scipy.optimize.linear_sum_assignment #
Solve the linear sum assignment problem.
The cost matrix of the bipartite graph.
Calculates a maximum weight matching if true.
An array of row indices and one of corresponding column indices giving the optimal assignment. The cost of the assignment can be computed as cost_matrix[row_ind, col_ind].sum() . The row indices will be sorted; in the case of a square cost matrix they will be equal to numpy.arange(cost_matrix.shape[0]) .
for sparse inputs
The linear sum assignment problem [1] is also known as minimum weight matching in bipartite graphs. A problem instance is described by a matrix C, where each C[i,j] is the cost of matching vertex i of the first partite set (a ‘worker’) and vertex j of the second set (a ‘job’). The goal is to find a complete assignment of workers to jobs of minimal cost.
Formally, let X be a boolean matrix where \(X[i,j] = 1\) iff row i is assigned to column j. Then the optimal assignment has cost
where, in the case where the matrix X is square, each row is assigned to exactly one column, and each column to exactly one row.
This function can also solve a generalization of the classic assignment problem where the cost matrix is rectangular. If it has more rows than columns, then not every row needs to be assigned to a column, and vice versa.
This implementation is a modified Jonker-Volgenant algorithm with no initialization, described in ref. [2] .
New in version 0.17.0.
https://en.wikipedia.org/wiki/Assignment_problem
DF Crouse. On implementing 2D rectangular assignment algorithms. IEEE Transactions on Aerospace and Electronic Systems , 52(4):1679-1696, August 2016, DOI:10.1109/TAES.2016.140952
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
- Responsive Chart with Bokeh, Flask and Python
- Handling Categorical Data in Python
- Remove all columns where the entire column is null in PySpark DataFrame
- Precision, Recall and F1-Score using R
- Merge and Unmerge Excel Cells using openpyxl in R
- Microsoft Stock Price Prediction with Machine Learning
- Delete pages from a PDF file in Python
- How to Build a Web App using Flask and SQLite in Python
- Hidden Layer Perceptron in TensorFlow
- What is setup.py in Python?
- Ledoit-Wolf vs OAS Estimation in Scikit Learn
- Top 10 String methods in Pandas
- Training of Recurrent Neural Networks (RNN) in TensorFlow
- Make Scatter Plot From Set of Points in Python Tuples
- Fill a Python String with Spaces
- Python Django Queryset Filtering
- Python OpenCV - distanceTransform() Function
- SciPy - Stats
- Calculate Time Difference in Python

Optimization in SciPy
SciPy is a Python library that is available for free and open source and is used for technical and scientific computing. It is a set of useful functions and mathematical methods created using Python’s NumPy module.
Features of SciPy:
- Creating complex programs and specialized applications is a benefit of building SciPy on Python.
- SciPy contains varieties of sub-packages that help to solve the most common issue related to Scientific Computation.
- SciPy users benefit from the inclusion of new modules created by programmers all around the world in a variety of software-related fields.
- Easy to use and understand as well as fast computational power.
- It can operate on an array of NumPy libraries.
Sub-packages of SciPy:
In this article, we will learn the scipy.optimize sub-package.
This package includes functions for minimizing and maximizing objective functions subject to given constraints. Let’s understand this package with the help of examples.
SciPy – Root Finding
func : callable The function whose root is required. It must be a function of a single variable of the form f(x, a, b, c, . . . ), where a, b, c, . . . are extra arguments that can be passed in the args parameter. x0 : float, sequence, or ndarray Initial point from where you want to find the root of the function. It will somewhere near the actual root. Our initial guess is 0.
Import the optimize.newton package using the below command. Newton package contains a function that will calculate the root using the Newton Raphson method . Define a function for the given objective function. Use the newton function. This function will return the result object which contains the smallest positive root for the given function f1.
SciPy – Linear Programming
Maximize : Z = 5x + 4y Constraints : 2y ≤ 50 x + 2y ≤ 25 x + y ≤ 15 x ≥ 0 and y ≥ 0
Import the optimize.linprog module using the following command. Create an array of the objective function’s coefficients. Before that convert the objective function in minimization form by multiplying it with a negative sign in the equation. Now create the two arrays for the constraints equations. One array will be for left-hand equations and the second array for right-hand side values.
Use the linprog inbuilt function and pass the arrays that we have created an addition mention the method.
This function will return an object that contains the optimal answer for the given problem.
SciPy – Assignment Problem
A city corporation has decided to carry out road repairs on main four arteries of the city. The government has agreed to make a special grant of Rs. 50 lakh towards the cost with a condition that repairs are done at the lowest cost and quickest time. If the conditions warrant, a supplementary token grant will also be considered favourably. The corporation has floated tenders and five contractors have sent in their bids. In order to expedite work, one road will be awarded to only one contractor. Cost of Repairs ( Rs in lakh) Contractors R1 R2 R3 R4 R5 C1 9 14 19 15 13 C2 7 17 20 19 18 C3 8 18 21 18 17 C4 10 12 18 19 18 C5 10 15 21 16 15 Find the best way of assigning the repair work to the contractors and the costs. If it is necessary to seek supplementary grants,what should be the amount sought?
In this code, we required the NumPy array so first install the NumPy module and then import the required modules. Create a multidimensional NumPy array of given data. Use the optimize.linear_sum_assignment() function. This function returns two NumPy arrays (Optimal solution) – one is the row ( Contactors) and the second is the column ( Corresponding Repair Cost).
Use the sum() function and calculate the total minimum optimal cost.
How the assignment will be done can be concluded below from the obtained data.
- Contractor C1 will assign road 1 with a repair cost of 13Rs Lakh.
- Contractor C2 will assign road 2 with a repair cost of 7RS lakh.
- Contractor C3 will assign road 3 with a repair cost of 21RS lakh.
- Contractor C4 will assign road 4 with a repair cost of 12RS lakh.
- Contractor C5 will assign road 5 with a repair cost of 16RS lakh.
Hence Final Minimal cost will be,
SciPy – Minimize
Broyden-fletcher-goldfarb-shanno ( bfgs ).
This algorithm deals with the Minimization of a scalar function of one or more variables. The BFGS algorithm is one of the most widely used second-order algorithms for numerical optimization, and it is frequently used to fit machine learning algorithms such as the logistic regression algorithm.
Objective Function: z = sin( X ) + cos( Y )
SciPy – curve_fit
Curve fitting requires that you define the function that maps examples of inputs to outputs like Machine Learning supervised learning . The mapping function can have a straight line ( Linear Regression ), a Curve line ( Polynomial Regression ), and much more. A detailed GeeksForGeeks article on Scipy Curve_fit is available here SciPy | Curve Fitting .
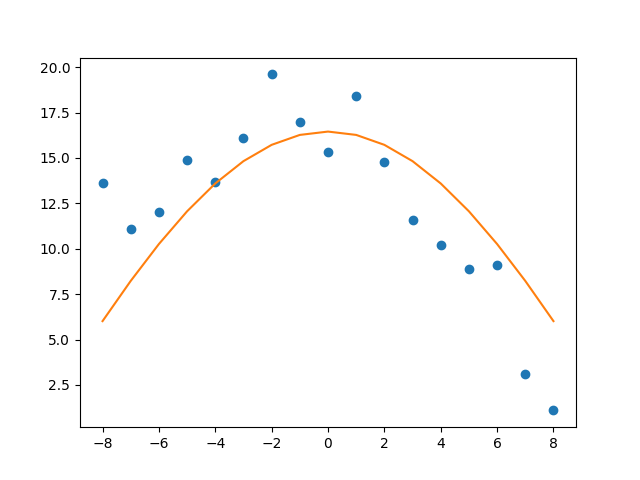
Approximate curve fitted to the input points
SciPy – Univariate Function Minimizers
Non-linear optimization with no constraint and there is only one decision variable in this optimization that we are trying to find a value for.
Nelder-Mead Simplex Search – Machine Learning
Noisy Optimization Problem – A noisy objective function is a function that gives different answers each time the same input is evaluated.
Please Login to comment...
Similar reads.
- Technical Scripter 2022
- Technical Scripter
- 5 Reasons to Start Using Claude 3 Instead of ChatGPT
- 6 Ways to Identify Who an Unknown Caller
- 10 Best Lavender AI Alternatives and Competitors 2024
- The 7 Best AI Tools for Programmers to Streamline Development in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
404 Not found
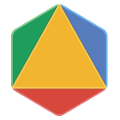
- Google OR-Tools
- Español – América Latina
- Português – Brasil
- Tiếng Việt
Linear Sum Assignment Solver
This section describes the linear sum assignment solver , a specialized solver for the simple assignment problem, which can be faster than either the MIP or CP-SAT solver. However, the MIP and CP-SAT solvers can handle a much wider array of problems, so in most cases they are the best option.
Cost matrix
The costs for workers and task are given in the table below.
The following sections present a Python program that solves an assignment problem using the linear sum assignment solver.
Import the libraries
The code that imports the required library is shown below.
Define the data
The following code creates the data for the program.
The array is the cost matrix , whose i , j entry is the cost for worker i to perform task j . There are four workers, corresponding to the rows of the matrix, and four tasks, corresponding to the columns.
Create the solver
The program uses the linear assignment solver, a specialized solver for the assignment problem.
The following code creates the solver.
Add the constraints
The following code adds the costs to the solver by looping over workers and tasks.
Invoke the solver
The following code invokes the solver.

Display the results
The following code displays the solution.
The output below shows the optimal assignment of workers to tasks.
The following graph shows the solution as the dashed edges in the graph. The numbers next to the dashed edges are their costs. The total wait time of this assignment is the sum of the costs for the dashed edges, which is 265.
In graph theory, a set of edges in a bipartite graph that matches every node on the left with exactly one node on the right is called a perfect matching .
The entire program
Here is the entire program.
Solution when workers can't perform all tasks
In the previous example, we assumed that all workers can perform all tasks. But this not always the case - a worker might be unable to perform one or more tasks for various reasons. However, it is easy to modify the program above to handle this.
As an example, suppose that worker 0 is unable to perform task 3. To modify the program to take this into account, make the following changes:
- Change the 0, 3 entry of the cost matrix to the string 'NA' . (Any string will work.) cost = [[90, 76, 75, 'NA'], [35, 85, 55, 65], [125, 95, 90, 105], [45, 110, 95, 115]]
- In the section of the code that assigns costs to the solver, add the line if cost[worker][task] != 'NA': , as shown below. for worker in range(0, rows): for task in range(0, cols): if cost[worker][task] != 'NA': assignment.AddArcWithCost(worker, task, cost[worker][task]) The added line prevents any edge whose entry in the cost matrix is 'NA' from being added to the solver.
After making these changes and running the modified code, you see the following output:
Notice that the total cost is higher now than it was for the original problem. This is not surprising, since in the original problem the optimal solution assigned worker 0 to task 3, while in the modified problem that assignment is not allowed.
To see what happens if more workers are unable to perform tasks, you can replace more entries of the cost matrix with 'NA' , to denote additional workers who can't perform certain tasks:
When you run the program this time, you get a negative result:
This means there is no way to assign workers to tasks so that each worker performs a different task. You can see why this is so by looking at the graph for the problem (in which there are no edges corresponding to values of 'NA' in the cost matrix).
Since the nodes for the three workers 0, 1, and 2 are only connected to the two nodes for tasks 0 and 1, it not possible to assign distinct tasks to these workers.
The Marriage Theorem
There is a well-known result in graph theory, called The Marriage Theorem , which tells us exactly when you can assign each node on the left to a distinct node on the right, in a bipartite graph like the one above. Such an assignment is called a perfect matching . In a nutshell, the theorem says this is possible if there is no subset of nodes on the left (like the one in the previous example ) whose edges lead to a smaller set of nodes on the right.
More precisely, the theorem says that a bipartite graph has a perfect matching if and only if for any subset S of the nodes on the left side of the graph, the set of nodes on the right side of the graph that are connected by an edge to a node in S is at least as large as S.
Except as otherwise noted, the content of this page is licensed under the Creative Commons Attribution 4.0 License , and code samples are licensed under the Apache 2.0 License . For details, see the Google Developers Site Policies . Java is a registered trademark of Oracle and/or its affiliates.
Last updated 2023-01-18 UTC.
scipy.optimize.linear_sum_assignment #
Solve the linear sum assignment problem.
The cost matrix of the bipartite graph.
Calculates a maximum weight matching if true.
An array of row indices and one of corresponding column indices giving the optimal assignment. The cost of the assignment can be computed as cost_matrix[row_ind, col_ind].sum() . The row indices will be sorted; in the case of a square cost matrix they will be equal to numpy.arange(cost_matrix.shape[0]) .
for sparse inputs
The linear sum assignment problem [1] is also known as minimum weight matching in bipartite graphs. A problem instance is described by a matrix C, where each C[i,j] is the cost of matching vertex i of the first partite set (a ‘worker’) and vertex j of the second set (a ‘job’). The goal is to find a complete assignment of workers to jobs of minimal cost.
Formally, let X be a boolean matrix where \(X[i,j] = 1\) iff row i is assigned to column j. Then the optimal assignment has cost
where, in the case where the matrix X is square, each row is assigned to exactly one column, and each column to exactly one row.
This function can also solve a generalization of the classic assignment problem where the cost matrix is rectangular. If it has more rows than columns, then not every row needs to be assigned to a column, and vice versa.
This implementation is a modified Jonker-Volgenant algorithm with no initialization, described in ref. [2] .
New in version 0.17.0.
https://en.wikipedia.org/wiki/Assignment_problem
DF Crouse. On implementing 2D rectangular assignment algorithms. IEEE Transactions on Aerospace and Electronic Systems , 52(4):1679-1696, August 2016, DOI:10.1109/TAES.2016.140952
nanolsap 0.1.3
pip install nanolsap Copy PIP instructions
Released: Aug 9, 2023
Python module to solve the linear sum assignment problem (LSAP) low memory friendly
Verified details
Maintainers.
Unverified details
Project links, github statistics.
- Open issues:
View statistics for this project via Libraries.io , or by using our public dataset on Google BigQuery
License: BSD License (BSD-3-Clause)
Author: hzqmwne
Tags lsap, munkres, kuhn-munkres, linear sum assignment problem
Requires: Python >=3.7
Classifiers
- OSI Approved :: BSD License
- Python :: 3
- Python :: 3.7
Project description
A Python module to solve the linear sum assignment problem (LSAP) efficiently. Extracted from SciPy, and modified to minimal memory cost.
Description
This module is useful in cases when you need an efficient LSAP solver on very large cost_matrix and limited memory.
For a cost_matrix with dtype float64 has shape of 30000*30000, it needs 30000*30000*8 / 1024**3 = 6.7GB memory to store it. In scipy.optimite.linear_sum_assignment, it will first convert it to float64 contiguous numpy 2-D array, then do a copy, and finally starts the solver. So, the actual memory cost is at least 6.7*2 = 13.4GB. if the origin cost_matrix does not match, for example a float32 2-D array, the first step here will cause one extra copy, increases the actual memory cost to 6.7/2+6.7*2 = 16.75GB.
In this module, When input cost_matrix is a contiguous numpy 2-D array, the solver can run on it directly without any copy. Also, cost_matrix can use small dtype like float32 to half reduce memory, so 3.35GB memory is enough.
Notice: when nr > nc, scipy.optimize.linear_sum_assignment will copy then transpose and rearrange cost matrix so keeps memory access locality, but this module do not do this, so it is about 2x slower in this situation. For nr <= nc, this module has almost no performance drop, so you can manually construct a transposed cost matrix for this module, and manually swap row_ind and col_ind result if nr > nc to get better performance.
The subrows and subcols arguments allow solver run on only a subgroup of row and cols on cost_matrix. The result should be same as scipy.optimize.linear_sum_assignment(cost_matrix[np.ix_(subrows, subcols)]), but it avoids the expensive construct of sub cost_matrix.
The code in this repository is licensed under the 3-clause BSD license, except for files including a different license header.
The LSAP solver copied from SciPy is also licensed under the 3-clause BSD license.
Some files copied from minilsap are also licensed under the 3-clause BSD license.
Project details
Release history release notifications | rss feed.
Aug 9, 2023
Jul 9, 2023
Jun 24, 2023
Jun 20, 2023
Download files
Download the file for your platform. If you're not sure which to choose, learn more about installing packages .
Source Distribution
Uploaded Aug 9, 2023 Source
Built Distributions
Uploaded Aug 9, 2023 CPython 3.7+ Windows x86-64
Uploaded Aug 9, 2023 CPython 3.7+ Windows x86
Uploaded Aug 9, 2023 CPython 3.7+ manylinux: glibc 2.17+ x86-64 manylinux: glibc 2.5+ x86-64
Uploaded Aug 9, 2023 CPython 3.7+ manylinux: glibc 2.17+ i686 manylinux: glibc 2.5+ i686
Uploaded Aug 9, 2023 CPython 3.7+ macOS 10.9+ universal2 (ARM64, x86-64)
Hashes for nanolsap-0.1.3.tar.gz
Hashes for nanolsap-0.1.3-cp37-abi3-win_amd64.whl, hashes for nanolsap-0.1.3-cp37-abi3-win32.whl, hashes for nanolsap-0.1.3-cp37-abi3-manylinux_2_5_x86_64.manylinux1_x86_64.manylinux_2_17_x86_64.manylinux2014_x86_64.whl, hashes for nanolsap-0.1.3-cp37-abi3-manylinux_2_5_i686.manylinux1_i686.manylinux_2_17_i686.manylinux2014_i686.whl, hashes for nanolsap-0.1.3-cp37-abi3-macosx_10_9_universal2.whl.
- português (Brasil)
Supported by
Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Fast linear assignment problem (LAP) solvers for Python based on c-extensions
cheind/py-lapsolver
Folders and files, repository files navigation, py-lapsolver.
py-lapsolver implements a Linear sum Assignment Problem (LAP) solver for dense matrices based on shortest path augmentation in Python. In practice, it solves 5000x5000 problems in around 3 seconds.
Windows binary wheels are provided for Python 3.5/3.6. Source wheels otherwise.
Install from source
Clone this repository
Then build the project and exectute tests
Executing the tests requires pytest and optionally pytest-benchmark for generating benchmarks.
You may also want to mark certain pairings impossible
Comparisons below are generated by scripts in ./lapsolver/benchmarks .
Currently, the following solvers are tested
- lapjv - https://github.com/gatagat/lap
- munkres - http://software.clapper.org/munkres/
- ortools - https://github.com/google/or-tools **
- scipy - https://github.com/scipy/scipy/tree/master/scipy
- lapsolver - this project
**reduced performance due to costly dense matrix to graph conversion. If you know a better way, please let me know.

Additional Benchmarks
Berhane performs an in depth analysis of Python3 linear assignment problem solver at https://github.com/berhane/LAP-solvers
py-lapsolver heavily relies on code published by @jaehyunp at https://github.com/jaehyunp/
Contributors 3
- Python 62.9%

IMAGES
VIDEO
COMMENTS
The linear sum assignment problem [1] is also known as minimum weight matching in bipartite graphs. A problem instance is described by a matrix C, where each C [i,j] is the cost of matching vertex i of the first partite set (a 'worker') and vertex j of the second set (a 'job'). The goal is to find a complete assignment of workers to ...
NOTE: I am aware I can solve this through the function scipy.optimize.linear_sum_assignment() and have done this but wanted to compare my answer by running this problem as a linear program. python scipy
SciPy is a Python library that is available for free and open source and is used for technical and scientific computing. ... Use the optimize.linear_sum_assignment() function. This function returns two NumPy arrays (Optimal solution) - one is the row ( Contactors) and the second is the column ( Corresponding Repair Cost).
minilsap. A Python module to solve the linear sum assignment problem (LSAP) efficiently. Extracted from SciPy, without significant modifications. This module is useful in cases when you need an efficient LSAP solver but you do not want to depend on the full SciPy library. Currently, the module depends on NumPy for array management.
Purpose. The script benchmarks the performance of Python3 linear assignment problem solvers for random cost matrices of different sizes. These solvers are: lapjv.lapjv - a wrapper to a C++ implementation of Jonker-Volgenant algorithm re-written for Python 3 and optimized to take advantage of AVX2 instruction sets by Vadim Markovtsev at src {d}.
minilsap. A Python module to solve the linear sum assignment problem (LSAP) efficiently. Extracted from SciPy, without significant modifications. This module is useful in cases when you need an efficient LSAP solver but you do not want to depend on the full SciPy library. Currently, the module depends on NumPy for array management.
Linear assignment with non-perfect matching. Dec 8, 2020. The linear assignment report (or simply associations problem) be the problem of finding one matching between two sets that minimizes the whole of pair-wise assignment costs. This can must expressed as finding adenine matching (or independent edge set) in a two-piece graph \(G = (U, V, E)\) that minimizes the sum of edge weights.
Google Optimization Tools (a.k.a., OR-Tools) is an open-source, fast and portable software suite for solving combinatorial optimization problems. The suite contains: Graph algorithms (shortest paths, min cost flow, max flow, linear sum assignment). We wrote OR-Tools in C++, but provide wrappers in Python, C# and Java.
The program uses the linear assignment solver, a specialized solver for the assignment problem. The following code creates the solver. Note: The linear sum assignment solver only accepts integer values for the weights and values. The section Using a solver with non-integer data shows how to use the solver if your data contains non-integer values.
The linear sum assignment problem is also known as minimum weight matching in bipartite graphs. A problem instance is described by a matrix C, where each C[i,j] is the cost of matching vertex i of the first partite set (a 'worker') and vertex j of the second set (a 'job'). The goal is to find a complete assignment of workers to jobs of ...
This Jonker-Volgenant algorithm is provided by Scipy: scipy.optimize.linear_sum_assignment. Murty's algorithm yields the top K solutions to a size n assignment problem. See [2] for an introduction to assignment problems. This implementation requires a square (nxn) matrix.
A Python module to solve the linear sum assignment problem (LSAP) efficiently. Extracted from SciPy, and modified to minimal memory cost. Description from nanolsap import linear_sum_assignment Solve the linear sum assignment problem. Parameters ----- cost_matrix : array The cost matrix of the bipartite graph.
22. You need to replace the sklearn.utils.linear_assignment_.linear_assignment function by the scipy.optimize.linear_sum_assignment function. The difference is in the return format: linear_assignment() is returning a numpy array and linear_sum_assignment() a tuple of numpy arrays. You obtain the same output by converting the output of linear ...
py-lapsolver implements a Linear sum Assignment Problem (LAP) solver for dense matrices based on shortest path augmentation in Python. In practice, it solves 5000x5000 problems in around 3 seconds. In practice, it solves 5000x5000 problems in around 3 seconds.
Python # scipy # 二部マッチング. tech. こちらのサイトで紹介されている 二部マッチング ライブラリ scipy.optimize.linear_sum_assignment で遊んでみました。 ...
I believe what you are looking for is cdist.Scipy cdist. Y = cdist(XA, XB, 'euclidean') Here is your example with working code: import numpy as np from scipy.optimize import linear_sum_assignment from scipy.spatial.distance import cdist np.random.seed(0) # define tasks t = np.random.rand(5) # define workers w = np.random.rand(3) # cost_matrix = np.array([[np.linalg.norm(x-y) for x in w] for y ...
The linear_assignment function is deprecated in 0.21 and will be removed from 0.23, but sklearn.utils.linear_assignment_ can be replaced by scipy.optimize.linear_sum_assignment. You can use: from scipy.optimize import linear_sum_assignment as linear_assignment. then you can run the file and don't need to change the code.