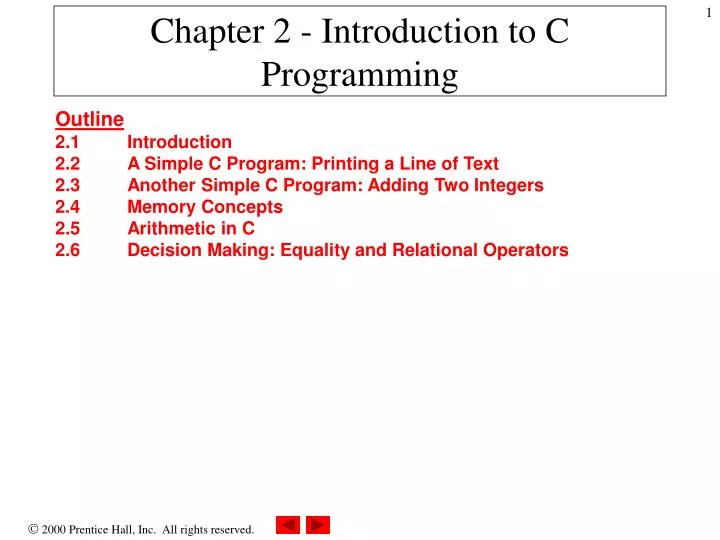

Chapter 2 - Introduction to C Programming
Nov 02, 2012
430 likes | 1.85k Views
Chapter 2 - Introduction to C Programming. Outline 2.1 Introduction 2.2 A Simple C Program: Printing a Line of Text 2.3 Another Simple C Program: Adding Two Integers 2.4 Memory Concepts 2.5 Arithmetic in C 2.6 Decision Making: Equality and Relational Operators. 2.1 Introduction.
Share Presentation
- input output
- print program output
- simple c program printing
- executable statement references
- integer2 sum
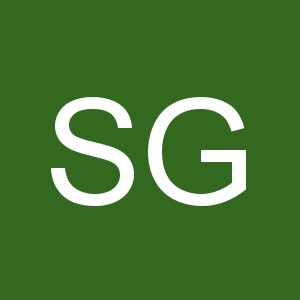
Presentation Transcript
Chapter 2 - Introduction to C Programming Outline 2.1 Introduction 2.2 A Simple C Program: Printing a Line of Text 2.3 Another Simple C Program: Adding Two Integers 2.4 Memory Concepts 2.5 Arithmetic in C 2.6 Decision Making: Equality and Relational Operators
2.1 Introduction • C programming language • Structured and disciplined approach to program design • Structured programming • Introduced in chapters 3 and 4 • Used throughout the remainder of the book
1 /* Fig. 2.1: fig02_01.c 2 A first program in C */ 3 #include <stdio.h> 4 5 int main() 6 { 7 printf( "Welcome to C!\n" ); 8 9 return 0; 10 } 2.2 A Simple C Program:Printing a Line of Text • Comments • Text surrounded by /* and */ is ignored by computer • Used to describe program • #include <stdio.h> • Preprocessor directive • Tells computer to load contents of a certain file • <stdio.h> allows standard input/output operations Welcome to C!
2.2 A Simple C Program:Printing a Line of Text • int main() • C++ programs contain one or more functions, exactly one of which must be main • Parenthesis used to indicate a function • int means that main "returns" an integer value • Braces ({ and }) indicate a block • The bodies of all functions must be contained in braces
2.2 A Simple C Program:Printing a Line of Text • printf("Welcome to C!\n"); • Instructs computer to perform an action • Specifically, prints the string of characters within quotes (“”) • Entire line called a statement • All statements must end with a semicolon (;) • Escape character (\) • Indicates that printf should do something out of the ordinary • \n is the newline character
2.2 A Simple C Program:Printing a Line of Text • return0; • A way to exit a function • return0, in this case, means that the program terminated normally • Right brace } • Indicates end of main has been reached • Linker • When a function is called, linker locates it in the library • Inserts it into object program • If function name is misspelled, the linker will produce an error because it will not be able to find function in the library
1 /* Fig. 2.5: fig02_05.c 2 Addition program */ 3 #include <stdio.h> 4 5 int main() 6 { 7 int integer1, integer2, sum; /* declaration */ 8 9 printf( "Enter first integer\n" ); /* prompt */ 10 scanf( "%d", &integer1 ); /* read an integer */ 11 printf( "Enter second integer\n" ); /* prompt */ 12 scanf( "%d", &integer2 ); /* read an integer */ 13 sum = integer1 + integer2; /* assignment of sum */ 14 printf( "Sum is %d\n", sum ); /* print sum */ 15 16 return 0; /* indicate that program ended successfully */ 17 } 1. Initialize variables 2. Input 2.1 Sum 3. Print Program Output Enter first integer 45 Enter second integer 72 Sum is 117
2.3 Another Simple C Program:Adding Two Integers • As before • Comments, #include <stdio.h> and main • intinteger1,integer2,sum; • Declaration of variables • Variables: locations in memory where a value can be stored • int means the variables can hold integers (-1, 3, 0, 47) • Variable names (identifiers) • integer1, integer2, sum • Identifiers: consist of letters, digits (cannot begin with a digit) and underscores( _ ) • Case sensitive • Declarations appear before executable statements • If an executable statement references and undeclared variable it will produce a syntax (compiler) error
2.3 Another Simple C Program:Adding Two Integers • scanf( "%d", &integer1 ); • Obtains a value from the user • scanf uses standard input (usually keyboard) • This scanf statement has two arguments • %d - indicates data should be a decimal integer • &integer1 - location in memory to store variable • & is confusing in beginning – for now, just remember to include it with the variable name in scanf statements • When executing the program the user responds to the scanf statement by typing in a number, then pressing the enter (return) key
2.3 Another Simple C Program:Adding Two Integers • = (assignment operator) • Assigns a value to a variable • Is a binary operator (has two operands) sum = variable1 + variable2; sum gets variable1 + variable2; • Variable receiving value on left • printf("Sum is %d\n",sum); • Similar to scanf • %d means decimal integer will be printed • sum specifies what integer will be printed • Calculations can be performed inside printf statements printf( "Sum is %d\n", integer1 + integer2 );
integer1 45 2.4 Memory Concepts • Variables • Variable names correspond to locations in the computer's memory • Every variable has a name, a type, a size and a value • Whenever a new value is placed into a variable (through scanf, for example), it replaces (and destroys) the previous value • Reading variables from memory does not change them • A visual representation
2.5 Arithmetic • Arithmetic calculations • Use * for multiplication and / for division • Integer division truncates remainder • 7/5 evaluates to 1 • Modulus operator(%) returns the remainder • 7%5 evaluates to 2 • Operator precedence • Some arithmetic operators act before others (i.e., multiplication before addition) • Use parenthesis when needed • Example: Find the average of three variables a, b and c • Do not use: a + b + c / 3 • Use: (a + b + c ) / 3
2.5 Arithmetic • Arithmetic operators: • Rules of operator precedence:
2.6 Decision Making: Equality and Relational Operators • Executable statements • Perform actions (calculations, input/output of data) • Perform decisions • May want to print "pass" or "fail" given the value of a test grade • if control structure • Simple version in this section, more detail later • If a condition is true, then the body of the if statement executed • 0 is false, non-zero is true • Control always resumes after the if structure • Keywords • Special words reserved for C • Cannot be used as identifiers or variable names
2.6 Decision Making: Equality and Relational Operators
1 /* Fig. 2.13: fig02_13.c 2 Using if statements, relational 3 operators, and equality operators */ 4 #include <stdio.h> 5 6 int main() 7 { 8 int num1, num2; 9 10 printf( "Enter two integers, and I will tell you\n" ); 11 printf( "the relationships they satisfy: " ); 12 scanf( "%d%d", &num1, &num2 ); /* read two integers */ 13 14 if ( num1 == num2 ) 15 printf( "%d is equal to %d\n", num1, num2 ); 16 17 if ( num1 != num2 ) 18 printf( "%d is not equal to %d\n", num1, num2 ); 19 20 if ( num1 < num2 ) 21 printf( "%d is less than %d\n", num1, num2 ); 22 23 if ( num1 > num2 ) 24 printf( "%d is greater than %d\n", num1, num2 ); 25 26 if ( num1 <= num2 ) 27 printf( "%d is less than or equal to %d\n", 28 num1, num2 ); 1. Declare variables 2. Input 2.1 if statements 3. Print
29 30 if ( num1 >= num2 ) 31 printf( "%d is greater than or equal to %d\n", 32 num1, num2 ); 33 34 return 0; /* indicate program ended successfully */ 35 } 3.1 Exit main Program Output Enter two integers, and I will tell you the relationships they satisfy: 3 7 3 is not equal to 7 3 is less than 7 3 is less than or equal to 7 Enter two integers, and I will tell you the relationships they satisfy: 22 12 22 is not equal to 12 22 is greater than 12 22 is greater than or equal to 12
- More by User
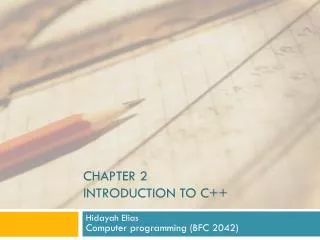
Chapter 2 introduction to C++
Chapter 2 introduction to C++. Hidayah Elias Computer programming (BFC 2042). Subtopics. Parts of a C++ Program Classes and Objects The #include Directive Variables and Literals Identifiers. Data Types Integer Char Floating point bool Arithmetic Operators Comments. preprocessor
568 views • 36 slides
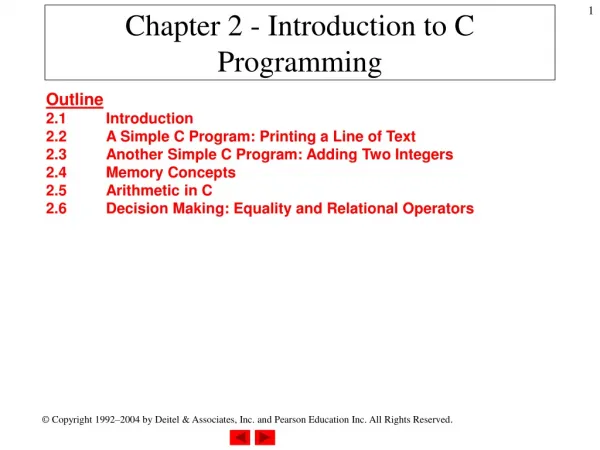
Chapter 2 - Introduction to C Programming. Outline 2.1 Introduction 2.2 A Simple C Program: Printing a Line of Text 2.3 Another Simple C Program: Adding Two Integers 2.4 Memory Concepts 2.5 Arithmetic in C 2.6 Decision Making: Equality and Relational Operators. Objectives.
590 views • 27 slides
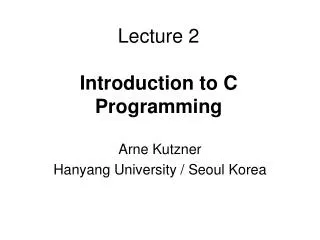
Lecture 2 Introduction to C Programming
Lecture 2 Introduction to C Programming. Arne Kutzner Hanyang University / Seoul Korea. This week …. You will learn: From the first Computers to the C Programming Languages Von Neumann Architecture The Idea of “binary computing” Origins of Programming Languages
857 views • 43 slides
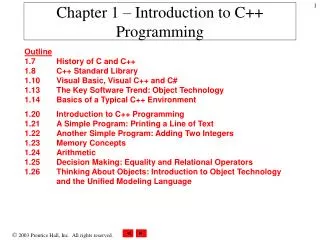
Chapter 1 – Introduction to C++ Programming
Chapter 1 – Introduction to C++ Programming. Outline 1.7 History of C and C++ 1.8 C++ Standard Library 1.10 Visual Basic, Visual C++ and C# 1.13 The Key Software Trend: Object Technology 1.14 Basics of a Typical C++ Environment
1.26k views • 34 slides
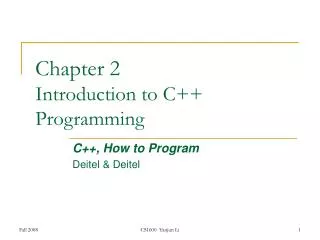
Chapter 2 Introduction to C++ Programming
Chapter 2 Introduction to C++ Programming. C++, How to Program Deitel & Deitel. Learn C++ by Examples. Five examples demonstrate How to display messages How to obtain information from the user How to perform arithmetic calculations. Important Parts of a C++ program.
585 views • 35 slides
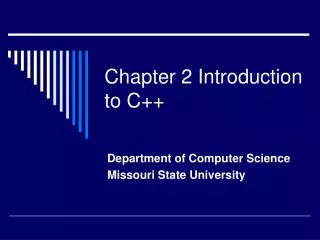

Chapter 2 Introduction to C++
Chapter 2 Introduction to C++. Department of Computer Science Missouri State University. Outline. The structure of C++ programs Cout Objects The #include directive Variables and constants Data Types Operations Comments Programming Style. An example.
366 views • 28 slides
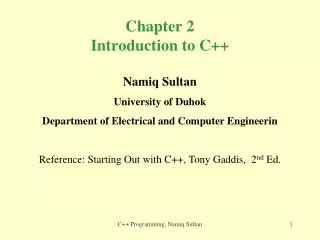
Chapter 2 Introduction to C++. Namiq Sultan University of Duhok Department of Electrical and Computer Engineerin Reference: Starting Out with C++, Tony Gaddis, 2 nd Ed. 2.1 The Parts of a C++ Program. C++ programs have parts and components that serve specific purposes. Program 2-1.
599 views • 51 slides
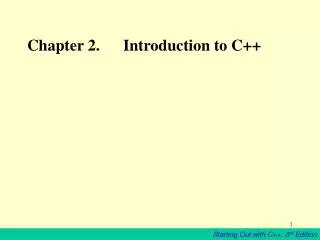
Chapter 2. Introduction to C++
Chapter 2. Introduction to C++. 2.1 The Parts of a C++ Program. C++ programs have parts and components that serve specific purposes. Program 2-1. //A simple C++ program #include <iostream.h> void main (void) { cout<< “Programming is great fun!”; } Program Output:
969 views • 70 slides
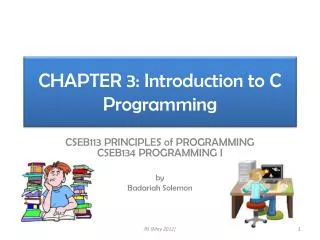
CHAPTER 3: Introduction to C Programming
CHAPTER 3: Introduction to C Programming. CSEB113 PRINCIPLES of PROGRAMMING CSEB134 PROGRAMMING I by Badariah Solemon. Topics. Steps for Creating, Compiling and Executing a C program Basic Structure of a C Program Formatting Output Writing Comments Errors and Debugging. Topic 1.
706 views • 40 slides
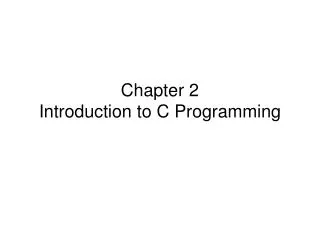
Chapter 2 Introduction to C Programming
Chapter 2 Introduction to C Programming. Objectives. In this chapter, you will learn: To be able to write simple computer programs in C. To be able to use simple input and output statements. To become familiar with fundamental data types. To understand computer memory concepts.
518 views • 38 slides
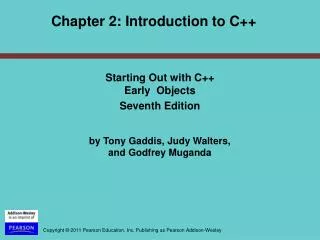
Chapter 2: Introduction to C++
Chapter 2: Introduction to C++. Starting Out with C++ Early Objects Seventh Edition by Tony Gaddis, Judy Walters, and Godfrey Muganda. Topics. 2.1 The Parts of a C++ Program 2.2 The cout Object 2.3 The #include Directive 2.4 Standard and Prestandard C++
473 views • 40 slides
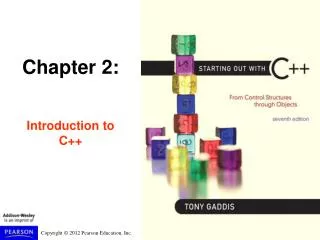
Chapter 2: Introduction to C++. 2.1. The Part of a C++ Program. preprocessor directive. which namespace to use. beginning of function named main. beginning of block for main. output statement. string literal. send 0 to operating system. end of block for main.
908 views • 79 slides
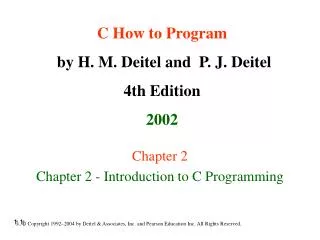
Chapter 2 Chapter 2 - Introduction to C Programming
Chapter 2 Chapter 2 - Introduction to C Programming. C How to Program by H. M. Deitel and P. J. Deitel 4th Edition 2002. 1.1. Chapter 2 - Introduction to C Programming. Outline 2.1 Introduction 2.2 A Simple C Program: Printing a Line of Text
817 views • 30 slides
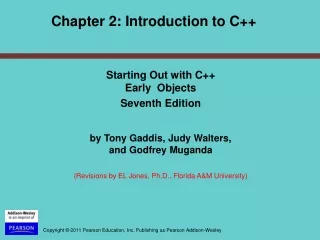
Chapter 2: Introduction to C++. Starting Out with C++ Early Objects Seventh Edition by Tony Gaddis, Judy Walters, and Godfrey Muganda (Revisions by EL Jones, Ph.D., Florida A&M University). Topics (where discussed in book). 2.1 The Parts of a C++ Program 2.2 Output: the cout Object
579 views • 50 slides
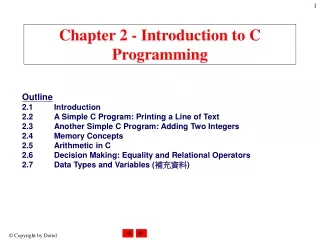
Chapter 2 - Introduction to C Programming. Outline 2.1 Introduction 2.2 A Simple C Program: Printing a Line of Text 2.3 Another Simple C Program: Adding Two Integers 2.4 Memory Concepts 2.5 Arithmetic in C 2.6 Decision Making: Equality and Relational Operators
783 views • 49 slides
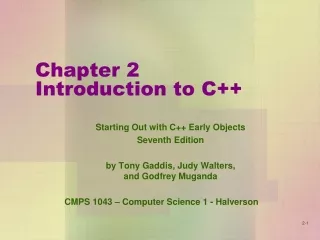
Chapter 2 Introduction to C++. Starting Out with C++ Early Objects Seventh Edition by Tony Gaddis, Judy Walters, and Godfrey Muganda CMPS 1043 – Computer Science 1 - Halverson. comment. preprocessor directive. which namespace to use. beginning of function named main.
463 views • 44 slides
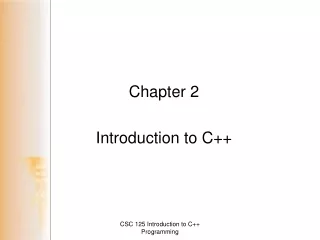
Chapter 2 Introduction to C++. Topics. 2.1 Parts of a C++ Program 2.2 The cout Object 2.3 The #include Directive 2.4 Variables and Constants 2.5 Identifiers 2.6 Integer Data Types 2.7 The char Data Type 2.8 Floating-Point Data Types. Topics (continued). 2.9 The bool Data Type
377 views • 33 slides
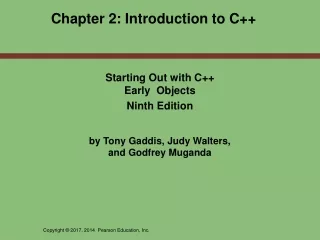
Chapter 2: Introduction to C++. Starting Out with C++ Early Objects Ninth Edition by Tony Gaddis, Judy Walters, and Godfrey Muganda. Topics. 2.1 The Parts of a C++ Program 2.2 The cout Object 2.3 The #include Directive 2.4 Variables and the Assignment Statement 2.5 Literals
498 views • 47 slides
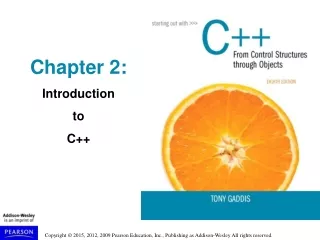
Chapter 2: Introduction to C++. string literal. The Parts of a C++ Program. // sample C++ program #include <iostream> using namespace std; int main() { cout << "Hello, there!"; return 0; }. comment. preprocessor directive. which namespace to use.
639 views • 59 slides

- Preferences
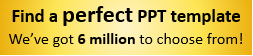
Chapter 3: Introduction to C Programming Language - PowerPoint PPT Presentation

Chapter 3: Introduction to C Programming Language
Chapter 3: introduction to c programming language c development environment a simple program example characters and tokens structure of a c program – powerpoint ppt presentation.
- C development environment
- A simple program example
- Characters and tokens
- Structure of a C program
- comment and preprocessor directives
- basic data types
- data declarations
- Basic functions
- NOTE ABOUT C PROGRAMS
- In C, lowercase and uppercase characters are very important! All commands in C must be lowercase. The C programs starting point is identified by the word
- This informs the computer as to where the program actually starts. The brackets that follow the keyword main indicate that there are no arguments supplied to this program (this will be examined later on).
- The two braces, and , signify the begin and end segments of the program.
- Summary of major points so far
- program execution begins at main()
- keywords are written in lower-case
- statements are terminated with a semi-colon
- text strings are enclosed in double quotes
- Characters are the basic building blocks in C program, equivalent to letters in English language
- Includes every printable character on the standard English language keyboard except , and _at_
- Example of characters
- Numeric digits 0 - 9
- Lowercase/uppercase letters a - z and A - Z
- Space (blank)
- Special characters , . ? / ( ) lt gt etc
- A token is a language element that can be used in forming higher level language constructs
- Equivalent to a word in English language
- Several types of tokens can be used to build a higher level C language construct such as expressions and statements
- There are 6 kinds of tokens in C
- Reserved words (keywords)
- Identifiers
- String literals
- Punctuators
- Keywords that identify language entities such as statements, data types, language attributes, etc.
- Have special meaning to the compiler, cannot be used as identifiers in our program.
- Should be typed in lowercase.
- Example const, double, int, main, void, while, for, else (etc..)
- Words used to represent certain program entities (program variables, function names, etc).
- int my_name
- my_name is an identifier used as a program variable
- void CalculateTotal(int value)
- CalculateTotal is an identifier used as a function name
- Entities that appear in the program code as fixed values.
- 4 types of constants
- Integer constants
- Positive or negative whole numbers with no fractional part
- const int MAX_NUM 10
- const int MIN_NUM -90
- Floating-point constants
- Positive or negative decimal numbers with an integer part, a decimal point and a fractional part
- const double VAL 0.5877e2 (stands for 0.5877 x 102)
- Character constants
- A character enclosed in a single quotation mark
- const char letter n
- const char number 1
- printf(c, S)
- Output would be S
- Enumeration
- Values are given as a list
- A sequence of any number of characters surrounded by double quotation marks.
- My name is Salman
- Example of usage in C program
- printf(My room number is BN-1-012\n)
- Output My room number is BN-1-012
- Symbols used to separate different parts of the C program.
- These punctuators include
- Usage example
- Tokens that result in some kind of computation or action when applied to variables or or other elements in an expression.
- Example of operators
- result total1 total2
- Explanations or annotations that are included in a program for documentation and clarification purpose.
- Completely ignored by the compiler during compilation and have no effect on program execution.
- Starts with / and ends with /
- Some compiler support comments starting with //
- The first thing to be checked by the compiler.
- Starts with .
- Tell the compiler about specific options that it needs to be aware of during compilation.
- There are a few compiler directives. But only 2 of them will be discussed here.
- include ltstdio.hgt
- Tell the compiler to include the file stdio.h during compilation
- Anything in the header file is considered a part of the program
- define VALUE 10
- Tell the compiler to substitute the word VALUE with 10 during compilation
- 3 examples of basic data types
- int (used to declare numeric program variables of integer type)
- char (used to declare character variable)
- double (used to declare floating point variable)
- In addition, there are float, void, short, long, etc.
- Declaration specifies the type of a variable.
- Example int local_var
- Definition assigning a value to the declared variable.
- Example local_var 5
- A variable can be declared globally or locally.
- A globally declared variable can be accessed from all parts of the program.
- A locally declared variable can only be accessed from inside the function in which the variable is declared.
- A specification of an action to be taken by the computer as the program executes.
- In the previous example, there are 2 lines following variable declaration and variable definition that terminate with semicolon .
- global_var local_var VALUE
- printf (Total sum is d\n, global_var)
- Each line is a statement.
- A C program consists of one or more functions that contain a group of statements which perform a specific task.
- A C program must at least have one function the function main.
- We can create our own function or use the functions that has been created in the library, in which case we have to include the appropriate header file (example stdio.h).
- In this section, we will learn a few functions that are pre-defined in the header file stdio.h
- These functions are
- getchar() putchar()
- In addition to those functions, we will also learn about Format Specifier and Escape Sequence which are used with printf() and scanf().
- Used to send data to the standard output (usually the monitor) to be printed according to specific format.
- General format
- printf(control string, variables)
- Control string is a combination of text, format specifier and escape sequence.
- printf(Thank you)
- d is a format specifier
- \n is an escape sequence
- Read data from the standard input device (usually keyboard) and store it in a variable.
- scanf(Control string, variable)
- The general format is pretty much the same as printf() except that it passes the address of the variable (notice the sign) instead of the variable itself to the second function argument.
- getchar() - read a character from standard input
- putchar() - write a character to standard output
PowerShow.com is a leading presentation sharing website. It has millions of presentations already uploaded and available with 1,000s more being uploaded by its users every day. Whatever your area of interest, here you’ll be able to find and view presentations you’ll love and possibly download. And, best of all, it is completely free and easy to use.
You might even have a presentation you’d like to share with others. If so, just upload it to PowerShow.com. We’ll convert it to an HTML5 slideshow that includes all the media types you’ve already added: audio, video, music, pictures, animations and transition effects. Then you can share it with your target audience as well as PowerShow.com’s millions of monthly visitors. And, again, it’s all free.
About the Developers
PowerShow.com is brought to you by CrystalGraphics , the award-winning developer and market-leading publisher of rich-media enhancement products for presentations. Our product offerings include millions of PowerPoint templates, diagrams, animated 3D characters and more.

