theory, let us learn how to use this solve() method to solve algebraic equations with the help of some examples. Refer to the following code for the same.
To solve algebraic equations in multiple variables, we need multiple equations.
For example, we need at least two such equations if we have to solve algebraic equations in two variables. In solving algebraic equations in three variables, we need at least three such equations.
And solving such equations, we need three utilities, namely, symbols , Eq , solve , from the SymPy module.
The symbols function transforms strings of variables into instances of the Symbol class.
The Eq class represents an equal relationship between two objects, specifically expressions. For example, if we have an equation x + y = 3 , then x + y and 3 are the two objects or expressions. For x + y - 5 = x + 6 , x + y - 5 and x + 6 are the two objects.
And, the solve() method uses the above two to solve the equations.
Let’s see how we can use these utilities to solve algebraic equations in two and three variables with the help of some relevant examples.
To understand how to solve algebraic equations in two values using the utilities discussed above, we will consider the following two examples.
Refer to the following Python code for the first example.
Refer to the following Python code for the second example.
To understand how to solve algebraic equations in three values using the utilities discussed above, we will consider the following two examples.
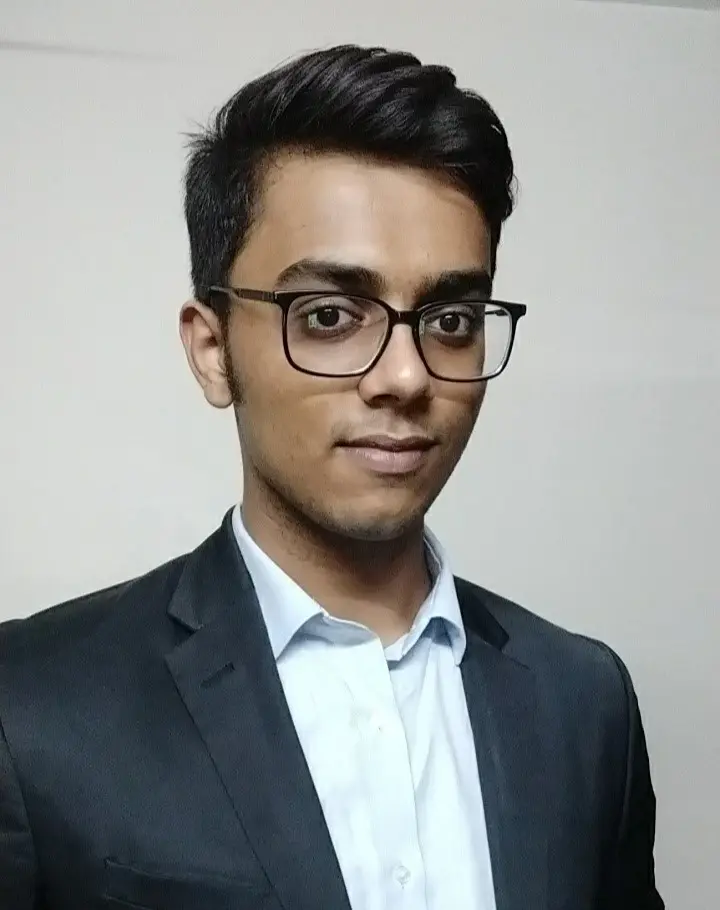
Vaibhav is an artificial intelligence and cloud computing stan. He likes to build end-to-end full-stack web and mobile applications. Besides computer science and technology, he loves playing cricket and badminton, going on bike rides, and doodling.
Related Article - Python Math
- How to Calculate Factorial in Python
- How to Calculate Inverse of Cosine in Python
- How to Calculate Modular Multiplicative Inverse in Python
- How to Fit Poisson Distribution to Different Datasets in Python
- How to Reduce Fractions in Python
- How to Define an Infinite Value in Python
How to Apply Math with Python – Numerical Analysis Explained
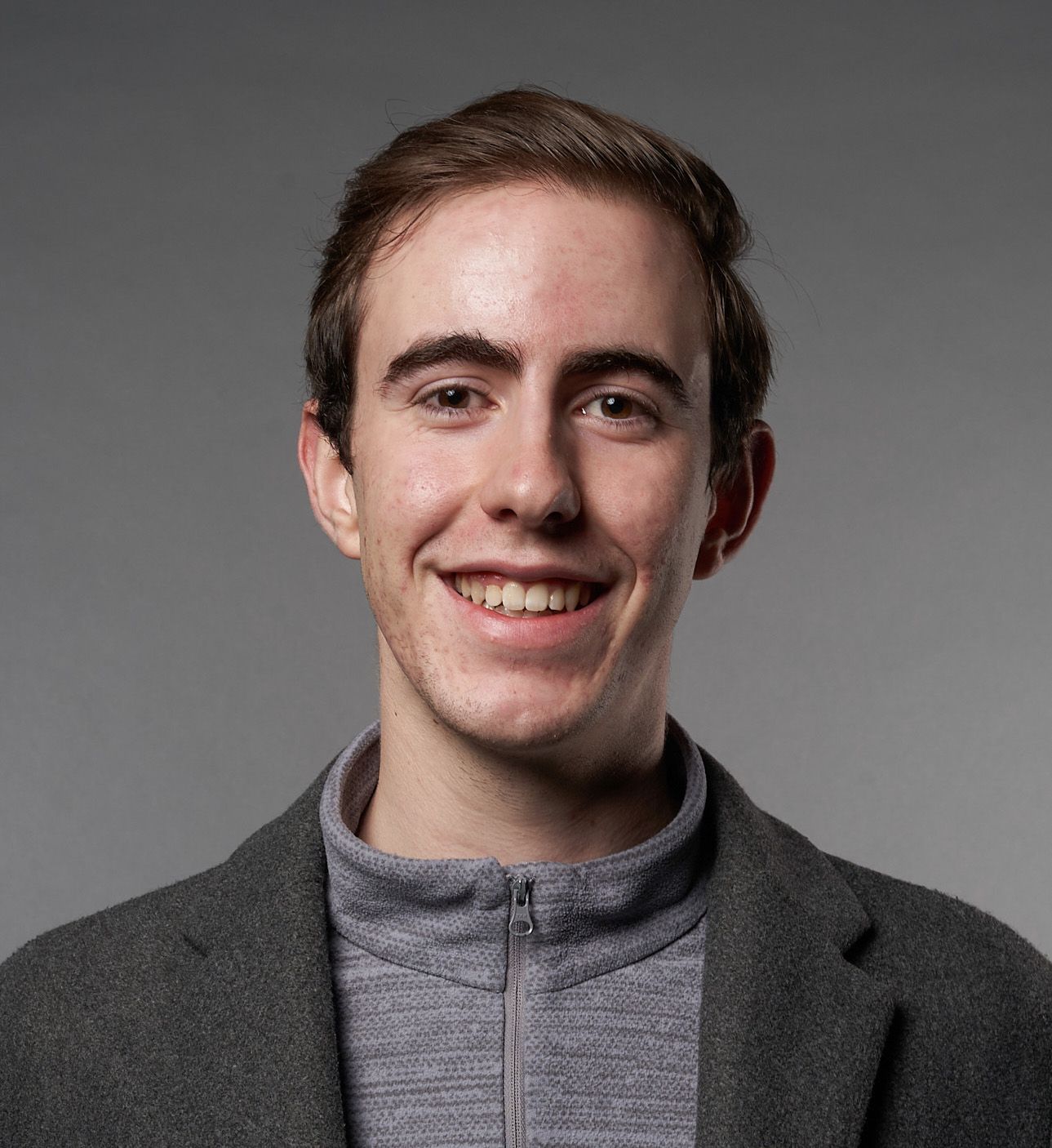
Numerical analysis is the bridge between math and computer science.
Essentially, it is the development of algorithms that approximate solutions that pure math would also solve, but using less computational resources and faster.
This field is very important. Because for most solutions in the real world, we only need good approximations and not the exact solutions.
In this article, we will explore:
- Analogy Illustrating the Importance of Numerical Analysis
Fundamentals of Numerical Analysis
Application of numerical analysis in real-world problems.
- Introduction to Partial Differential Equations (PDEs)
- Introduction to Optimization in Numerical Analysis
An Analogy that Illustrates the Importance of Numerical Analysis
How can we measure the coastline of an island?
If we try to measure every centimeter of every small segment, it would be impossible and probably time-consuming.
Because of the sea, the coastline is always changing at that level of detail.
However, by approximating and measuring in larger segments, we can get a practical measurement of the coastline.
This situation mirrors numerical analysis.
Approximation gives insights in situations where precise measurement is impossible or impractical.
Just as we accept a good estimation of the coastline length, numerical analysis uses approximation to solve hard problems.
Numerical analysis is all about approximation. It is like using binoculars to see a landscape that is very far away. We can't see every leaf. But we get a good enough picture to understand the terrain.
This is crucial in numerical analysis.
In this, we solve hard math problems where exact solutions are either impossible or extremely resource-intensive.
By approximating, we get sufficient good results with less computational effort.
There are many applications of numerical analysis
- In engineering, it enables simulation of structures and fluids.
- In finance, for risk assessment and portfolio optimization.
- In environmental science, it predicts climate patterns.
In each field, numerical analysis is a toolkit to solve problems where pure math just takes too much time, or it is impossible to give good results.
An Introduction to Partial Differential Equations (PDEs)
Partial Differential Equations (PDEs) are equations that describe how quantities like heat, sound, or electricity change in different places and as time goes on.
Solving PDEs is very important. Because it allows us to control these changes.
By allowing us to control them, we can:
- Predict weather patterns.
- Understand sound propagation in different environments.
- Design efficient transportation systems.
- Optimize energy distribution.
However, most PDE can only be approximated with numerical methods.
It is either too hard or impossible to find through normal calculations.
This way, with numerical methods, we are able to solve PDEs which in turn allows us to solve many real life problems.
Numerical Solutions of PDEs with SciPy
Solving PDEs with numerical methods often involves dividing the PDEs in small, manageable parts. Solve each one and then add them up.
SciPy, a Python library for scientific and technical computing, gives many tools for this purpose.
Now, let's solve a heat transfer problem in a rod.
In the below code, we will see line by line how it allows us to know how heat spreads in a rod:
Lets see how thhe code works block by block in the following sections.
How to importing libraries

Here we import 2 python libraries:
These two python libraries are some of the most used in data science.
How to define the head equation and boundary conditions

We create heat_equation(x, y) and boundary_conditions(ya, yb) .
In heat_equation(x, y) we are defining the differential equation we want to solve.
The boundary_conditions(ya, yb) function defines constrains at the start and end of a solution. The condition is that the end of the solution needs to be one unit less than the start.
How to solve the equation
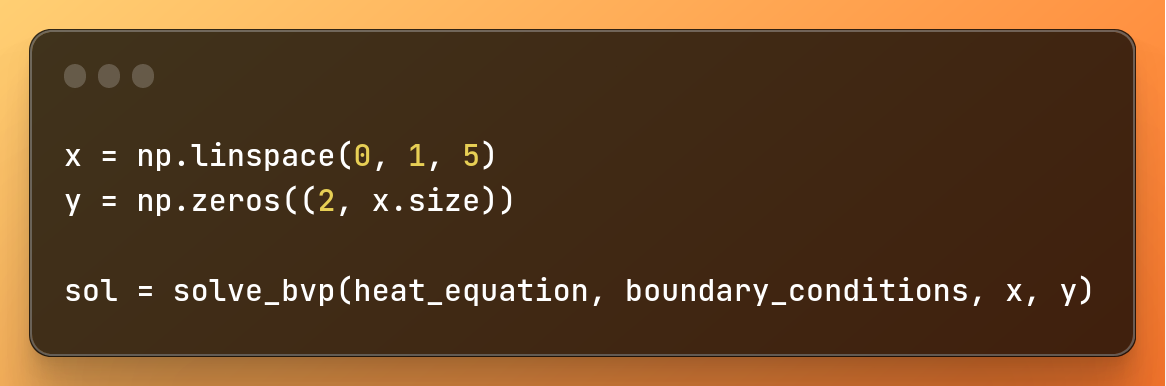
The line sol = solve_bvp(heat_equation, boundary_conditions, x, y) is the solution.
The code solve_bvp stands for solve boundary value problem .
It takes four arguments:
- heat_equation : This is the main problem we are trying to solve.
- boundary_conditions : These are the mathematical constrains at the start and end of a solution.
- x : Are the spots we choose to explore our answers.
- y : Are initial attempts to solve the problem, based on your chosen x values.
An Introduction to Optimization in Numerical Analysis
Optimization is finding the best solution from all solutions. It is like finding the most efficient route in a complex network of roads.
Numerical optimization methods find the most efficient or cost-effective solution to a problem, whether that is:
- Minimizing waste in production.
- Maximizing efficiency in a logistic network.
- Finding best fit for a certain data model.
An Overview of Numerical Optimization Techniques with SciPy
The goal in this example is to minimize transportation cost across a network.
For instance, let's consider an optimization problem in logistics, where the goal is to minimize transportation cost across a network.
SciPy's minimize function can be used to find the best strategy to minimizes cost while meeting all constraints:
Lets explain how the code works block by block.
How to importing the library
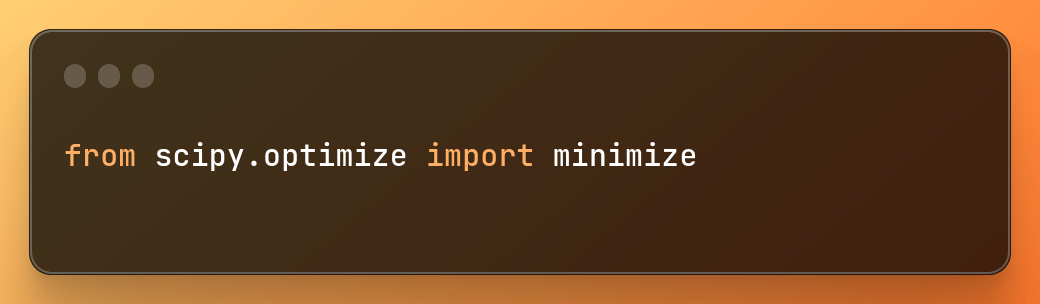
Once again we import the necessary library:
How to defining objective and constraint equation
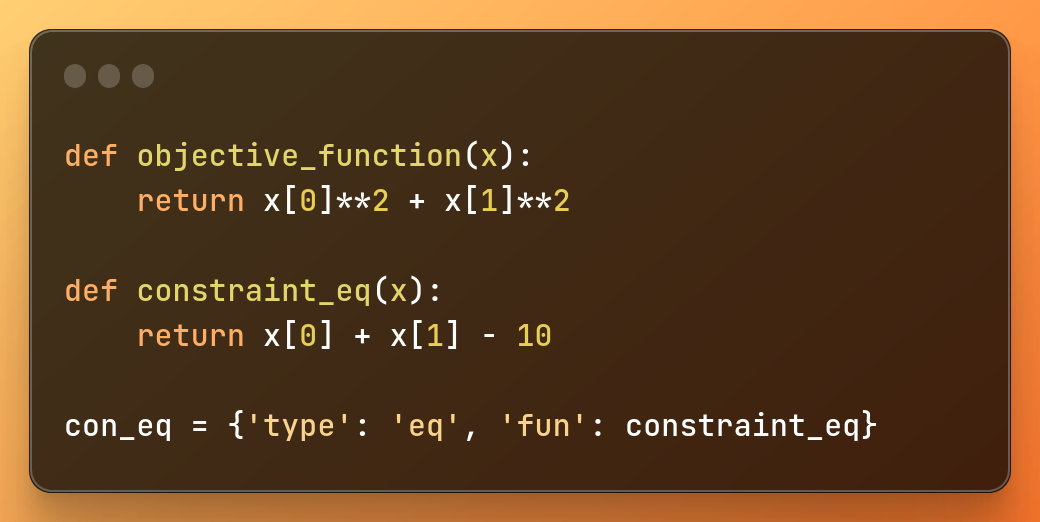
- The objective function is the function we want to minimize to find the best answer.
- The constraint equation is the equation that limits the search space to those x values that fulfill this equation.
con_eq is defined by the following:
- 'type': 'eq' indicates the type of constraint. 'eq' means equality, in other words, the function must equal zero at the solution.
- 'fun': constraint_eq assigns the constraint function.
We will see in the next block of code, it is where we constrain the possible solutions of the problem.
How to define an initial condition and result
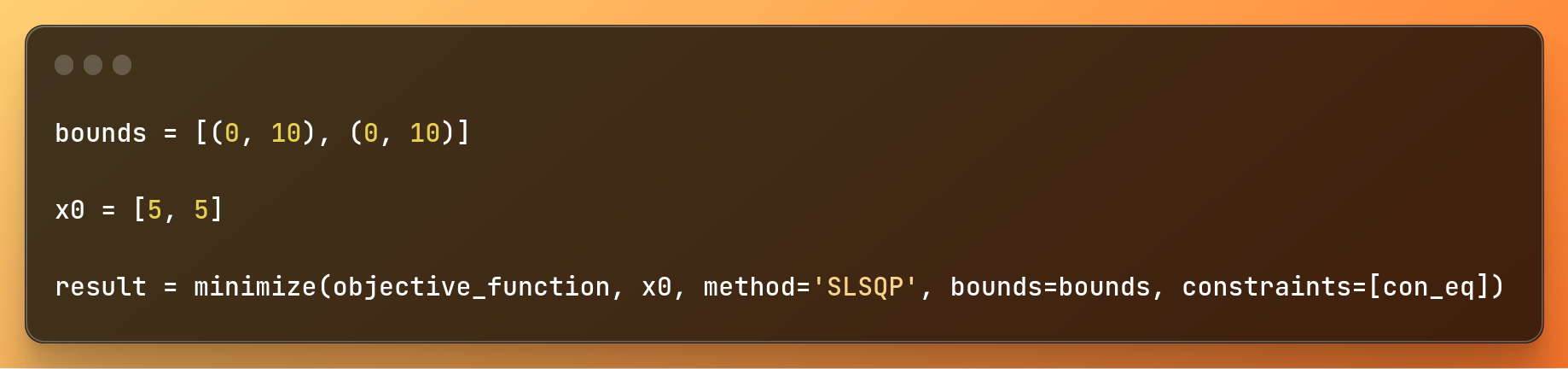
To understand this block of code, let's understand each parameter of result = minimize(objective_function, x0, method='SLSQP', bounds=bounds, constraints=[con_eq]) :
- objective_function : Is the function to be minimized.
- x0 : Is the initial guess for the variables.
- method='SLSQP' : This specifies the optimization algorithm we are using. In this case, we use SLSQP (Sequential Least SQuares Programming) .
- bounds=bounds : This parameter specifies the bounds for each of the decision variables.
- constraints=[con_eq] : This parameter tells us the constraints applied in the optimization problem.
This is how many real life problems are solved
Many things in real life are modeled with partial differential equation.
Then, with optimization methods developed with numerical analysis, they are optimized.
I am writing this because I know math can be boring for some people, and they may not be aware of where it is applied to solve real problems. The Calculus they learn can be applied in non-ideal situations outside the exams exercises.
Here, we can see finally see why math is important in two scenarios:
- To model systems to get solutions from it
- To optimize a certain system
Numerical analysis is one of the most important areas of applied math in STEM.
From solving PDE to optimize problems, numerical analysis is everywhere.
With more complex problems, numerical analysis is growing in importance to get faster algorithms that approximate pure math solutions.
This way, it is a bridge between theoretical mathematics and practical application.
If you want to, you can get the full code used in this article on GitHub .
3º year electrical and computer engineering student at Nova School of Science and Technology in Portugal | Vice Chair of IEEE NOVA SB | IEEE NOVA SB represents IEEE | https://www.ieee.org/
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
SymPy: Solving Math Equations in Python and Jupyter
Sympy alternatives , sympy vs. scipy , sympy vs. sagemath , using sympy and jupyter: a quick start tutorial .
There’s a lot more to SymPy than what we’ll cover here. This tutorial will focus on the mathematics I’m most familiar with, i.e., nothing beyond first-year university math. Those of you who are more advanced – and there are lots of folks who are – will want to spend some time digging into some resources I’ll point out as I go along.
Installing SymPy
Sympy first steps .
This explicit approach is advantageous if you need to embed SymPy in another application since you can control what parts you need. However, if you’re using SymPy interactively, it’s nice to have it preconfigure a few “smart defaults.” You can set up these defaults easily, as shown here:
The excellent SymPy documentation can give you more information about these, but since we’re in a Jupyter notebook, you can also view the docstring for any function by creating a new notebook cell and running function_name?
Solving Equations and Working With Math Functions
Now that we have a function defined, we can do several things with it. Let’s enter the variable name in a new cell. We see the function body displayed as nicely formatted LaTeX (in JupyterLab, SymPy uses MathJax to render the output this way).
That solution looks pretty good on the face of it, but we can check it by factoring the expression using factor :
SymPy as a Python Graphing Calculator
Sympy and latex .
I mentioned how much I like the default formatting you get when you use SymPy in Jupyter Lab. (Incidentally, it also works in Jupyter Notebook, too).
You may want to view the raw LaTeX output from time to time, as I did earlier when I prepared the screenshot for our “difference of squares” function. To do that, call either latex or print_latex .
SymPy and LaTeX: A Caveat
Incidentally, if you want to get more insight into how SymPy is managing the result, you can use the srepr function to see how the function is represented internally. This is a great tool for getting familiar with SymPy internals, but it can sometimes be a bit hard to follow. Therefore, I recommend trying it out on some simple functions first. For example, here we revisit our friend, the difference of squares function:
Python Calculus Made (Almost) Easy
Differentiation and integration , closing thoughts , companion source .
Mathematics Institute
Ma3k7 problem solving with python.
Lecturer: Siri Chongchitnan and Mark Cummings
Term(s): Term 2
Status for Mathematics students: Mathematics BSc (G100) List A, MMath (G103) List B
Note: There is a cap of 80 students due to the way this module is taught. 3rd year Maths students (G100) have priority. There is already no room for 4th year MMath students or students outside of the Maths department (including joint degree students) so we are sorry that they will not be able to register for this module. 3rd year MMath students may also need to deregister.
Commitment: 30 lectures
Assessment: 100% Coursework (50% from 5 problem sets, 50% from project)
Formal registration prerequisites: None
Assumed knowledge:
- Basic Python knowledge from MA124 Maths by Computer is assumed
Aims : We will examine the process of mathematical problem solving, with the use of Python as an exploratory tool. Problem-solving skills will be developed through a system of rubrics, which allow each problem to be approached systematically in distinct phases. Python will used not just as a tool to perform elaborate calculations, but also for visualisation and simulation, hence allowing each problem to be explored more freely, deeply and efficiently than the pen-and-paper approach. .
You will work both individually and in groups on mathematical problems that are challenging, unfamiliar and often open-ended. The problem-solving, critical-thinking and programming skills learnt in this course are highly desirable and transferrable, whether you go on to further study or into the job market.
Content : Mathematicians are used to solving problems, but few have really thought about what the problem-solving process really involves. The great mathematician George Pòlya was one of the pioneers who regarded problem solving as a subject worthy of studying on its own. He subsequently developed what he called heuristics (i.e. a recipe) for problem solving, and this was further refined by mathematicians over the past decades. In this module, we will not only explore these problem-solving strategies in detail, but we will also learn how even a basic knowledge of Python can be used to greatly enhance these strategies and make problem solving an enjoyable and mathematically enriching experience.
Indicative syllabus:
- The phases of problem-solving according to Pólya, Mason and others - Using Python to enhance the problem-solving phases - Writing a problem-solving rubric - Making conjectures and dealing with being stuck - Justifying and convincing - Asking question and extending a given mathematical problem
Objectives :
- Use an explicit scheme to organise your approach to solving mathematical problems.
- Use Python for calculations and visualisation of problems, aiding and enriching your solution.
- Critically evaluate your own problem-solving practice
- Mason, Burton and Stacey, Thinking Mathematically , 2nd ed., Pearson (2010)
- Linge and Langtangen, Programming for Computations - Python, 2nd ed ., Springer (2019)
- Pólya, How To Solve It , 2nd ed., (1990)
- Grieser, Exploring Mathematics: Problem-Solving and Proof , Springer (2018)
- Chongchitnan, Exploring University Mathematics with Python, Springer (2023)
Additional Resources
Year 1 regs and modules G100 G103 GL11 G1NC
Year 2 regs and modules G100 G103 GL11 G1NC
Year 3 regs and modules G100 G103
Year 4 regs and modules G103
Exam information Core module averages

Hands-On Mathematical Optimization with Python
Hands-on mathematical optimization with python #.
Welcome to this repository of companion notebooks for the book Hands-On Mathematical Optimization with Python , which will be published by Cambridge University press in October 2024.
This book introduces the concepts and tools of mathematical optimization with examples from a range of disciplines. The goals of these companion notebooks are to:
Provide a foundation for hands-on learning of mathematical optimization,
Demonstrate the tools and concepts of optimization with practical examples,
Help readers to develop the practical skills needed to build models and solving problem using state-of-the-art modeling languages and solvers.
Getting started #
The notebooks in this repository make extensive use of Pyomo which is a complete and versatile mathematical optimization package for the Python ecosystem. Pyomo provides a means to build models for optimization using the concepts of decision variables, constraints, and objectives from mathematical optimization, then transform and generate solutions using open source or commercial solvers.
All notebooks in this repository can be opened and run in Google Colab. A launch icon appearing at the top of a page (look for the rocket) indicates the notebook can be opened as an executable document. Selecting Colab will reopen the notebook in Google Colab. Cells inside the notebooks will perform any necessary installations of Pyomo and solvers needed to execute the code within the notebook.
Start your journey with the first chapter !
We seek your feedback! If you encounter an issue or have suggestions on how to make these examples better, please open an issue using the link at the top of every page (look for the Github cat icon).
We are a group of researchers and educators who came together with a common purpose of developing materials for use in our classroom teaching. Hopefully, these materials will find use in other classrooms and, most importantly, by those seeking entry into the world of building optimization models for data-rich applications.
Krzysztof Postek, Boston Consulting Group (formerly TU Delft)
Alessandro Zocca, VU Amsterdam
Joaquim Gromicho, ORTEC and the University of Amsterdam
Jeffrey Kantor, University of Notre Dame
If you wish to cite this work, please use
Problem Solving with Python
If you like this book, please consider purchasing a hard copy version on amazon.com .
- You will find the book chapters on the left hand menu
- You will find navigation within a section of a chapter (one webpage) on the righthand menu
- Sources for this text are stored on GitHub at github.com/professorkazarinoff/Problem-Solving-with-Python-37-Edition
If you find the text useful, please consider supporting the work by purchasing a hard copy of the text .
This work is licensed under a GNU General Public License v3.0
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
A Handbook of Mathematical Models with Python, published by Packt
PacktPublishing/A-Handbook-of-Mathematical-Models-with-Python
Folders and files.
Name | Name | |||
---|---|---|---|---|
77 Commits | ||||
Repository files navigation
Packt conference : put generative ai to work on oct 11-13 (virtual).
Code: USD75OFF
A Handbook of Mathematical Models with Python
This is the code repository for A Handbook of Mathematical Models with Python , published by Packt.
Elevate your machine learning projects with NetworkX, PuLP, and linalg
What is this book about?
Mathematical modeling is the art of transforming a business problem into a well-defined mathematical formulation. Its emphasis on interpretability is particularly crucial when deploying a model to support high-stake decisions in sensitive sectors like pharmaceuticals and healthcare.
This book covers the following exciting features:
- Understand core concepts of mathematical models and their relevance in solving problems
- Explore various approaches to modeling and learning using Python
- Work with tested mathematical tools to gather meaningful insights
- Blend mathematical modeling with machine learning to find optimal solutions to business problems
- Optimize ML models built with business data, apply them to understand their impact on the business, and address critical questions
- Apply mathematical optimization for data-scarce problems where the objective and constraints are known
If you feel this book is for you, get your copy today!

Instructions and Navigations
All of the code is organized into folders.
The code will look like the following:
Following is what you need for this book: If you are a budding data scientist seeking to augment your journey with mathematics, this book is for you. Researchers and R&D scientists will also be able to harness the concepts covered to their full potential. To make the best use of this book, a background in linear algebra, differential equations, basics of statistics, data types, data structures, and numerical algorithms will be useful.
With the following software and hardware list you can run all code files present in the book (Chapters 3-9).
Software and Hardware List
You will need Python 3.0 or higher versions to run the code in respective chapters. Python libraries required to execute a particular method have been imported (compatible versions with Python 3.0) in the code, which can be installed readily in the notebook or Python environment of your system.
System requirements are mentioned in the following table:
Software/Hardware | Operating System requirements |
---|---|
Python 3.0 or higher | Windows, Mac OS X, and Linux (Any) |
Python libraries | Windows, Mac OS X, and Linux (Any) |
Related products
Machine Learning Security Principles [Packt] [Amazon]
Machine Learning Model Serving Patterns and Best Practices [Packt] [Amazon]
Get to Know the Author
Dr. Ranja Sarkar is a delivery manager (data science consulting) at MathCo. She is a scientist with a focus on the intersection of data science and technology, working on a variety of projects in sectors ranging from manufacturing to healthcare to retail. Prior to this, Ranja worked as a data scientist at Shell plc, the most diversified global group of energy and petrochemical companies. Ranja has a decade of experience in academic scientific research encompassing physics, biophysics, statistical physics, and mathematics with publications in peer-reviewed internationally acclaimed journals. She has also worked at Deloitte - USI (Offices of the US) as a consultant for a year.
Additional Information
This book has been divided into three parts.
Part I - Mathematical Modeling: It has 2 chapters and introduces the theory and concepts of mathematical modeling and discusses how a business problem can be formulated mathematically.
Part II - Mathematical Tools: It consists of 6 chapters (all have python code examples) about the most tried and tested tools that enable mathematical modeling and how mathematical models are complementary to machine learning models.
Part III - Mathematical Optimization: It has 2 chapters (1 has python code examples) introduces the readers to optimization techniques in general, those used in machine learning and in operations research.
Contributors 4
- Python 100.0%
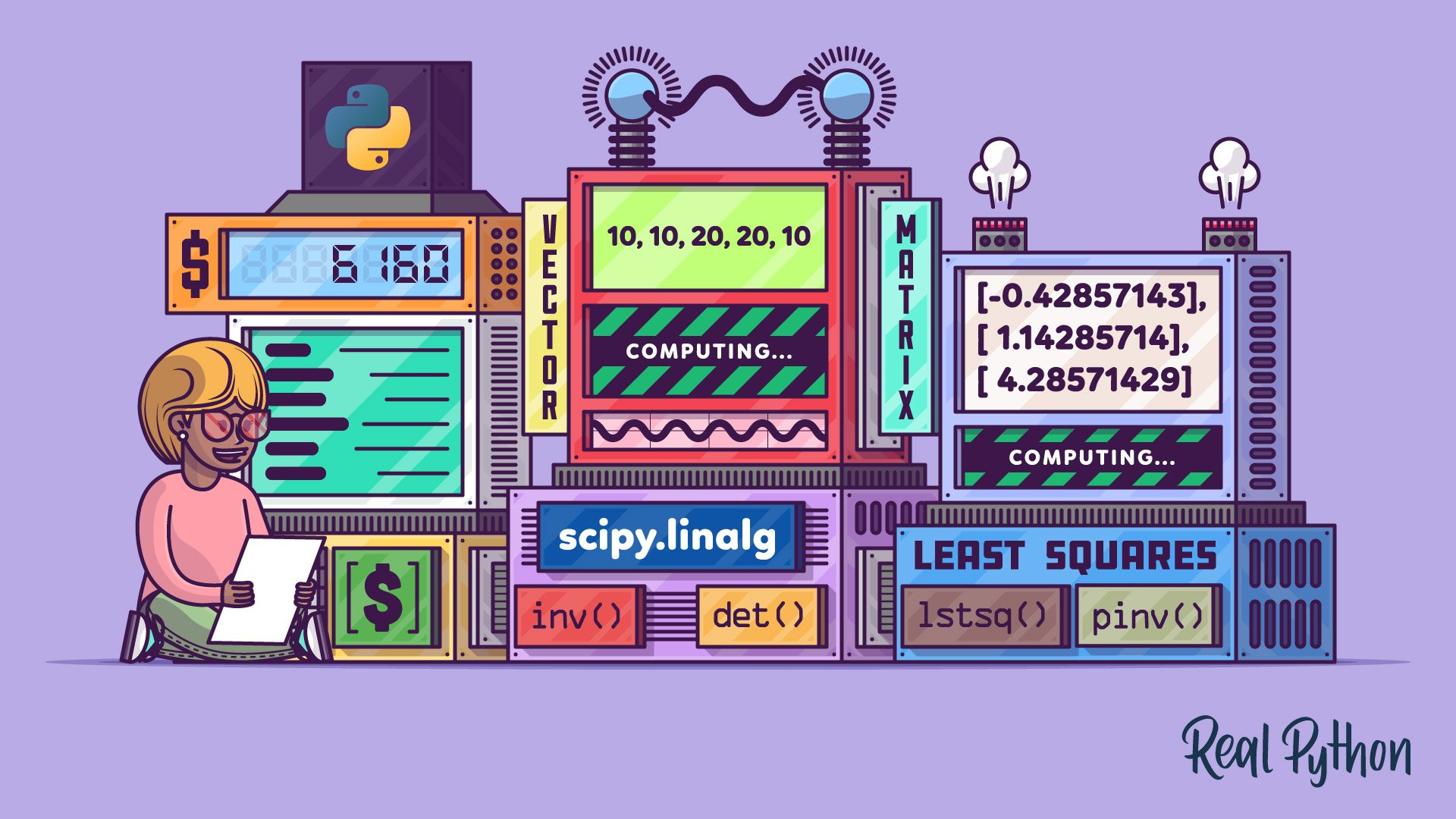
Linear Algebra in Python: Matrix Inverses and Least Squares
Table of Contents
Getting Started With Linear Algebra in Python
Understanding vectors, matrices, and the role of linear algebra, using determinants to study linear systems, using matrix inverses to solve linear systems, calculating inverses and determinants with scipy.linalg, interpolating polynomials with linear systems, building least squares models using scipy.linalg, obtaining least squares solutions using a pseudoinverse, preparing the data, building the model, predicting prices.
Linear algebra is an important topic across a variety of subjects. It allows you to solve problems related to vectors , matrices , and linear equations . In Python, most of the routines related to this subject are implemented in scipy.linalg , which offers very fast linear algebra capabilities.
In particular, linear models play an important role in a variety of real-world problems, and scipy.linalg provides tools to compute them in an efficient way.
In this tutorial, you’ll learn how to:
- Study linear systems using determinants and solve problems using matrix inverses
- Interpolate polynomials to fit a set of points using linear systems
- Use Python to solve linear regression problems
- Use linear regression to predict prices based on historical data
This is the second part of a series of tutorials on linear algebra using scipy.linalg . So, before continuing, make sure to take a look at the first tutorial of the series before reading this one.
Free Source Code: Click here to download the free code and dataset that you’ll use to work with linear systems and algebra in Python with scipy.linalg .
Now you’re ready to get started!
Linear algebra is a branch of mathematics that deals with linear equations and their representations using vectors and matrices . It’s a fundamental subject in several areas of engineering, and it’s a prerequisite to a deeper understanding of machine learning .
To work with linear algebra in Python, you can count on SciPy , which is an open-source Python library used for scientific computing, including several modules for common tasks in science and engineering.
Of course, SciPy includes modules for linear algebra , but that’s not all. It also offers optimization , integration , interpolation , and signal processing capabilities. It’s part of the SciPy stack , which includes several other packages for scientific computing, such as NumPy , Matplotlib , SymPy , IPython , and pandas .
scipy.linalg includes several tools for working with linear algebra problems, including functions for performing matrix calculations, such as determinants , inverses , eigenvalues, eigenvectors , and the singular value decomposition .
In the previous tutorial of this series , you learned how to work with matrices and vectors in Python to model practical problems using linear systems. You solved these problems using scipy.linalg .
In this tutorial, you’re going a step further, using scipy.linalg to study linear systems and build linear models for real-world problems.
In order to use scipy.linalg , you have to install and set up the SciPy library. Besides that, you’re going to use Jupyter Notebook to run the code in an interactive environment. SciPy and Jupyter Notebook are third-party packages that you need to install. For installation, you can use the conda or pip package manager. Revisit Working With Linear Systems in Python With scipy.linalg for installation details.
Note: Using Jupyter Notebook to run the code isn’t mandatory, but it facilitates working with numerical and scientific applications.
For a refresher on working with Jupyter Notebooks, take a look at Jupyter Notebook: An Introduction .
Next, you’ll go through some fundamental concepts of linear algebra and explore how to use Python to work with these concepts.
A vector is a mathematical entity used to represent physical quantities that have both magnitude and direction. It’s a fundamental tool for solving engineering and machine learning problems. So are matrices , which are used to represent vector transformations, among other applications.
Note: In Python, NumPy is the most used library for working with matrices and vectors. It uses a special type called ndarray to represent them. As an example, imagine that you need to create the following matrix:

With NumPy, you can use np.array() to create it, providing a nested list containing the elements of each row of the matrix:
NumPy provides several functions to facilitate working with vector and matrix computations. You can find more information on how to use NumPy to represent vectors and matrices and perform operations with them in the previous tutorial in this series .
A linear system or, more precisely, a system of linear equations, is a set of equations linearly relating to a set of variables. Here’s an example of a linear system relating to the variables x ₁ and x ₂:

Here you have two equations involving two variables. In order to have a linear system, the values that multiply the variables x ₁ and x ₂ must be constants, like the ones in this example. It’s common to write linear systems using matrices and vectors. For example, you can write the previous system as the following matrix product :

Comparing the matrix product form with the original system, you can notice the elements of matrix A correspond to the coefficients that multiply x ₁ and x ₂. Besides that, the values in the right-hand side of the original equations now make up vector b .
Linear algebra is a mathematical discipline that deals with vectors, matrices, and vector spaces and linear transformations more generally. By using linear algebra concepts, it’s possible to build algorithms to perform computations for several applications, including solving linear systems.
When there are just two or three equations and variables, it’s feasible to perform the calculations manually , combine the equations, and find the values for the variables.
However, in real-world applications, the number of equations can be very large, making it infeasible to do calculations manually. That’s precisely when linear algebra concepts and algorithms come handy, allowing you to develop usable applications for engineering and machine learning , for example.
In Working With Linear Systems in Python With scipy.linalg , you’ve seen how to solve linear systems using scipy.linalg.solve() . Now you’re going to learn how to use determinants to study the possible solutions and how to solve problems using the concept of matrix inverses.
Solving Problems Using Matrix Inverses and Determinants
Matrix inverses and determinants are tools that allow you to get some information about the linear system and also to solve it. Before going through the details on how to calculate matrix inverses and determinants using scipy.linalg , take some time to remember how to use these structures.
As you may recall from your math classes, not every linear system can be solved. You may have a combination of equations that’s inconsistent and has no solution. For example, a system with two equations given by x ₁ + x ₂ = 2 and x ₁ + x ₂ = 3 is inconsistent and has no solution. This happens because no two numbers x ₁ and x ₂ can add up to both 2 and 3 at the same time.
Besides that, some systems can be solved but have more than one solution. For example, if you have a system with two equivalent equations, such as x ₁ + x ₂ = 2 and 2 x ₁ + 2 x ₂ = 4, then you can find an infinite number of solutions, such as ( x ₁=1, x ₂=1), ( x ₁=0, x ₂=2), ( x ₁=2, x ₂=0), and so on.
A determinant is a number, calculated using the matrix of coefficients , that tells you if there’s a solution for the system. Because you’ll be using scipy.linalg to calculate it, you don’t need to care much about the details on how to make the calculation. However, keep the following in mind:
- If the determinant of a coefficients matrix of a linear system is different from zero , then you can say the system has a unique solution .
- If the determinant of a coefficients matrix of a linear system is equal to zero , then the system may have either zero solutions or an infinite number of solutions .
Now that you have this in mind, you’ll learn how to solve linear systems using matrices.
To understand the idea behind the inverse of a matrix, start by recalling the concept of the multiplicative inverse of a number. When you multiply a number by its inverse, you get 1 as the result. Take 3 as an example. The inverse of 3 is 1/3, and when you multiply these numbers, you get 3 × 1/3 = 1.
With square matrices, you can think of a similar idea. However, instead of 1, you’ll get an identity matrix as the result. An identity matrix has ones in its diagonal and zeros in the elements outside of the diagonal, like the following examples:

The identity matrix has an interesting property: when multiplied by another matrix A of the same dimensions, the obtained result is A . Recall that this is also true for the number 1, when you consider the multiplication of numbers.
This allows you to solve a linear system by following the same steps used to solve an equation. As an example, consider the following linear system, written as a matrix product:
By calling A ⁻¹ the inverse of matrix A , you could multiply both sides of the equation by A ⁻¹, which would give you the following result:

This way, by using the inverse, A ⁻¹, you can obtain the solution x for the system by calculating A ⁻¹ b .
It’s worth noting that while non-zero numbers always have an inverse, not all matrices have an inverse. When the system has no solution or when it has multiple solutions, the determinant of A will be zero, and the inverse, A ⁻¹, won’t exist.
Now you’ll see how to use Python with scipy.linalg to make these calculations.
You can calculate matrix inverses and determinants using scipy.linalg.inv() and scipy.linalg.det() .
For example, consider the meal plan problem that you worked on in the previous tutorial of this series . Recall that the linear system for this problem could be written as a matrix product:
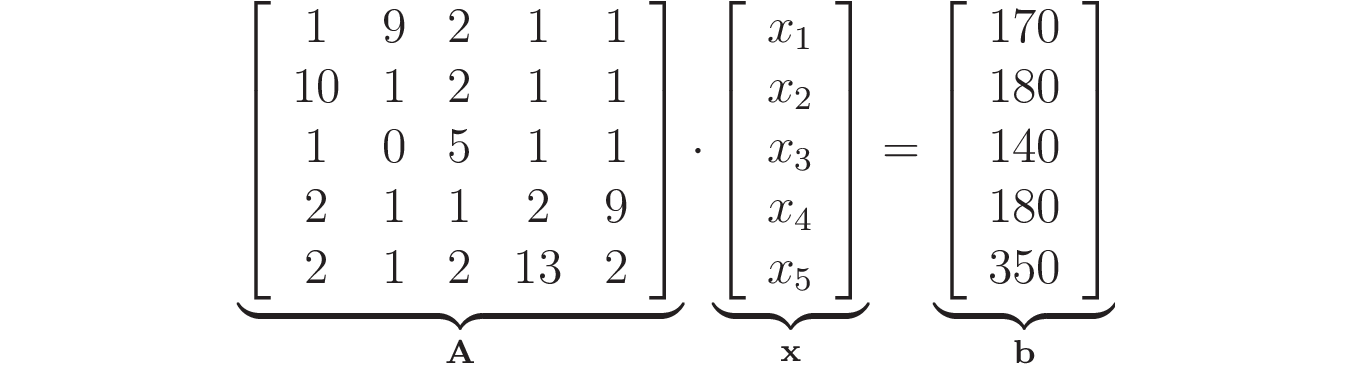
Previously, you used scipy.linalg.solve() to obtain the solution 10 , 10 , 20 , 20 , 10 for the variables x ₁ to x ₅, respectively. But as you’ve just learned, it’s also possible to use the inverse of the coefficients matrix to obtain vector x , which contains the solutions for the problem. You have to calculate x = A ⁻¹ b , which you can do with the following program:
Here’s a breakdown of what’s happening:
Lines 1 and 2 import NumPy as np , along with linalg from scipy . These imports allow you to use linalg.inv() .
Lines 4 to 12 create the coefficients matrix as a NumPy array called A .
Line 14 creates the independent terms vector as a NumPy array called b . To make it a column vector with five elements, you use .reshape((5, 1)) .
Line 16 uses linalg.inv() to obtain the inverse of matrix A .
Lines 18 and 19 use the @ operator to perform the matrix product in order to solve the linear system characterized by A and b . You store the result in x , which is printed.
You get exactly the same solution as the one provided by scipy.linalg.solve() . Because this system has a unique solution, the determinant of matrix A must be different from zero. You can confirm that it is by calculating it using det() from scipy.linalg :
As expected, the determinant isn’t zero. This indicates that the inverse of A , denoted as A ⁻¹ and calculated with inv(A) , exists, so the system has a unique solution. A ⁻¹ is a square matrix with the same dimensions as A , so the product of A ⁻¹ and A results in an identity matrix. In this example, it’s given by the following:
Now that you know the basics of using matrix inverses and determinants, you’ll see how to use these tools to find the coefficients of polynomials.
You can use linear systems to calculate polynomial coefficients so that these polynomials include some specific points.
For example, consider the second-degree polynomial y = P ( x ) = a ₀ + a ₁ x + a ₂ x ². Recall that when you plot a second-degree polynomial, you get a parabola, which will be different depending on the coefficients a ₀, a ₁, and a ₂.
Now, suppose that you’d like to find a specific second-degree polynomial that includes the ( x , y ) points (1, 5), (2, 13), and (3, 25). How could you calculate a ₀, a ₁, and a ₂, such that P ( x ) includes these points in its parabola? In other words, you want to find the coefficients of the polynomial in this figure:
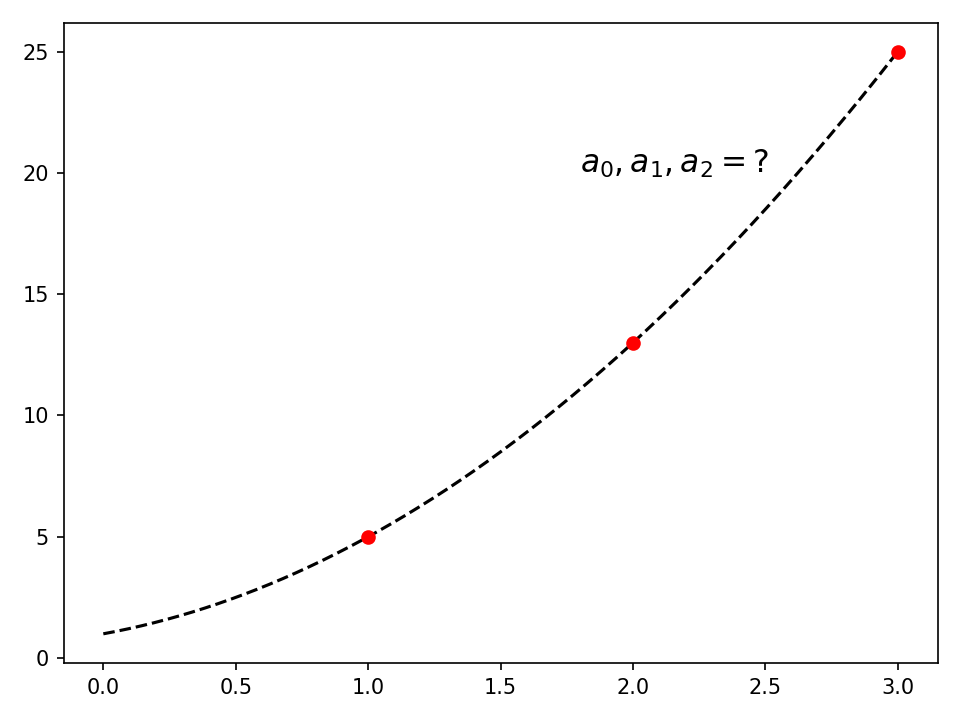
For each point that you’d like to include in the parabola, you can use the general expression of the polynomial in order to get a linear equation. For example, taking the second point, ( x =2, y =13), and considering that y = a ₀ + a ₁ x + a ₂ x ², you could write the following equation:

This way, for each point ( x , y ), you’ll get an equation involving a ₀, a ₁, and a ₂. Because you’re considering three different points, you’ll end up with a system of three equations:

To check if this system has a unique solution, you can calculate the determinant of the coefficients matrix and check if it’s not zero. You can do that with the following code:
It’s worth noting that the existence of the solution only depends on A . Because the value of the determinant isn’t zero, you can be sure that there’s a unique solution for the system. You can solve it using the matrix inverse method with the following code:
This result tells you that a ₀ = 1, a ₁ = 2, and a ₂ = 2 is a solution for the system. In other words, the polynomial that includes the points (1, 5), (2, 13), and (3, 25) is given by y = P ( x ) = 1 + 2 x + 2 x ². You can test the solution for each point by inputting x and verifying that P ( x ) is equal to y .
As an example of a system without any solution, say that you’re trying to interpolate a parabola with the ( x , y ) points given by (1, 5), (2, 13), and (2, 25). If you look carefully at these numbers, you’ll notice that the second and third points consider x = 2 and different values for y , which makes it impossible to find a function that includes both points.
Following the same steps as before, you’ll arrive at the equations for this system, which are the following:

To confirm that this system doesn’t present a unique solution, you can calculate the determinant of the coefficients matrix with the following code:
You may notice that the value of the determinant is zero, which means that the system doesn’t have a unique solution. This also means that the inverse of the coefficients matrix doesn’t exist. In other words, the coefficients matrix is singular .
Depending on your computer architecture, you may get a very small number instead of zero. This happens due to the numerical algorithms that det() uses to calculate the determinant. In these algorithms, numeric precision errors make this result not exactly equal to zero.
In general, whenever you come across a tiny number, you can conclude that the system doesn’t have a unique solution.
You can try to solve the linear system using the matrix inverse method with the following code:
Because the system has no solution, you get an exception telling you that the coefficients matrix is singular.
When the system has more than one solution, you’ll come across a similar result. The value of the determinant of the coefficients matrix will be zero or very small, indicating that the coefficients matrix again is singular.
As an example of a system with more than one solution, you can try to interpolate a parabola considering the points ( x , y ) given by (1, 5), (2, 13), and (2, 13). As you may notice, here you’re considering two points at the same position, which allows an infinite number of solutions for a ₀, a ₁, and a ₂.
Now that you’ve gone through how to work with polynomial interpolation using linear systems, you’ll see another technique that makes an effort to find the coefficients for any set of points.
Minimizing Error With Least Squares
You’ve seen that sometimes you can’t find a polynomial that fits precisely to a set of points. However, usually when you’re trying to interpolate a polynomial, you’re not interested in a precise fit. You’re just looking for a solution that approximates the points, providing the minimum error possible.
This is generally the case when you’re working with real-world data. Usually, it includes some noise caused by errors that occur in the collecting process, like imprecision or malfunction in sensors, and typos when users are inputting data manually.
Using the least squares method, you can find a solution for the interpolation of a polynomial, even when the coefficients matrix is singular. By using this method, you’ll be looking for the coefficients of the polynomial that provides the minimum squared error when comparing the polynomial curve to your data points.
Actually, the least squares method is generally used to fit polynomials to large sets of data points. The idea is to try to design a model that represents some observed behavior.
Note: If a linear system has a unique solution, then the least squares solution will be equal to that unique solution.
For example, you could design a model to try to predict car prices. For that, you could collect some real-world data, including the car price and some other features like the mileage, the year, and the type of car. With this data, you can design a polynomial that models the price as a function of the other features and use least squares to find the optimal coefficients of this model.
Soon, you’re going to work on a model to address this problem. But first, you’re going to see how to use scipy.linalg to build models using least squares.
To solve least squares problems, scipy.linalg provides a function called lstsq() . To see how it works, consider the previous example, in which you tried to fit a parabola to the points ( x , y ) given by (1, 5), (2, 13), and (2, 25). Remember that this system has no solution, since there are two points with the same value for x .
Just like you did before, using the model y = a ₀ + a ₁ x + a ₂ x ², you arrive at the following linear system:
Using the least squares method, you can find a solution for the coefficients a ₀, a ₁, and a ₂ that provides a parabola that minimizes the squared difference between the curve and the data points. For that, you can use the following code:
In this program, you’ve set up the following:
Lines 1 to 2: You import numpy as np and linalg from scipy in order to use linalg.lstsq() .
Lines 4 to 5: You create the coefficients matrix A using a NumPy array called A and the vector with the independent terms b using a NumPy array called b .
Line 7: You calculate the least squares solution for the problem using linalg.lstsq() , which takes the coefficients matrix and the vector with the independent terms as input.
lstsq() provides several pieces of information about the system, including the residues, rank, and singular values of the coefficients matrix. In this case, you’re interested only in the coefficients of the polynomial to solve the problem according to the least squares criteria, which are stored in p .
As you can see, even considering a linear system that has no exact solution, lstsq() provides the coefficients that minimize the squared errors. With the following code, you can visualize the solution provided by plotting the parabola and the data points:
This program uses matplotlib to plot the results:
Line 1: You import matplotlib.pyplot as plt , which is typical.
Lines 3 to 4: You create a NumPy array named x , with values ranging from 0 to 3 , containing 1000 points. You also create a NumPy array named y with the corresponding values of the model.
Line 6: You plot the curve for the parabola obtained with the model given by the points in the arrays x and y .
Lines 7 to 9: In red ( "ro" ), you plot the three points used to build the model.
The output should be the following figure:
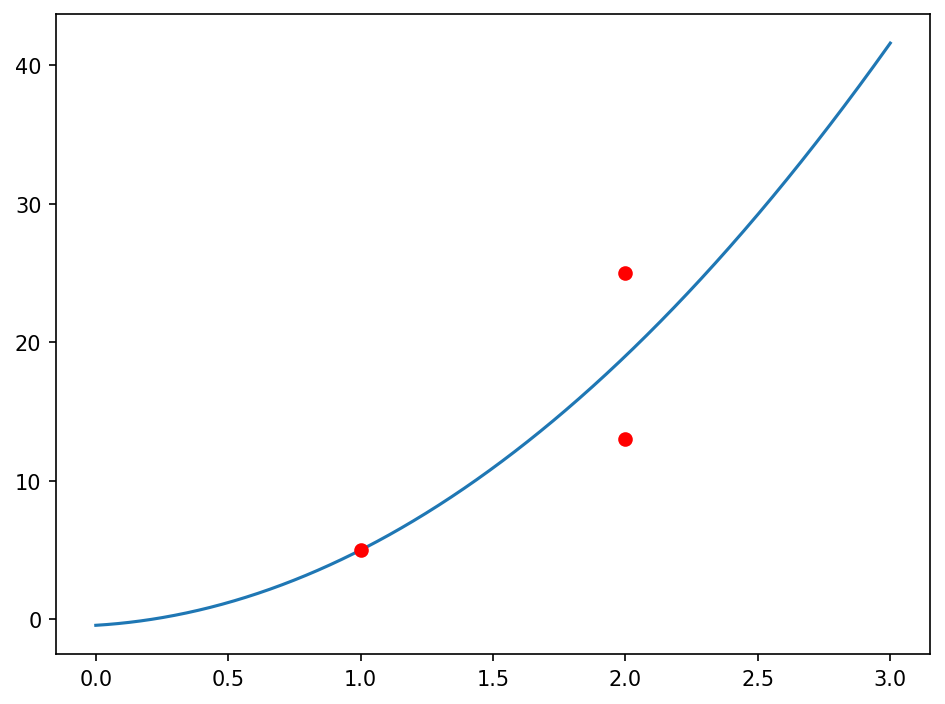
Notice how the curve provided by the model tries to approximate the points as well as possible.
Besides lstsq() , there are other ways to calculate least squares solutions using SciPy. One of the alternatives is using a pseudoinverse , which you’ll explore next.
Another way to compute the least squares solution is by using the Moore-Penrose pseudoinverse of a matrix.
You can think of a pseudoinverse as a generalization of the matrix inverse, as it’s equal to the usual matrix inverse when the matrix isn’t singular.
However, when the matrix is singular, which is the case in linear systems that lack a unique solution, then the pseudoinverse computes the matrix that provides the best fit, leading to the least squares solution.
Using the pseudoinverse, you can find the coefficients for the parabola used in the previous example:
This code is very similar to the code from the previous section, except for the highlighted lines:
Line 7: You calculate the pseudoinverse of the coefficients matrix and store it in A_pinv .
Line 9: Following the same approach used to solve linear systems with the inverse of a matrix, you calculate the coefficients of the parabola equation using the pseudoinverse and store them in the vector p2 .
As you’d expect, the least squares solution is the same as the lstsq() solution. In this case, because A is a square matrix, pinv() will provide a square matrix with the same dimensions as A , optimizing for the best fit in the least squares sense:
However, it’s worth noting that you can also calculate pinv() for non-square matrices, which is usually the case in practice. You’ll dive into that next, with an example using real-world data.
Example: Predicting Car Prices With Least Squares
In this example, you’re going to build a model using least squares to predict the price of used cars using the data from the Used Cars Dataset . This dataset is a huge collection with 957 MB of vehicle listings from craigslist.org, including very different types of vehicles.
When working with real data, it’s often necessary to perform some steps of filtering and cleaning in order to use the data to build a model. In this case, it’s necessary to narrow down the types of vehicles that you’ll include, in order to get better results with your model.
Since your main focus here is on using least squares to build the model, you’ll start with a cleaned dataset, which is a small subset from the original one. Before you start working on the code, get the cleaned data CSV file by clicking the link below and navigating to vehicles_cleaned.csv :
In the downloadable materials, you can also check out the Jupyter Notebook to learn more about data preparation.
To load the CSV file and process the data, you’ll use pandas . So, make sure to install it in the conda environment linalg as follows:
After downloading the data and setting up pandas, you can start a new Jupyter Notebook and load the data by running the following code block:
This will create a pandas DataFrame named cars_data containing the data from the CSV file. From this DataFrame, you’ll generate the NumPy arrays that you’ll use as inputs to lstsq() and pinv() to obtain the least squares solution. To learn more on how to use pandas to process data, take a look at Using pandas and Python to Explore Your Dataset .
A DataFrame object includes an attribute named columns that allows you to consult the names of the columns included in the data. That means you can check the columns included in this dataset with the following code:
You can take a look into one of the lines of the DataFrame using .iloc :
As you can see, this dataset includes nine columns, with the following data:
Column Name | Description |
---|---|
The price of the vehicle, which is the column that you want to predict with your model | |
The production year of the vehicle | |
A categorical variable that can take the values , , , , , or | |
A categorical variable that can take the values or | |
A categorical variable that can take the values or | |
The mileage of the vehicle indicated by the odometer | |
A categorical variable that can take the values or | |
A categorical value that can take the values , , , or | |
A categorical value that can take the values , , , or |
To use this data to build a least squares model, you’ll need to represent the categorical data in a numeric way. In most cases, categorical data is transformed to a set of dummy variables , which are variables that can take a value of 0 or 1 .
As an example of this transformation, consider the column fuel , which can take the value gas or diesel . You could transform this categorical column to a dummy column named fuel_gas that takes the value 1 when fuel is gas and 0 when fuel is diesel .
Note that you’ll need just one dummy column to represent a categorical column that can take two different values. Similarly, for a categorical column that can take N values, you’re going to need N-1 dummy columns, as one of the values will be assumed as the default.
In pandas, you can transform these categorical columns to dummy columns with get_dummies() :
Here, you’re creating a new DataFrame named cars_data_dummies , which includes dummy variables for the columns specified in the columns argument. You can now check the new columns included in this DataFrame:
Now that you’ve transformed the categorical variables to sets of dummy variables, you can use this information to build your model. Basically, the model will include a coefficient for each of these columns—except price , which will be used as the model output. The price will be given by a weighted combination of the other variables, where the weights are given by the model’s coefficients.
However, it’s customary to consider an extra coefficient that represents a constant value that’s added to the weighted combination of the other variables. This coefficient is known as the intercept , and you can include it in your model by adding an extra column to the data, with all the rows equal to 1 :
Now that you have all the data organized, you can generate the NumPy arrays to build your model using scipy.linalg . That’s what you’ll do next.
To generate the NumPy arrays to input in lstsq() or pinv() , you can use .to_numpy() :
The coefficients matrix A is given by all the columns, except price . Vector b , with the independent terms, is given by the values that you want to predict, which is the price column in this case. With A and b set, you can use lstsq() to find the least squares solution for the coefficients:
These are the coefficients that you should use to model price in terms of a weighted combination of the other variables in order to minimize the squared error. As you’ve seen, it’s also possible to get these coefficients by using pinv() with the following code:
One of the nice characteristics of a linear regression model is that it’s fairly easy to interpret. In this case, you can conclude from the coefficients that the value of the car increases approximately $847 as year increases by 1 , which means that the value of the car decreases $847 per year of car age. Similarly, according to the second coefficient, the value of the car decreases approximately $35.39 per 1,000 miles.
Now that you’ve obtained the model, you’ll use it to predict the price of a car.
Using the model given by the least squares solution, you can predict the price for a car represented by a vector with the values for each of the variables used in the model:
So, a 2010 4-cylinder hatchback, with automatic transmission, gas fuel, and 50,000 miles, in good condition, can be represented with the following vector:
You can obtain the prediction of the price by calculating the dot product between the car vector and the vector p of the coefficients. Because both vectors are one-dimensional NumPy arrays, you can use @ to obtain the dot product:
In this example, the predicted price for the hatchback is approximately $6,160. It’s worth noting that the model coefficients include some uncertainty because the data used to obtain the model could be biased toward a particular type of car, for example.
Besides that, the model choice plays a big role in the quality of the estimates. Least squares is one of the most-used techniques to build models because it’s simple and yields explainable models. In this example, you’ve seen how to use scipy.linalg to build such models. For more details on least squares models, take a look at Linear Regression in Python .
Congratulations! You’ve learned how to use some linear algebra concepts with Python to solve problems involving linear models. You’ve discovered that vectors and matrices are useful for representing data and that, by using linear systems, you can model practical problems and solve them in an efficient manner.
In this tutorial, you’ve learned how to:
Linear algebra is a very broad topic. For more information on some other linear algebra applications, check out the following resources:
- Working With Linear Systems in Python With scipy.linalg
- Scientific Python: Using SciPy for Optimization
- Hands-On Linear Programming: Optimization With Python
- NumPy, SciPy, and pandas: Correlation With Python
Keep studying, and feel free to leave any questions or comments below!
🐍 Python Tricks 💌
Get a short & sweet Python Trick delivered to your inbox every couple of days. No spam ever. Unsubscribe any time. Curated by the Real Python team.
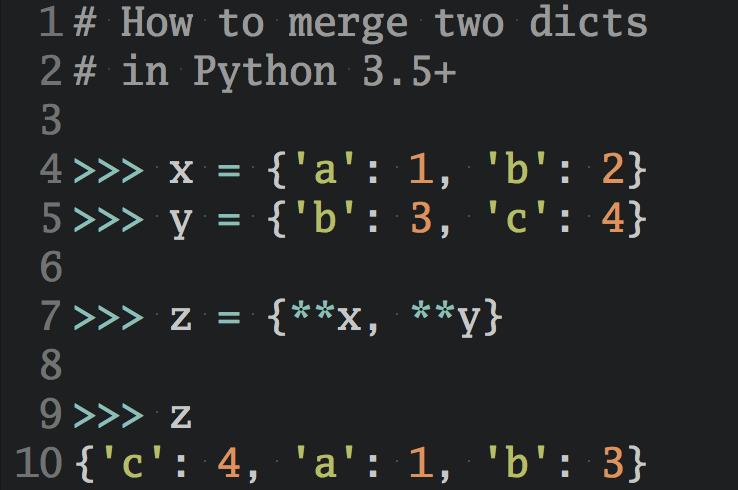
About Renato Candido
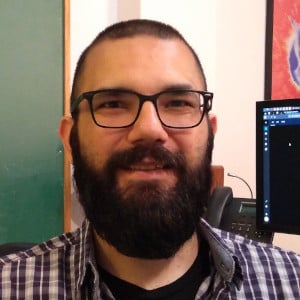
Researcher in digital signal processing, telecommunications and machine learning. {free, libre, open source} {software, hardware, culture, science} enthusiast.
Each tutorial at Real Python is created by a team of developers so that it meets our high quality standards. The team members who worked on this tutorial are:
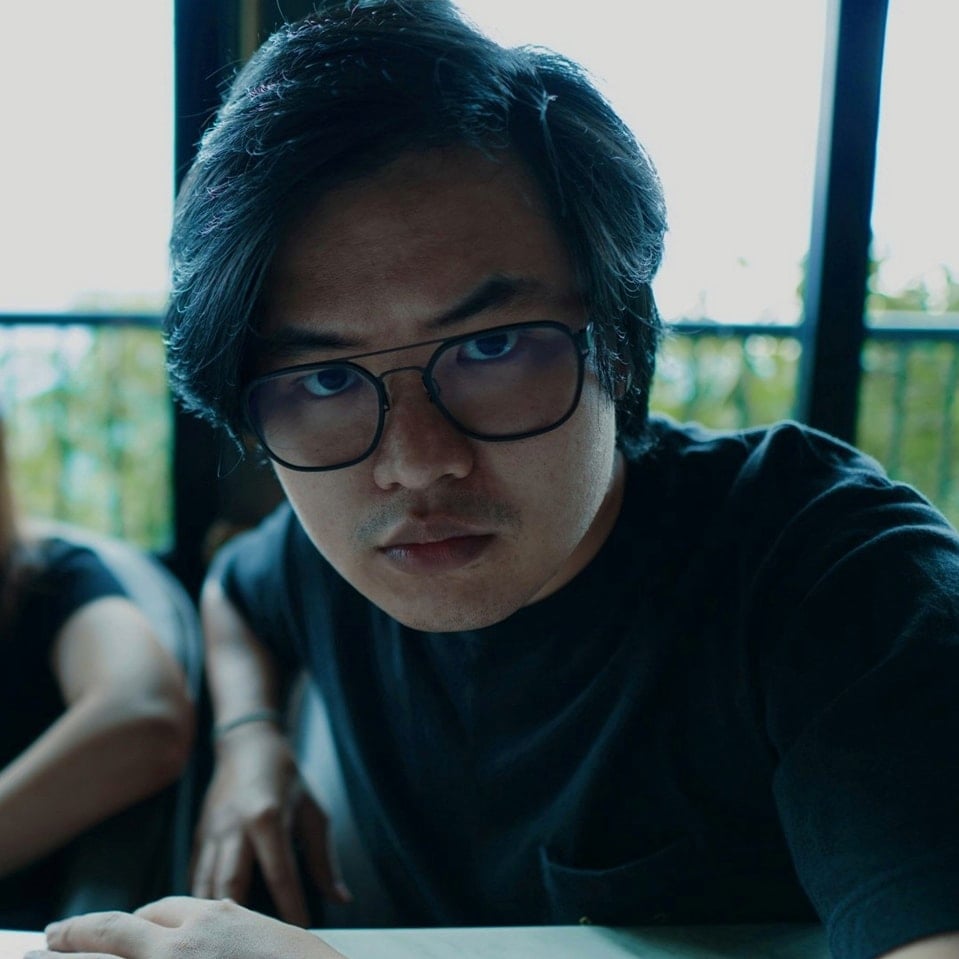
Master Real-World Python Skills With Unlimited Access to Real Python
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
What Do You Think?
What’s your #1 takeaway or favorite thing you learned? How are you going to put your newfound skills to use? Leave a comment below and let us know.
Commenting Tips: The most useful comments are those written with the goal of learning from or helping out other students. Get tips for asking good questions and get answers to common questions in our support portal . Looking for a real-time conversation? Visit the Real Python Community Chat or join the next “Office Hours” Live Q&A Session . Happy Pythoning!
Keep Learning
Related Topics: intermediate data-science numpy
Keep reading Real Python by creating a free account or signing in:
Already have an account? Sign-In
Almost there! Complete this form and click the button below to gain instant access:
Linear Systems and Algebra in Python (Source Code)
🔒 No spam. We take your privacy seriously.
Python Programming
Practice Python Exercises and Challenges with Solutions
Free Coding Exercises for Python Developers. Exercises cover Python Basics , Data structure , to Data analytics . As of now, this page contains 18 Exercises.
What included in these Python Exercises?
Each exercise contains specific Python topic questions you need to practice and solve. These free exercises are nothing but Python assignments for the practice where you need to solve different programs and challenges.
- All exercises are tested on Python 3.
- Each exercise has 10-20 Questions.
- The solution is provided for every question.
- Practice each Exercise in Online Code Editor
These Python programming exercises are suitable for all Python developers. If you are a beginner, you will have a better understanding of Python after solving these exercises. Below is the list of exercises.
Select the exercise you want to solve .
Basic Exercise for Beginners
Practice and Quickly learn Python’s necessary skills by solving simple questions and problems.
Topics : Variables, Operators, Loops, String, Numbers, List
Python Input and Output Exercise
Solve input and output operations in Python. Also, we practice file handling.
Topics : print() and input() , File I/O
Python Loop Exercise
This Python loop exercise aims to help developers to practice branching and Looping techniques in Python.
Topics : If-else statements, loop, and while loop.
Python Functions Exercise
Practice how to create a function, nested functions, and use the function arguments effectively in Python by solving different questions.
Topics : Functions arguments, built-in functions.

Python String Exercise
Solve Python String exercise to learn and practice String operations and manipulations.
Python Data Structure Exercise
Practice widely used Python types such as List, Set, Dictionary, and Tuple operations in Python
Python List Exercise
This Python list exercise aims to help Python developers to learn and practice list operations.
Python Dictionary Exercise
This Python dictionary exercise aims to help Python developers to learn and practice dictionary operations.
Python Set Exercise
This exercise aims to help Python developers to learn and practice set operations.
Python Tuple Exercise
This exercise aims to help Python developers to learn and practice tuple operations.
Python Date and Time Exercise
This exercise aims to help Python developers to learn and practice DateTime and timestamp questions and problems.
Topics : Date, time, DateTime, Calendar.
Python OOP Exercise
This Python Object-oriented programming (OOP) exercise aims to help Python developers to learn and practice OOP concepts.
Topics : Object, Classes, Inheritance
Python JSON Exercise
Practice and Learn JSON creation, manipulation, Encoding, Decoding, and parsing using Python
Python NumPy Exercise
Practice NumPy questions such as Array manipulations, numeric ranges, Slicing, indexing, Searching, Sorting, and splitting, and more.
Python Pandas Exercise
Practice Data Analysis using Python Pandas. Practice Data-frame, Data selection, group-by, Series, sorting, searching, and statistics.
Python Matplotlib Exercise
Practice Data visualization using Python Matplotlib. Line plot, Style properties, multi-line plot, scatter plot, bar chart, histogram, Pie chart, Subplot, stack plot.
Random Data Generation Exercise
Practice and Learn the various techniques to generate random data in Python.
Topics : random module, secrets module, UUID module
Python Database Exercise
Practice Python database programming skills by solving the questions step by step.
Use any of the MySQL, PostgreSQL, SQLite to solve the exercise
Exercises for Intermediate developers
The following practice questions are for intermediate Python developers.
If you have not solved the above exercises, please complete them to understand and practice each topic in detail. After that, you can solve the below questions quickly.
Exercise 1: Reverse each word of a string
Expected Output
- Use the split() method to split a string into a list of words.
- Reverse each word from a list
- finally, use the join() function to convert a list into a string
Steps to solve this question :
- Split the given string into a list of words using the split() method
- Use a list comprehension to create a new list by reversing each word from a list.
- Use the join() function to convert the new list into a string
- Display the resultant string
Exercise 2: Read text file into a variable and replace all newlines with space
Given : Assume you have a following text file (sample.txt).
Expected Output :
- First, read a text file.
- Next, use string replace() function to replace all newlines ( \n ) with space ( ' ' ).
Steps to solve this question : -
- First, open the file in a read mode
- Next, read all content from a file using the read() function and assign it to a variable.
- Display final string
Exercise 3: Remove items from a list while iterating
Description :
In this question, You need to remove items from a list while iterating but without creating a different copy of a list.
Remove numbers greater than 50
Expected Output : -
- Get the list's size
- Iterate list using while loop
- Check if the number is greater than 50
- If yes, delete the item using a del keyword
- Reduce the list size
Solution 1: Using while loop
Solution 2: Using for loop and range()
Exercise 4: Reverse Dictionary mapping
Exercise 5: display all duplicate items from a list.
- Use the counter() method of the collection module.
- Create a dictionary that will maintain the count of each item of a list. Next, Fetch all keys whose value is greater than 2
Solution 1 : - Using collections.Counter()
Solution 2 : -
Exercise 6: Filter dictionary to contain keys present in the given list
Exercise 7: print the following number pattern.
Refer to Print patterns in Python to solve this question.
- Use two for loops
- The outer loop is reverse for loop from 5 to 0
- Increment value of x by 1 in each iteration of an outer loop
- The inner loop will iterate from 0 to the value of i of the outer loop
- Print value of x in each iteration of an inner loop
- Print newline at the end of each outer loop
Exercise 8: Create an inner function
Question description : -
- Create an outer function that will accept two strings, x and y . ( x= 'Emma' and y = 'Kelly' .
- Create an inner function inside an outer function that will concatenate x and y.
- At last, an outer function will join the word 'developer' to it.
Exercise 9: Modify the element of a nested list inside the following list
Change the element 35 to 3500
Exercise 10: Access the nested key increment from the following dictionary
Under Exercises: -
Python Object-Oriented Programming (OOP) Exercise: Classes and Objects Exercises
Updated on: December 8, 2021 | 52 Comments
Python Date and Time Exercise with Solutions
Updated on: December 8, 2021 | 11 Comments
Python Dictionary Exercise with Solutions
Updated on: May 6, 2023 | 57 Comments
Python Tuple Exercise with Solutions
Updated on: December 8, 2021 | 96 Comments
Python Set Exercise with Solutions
Updated on: October 20, 2022 | 27 Comments
Python if else, for loop, and range() Exercises with Solutions
Updated on: July 6, 2024 | 296 Comments
Updated on: August 2, 2022 | 153 Comments
Updated on: September 6, 2021 | 111 Comments
Python List Exercise with Solutions
Updated on: December 8, 2021 | 200 Comments
Updated on: December 8, 2021 | 7 Comments
Python Data Structure Exercise for Beginners
Updated on: December 8, 2021 | 116 Comments
Python String Exercise with Solutions
Updated on: October 6, 2021 | 222 Comments
Updated on: March 9, 2021 | 23 Comments
Updated on: March 9, 2021 | 51 Comments
Updated on: July 20, 2021 | 29 Comments
Python Basic Exercise for Beginners
Updated on: August 31, 2023 | 493 Comments
Useful Python Tips and Tricks Every Programmer Should Know
Updated on: May 17, 2021 | 37 Comments
Python random Data generation Exercise
Updated on: December 8, 2021 | 13 Comments
Python Database Programming Exercise
Updated on: March 9, 2021 | 17 Comments
- Online Python Code Editor
Updated on: June 1, 2022 |
About PYnative
PYnative.com is for Python lovers. Here, You can get Tutorials, Exercises, and Quizzes to practice and improve your Python skills .
Explore Python
- Learn Python
- Python Basics
- Python Databases
- Python Exercises
- Python Quizzes
- Python Tricks
To get New Python Tutorials, Exercises, and Quizzes
Legal Stuff
We use cookies to improve your experience. While using PYnative, you agree to have read and accepted our Terms Of Use , Cookie Policy , and Privacy Policy .
Copyright © 2018–2024 pynative.com
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Solve Complex Equations in Python
Complex numbers seem scary, but Python can help you understand and solve equations with them. This article explores the concept of solving complex equations in Python and various approaches to solve complex equations.
Below, are the approaches for solving complex equations in Python :
- Using Symbolic Mathematics with SymPy
- Using Numerical Solver with SciPy
- Using Numpy for Roots of Polynomials
- Using Iterative Methods (e.g., Newton’s Method)
Solve Complex Equations Using Symbolic Mathematics with SymPy
The below approach code uses the SymPy library to solve a complex equation (z**2 + 1 = 0) symbolically. It defines the variable z , sets up the equation, and utilizes SymPy’s solve function to find the solutions, printing the results. In this specific example, it finds the solutions for the equation z**2 + 1 = 0 , which corresponds to complex numbers satisfying the equation.
Solve Complex Equations Using Numerical Solver with SciPy
This below approach code uses SciPy’s fsolve to find the root of a system of complex equations. The complex_equation_to_solve function defines the equations, and the initial guess is provided with real and imaginary parts, yielding a complex solution that is then printed.
Solve Complex Equations Using Numpy for Roots of Polynomials
The below approach code uses NumPy’s np.roots to find the complex roots of the polynomial equation z**2 + 1 = 0 , represented by the coefficients [1, 0, 1] . The complex roots are then printed as the output.
Solve Complex Equations Using Iterative Methods (e.g., Newton’s Method)
The below approach code applies Newton’s method iteratively to find the complex root of the equation z 2 +1=0 , starting with an initial guess of 0.0+1.0 j. The result is printed as the complex solution after the specified maximum number of iterations (100) . We can adjust the equation and initial guess as needed for different complex problems.
In conclusion, Python offers a variety of powerful tools and libraries for solving complex equations efficiently. libraries such as SymPy, SciPy, and NumPy, users can tackle mathematical problems with ease. SymPy provides symbolic mathematics capabilities, allowing users to manipulate algebraic expressions and solve equations symbolically.
Please Login to comment...
Similar reads.
- Python Programs
- Python-numpy
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Python »
- 3.12.4 Documentation »
- The Python Standard Library »
- Numeric and Mathematical Modules »
- math — Mathematical functions
- Theme Auto Light Dark |
math — Mathematical functions ¶
This module provides access to the mathematical functions defined by the C standard.
These functions cannot be used with complex numbers; use the functions of the same name from the cmath module if you require support for complex numbers. The distinction between functions which support complex numbers and those which don’t is made since most users do not want to learn quite as much mathematics as required to understand complex numbers. Receiving an exception instead of a complex result allows earlier detection of the unexpected complex number used as a parameter, so that the programmer can determine how and why it was generated in the first place.
The following functions are provided by this module. Except when explicitly noted otherwise, all return values are floats.
Number-theoretic and representation functions ¶
Return the ceiling of x , the smallest integer greater than or equal to x . If x is not a float, delegates to x.__ceil__ , which should return an Integral value.
Return the number of ways to choose k items from n items without repetition and without order.
Evaluates to n! / (k! * (n - k)!) when k <= n and evaluates to zero when k > n .
Also called the binomial coefficient because it is equivalent to the coefficient of k-th term in polynomial expansion of (1 + x)ⁿ .
Raises TypeError if either of the arguments are not integers. Raises ValueError if either of the arguments are negative.
Added in version 3.8.
Return a float with the magnitude (absolute value) of x but the sign of y . On platforms that support signed zeros, copysign(1.0, -0.0) returns -1.0 .
Return the absolute value of x .
Return n factorial as an integer. Raises ValueError if n is not integral or is negative.
Deprecated since version 3.9: Accepting floats with integral values (like 5.0 ) is deprecated.
Return the floor of x , the largest integer less than or equal to x . If x is not a float, delegates to x.__floor__ , which should return an Integral value.
Return fmod(x, y) , as defined by the platform C library. Note that the Python expression x % y may not return the same result. The intent of the C standard is that fmod(x, y) be exactly (mathematically; to infinite precision) equal to x - n*y for some integer n such that the result has the same sign as x and magnitude less than abs(y) . Python’s x % y returns a result with the sign of y instead, and may not be exactly computable for float arguments. For example, fmod(-1e-100, 1e100) is -1e-100 , but the result of Python’s -1e-100 % 1e100 is 1e100-1e-100 , which cannot be represented exactly as a float, and rounds to the surprising 1e100 . For this reason, function fmod() is generally preferred when working with floats, while Python’s x % y is preferred when working with integers.
Return the mantissa and exponent of x as the pair (m, e) . m is a float and e is an integer such that x == m * 2**e exactly. If x is zero, returns (0.0, 0) , otherwise 0.5 <= abs(m) < 1 . This is used to “pick apart” the internal representation of a float in a portable way.
Return an accurate floating-point sum of values in the iterable. Avoids loss of precision by tracking multiple intermediate partial sums.
The algorithm’s accuracy depends on IEEE-754 arithmetic guarantees and the typical case where the rounding mode is half-even. On some non-Windows builds, the underlying C library uses extended precision addition and may occasionally double-round an intermediate sum causing it to be off in its least significant bit.
For further discussion and two alternative approaches, see the ASPN cookbook recipes for accurate floating-point summation .
Return the greatest common divisor of the specified integer arguments. If any of the arguments is nonzero, then the returned value is the largest positive integer that is a divisor of all arguments. If all arguments are zero, then the returned value is 0 . gcd() without arguments returns 0 .
Added in version 3.5.
Changed in version 3.9: Added support for an arbitrary number of arguments. Formerly, only two arguments were supported.
Return True if the values a and b are close to each other and False otherwise.
Whether or not two values are considered close is determined according to given absolute and relative tolerances.
rel_tol is the relative tolerance – it is the maximum allowed difference between a and b , relative to the larger absolute value of a or b . For example, to set a tolerance of 5%, pass rel_tol=0.05 . The default tolerance is 1e-09 , which assures that the two values are the same within about 9 decimal digits. rel_tol must be greater than zero.
abs_tol is the minimum absolute tolerance – useful for comparisons near zero. abs_tol must be at least zero.
If no errors occur, the result will be: abs(a-b) <= max(rel_tol * max(abs(a), abs(b)), abs_tol) .
The IEEE 754 special values of NaN , inf , and -inf will be handled according to IEEE rules. Specifically, NaN is not considered close to any other value, including NaN . inf and -inf are only considered close to themselves.
PEP 485 – A function for testing approximate equality
Return True if x is neither an infinity nor a NaN, and False otherwise. (Note that 0.0 is considered finite.)
Added in version 3.2.
Return True if x is a positive or negative infinity, and False otherwise.
Return True if x is a NaN (not a number), and False otherwise.
Return the integer square root of the nonnegative integer n . This is the floor of the exact square root of n , or equivalently the greatest integer a such that a ² ≤ n .
For some applications, it may be more convenient to have the least integer a such that n ≤ a ², or in other words the ceiling of the exact square root of n . For positive n , this can be computed using a = 1 + isqrt(n - 1) .
Return the least common multiple of the specified integer arguments. If all arguments are nonzero, then the returned value is the smallest positive integer that is a multiple of all arguments. If any of the arguments is zero, then the returned value is 0 . lcm() without arguments returns 1 .
Added in version 3.9.
Return x * (2**i) . This is essentially the inverse of function frexp() .
Return the fractional and integer parts of x . Both results carry the sign of x and are floats.
Return the floating-point value steps steps after x towards y .
If x is equal to y , return y , unless steps is zero.
math.nextafter(x, math.inf) goes up: towards positive infinity.
math.nextafter(x, -math.inf) goes down: towards minus infinity.
math.nextafter(x, 0.0) goes towards zero.
math.nextafter(x, math.copysign(math.inf, x)) goes away from zero.
See also math.ulp() .
Changed in version 3.12: Added the steps argument.
Return the number of ways to choose k items from n items without repetition and with order.
Evaluates to n! / (n - k)! when k <= n and evaluates to zero when k > n .
If k is not specified or is None , then k defaults to n and the function returns n! .
Calculate the product of all the elements in the input iterable . The default start value for the product is 1 .
When the iterable is empty, return the start value. This function is intended specifically for use with numeric values and may reject non-numeric types.
Return the IEEE 754-style remainder of x with respect to y . For finite x and finite nonzero y , this is the difference x - n*y , where n is the closest integer to the exact value of the quotient x / y . If x / y is exactly halfway between two consecutive integers, the nearest even integer is used for n . The remainder r = remainder(x, y) thus always satisfies abs(r) <= 0.5 * abs(y) .
Special cases follow IEEE 754: in particular, remainder(x, math.inf) is x for any finite x , and remainder(x, 0) and remainder(math.inf, x) raise ValueError for any non-NaN x . If the result of the remainder operation is zero, that zero will have the same sign as x .
On platforms using IEEE 754 binary floating point, the result of this operation is always exactly representable: no rounding error is introduced.
Added in version 3.7.
Return the sum of products of values from two iterables p and q .
Raises ValueError if the inputs do not have the same length.
Roughly equivalent to:
For float and mixed int/float inputs, the intermediate products and sums are computed with extended precision.
Added in version 3.12.
Return x with the fractional part removed, leaving the integer part. This rounds toward 0: trunc() is equivalent to floor() for positive x , and equivalent to ceil() for negative x . If x is not a float, delegates to x.__trunc__ , which should return an Integral value.
Return the value of the least significant bit of the float x :
If x is a NaN (not a number), return x .
If x is negative, return ulp(-x) .
If x is a positive infinity, return x .
If x is equal to zero, return the smallest positive denormalized representable float (smaller than the minimum positive normalized float, sys.float_info.min ).
If x is equal to the largest positive representable float, return the value of the least significant bit of x , such that the first float smaller than x is x - ulp(x) .
Otherwise ( x is a positive finite number), return the value of the least significant bit of x , such that the first float bigger than x is x + ulp(x) .
ULP stands for “Unit in the Last Place”.
See also math.nextafter() and sys.float_info.epsilon .
Note that frexp() and modf() have a different call/return pattern than their C equivalents: they take a single argument and return a pair of values, rather than returning their second return value through an ‘output parameter’ (there is no such thing in Python).
For the ceil() , floor() , and modf() functions, note that all floating-point numbers of sufficiently large magnitude are exact integers. Python floats typically carry no more than 53 bits of precision (the same as the platform C double type), in which case any float x with abs(x) >= 2**52 necessarily has no fractional bits.
Power and logarithmic functions ¶
Return the cube root of x .
Added in version 3.11.
Return e raised to the power x , where e = 2.718281… is the base of natural logarithms. This is usually more accurate than math.e ** x or pow(math.e, x) .
Return 2 raised to the power x .
Return e raised to the power x , minus 1. Here e is the base of natural logarithms. For small floats x , the subtraction in exp(x) - 1 can result in a significant loss of precision ; the expm1() function provides a way to compute this quantity to full precision:
With one argument, return the natural logarithm of x (to base e ).
With two arguments, return the logarithm of x to the given base , calculated as log(x)/log(base) .
Return the natural logarithm of 1+x (base e ). The result is calculated in a way which is accurate for x near zero.
Return the base-2 logarithm of x . This is usually more accurate than log(x, 2) .
Added in version 3.3.
int.bit_length() returns the number of bits necessary to represent an integer in binary, excluding the sign and leading zeros.
Return the base-10 logarithm of x . This is usually more accurate than log(x, 10) .
Return x raised to the power y . Exceptional cases follow the IEEE 754 standard as far as possible. In particular, pow(1.0, x) and pow(x, 0.0) always return 1.0 , even when x is a zero or a NaN. If both x and y are finite, x is negative, and y is not an integer then pow(x, y) is undefined, and raises ValueError .
Unlike the built-in ** operator, math.pow() converts both its arguments to type float . Use ** or the built-in pow() function for computing exact integer powers.
Changed in version 3.11: The special cases pow(0.0, -inf) and pow(-0.0, -inf) were changed to return inf instead of raising ValueError , for consistency with IEEE 754.
Return the square root of x .
Trigonometric functions ¶
Return the arc cosine of x , in radians. The result is between 0 and pi .
Return the arc sine of x , in radians. The result is between -pi/2 and pi/2 .
Return the arc tangent of x , in radians. The result is between -pi/2 and pi/2 .
Return atan(y / x) , in radians. The result is between -pi and pi . The vector in the plane from the origin to point (x, y) makes this angle with the positive X axis. The point of atan2() is that the signs of both inputs are known to it, so it can compute the correct quadrant for the angle. For example, atan(1) and atan2(1, 1) are both pi/4 , but atan2(-1, -1) is -3*pi/4 .
Return the cosine of x radians.
Return the Euclidean distance between two points p and q , each given as a sequence (or iterable) of coordinates. The two points must have the same dimension.
Return the Euclidean norm, sqrt(sum(x**2 for x in coordinates)) . This is the length of the vector from the origin to the point given by the coordinates.
For a two dimensional point (x, y) , this is equivalent to computing the hypotenuse of a right triangle using the Pythagorean theorem, sqrt(x*x + y*y) .
Changed in version 3.8: Added support for n-dimensional points. Formerly, only the two dimensional case was supported.
Changed in version 3.10: Improved the algorithm’s accuracy so that the maximum error is under 1 ulp (unit in the last place). More typically, the result is almost always correctly rounded to within 1/2 ulp.
Return the sine of x radians.
Return the tangent of x radians.
Angular conversion ¶
Convert angle x from radians to degrees.
Convert angle x from degrees to radians.
Hyperbolic functions ¶
Hyperbolic functions are analogs of trigonometric functions that are based on hyperbolas instead of circles.
Return the inverse hyperbolic cosine of x .
Return the inverse hyperbolic sine of x .
Return the inverse hyperbolic tangent of x .
Return the hyperbolic cosine of x .
Return the hyperbolic sine of x .
Return the hyperbolic tangent of x .
Special functions ¶
Return the error function at x .
The erf() function can be used to compute traditional statistical functions such as the cumulative standard normal distribution :
Return the complementary error function at x . The complementary error function is defined as 1.0 - erf(x) . It is used for large values of x where a subtraction from one would cause a loss of significance .
Return the Gamma function at x .
Return the natural logarithm of the absolute value of the Gamma function at x .
Constants ¶
The mathematical constant π = 3.141592…, to available precision.
The mathematical constant e = 2.718281…, to available precision.
The mathematical constant τ = 6.283185…, to available precision. Tau is a circle constant equal to 2 π , the ratio of a circle’s circumference to its radius. To learn more about Tau, check out Vi Hart’s video Pi is (still) Wrong , and start celebrating Tau day by eating twice as much pie!
Added in version 3.6.
A floating-point positive infinity. (For negative infinity, use -math.inf .) Equivalent to the output of float('inf') .
A floating-point “not a number” (NaN) value. Equivalent to the output of float('nan') . Due to the requirements of the IEEE-754 standard , math.nan and float('nan') are not considered to equal to any other numeric value, including themselves. To check whether a number is a NaN, use the isnan() function to test for NaNs instead of is or == . Example:
Changed in version 3.11: It is now always available.
CPython implementation detail: The math module consists mostly of thin wrappers around the platform C math library functions. Behavior in exceptional cases follows Annex F of the C99 standard where appropriate. The current implementation will raise ValueError for invalid operations like sqrt(-1.0) or log(0.0) (where C99 Annex F recommends signaling invalid operation or divide-by-zero), and OverflowError for results that overflow (for example, exp(1000.0) ). A NaN will not be returned from any of the functions above unless one or more of the input arguments was a NaN; in that case, most functions will return a NaN, but (again following C99 Annex F) there are some exceptions to this rule, for example pow(float('nan'), 0.0) or hypot(float('nan'), float('inf')) .
Note that Python makes no effort to distinguish signaling NaNs from quiet NaNs, and behavior for signaling NaNs remains unspecified. Typical behavior is to treat all NaNs as though they were quiet.
Complex number versions of many of these functions.
Table of Contents
- Number-theoretic and representation functions
- Power and logarithmic functions
- Trigonometric functions
- Angular conversion
- Hyperbolic functions
- Special functions
Previous topic
numbers — Numeric abstract base classes
cmath — Mathematical functions for complex numbers
- Report a Bug
- Show Source
MathChat - An Conversational Framework to Solve Math Problems
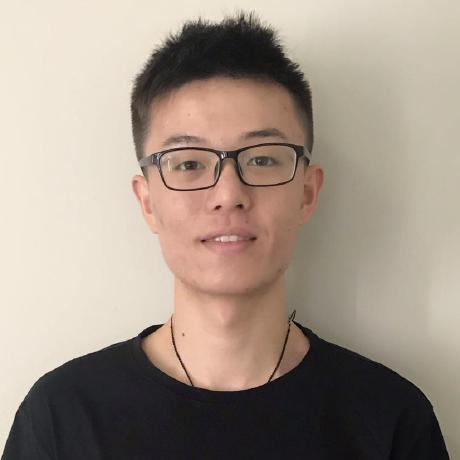
- We introduce MathChat, a conversational framework leveraging Large Language Models (LLMs), specifically GPT-4, to solve advanced mathematical problems.
- MathChat improves LLM's performance on challenging math problem-solving, outperforming basic prompting and other strategies by about 6%. The improvement was especially notable in the Algebra category, with a 15% increase in accuracy.
- Despite the advancement, GPT-4 still struggles to solve very challenging math problems, even with effective prompting strategies. Further improvements are needed, such as the development of more specific assistant models or the integration of new tools and prompts.
Recent Large Language Models (LLMs) like GTP-3.5 and GPT-4 have demonstrated astonishing abilities over previous models on various tasks, such as text generation, question answering, and code generation. Moreover, these models can communicate with humans through conversations and remember previous contexts, making it easier for humans to interact with them. These models play an increasingly important role in our daily lives assisting people with different tasks, such as writing emails, summarizing documents, and writing code.
In this blog post, we probe into the problem-solving capabilities of LLMs. Specifically, we are interested in their capabilities to solve advanced math problems, which could be representative of a broader class of problems that require precise reasoning and also have deterministic solutions.
We introduce MathChat, a conversational framework designed for solving challenging math problems with LLMs. This framework takes advantage of the chat-optimized feature of state-of-the-art LLMs, where a user proxy agent and an LLM assistant work together to tackle math problems. We also test previous prompting techniques for comparison.
The MathChat Framework
MathChat simulates a conversation between the LLM assistant and a user proxy agent. As the name indicates, the user proxy agent acts as a proxy for the user, which is responsible for communicating with the LLM assistant and continuing the conversation in a desired manner.
The proxy agent first presents a math problem to the LLM assistant, framed by an initial prompt that guides the assistant in how it should work collaboratively to solve the problem. We incorporate existing prompting methods such as CoT (chain-of-thought) and tool-using are incorporated in the initial prompt. Key components of the prompt include:
Tool-using Prompt: This guides the LLM assistant to use Python code in the correct format to address the problem, allowing the user proxy agent to parse the code and provide the appropriate results.
Problem-Solving Strategy Selection Prompt: The assistant is instructed to choose one of three potential problem-solving strategies, including:
- Writing a Python program to solve the problem directly.
- Solving the problem directly without using Python, which exercises LLMs' inherent reasoning capabilities.
- Solving the problem step by step with Python. This should be used when the first two strategies aren't suitable.
Final Answer Encapsulation Prompt: This part instructs the assistant to put the final answer in \boxed .
The prompt design in MathChat is flexible and can easily be refined to include other tools, such as Wolfram Alpha.
Let's take a look at an example between the User Proxy Agent and the LLM Assistant (GPT-4). The conversation focuses on how to solve inequality using Python. (The conversation is modified for readability.)
Participant | Dialogue |
---|---|
User Proxy Agent | (Initial Prompt: Let's solve a math problem with Python...) Problem: Find all x that satisfy the inequality (2x+10)(x+3) < (3x+9)(x+8). Express your answer in interval notation. |
LLM assistant | The key idea to solve this problem is to find the critical points, which are the points where the inequality is equal. Then, test the intervals between the critical points to find the interval for which the inequality holds. |
LLM assistant (continued) | Here's Python code to find the critical points: |
User Proxy Agent | The critical points are: [-14, -3] |
LLM assistant | We found the critical points to be x = -14 and x = -3. Now we'll test the intervals between these critical points as well as points before and after the critical points. The intervals are: x < -14, -14 < x < -3, x > -3. |
LLM assistant (continued) | Here's Python code to test the inequality for each interval: |
User Proxy Agent | The intervals where the inequality holds true are for test points: [-15, 0] |
LLM assistant | We have tested the intervals and found that the inequality holds for: 1. x < -14 (test point: -15) 2. x > -3 (test point: 0). Therefore, the solution to the inequality (2x+10)(x+3) < (3x+9)(x+8) is x ∈ (-∞, -14) ∪ (-3, ∞) |
Experiment Setup
We evaluate the improvement brought by MathChat.
For the experiment, we focus on the level-5 problems from the MATH dataset, which are composed of high school competition problems. These problems include the application of theorems and complex equation derivation and are challenging even for undergraduate students. We evaluate 6 of 7 categories from the dataset (excluding Geometry): Prealgebra, Algebra, Number Theory, Counting and Probability, Intermediate Algebra, and Precalculus.
We evaluate GPT-4 and use the default configuration of the OpenAI API. To access the final performance, we manually compare the final answer with the correct answer. For the vanilla prompt, Program Synthesis, and MathChat, we have GPT-4 enclose the final answer in \boxed , and we take the return of the function in PoT as the final answer.
We also evaluate the following methods for comparison:
Vanilla prompting: Evaluates GPT-4's direct problem-solving capability. The prompt used is: " Solve the problem carefully. Put the final answer in \boxed " .
Program of Thoughts (PoT): Uses a zero-shot PoT prompt that requests the model to create a Solver function to solve the problem and return the final answer.
Program Synthesis (PS) prompting: Like PoT, it prompts the model to write a program to solve the problem. The prompt used is: "Write a program that answers the following question: {Problem}" .
Experiment Results
The accuracy on all the problems with difficulty level-5 from different categories of the MATH dataset with different methods is shown below:
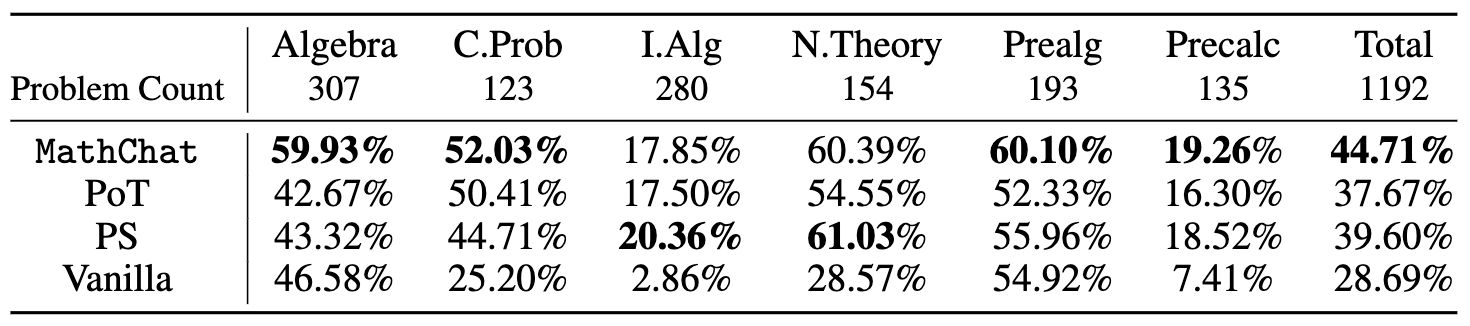
We found that compared to basic prompting, which demonstrates the innate capabilities of GPT-4, utilizing Python within the context of PoT or PS strategy improved the overall accuracy by about 10%. This increase was mostly seen in categories involving more numerical manipulations, such as Counting & Probability and Number Theory, and in more complex categories like Intermediate Algebra and Precalculus.
For categories like Algebra and Prealgebra, PoT and PS showed little improvement, and in some instances, even led to a decrease in accuracy. However, MathChat was able to enhance total accuracy by around 6% compared to PoT and PS, showing competitive performance across all categories. Remarkably, MathChat improved accuracy in the Algebra category by about 15% over other methods. Note that categories like Intermediate Algebra and Precalculus remained challenging for all methods, with only about 20% of problems solved accurately.
The code for experiments can be found at this repository . We now provide an implementation of MathChat using the interactive agents in AutoGen. See this notebook for example usage.
Future Directions
Despite MathChat's improvements over previous methods, the results show that complex math problem is still challenging for recent powerful LLMs, like GPT-4, even with help from external tools.
Further work can be done to enhance this framework or math problem-solving in general:
- Although enabling the model to use tools like Python can reduce calculation errors, LLMs are still prone to logic errors. Methods like self-consistency (Sample several solutions and take a major vote on the final answer), or self-verification (use another LLM instance to check whether an answer is correct) might improve the performance.
- Sometimes, whether the LLM can solve the problem depends on the plan it uses. Some plans require less computation and logical reasoning, leaving less room for mistakes.
- MathChat has the potential to be adapted into a copilot system, which could assist users with math problems. This system could allow users to be more involved in the problem-solving process, potentially enhancing learning.
For Further Reading
- Research paper of MathChat
- Documentation about autogen
Are you working on applications that involve math problem-solving? Would you appreciate additional research or support on the application of LLM-based agents for math problem-solving? Please join our Discord server for discussion.
- The MathChat Framework
- Experiment Setup
- Experiment Results
- Future Directions
- For Further Reading

COMMENTS
We use a library called math, which is a built-in python library to do quick mathematical operations. The function math.sqrt takes the square root of the result y that we got from (task 1) E ...
Expected Output : Quadratic function : (a * x^2) + b*x + c a: 25 b: 64 c: 36 There are 2 roots: -0.834579 and -1.725421. Click me to see the sample solution. 31. Write a Python program to convert a decimal number to a binary number. Expected Output : Input a binary number: 101011 The decimal value of the number is 43.
The SymPy symbolic math library in Python can do pretty much any kind of math, solving equations, simplifying, factoring, substituting values for variables, pretty printing, converting to LaTeX format, etc. etc. It seems to be a pretty robust solver in my very limited use so far. I recommend trying it out.
Getting to Know the Python math Module. The Python math module is an important feature designed to deal with mathematical operations. It comes packaged with the standard Python release and has been there from the beginning. Most of the math module's functions are thin wrappers around the C platform's mathematical functions. Since its underlying functions are written in CPython, the math ...
Solve Algebraic Equations in One Variable Using the solve() Method From the SymPy Package. The SymPy library has a solve() function that can solve algebraic equations. This function accepts the following main arguments. f: An algebraic equation. symbols: The variables for which the equation has to be solved.
The line sol = solve_bvp(heat_equation, boundary_conditions, x, y) is the solution. The code solve_bvp stands for solve boundary value problem. It takes four arguments: heat_equation: This is the main problem we are trying to solve. boundary_conditions: These are the mathematical constrains at the start and end of a solution.
Linear programming is a set of techniques used in mathematical programming, sometimes called mathematical optimization, to solve systems of linear equations and inequalities while maximizing or minimizing some linear function. It's important in fields like scientific computing, economics, technical sciences, manufacturing, transportation ...
Solving Equations Solving Equations. SymPy's solve() function can be used to solve equations and expressions that contain symbolic math variables.. Equations with one solution. A simple equation that contains one variable like x-4-2 = 0 can be solved using the SymPy's solve() function. When only one value is part of the solution, the solution is in the form of a list.
00:00 Welcome to this video course featuring exercises on using numbers and math in Python. In this short course, you'll have the opportunity to test yourself by solving a few basic mathematical problems with Python. 00:14 This is a follow-up to another video course with a similar title that you should watch first. If you haven't, then please follow the link listed in the description below ...
Problem solving in Python. Learn problem solving in Python from our online course and tutorial. You will learn basic math, conditionals and step by step logic building to solve problems easily. 4.5 (3658 reviews) 18 lessons Beginner level. 46.7k Learners.
There are 5 modules in this course. This course is designed for the Python programmer who wants to develop the foundations of Calculus to help solve challenging problems as well as the student of mathematics looking to learn the theory and numerical techniques of applied calculus implemented in Python. By the end of this course, you will have ...
SymPy: Solving Math Equations in Python and Jupyter. SymPy is a Python library for symbolic mathematics. It's free and open source, and because it's written entirely in Python, it's easy to install and use. Symbolic math software tools, also called Computer Algebra Systems (CAS), allow you to work with mathematical equations more or less ...
Indicative syllabus: - The phases of problem-solving according to Pólya, Mason and others. - Using Python to enhance the problem-solving phases. - Writing a problem-solving rubric. - Making conjectures and dealing with being stuck. - Justifying and convincing. - Asking question and extending a given mathematical problem.
Help readers to develop the practical skills needed to build models and solving problem using state-of-the-art modeling languages and solvers. Getting started# The notebooks in this repository make extensive use of Pyomo which is a complete and versatile mathematical optimization package for the Python ecosystem. Pyomo provides a means to build ...
Website companion for the book Problem Solving with Python by Peter D. Kazarinoff ... You can define equations in Python using SymPy and symbolic math variables. Equations in SymPy are different than expressions. An expression does not have equality. An expression is a collection of symbols and operators, but expressions are not equal to anything.
Website companion for the book Problem Solving with Python by Peter D. Kazarinoff ... LaTeX Math Problem Solving with Python Book Construction Contributions Cover Artwork About the Author Table of contents. Overview Hard Copy License ...
Part I - Mathematical Modeling: It has 2 chapters and introduces the theory and concepts of mathematical modeling and discusses how a business problem can be formulated mathematically. Part II - Mathematical Tools: It consists of 6 chapters (all have python code examples) about the most tried and tested tools that enable mathematical modeling ...
Linear algebra is an important topic across a variety of subjects. It allows you to solve problems related to vectors, matrices, and linear equations.In Python, most of the routines related to this subject are implemented in scipy.linalg, which offers very fast linear algebra capabilities.. In particular, linear models play an important role in a variety of real-world problems, and scipy ...
Each exercise has 10-20 Questions. The solution is provided for every question. Practice each Exercise in Online Code Editor. These Python programming exercises are suitable for all Python developers. If you are a beginner, you will have a better understanding of Python after solving these exercises. Below is the list of exercises.
Rhombus 1. A matrix with rhombus with a radius of 2. Rhombus 1 is highlighted with the blue rhombus. The digits contained in rhombus 1 are summed as follows: 99 + 19 + 78 +12 + 12 = 220. Rhombus 2 ...
Using Symbolic Mathematics with SymPy; Using Numerical Solver with SciPy; Using Numpy for Roots of Polynomials; Using Iterative Methods (e.g., Newton's Method) Solve Complex Equations Using Symbolic Mathematics with SymPy. The below approach code uses the SymPy library to solve a complex equation (z**2 + 1 = 0) symbolically. It defines the variable z, sets up the equation, and utilizes SymPy ...
math. trunc (x) ¶ Return x with the fractional part removed, leaving the integer part. This rounds toward 0: trunc() is equivalent to floor() for positive x, and equivalent to ceil() for negative x.If x is not a float, delegates to x.__trunc__, which should return an Integral value.. math. ulp (x) ¶ Return the value of the least significant bit of the float x:. If x is a NaN (not a number ...
Problem-Solving Strategy Selection Prompt: The assistant is instructed to choose one of three potential problem-solving strategies, including: Writing a Python program to solve the problem directly. Solving the problem directly without using Python, which exercises LLMs' inherent reasoning capabilities. Solving the problem step by step with Python.