Go if..else, if..else..if, nested if with Best Practices
September 7, 2022

Introduction
In computer programming, conditional statements help you make decisions based on a given condition. The conditional statement evaluates if a condition is true or false, therefore it is worth noting that if statements work with boolean values. Just like other programming languages, Go has its own construct for conditional statements. In this tutorial , we will learn about different types of conditional statements in Go.
Below are the topics that we will cover
- If statement
- If - Else statement
- If -else if else statement
- Nested If statement
- Logical operators AND , OR and NOT
- Using multiple conditions if if else
Supported Comparison Operators in GO
Before we go ahead learning about golang if else statement, let us be familiar with supported comparison operators as you may need to use them in the if else conditions for decision making:
Operator | Description | Example |
---|---|---|
== | Equal to | num == 0 |
!= | Not equal to | num != 0 |
< | Less than | num < 0 |
<= | Less than or equal to | num <= 0 |
> | Greater than | num > 0 |
>= | Greater than or equal to | num >= 0 |
if statement
If a statement is used to specify a block of code that should be executed if a certain condition is true .
Explanation
In the above syntax, the keyword if is used to declare the beginning of an if statement, followed by a condition that is being tested. The condition should be a value of boolean type , the the block of code between opening and closing curly brackets will be executed only if the condition is true . In the next example, we define code that will only run if age is above 18 years.
In the preceding example, we define the age and ageLimit variables. In the if statement we compare the value of age and ageLimit by checking which is greater. The statement age > ageLimit will evaluate to true because 19 is greater than 18. The code block will be executed because the condition is true .
One liner if statement
We can also convert our if condition to run in one line, for example here I have written the same if condition in 2 different ways, one is multi line while the other is using single line:
if else Statement
If Else statement is used to evaluate both conditions that might be true or false. In the previous example, we did not really care if a condition evaluates to false. We use If Else conditional statements to handle both true and false outcomes.
In the preceding example, we define the age and ageLimit variables. In the if statement we compare the value of age and ageLimit by checking which is greater. The statement age > ageLimit will evaluate to false because 12 is less than 18. The second code block will be executed because the condition is false .
if else if statement
If Else If statement is used to specify a new condition if the first condition is false. When using If Else If statement, you can nest as many If Else conditions as you desire.
In the above example, the first if statement evaluates to false because age (17) is not greater than ageLimit(18) . We then check again if age is equal to 17, which evaluates to true . The block of code inside this section will be executed.
Nested if Statement
Nested if Statement, is a If conditional statement that has an If statement inside another If statement. The nested If statement will always be executed only if the outer If statement evaluates to true.
In the above syntax, the second if statement with condition labeled conditionY is housed inside the outer If statement with condition labeled conditionX . As long as condition labeled conditionX is true , if conditionY will be executed.
Supported Logical Operators in GO
We also have some logical operators on golang which help us when we have to check for multiple conditions:
if else statements using logical operator
In Go we use logical operators together with If conditional statements to achieve certain goals in our code. Logical operators return/execute to boolean values, hence making more sense using them conditional statements. Below are definitions of the three logical operators in Go.
Using logical AND operator
Checks of both operands are none zero. In case both operands are none-zero, a true value will be returned, else a false value will be returned. The operator representing logical AND is && .
Using logical OR operator
Check if one operand is true. In case one operand is true code will be executed. The operator representing logical OR is || .
Using logical NOT operator
Reverses the logical state of its operands. If a condition is true, the logical operator NOT will change it to false. The operator representing logical Not is ! .
Using multiple condition in golang if else statements
We can also add multiple conditional blocks inside the if else statements to further enhance the decision making. Let us check some more examples covering if else multiple condition:
if statement with 2 logical OR Operator
Here we are checking if our num is more than 100 or less than 900:
if statement with 3 logical OR Operator
In this example we add one more OR condition to the if condition:
Using both logical AND and OR operator in single if statement
We can also use both AND and OR operator inside a single if statement, here is an example:
Using multiple conditions with if..else..if..else statement
Now we have used multiple conditions in our if condition but you can use the same inside else..if condition or combine it with if..else..if statements.
In Go, we use conditional statements to handle decisions precisely in code. They enable our code to perform different computations or actions depending on whether a condition is true or false . In this article we have learned about different if conditional statements like If, If Else, If Else If Else, Nested If statements
https://go.dev/tour/flowcontrol https://www.golangprograms.com/golang-if-else-statements.html
Related Keywords: golang if else shorthand, golang if else one line, golang if else multiple conditions, golang if else string, golang if true, golang if else best practices, golang if statement with assignment, golang if not
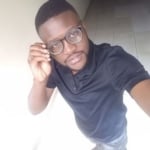
Antony Shikubu
He is highly skilled software developer with expertise in Python, Golang, and AWS cloud services. Skilled in building scalable solutions, he specializes in Django, Flask, Pandas, and NumPy for web apps and data processing, ensuring robust and maintainable code for diverse projects. You can reach out to him on his LinkedIn profile.
Can't find what you're searching for? Let us assist you.
Enter your query below, and we'll provide instant results tailored to your needs.
If my articles on GoLinuxCloud has helped you, kindly consider buying me a coffee as a token of appreciation.
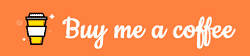
For any other feedbacks or questions you can send mail to [email protected]
Thank You for your support!!
Leave a Comment Cancel reply
Save my name and email in this browser for the next time I comment.
Notify me via e-mail if anyone answers my comment.
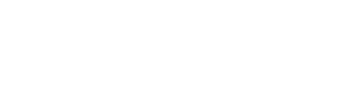
We try to offer easy-to-follow guides and tips on various topics such as Linux, Cloud Computing, Programming Languages, Ethical Hacking and much more.
Recent Comments
Popular posts, 7 tools to detect memory leaks with examples, 100+ linux commands cheat sheet & examples, tutorial: beginners guide on linux memory management, top 15 tools to monitor disk io performance with examples, overview on different disk types and disk interface types, 6 ssh authentication methods to secure connection (sshd_config), how to check security updates list & perform linux patch management rhel 6/7/8, 8 ways to prevent brute force ssh attacks in linux (centos/rhel 7).
Privacy Policy
HTML Sitemap
- Data Types in Go
- Go Keywords
- Go Control Flow
- Go Functions
- GoLang Structures
- GoLang Arrays
- GoLang Strings
- GoLang Pointers
- GoLang Interface
- GoLang Concurrency
Combining Conditional Statements in Golang
Go is a open-source programming language developed at Google by Robert Griesemer, Rob Pike, and Ken Thompson. Go is similar to C syntactically but with CSP style concurrency and many features of other robust programming language. Often refereed to as Golang because of the domain name, this language also has the If/else conditions. Usually the If/else/else if condition when written with one condition makes the program lengthy and increases the complexity thus we can combine two conditions. The conditions can be combined in the following ways :-
Using &&(AND) Operator
The And (&&) is a way of combining conditional statement has the following syntax:
Here, condition1 represents the first condition and condition2 represents the second condition. The && statement combines the conditions and gives the results in the following way:
- If condition1 is True and condition2 is also True , the result is True .
- If condition1 is True and condition2 is False , the result is False .
- If condition1 is False and condition2 is True , the result is False .
- If condition1 is False and condition2 is also False , the result is False .
Using OR operator
The OR way of combining conditional statement has the following syntax:
Here, condition1 represents the first condition and condition2 represents the second condition. The || statement combines the conditions and gives the results in the following way:
- If condition1 is True and condition2 is False , the result is Tru e .
- If condition1 is False and condition2 is True , the result is Tru e .
Please Login to comment...
Similar reads.
- Go Language
- How to Delete Discord Servers: Step by Step Guide
- Google increases YouTube Premium price in India: Check our the latest plans
- California Lawmakers Pass Bill to Limit AI Replicas
- Best 10 IPTV Service Providers in Germany
- 15 Most Important Aptitude Topics For Placements [2024]
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
The ternary operator in Golang
In most of the programming languages, there is an operator (?:) called ternary operator which evaluates like an if-else chain will do. But, Go does not have a ternary operator . In this post, we will dive into why it is absent in Go.
What is the ternary operator?
The ternary operator is the ?: operator used for the conditional value. Here is an example of how one would use it in code.
This operator is absent in Golang.
Why is it absent in Go?
The reason is a simple design choice. The operator although once understood, is an easy one is in fact a complicated construct for someone who is new to code. The Go programming language chose the simple approach of if-else. This is a longer version of the operator, but it is more readable .
The solution
The solution is obviously an if-else block. It represents the same code in a much cleaner way. Here is a simple if-else block representing the above expression.
The solution is verbose but it is a design choice made by the Go creators. One of the reasons being overuse and abuse of that operator to create extremely complex code that is hard to read. This decision improves over it and forces a cleaner and readable approach over that.
If else statement
Welcome to tutorial number 8 of our Golang tutorial series .
if statement has a condition and it executes a block of code if that condition evaluates to true . It executes an alternate else block if the condition evaluates to false . In this tutorial we will look at the various syntaxes for using a if statement.
If statement syntax
The syntax of the if statement is provided below
If the condition evaluates to true , the block of code between the braces { and } is executed.
Unlike in other languages like C, the braces { } are not optional and they are mandatory even if there is only one line of code between the braces { } .
Let’s write a simple program to find out whether a number is even or odd.
Run in Playground
In the above program, the condition num%2 in line no. 9 finds whether the remainder of dividing num by 2 is zero or not. Since it is 0 in this case, the text The number 10 is even is printed and the program exits.
The if statement has an optional else construct which will be executed if the condition in the if statement evaluates to false .
In the above snippet, if condition evaluates to false , then the block of code between else { and } will be executed.
Let’s rewrite the program to find whether the number is odd or even using if else statement.
Run in playground
In the above code, instead of returning if the condition is true as we did in the previous section , we create an else statement that will be executed if the condition is false . In this case, since 11 is odd, the if condition is false and the lines of code within the else statement is executed. The above program will print.
If … else if … else statement
The if statement also has optional else if and else components. The syntax for the same is provided below
The condition is evaluated for the truth from the top to bottom.
In the above statement, if condition1 is true, then the block of code within if condition1 { and the closing brace } is executed.
If condition1 is false and condition2 is true, then the block of code within else if condition2 { and the next closing brace } is executed.
If both condition1 and condition2 are false, then the block of code in the else statement between else { and } is executed.
There can be any number of else if statements.
In general, whichever if or else if ’s condition evaluates to true , it’s corresponding code block is executed. If none of the conditions are true then else block is executed.
Let’s write a bus ticket pricing program using else if . The program must satisfy the following requirements.
- If the age of the passenger is less than 5 years, the ticket is free.
- If the age of the passenger is between 5 and 22 years, then the ticket is $10.
- If the age of the passenger is above 22 years, then the ticket price is $15.
In the above program, the age of the passenger is set to 10 . The condition in line no. 12 is true and hence the program will print
Please try changing the age to test whether the different blocks of the if else statement are executed as expected.
If with assignment
There is one more variant of if which includes an optional shorthand assignment statement that is executed before the condition is evaluated. Its syntax is
In the above snippet, assignment-statement is first executed before the condition is evaluated.
Let’s rewrite the program which calculates the bus ticket price using the shorthand syntax.
In the above program age is initialized in the if statement in line no. 9. age can be accessed from only within the if construct. i.e. the scope of age is limited to the if , else if and else blocks. If we try to access age outside the if , else if or else blocks, the compiler will complain. This syntax often comes in handy when we declare a variable just for the purpose of if else construct. Using this syntax in such cases ensures that the scope of the variable is only within the if statement.
The else statement should start in the same line after the closing curly brace } of the if statement. If not the compiler will complain.
Let’s understand this by means of a program.
In the program above, the else statement does not start in the same line after the closing } of the if statement in line no. 11 . Instead, it starts in the next line. This is not allowed in Go. If you run this program, the compiler will print the error,
The reason is because of the way Go inserts semicolons automatically. You can read about the semicolon insertion rule here https://go.dev/ref/spec#Semicolons .
In the rules, it’s specified that a semicolon will be inserted after closing brace } , if that is the final token of the line. So a semicolon is automatically inserted after the if statement’s closing braces } in line no. 11 by the Go compiler.
So our program actually becomes
after semicolon insertion. The compiler would have inserted a semicolon in line no. 4 of the above snippet.
Since if{...} else {...} is one single statement, a semicolon should not be present in the middle of it. Hence this program fails to compile. Therefore it is a syntactical requirement to place the else in the same line after the if statement’s closing brace } .
I have rewritten the program by moving the else after the closing } of the if statement to prevent the automatic semicolon insertion.
Now the compiler will be happy and so are we 😃.
Idiomatic Go
We have seen various if-else constructs and we have in fact seen multiple ways to write the same program. For example, we have seen multiple ways to write a program that checks whether the number is even or odd using different if else constructs. Which one is the idiomatic way of coding in Go? In Go’s philosophy, it is better to avoid unnecessary branches and indentation of code. It is also considered better to return as early as possible. I have provided the program from the previous section below,
The idiomatic way of writing the above program in Go’s philosophy is to avoid the else and return from the if if the condition is true .
In the above program, as soon as we find out the number is even, we return immediately. This avoids the unnecessary else code branch. This is the way things are done in Go 😃. Please keep this in mind whenever writing Go programs.
I hope you liked this tutorial. Please leave your feedback and comments. Please consider sharing this tutorial on twitter or LinkedIn . Have a good day.
Next tutorial - Loops
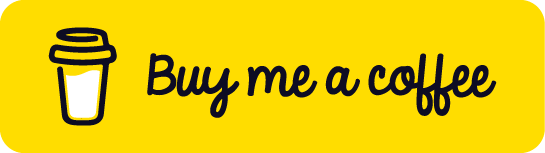
🦥 Ternary operator in Go
In short, the ternary operator known from languages like PHP or JavaScript is not available in Go. But you can express the same condition using the if {..} else {..} block, which is longer but easier to understand. The fact that it is not in the syntax is the result of a deliberate choice by the language designers , who noticed that the ternary operator is often used to create overly complicated expressions.
As a result, there is only one conditional control flow construct in Go: if {..} else {..} . So, instead of writing:
you need to write:
Thank you for being on our site 😊. If you like our tutorials and examples, please consider supporting us with a cup of coffee and we'll turn it into more great Go examples.
Have a great day!
❤️🩹 Recover function in Go
Learn what it is for and how to use the built-in recover() function, ➰ foreach loop in go, learn how to construct the popular programming loop, 🫘 count the occurrences of an element in a slice in go, learn how to count elements in a slice that meet certain conditions.

DEV Community

Posted on Mar 14, 2022
If/Else and Conditional Statements in Go
In Go, we can use conditional statements to execute code based on different conditions. We can also use if , else if and if else statements to execute code provided if a condition is true or false. If/else statements are commonplace in programming, so if you're already a seasoned programmer you won't spend long here. But as always, we'll take our time and make sure we understand fully what's going on.
In this article we'll cover:
- The if statement
- The if else statement
- The else if statement
- Conditional statements
- if statements inside if statements (Ifception).
As always, I have a simple Go file that shows how if statements and conditions work on my GitHub .
The If Statement
We can use if statements to execute pieces of code if a condition is true. For example:
Here, we have two int variables: number1 set to 10 and number2 set to 20. Here we evaluate whether or not number1 is a bigger number than number2 . Since 10 isn't bigger than 20, our Println statement won't be executed.
The If Else Statement
What if we wanted another piece of code to execute to show the user that the condition we just evaluate was false, rather than doing nothing? This is where we can use the else statement to do this for us. For example:
Thanks to this else statement, our program will now print out a response telling us that our condition is false.
The Else If Statement
What if we have multiple conditions we wish to evaluate? If we have several conditions, we should use a switch statement. But if we have two or three conditions, we can use a else if statement like so:
Here, our first condition will fail since the remainder of 9 divided by 2 is not equal 0, so Go will then evaluate our second condition.
Here 9 is smaller than 10, so our Go program will print out that 9 is smaller than 10.
The else if statement will test a new condition so our first condition evaluated by an if statement fails.
Conditional Statements
Let's talk about conditions. A condition in Go can be true or false.
Go supports these mathematical comparison operators:
- Less than <
- Less than or equal <=
- Greater than >
- Greater than or equal >=
- Equal to ==
- Not equal to !=
Go also supports these logical operators:
- AND &&
So in code, we can write conditions like so:
Ifception (Ifs inside Ifs)
We can even has if statements inside if statements. This is good when we want to evaluate conditions after a previous condition was evaluated. Here's an example:
In this code block, we first evaluate whether or not number1 is bigger than 9. Since this is true, we proceed to our second if statement and test whether our number2 variable is bigger than 19. Since it is, we'll print out that both conditions are true.
As you can see here, if we had multiple conditions our code would get very messy.
In this article, we talked about how we can use if statements and conditionals to control what code gets executed based on certain conditions in Go.
And no, I didn't just write this article to make a crappy pun on Inception. I've never watched the film. Learning control flow and conditional statements are an important milestone in any programming language, so well done you for taking the time to learn how it works in Go!
If you have any questions, feel free to reach out to me on twitter @willvelida and I'll try and help out the best I can.
In my next post, we'll start building Go programs using If, if/else and else/if statements.
Until next time, Happy coding! 🤓🖥️
Top comments (0)
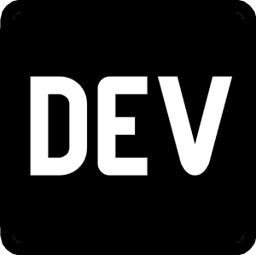
Templates let you quickly answer FAQs or store snippets for re-use.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
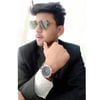
🌤️ Stop Using Margin-Top: Use Margin-Bottom and Gap Instead
Safdar Ali - Aug 29
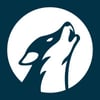
Create A Sports Team Roster From Google Docs Template Using Google Sheets Data
nightwolfdev - Aug 21
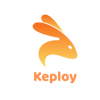
Understanding the Token Bucket Algorithm
keploy - Aug 16

Intro to GoLang Structs
Nerdherd - Aug 29
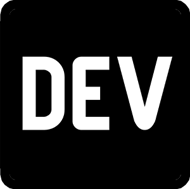
We're a place where coders share, stay up-to-date and grow their careers.
4 basic if-else statement patterns
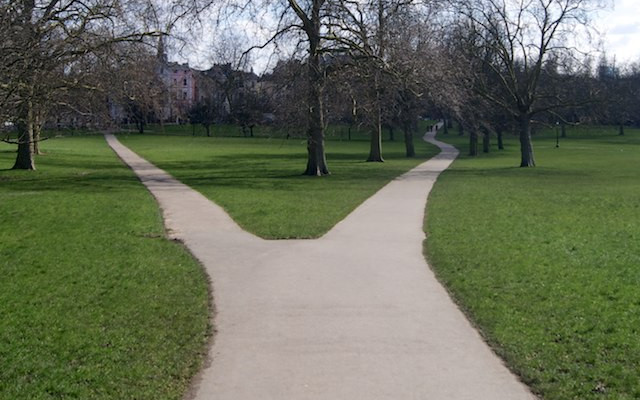
Basic syntax
With init statement, nested if statements, ternary operator alternatives.
An if statement executes one of two branches according to a boolean expression.
- If the expression evaluates to true, the if branch is executed,
- otherwise, if present, the else branch is executed.
The expression may be preceded by a simple statement , which executes before the expression is evaluated. The scope of x is limited to the if statement.
Complicated conditionals are often best expressed in Go with a switch statement . See 5 switch statement patterns for details.
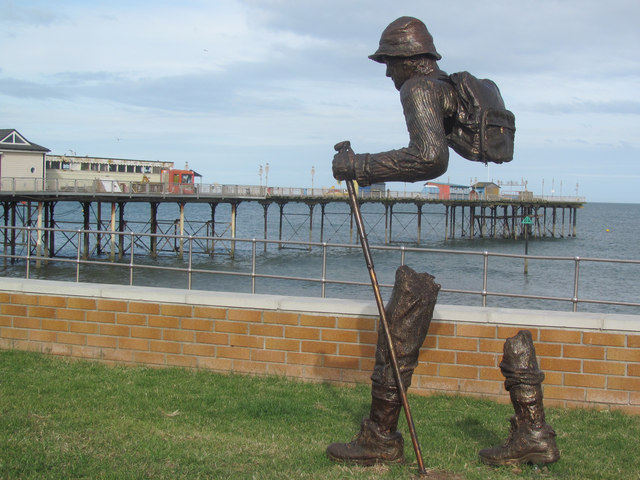
You can’t write a short one-line conditional in Go; there is no ternary conditional operator. Instead of
In some cases, you may want to create a dedicated function.
How to Use Conditions in Go

Your changes have been saved
Email is sent
Email has already been sent
Please verify your email address.
You’ve reached your account maximum for followed topics.
Conditional statements allow you to execute decisions based on logic that evaluates to true or false. Some examples of conditional statements include if statements, if…else, if…else if.. else, and switch-case statements. Go has full support for these conditionals with a familiar and straightforward syntax.
Getting Started With Golang
To follow along with this tutorial, install Go in your local environment if you haven't already. You can also test the code snippets in this article using the Go playground .
If Statement
The if statement executes a block of code only if a certain condition is met. Here is the syntax:
Here is an example that prints “Pass” if the value of the marks variable is more than 50:
You could also declare the marks variable in the if statement like this:
When combining conditions, Go allows you to use logical operators familiar from other languages like AND(&&), OR (||), and NOT(!).
The AND operator returns true only if the value on its right and left are true:
The OR operator returns true if one of the values either on the right or left is true:
The NOT operator returns true only if the value is false:
If…Else Statement
The if…else statement executes the corresponding code depending on whether the condition is met.
In the example below, the program prints ‘Pass’ if the total marks value is above 50 and ‘Fail’ if it’s below.
If…Else If…Else Statement
The if…else if…else statement allows you to combine multiple if statements.
Extending the if…else example, the program below also checks whether the marks are above 80 and prints “Passed with distinction” if so:
Switch Statement
The switch statement is a conditional statement that allows you to execute different actions based on the value of an expression. You can identify significant values as “cases” and act on them accordingly.
The switch statement in Go is slightly different from in other programming languages like C#, JavaScript, and Python. This is because it only executes the block of code under the met case. The break keyword, required by other languages, isn't necessary here:
The above code evaluates the expression after the switch keyword, then compares it to each case value. If a value matches, the following block runs. You can have as many cases as you need, but they must all be unique. The default block runs if there is no match.
The following example uses a switch statement to display a “todo” item for each day of the week.
Here, Go’s time package provides the day of the week as an integer and, depending on that value, the switch statement prints a certain task.
When to Use Conditional Statements
Conditional statements help you create a decision flow in your program. Like many other languages, Go supports several types of conditional statement. You can use them to streamline your program and ensure its logical operation is correct.
Use conditional statements when the flow of your program depends on a certain value such as user input.
- Programming

Go by Example : If/Else
Branching with and in Go is straight-forward. | |
main | |
"fmt" | |
main() { | |
Here’s a basic example. | 7%2 == 0 { fmt.Println("7 is even") } else { fmt.Println("7 is odd") } |
You can have an statement without an else. | 8%4 == 0 { fmt.Println("8 is divisible by 4") } |
Logical operators like and are often useful in conditions. | 8%2 == 0 || 7%2 == 0 { fmt.Println("either 8 or 7 are even") } |
A statement can precede conditionals; any variables declared in this statement are available in the current and all subsequent branches. | num := 9; num < 0 { fmt.Println(num, "is negative") } else if num < 10 { fmt.Println(num, "has 1 digit") } else { fmt.Println(num, "has multiple digits") } } |
Note that you don’t need parentheses around conditions in Go, but that the braces are required. |
go run if-else.go 7 is odd 8 is divisible by 4 either 8 or 7 are even 9 has 1 digit | |
There is no in Go, so you’ll need to use a full statement even for basic conditions. |
Next example: Switch .
by Mark McGranaghan and Eli Bendersky | source | license
Get the Reddit app
Ask questions and post articles about the Go programming language and related tools, events etc.
Idiomatic use of assignment in conditional statements
My coworkers and I have some disagreement about when to use assignment in the conditional when it results in an else clause that could be elided due to early return in the first branch. I wrote something similar to this:
They preferred this style:
Is there a generally-accepted practice in the community? I can find examples of both in popular libraries, and can't say I actually feel strongly one way or the other, but was curious whether there's a generally considered best practice.
By continuing, you agree to our User Agreement and acknowledge that you understand the Privacy Policy .
Enter the 6-digit code from your authenticator app
You’ve set up two-factor authentication for this account.
Enter a 6-digit backup code
Create your username and password.
Reddit is anonymous, so your username is what you’ll go by here. Choose wisely—because once you get a name, you can’t change it.
Reset your password
Enter your email address or username and we’ll send you a link to reset your password
Check your inbox
An email with a link to reset your password was sent to the email address associated with your account
Choose a Reddit account to continue
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
proposal: Go 2: spec: conditional-assign (?=) for limited ternary operator functionality #31659
ugorji commented Apr 24, 2019 • edited Loading
Introduce a operator, to support the limited use-case of a conditional assignment For example: By using commas in the RHS, it limits this syntax to only be usable on assignment to a declared variable and consequently limits the LHS to only have one declared variable. Also, because does not permit variable assignment to declare variables on the RHS, this be abused. .I appreciate the resistance to do a ternary operator (see ), and I have also looked at some proposals and conversations to add it back in some form: (see ) . We all can clearly see the benefit of the ternary operator as seen in ( ) where it translates: n T if expr { n = trueVal } else { n = falseVal }to a 1-liner n = expr ? trueVal : falseValThe drawback is that it permits the creation of incredibly complex expressions as seen in ( ) b1, b2, b3 bool s = b1 || b2 ? b2 && b3 ? b1 && b3 : "s1" : "s2" : "s3": "s4" // impossible to readVERSUS s string if b1 || b2 { if b2 && b3 { if b1 && b3 { s = "s4" } else { s = "s3" } } else { s = "s2" } } else { s = "s1" }Clearly, it's incredibly hard to understand the one-liner in . Its longer version, while many lines, is clearly more readable. I have looked at the one-liner many times, and I don't even know if it is even correct, or if the syntax is correct. Clearly, the one-liner is unreadable, and it was great that it was disallowed in the language. operator, so it can only occur during explicit variable assignment.We know the common use of ternary operators by pragmatic programmers is . What if we can allow that use-case, and just that alone? It could dramatically reduce the number of lines of code. This is what this proposal should permit. ^^ cc'ing var s string = b1 ? "s1" : b2 ? "s2" : b3 ? "s3" : "s4000" // equivalent to if b1 { s = "s1" } else if b2 { s = "s2" } else if b3 { s = "s3" } else { s = "s4000" } |
The text was updated successfully, but these errors were encountered: |
- 👍 10 reactions
- 👎 16 reactions
- 😕 2 reactions
- ❤️ 1 reaction
urandom commented Apr 25, 2019
I'd honestly prefer for the if and switch statements to be turned into expressions instead. There are quite a few mainstream languages that either started with these things as expressions or migrated to them, and the syntax will not be confusing for a lot of people. And something like this would suffice for ternary: And of course, if you have a lot of conditions: |
- 👍 19 reactions
- 👎 6 reactions
- ❤️ 2 reactions
Sorry, something went wrong.
alanfo commented Apr 25, 2019
I like this proposal which is simple, clear, generic, backwards-compatible and would cut down on verbosity whilst avoiding the excesses of the C-style ternary operator. It should also be possible to implement it efficiently in the compiler. In the absence of the ternary operator, I often write a function instead but, lacking generics as well, this isn't a great solution. I wondered at first whether there might be a case for having a operator as well so that the variable could be defined on the same line without the need for . But I think on balance it's best to stick with a single operator and to require the use of in this scenario as type inference can, of course, still be used. TBH I'm not a fan of and doubling up as expressions having used them in this guise in Kotlin (which uses instead of ). They tend to produce rather untidy code as well as blurring the distinction between statements and expressions which I personally dislike. In fact, this is a very heated topic in the Kotlin community half of which would like to see the ternary operator (or some form of it) introduced whereas the other half prefer the status quo. |
- 👍 1 reaction
ntrrg commented Apr 25, 2019 • edited Loading
What about the Python way? := "yes" if true else "no"Some people don't like the sintax |
- 👎 12 reactions
ugorji commented Apr 25, 2019
:= "yes" if true else "no" Some people don't like the sintax This is not about the syntax at all. This is a proposal to introduce a ternary operator. Please re-read the whole proposal. This is a proposal to allow a to a variable, which will accommodate most of the pragmatic use-cases of ternary operator, while bypassing the drawbacks. If the syntax is available as part of an expression, then it can be misused. |
And something like this would suffice for ternary: And of course, if you have a lot of conditions: if/switch expressions do not help eliminate the drawbacks. By definition, an expression can be nested, so the drawbacks are there. It is just a different syntax for the ternary operator, with all the drawbacks it has when used as part of an expression. The reason this proposal can work, is that the operator cannot be part of an expression, as it is an , like or , which MUST have exactly one declared variable on the LHS. |
- 👍 2 reactions
josharian commented Apr 25, 2019
This is necessary to avoid ambiguity with the syntax you chose, but it is a bit unfortunate. Consider (using the python syntax for clarity only):
The restriction that does the work “to avoid abuse” is that of being a statement instead of an expression, not of having a single variable on the LHS. Some other questions and observations. Can you declare variables this way, using ? Other syntax in Go like modifies the LHS ( , , etc.). That makes this a bit of an anomaly. |
Sorry, I didn't mean you were talking about including the ternary operator, I just was saying that writing: s ?= boolean_expr, ifTrueVal, ifFalseValCould be easier to read if you write: s ?= ifTrueVal if boolean_expr else ifFalseValIf you see is like writing a |
This is necessary to avoid ambiguity with the syntax you chose, but it is a bit unfortunate. Consider (using the python syntax for clarity only):
The restriction that does the work “to avoid abuse” is that of being a statement instead of an expression, not of having a single variable on the LHS. Some other questions and observations. Can you declare variables this way, using ? Other syntax in Go like modifies the LHS ( , , etc.). That makes this a bit of an anomaly. Right. I tried to avoid using expression vs statement because many folks get confused. So I wanted to use lay terms ie RHS LHS that anyone understands. I see just like I see . Within , the spec makes clear that op= works when op is an arithmetic operator. and both work in this context, because neither nor is an arithmetic operator, so there's no ambiguity/confusion. It becomes a singular multi-byte operator, just like or or or . And there's no ambiguity, and there's precedence in this specific limited case of "richer assignment" like does. Remember: there can only be one variable on the LHS. The syntax is really only applicable for: Given that already prevents if/switch expressions, the example you suggested is not possible in go in any syntax, and so doesn't apply. |
- 😕 1 reaction
if boolean_expr else ifFalseVal s ?= boolean_expr, ifTrueVal, ifFalseVal Could be easier to read if you write: s ?= ifTrueVal if boolean_expr else ifFalseValIf you see is like writing a Key message in the proposal is that this is an operator that doesn't fit into an expression at all, because once it does, it can be misused. See example of misuse: s ?= trueVal1 if boolean_expr_1 else trueVal2 if boolean_expr_2 else trueVal3 if boolean_expr_3 else falseValLooking at above, I don't even know if the syntax is correct. The compiler might let me know. And this is why folks do not like the ternary operator when nesting is allowed. By using commas, there cannot be any nesting. The operator is strictly attached to assignment to exactly one variable. It's like a function call that does: ternary(b bool, s1 string, s2 string) string { if b { return s1 } else { return s2 } } var s = ternary(boolean_expr, trueVal, falseVal)This is the limited scope that this proposal allows. |
nigeltao commented Apr 26, 2019
I'm still undecided whether or not I like the proposal, but as for 's proposed syntax: This doesn't necessitate having if/switch . Instead, this could be a peculiar form of : an assign-if or assign-switch. Essentially, or is the atomic syntactic concept, and the curlies and afterwards are basically ceremony that makes the whole thing look familiar, similar to (but different than) the existing if . For example, this would allow (and we could possibly also allow ) but this would still prohibit . It would definitely prohibit the abuse of nested ternaries. Note that the true/false arms of an assign-if would have to be expressions, not the statement lists you can have in an if statement's arms. The could be optional, implying that is the zero value of . OTOH that could be confusing if people read to mean a no-op if is false. Saying instead of as per the original proposal also lets us allow assign-switch, as already noted. This would be similar to allowing (but not mandating) 1-liner functions. Both of these are acceptible to gofmt: |
- 👍 8 reactions
beoran commented Apr 26, 2019
Another, slightly more general way to have something similar would be to introduce the if modifier at the end of a statement, much like in Ruby. An if at the end of a statement is executed only when the if condition is true. This would be backwards compatible as well. So: FooOrBar(isBar bool) { var a string a = "bar" if a = "foo" ; isBar return a }would then be syntactic sugar for this: FooOrBar(isBar bool) { var a string if a = "foo" ; isBar { a = "bar" } return a }Well, just a wild idea. |
alanfo commented Apr 26, 2019
Well there may be different ways of looking at this but the fact remains that, as soon as you try to use if/else and switch in this fashion, various difficulties present themselves and it defeats the basic purpose of this proposal (to express simple conditionals as one liners in a Go-like way). Frankly I'd rather things were left as they are than reuse the conditional keywords in this way. For me the beauty of this proposal is that it addresses what is clearly a pain-point for many people (you only have to look at discussions on golang-nuts to see that this is so) and does so in a simple way which avoids the main objection to having a 'standard' ternary operator in the first place. It's not as if this is a rare scenario - it comes up all the time. Personally, I don't see it as a drawback that you can't assign values to more than one variable at a time with this proposal as, apart from simple swaps, I regard assignments where the variables refer to each other on the RHS as potentially confusing any way. |
ugorji commented Apr 26, 2019
wrote: This doesn't necessitate having if/switch . Instead, this could be a peculiar form of : an assign-if or assign-switch. Essentially, or is the atomic syntactic concept, and the curlies and afterwards are basically ceremony that makes the whole thing look familiar, similar to (but different than) the existing if . I actually do like this, and I will be fine if this is an accepted substitute. I like how it can allow for multiple assignments. However, when I write them side by side, the ?= looks simpler. See: = if valueIsOk { "abc" } else { "def" } a ?= valueIsOK, "abc", "def"There is also more possible confusion with like: does go now support if expressions, why can't I use it in if expressions fully, why can't i use the construct outside of assignment, etc. E.g. why not? = if valueIs1 { "a1" } else if valueIs2 { "b2" } else { "b3" } a = if valueIs1 { "a1" } else { if valueIs2 { "b2" } else { "b3" } } func doSomething(s string) { ... } doSomething(if valueIs1 { "a1"} else { "b2" })Finally, I also love that go is about orthogonality. I agree that the would not be orthogonal, as we will be overloading the if keyword and statement and restricting where it's full power can be used. We typically mostly do this for built-in functions e.g. make, new, etc. However, as an operator, it is still kinda orthogonal, as it is just fulfilling a missing feature available in other languages, like , , etc and acting as syntactic sugar. i.e. valueIsOk boolean var s ?= valueIsOk ? "s1" : "s2" // is just syntactic sugar for var s string if valueIsOk { s = "s1" } else { s = "s2" } |
josharian commented Apr 26, 2019
I like your syntax; I share your uncertainty about whether I like the proposal. FWIW, here are a few interesting cases. Comma, ok variant: m map[string]int x, ok := if b { m["abc"] } else { m["def"] }containing a statement: := if c := f(); c != 0 { ... } else { ... }Using the results of a function call returning multiple values: f() (int, int) x, y := if b { 0, 0 } else { f() }It seems like all of these are things we would probably want to permit, and the semantics for them seem reasonably clear. |
randall77 commented Apr 26, 2019
This proposal doesn't mention anything about short-circuiting (ala and ). Are and evaluated unconditionally, or are they only evaluated based on the result of evaluating ? In the C and Java ternary operators, at least, it is the latter. This has a large impact on the syntax; the syntax should be consistent with the answer. When I read , it looks like , , and are all unconditionally evaluated (as they are in a multiassignment). On the other side, Implies that only one of or is evaluated. |
- 👍 5 reactions
josharian commented Apr 26, 2019 • edited Loading
Another interesting case: mixed type inference. := if b { 0 } else { byte(1) }Presumably, has type , even though the default type for the constant is . I don't think there are any cases in which we currently do dual/paired type inference. (And if we allow switches, n-ary type inference.) EDIT: Even more interesting is := if b { 0 } else { 1.5 } |
Regarding 's point, I think the proposed operator should definitely short-circuit. This is the more efficient option and is what people will expect. I don't see why there is a consistency problem with: as it's not a multi-assignment - there's only one variable on the LHS. Turning now to 's examples, I think that type inference should take place from left to right and so neither example should compile. In both cases is inferred to be an and so can't be assigned a or value. Of course, if x had already been declared to be a or variable, then there would be no problem as assigning to it would then be allowed. |
randall77 commented Apr 27, 2019
Yes, but on the RHS there's no visual distinction between the three values. They are just three comma-separated expressions, just like the RHS of a multiassignment or the args of a function. So in , we infer the type of from the type of , and then check that is assignable to that type? That would work. It's a little bit unsatisfying that it isn't symmetrical in and . It might cause people to invert the condition unnecessarily to get the type they want inferred into the slot. But that's a relatively small objection. |
josharian commented Apr 27, 2019
An alternative, which fits a little better with Go's preference for explicitness, is to typecheck both halves independently, and fail if they are different. That will also make the job of refactoring (automated or not) easier. Among other things, it means that it is sound to invert the condition and flip the slots. |
alanfo commented Apr 27, 2019 • edited Loading
, OK, I understand the point you're making and, if a succinct way can be found to achieve that, I'd have no problem with it. The way you've suggested should work and another possibility (borrowing some syntax from the ternary operator itself) would be: |
, I agree that it would be preferable to look at the 'true' and 'false' values independently as there would then be no distinction in the programmer's mind between assigning to a new implicitly typed variable, to a new explicitly typed variable or to an existing variable. However, the problem is that when untyped numeric literals are used (which would be commonplace if this proposal, or something like it, were accepted) the 'true' and 'false' values aren't necessarily independent and the compiler might therefore need to examine the matter from both perspectives when performing type inference to see whether a 'matching type' existed. So, in the examples you cited earlier, there would be no match from the 'true' value's perspective but there would be a match from the 'false' value's perspective. So I think there would be more work for the compiler compared to what I suggested earlier, though it should still be doable. |
Typechecking is cheap. |
alanfo commented Apr 28, 2019
Whilst that's true when you only have a single expression, as you said yourself in an earlier post this would be the first time that dual type inference were needed. However, if generic functions are eventually introduced on something like the lines of the draft design paper, then you'd have exactly the same problem there - except worse as you could have any number of parameters to deal with. For example, if one introduced a generic function to replace the ternary operator, like so: iff(type T) (cond bool, trueValue, falseValue T) T { if cond { return trueValue } return falseValue }then it would be disappointing if one couldn't write this: := iff(b, 0, 1.5)where is inferred to be a float64 variable. Incidentally, I'm not advocating the use of such a function instead of this proposal as the former would suffer from the dual problems of permitting nesting of 'iff's and (unless it was a built-in) there would be no short-circuiting - all parameters would need to be evaluated. However, it's something I would consider doing for my own code rather than having to write multiple lines for simple conditionals. |
stevef1uk commented Apr 28, 2019
I would also like to see a ternary operator in Go. I am writing some code where the structure I am processing may or may not have been initialised e.g. tmp_Tsimple_14 := &Simples{ If the fields within params.Body have not been set I obviously get runtime errors. I would like to be able to generate code such that I can use a ternary condition to ensure I can pass the field e.g. tmp_Tsimple_14 := &Simples{ |
bcmills commented Apr 29, 2019
That seems like more of an issue for than for the language spec itself: is free to add separating newlines as appropriate. |
networkimprov commented Apr 29, 2019
Data point; I use: Sadly that always evaluates and copies . I would love to see an efficient way to do this. (I ban in my projects for this and other reasons.) is on record that (paraphrased) "Go does not allow control flow in expressions." But in fact, it does, as and may not evaluate the second operand. |
josharian commented Apr 29, 2019
One important question for all language change proposals is: What can you do significantly more easily if the proposal is adopted? There is one case in which this syntax accomplishes something more than saving a line or two of code: initializing global variables. Consider this at the top level: v = if b { x } else { y }Currently this initialization requires an function. In addition to the reduced verbosity, it would be easier for the compiler to convert this to static data in cases in which can be evaluated to a constant. (We currently make no effort to understand the contents of an function, and I don't see that changing.) |
ianlancetaylor commented Apr 29, 2019
I'm not really in favor of this proposal, but I don't see type inferencing as a problem. It's not fundamentally different from cases like := 1 + 2 b := int32(1) + 2 c := 1 + int64(2)In these cases we need to infer the type of at least one of the values on the right hand side, and we know how to do that. For := if b { 0 } else { byte(1) }the type of is , just as it is for := 0 + byte(1)For := if b { 0 } else { 1.5 }the type of is , just as it is for := 0 + 1.5 |
- 👍 6 reactions
nigeltao commented Apr 30, 2019
Once again, I haven't decided on whether I like this (or any suggestion above), but for the record, here's a comment that a colleague made, off-list... If you dislike ternaries because of nesting, you could at least require parentheses to help accentuate the nesting. Alternatively, you could require that the to be a 'simple' expression, for some definition of 'simple'. Even if you have nesting, it'd always read left-to-right, following the false arms: |
ugorji commented Apr 30, 2019
wrote ... to be a 'simple' expression, for some definition of 'simple'. Even if you have nesting, it'd always read left-to-right, following the false arms:This is ... not bad at all. Maybe etc can comment on whether they find this acceptable. It definitely seems to resolve the common complaint with ternary operators. |
wrote ... to be a 'simple' expression, for some definition of 'simple'. Even if you have nesting, it'd always read left-to-right, following the false arms: This is ... not bad at all. Maybe etc can comment on whether they find this acceptable. It definitely seems to resolve the common complaint with ternary operators. This is really nice, because by limiting this to a expression i.e. one without a nested conditional, then this reads just as simply as a if/(else-if/)*else block (with no nesting). Really nice! is equivalent to OR This gives ternary operators usable in multiple contexts (not just assignments) but clearly legible and with none of the drawbacks that the go designers were concerned about when they left it out. I would love to hear what the go team thinks about this. Thanks for highlighting this idea . I really like it, way more than my original proposal. |
nigeltao commented May 1, 2019
Yet another syntax idea (and not necessarily a good idea) for the pot... If you wanted to discriminate between an and an , the latter could require parentheses: |
- 👎 3 reactions
deanveloper commented May 7, 2019
What designates a "simple" expression? Nested statements are not the only things that make ternary operators unreadable: |
urandom commented May 7, 2019
That actually looks quite readable (the ternary), definitely on the level of the actual if block |
I personally don't find it very readable, but I guess readability is subjective and the expression isn't complex. |
ugorji commented May 7, 2019
Yea, I find it readable too. Once your eyes get used to scanning for the ? and :, it became easy for me to read, or at least just as readable as the long form. |
networkimprov commented May 7, 2019
In JS I add a line break for convoluted ternaries |
ianlancetaylor commented Jun 4, 2019
One way to possibly address the syntactic and readability issues would be to use a builtin function. For example, Because it is a builtin function, it could have the lazy evaluation of the true and false values that we want (only one of the true/false values is evaluated, depending on the condition's value). On the other hand, it would be the only builtin function with lazy evaluation (which is also why we could not implement this function using generics, if we had generics). |
alanfo commented Jun 5, 2019 • edited Loading
For me that would be a perfect solution. We'd have the brevity and efficiency of the ternary operator but in a more readable form. I think people would be less likely to pass nested expressions to a function than to the ternary operator itself but, even if they did, it should still be readable because the nested 's would stand out in an editor or IDE using syntax highlighting. I'm not sure whether would be the best name for it as it's quite commonly used as a bool variable or parameter in my experience. Other possibilities would be (or ) which is common in BASIC-like languages or perhaps as an easy reminder of what it does. So, yeah, bring it on and soon :) |
MrTravisB commented Jun 5, 2019
One of the biggest frustrations with Go is the verbosity and how much boilerplate you have to write over and over again. Error checking (which is being addressed in some way) and generics (also eventually being addressed) will go a long way to helping this. Arrow functions and ternary operator would be equally as helpful. I understand the drawbacks of ternary operators. I have worked in PHP, Ruby, Python and Javascript a lot and have seen some atrocious ternary statements. But at some point we need to let people decide how they want to write their code. Individual projects should be able to set their own standards for how to write code. Go already has features which, in my opinion, when used make the code more difficult to read. Named return parameters and naked return statements being the biggest culprits. Defer statements a close second. If Go allowed configuration of configuration of golint and gofmt, it could allow individual projects to decide if they wanted to allow ternary operators (or named return params, naked returns, etc). Personally I prefer the Python ternary over the more common ternary |
- 👍 3 reactions
ugorji commented Jun 6, 2019
Because it is a builtin function, it could have the lazy evaluation of the true and false values that we want (only one of the true/false values is evaluated, depending on the condition's value). On the other hand, it would be the only builtin function with lazy evaluation (which is also why we could not implement this function using generics, if we had generics). The concern with the function call is that it affords nesting, which is a major concern that the go team identified with the ternary operator. |
networkimprov commented Jun 6, 2019 • edited Loading
Having a function which doesn't always evaluate all arguments would be surprising. |
alanfo commented Jun 6, 2019
Although the risk would still be there, I think it would be much lower in the case of the function because you don't often see very complicated expressions passed as arguments to functions - they tend to be assigned to intermediate variables first to make the code more readable. It would be surprising if it were a normal function but it will be a built-in and these often have features (for example function overloading) which are not found in the normal language. There would, of course, be three arguments passed to the function including the bool condition so at least two would be evaluated which is the same as the ternary operator itself. |
ugorji commented Jun 14, 2019
On further thought, I like the function with the caveat that it cannot embed other . |
beoran commented Jun 14, 2019 via email
. |
ianlancetaylor commented Jul 9, 2019
Thanks for the suggestion. This proposal has some minor issues mentioned above. Paraphrasing ( ), the new functionality is not particularly orthogonal. It is a new kind of declaration, but although it provides general functionality doesn't extend past declarations. Paraphrasing ( ), the statement is presumably intended to short-circuit in that the unselected branch is not evaluated, but the syntax does not indicate that in any clear way. The syntax is also unusual: currently Go only has comma separated expressions in function calls and composite literals, in which each element of the list becomes part of some value; here the comma separated list has a different purpose. Setting those minor issues aside, the benefit of this proposal is not high. You can already write this kind of code in just a few lines. Even initializing a top level variable can be done using an function. While the cases where this syntax would help clearly do exist, it's not obvious that they are common enough to justify new syntax. The lack of a conditional ternary operator in Go comes up from time to time but it is not a common complaint. And the people who do make the complaint most likely want the general operator, not a specific initialization syntax. Writing for @golang/proposal-review . |
No branches or pull requests
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Multiple assignment by if statement
It is possible to execute multiple assignment by if condition, like the following code?
- 3 Doesn't that look a little hard to decipher ? – Denys Séguret Commented Aug 13, 2014 at 9:23
- 3 I don't think so. Your code is a bit hard to read, too. Maybe you want to split it up into multiple if-statements. – fuz Commented Aug 13, 2014 at 9:24
- ok, I think it would be better for read too. – softshipper Commented Aug 13, 2014 at 9:24
2 Answers 2
It looks like you want something like this:
No. Only one 'simple statement' is permitted at the beginning of an if-statement, per the spec .
The recommended approach is multiple tests which might return an error, so I think you want something like:
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged go or ask your own question .
- The Overflow Blog
- At scale, anything that could fail definitely will
- Featured on Meta
- Announcing a change to the data-dump process
- Bringing clarity to status tag usage on meta sites
- What does a new user need in a homepage experience on Stack Overflow?
- Feedback requested: How do you use tag hover descriptions for curating and do...
- Staging Ground Reviewer Motivation
Hot Network Questions
- Definition clarification on topology regarding closed set
- Doesn't counting hole and electron current lead to double-counting of actual current?
- How to run only selected lines of a shell script?
- Does an airplane fly less or more efficiently after a mid-flight engine failure?
- What does "if you ever get up this way" mean?
- quantulum abest, quo minus . .
- Is loss of availability automatically a security incident?
- Lore reasons for being faithless
- How to resolve hostname by mDNS?
- A story where SETI finds a signal but it's just a boring philosophical treatise
- Multiple alien species on Earth at the same time: one species destroys Earth but the other preserves a small group of humans
- Could an empire rise by economic power?
- What is the significance of the phrase " in the name of the Lord " as in Psalm 124:8?
- Does an unseen creature with a burrow speed have advantage when attacking from underground?
- Uniqueness of Neumann series
- Is it safe to install programs other than with a distro's package manager?
- How do I keep my tikz drawing on the page?
- How would humans actually colonize mars?
- How can I find Mean[data] Median[data] Quartiles[data] Variance[data] StandardDeviation[data] of a grouped frequency table
- Why is the stall speed of an aircraft a specific speed?
- Is it possible to travel to USA with legal cannabis?
- How should I secure ceiling drywall with no edge backing?
- How best to cut (slightly) varying size notches in long piece of trim
- Can Christian Saudi Nationals Visit Mecca?

IMAGES
VIDEO
COMMENTS
The C conditional expression (commonly known as the ternary operator) has three operands: expr1 ? expr2 : expr3. If expr1 evaluates to true, expr2 is evaluated and is the result of the expression. Otherwise, expr3 is evaluated and provided as the result. This is from the ANSI C Programming Language section 2.11 by K&R.
12. One possible way to do this in just one line by using a map, simple I am checking whether a > b if it is true I am assigning c to a otherwise b. c := map[bool]int{true: a, false: b}[a > b] However, this looks amazing but in some cases it might NOT be the perfect solution because of evaluation order.
If Statements. We will start with the if statement, which will evaluate whether a statement is true or false, and run code only in the case that the statement is true. In a plain text editor, open a file and write the following code: import "fmt" func main() {. grade := 70 if grade >= 65 {.
If a statement is used to specify a block of code that should be executed if a certain condition is true. Syntax. go. if condition {. code to execute. } Explanation. In the above syntax, the keyword if is used to declare the beginning of an if statement, followed by a condition that is being tested. The condition should be a value of boolean ...
Usually the If/else/else if condition when written with one condition makes the program lengthy and increases the complexity thus we can combine two conditions. The conditions can be combined in the following ways :-. Using && (AND) Operator. The And (&&) is a way of combining conditional statement has the following syntax:
The ternary operator is the ?: operator used for the conditional value. Here is an example of how one would use it in code. 1. v = f > 0 ? 1 : 0 // if f > 0 then v is 1 else v is 0. This operator is absent in Golang.
Welcome to tutorial number 8 of our Golang tutorial series.. if statement has a condition and it executes a block of code if that condition evaluates to true.It executes an alternate else block if the condition evaluates to false. In this tutorial we will look at the various syntaxes for using a if statement.. If statement syntax
In short, the ternary operator known from languages like PHP or JavaScript is not available in Go. But you can express the same condition using the if {..} else {..} block, which is longer but easier to understand. The fact that it is not in the syntax is the result of a deliberate choice by the language designers, who noticed that the ternary ...
In Go, we can use conditional statements to execute code based on different conditions. We can also use if, else if and if else statements to execute code provided if a condition is true or false. If/else statements are commonplace in programming, so if you're already a seasoned programmer you won't spend long here.
Introduction. Conditional statements give programmers the ability to direct their programs to take some action if a condition is true and another action if the condition is false. Frequently, we want to compare some variable against multiple possible values, taking different actions in each circumstance. It's possible to make this work using if statements alone.
12. From Unsplash donio3d. If you were like me, a pure Java developer before writing Go, you must be wondering why Go doesn't support the ternary operator like return a > 1 ? 0 : 1. Most mainstream languages like C and Java are supportive of ternary operators; languages like Python and Ruby support the simplified if-else one-liner, such as a ...
The if/else conditional statement. Go provides if, if-else, if-else if-else variants of if/else statement we are familiar with. It is used to check a condition, and execute some code when the ...
Basic syntax; With init statement; Nested if statements; Ternary ? operator alternatives; Basic syntax if x > max {x = max } if x = y {min = x } else {min = y }. An if statement executes one of two branches according to a boolean expression.. If the expression evaluates to true, the if branch is executed,; otherwise, if present, the else branch is executed.; With init statement
Here is the syntax: Here is an example that prints "Pass" if the value of the marks variable is more than 50: fmt.Println( "Pass") You could also declare the marks variable in the if statement like this: fmt.Println( "Pass") When combining conditions, Go allows you to use logical operators familiar from other languages like AND (&&), OR ...
Go by Example. : If/Else. Branching with if and else in Go is straight-forward. Here's a basic example. You can have an if statement without an else. Logical operators like && and || are often useful in conditions. A statement can precede conditionals; any variables declared in this statement are available in the current and all subsequent ...
If with a short statement. Like for, the if statement can start with a short statement to execute before the condition. Variables declared by the statement are only in scope until the end of the if. (Try using v in the last return statement.) < 6/14 >. if-with-a-short-statement.go Syntax Imports. 21.
If you're still set on assignment in the conditional (e.g., if you've declared err previously above) then: ... So, it helps to know how Golang scopes work. An if statement would create a block scoped variable, only accessible from within the statements block. If you had to do something with "fp" in your example you couldn't unless it ...
Then you can also declare methods for NormalResult which will be promoted to AdminResult as well: func (r *NormalResult) doSomething() {. // Doing something. } Edit. And, no, it is not possible to have conditional types in Go as you suggested. A variable can only be of one type, be it NormalResult, AdminResult or interface{}
Some people don't like the ? : sintax. This is not about the ? : syntax at all. This is NOT a proposal to introduce a ternary operator. Please re-read the whole proposal. This is a proposal to allow a conditional assignment to a declared variable, which will accommodate most of the pragmatic use-cases of ternary operator, while bypassing the drawbacks.. If the syntax is available as part of an ...
done = true. } else {. select {. case value := <- somechannel: // Do something with value. default: done = true. That is, if nothing is pending on the channel, and I haven't set a timeout, I exit. If a timeout is set and nothing is available, then I wait for either the timeout or for something to be available on the channel.
No. Only one 'simple statement' is permitted at the beginning of an if-statement, per the spec. The recommended approach is multiple tests which might return an error, so I think you want something like: genUri := buildUri() if err := setRedisIdentity(genUri, email); err != nil {. return "", err.