Assigning Service Principals to Groups and Roles with the Azure CLI
The more I use Azure the more often I find myself needing to assign various managed identities / service principals to various groups and roles, and while that can be done in the Portal, it's cumbersome and I'd prefer to automate it.
So in this post I'll sharing a few Azure CLI commands that should prove useful whenever you're configuring Service Principals.

Getting a service principal's object id
Suppose you know the name of the service principal, but not the "object id", which is required for assigning it to groups and roles. You can use a filter with the az ad sp list command to find that service principal and then a query to pick out just the object id.
Note that you should avoid trying to use the query parameter to find the matching name, as that will likely not find it as it only applies to the first page of results .
Note that the object id is different from the app id. If you do need the app id for any reason you just need to change the query parameter:
Adding to a group
Suppose we want to add the service principal to a group. We need the group id to do that, and if we need to look it up, we can do so with the az ad group list command and using a filter .
Then the az ad group member add command allows us to add the object id of our service principal to the group.
Creating a role assignment
If we want to create a role assignment, then as well as knowing the user we're assigning the role to and the name of the role, we also need to provide a " scope " for that to apply to. This is typically a long / delimited path to an Azure resource. So for a KeyVault it might look like this:
You can of course construct this string yourself, but actually this is quite often just the "ID" of the resource as returned by the Azure CLI. So we could get the above value with the following command:
And now that we have the scope, we can simply use the az role assignment create to assign the role to our service principal, and we can pass the role name directly (in this example it's "Key Vault Administrator"):
Hope this proves useful to you.
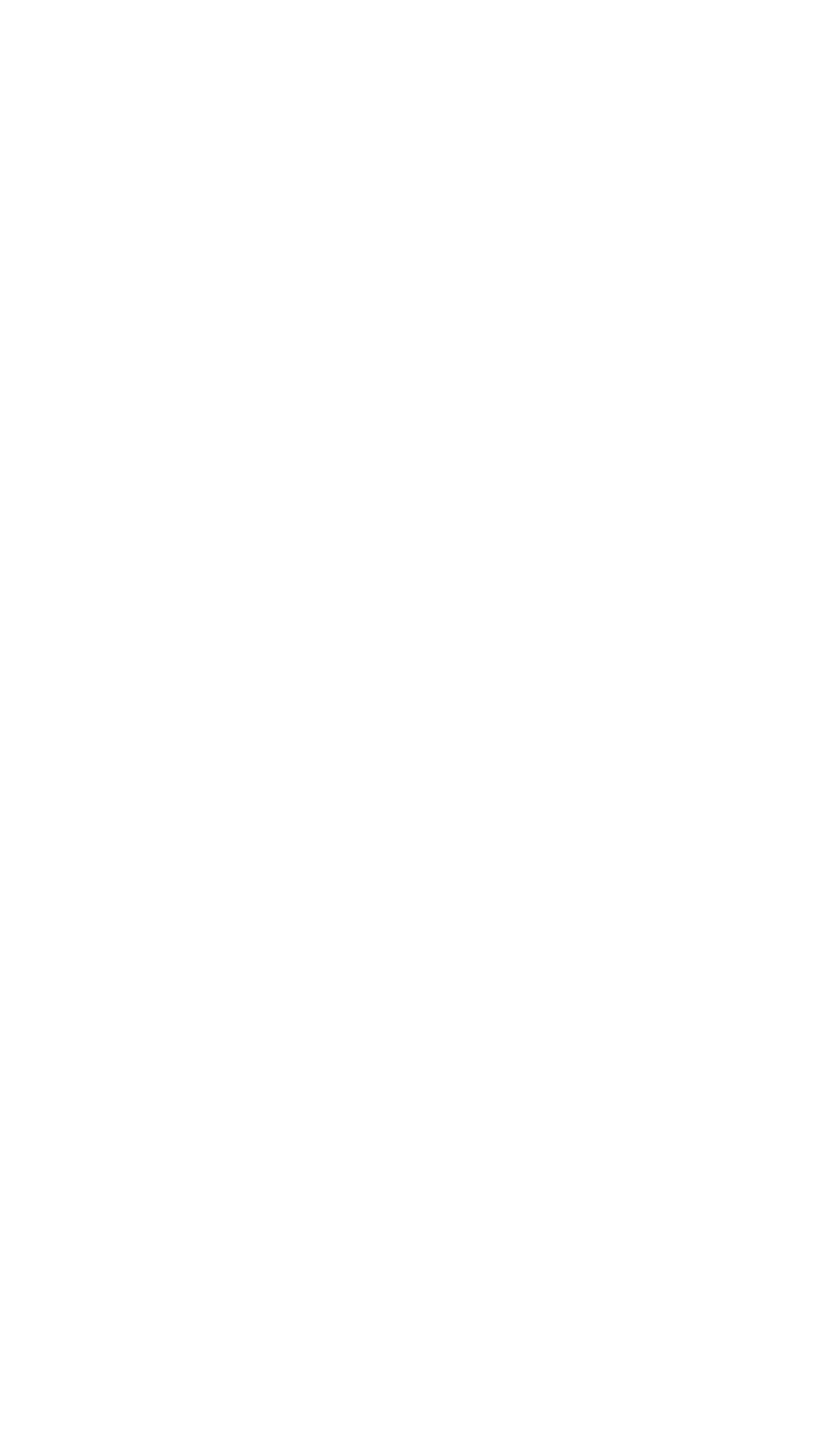
{ | |
"description": "Grant service principal X reader access on application Z.", | |
"client_type" : "application", | |
"client_principal_name": "service-principal-x-prod", | |
"server_app_registration_name": "app-registration-z-prod", | |
"role_name": "reader" | |
}, |
You can use the script like this:
- Download the script and the config file.
- Update the config files to your needs
- Trigger the script via PowerShell
If you are interested, this is how the script looks like:
param ( | |
[string] $TenantId, | |
[string] $ConfigFilePath | |
) | |
$ErrorActionPreference = "Stop" | |
Write-Host Start Azure AD role assignment script | |
Write-Host "-Tenant Id:" $TenantId -ForegroundColor Gray | |
Write-Host "-Config File Path:" $ConfigFilePath -ForegroundColor Gray | |
Write-Host Installing and importing AzureAD Module | |
if (Get-Module -ListAvailable -Name AzureAD) { | |
Import-Module -Name "AzureAD" | |
} | |
else { | |
Install-Module -Name "AzureAD" -Force | |
} | |
Write-Host Connecting to Azure AD Tenant within current security context | |
$azure_context = Get-AzContext | |
$account_id = $azure_context.Account.Id | |
Write-Host "-Account Id:" $azure_context.Account.Id -ForegroundColor Gray | |
Connect-AzureAD -TenantId $TenantId -AccountId $account_id | |
Write-Host Loading role assignments from config file | |
$role_assignments = (Get-Content $ConfigFilePath -Raw) | ConvertFrom-Json | |
Write-Host Looping each configured role assignment | |
foreach($role_assignment in $role_assignments) | |
{ | |
Write-Host Applying role assigment... started -ForegroundColor Green | |
Write-Host "-Description:" $role_assignment.description -ForegroundColor Gray | |
Write-Host "-Client principal Name:" $role_assignment.client_principal_name -ForegroundColor Gray | |
Write-Host "-Server App Registration Name:" $role_assignment.server_app_registration_name -ForegroundColor Gray | |
Write-Host "-Role Name:" $role_assignment.role_name -ForegroundColor Gray | |
Write-Host Getting the server application registration | |
$aad_filter = "DisplayName eq '" + $role_assignment.server_app_registration_name + "'" | |
$server_application_registration = Get-AzureADApplication -Filter $aad_filter | |
if (!$server_application_registration) { throw "Cannot find configured server application registration with name '" + $role_assignment.server_app_registration_name + "'" } | |
Write-Host Getting the server service principal id | |
$aad_filter = "AppId eq '" + $server_application_registration.AppId + "'" | |
$server_service_principal = Get-AzureADServicePrincipal -Filter $aad_filter | |
$server_service_principal_id = $server_service_principal.ObjectId | |
Write-Host "-Server service principal Id: " $server_service_principal_id -ForegroundColor Gray | |
Write-Host Getting the Id for the configured application role | |
$role_id = ($server_application_registration.AppRoles | Where-Object DisplayName -eq $role_assignment.role_name).Id | |
if (!$role_id) { throw "Cannot find configured application role with name '" + $role_assignment.role_name + "'" } | |
Write-Host "-Role Id: " $role_id -ForegroundColor Gray | |
if(($role_assignment.client_type -ne "application") -and ($role_assignment.client_type -ne "user")) { throw "Incorrect client_type '" + $role_assignment.client_type + "' provided." } | |
switch ($role_assignment.client_type) | |
{ | |
"application" | |
{ | |
Write-Host Getting the configured client service principal | |
$aad_filter = "DisplayName eq '" + $role_assignment.client_principal_name + "'" | |
$client_service_principal = (Get-AzureADServicePrincipal -Filter $aad_filter) | |
if (!$client_service_principal) { throw "Cannot find configured client service principal with name '" + $role_assignment.client_principal_name + "'" } | |
$client_service_principal_id = $client_service_principal.ObjectId | |
$client_service_principal_name = $client_service_principal.DisplayName | |
Write-Host "-Client service principal Id:" $client_service_principal_id -ForegroundColor Gray | |
Write-Host Assigning the Azure Ad role to the configured service principal | |
try | |
{ | |
New-AzureADServiceAppRoleAssignment -Id $role_id -ResourceId $server_service_principal_id -ObjectId $client_service_principal_id -PrincipalId $client_service_principal_id | |
} | |
catch | |
{ | |
if( $_.Exception.Message -like '*Permission being assigned already exists on the object*') | |
{ | |
Write-Host Permission already exists | |
} | |
else | |
{ | |
Write-Error $_.Exception.Message | |
} | |
} | |
} | |
"user" | |
{ | |
Write-Host Getting the configured client user | |
$user = Get-AzureADUser -searchstring $role_assignment.client_principal_name | |
if (!$user) { throw "Cannot find configured client users with name '" + $role_assignment.client_principal_name + "'" } | |
$user_id = $user.ObjectId | |
Write-Host "-User Id:" $user_id -ForegroundColor Gray | |
Write-Host Assigning the Azure Ad role to the configured user | |
try | |
{ | |
New-AzureADUserAppRoleAssignment -Id $role_id -ResourceId $server_service_principal_id -ObjectId $user_id -PrincipalId $user_id | |
} | |
catch | |
{ | |
if( $_.Exception.Message -like '*Permission being assigned already exists on the object*') | |
{ | |
Write-Host Permission already exists | |
} | |
else | |
{ | |
Write-Error $_.Exception.Message | |
} | |
} | |
} | |
} | |
Write-Host Applying role assigment... done -ForegroundColor Green | |
} |
I hope that this script helps you to accelerate your security automation.
Cheers Toon
UPCOMING TRAININGS
CHECK OUT OUR TRAININGS
Azure Integration Services
Azure migration.
- Azure Governance
Azure Security
Azure foundations, recent posts.
- Looking back at INTEGRATE 2024
- Azure Service Bus vs Event Grid Pull Delivery
- Trying the new Microsoft Applied Skills
- Finally a correct way to configure RBAC for DevOps agents!
- What do the new API Management v2 tiers mean for you?
- Announcement
- API Management
- Architecture
- Azure App Service
- Azure Data Factory
- Azure DevOps
- Azure Event Grid
- Azure Functions
- Azure Kubernetes Service
- Azure Policy
- Azure Resource Graph
- Azure Resource Manager
- Azure Service Bus
- Azure Stream Analytics
- BizTalk Server
- Container Apps
- Geen categorie
- Home Automation
- Microsoft Learn
- Service Bus
MEET THE YOUR AZURE COACH TEAM
Your Azure Coach is specialized in organizing Azure trainings that are infused with real-life experience. All our coaches are active consultants, who are very passionate and who love to share their Azure expertise with you.
Toon Vanhoutte
Azure integration services & serverless.
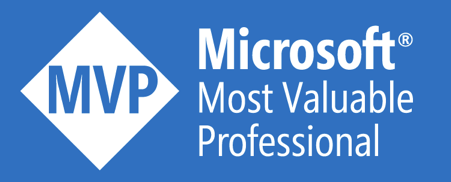
Wim Matthyssen
Azure infra, security & governance, azure development and ai/ml, azure identity and security, stéphane eyskens, cloud-native azure architecture, geert baeke, azure kubernetes service & containerization, maik van der gaag, azure infrastructure as code & devops, bart verboven, sammy deprez, azure ai, ml & cognitive services, sander van de velde.
- Career Model
- Proactive Mentorship
- Productivity
- Review Model
- Work:Life Balance
- 3D Printing
- Announcements
- Conferences
How to find all the Azure Built-In Roles for Azure RBAC with Azure CLI, PowerShell, Docs, or AzAdvertizer
Here are a bunch of ways you can find which roles are built into Azure. This will come in super handy when you need to assign a role to a service principal or user with Azure CLI commands like this:
- Query the big honking json
- Query all, but only return Name and Id in a nice table
- Filter by name contains:
This one filters for roles with “Map” in the name:
Azure PowerShell
https://docs.microsoft.com/en-us/powershell/module/az.resources/get-azroledefinition?view=azps-3.8.0
This page has all the built in roles: https://docs.microsoft.com/azure/role-based-access-control/built-in-roles
AzAdvertizer
Just found this site today by Julian Hayward. It’s a great way to find roles
https://www.azadvertizer.net/azrolesadvertizer_all.html

Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
What is the default RBAC scope used when assigning a role in Azure with the CLI?
This is the documentation for the az role assignment create command: https://docs.microsoft.com/en-us/cli/azure/role/assignment?view=azure-cli-latest#az-role-assignment-create
--score is an optional parameter. This is what the documentation says about it:
Scope at which the role assignment or definition applies to, e.g., /subscriptions/0b1f6471-1bf0-4dda-aec3-111122223333, /subscriptions/0b1f6471-1bf0-4dda-aec3-111122223333/resourceGroups/myGroup, or /subscriptions/0b1f6471-1bf0-4dda-aec3-111122223333/resourceGroups/myGroup/providers/Microsoft.Compute/virtualMachines/myVM.
As you can see, it doesn't say what the default value for this parameter is. I can't find it anywhere, so I found myself forced to ask here.
- I can't select my own answer as the correct one because I have to wait 2 days, but that's the correct answer anyways. – Adrian Commented Jan 22, 2022 at 4:25
https://docs.microsoft.com/en-us/azure/role-based-access-control/role-assignments-cli#step-4-assign-role
Apparently, when the --scope parameter is not provided its value depends on whether the --resource-group parameter is provided or not. If you provide that parameter, then it's like if you specified the resource group scope. Else, the subscription scope is assumed.
You must log in to answer this question.
Not the answer you're looking for browse other questions tagged azure entra-id azure-cli ..
- The Overflow Blog
- The framework helping devs build LLM apps
- How to bridge the gap between Web2 skills and Web3 workflows
- Featured on Meta
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
- Announcing a change to the data-dump process
Hot Network Questions
- Why does "They be naked" use the base form of "be"?
- Were ancient Greece tridents different designs from other historical examples?
- How to request for a package to be added to the Fedora repositories?
- Mutual Life Insurance Company of New York -- What is it now? How can I reach them?
- The book where someone can serve a sentence in advance
- Can loops/cycles (in a temporal sense) exist without beginnings?
- Is the XOR of hashes a good hash function?
- Welch t-test p-values are poorly calibrated for N=2 samples
- A Professor's Presentation
- What goods yield the best profit for time-travel arbitrage?
- Is deciding to use google fonts the sort of decision that makes an entity a controller rather than a processor?
- Infinite output impedance in amplifier, instead of 0?
- Book series where soldiers or pilots from Earth are teleported to a world where they learn how to use crystal magic technology
- Are the hangers on these joists sized and installed properly?
- What are good reasons for declining to referee a manuscript that hasn't been posted on arXiv?
- What kind of pressures would make a culture force its members to always wear power armour
- Can every kind of object perform actions?
- Why is restructure of mempool required with cluster mempool?
- Is it worth it to apply to jobs that have over 100 applicants or have been posted for few days?
- Mass driver - reducing required length using loop?
- How wrong is NIST 800-89's Partial Public Key Validation for RSA step e?
- smartctl lies that NVME has lifespan of ~2800TBW? What is the real lifespan of my NVME?
- A publicly accessible place to photo Cologne Cathedral's west facade
- Fourier series using DFT
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.

Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Role assignment creation failed through az ad sp create-for-rbac command #22837
francisrod01 commented Jun 11, 2022
I'm following the Azure container Apps doc to .
Steps to reproduce the behavior. Note that argument values have been redacted, as they may contain sensitive information. |
The text was updated successfully, but these errors were encountered: |
yonzhan commented Jun 11, 2022
for awareness |
Sorry, something went wrong.
francisrod01 commented Jun 11, 2022 • edited Loading
More to add: ⚠ I've tried downgrades but it didn't work, previous versions aren't able to run the comment mentioned above. |
francisrod01 commented Jun 12, 2022
I think you guys are working on it, |
jiasli commented Jun 13, 2022
Duplicate of |
- 👍 1 reaction
spanchal1980 commented Sep 18, 2023
Any update on this. I am getting same issue. |
No branches or pull requests
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Tutorial: Create an Azure custom role using Azure CLI
- 6 contributors
If the Azure built-in roles don't meet the specific needs of your organization, you can create your own custom roles. For this tutorial, you create a custom role named Reader Support Tickets using Azure CLI. The custom role allows the user to view everything in the control plane of a subscription and also open support tickets.
In this tutorial, you learn how to:
Create a custom role
List custom roles, update a custom role, delete a custom role.
If you don't have an Azure subscription, create a free account before you begin.
Prerequisites
To complete this tutorial, you will need:
- Permissions to create custom roles, such as User Access Administrator
- Azure Cloud Shell or Azure CLI
Sign in to Azure CLI
Sign in to Azure CLI .
The easiest way to create a custom role is to start with a JSON template, add your changes, and then create a new role.
Review the list of actions for the Microsoft.Support resource provider . It's helpful to know the actions that are available to create your permissions.
Action | Description |
---|---|
Microsoft.Support/register/action | Registers to Support Resource Provider |
Microsoft.Support/supportTickets/read | Gets Support Ticket details (including status, severity, contact details and communications) or gets the list of Support Tickets across subscriptions. |
Microsoft.Support/supportTickets/write | Creates or Updates a Support Ticket. You can create a Support Ticket for Technical, Billing, Quotas or Subscription Management related issues. You can update severity, contact details and communications for existing support tickets. |
Create a new file named ReaderSupportRole.json .
Open ReaderSupportRole.json in an editor and add the following JSON.
For information about the different properties, see Azure custom roles .
Add the following actions to the Actions property. These actions allow the user to view everything in the subscription and create support tickets.
Get the ID of your subscription using the az account list command.
In AssignableScopes , replace {subscriptionId1} with your subscription ID.
You must add explicit subscription IDs, otherwise you won't be allowed to import the role into your subscription.
Change the Name and Description properties to "Reader Support Tickets" and "View everything in the subscription and also open support tickets."
Your JSON file should look like the following:
To create the new custom role, use the az role definition create command and specify the JSON role definition file.
The new custom role is now available and can be assigned to users, groups, or service principals just like built-in roles.
To list all your custom roles, use the az role definition list command with the --custom-role-only parameter.
You can also see the custom role in the Azure portal.

To update the custom role, update the JSON file and then update the custom role.
Open the ReaderSupportRole.json file.
In Actions , add the action to create and manage resource group deployments "Microsoft.Resources/deployments/*" . Be sure to include a comma after the previous action.
Your updated JSON file should look like the following:
To update the custom role, use the az role definition update command and specify the updated JSON file.
Use the az role definition delete command and specify the role name or role ID to delete the custom role.
Create or update Azure custom roles using Azure CLI
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Renew JSON authentication key of Service Principal
I have created a Service Principal to access resources in CI before using the az ad sp create-for-rbac command:
This command yields a JSON object with the secret, a few IDs, and a few endpoint URL.
Now that secret is close to expiring. I know that I can renew the secret using az ad app credential reset --id <my-sp-id> . However, that command outputs a different JSON schema.
How do I obtain a drop-in replacement for the --json-auth token that az ad sp create-for-rbac generated?
- azure-active-directory

- 2 What's your exact requirement? Could you brief more regarding it? If you are looking for equivalent command to get similar json response while resetting secret, it's not possible. – Sridevi Commented yesterday
Initially, I ran same CLI command in my environment and got below JSON response:

When you reset the client secret with below CLI command, you will get only few values in response as the remaining will stay same:

Note that, there is no direct CLI command to get similar JSON response while resetting client secret. Alternatively, you can make use of below script that adds remaining values manually in response:

Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged azure azure-active-directory azure-cli or ask your own question .
- The Overflow Blog
- The framework helping devs build LLM apps
- How to bridge the gap between Web2 skills and Web3 workflows
- Featured on Meta
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
- Announcing a change to the data-dump process
- What makes a homepage useful for logged-in users
Hot Network Questions
- Can loops/cycles (in a temporal sense) exist without beginnings?
- Mutual Life Insurance Company of New York -- What is it now? How can I reach them?
- Formula for bump function
- Left crank arm misaligned on climb
- Are 自分で来ました and 自分で来た grammatical?
- QGIS: Horizontal alignment of legend symbol to multi-line label
- How wrong is NIST 800-89's Partial Public Key Validation for RSA step e?
- Are Windows ReFS file-level snapshots what File History should have been?
- How could double damage be explained in-universe?
- I can't find a nice literal translation for "Stella caelis exstirpavit"
- What are good reasons for declining to referee a manuscript that hasn't been posted on arXiv?
- Knights, Knaves, and Spies
- Reorder for smallest largest prefix sum
- Meaning of general Lorentz transformations
- Verbs for to punish
- What sort of security does Docusign provide?
- What concretely does it mean to "do mathematics in a topos X"?
- Why does "They be naked" use the base form of "be"?
- A story about a personal mode of teleportation, called "jaunting," possibly in Analog or Amazing Stories
- Complexity of permutation group intersection
- Diminished/Half diminished
- Objects proven finiteness yet no algorithm discovered?
- What's to prevent us from concluding that Revelation 13:3 has been fulfilled through Trump?
- Why is restructure of mempool required with cluster mempool?

IMAGES
VIDEO
COMMENTS
To assign a role, you might need to specify the unique ID of the object. The ID has the format: 11111111-1111-1111-1111-111111111111. You can get the ID using the Azure portal or Azure CLI. User. For a Microsoft Entra user, get the user principal name, such as [email protected] or the user object ID.
Name Description Type Status; az role assignment create: Create a new role assignment for a user, group, or service principal. Core GA az role assignment delete
To list a custom role definition, use az role definition list. This command is the same command you would use for a built-in role. Azure CLI. Copy. az role definition list --name {roleName} The following example lists the Virtual Machine Operator role definition: Azure CLI. Copy.
This is the Azure CLI Command to create a new role in Azure, where RoleInfo.json is the local file with all the configurations, scope, actions, data actions regarding that role. You need to follow the Microsoft's Custom Role Creation Documentation to make sure everything is setup in a proper way. az role definition create --role-definition ...
Adding to a group. Suppose we want to add the service principal to a group. We need the group id to do that, and if we need to look it up, we can do so with the az ad group list command and using a filter. --query "[].id" -o tsv. Then the az ad group member add command allows us to add the object id of our service principal to the group.
The Azure-CLI command documentation can be found here. az role definition create --role-definition vm-restart.json . Once the role has been create you can use the following command to assign it to a group or user(s) az role assignment create --role "Restart Virtual Machines" --assignee [email protected] or assign it using the portal.
.\aad-apply-role-assignments.ps1 -TenantId xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx -ConfigFilePath ".\aad-role-assignments.json" If you are interested, this is how the script looks like: This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below.
Here are a bunch of ways you can find which roles are built into Azure. This will come in super handy when you need to assign a role to a service principal or user with Azure CLI commands like this: az role assignment create --assignee 3db3ad97-06be-4c28-aa96-f1bac93aeed3 --role "Azure Maps Data Reader" Azure CLI. Query the big honking json
Manage role assignments. Create a new role assignment for a user, group, or service principal. Delete role assignments. List role assignments. List changelogs for role assignments. Update an existing role assignment for a user, group, or service principal. Manage role definitions. Create a custom role definition.
Tour Start here for a quick overview of the site Help Center Detailed answers to any questions you might have Meta Discuss the workings and policies of this site
This is autogenerated. Please review and update as needed. Describe the bug I'm following the Azure container Apps doc to Create a service principal and store credential. Command Name az ad sp create-for-rbac az ad sp create-for-rbac \ -...
List role assignments for a user. To list the role assignments for a specific user, use az role assignment list: Azure CLI. Copy. az role assignment list --assignee {assignee} By default, only role assignments for the current subscription will be displayed. To view role assignments for the current subscription and below, add the --all parameter.
In this article. An Azure role assignment condition is an additional check that you can optionally add to your role assignment to provide more fine-grained access control. For example, you can add a condition that requires an object to have a specific tag to read the object. This article describes how to add, edit, list, or delete conditions for your role assignments using Azure CLI.
Select + Create. Add Contributor role assignment to Managed Identity. Navigate to the Managed Identity resource that you created. Select Azure role assignments from the left side tab. Select +Add role assignment from the navigation menu. Inside Add role assignment page, Perform the following tasks: Select the Scope to Resource group.
In this article. If the Azure built-in roles don't meet the specific needs of your organization, you can create your own custom roles. For this tutorial, you create a custom role named Reader Support Tickets using Azure CLI. The custom role allows the user to view everything in the control plane of a subscription and also open support tickets.
Note that, there is no direct Azure CLI command to create custom directory role in Microsoft Entra ID. Alternatively, you can make use of either curl or az rest by calling Microsoft Graph API. In my case, I used below bash script that calls curl request and got response like this: TOKEN=$(az account get-access-token --resource https://graph ...
The Global admin is an Administrator role in Azure AD, and the Owner is an RBAC role in the subscription. They are different things. When you use the azure cli like below to create the role assignment, it adds the service principal joytestsp as an Owner to the storage account. The service principal which you login needs to call the AAD Graph to ...
I have created a Service Principal to access resources in CI before using the az ad sp create-for-rbac command: az ad sp create-for-rbac --name "my-ci-sp" --role contributor \ --scopes /