404 Not found

Assignment Statements - Intermediate Code Generation, Computer Science and IT Engineering - Computer Science Engineering (CSE) PDF Download
ASSIGNMENT STATEMENTS
Suppose that the context in which an assignment appears is given by the following grammar.
P->M D
M->ɛ
D->D ; D | id: T | proc id; N D ; S
N->ɛ
Nonterminal P becomes the new start symbol when these productions are added to those in the tran slation scheme shown below.
Translation scheme to produce three-address code for assignments
S->id : = E { p : = lookup ( id.name); if p ≠ nil then
emit( p ‘ : =’ E.place) else error }
E->E1 + E2 { E.place : = newtemp;
emit( E.place ‘: =’ E1.place ‘ + ‘ E2.place ) }
E->E1 * E2 { E.place : = newtemp;
emit( E.place ‘: =’ E1.place ‘ * ‘ E2.place ) }
E->-E1 { E.place : = newtemp;
emit ( E.place ‘: =’ ‘uminus’ E1.place ) }
E-> ( E1) { E.place : = E1.place }
E->id { p : = lookup ( id.name); if p ≠ nil then
E.place : = p else error }
Reusing Temporary Names
The temporaries used to hold intermediate values in expression calculations tend to clutter up the symbol table, and space has to be allocated to hold their values. Temporaries can be reused by changing newtemp. The code generated by the rules for E E1 + E2 has the general form:
evaluate E1 into t1
evaluate E2 into t2
t : = t1 + t2
- The lifetimes of these temporaries are nested like matching pairs of balanced parentheses.
- Keep a count c , initialized to zero. Whenever a temporary name is used as an operand, decrement c by 1. Whenever a new temporary name is generated, use $c and increase c by 1.
- For example, consider the assignment x := a * b + c * d - e * f
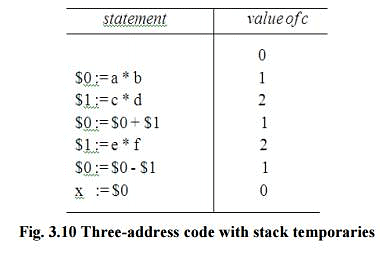
Addressing Array Elements:
Elements of an array can be accessed quickly if the elements are stored in a block of consecutive locations. If the width of each array element is w, then the ith element of array A begins in location
base + ( i - low ) x w
where low is the lower bound on the subscript and base is the relative address of the storage allocated for the array. That is, base is the relative address of A[low].
The expression can be partially evaluated at compile time if it is rewritten
ixw+ (base-low x w)
The subexpression c = base - low x w can be evaluated when the declaration of the array is seen. We assume that c is saved in the symbol table entry for A , so the relative address of A[i] is obtained by simply adding i x w to c.
Address calculation of multi-dimensional arrays:
A two-dimensional array is stored in of the two forms :
Row-major (row-by-row)
Column-major (column-by-column)
In the case of row-major form, the relative address of A[ i1 , i2] can be calculated by the formula base + ((i1 - low1) x n2 + i2 - low2) x w
where, low1 and low2 are the lower bounds on the values of i1 and i2 and n2 is the number of values that i2 can take. That is, if high2 is the upper bound on the value of i2, then n2 = high2 - low2 + 1.
Assuming that i1 and i2 are the only values that are known at compile time, we can rewrite the above expression as
(( i1 x n2 ) + i2 ) x w + ( base - (( low1 x n2 ) + low2 ) x w)
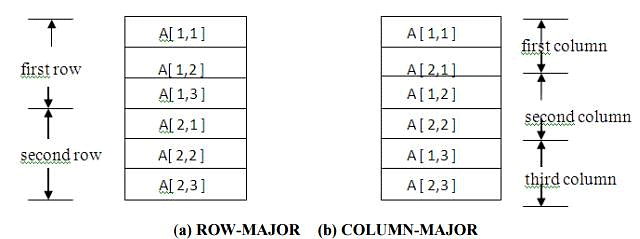
Fig. 3.11 Layouts for a 2 x 3 array
Generalized formula:
The expression generalizes to the following expression for the relative address of A[i1,i2,…,ik] (( . . . (( i1n2 + i2 ) n3 + i3) . . . ) nk + ik ) x w + base - (( . . .((low1n2 + low2)n3 + low3) . . .) nk + lowk) x w
for all j, nj = highj - lowj + 1
The Translation Scheme for Addressing Array Elements :
Semantic actions will be added to the grammar :
(1) S-> L : = E
(2) E-> E + E
(3) E-> (E)
(4) E-> L
(5) L-> Elist ]
(6) L-> id
(7) Elist-> Elist , E
(8) Elist-> id [ E
We generate a normal assignment if L is a simple name, and an indexed assignment into the location denoted by L otherwise :
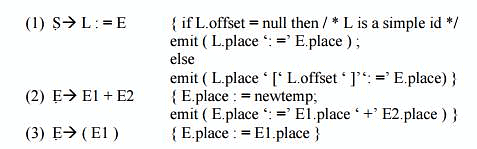
When an array reference L is reduced to E , we want the r-value of L. Therefore we use indexing to obtain the contents of the location L.place [ L.offset ] :
(4) E-> L { if L.offset = null then /* L is a simple id* / E.place : = L.place
E.place : = newtemp;
emit ( E.place ‘: =’ L.place ‘ [‘ L.offset ‘]’) end }
(5) L-> Elist ] { L.place : = newtemp;
L.offset : = newtemp;
emit (L.place ‘: =’ c( Elist.array ));
(6) L-> id emit (L.offset ‘: =’ Elist.place ‘*’ width (Elist.array)) }
{ L.place := id.place;
L.offset := null }
(7) Elist-> Elist1 , E { t := newtemp;
m : = Elist1.ndim + 1;
emit ( t ‘: =’ Elist1.place ‘*’ limit (Elist1.array,m)); emit ( t ‘: =’ t ‘+’ E.place);
Elist.array : = Elist1.array;
Elist.place : = t;
(8) Elist-> id [ E Elist.ndim : = m }
{ Elist.array : = id.place;
Elist.place : = E.place; Elist.ndim : = 1 }
Type conversion within Assignments :
Consider the grammar for assignment statements as above, but suppose there are two types - real and integer , with integers converted to reals when necessary. We have another attribute E.type, whose value is either real or integer. The semantic rule for E.type associated with the production
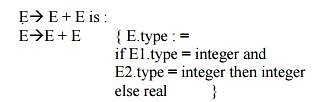
The entire semantic rule for E = E+E ans most o the other productions must modified to generate, when necessary, three-address statements of the form x : = inttoreal y, whose effect is to convert integer y to a real of equal value, called x.
Semantic action for E-> E1+E2
E.place := newtemp;
if E1.type = integer and E2.type = integer then begin emit( E.place ‘: =’ E1.place ‘int +’ E2.place);
E.type : = integer
else if E1.type = real and E2.type = real then begin
emit( E.place ‘: =’ E1.place ‘real +’ E2.place);
E.type : = real
else if E1.type = integer and E2.type = real then begin
u : = newtemp;
emit( u ‘: =’ ‘inttoreal’ E1.place);
emit( E.place ‘: =’ u ‘ real +’ E2.place); E.type : = real end
else if E1.type = real and E2.type =integer then begin
emit( u ‘: =’ ‘inttoreal’ E2.place);
emit( E.place ‘: =’ E1.place ‘ real +’ u); E.type : = real end
E.type : = type_error;
For example, for the input x : = y + i * j
assuming x and y have type real, and i and j have type integer, the output would look like
t1 : = i int* j
t3 : = inttoreal t1
t2 : = y real+ t3
x : = t2
Top Courses for Computer Science Engineering (CSE)
Faqs on assignment statements - intermediate code generation, computer science and it engineering - computer science engineering (cse), mock tests for examination, practice quizzes, previous year questions with solutions, assignment statements - intermediate code generation, computer science and it engineering - computer science engineering (cse), past year papers, objective type questions, study material, extra questions, important questions, viva questions, video lectures, sample paper, semester notes, shortcuts and tricks.
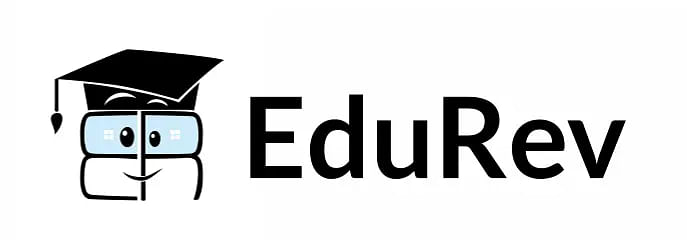
Assignment Statements - Intermediate Code Generation, Computer Science and IT Engineering Free PDF Download
Importance of assignment statements - intermediate code generation, computer science and it engineering, assignment statements - intermediate code generation, computer science and it engineering notes, assignment statements - intermediate code generation, computer science and it engineering computer science engineering (cse) questions, study assignment statements - intermediate code generation, computer science and it engineering on the app, welcome back, create your account for free.

Forgot Password
Unattempted tests, change country, practice & revise.
- Engineering Mathematics
- Discrete Mathematics
- Operating System
- Computer Networks
- Digital Logic and Design
- C Programming
- Data Structures
- Theory of Computation
- Compiler Design
- Computer Org and Architecture
- Compiler Design Tutorial
Introduction
- Introduction of Compiler Design
- Compiler construction tools
- Phases of a Compiler
- Symbol Table in Compiler
- Error detection and Recovery in Compiler
- Error Handling in Compiler Design
- Language Processors: Assembler, Compiler and Interpreter
- Generation of Programming Languages
Lexical Analysis
- Introduction of Lexical Analysis
- Flex (Fast Lexical Analyzer Generator )
- Introduction of Finite Automata
- Ambiguous Grammar
Syntax Analysis
- Introduction to Syntax Analysis in Compiler Design
- Why FIRST and FOLLOW in Compiler Design?
- FIRST Set in Syntax Analysis
- FOLLOW Set in Syntax Analysis
- Classification of Context Free Grammars
- Parsing | Set 1 (Introduction, Ambiguity and Parsers)
- Classification of Top Down Parsers
- Bottom-up or Shift Reduce Parsers | Set 2
- Shift Reduce Parser in Compiler
- SLR Parser (with Examples)
- CLR Parser (with Examples)
- Construction of LL(1) Parsing Table
- LALR Parser (with Examples)
Syntax Directed Translation
- Syntax Directed Translation in Compiler Design
- S - attributed and L - attributed SDTs in Syntax directed translation
- Parse Tree in Compiler Design
- Parse Tree and Syntax Tree
- Code Generation and Optimization
- Code Optimization in Compiler Design
Intermediate Code Generation in Compiler Design
- Issues in the design of a code generator
- Three address code in Compiler
- Data flow analysis in Compiler
- Compiler Design | Detection of a Loop in Three Address Code
- Introduction of Object Code in Compiler Design
Runtime Environments
- Static and Dynamic Scoping
- Runtime Environments in Compiler Design
- Loader in C/C++
Compiler Design LMN
- Last Minute Notes - Compiler Design
Compiler Design GATE PYQ's and MCQs
- Lexical analysis
- Parsing and Syntax directed translation
- Compiler Design - GATE CSE Previous Year Questions
In the analysis-synthesis model of a compiler, the front end of a compiler translates a source program into an independent intermediate code, then the back end of the compiler uses this intermediate code to generate the target code (which can be understood by the machine). The benefits of using machine-independent intermediate code are:
- Because of the machine-independent intermediate code, portability will be enhanced. For ex, suppose, if a compiler translates the source language to its target machine language without having the option for generating intermediate code, then for each new machine, a full native compiler is required. Because, obviously, there were some modifications in the compiler itself according to the machine specifications.
- Retargeting is facilitated.
- It is easier to apply source code modification to improve the performance of source code by optimizing the intermediate code.
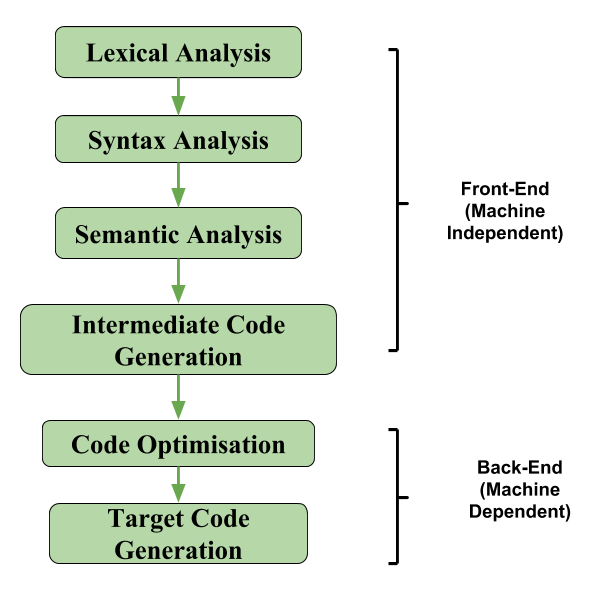
- Also known as reverse Polish notation or suffix notation.
- In the infix notation, the operator is placed between operands, e.g., a + b. Postfix notation positions the operator at the right end, as in ab + .
- For any postfix expressions e1 and e2 with a binary operator (+) , applying the operator yields e1e2+.
- Postfix notation eliminates the need for parentheses, as the operator’s position and arity allow unambiguous expression decoding.
- In postfix notation, the operator consistently follows the operand. Example 1: The postfix representation of the expression (a + b) * c is : ab + c * Example 2: The postfix representation of the expression (a – b) * (c + d) + (a – b) is : ab – cd + *ab -+ Read more: Infix to Postfix
- A three address statement involves a maximum of three references, consisting of two for operands and one for the result.
- A sequence of three address statements collectively forms a three address code.
- The typical form of a three address statement is expressed as x = y op z , where x, y , and z represent memory addresses.
- Each variable (x, y, z) in a three address statement is associated with a specific memory location.
- A syntax tree serves as a condensed representation of a parse tree.
- The operator and keyword nodes present in the parse tree undergo a relocation process to become part of their respective parent nodes in the syntax tree. the internal nodes are operators and child nodes are operands.
- Creating a syntax tree involves strategically placing parentheses within the expression. This technique contributes to a more intuitive representation, making it easier to discern the sequence in which operands should be processed.
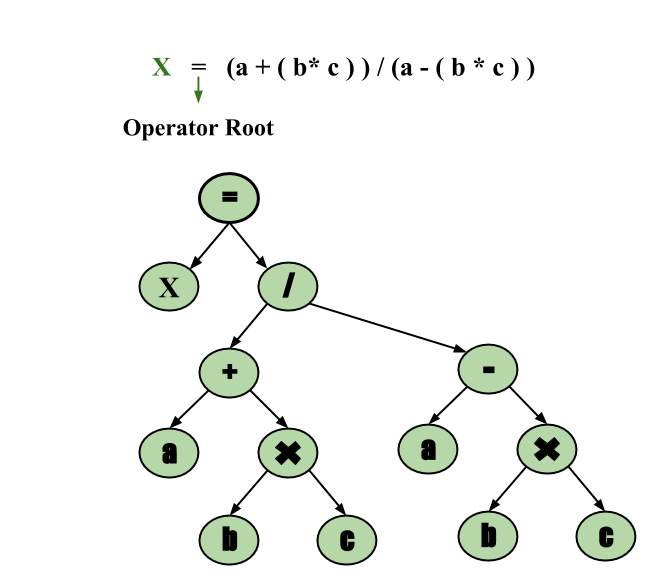
Advantages of Intermediate Code Generation:
Easier to implement: Intermediate code generation can simplify the code generation process by reducing the complexity of the input code, making it easier to implement.
Facilitates code optimization: Intermediate code generation can enable the use of various code optimization techniques, leading to improved performance and efficiency of the generated code.
Platform independence: Intermediate code is platform-independent, meaning that it can be translated into machine code or bytecode for any platform.
Code reuse: Intermediate code can be reused in the future to generate code for other platforms or languages.
Easier debugging: Intermediate code can be easier to debug than machine code or bytecode, as it is closer to the original source code.
Disadvantages of Intermediate Code Generation:
Increased compilation time: Intermediate code generation can significantly increase the compilation time, making it less suitable for real-time or time-critical applications.
Additional memory usage: Intermediate code generation requires additional memory to store the intermediate representation, which can be a concern for memory-limited systems.
Increased complexity: Intermediate code generation can increase the complexity of the compiler design, making it harder to implement and maintain.
Reduced performance: The process of generating intermediate code can result in code that executes slower than code generated directly from the source code.
Please Login to comment...
Similar reads.
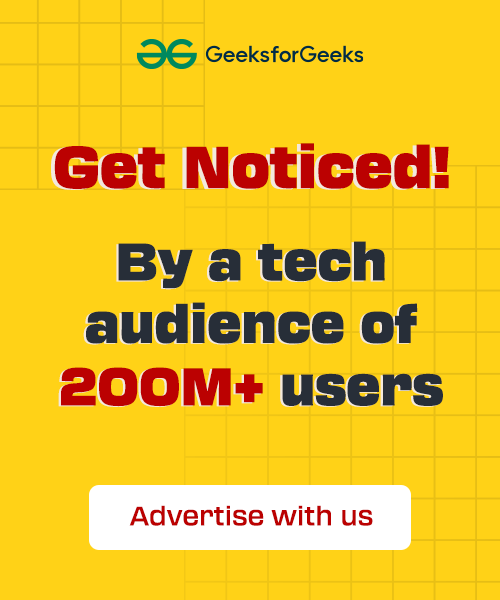
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Intermediate-Code Generation
- First Online: 01 January 2024
Cite this chapter
- Torben Ægidius Mogensen 12
Part of the book series: Undergraduate Topics in Computer Science ((UTICS))
551 Accesses
The ultimate goal of a compiler is to get programs written in a high-level language to run on a computer. This means that, eventually, the program will have to be expressed as machine code that can run on the computer. This does not mean that we need to translate directly from the high-level abstract syntax to machine code. Many compilers use a medium-level language as a stepping-stone between the high-level language and the very low-level machine code. Such stepping-stone languages are called intermediate code . We will generate intermediate code using translation functions for each syntactic category, similar to the functions we used for interpretation and type checking. We generate code for a syntactic construct independently of the constructs around it, except that the parameters of a translation function may hold information about the context (such as symbol tables) and the result of a translation function may (in addition to the generated code) hold information about how the generated code interfaces with its context (such as which variables it uses).
The art of free society consists first in the maintenance of the symbolic code; and secondly in fearlessness of revision, to secure that the code serves those purposes which satisfy an enlightened reason. Alfred North Whitehead (1869–1947)
This is a preview of subscription content, log in via an institution to check access.
Access this chapter
- Available as PDF
- Read on any device
- Instant download
- Own it forever
- Available as EPUB and PDF
- Compact, lightweight edition
- Dispatched in 3 to 5 business days
- Free shipping worldwide - see info
Tax calculation will be finalised at checkout
Purchases are for personal use only
Institutional subscriptions
Note that the coordinate system is rotated 90° clockwise compared to mathematical tradition.
Aït-Kaci, H.: Warren’s Abstract Machine – A Tutorial Reconstruction. MIT Press (1991)
Google Scholar
Appel, A.W.: Compiling with Continuations. Cambridge University Press (1992)
Jones, S.L.P., Lester, D.: Implementing Functional Languages – A Tutorial. Prentice Hall (1992)
Lattner, C.: LLVM language reference manual (2011). http://llvm.org/docs/LangRef.html
Lindholm, T., Yellin, F.: The Java Virtual Machine Specification, 2nd edn. Addison-Wesley, Reading, Massachusetts (1999)
Muchnick, S.S.: Advanced Compiler Design and Implementation. Morgan Kaufmann (1997)
Download references
Author information
Authors and affiliations.
Datalogisk Institut, Københavns Universitet, Copenhagen, Denmark
Torben Ægidius Mogensen
You can also search for this author in PubMed Google Scholar

Corresponding author
Correspondence to Torben Ægidius Mogensen .
Rights and permissions
Reprints and permissions
Copyright information
© 2024 Springer International Publishing AG
About this chapter
Mogensen, T.Æ. (2024). Intermediate-Code Generation. In: Introduction to Compiler Design. Undergraduate Topics in Computer Science. Springer, Cham. https://doi.org/10.1007/978-3-031-46460-7_6
Download citation
DOI : https://doi.org/10.1007/978-3-031-46460-7_6
Published : 01 January 2024
Publisher Name : Springer, Cham
Print ISBN : 978-3-031-46459-1
Online ISBN : 978-3-031-46460-7
eBook Packages : Computer Science Computer Science (R0)
Share this chapter
Anyone you share the following link with will be able to read this content:
Sorry, a shareable link is not currently available for this article.
Provided by the Springer Nature SharedIt content-sharing initiative
- Publish with us
Policies and ethics
- Find a journal
- Track your research
Declaration and assignment in intermediate code generation
By Team EasyExamNotes
In intermediate code generation, the process of declaring variables and assigning values to them involves creating appropriate intermediate code statements.
Here’s how declaration and assignment are typically handled in intermediate code generation:
1. Declaration:
- When a variable is encountered in the source code, a corresponding declaration statement is generated in the intermediate code.
- The declaration statement typically includes the variable name, data type, and any necessary information for memory allocation, such as the size of the variable.
- The declaration statement ensures that space is allocated for the variable in memory during the execution of the program.
2. Assignment:
- When an assignment statement is encountered in the source code, an equivalent intermediate code statement is generated.
- The assignment statement typically consists of the target variable (left-hand side) and the expression or value to be assigned (right-hand side).
- The intermediate code statement captures the intent of assigning a value to the target variable .
3. Expression Evaluation:
- In many cases, assignment statements involve expressions that need to be evaluated.
- Intermediate code generation handles expression evaluation by breaking down the expression into a sequence of intermediate code statements.
- The sequence of intermediate code statements performs the necessary computations, including arithmetic operations, function calls, and handling of variables or constants.
Example to illustrate the intermediate code generation for declaration and assignment:
Sourc code:, intermediate code:.
In the above example, the DECLARE statements generate intermediate code for variable declaration.
The assignment statements a = 5 and b = t1 generate intermediate code for assignment, where t1 is a temporary variable holding the result of the expression a + 3.
The specific format and representation of intermediate code can vary depending on the chosen intermediate representation (e.g., three-address code, quadruples, or bytecode). The key idea is to generate intermediate code statements that capture the necessary information for subsequent stages of compilation or interpretation.
Share this:
- Click to share on Facebook (Opens in new window)
- Click to share on Telegram (Opens in new window)
- Click to share on WhatsApp (Opens in new window)
Search anything:
Types and Declarations in Intermediate Code Generation
Compiler design.
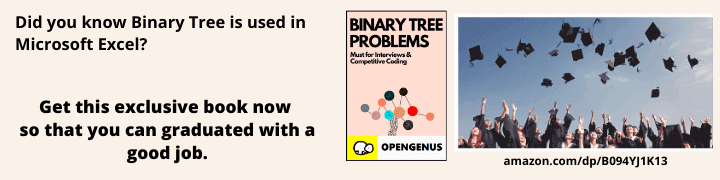
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we learn about types and the storage layouts for names declared within a procedure or class which are allocated at run time when the procedure is called or an object is created.
Table of contents.
Introduction..
- Type expressions.
- Type equivalence.
- Declarations.
- Storage layout for local names.
- Sequences and declarations.
- Records and classes fields.
References.
Applications of types are grouped under checking and translation . First type checking is used to reason about the behavior of a program at runtime by using logical rules.
In applications of translation, given the type of a name, the compiler can determine the storage needed for the name during runtime.
In this article, we learn about types and storage layouts for names declared within classes or procedures. The storage procedure call or an object is allocated during runtime when the procedure is called or when the object is created.
Type expressions
Types have a structure we will represent using type expressions , a type expression can either be formed by applying a type constructor operator to a type expression or can be a basic type.
Basic types are determined by the language that is being checked.
An example; We have an array of type int[2][3] that is read as array of 2 arrays of 3 integers each . It is written as a type expression as follows; array(2, array(3, integer)) We represent it as a tree as shown below (1);
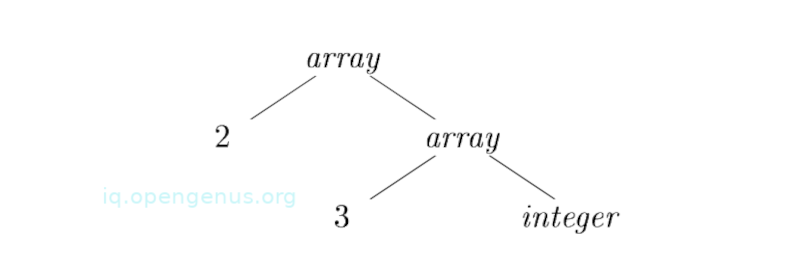
The following definitions are used for type expressions;
- A basic type expression. These include, boolean , char , integer , float and void .
- A type name is a type expression.
- A type expression is formed by applying an array type constructor to a number and type expression.
- A record is a data structure with named fields. A type expression is formed by applying the record type constructor to the fields' names and their types.
- A type expression is formed by using the type constructor → for function types. That is, we write s → t for 'function from type s to type t' .
- If s and t are type expressions, their cartesian product s x t is a type expression.
- Type expressions may contain variables whose values are type expressions.
Type equivalence
Type-checking rules are of the form; 'if two type expressions are equal then return a certain type, else return an error' .
When similar names are used for type expressions and other subsequent type expressions, ambiguities arise.
The problem is whether a name in a type expression represents itself or represents an abbreviation for another type expression.
When representing type expressions using graphs, we say that two types are structurally equivalent if and only if either of the conditions is true;
- They have the same basic type.
- They are formed by applying a similar constructor to structurally equivalent types.
- One is a type name that denotes the other.
If we treat type names as standing for themselves, then the first two conditions in the definition above lead to the name equivalence of type expressions.
Declarations
We learn about types and declarations using simplified grammar that declares a single name at a time. We have the following grammar; (2)
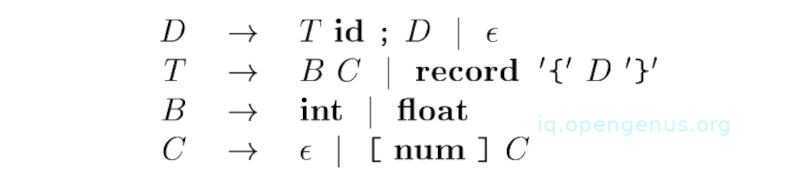
The non-terminal D generates a sequence of declarations. Non-terminal T generates basic types, array, or record types. The non-terminal B generates a basic type either an int or float . The non-terminal C generates strings of zero or more integers, each surrounded by brackets. An array type consists of the basic type specified by B followed by array components specified by a non-terminal C . A record type is a sequence of declarations for fields of the record surrounded by curly braces.
Storage layout for local names
Given a type name, we can determine the amount of storage that is needed for the name at runtime. During compile time we use these amounts to assign each name a relative address.
Type and relative addresses are stored in a symbol table entry for the name. Varying-length data such as strings or data whose size cannot be determined until runtime such as dynamic arrays is handled by keeping a fixed amount of storage for a pointer to data.
Assuming that storage is in blocks of contiguous bytes whereby a byte is the smallest unit of addressable memory. Multibyte objects are stored in consecutive bytes and given the address of the first byte.
We have the following SDT(Syntax Directed Translation) that computes types and their widths for basic and array types. (3)
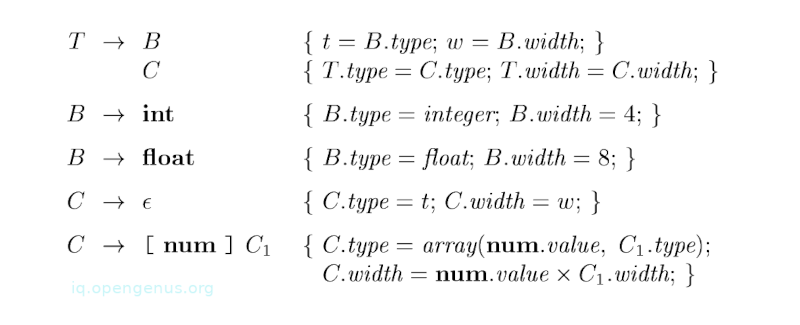
The above SDT uses synthesized attributes type and width for each non-terminal and two variables t and w to pass type and width information from B node in a parse tree to a node fo the production C → ϵ . In an SDD(Syntax-Directed Definition) , t and w would be inherited attributes for C .
The body of the T-production consists of a non-terminal B , an action, and a non-terminal C that appears on the next line. The action between B and C sets t to B-type and w to B.width . If B → int , B.type is set to an integer and B.width is set to 4 which is the width of an integer. Similarly, if B → float , B.type is float and B.width is 8 , which is the width of a float.
Productions for C determine whether T generates a basic type or an array type. If C → ϵ , then t is C.type and w is C.width .
Otherwise, C specifies an array component. The action for C → [num] C 1 forms C.type by applying the type constructor array to the operands num.value and C 1 .type . For example, the resulting tree structure for applying an array can be seen from the first image.
To obtain the width of an array we multiply the width of an element by the number of elements in an array. If addresses of consecutive integers differ by 4 , then the address calculations for an array of integers include multiplications by 4 . These multiplications give opportunities for optimization and therefore the front end needs to make them explicit.
Sequences and declarations
In programming languages such as C and Java , declarations in a single group are processed as a group. These declarations can be distributed within a Java procedure but can still be processed when the procedure s analyzed. We can use a variable to track the next available relative address.
The following translation scheme(4) deals with a sequence of declarations in the form of T id where T generates a type as shown in image (3).
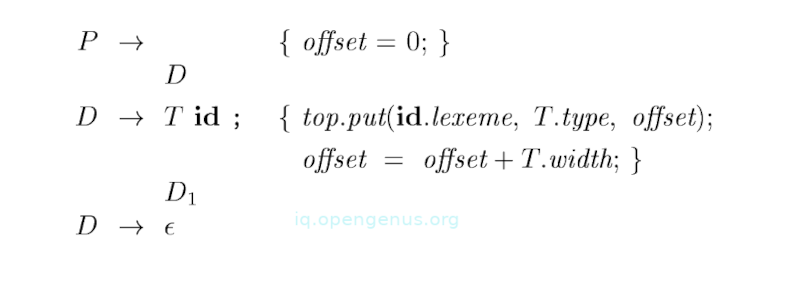
Before the first declaration is considered, a variable offset that tracks the next available relative address is set to 0 . The value of offset is incremented by the width of the type of x , x is a new name entered into the symbol table with its relative address set to the current value of offset .
The semantic action, within the production D → T id , D 1 creates a symbol table entry by executing top.put(id.lexeme, T.type, offset) . top denotes the current symbol table. top.put creates a symbol table entry for id.lexeme with T.type and relative address offset in its data area.
The initialization of offset in image (4) is more evident in the first production that appears as; P → {offset = 0;} D
Non-terminals generating ϵ referred to as marker non-terminals are used to rewrite productions so that all actions appear at the right ends. By using a maker non-terminal M , the above production is restated as; P → M D *M → ϵ { offset = 0; }
Records and classes fields
The translation of declarations in image (4) carries over to fields in records and classes. Record types are added to the grammar in image (3) by adding the following production;
T → record '{' D '}' .
The fields in the record type are specified by a sequence of declarations that are generated by D . The approach used in image (4) can be used to determine the types and relative addresses of fields as long as we are careful about the following;
- Field names within a record must be distinct, that is, a name can only appear once in declarations generated by D .
- The offset or relative address for a field name is relative to the data area for that record.
Graphs are useful for representing type expressions.
Applications of types can be under checking or translation . Checking reasons about the behavior of a program at runtime by using logical rules.
For translation , given the type of a name, the compiler can determine the storage needed for the name during runtime.
- Basics of Compiler Design - Torben Mogensen
- Compilers Principles, Techniques, & Tools - Alfred V. Aho Monica
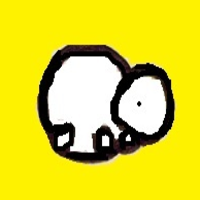

IMAGES
VIDEO
COMMENTS
The assignment statements one = 5 and b = t1 generate intermediate code for assignment, where t1 is a temporary variable holding the result of the expression a + 3. The specified format also representation of intermediate code can vary depending on the chosen intermediate representation (e.g., three-address codes, quadruples, or bytecode).
The "Assignment Statements - Intermediate Code Generation, Computer Science and IT Engineering Computer Science Engineering (CSE) Questions" guide is a valuable resource for all aspiring students preparing for the Computer Science Engineering (CSE) exam.
Platform independence: Intermediate code is platform-independent, meaning that it can be translated into machine code or bytecode for any platform. Code reuse: Intermediate code can be reused in the future to generate code for other platforms or languages. Easier debugging: Intermediate code can be easier to debug than machine code or bytecode, as it is closer to the original source code.
Intermediate Code Generation Rupesh Nasre. CS3300 Compiler Design IIT Madras August 2020. 2 Intermediate Code Generator Intermediate Code Generator ... Assignment statement: a = b * - c + b * - c; t1 = minus c t2 = b * t1 t3 = minus c t4 = b * t3 t5 = t2 + t4 a = t5 op arg1 arg2 result minus c * b (0) minus c
Other handy instructions. drop. An intermediate representation (IR) provides the connection between the front end and the back end of the compiler, and continues to represent the program during the various back-end phases. IRs can be classified in terms of their level, or degree of machine dependence. High-level IRs.
Intermediate representation (AST vs DAG, three-address code) Translating expressions, control flow, declarations, and statements. Not specific to Mini-Go ... Assignment statement x = y op z 2.) Unary assignment x = op y 3.) Copy statement x = y 4.) Unconditional jump goto L 5.) Conditional jump if x rel y goto L
Code Generation Intermediate code generation: Declarations, Assignment statements, Boolean expressions, Case statements, Back patching, Procedure calls Code Generation: Issues in the design of code generator, Basic block and flow graphs, Register allocation and assignment, DAG
Intermediate code generation! Purpose: translate ASTs into linear sequence of simple statements called intermediate code • Can optimize intermediate code in place • A later pass translates intermediate code into target code! Intermediate code is machine-independent • Don't worry about details of the target machine (e.g., number of
Lecture IX—Intermediate Code Generation Alessandro Artale Free University of Bozen-Bolzano Faculty of Computer Science - POS Building, Room: 2.03 ... 1 Assignment Statements: x := y op z. 2 Unary Assignment Statements: x := op y. 3 Copy Statements: x := y. 4 Unconditional Jump: goto L (with L a label of a statement).
Intermediate Code Generation Intermediate Representation (IR): ... Three-Address Code & Static Single Assignment Most register-based IRs use three-address code: Arithmetic instructions have (up to) three operands: two ... Three-Address Code (TAC) Generation Goal: take statements (AST) and produce a sequence of TAC. Example: a :=b + c * d;
INTRODUCTION - Intermediate Code generator The front end translates a source program into an intermediate representation from which the back end generates target code. Benefits of using a machine-independent intermediate form are: ... A syntax tree and dag for the assignment statement a : = b * - c + b * - c are as follows:
Intermediate-Code Generation Mohamed Zahran (aka Z) [email protected]. Back-end and Front-end of A Compiler. Back-end and Front-end of A Compiler ... path that was taken to get to the assignment statement containing the Ø-function. Example. Types and Declarations •Type checking: to ensure that types of operands match the type expected by
When we generate intermediate code, we want, for simplicity, to treat each subexpression independently of its context. This may lead to such superfluous assignments. We will look at ways of getting rid of these when we treat machine code generation and register allocation in Chaps. 7 and 8. A more complex expression is 3+f(x-y,z). Using the ...
Transform AST to lower-level intermediate representation. Basic Goals: Separation of Concerns. Generate efficient code sequences for individual operations. Keep it fast and simple: leave most optimizations to later phases. Provide clean, easy-to-optimize code. IR forms the basis for code optimization and target code generation.
Intermediate-Code Generation Three-Address Code • In three-address code, there is at most one operator on the right ... Three-address code for an assignment statement When an expression is a single identifier, say x, then x itself holds the value of the expression.
Intermediate Code Generation Intermediate Representation (IR): ... Three-Address Code & Static Single Assignment SSA Form simplifies various compiler optimizations. Three-Address: y = 1 y = 2 x = y ... Three-Address Code (TAC) Generation Goal: take statements (AST) and produce a sequence of TAC. Example: a :=b + c * d;
Intermediate Code Generation We could translate the source code directly into the target Language, but there are benefits to having an intermediate, ... Assignment statements of the form x := y op z Assignment statements of the form x := op z where op is a unary operation (e.g. unary minus, logical negation, shift and convert ...
This video is about Assignment Statements in intermediate code generation in compiler design
code generation • Semantic Action (or, Semantic Routine): Each action symbol is associated with a sub-routine to perform • Semantic Stack (here referred to by "ss"): a stack dedicated to the both semantic analyzer and intermediate code generator to store and use the required information
The reason for the term "three-address code" is that each statement usually contains three addresses, two for the operands and one for the result. Types of Three-Address Statements: The common three-address statements are: 1. Assignment statements of the form x : = y op z, where op is a binary arithmetic or logical operation. 2.
We will generate intermediate code using translation functions for each syntactic category, similar to the functions we used for interpretation and type checking. ... When translating statements, we will need the symbol table for variables (for translating assignment), and since statements contain expressions, we also need \( ftable \) so we ...
The assignment statements a = 5 and b = t1 generate intermediate code for assignment, where t1 is a temporary variable holding the result of the expression a + 3. The specific format and representation of intermediate code can vary depending on the chosen intermediate representation (e.g., three-address code, quadruples, or bytecode).
We learn about types and declarations using simplified grammar that declares a single name at a time. We have the following grammar; (2) The non-terminal D generates a sequence of declarations. Non-terminal T generates basic types, array, or record types. The non-terminal B generates a basic type either an int or float.